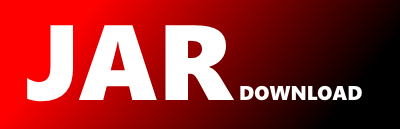
cz.proto.SpillStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: operator.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.SpillStats}
*/
public final class SpillStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.SpillStats)
SpillStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use SpillStats.newBuilder() to construct.
private SpillStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SpillStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SpillStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SpillStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
compressedSize_ = input.readUInt64();
break;
}
case 16: {
rawSize_ = input.readUInt64();
break;
}
case 24: {
spillCount_ = input.readUInt64();
break;
}
case 32: {
rowCount_ = input.readUInt64();
break;
}
case 40: {
runCount_ = input.readUInt64();
break;
}
case 48: {
fileCount_ = input.readUInt64();
break;
}
case 58: {
cz.proto.Timing.Builder subBuilder = null;
if (writeTiming_ != null) {
subBuilder = writeTiming_.toBuilder();
}
writeTiming_ = input.readMessage(cz.proto.Timing.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(writeTiming_);
writeTiming_ = subBuilder.buildPartial();
}
break;
}
case 66: {
cz.proto.Timing.Builder subBuilder = null;
if (readTiming_ != null) {
subBuilder = readTiming_.toBuilder();
}
readTiming_ = input.readMessage(cz.proto.Timing.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(readTiming_);
readTiming_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_SpillStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_SpillStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.SpillStats.class, cz.proto.SpillStats.Builder.class);
}
public static final int COMPRESSED_SIZE_FIELD_NUMBER = 1;
private long compressedSize_;
/**
* uint64 compressed_size = 1;
* @return The compressedSize.
*/
@java.lang.Override
public long getCompressedSize() {
return compressedSize_;
}
public static final int RAW_SIZE_FIELD_NUMBER = 2;
private long rawSize_;
/**
* uint64 raw_size = 2;
* @return The rawSize.
*/
@java.lang.Override
public long getRawSize() {
return rawSize_;
}
public static final int SPILL_COUNT_FIELD_NUMBER = 3;
private long spillCount_;
/**
*
* deprecated
*
*
* uint64 spill_count = 3;
* @return The spillCount.
*/
@java.lang.Override
public long getSpillCount() {
return spillCount_;
}
public static final int ROW_COUNT_FIELD_NUMBER = 4;
private long rowCount_;
/**
* uint64 row_count = 4;
* @return The rowCount.
*/
@java.lang.Override
public long getRowCount() {
return rowCount_;
}
public static final int RUN_COUNT_FIELD_NUMBER = 5;
private long runCount_;
/**
* uint64 run_count = 5;
* @return The runCount.
*/
@java.lang.Override
public long getRunCount() {
return runCount_;
}
public static final int FILE_COUNT_FIELD_NUMBER = 6;
private long fileCount_;
/**
* uint64 file_count = 6;
* @return The fileCount.
*/
@java.lang.Override
public long getFileCount() {
return fileCount_;
}
public static final int WRITE_TIMING_FIELD_NUMBER = 7;
private cz.proto.Timing writeTiming_;
/**
* .cz.proto.Timing write_timing = 7;
* @return Whether the writeTiming field is set.
*/
@java.lang.Override
public boolean hasWriteTiming() {
return writeTiming_ != null;
}
/**
* .cz.proto.Timing write_timing = 7;
* @return The writeTiming.
*/
@java.lang.Override
public cz.proto.Timing getWriteTiming() {
return writeTiming_ == null ? cz.proto.Timing.getDefaultInstance() : writeTiming_;
}
/**
* .cz.proto.Timing write_timing = 7;
*/
@java.lang.Override
public cz.proto.TimingOrBuilder getWriteTimingOrBuilder() {
return getWriteTiming();
}
public static final int READ_TIMING_FIELD_NUMBER = 8;
private cz.proto.Timing readTiming_;
/**
* .cz.proto.Timing read_timing = 8;
* @return Whether the readTiming field is set.
*/
@java.lang.Override
public boolean hasReadTiming() {
return readTiming_ != null;
}
/**
* .cz.proto.Timing read_timing = 8;
* @return The readTiming.
*/
@java.lang.Override
public cz.proto.Timing getReadTiming() {
return readTiming_ == null ? cz.proto.Timing.getDefaultInstance() : readTiming_;
}
/**
* .cz.proto.Timing read_timing = 8;
*/
@java.lang.Override
public cz.proto.TimingOrBuilder getReadTimingOrBuilder() {
return getReadTiming();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (compressedSize_ != 0L) {
output.writeUInt64(1, compressedSize_);
}
if (rawSize_ != 0L) {
output.writeUInt64(2, rawSize_);
}
if (spillCount_ != 0L) {
output.writeUInt64(3, spillCount_);
}
if (rowCount_ != 0L) {
output.writeUInt64(4, rowCount_);
}
if (runCount_ != 0L) {
output.writeUInt64(5, runCount_);
}
if (fileCount_ != 0L) {
output.writeUInt64(6, fileCount_);
}
if (writeTiming_ != null) {
output.writeMessage(7, getWriteTiming());
}
if (readTiming_ != null) {
output.writeMessage(8, getReadTiming());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (compressedSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, compressedSize_);
}
if (rawSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, rawSize_);
}
if (spillCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, spillCount_);
}
if (rowCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, rowCount_);
}
if (runCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, runCount_);
}
if (fileCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, fileCount_);
}
if (writeTiming_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getWriteTiming());
}
if (readTiming_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getReadTiming());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.SpillStats)) {
return super.equals(obj);
}
cz.proto.SpillStats other = (cz.proto.SpillStats) obj;
if (getCompressedSize()
!= other.getCompressedSize()) return false;
if (getRawSize()
!= other.getRawSize()) return false;
if (getSpillCount()
!= other.getSpillCount()) return false;
if (getRowCount()
!= other.getRowCount()) return false;
if (getRunCount()
!= other.getRunCount()) return false;
if (getFileCount()
!= other.getFileCount()) return false;
if (hasWriteTiming() != other.hasWriteTiming()) return false;
if (hasWriteTiming()) {
if (!getWriteTiming()
.equals(other.getWriteTiming())) return false;
}
if (hasReadTiming() != other.hasReadTiming()) return false;
if (hasReadTiming()) {
if (!getReadTiming()
.equals(other.getReadTiming())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COMPRESSED_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCompressedSize());
hash = (37 * hash) + RAW_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRawSize());
hash = (37 * hash) + SPILL_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSpillCount());
hash = (37 * hash) + ROW_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRowCount());
hash = (37 * hash) + RUN_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRunCount());
hash = (37 * hash) + FILE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFileCount());
if (hasWriteTiming()) {
hash = (37 * hash) + WRITE_TIMING_FIELD_NUMBER;
hash = (53 * hash) + getWriteTiming().hashCode();
}
if (hasReadTiming()) {
hash = (37 * hash) + READ_TIMING_FIELD_NUMBER;
hash = (53 * hash) + getReadTiming().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.SpillStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.SpillStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.SpillStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.SpillStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.SpillStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.SpillStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.SpillStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.SpillStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.SpillStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.SpillStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.SpillStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.SpillStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.SpillStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.SpillStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.SpillStats)
cz.proto.SpillStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.OperatorProto.internal_static_cz_proto_SpillStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.OperatorProto.internal_static_cz_proto_SpillStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.SpillStats.class, cz.proto.SpillStats.Builder.class);
}
// Construct using cz.proto.SpillStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
compressedSize_ = 0L;
rawSize_ = 0L;
spillCount_ = 0L;
rowCount_ = 0L;
runCount_ = 0L;
fileCount_ = 0L;
if (writeTimingBuilder_ == null) {
writeTiming_ = null;
} else {
writeTiming_ = null;
writeTimingBuilder_ = null;
}
if (readTimingBuilder_ == null) {
readTiming_ = null;
} else {
readTiming_ = null;
readTimingBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.OperatorProto.internal_static_cz_proto_SpillStats_descriptor;
}
@java.lang.Override
public cz.proto.SpillStats getDefaultInstanceForType() {
return cz.proto.SpillStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.SpillStats build() {
cz.proto.SpillStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.SpillStats buildPartial() {
cz.proto.SpillStats result = new cz.proto.SpillStats(this);
result.compressedSize_ = compressedSize_;
result.rawSize_ = rawSize_;
result.spillCount_ = spillCount_;
result.rowCount_ = rowCount_;
result.runCount_ = runCount_;
result.fileCount_ = fileCount_;
if (writeTimingBuilder_ == null) {
result.writeTiming_ = writeTiming_;
} else {
result.writeTiming_ = writeTimingBuilder_.build();
}
if (readTimingBuilder_ == null) {
result.readTiming_ = readTiming_;
} else {
result.readTiming_ = readTimingBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.SpillStats) {
return mergeFrom((cz.proto.SpillStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.SpillStats other) {
if (other == cz.proto.SpillStats.getDefaultInstance()) return this;
if (other.getCompressedSize() != 0L) {
setCompressedSize(other.getCompressedSize());
}
if (other.getRawSize() != 0L) {
setRawSize(other.getRawSize());
}
if (other.getSpillCount() != 0L) {
setSpillCount(other.getSpillCount());
}
if (other.getRowCount() != 0L) {
setRowCount(other.getRowCount());
}
if (other.getRunCount() != 0L) {
setRunCount(other.getRunCount());
}
if (other.getFileCount() != 0L) {
setFileCount(other.getFileCount());
}
if (other.hasWriteTiming()) {
mergeWriteTiming(other.getWriteTiming());
}
if (other.hasReadTiming()) {
mergeReadTiming(other.getReadTiming());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.SpillStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.SpillStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long compressedSize_ ;
/**
* uint64 compressed_size = 1;
* @return The compressedSize.
*/
@java.lang.Override
public long getCompressedSize() {
return compressedSize_;
}
/**
* uint64 compressed_size = 1;
* @param value The compressedSize to set.
* @return This builder for chaining.
*/
public Builder setCompressedSize(long value) {
compressedSize_ = value;
onChanged();
return this;
}
/**
* uint64 compressed_size = 1;
* @return This builder for chaining.
*/
public Builder clearCompressedSize() {
compressedSize_ = 0L;
onChanged();
return this;
}
private long rawSize_ ;
/**
* uint64 raw_size = 2;
* @return The rawSize.
*/
@java.lang.Override
public long getRawSize() {
return rawSize_;
}
/**
* uint64 raw_size = 2;
* @param value The rawSize to set.
* @return This builder for chaining.
*/
public Builder setRawSize(long value) {
rawSize_ = value;
onChanged();
return this;
}
/**
* uint64 raw_size = 2;
* @return This builder for chaining.
*/
public Builder clearRawSize() {
rawSize_ = 0L;
onChanged();
return this;
}
private long spillCount_ ;
/**
*
* deprecated
*
*
* uint64 spill_count = 3;
* @return The spillCount.
*/
@java.lang.Override
public long getSpillCount() {
return spillCount_;
}
/**
*
* deprecated
*
*
* uint64 spill_count = 3;
* @param value The spillCount to set.
* @return This builder for chaining.
*/
public Builder setSpillCount(long value) {
spillCount_ = value;
onChanged();
return this;
}
/**
*
* deprecated
*
*
* uint64 spill_count = 3;
* @return This builder for chaining.
*/
public Builder clearSpillCount() {
spillCount_ = 0L;
onChanged();
return this;
}
private long rowCount_ ;
/**
* uint64 row_count = 4;
* @return The rowCount.
*/
@java.lang.Override
public long getRowCount() {
return rowCount_;
}
/**
* uint64 row_count = 4;
* @param value The rowCount to set.
* @return This builder for chaining.
*/
public Builder setRowCount(long value) {
rowCount_ = value;
onChanged();
return this;
}
/**
* uint64 row_count = 4;
* @return This builder for chaining.
*/
public Builder clearRowCount() {
rowCount_ = 0L;
onChanged();
return this;
}
private long runCount_ ;
/**
* uint64 run_count = 5;
* @return The runCount.
*/
@java.lang.Override
public long getRunCount() {
return runCount_;
}
/**
* uint64 run_count = 5;
* @param value The runCount to set.
* @return This builder for chaining.
*/
public Builder setRunCount(long value) {
runCount_ = value;
onChanged();
return this;
}
/**
* uint64 run_count = 5;
* @return This builder for chaining.
*/
public Builder clearRunCount() {
runCount_ = 0L;
onChanged();
return this;
}
private long fileCount_ ;
/**
* uint64 file_count = 6;
* @return The fileCount.
*/
@java.lang.Override
public long getFileCount() {
return fileCount_;
}
/**
* uint64 file_count = 6;
* @param value The fileCount to set.
* @return This builder for chaining.
*/
public Builder setFileCount(long value) {
fileCount_ = value;
onChanged();
return this;
}
/**
* uint64 file_count = 6;
* @return This builder for chaining.
*/
public Builder clearFileCount() {
fileCount_ = 0L;
onChanged();
return this;
}
private cz.proto.Timing writeTiming_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder> writeTimingBuilder_;
/**
* .cz.proto.Timing write_timing = 7;
* @return Whether the writeTiming field is set.
*/
public boolean hasWriteTiming() {
return writeTimingBuilder_ != null || writeTiming_ != null;
}
/**
* .cz.proto.Timing write_timing = 7;
* @return The writeTiming.
*/
public cz.proto.Timing getWriteTiming() {
if (writeTimingBuilder_ == null) {
return writeTiming_ == null ? cz.proto.Timing.getDefaultInstance() : writeTiming_;
} else {
return writeTimingBuilder_.getMessage();
}
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public Builder setWriteTiming(cz.proto.Timing value) {
if (writeTimingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
writeTiming_ = value;
onChanged();
} else {
writeTimingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public Builder setWriteTiming(
cz.proto.Timing.Builder builderForValue) {
if (writeTimingBuilder_ == null) {
writeTiming_ = builderForValue.build();
onChanged();
} else {
writeTimingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public Builder mergeWriteTiming(cz.proto.Timing value) {
if (writeTimingBuilder_ == null) {
if (writeTiming_ != null) {
writeTiming_ =
cz.proto.Timing.newBuilder(writeTiming_).mergeFrom(value).buildPartial();
} else {
writeTiming_ = value;
}
onChanged();
} else {
writeTimingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public Builder clearWriteTiming() {
if (writeTimingBuilder_ == null) {
writeTiming_ = null;
onChanged();
} else {
writeTiming_ = null;
writeTimingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public cz.proto.Timing.Builder getWriteTimingBuilder() {
onChanged();
return getWriteTimingFieldBuilder().getBuilder();
}
/**
* .cz.proto.Timing write_timing = 7;
*/
public cz.proto.TimingOrBuilder getWriteTimingOrBuilder() {
if (writeTimingBuilder_ != null) {
return writeTimingBuilder_.getMessageOrBuilder();
} else {
return writeTiming_ == null ?
cz.proto.Timing.getDefaultInstance() : writeTiming_;
}
}
/**
* .cz.proto.Timing write_timing = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>
getWriteTimingFieldBuilder() {
if (writeTimingBuilder_ == null) {
writeTimingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>(
getWriteTiming(),
getParentForChildren(),
isClean());
writeTiming_ = null;
}
return writeTimingBuilder_;
}
private cz.proto.Timing readTiming_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder> readTimingBuilder_;
/**
* .cz.proto.Timing read_timing = 8;
* @return Whether the readTiming field is set.
*/
public boolean hasReadTiming() {
return readTimingBuilder_ != null || readTiming_ != null;
}
/**
* .cz.proto.Timing read_timing = 8;
* @return The readTiming.
*/
public cz.proto.Timing getReadTiming() {
if (readTimingBuilder_ == null) {
return readTiming_ == null ? cz.proto.Timing.getDefaultInstance() : readTiming_;
} else {
return readTimingBuilder_.getMessage();
}
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public Builder setReadTiming(cz.proto.Timing value) {
if (readTimingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
readTiming_ = value;
onChanged();
} else {
readTimingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public Builder setReadTiming(
cz.proto.Timing.Builder builderForValue) {
if (readTimingBuilder_ == null) {
readTiming_ = builderForValue.build();
onChanged();
} else {
readTimingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public Builder mergeReadTiming(cz.proto.Timing value) {
if (readTimingBuilder_ == null) {
if (readTiming_ != null) {
readTiming_ =
cz.proto.Timing.newBuilder(readTiming_).mergeFrom(value).buildPartial();
} else {
readTiming_ = value;
}
onChanged();
} else {
readTimingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public Builder clearReadTiming() {
if (readTimingBuilder_ == null) {
readTiming_ = null;
onChanged();
} else {
readTiming_ = null;
readTimingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public cz.proto.Timing.Builder getReadTimingBuilder() {
onChanged();
return getReadTimingFieldBuilder().getBuilder();
}
/**
* .cz.proto.Timing read_timing = 8;
*/
public cz.proto.TimingOrBuilder getReadTimingOrBuilder() {
if (readTimingBuilder_ != null) {
return readTimingBuilder_.getMessageOrBuilder();
} else {
return readTiming_ == null ?
cz.proto.Timing.getDefaultInstance() : readTiming_;
}
}
/**
* .cz.proto.Timing read_timing = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>
getReadTimingFieldBuilder() {
if (readTimingBuilder_ == null) {
readTimingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>(
getReadTiming(),
getParentForChildren(),
isClean());
readTiming_ = null;
}
return readTimingBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.SpillStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.SpillStats)
private static final cz.proto.SpillStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.SpillStats();
}
public static cz.proto.SpillStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SpillStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SpillStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.SpillStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy