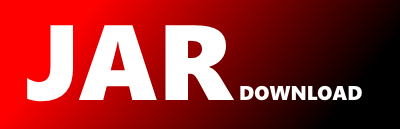
cz.proto.TableScanSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: job.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.TableScanSummary}
*/
public final class TableScanSummary extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.TableScanSummary)
TableScanSummaryOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableScanSummary.newBuilder() to construct.
private TableScanSummary(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableScanSummary() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableScanSummary();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableScanSummary(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.OperatorStatistics.Builder subBuilder = null;
if (inputBytes_ != null) {
subBuilder = inputBytes_.toBuilder();
}
inputBytes_ = input.readMessage(cz.proto.OperatorStatistics.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(inputBytes_);
inputBytes_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.OperatorStatistics.Builder subBuilder = null;
if (splitCnt_ != null) {
subBuilder = splitCnt_.toBuilder();
}
splitCnt_ = input.readMessage(cz.proto.OperatorStatistics.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(splitCnt_);
splitCnt_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.TableScanSummary.TableScanSourceStats.Builder subBuilder = null;
if (tableScanSourceStats_ != null) {
subBuilder = tableScanSourceStats_.toBuilder();
}
tableScanSourceStats_ = input.readMessage(cz.proto.TableScanSummary.TableScanSourceStats.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableScanSourceStats_);
tableScanSourceStats_ = subBuilder.buildPartial();
}
break;
}
case 34: {
cz.proto.TableScanSummary.ParquetRowGroupStats.Builder subBuilder = null;
if (parquetRowGroupStats_ != null) {
subBuilder = parquetRowGroupStats_.toBuilder();
}
parquetRowGroupStats_ = input.readMessage(cz.proto.TableScanSummary.ParquetRowGroupStats.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(parquetRowGroupStats_);
parquetRowGroupStats_ = subBuilder.buildPartial();
}
break;
}
case 42: {
cz.proto.TableScanSummary.ParquetRowCountStats.Builder subBuilder = null;
if (parquetRowCountStats_ != null) {
subBuilder = parquetRowCountStats_.toBuilder();
}
parquetRowCountStats_ = input.readMessage(cz.proto.TableScanSummary.ParquetRowCountStats.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(parquetRowCountStats_);
parquetRowCountStats_ = subBuilder.buildPartial();
}
break;
}
case 50: {
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder subBuilder = null;
if (parquetPrunedSplitsStats_ != null) {
subBuilder = parquetPrunedSplitsStats_.toBuilder();
}
parquetPrunedSplitsStats_ = input.readMessage(cz.proto.TableScanSummary.ParquetPrunedSplitsStats.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(parquetPrunedSplitsStats_);
parquetPrunedSplitsStats_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.class, cz.proto.TableScanSummary.Builder.class);
}
public interface ParquetRowGroupStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.TableScanSummary.ParquetRowGroupStats)
com.google.protobuf.MessageOrBuilder {
/**
* int64 bloom_pruned_row_group = 1;
* @return The bloomPrunedRowGroup.
*/
long getBloomPrunedRowGroup();
/**
* int64 stats_pruned_row_group = 2;
* @return The statsPrunedRowGroup.
*/
long getStatsPrunedRowGroup();
/**
* int64 dict_pruned_row_group = 3;
* @return The dictPrunedRowGroup.
*/
long getDictPrunedRowGroup();
/**
* int64 request_row_group = 4;
* @return The requestRowGroup.
*/
long getRequestRowGroup();
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetRowGroupStats}
*/
public static final class ParquetRowGroupStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.TableScanSummary.ParquetRowGroupStats)
ParquetRowGroupStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParquetRowGroupStats.newBuilder() to construct.
private ParquetRowGroupStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParquetRowGroupStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParquetRowGroupStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ParquetRowGroupStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bloomPrunedRowGroup_ = input.readInt64();
break;
}
case 16: {
statsPrunedRowGroup_ = input.readInt64();
break;
}
case 24: {
dictPrunedRowGroup_ = input.readInt64();
break;
}
case 32: {
requestRowGroup_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowGroupStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowGroupStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetRowGroupStats.class, cz.proto.TableScanSummary.ParquetRowGroupStats.Builder.class);
}
public static final int BLOOM_PRUNED_ROW_GROUP_FIELD_NUMBER = 1;
private long bloomPrunedRowGroup_;
/**
* int64 bloom_pruned_row_group = 1;
* @return The bloomPrunedRowGroup.
*/
@java.lang.Override
public long getBloomPrunedRowGroup() {
return bloomPrunedRowGroup_;
}
public static final int STATS_PRUNED_ROW_GROUP_FIELD_NUMBER = 2;
private long statsPrunedRowGroup_;
/**
* int64 stats_pruned_row_group = 2;
* @return The statsPrunedRowGroup.
*/
@java.lang.Override
public long getStatsPrunedRowGroup() {
return statsPrunedRowGroup_;
}
public static final int DICT_PRUNED_ROW_GROUP_FIELD_NUMBER = 3;
private long dictPrunedRowGroup_;
/**
* int64 dict_pruned_row_group = 3;
* @return The dictPrunedRowGroup.
*/
@java.lang.Override
public long getDictPrunedRowGroup() {
return dictPrunedRowGroup_;
}
public static final int REQUEST_ROW_GROUP_FIELD_NUMBER = 4;
private long requestRowGroup_;
/**
* int64 request_row_group = 4;
* @return The requestRowGroup.
*/
@java.lang.Override
public long getRequestRowGroup() {
return requestRowGroup_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (bloomPrunedRowGroup_ != 0L) {
output.writeInt64(1, bloomPrunedRowGroup_);
}
if (statsPrunedRowGroup_ != 0L) {
output.writeInt64(2, statsPrunedRowGroup_);
}
if (dictPrunedRowGroup_ != 0L) {
output.writeInt64(3, dictPrunedRowGroup_);
}
if (requestRowGroup_ != 0L) {
output.writeInt64(4, requestRowGroup_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (bloomPrunedRowGroup_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, bloomPrunedRowGroup_);
}
if (statsPrunedRowGroup_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, statsPrunedRowGroup_);
}
if (dictPrunedRowGroup_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, dictPrunedRowGroup_);
}
if (requestRowGroup_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, requestRowGroup_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.TableScanSummary.ParquetRowGroupStats)) {
return super.equals(obj);
}
cz.proto.TableScanSummary.ParquetRowGroupStats other = (cz.proto.TableScanSummary.ParquetRowGroupStats) obj;
if (getBloomPrunedRowGroup()
!= other.getBloomPrunedRowGroup()) return false;
if (getStatsPrunedRowGroup()
!= other.getStatsPrunedRowGroup()) return false;
if (getDictPrunedRowGroup()
!= other.getDictPrunedRowGroup()) return false;
if (getRequestRowGroup()
!= other.getRequestRowGroup()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BLOOM_PRUNED_ROW_GROUP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBloomPrunedRowGroup());
hash = (37 * hash) + STATS_PRUNED_ROW_GROUP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStatsPrunedRowGroup());
hash = (37 * hash) + DICT_PRUNED_ROW_GROUP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDictPrunedRowGroup());
hash = (37 * hash) + REQUEST_ROW_GROUP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRequestRowGroup());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.TableScanSummary.ParquetRowGroupStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetRowGroupStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.TableScanSummary.ParquetRowGroupStats)
cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowGroupStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowGroupStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetRowGroupStats.class, cz.proto.TableScanSummary.ParquetRowGroupStats.Builder.class);
}
// Construct using cz.proto.TableScanSummary.ParquetRowGroupStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bloomPrunedRowGroup_ = 0L;
statsPrunedRowGroup_ = 0L;
dictPrunedRowGroup_ = 0L;
requestRowGroup_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowGroupStats_descriptor;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStats getDefaultInstanceForType() {
return cz.proto.TableScanSummary.ParquetRowGroupStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStats build() {
cz.proto.TableScanSummary.ParquetRowGroupStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStats buildPartial() {
cz.proto.TableScanSummary.ParquetRowGroupStats result = new cz.proto.TableScanSummary.ParquetRowGroupStats(this);
result.bloomPrunedRowGroup_ = bloomPrunedRowGroup_;
result.statsPrunedRowGroup_ = statsPrunedRowGroup_;
result.dictPrunedRowGroup_ = dictPrunedRowGroup_;
result.requestRowGroup_ = requestRowGroup_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.TableScanSummary.ParquetRowGroupStats) {
return mergeFrom((cz.proto.TableScanSummary.ParquetRowGroupStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.TableScanSummary.ParquetRowGroupStats other) {
if (other == cz.proto.TableScanSummary.ParquetRowGroupStats.getDefaultInstance()) return this;
if (other.getBloomPrunedRowGroup() != 0L) {
setBloomPrunedRowGroup(other.getBloomPrunedRowGroup());
}
if (other.getStatsPrunedRowGroup() != 0L) {
setStatsPrunedRowGroup(other.getStatsPrunedRowGroup());
}
if (other.getDictPrunedRowGroup() != 0L) {
setDictPrunedRowGroup(other.getDictPrunedRowGroup());
}
if (other.getRequestRowGroup() != 0L) {
setRequestRowGroup(other.getRequestRowGroup());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.TableScanSummary.ParquetRowGroupStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.TableScanSummary.ParquetRowGroupStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long bloomPrunedRowGroup_ ;
/**
* int64 bloom_pruned_row_group = 1;
* @return The bloomPrunedRowGroup.
*/
@java.lang.Override
public long getBloomPrunedRowGroup() {
return bloomPrunedRowGroup_;
}
/**
* int64 bloom_pruned_row_group = 1;
* @param value The bloomPrunedRowGroup to set.
* @return This builder for chaining.
*/
public Builder setBloomPrunedRowGroup(long value) {
bloomPrunedRowGroup_ = value;
onChanged();
return this;
}
/**
* int64 bloom_pruned_row_group = 1;
* @return This builder for chaining.
*/
public Builder clearBloomPrunedRowGroup() {
bloomPrunedRowGroup_ = 0L;
onChanged();
return this;
}
private long statsPrunedRowGroup_ ;
/**
* int64 stats_pruned_row_group = 2;
* @return The statsPrunedRowGroup.
*/
@java.lang.Override
public long getStatsPrunedRowGroup() {
return statsPrunedRowGroup_;
}
/**
* int64 stats_pruned_row_group = 2;
* @param value The statsPrunedRowGroup to set.
* @return This builder for chaining.
*/
public Builder setStatsPrunedRowGroup(long value) {
statsPrunedRowGroup_ = value;
onChanged();
return this;
}
/**
* int64 stats_pruned_row_group = 2;
* @return This builder for chaining.
*/
public Builder clearStatsPrunedRowGroup() {
statsPrunedRowGroup_ = 0L;
onChanged();
return this;
}
private long dictPrunedRowGroup_ ;
/**
* int64 dict_pruned_row_group = 3;
* @return The dictPrunedRowGroup.
*/
@java.lang.Override
public long getDictPrunedRowGroup() {
return dictPrunedRowGroup_;
}
/**
* int64 dict_pruned_row_group = 3;
* @param value The dictPrunedRowGroup to set.
* @return This builder for chaining.
*/
public Builder setDictPrunedRowGroup(long value) {
dictPrunedRowGroup_ = value;
onChanged();
return this;
}
/**
* int64 dict_pruned_row_group = 3;
* @return This builder for chaining.
*/
public Builder clearDictPrunedRowGroup() {
dictPrunedRowGroup_ = 0L;
onChanged();
return this;
}
private long requestRowGroup_ ;
/**
* int64 request_row_group = 4;
* @return The requestRowGroup.
*/
@java.lang.Override
public long getRequestRowGroup() {
return requestRowGroup_;
}
/**
* int64 request_row_group = 4;
* @param value The requestRowGroup to set.
* @return This builder for chaining.
*/
public Builder setRequestRowGroup(long value) {
requestRowGroup_ = value;
onChanged();
return this;
}
/**
* int64 request_row_group = 4;
* @return This builder for chaining.
*/
public Builder clearRequestRowGroup() {
requestRowGroup_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.TableScanSummary.ParquetRowGroupStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.TableScanSummary.ParquetRowGroupStats)
private static final cz.proto.TableScanSummary.ParquetRowGroupStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.TableScanSummary.ParquetRowGroupStats();
}
public static cz.proto.TableScanSummary.ParquetRowGroupStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParquetRowGroupStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ParquetRowGroupStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ParquetRowCountStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.TableScanSummary.ParquetRowCountStats)
com.google.protobuf.MessageOrBuilder {
/**
* int64 parquet_read_row_cnt = 1;
* @return The parquetReadRowCnt.
*/
long getParquetReadRowCnt();
/**
* int64 parquet_request_row_cnt = 2;
* @return The parquetRequestRowCnt.
*/
long getParquetRequestRowCnt();
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetRowCountStats}
*/
public static final class ParquetRowCountStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.TableScanSummary.ParquetRowCountStats)
ParquetRowCountStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParquetRowCountStats.newBuilder() to construct.
private ParquetRowCountStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParquetRowCountStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParquetRowCountStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ParquetRowCountStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
parquetReadRowCnt_ = input.readInt64();
break;
}
case 16: {
parquetRequestRowCnt_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowCountStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowCountStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetRowCountStats.class, cz.proto.TableScanSummary.ParquetRowCountStats.Builder.class);
}
public static final int PARQUET_READ_ROW_CNT_FIELD_NUMBER = 1;
private long parquetReadRowCnt_;
/**
* int64 parquet_read_row_cnt = 1;
* @return The parquetReadRowCnt.
*/
@java.lang.Override
public long getParquetReadRowCnt() {
return parquetReadRowCnt_;
}
public static final int PARQUET_REQUEST_ROW_CNT_FIELD_NUMBER = 2;
private long parquetRequestRowCnt_;
/**
* int64 parquet_request_row_cnt = 2;
* @return The parquetRequestRowCnt.
*/
@java.lang.Override
public long getParquetRequestRowCnt() {
return parquetRequestRowCnt_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (parquetReadRowCnt_ != 0L) {
output.writeInt64(1, parquetReadRowCnt_);
}
if (parquetRequestRowCnt_ != 0L) {
output.writeInt64(2, parquetRequestRowCnt_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (parquetReadRowCnt_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, parquetReadRowCnt_);
}
if (parquetRequestRowCnt_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, parquetRequestRowCnt_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.TableScanSummary.ParquetRowCountStats)) {
return super.equals(obj);
}
cz.proto.TableScanSummary.ParquetRowCountStats other = (cz.proto.TableScanSummary.ParquetRowCountStats) obj;
if (getParquetReadRowCnt()
!= other.getParquetReadRowCnt()) return false;
if (getParquetRequestRowCnt()
!= other.getParquetRequestRowCnt()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PARQUET_READ_ROW_CNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getParquetReadRowCnt());
hash = (37 * hash) + PARQUET_REQUEST_ROW_CNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getParquetRequestRowCnt());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetRowCountStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.TableScanSummary.ParquetRowCountStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetRowCountStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.TableScanSummary.ParquetRowCountStats)
cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowCountStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowCountStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetRowCountStats.class, cz.proto.TableScanSummary.ParquetRowCountStats.Builder.class);
}
// Construct using cz.proto.TableScanSummary.ParquetRowCountStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
parquetReadRowCnt_ = 0L;
parquetRequestRowCnt_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetRowCountStats_descriptor;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStats getDefaultInstanceForType() {
return cz.proto.TableScanSummary.ParquetRowCountStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStats build() {
cz.proto.TableScanSummary.ParquetRowCountStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStats buildPartial() {
cz.proto.TableScanSummary.ParquetRowCountStats result = new cz.proto.TableScanSummary.ParquetRowCountStats(this);
result.parquetReadRowCnt_ = parquetReadRowCnt_;
result.parquetRequestRowCnt_ = parquetRequestRowCnt_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.TableScanSummary.ParquetRowCountStats) {
return mergeFrom((cz.proto.TableScanSummary.ParquetRowCountStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.TableScanSummary.ParquetRowCountStats other) {
if (other == cz.proto.TableScanSummary.ParquetRowCountStats.getDefaultInstance()) return this;
if (other.getParquetReadRowCnt() != 0L) {
setParquetReadRowCnt(other.getParquetReadRowCnt());
}
if (other.getParquetRequestRowCnt() != 0L) {
setParquetRequestRowCnt(other.getParquetRequestRowCnt());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.TableScanSummary.ParquetRowCountStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.TableScanSummary.ParquetRowCountStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long parquetReadRowCnt_ ;
/**
* int64 parquet_read_row_cnt = 1;
* @return The parquetReadRowCnt.
*/
@java.lang.Override
public long getParquetReadRowCnt() {
return parquetReadRowCnt_;
}
/**
* int64 parquet_read_row_cnt = 1;
* @param value The parquetReadRowCnt to set.
* @return This builder for chaining.
*/
public Builder setParquetReadRowCnt(long value) {
parquetReadRowCnt_ = value;
onChanged();
return this;
}
/**
* int64 parquet_read_row_cnt = 1;
* @return This builder for chaining.
*/
public Builder clearParquetReadRowCnt() {
parquetReadRowCnt_ = 0L;
onChanged();
return this;
}
private long parquetRequestRowCnt_ ;
/**
* int64 parquet_request_row_cnt = 2;
* @return The parquetRequestRowCnt.
*/
@java.lang.Override
public long getParquetRequestRowCnt() {
return parquetRequestRowCnt_;
}
/**
* int64 parquet_request_row_cnt = 2;
* @param value The parquetRequestRowCnt to set.
* @return This builder for chaining.
*/
public Builder setParquetRequestRowCnt(long value) {
parquetRequestRowCnt_ = value;
onChanged();
return this;
}
/**
* int64 parquet_request_row_cnt = 2;
* @return This builder for chaining.
*/
public Builder clearParquetRequestRowCnt() {
parquetRequestRowCnt_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.TableScanSummary.ParquetRowCountStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.TableScanSummary.ParquetRowCountStats)
private static final cz.proto.TableScanSummary.ParquetRowCountStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.TableScanSummary.ParquetRowCountStats();
}
public static cz.proto.TableScanSummary.ParquetRowCountStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParquetRowCountStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ParquetRowCountStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ParquetPrunedSplitsStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.TableScanSummary.ParquetPrunedSplitsStats)
com.google.protobuf.MessageOrBuilder {
/**
* int64 pruned_file_cnt = 1;
* @return The prunedFileCnt.
*/
long getPrunedFileCnt();
/**
* int64 bitmap_pruned_splits = 2;
* @return The bitmapPrunedSplits.
*/
long getBitmapPrunedSplits();
/**
* int64 bloom_filter_pruned_splits = 3;
* @return The bloomFilterPrunedSplits.
*/
long getBloomFilterPrunedSplits();
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetPrunedSplitsStats}
*/
public static final class ParquetPrunedSplitsStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.TableScanSummary.ParquetPrunedSplitsStats)
ParquetPrunedSplitsStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParquetPrunedSplitsStats.newBuilder() to construct.
private ParquetPrunedSplitsStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParquetPrunedSplitsStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParquetPrunedSplitsStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ParquetPrunedSplitsStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
prunedFileCnt_ = input.readInt64();
break;
}
case 16: {
bitmapPrunedSplits_ = input.readInt64();
break;
}
case 24: {
bloomFilterPrunedSplits_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetPrunedSplitsStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetPrunedSplitsStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.class, cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder.class);
}
public static final int PRUNED_FILE_CNT_FIELD_NUMBER = 1;
private long prunedFileCnt_;
/**
* int64 pruned_file_cnt = 1;
* @return The prunedFileCnt.
*/
@java.lang.Override
public long getPrunedFileCnt() {
return prunedFileCnt_;
}
public static final int BITMAP_PRUNED_SPLITS_FIELD_NUMBER = 2;
private long bitmapPrunedSplits_;
/**
* int64 bitmap_pruned_splits = 2;
* @return The bitmapPrunedSplits.
*/
@java.lang.Override
public long getBitmapPrunedSplits() {
return bitmapPrunedSplits_;
}
public static final int BLOOM_FILTER_PRUNED_SPLITS_FIELD_NUMBER = 3;
private long bloomFilterPrunedSplits_;
/**
* int64 bloom_filter_pruned_splits = 3;
* @return The bloomFilterPrunedSplits.
*/
@java.lang.Override
public long getBloomFilterPrunedSplits() {
return bloomFilterPrunedSplits_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (prunedFileCnt_ != 0L) {
output.writeInt64(1, prunedFileCnt_);
}
if (bitmapPrunedSplits_ != 0L) {
output.writeInt64(2, bitmapPrunedSplits_);
}
if (bloomFilterPrunedSplits_ != 0L) {
output.writeInt64(3, bloomFilterPrunedSplits_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (prunedFileCnt_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, prunedFileCnt_);
}
if (bitmapPrunedSplits_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, bitmapPrunedSplits_);
}
if (bloomFilterPrunedSplits_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, bloomFilterPrunedSplits_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.TableScanSummary.ParquetPrunedSplitsStats)) {
return super.equals(obj);
}
cz.proto.TableScanSummary.ParquetPrunedSplitsStats other = (cz.proto.TableScanSummary.ParquetPrunedSplitsStats) obj;
if (getPrunedFileCnt()
!= other.getPrunedFileCnt()) return false;
if (getBitmapPrunedSplits()
!= other.getBitmapPrunedSplits()) return false;
if (getBloomFilterPrunedSplits()
!= other.getBloomFilterPrunedSplits()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PRUNED_FILE_CNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPrunedFileCnt());
hash = (37 * hash) + BITMAP_PRUNED_SPLITS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBitmapPrunedSplits());
hash = (37 * hash) + BLOOM_FILTER_PRUNED_SPLITS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBloomFilterPrunedSplits());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.TableScanSummary.ParquetPrunedSplitsStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.ParquetPrunedSplitsStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.TableScanSummary.ParquetPrunedSplitsStats)
cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetPrunedSplitsStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetPrunedSplitsStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.class, cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder.class);
}
// Construct using cz.proto.TableScanSummary.ParquetPrunedSplitsStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
prunedFileCnt_ = 0L;
bitmapPrunedSplits_ = 0L;
bloomFilterPrunedSplits_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_ParquetPrunedSplitsStats_descriptor;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats getDefaultInstanceForType() {
return cz.proto.TableScanSummary.ParquetPrunedSplitsStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats build() {
cz.proto.TableScanSummary.ParquetPrunedSplitsStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats buildPartial() {
cz.proto.TableScanSummary.ParquetPrunedSplitsStats result = new cz.proto.TableScanSummary.ParquetPrunedSplitsStats(this);
result.prunedFileCnt_ = prunedFileCnt_;
result.bitmapPrunedSplits_ = bitmapPrunedSplits_;
result.bloomFilterPrunedSplits_ = bloomFilterPrunedSplits_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.TableScanSummary.ParquetPrunedSplitsStats) {
return mergeFrom((cz.proto.TableScanSummary.ParquetPrunedSplitsStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.TableScanSummary.ParquetPrunedSplitsStats other) {
if (other == cz.proto.TableScanSummary.ParquetPrunedSplitsStats.getDefaultInstance()) return this;
if (other.getPrunedFileCnt() != 0L) {
setPrunedFileCnt(other.getPrunedFileCnt());
}
if (other.getBitmapPrunedSplits() != 0L) {
setBitmapPrunedSplits(other.getBitmapPrunedSplits());
}
if (other.getBloomFilterPrunedSplits() != 0L) {
setBloomFilterPrunedSplits(other.getBloomFilterPrunedSplits());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.TableScanSummary.ParquetPrunedSplitsStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.TableScanSummary.ParquetPrunedSplitsStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long prunedFileCnt_ ;
/**
* int64 pruned_file_cnt = 1;
* @return The prunedFileCnt.
*/
@java.lang.Override
public long getPrunedFileCnt() {
return prunedFileCnt_;
}
/**
* int64 pruned_file_cnt = 1;
* @param value The prunedFileCnt to set.
* @return This builder for chaining.
*/
public Builder setPrunedFileCnt(long value) {
prunedFileCnt_ = value;
onChanged();
return this;
}
/**
* int64 pruned_file_cnt = 1;
* @return This builder for chaining.
*/
public Builder clearPrunedFileCnt() {
prunedFileCnt_ = 0L;
onChanged();
return this;
}
private long bitmapPrunedSplits_ ;
/**
* int64 bitmap_pruned_splits = 2;
* @return The bitmapPrunedSplits.
*/
@java.lang.Override
public long getBitmapPrunedSplits() {
return bitmapPrunedSplits_;
}
/**
* int64 bitmap_pruned_splits = 2;
* @param value The bitmapPrunedSplits to set.
* @return This builder for chaining.
*/
public Builder setBitmapPrunedSplits(long value) {
bitmapPrunedSplits_ = value;
onChanged();
return this;
}
/**
* int64 bitmap_pruned_splits = 2;
* @return This builder for chaining.
*/
public Builder clearBitmapPrunedSplits() {
bitmapPrunedSplits_ = 0L;
onChanged();
return this;
}
private long bloomFilterPrunedSplits_ ;
/**
* int64 bloom_filter_pruned_splits = 3;
* @return The bloomFilterPrunedSplits.
*/
@java.lang.Override
public long getBloomFilterPrunedSplits() {
return bloomFilterPrunedSplits_;
}
/**
* int64 bloom_filter_pruned_splits = 3;
* @param value The bloomFilterPrunedSplits to set.
* @return This builder for chaining.
*/
public Builder setBloomFilterPrunedSplits(long value) {
bloomFilterPrunedSplits_ = value;
onChanged();
return this;
}
/**
* int64 bloom_filter_pruned_splits = 3;
* @return This builder for chaining.
*/
public Builder clearBloomFilterPrunedSplits() {
bloomFilterPrunedSplits_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.TableScanSummary.ParquetPrunedSplitsStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.TableScanSummary.ParquetPrunedSplitsStats)
private static final cz.proto.TableScanSummary.ParquetPrunedSplitsStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.TableScanSummary.ParquetPrunedSplitsStats();
}
public static cz.proto.TableScanSummary.ParquetPrunedSplitsStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParquetPrunedSplitsStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ParquetPrunedSplitsStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TableScanSourceStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.TableScanSummary.TableScanSourceStats)
com.google.protobuf.MessageOrBuilder {
/**
* int64 short_circuit_bytes = 1;
* @return The shortCircuitBytes.
*/
long getShortCircuitBytes();
/**
* float short_circuit_percentage = 2;
* @return The shortCircuitPercentage.
*/
float getShortCircuitPercentage();
/**
* int64 rpc_bytes = 3;
* @return The rpcBytes.
*/
long getRpcBytes();
/**
* float rpc_percentage = 4;
* @return The rpcPercentage.
*/
float getRpcPercentage();
/**
* int64 object_storage_bytes = 5;
* @return The objectStorageBytes.
*/
long getObjectStorageBytes();
/**
* float object_storage_percentage = 6;
* @return The objectStoragePercentage.
*/
float getObjectStoragePercentage();
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.TableScanSourceStats}
*/
public static final class TableScanSourceStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.TableScanSummary.TableScanSourceStats)
TableScanSourceStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableScanSourceStats.newBuilder() to construct.
private TableScanSourceStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableScanSourceStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableScanSourceStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableScanSourceStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
shortCircuitBytes_ = input.readInt64();
break;
}
case 21: {
shortCircuitPercentage_ = input.readFloat();
break;
}
case 24: {
rpcBytes_ = input.readInt64();
break;
}
case 37: {
rpcPercentage_ = input.readFloat();
break;
}
case 40: {
objectStorageBytes_ = input.readInt64();
break;
}
case 53: {
objectStoragePercentage_ = input.readFloat();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_TableScanSourceStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_TableScanSourceStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.TableScanSourceStats.class, cz.proto.TableScanSummary.TableScanSourceStats.Builder.class);
}
public static final int SHORT_CIRCUIT_BYTES_FIELD_NUMBER = 1;
private long shortCircuitBytes_;
/**
* int64 short_circuit_bytes = 1;
* @return The shortCircuitBytes.
*/
@java.lang.Override
public long getShortCircuitBytes() {
return shortCircuitBytes_;
}
public static final int SHORT_CIRCUIT_PERCENTAGE_FIELD_NUMBER = 2;
private float shortCircuitPercentage_;
/**
* float short_circuit_percentage = 2;
* @return The shortCircuitPercentage.
*/
@java.lang.Override
public float getShortCircuitPercentage() {
return shortCircuitPercentage_;
}
public static final int RPC_BYTES_FIELD_NUMBER = 3;
private long rpcBytes_;
/**
* int64 rpc_bytes = 3;
* @return The rpcBytes.
*/
@java.lang.Override
public long getRpcBytes() {
return rpcBytes_;
}
public static final int RPC_PERCENTAGE_FIELD_NUMBER = 4;
private float rpcPercentage_;
/**
* float rpc_percentage = 4;
* @return The rpcPercentage.
*/
@java.lang.Override
public float getRpcPercentage() {
return rpcPercentage_;
}
public static final int OBJECT_STORAGE_BYTES_FIELD_NUMBER = 5;
private long objectStorageBytes_;
/**
* int64 object_storage_bytes = 5;
* @return The objectStorageBytes.
*/
@java.lang.Override
public long getObjectStorageBytes() {
return objectStorageBytes_;
}
public static final int OBJECT_STORAGE_PERCENTAGE_FIELD_NUMBER = 6;
private float objectStoragePercentage_;
/**
* float object_storage_percentage = 6;
* @return The objectStoragePercentage.
*/
@java.lang.Override
public float getObjectStoragePercentage() {
return objectStoragePercentage_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (shortCircuitBytes_ != 0L) {
output.writeInt64(1, shortCircuitBytes_);
}
if (java.lang.Float.floatToRawIntBits(shortCircuitPercentage_) != 0) {
output.writeFloat(2, shortCircuitPercentage_);
}
if (rpcBytes_ != 0L) {
output.writeInt64(3, rpcBytes_);
}
if (java.lang.Float.floatToRawIntBits(rpcPercentage_) != 0) {
output.writeFloat(4, rpcPercentage_);
}
if (objectStorageBytes_ != 0L) {
output.writeInt64(5, objectStorageBytes_);
}
if (java.lang.Float.floatToRawIntBits(objectStoragePercentage_) != 0) {
output.writeFloat(6, objectStoragePercentage_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (shortCircuitBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, shortCircuitBytes_);
}
if (java.lang.Float.floatToRawIntBits(shortCircuitPercentage_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(2, shortCircuitPercentage_);
}
if (rpcBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, rpcBytes_);
}
if (java.lang.Float.floatToRawIntBits(rpcPercentage_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(4, rpcPercentage_);
}
if (objectStorageBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, objectStorageBytes_);
}
if (java.lang.Float.floatToRawIntBits(objectStoragePercentage_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(6, objectStoragePercentage_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.TableScanSummary.TableScanSourceStats)) {
return super.equals(obj);
}
cz.proto.TableScanSummary.TableScanSourceStats other = (cz.proto.TableScanSummary.TableScanSourceStats) obj;
if (getShortCircuitBytes()
!= other.getShortCircuitBytes()) return false;
if (java.lang.Float.floatToIntBits(getShortCircuitPercentage())
!= java.lang.Float.floatToIntBits(
other.getShortCircuitPercentage())) return false;
if (getRpcBytes()
!= other.getRpcBytes()) return false;
if (java.lang.Float.floatToIntBits(getRpcPercentage())
!= java.lang.Float.floatToIntBits(
other.getRpcPercentage())) return false;
if (getObjectStorageBytes()
!= other.getObjectStorageBytes()) return false;
if (java.lang.Float.floatToIntBits(getObjectStoragePercentage())
!= java.lang.Float.floatToIntBits(
other.getObjectStoragePercentage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SHORT_CIRCUIT_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getShortCircuitBytes());
hash = (37 * hash) + SHORT_CIRCUIT_PERCENTAGE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getShortCircuitPercentage());
hash = (37 * hash) + RPC_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRpcBytes());
hash = (37 * hash) + RPC_PERCENTAGE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getRpcPercentage());
hash = (37 * hash) + OBJECT_STORAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getObjectStorageBytes());
hash = (37 * hash) + OBJECT_STORAGE_PERCENTAGE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getObjectStoragePercentage());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary.TableScanSourceStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.TableScanSummary.TableScanSourceStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.TableScanSummary.TableScanSourceStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.TableScanSummary.TableScanSourceStats)
cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_TableScanSourceStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_TableScanSourceStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.TableScanSourceStats.class, cz.proto.TableScanSummary.TableScanSourceStats.Builder.class);
}
// Construct using cz.proto.TableScanSummary.TableScanSourceStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
shortCircuitBytes_ = 0L;
shortCircuitPercentage_ = 0F;
rpcBytes_ = 0L;
rpcPercentage_ = 0F;
objectStorageBytes_ = 0L;
objectStoragePercentage_ = 0F;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_TableScanSourceStats_descriptor;
}
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStats getDefaultInstanceForType() {
return cz.proto.TableScanSummary.TableScanSourceStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStats build() {
cz.proto.TableScanSummary.TableScanSourceStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStats buildPartial() {
cz.proto.TableScanSummary.TableScanSourceStats result = new cz.proto.TableScanSummary.TableScanSourceStats(this);
result.shortCircuitBytes_ = shortCircuitBytes_;
result.shortCircuitPercentage_ = shortCircuitPercentage_;
result.rpcBytes_ = rpcBytes_;
result.rpcPercentage_ = rpcPercentage_;
result.objectStorageBytes_ = objectStorageBytes_;
result.objectStoragePercentage_ = objectStoragePercentage_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.TableScanSummary.TableScanSourceStats) {
return mergeFrom((cz.proto.TableScanSummary.TableScanSourceStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.TableScanSummary.TableScanSourceStats other) {
if (other == cz.proto.TableScanSummary.TableScanSourceStats.getDefaultInstance()) return this;
if (other.getShortCircuitBytes() != 0L) {
setShortCircuitBytes(other.getShortCircuitBytes());
}
if (other.getShortCircuitPercentage() != 0F) {
setShortCircuitPercentage(other.getShortCircuitPercentage());
}
if (other.getRpcBytes() != 0L) {
setRpcBytes(other.getRpcBytes());
}
if (other.getRpcPercentage() != 0F) {
setRpcPercentage(other.getRpcPercentage());
}
if (other.getObjectStorageBytes() != 0L) {
setObjectStorageBytes(other.getObjectStorageBytes());
}
if (other.getObjectStoragePercentage() != 0F) {
setObjectStoragePercentage(other.getObjectStoragePercentage());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.TableScanSummary.TableScanSourceStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.TableScanSummary.TableScanSourceStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long shortCircuitBytes_ ;
/**
* int64 short_circuit_bytes = 1;
* @return The shortCircuitBytes.
*/
@java.lang.Override
public long getShortCircuitBytes() {
return shortCircuitBytes_;
}
/**
* int64 short_circuit_bytes = 1;
* @param value The shortCircuitBytes to set.
* @return This builder for chaining.
*/
public Builder setShortCircuitBytes(long value) {
shortCircuitBytes_ = value;
onChanged();
return this;
}
/**
* int64 short_circuit_bytes = 1;
* @return This builder for chaining.
*/
public Builder clearShortCircuitBytes() {
shortCircuitBytes_ = 0L;
onChanged();
return this;
}
private float shortCircuitPercentage_ ;
/**
* float short_circuit_percentage = 2;
* @return The shortCircuitPercentage.
*/
@java.lang.Override
public float getShortCircuitPercentage() {
return shortCircuitPercentage_;
}
/**
* float short_circuit_percentage = 2;
* @param value The shortCircuitPercentage to set.
* @return This builder for chaining.
*/
public Builder setShortCircuitPercentage(float value) {
shortCircuitPercentage_ = value;
onChanged();
return this;
}
/**
* float short_circuit_percentage = 2;
* @return This builder for chaining.
*/
public Builder clearShortCircuitPercentage() {
shortCircuitPercentage_ = 0F;
onChanged();
return this;
}
private long rpcBytes_ ;
/**
* int64 rpc_bytes = 3;
* @return The rpcBytes.
*/
@java.lang.Override
public long getRpcBytes() {
return rpcBytes_;
}
/**
* int64 rpc_bytes = 3;
* @param value The rpcBytes to set.
* @return This builder for chaining.
*/
public Builder setRpcBytes(long value) {
rpcBytes_ = value;
onChanged();
return this;
}
/**
* int64 rpc_bytes = 3;
* @return This builder for chaining.
*/
public Builder clearRpcBytes() {
rpcBytes_ = 0L;
onChanged();
return this;
}
private float rpcPercentage_ ;
/**
* float rpc_percentage = 4;
* @return The rpcPercentage.
*/
@java.lang.Override
public float getRpcPercentage() {
return rpcPercentage_;
}
/**
* float rpc_percentage = 4;
* @param value The rpcPercentage to set.
* @return This builder for chaining.
*/
public Builder setRpcPercentage(float value) {
rpcPercentage_ = value;
onChanged();
return this;
}
/**
* float rpc_percentage = 4;
* @return This builder for chaining.
*/
public Builder clearRpcPercentage() {
rpcPercentage_ = 0F;
onChanged();
return this;
}
private long objectStorageBytes_ ;
/**
* int64 object_storage_bytes = 5;
* @return The objectStorageBytes.
*/
@java.lang.Override
public long getObjectStorageBytes() {
return objectStorageBytes_;
}
/**
* int64 object_storage_bytes = 5;
* @param value The objectStorageBytes to set.
* @return This builder for chaining.
*/
public Builder setObjectStorageBytes(long value) {
objectStorageBytes_ = value;
onChanged();
return this;
}
/**
* int64 object_storage_bytes = 5;
* @return This builder for chaining.
*/
public Builder clearObjectStorageBytes() {
objectStorageBytes_ = 0L;
onChanged();
return this;
}
private float objectStoragePercentage_ ;
/**
* float object_storage_percentage = 6;
* @return The objectStoragePercentage.
*/
@java.lang.Override
public float getObjectStoragePercentage() {
return objectStoragePercentage_;
}
/**
* float object_storage_percentage = 6;
* @param value The objectStoragePercentage to set.
* @return This builder for chaining.
*/
public Builder setObjectStoragePercentage(float value) {
objectStoragePercentage_ = value;
onChanged();
return this;
}
/**
* float object_storage_percentage = 6;
* @return This builder for chaining.
*/
public Builder clearObjectStoragePercentage() {
objectStoragePercentage_ = 0F;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.TableScanSummary.TableScanSourceStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.TableScanSummary.TableScanSourceStats)
private static final cz.proto.TableScanSummary.TableScanSourceStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.TableScanSummary.TableScanSourceStats();
}
public static cz.proto.TableScanSummary.TableScanSourceStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableScanSourceStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableScanSourceStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int INPUT_BYTES_FIELD_NUMBER = 1;
private cz.proto.OperatorStatistics inputBytes_;
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
* @return Whether the inputBytes field is set.
*/
@java.lang.Override
public boolean hasInputBytes() {
return inputBytes_ != null;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
* @return The inputBytes.
*/
@java.lang.Override
public cz.proto.OperatorStatistics getInputBytes() {
return inputBytes_ == null ? cz.proto.OperatorStatistics.getDefaultInstance() : inputBytes_;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
@java.lang.Override
public cz.proto.OperatorStatisticsOrBuilder getInputBytesOrBuilder() {
return getInputBytes();
}
public static final int SPLIT_CNT_FIELD_NUMBER = 2;
private cz.proto.OperatorStatistics splitCnt_;
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
* @return Whether the splitCnt field is set.
*/
@java.lang.Override
public boolean hasSplitCnt() {
return splitCnt_ != null;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
* @return The splitCnt.
*/
@java.lang.Override
public cz.proto.OperatorStatistics getSplitCnt() {
return splitCnt_ == null ? cz.proto.OperatorStatistics.getDefaultInstance() : splitCnt_;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
@java.lang.Override
public cz.proto.OperatorStatisticsOrBuilder getSplitCntOrBuilder() {
return getSplitCnt();
}
public static final int TABLE_SCAN_SOURCE_STATS_FIELD_NUMBER = 3;
private cz.proto.TableScanSummary.TableScanSourceStats tableScanSourceStats_;
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
* @return Whether the tableScanSourceStats field is set.
*/
@java.lang.Override
public boolean hasTableScanSourceStats() {
return tableScanSourceStats_ != null;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
* @return The tableScanSourceStats.
*/
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStats getTableScanSourceStats() {
return tableScanSourceStats_ == null ? cz.proto.TableScanSummary.TableScanSourceStats.getDefaultInstance() : tableScanSourceStats_;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
@java.lang.Override
public cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder getTableScanSourceStatsOrBuilder() {
return getTableScanSourceStats();
}
public static final int PARQUET_ROW_GROUP_STATS_FIELD_NUMBER = 4;
private cz.proto.TableScanSummary.ParquetRowGroupStats parquetRowGroupStats_;
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
* @return Whether the parquetRowGroupStats field is set.
*/
@java.lang.Override
public boolean hasParquetRowGroupStats() {
return parquetRowGroupStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
* @return The parquetRowGroupStats.
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStats getParquetRowGroupStats() {
return parquetRowGroupStats_ == null ? cz.proto.TableScanSummary.ParquetRowGroupStats.getDefaultInstance() : parquetRowGroupStats_;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder getParquetRowGroupStatsOrBuilder() {
return getParquetRowGroupStats();
}
public static final int PARQUET_ROW_COUNT_STATS_FIELD_NUMBER = 5;
private cz.proto.TableScanSummary.ParquetRowCountStats parquetRowCountStats_;
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
* @return Whether the parquetRowCountStats field is set.
*/
@java.lang.Override
public boolean hasParquetRowCountStats() {
return parquetRowCountStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
* @return The parquetRowCountStats.
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStats getParquetRowCountStats() {
return parquetRowCountStats_ == null ? cz.proto.TableScanSummary.ParquetRowCountStats.getDefaultInstance() : parquetRowCountStats_;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder getParquetRowCountStatsOrBuilder() {
return getParquetRowCountStats();
}
public static final int PARQUET_PRUNED_SPLITS_STATS_FIELD_NUMBER = 6;
private cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquetPrunedSplitsStats_;
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
* @return Whether the parquetPrunedSplitsStats field is set.
*/
@java.lang.Override
public boolean hasParquetPrunedSplitsStats() {
return parquetPrunedSplitsStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
* @return The parquetPrunedSplitsStats.
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats getParquetPrunedSplitsStats() {
return parquetPrunedSplitsStats_ == null ? cz.proto.TableScanSummary.ParquetPrunedSplitsStats.getDefaultInstance() : parquetPrunedSplitsStats_;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
@java.lang.Override
public cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder getParquetPrunedSplitsStatsOrBuilder() {
return getParquetPrunedSplitsStats();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (inputBytes_ != null) {
output.writeMessage(1, getInputBytes());
}
if (splitCnt_ != null) {
output.writeMessage(2, getSplitCnt());
}
if (tableScanSourceStats_ != null) {
output.writeMessage(3, getTableScanSourceStats());
}
if (parquetRowGroupStats_ != null) {
output.writeMessage(4, getParquetRowGroupStats());
}
if (parquetRowCountStats_ != null) {
output.writeMessage(5, getParquetRowCountStats());
}
if (parquetPrunedSplitsStats_ != null) {
output.writeMessage(6, getParquetPrunedSplitsStats());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (inputBytes_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getInputBytes());
}
if (splitCnt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getSplitCnt());
}
if (tableScanSourceStats_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTableScanSourceStats());
}
if (parquetRowGroupStats_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getParquetRowGroupStats());
}
if (parquetRowCountStats_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getParquetRowCountStats());
}
if (parquetPrunedSplitsStats_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getParquetPrunedSplitsStats());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.TableScanSummary)) {
return super.equals(obj);
}
cz.proto.TableScanSummary other = (cz.proto.TableScanSummary) obj;
if (hasInputBytes() != other.hasInputBytes()) return false;
if (hasInputBytes()) {
if (!getInputBytes()
.equals(other.getInputBytes())) return false;
}
if (hasSplitCnt() != other.hasSplitCnt()) return false;
if (hasSplitCnt()) {
if (!getSplitCnt()
.equals(other.getSplitCnt())) return false;
}
if (hasTableScanSourceStats() != other.hasTableScanSourceStats()) return false;
if (hasTableScanSourceStats()) {
if (!getTableScanSourceStats()
.equals(other.getTableScanSourceStats())) return false;
}
if (hasParquetRowGroupStats() != other.hasParquetRowGroupStats()) return false;
if (hasParquetRowGroupStats()) {
if (!getParquetRowGroupStats()
.equals(other.getParquetRowGroupStats())) return false;
}
if (hasParquetRowCountStats() != other.hasParquetRowCountStats()) return false;
if (hasParquetRowCountStats()) {
if (!getParquetRowCountStats()
.equals(other.getParquetRowCountStats())) return false;
}
if (hasParquetPrunedSplitsStats() != other.hasParquetPrunedSplitsStats()) return false;
if (hasParquetPrunedSplitsStats()) {
if (!getParquetPrunedSplitsStats()
.equals(other.getParquetPrunedSplitsStats())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInputBytes()) {
hash = (37 * hash) + INPUT_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getInputBytes().hashCode();
}
if (hasSplitCnt()) {
hash = (37 * hash) + SPLIT_CNT_FIELD_NUMBER;
hash = (53 * hash) + getSplitCnt().hashCode();
}
if (hasTableScanSourceStats()) {
hash = (37 * hash) + TABLE_SCAN_SOURCE_STATS_FIELD_NUMBER;
hash = (53 * hash) + getTableScanSourceStats().hashCode();
}
if (hasParquetRowGroupStats()) {
hash = (37 * hash) + PARQUET_ROW_GROUP_STATS_FIELD_NUMBER;
hash = (53 * hash) + getParquetRowGroupStats().hashCode();
}
if (hasParquetRowCountStats()) {
hash = (37 * hash) + PARQUET_ROW_COUNT_STATS_FIELD_NUMBER;
hash = (53 * hash) + getParquetRowCountStats().hashCode();
}
if (hasParquetPrunedSplitsStats()) {
hash = (37 * hash) + PARQUET_PRUNED_SPLITS_STATS_FIELD_NUMBER;
hash = (53 * hash) + getParquetPrunedSplitsStats().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.TableScanSummary parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.TableScanSummary parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.TableScanSummary parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.TableScanSummary parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.TableScanSummary parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.TableScanSummary prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.TableScanSummary}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.TableScanSummary)
cz.proto.TableScanSummaryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.TableScanSummary.class, cz.proto.TableScanSummary.Builder.class);
}
// Construct using cz.proto.TableScanSummary.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (inputBytesBuilder_ == null) {
inputBytes_ = null;
} else {
inputBytes_ = null;
inputBytesBuilder_ = null;
}
if (splitCntBuilder_ == null) {
splitCnt_ = null;
} else {
splitCnt_ = null;
splitCntBuilder_ = null;
}
if (tableScanSourceStatsBuilder_ == null) {
tableScanSourceStats_ = null;
} else {
tableScanSourceStats_ = null;
tableScanSourceStatsBuilder_ = null;
}
if (parquetRowGroupStatsBuilder_ == null) {
parquetRowGroupStats_ = null;
} else {
parquetRowGroupStats_ = null;
parquetRowGroupStatsBuilder_ = null;
}
if (parquetRowCountStatsBuilder_ == null) {
parquetRowCountStats_ = null;
} else {
parquetRowCountStats_ = null;
parquetRowCountStatsBuilder_ = null;
}
if (parquetPrunedSplitsStatsBuilder_ == null) {
parquetPrunedSplitsStats_ = null;
} else {
parquetPrunedSplitsStats_ = null;
parquetPrunedSplitsStatsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_TableScanSummary_descriptor;
}
@java.lang.Override
public cz.proto.TableScanSummary getDefaultInstanceForType() {
return cz.proto.TableScanSummary.getDefaultInstance();
}
@java.lang.Override
public cz.proto.TableScanSummary build() {
cz.proto.TableScanSummary result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.TableScanSummary buildPartial() {
cz.proto.TableScanSummary result = new cz.proto.TableScanSummary(this);
if (inputBytesBuilder_ == null) {
result.inputBytes_ = inputBytes_;
} else {
result.inputBytes_ = inputBytesBuilder_.build();
}
if (splitCntBuilder_ == null) {
result.splitCnt_ = splitCnt_;
} else {
result.splitCnt_ = splitCntBuilder_.build();
}
if (tableScanSourceStatsBuilder_ == null) {
result.tableScanSourceStats_ = tableScanSourceStats_;
} else {
result.tableScanSourceStats_ = tableScanSourceStatsBuilder_.build();
}
if (parquetRowGroupStatsBuilder_ == null) {
result.parquetRowGroupStats_ = parquetRowGroupStats_;
} else {
result.parquetRowGroupStats_ = parquetRowGroupStatsBuilder_.build();
}
if (parquetRowCountStatsBuilder_ == null) {
result.parquetRowCountStats_ = parquetRowCountStats_;
} else {
result.parquetRowCountStats_ = parquetRowCountStatsBuilder_.build();
}
if (parquetPrunedSplitsStatsBuilder_ == null) {
result.parquetPrunedSplitsStats_ = parquetPrunedSplitsStats_;
} else {
result.parquetPrunedSplitsStats_ = parquetPrunedSplitsStatsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.TableScanSummary) {
return mergeFrom((cz.proto.TableScanSummary)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.TableScanSummary other) {
if (other == cz.proto.TableScanSummary.getDefaultInstance()) return this;
if (other.hasInputBytes()) {
mergeInputBytes(other.getInputBytes());
}
if (other.hasSplitCnt()) {
mergeSplitCnt(other.getSplitCnt());
}
if (other.hasTableScanSourceStats()) {
mergeTableScanSourceStats(other.getTableScanSourceStats());
}
if (other.hasParquetRowGroupStats()) {
mergeParquetRowGroupStats(other.getParquetRowGroupStats());
}
if (other.hasParquetRowCountStats()) {
mergeParquetRowCountStats(other.getParquetRowCountStats());
}
if (other.hasParquetPrunedSplitsStats()) {
mergeParquetPrunedSplitsStats(other.getParquetPrunedSplitsStats());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.TableScanSummary parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.TableScanSummary) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.OperatorStatistics inputBytes_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder> inputBytesBuilder_;
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
* @return Whether the inputBytes field is set.
*/
public boolean hasInputBytes() {
return inputBytesBuilder_ != null || inputBytes_ != null;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
* @return The inputBytes.
*/
public cz.proto.OperatorStatistics getInputBytes() {
if (inputBytesBuilder_ == null) {
return inputBytes_ == null ? cz.proto.OperatorStatistics.getDefaultInstance() : inputBytes_;
} else {
return inputBytesBuilder_.getMessage();
}
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public Builder setInputBytes(cz.proto.OperatorStatistics value) {
if (inputBytesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputBytes_ = value;
onChanged();
} else {
inputBytesBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public Builder setInputBytes(
cz.proto.OperatorStatistics.Builder builderForValue) {
if (inputBytesBuilder_ == null) {
inputBytes_ = builderForValue.build();
onChanged();
} else {
inputBytesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public Builder mergeInputBytes(cz.proto.OperatorStatistics value) {
if (inputBytesBuilder_ == null) {
if (inputBytes_ != null) {
inputBytes_ =
cz.proto.OperatorStatistics.newBuilder(inputBytes_).mergeFrom(value).buildPartial();
} else {
inputBytes_ = value;
}
onChanged();
} else {
inputBytesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public Builder clearInputBytes() {
if (inputBytesBuilder_ == null) {
inputBytes_ = null;
onChanged();
} else {
inputBytes_ = null;
inputBytesBuilder_ = null;
}
return this;
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public cz.proto.OperatorStatistics.Builder getInputBytesBuilder() {
onChanged();
return getInputBytesFieldBuilder().getBuilder();
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
public cz.proto.OperatorStatisticsOrBuilder getInputBytesOrBuilder() {
if (inputBytesBuilder_ != null) {
return inputBytesBuilder_.getMessageOrBuilder();
} else {
return inputBytes_ == null ?
cz.proto.OperatorStatistics.getDefaultInstance() : inputBytes_;
}
}
/**
* .cz.proto.OperatorStatistics input_bytes = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder>
getInputBytesFieldBuilder() {
if (inputBytesBuilder_ == null) {
inputBytesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder>(
getInputBytes(),
getParentForChildren(),
isClean());
inputBytes_ = null;
}
return inputBytesBuilder_;
}
private cz.proto.OperatorStatistics splitCnt_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder> splitCntBuilder_;
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
* @return Whether the splitCnt field is set.
*/
public boolean hasSplitCnt() {
return splitCntBuilder_ != null || splitCnt_ != null;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
* @return The splitCnt.
*/
public cz.proto.OperatorStatistics getSplitCnt() {
if (splitCntBuilder_ == null) {
return splitCnt_ == null ? cz.proto.OperatorStatistics.getDefaultInstance() : splitCnt_;
} else {
return splitCntBuilder_.getMessage();
}
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public Builder setSplitCnt(cz.proto.OperatorStatistics value) {
if (splitCntBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
splitCnt_ = value;
onChanged();
} else {
splitCntBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public Builder setSplitCnt(
cz.proto.OperatorStatistics.Builder builderForValue) {
if (splitCntBuilder_ == null) {
splitCnt_ = builderForValue.build();
onChanged();
} else {
splitCntBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public Builder mergeSplitCnt(cz.proto.OperatorStatistics value) {
if (splitCntBuilder_ == null) {
if (splitCnt_ != null) {
splitCnt_ =
cz.proto.OperatorStatistics.newBuilder(splitCnt_).mergeFrom(value).buildPartial();
} else {
splitCnt_ = value;
}
onChanged();
} else {
splitCntBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public Builder clearSplitCnt() {
if (splitCntBuilder_ == null) {
splitCnt_ = null;
onChanged();
} else {
splitCnt_ = null;
splitCntBuilder_ = null;
}
return this;
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public cz.proto.OperatorStatistics.Builder getSplitCntBuilder() {
onChanged();
return getSplitCntFieldBuilder().getBuilder();
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
public cz.proto.OperatorStatisticsOrBuilder getSplitCntOrBuilder() {
if (splitCntBuilder_ != null) {
return splitCntBuilder_.getMessageOrBuilder();
} else {
return splitCnt_ == null ?
cz.proto.OperatorStatistics.getDefaultInstance() : splitCnt_;
}
}
/**
* .cz.proto.OperatorStatistics split_cnt = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder>
getSplitCntFieldBuilder() {
if (splitCntBuilder_ == null) {
splitCntBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.OperatorStatistics, cz.proto.OperatorStatistics.Builder, cz.proto.OperatorStatisticsOrBuilder>(
getSplitCnt(),
getParentForChildren(),
isClean());
splitCnt_ = null;
}
return splitCntBuilder_;
}
private cz.proto.TableScanSummary.TableScanSourceStats tableScanSourceStats_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.TableScanSourceStats, cz.proto.TableScanSummary.TableScanSourceStats.Builder, cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder> tableScanSourceStatsBuilder_;
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
* @return Whether the tableScanSourceStats field is set.
*/
public boolean hasTableScanSourceStats() {
return tableScanSourceStatsBuilder_ != null || tableScanSourceStats_ != null;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
* @return The tableScanSourceStats.
*/
public cz.proto.TableScanSummary.TableScanSourceStats getTableScanSourceStats() {
if (tableScanSourceStatsBuilder_ == null) {
return tableScanSourceStats_ == null ? cz.proto.TableScanSummary.TableScanSourceStats.getDefaultInstance() : tableScanSourceStats_;
} else {
return tableScanSourceStatsBuilder_.getMessage();
}
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public Builder setTableScanSourceStats(cz.proto.TableScanSummary.TableScanSourceStats value) {
if (tableScanSourceStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableScanSourceStats_ = value;
onChanged();
} else {
tableScanSourceStatsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public Builder setTableScanSourceStats(
cz.proto.TableScanSummary.TableScanSourceStats.Builder builderForValue) {
if (tableScanSourceStatsBuilder_ == null) {
tableScanSourceStats_ = builderForValue.build();
onChanged();
} else {
tableScanSourceStatsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public Builder mergeTableScanSourceStats(cz.proto.TableScanSummary.TableScanSourceStats value) {
if (tableScanSourceStatsBuilder_ == null) {
if (tableScanSourceStats_ != null) {
tableScanSourceStats_ =
cz.proto.TableScanSummary.TableScanSourceStats.newBuilder(tableScanSourceStats_).mergeFrom(value).buildPartial();
} else {
tableScanSourceStats_ = value;
}
onChanged();
} else {
tableScanSourceStatsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public Builder clearTableScanSourceStats() {
if (tableScanSourceStatsBuilder_ == null) {
tableScanSourceStats_ = null;
onChanged();
} else {
tableScanSourceStats_ = null;
tableScanSourceStatsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public cz.proto.TableScanSummary.TableScanSourceStats.Builder getTableScanSourceStatsBuilder() {
onChanged();
return getTableScanSourceStatsFieldBuilder().getBuilder();
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
public cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder getTableScanSourceStatsOrBuilder() {
if (tableScanSourceStatsBuilder_ != null) {
return tableScanSourceStatsBuilder_.getMessageOrBuilder();
} else {
return tableScanSourceStats_ == null ?
cz.proto.TableScanSummary.TableScanSourceStats.getDefaultInstance() : tableScanSourceStats_;
}
}
/**
* .cz.proto.TableScanSummary.TableScanSourceStats table_scan_source_stats = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.TableScanSourceStats, cz.proto.TableScanSummary.TableScanSourceStats.Builder, cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder>
getTableScanSourceStatsFieldBuilder() {
if (tableScanSourceStatsBuilder_ == null) {
tableScanSourceStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.TableScanSourceStats, cz.proto.TableScanSummary.TableScanSourceStats.Builder, cz.proto.TableScanSummary.TableScanSourceStatsOrBuilder>(
getTableScanSourceStats(),
getParentForChildren(),
isClean());
tableScanSourceStats_ = null;
}
return tableScanSourceStatsBuilder_;
}
private cz.proto.TableScanSummary.ParquetRowGroupStats parquetRowGroupStats_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowGroupStats, cz.proto.TableScanSummary.ParquetRowGroupStats.Builder, cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder> parquetRowGroupStatsBuilder_;
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
* @return Whether the parquetRowGroupStats field is set.
*/
public boolean hasParquetRowGroupStats() {
return parquetRowGroupStatsBuilder_ != null || parquetRowGroupStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
* @return The parquetRowGroupStats.
*/
public cz.proto.TableScanSummary.ParquetRowGroupStats getParquetRowGroupStats() {
if (parquetRowGroupStatsBuilder_ == null) {
return parquetRowGroupStats_ == null ? cz.proto.TableScanSummary.ParquetRowGroupStats.getDefaultInstance() : parquetRowGroupStats_;
} else {
return parquetRowGroupStatsBuilder_.getMessage();
}
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public Builder setParquetRowGroupStats(cz.proto.TableScanSummary.ParquetRowGroupStats value) {
if (parquetRowGroupStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
parquetRowGroupStats_ = value;
onChanged();
} else {
parquetRowGroupStatsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public Builder setParquetRowGroupStats(
cz.proto.TableScanSummary.ParquetRowGroupStats.Builder builderForValue) {
if (parquetRowGroupStatsBuilder_ == null) {
parquetRowGroupStats_ = builderForValue.build();
onChanged();
} else {
parquetRowGroupStatsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public Builder mergeParquetRowGroupStats(cz.proto.TableScanSummary.ParquetRowGroupStats value) {
if (parquetRowGroupStatsBuilder_ == null) {
if (parquetRowGroupStats_ != null) {
parquetRowGroupStats_ =
cz.proto.TableScanSummary.ParquetRowGroupStats.newBuilder(parquetRowGroupStats_).mergeFrom(value).buildPartial();
} else {
parquetRowGroupStats_ = value;
}
onChanged();
} else {
parquetRowGroupStatsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public Builder clearParquetRowGroupStats() {
if (parquetRowGroupStatsBuilder_ == null) {
parquetRowGroupStats_ = null;
onChanged();
} else {
parquetRowGroupStats_ = null;
parquetRowGroupStatsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public cz.proto.TableScanSummary.ParquetRowGroupStats.Builder getParquetRowGroupStatsBuilder() {
onChanged();
return getParquetRowGroupStatsFieldBuilder().getBuilder();
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
public cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder getParquetRowGroupStatsOrBuilder() {
if (parquetRowGroupStatsBuilder_ != null) {
return parquetRowGroupStatsBuilder_.getMessageOrBuilder();
} else {
return parquetRowGroupStats_ == null ?
cz.proto.TableScanSummary.ParquetRowGroupStats.getDefaultInstance() : parquetRowGroupStats_;
}
}
/**
* .cz.proto.TableScanSummary.ParquetRowGroupStats parquet_row_group_stats = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowGroupStats, cz.proto.TableScanSummary.ParquetRowGroupStats.Builder, cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder>
getParquetRowGroupStatsFieldBuilder() {
if (parquetRowGroupStatsBuilder_ == null) {
parquetRowGroupStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowGroupStats, cz.proto.TableScanSummary.ParquetRowGroupStats.Builder, cz.proto.TableScanSummary.ParquetRowGroupStatsOrBuilder>(
getParquetRowGroupStats(),
getParentForChildren(),
isClean());
parquetRowGroupStats_ = null;
}
return parquetRowGroupStatsBuilder_;
}
private cz.proto.TableScanSummary.ParquetRowCountStats parquetRowCountStats_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowCountStats, cz.proto.TableScanSummary.ParquetRowCountStats.Builder, cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder> parquetRowCountStatsBuilder_;
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
* @return Whether the parquetRowCountStats field is set.
*/
public boolean hasParquetRowCountStats() {
return parquetRowCountStatsBuilder_ != null || parquetRowCountStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
* @return The parquetRowCountStats.
*/
public cz.proto.TableScanSummary.ParquetRowCountStats getParquetRowCountStats() {
if (parquetRowCountStatsBuilder_ == null) {
return parquetRowCountStats_ == null ? cz.proto.TableScanSummary.ParquetRowCountStats.getDefaultInstance() : parquetRowCountStats_;
} else {
return parquetRowCountStatsBuilder_.getMessage();
}
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public Builder setParquetRowCountStats(cz.proto.TableScanSummary.ParquetRowCountStats value) {
if (parquetRowCountStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
parquetRowCountStats_ = value;
onChanged();
} else {
parquetRowCountStatsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public Builder setParquetRowCountStats(
cz.proto.TableScanSummary.ParquetRowCountStats.Builder builderForValue) {
if (parquetRowCountStatsBuilder_ == null) {
parquetRowCountStats_ = builderForValue.build();
onChanged();
} else {
parquetRowCountStatsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public Builder mergeParquetRowCountStats(cz.proto.TableScanSummary.ParquetRowCountStats value) {
if (parquetRowCountStatsBuilder_ == null) {
if (parquetRowCountStats_ != null) {
parquetRowCountStats_ =
cz.proto.TableScanSummary.ParquetRowCountStats.newBuilder(parquetRowCountStats_).mergeFrom(value).buildPartial();
} else {
parquetRowCountStats_ = value;
}
onChanged();
} else {
parquetRowCountStatsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public Builder clearParquetRowCountStats() {
if (parquetRowCountStatsBuilder_ == null) {
parquetRowCountStats_ = null;
onChanged();
} else {
parquetRowCountStats_ = null;
parquetRowCountStatsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public cz.proto.TableScanSummary.ParquetRowCountStats.Builder getParquetRowCountStatsBuilder() {
onChanged();
return getParquetRowCountStatsFieldBuilder().getBuilder();
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
public cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder getParquetRowCountStatsOrBuilder() {
if (parquetRowCountStatsBuilder_ != null) {
return parquetRowCountStatsBuilder_.getMessageOrBuilder();
} else {
return parquetRowCountStats_ == null ?
cz.proto.TableScanSummary.ParquetRowCountStats.getDefaultInstance() : parquetRowCountStats_;
}
}
/**
* .cz.proto.TableScanSummary.ParquetRowCountStats parquet_row_count_stats = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowCountStats, cz.proto.TableScanSummary.ParquetRowCountStats.Builder, cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder>
getParquetRowCountStatsFieldBuilder() {
if (parquetRowCountStatsBuilder_ == null) {
parquetRowCountStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetRowCountStats, cz.proto.TableScanSummary.ParquetRowCountStats.Builder, cz.proto.TableScanSummary.ParquetRowCountStatsOrBuilder>(
getParquetRowCountStats(),
getParentForChildren(),
isClean());
parquetRowCountStats_ = null;
}
return parquetRowCountStatsBuilder_;
}
private cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquetPrunedSplitsStats_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetPrunedSplitsStats, cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder, cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder> parquetPrunedSplitsStatsBuilder_;
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
* @return Whether the parquetPrunedSplitsStats field is set.
*/
public boolean hasParquetPrunedSplitsStats() {
return parquetPrunedSplitsStatsBuilder_ != null || parquetPrunedSplitsStats_ != null;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
* @return The parquetPrunedSplitsStats.
*/
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats getParquetPrunedSplitsStats() {
if (parquetPrunedSplitsStatsBuilder_ == null) {
return parquetPrunedSplitsStats_ == null ? cz.proto.TableScanSummary.ParquetPrunedSplitsStats.getDefaultInstance() : parquetPrunedSplitsStats_;
} else {
return parquetPrunedSplitsStatsBuilder_.getMessage();
}
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public Builder setParquetPrunedSplitsStats(cz.proto.TableScanSummary.ParquetPrunedSplitsStats value) {
if (parquetPrunedSplitsStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
parquetPrunedSplitsStats_ = value;
onChanged();
} else {
parquetPrunedSplitsStatsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public Builder setParquetPrunedSplitsStats(
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder builderForValue) {
if (parquetPrunedSplitsStatsBuilder_ == null) {
parquetPrunedSplitsStats_ = builderForValue.build();
onChanged();
} else {
parquetPrunedSplitsStatsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public Builder mergeParquetPrunedSplitsStats(cz.proto.TableScanSummary.ParquetPrunedSplitsStats value) {
if (parquetPrunedSplitsStatsBuilder_ == null) {
if (parquetPrunedSplitsStats_ != null) {
parquetPrunedSplitsStats_ =
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.newBuilder(parquetPrunedSplitsStats_).mergeFrom(value).buildPartial();
} else {
parquetPrunedSplitsStats_ = value;
}
onChanged();
} else {
parquetPrunedSplitsStatsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public Builder clearParquetPrunedSplitsStats() {
if (parquetPrunedSplitsStatsBuilder_ == null) {
parquetPrunedSplitsStats_ = null;
onChanged();
} else {
parquetPrunedSplitsStats_ = null;
parquetPrunedSplitsStatsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder getParquetPrunedSplitsStatsBuilder() {
onChanged();
return getParquetPrunedSplitsStatsFieldBuilder().getBuilder();
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
public cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder getParquetPrunedSplitsStatsOrBuilder() {
if (parquetPrunedSplitsStatsBuilder_ != null) {
return parquetPrunedSplitsStatsBuilder_.getMessageOrBuilder();
} else {
return parquetPrunedSplitsStats_ == null ?
cz.proto.TableScanSummary.ParquetPrunedSplitsStats.getDefaultInstance() : parquetPrunedSplitsStats_;
}
}
/**
* .cz.proto.TableScanSummary.ParquetPrunedSplitsStats parquet_pruned_splits_stats = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetPrunedSplitsStats, cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder, cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder>
getParquetPrunedSplitsStatsFieldBuilder() {
if (parquetPrunedSplitsStatsBuilder_ == null) {
parquetPrunedSplitsStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TableScanSummary.ParquetPrunedSplitsStats, cz.proto.TableScanSummary.ParquetPrunedSplitsStats.Builder, cz.proto.TableScanSummary.ParquetPrunedSplitsStatsOrBuilder>(
getParquetPrunedSplitsStats(),
getParentForChildren(),
isClean());
parquetPrunedSplitsStats_ = null;
}
return parquetPrunedSplitsStatsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.TableScanSummary)
}
// @@protoc_insertion_point(class_scope:cz.proto.TableScanSummary)
private static final cz.proto.TableScanSummary DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.TableScanSummary();
}
public static cz.proto.TableScanSummary getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableScanSummary parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableScanSummary(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.TableScanSummary getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy