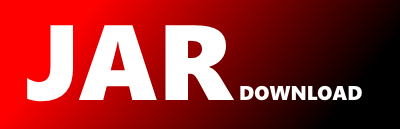
cz.proto.WorkerStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: job.proto
package cz.proto;
/**
* Protobuf type {@code cz.proto.WorkerStats}
*/
public final class WorkerStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.WorkerStats)
WorkerStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use WorkerStats.newBuilder() to construct.
private WorkerStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WorkerStats() {
operatorStats_ = java.util.Collections.emptyList();
driverStats_ = java.util.Collections.emptyList();
pipelineStats_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WorkerStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WorkerStats(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.Timing.Builder subBuilder = null;
if (timing_ != null) {
subBuilder = timing_.toBuilder();
}
timing_ = input.readMessage(cz.proto.Timing.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timing_);
timing_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
operatorStats_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
operatorStats_.add(
input.readMessage(cz.proto.OperatorStats.parser(), extensionRegistry));
break;
}
case 26: {
cz.proto.TimeRangeOuterClass.TimeRange.Builder subBuilder = null;
if (timeRangeMs_ != null) {
subBuilder = timeRangeMs_.toBuilder();
}
timeRangeMs_ = input.readMessage(cz.proto.TimeRangeOuterClass.TimeRange.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timeRangeMs_);
timeRangeMs_ = subBuilder.buildPartial();
}
break;
}
case 32: {
peakMemory_ = input.readUInt64();
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
driverStats_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
driverStats_.add(
input.readMessage(cz.proto.RuntimeDriverStats.parser(), extensionRegistry));
break;
}
case 48: {
runnerId_ = input.readUInt64();
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
pipelineStats_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
pipelineStats_.add(
input.readMessage(cz.proto.RuntimePipelineStats.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
operatorStats_ = java.util.Collections.unmodifiableList(operatorStats_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
driverStats_ = java.util.Collections.unmodifiableList(driverStats_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
pipelineStats_ = java.util.Collections.unmodifiableList(pipelineStats_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_WorkerStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_WorkerStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.WorkerStats.class, cz.proto.WorkerStats.Builder.class);
}
public static final int TIMING_FIELD_NUMBER = 1;
private cz.proto.Timing timing_;
/**
* .cz.proto.Timing timing = 1;
* @return Whether the timing field is set.
*/
@java.lang.Override
public boolean hasTiming() {
return timing_ != null;
}
/**
* .cz.proto.Timing timing = 1;
* @return The timing.
*/
@java.lang.Override
public cz.proto.Timing getTiming() {
return timing_ == null ? cz.proto.Timing.getDefaultInstance() : timing_;
}
/**
* .cz.proto.Timing timing = 1;
*/
@java.lang.Override
public cz.proto.TimingOrBuilder getTimingOrBuilder() {
return getTiming();
}
public static final int OPERATOR_STATS_FIELD_NUMBER = 2;
private java.util.List operatorStats_;
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
@java.lang.Override
public java.util.List getOperatorStatsList() {
return operatorStats_;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
@java.lang.Override
public java.util.List extends cz.proto.OperatorStatsOrBuilder>
getOperatorStatsOrBuilderList() {
return operatorStats_;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
@java.lang.Override
public int getOperatorStatsCount() {
return operatorStats_.size();
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
@java.lang.Override
public cz.proto.OperatorStats getOperatorStats(int index) {
return operatorStats_.get(index);
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
@java.lang.Override
public cz.proto.OperatorStatsOrBuilder getOperatorStatsOrBuilder(
int index) {
return operatorStats_.get(index);
}
public static final int TIME_RANGE_MS_FIELD_NUMBER = 3;
private cz.proto.TimeRangeOuterClass.TimeRange timeRangeMs_;
/**
* .cz.proto.TimeRange time_range_ms = 3;
* @return Whether the timeRangeMs field is set.
*/
@java.lang.Override
public boolean hasTimeRangeMs() {
return timeRangeMs_ != null;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
* @return The timeRangeMs.
*/
@java.lang.Override
public cz.proto.TimeRangeOuterClass.TimeRange getTimeRangeMs() {
return timeRangeMs_ == null ? cz.proto.TimeRangeOuterClass.TimeRange.getDefaultInstance() : timeRangeMs_;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
@java.lang.Override
public cz.proto.TimeRangeOuterClass.TimeRangeOrBuilder getTimeRangeMsOrBuilder() {
return getTimeRangeMs();
}
public static final int PEAK_MEMORY_FIELD_NUMBER = 4;
private long peakMemory_;
/**
* uint64 peak_memory = 4;
* @return The peakMemory.
*/
@java.lang.Override
public long getPeakMemory() {
return peakMemory_;
}
public static final int DRIVER_STATS_FIELD_NUMBER = 5;
private java.util.List driverStats_;
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
@java.lang.Override
public java.util.List getDriverStatsList() {
return driverStats_;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
@java.lang.Override
public java.util.List extends cz.proto.RuntimeDriverStatsOrBuilder>
getDriverStatsOrBuilderList() {
return driverStats_;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
@java.lang.Override
public int getDriverStatsCount() {
return driverStats_.size();
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
@java.lang.Override
public cz.proto.RuntimeDriverStats getDriverStats(int index) {
return driverStats_.get(index);
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
@java.lang.Override
public cz.proto.RuntimeDriverStatsOrBuilder getDriverStatsOrBuilder(
int index) {
return driverStats_.get(index);
}
public static final int RUNNER_ID_FIELD_NUMBER = 6;
private long runnerId_;
/**
* uint64 runner_id = 6;
* @return The runnerId.
*/
@java.lang.Override
public long getRunnerId() {
return runnerId_;
}
public static final int PIPELINE_STATS_FIELD_NUMBER = 7;
private java.util.List pipelineStats_;
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
@java.lang.Override
public java.util.List getPipelineStatsList() {
return pipelineStats_;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
@java.lang.Override
public java.util.List extends cz.proto.RuntimePipelineStatsOrBuilder>
getPipelineStatsOrBuilderList() {
return pipelineStats_;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
@java.lang.Override
public int getPipelineStatsCount() {
return pipelineStats_.size();
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
@java.lang.Override
public cz.proto.RuntimePipelineStats getPipelineStats(int index) {
return pipelineStats_.get(index);
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
@java.lang.Override
public cz.proto.RuntimePipelineStatsOrBuilder getPipelineStatsOrBuilder(
int index) {
return pipelineStats_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (timing_ != null) {
output.writeMessage(1, getTiming());
}
for (int i = 0; i < operatorStats_.size(); i++) {
output.writeMessage(2, operatorStats_.get(i));
}
if (timeRangeMs_ != null) {
output.writeMessage(3, getTimeRangeMs());
}
if (peakMemory_ != 0L) {
output.writeUInt64(4, peakMemory_);
}
for (int i = 0; i < driverStats_.size(); i++) {
output.writeMessage(5, driverStats_.get(i));
}
if (runnerId_ != 0L) {
output.writeUInt64(6, runnerId_);
}
for (int i = 0; i < pipelineStats_.size(); i++) {
output.writeMessage(7, pipelineStats_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (timing_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTiming());
}
for (int i = 0; i < operatorStats_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, operatorStats_.get(i));
}
if (timeRangeMs_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTimeRangeMs());
}
if (peakMemory_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, peakMemory_);
}
for (int i = 0; i < driverStats_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, driverStats_.get(i));
}
if (runnerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, runnerId_);
}
for (int i = 0; i < pipelineStats_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, pipelineStats_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.WorkerStats)) {
return super.equals(obj);
}
cz.proto.WorkerStats other = (cz.proto.WorkerStats) obj;
if (hasTiming() != other.hasTiming()) return false;
if (hasTiming()) {
if (!getTiming()
.equals(other.getTiming())) return false;
}
if (!getOperatorStatsList()
.equals(other.getOperatorStatsList())) return false;
if (hasTimeRangeMs() != other.hasTimeRangeMs()) return false;
if (hasTimeRangeMs()) {
if (!getTimeRangeMs()
.equals(other.getTimeRangeMs())) return false;
}
if (getPeakMemory()
!= other.getPeakMemory()) return false;
if (!getDriverStatsList()
.equals(other.getDriverStatsList())) return false;
if (getRunnerId()
!= other.getRunnerId()) return false;
if (!getPipelineStatsList()
.equals(other.getPipelineStatsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTiming()) {
hash = (37 * hash) + TIMING_FIELD_NUMBER;
hash = (53 * hash) + getTiming().hashCode();
}
if (getOperatorStatsCount() > 0) {
hash = (37 * hash) + OPERATOR_STATS_FIELD_NUMBER;
hash = (53 * hash) + getOperatorStatsList().hashCode();
}
if (hasTimeRangeMs()) {
hash = (37 * hash) + TIME_RANGE_MS_FIELD_NUMBER;
hash = (53 * hash) + getTimeRangeMs().hashCode();
}
hash = (37 * hash) + PEAK_MEMORY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPeakMemory());
if (getDriverStatsCount() > 0) {
hash = (37 * hash) + DRIVER_STATS_FIELD_NUMBER;
hash = (53 * hash) + getDriverStatsList().hashCode();
}
hash = (37 * hash) + RUNNER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRunnerId());
if (getPipelineStatsCount() > 0) {
hash = (37 * hash) + PIPELINE_STATS_FIELD_NUMBER;
hash = (53 * hash) + getPipelineStatsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.WorkerStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.WorkerStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.WorkerStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.WorkerStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.WorkerStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.WorkerStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.WorkerStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.WorkerStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.WorkerStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.WorkerStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.WorkerStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.WorkerStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.WorkerStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.WorkerStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.WorkerStats)
cz.proto.WorkerStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.JobProto.internal_static_cz_proto_WorkerStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.JobProto.internal_static_cz_proto_WorkerStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.WorkerStats.class, cz.proto.WorkerStats.Builder.class);
}
// Construct using cz.proto.WorkerStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOperatorStatsFieldBuilder();
getDriverStatsFieldBuilder();
getPipelineStatsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (timingBuilder_ == null) {
timing_ = null;
} else {
timing_ = null;
timingBuilder_ = null;
}
if (operatorStatsBuilder_ == null) {
operatorStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
operatorStatsBuilder_.clear();
}
if (timeRangeMsBuilder_ == null) {
timeRangeMs_ = null;
} else {
timeRangeMs_ = null;
timeRangeMsBuilder_ = null;
}
peakMemory_ = 0L;
if (driverStatsBuilder_ == null) {
driverStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
driverStatsBuilder_.clear();
}
runnerId_ = 0L;
if (pipelineStatsBuilder_ == null) {
pipelineStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
pipelineStatsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.JobProto.internal_static_cz_proto_WorkerStats_descriptor;
}
@java.lang.Override
public cz.proto.WorkerStats getDefaultInstanceForType() {
return cz.proto.WorkerStats.getDefaultInstance();
}
@java.lang.Override
public cz.proto.WorkerStats build() {
cz.proto.WorkerStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.WorkerStats buildPartial() {
cz.proto.WorkerStats result = new cz.proto.WorkerStats(this);
int from_bitField0_ = bitField0_;
if (timingBuilder_ == null) {
result.timing_ = timing_;
} else {
result.timing_ = timingBuilder_.build();
}
if (operatorStatsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
operatorStats_ = java.util.Collections.unmodifiableList(operatorStats_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.operatorStats_ = operatorStats_;
} else {
result.operatorStats_ = operatorStatsBuilder_.build();
}
if (timeRangeMsBuilder_ == null) {
result.timeRangeMs_ = timeRangeMs_;
} else {
result.timeRangeMs_ = timeRangeMsBuilder_.build();
}
result.peakMemory_ = peakMemory_;
if (driverStatsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
driverStats_ = java.util.Collections.unmodifiableList(driverStats_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.driverStats_ = driverStats_;
} else {
result.driverStats_ = driverStatsBuilder_.build();
}
result.runnerId_ = runnerId_;
if (pipelineStatsBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
pipelineStats_ = java.util.Collections.unmodifiableList(pipelineStats_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.pipelineStats_ = pipelineStats_;
} else {
result.pipelineStats_ = pipelineStatsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.WorkerStats) {
return mergeFrom((cz.proto.WorkerStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.WorkerStats other) {
if (other == cz.proto.WorkerStats.getDefaultInstance()) return this;
if (other.hasTiming()) {
mergeTiming(other.getTiming());
}
if (operatorStatsBuilder_ == null) {
if (!other.operatorStats_.isEmpty()) {
if (operatorStats_.isEmpty()) {
operatorStats_ = other.operatorStats_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureOperatorStatsIsMutable();
operatorStats_.addAll(other.operatorStats_);
}
onChanged();
}
} else {
if (!other.operatorStats_.isEmpty()) {
if (operatorStatsBuilder_.isEmpty()) {
operatorStatsBuilder_.dispose();
operatorStatsBuilder_ = null;
operatorStats_ = other.operatorStats_;
bitField0_ = (bitField0_ & ~0x00000001);
operatorStatsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOperatorStatsFieldBuilder() : null;
} else {
operatorStatsBuilder_.addAllMessages(other.operatorStats_);
}
}
}
if (other.hasTimeRangeMs()) {
mergeTimeRangeMs(other.getTimeRangeMs());
}
if (other.getPeakMemory() != 0L) {
setPeakMemory(other.getPeakMemory());
}
if (driverStatsBuilder_ == null) {
if (!other.driverStats_.isEmpty()) {
if (driverStats_.isEmpty()) {
driverStats_ = other.driverStats_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureDriverStatsIsMutable();
driverStats_.addAll(other.driverStats_);
}
onChanged();
}
} else {
if (!other.driverStats_.isEmpty()) {
if (driverStatsBuilder_.isEmpty()) {
driverStatsBuilder_.dispose();
driverStatsBuilder_ = null;
driverStats_ = other.driverStats_;
bitField0_ = (bitField0_ & ~0x00000002);
driverStatsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDriverStatsFieldBuilder() : null;
} else {
driverStatsBuilder_.addAllMessages(other.driverStats_);
}
}
}
if (other.getRunnerId() != 0L) {
setRunnerId(other.getRunnerId());
}
if (pipelineStatsBuilder_ == null) {
if (!other.pipelineStats_.isEmpty()) {
if (pipelineStats_.isEmpty()) {
pipelineStats_ = other.pipelineStats_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePipelineStatsIsMutable();
pipelineStats_.addAll(other.pipelineStats_);
}
onChanged();
}
} else {
if (!other.pipelineStats_.isEmpty()) {
if (pipelineStatsBuilder_.isEmpty()) {
pipelineStatsBuilder_.dispose();
pipelineStatsBuilder_ = null;
pipelineStats_ = other.pipelineStats_;
bitField0_ = (bitField0_ & ~0x00000004);
pipelineStatsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPipelineStatsFieldBuilder() : null;
} else {
pipelineStatsBuilder_.addAllMessages(other.pipelineStats_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.WorkerStats parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.WorkerStats) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.Timing timing_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder> timingBuilder_;
/**
* .cz.proto.Timing timing = 1;
* @return Whether the timing field is set.
*/
public boolean hasTiming() {
return timingBuilder_ != null || timing_ != null;
}
/**
* .cz.proto.Timing timing = 1;
* @return The timing.
*/
public cz.proto.Timing getTiming() {
if (timingBuilder_ == null) {
return timing_ == null ? cz.proto.Timing.getDefaultInstance() : timing_;
} else {
return timingBuilder_.getMessage();
}
}
/**
* .cz.proto.Timing timing = 1;
*/
public Builder setTiming(cz.proto.Timing value) {
if (timingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timing_ = value;
onChanged();
} else {
timingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Timing timing = 1;
*/
public Builder setTiming(
cz.proto.Timing.Builder builderForValue) {
if (timingBuilder_ == null) {
timing_ = builderForValue.build();
onChanged();
} else {
timingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Timing timing = 1;
*/
public Builder mergeTiming(cz.proto.Timing value) {
if (timingBuilder_ == null) {
if (timing_ != null) {
timing_ =
cz.proto.Timing.newBuilder(timing_).mergeFrom(value).buildPartial();
} else {
timing_ = value;
}
onChanged();
} else {
timingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Timing timing = 1;
*/
public Builder clearTiming() {
if (timingBuilder_ == null) {
timing_ = null;
onChanged();
} else {
timing_ = null;
timingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Timing timing = 1;
*/
public cz.proto.Timing.Builder getTimingBuilder() {
onChanged();
return getTimingFieldBuilder().getBuilder();
}
/**
* .cz.proto.Timing timing = 1;
*/
public cz.proto.TimingOrBuilder getTimingOrBuilder() {
if (timingBuilder_ != null) {
return timingBuilder_.getMessageOrBuilder();
} else {
return timing_ == null ?
cz.proto.Timing.getDefaultInstance() : timing_;
}
}
/**
* .cz.proto.Timing timing = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>
getTimingFieldBuilder() {
if (timingBuilder_ == null) {
timingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.Timing, cz.proto.Timing.Builder, cz.proto.TimingOrBuilder>(
getTiming(),
getParentForChildren(),
isClean());
timing_ = null;
}
return timingBuilder_;
}
private java.util.List operatorStats_ =
java.util.Collections.emptyList();
private void ensureOperatorStatsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
operatorStats_ = new java.util.ArrayList(operatorStats_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.OperatorStats, cz.proto.OperatorStats.Builder, cz.proto.OperatorStatsOrBuilder> operatorStatsBuilder_;
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public java.util.List getOperatorStatsList() {
if (operatorStatsBuilder_ == null) {
return java.util.Collections.unmodifiableList(operatorStats_);
} else {
return operatorStatsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public int getOperatorStatsCount() {
if (operatorStatsBuilder_ == null) {
return operatorStats_.size();
} else {
return operatorStatsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public cz.proto.OperatorStats getOperatorStats(int index) {
if (operatorStatsBuilder_ == null) {
return operatorStats_.get(index);
} else {
return operatorStatsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder setOperatorStats(
int index, cz.proto.OperatorStats value) {
if (operatorStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOperatorStatsIsMutable();
operatorStats_.set(index, value);
onChanged();
} else {
operatorStatsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder setOperatorStats(
int index, cz.proto.OperatorStats.Builder builderForValue) {
if (operatorStatsBuilder_ == null) {
ensureOperatorStatsIsMutable();
operatorStats_.set(index, builderForValue.build());
onChanged();
} else {
operatorStatsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder addOperatorStats(cz.proto.OperatorStats value) {
if (operatorStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOperatorStatsIsMutable();
operatorStats_.add(value);
onChanged();
} else {
operatorStatsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder addOperatorStats(
int index, cz.proto.OperatorStats value) {
if (operatorStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOperatorStatsIsMutable();
operatorStats_.add(index, value);
onChanged();
} else {
operatorStatsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder addOperatorStats(
cz.proto.OperatorStats.Builder builderForValue) {
if (operatorStatsBuilder_ == null) {
ensureOperatorStatsIsMutable();
operatorStats_.add(builderForValue.build());
onChanged();
} else {
operatorStatsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder addOperatorStats(
int index, cz.proto.OperatorStats.Builder builderForValue) {
if (operatorStatsBuilder_ == null) {
ensureOperatorStatsIsMutable();
operatorStats_.add(index, builderForValue.build());
onChanged();
} else {
operatorStatsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder addAllOperatorStats(
java.lang.Iterable extends cz.proto.OperatorStats> values) {
if (operatorStatsBuilder_ == null) {
ensureOperatorStatsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, operatorStats_);
onChanged();
} else {
operatorStatsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder clearOperatorStats() {
if (operatorStatsBuilder_ == null) {
operatorStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
operatorStatsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public Builder removeOperatorStats(int index) {
if (operatorStatsBuilder_ == null) {
ensureOperatorStatsIsMutable();
operatorStats_.remove(index);
onChanged();
} else {
operatorStatsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public cz.proto.OperatorStats.Builder getOperatorStatsBuilder(
int index) {
return getOperatorStatsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public cz.proto.OperatorStatsOrBuilder getOperatorStatsOrBuilder(
int index) {
if (operatorStatsBuilder_ == null) {
return operatorStats_.get(index); } else {
return operatorStatsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public java.util.List extends cz.proto.OperatorStatsOrBuilder>
getOperatorStatsOrBuilderList() {
if (operatorStatsBuilder_ != null) {
return operatorStatsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(operatorStats_);
}
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public cz.proto.OperatorStats.Builder addOperatorStatsBuilder() {
return getOperatorStatsFieldBuilder().addBuilder(
cz.proto.OperatorStats.getDefaultInstance());
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public cz.proto.OperatorStats.Builder addOperatorStatsBuilder(
int index) {
return getOperatorStatsFieldBuilder().addBuilder(
index, cz.proto.OperatorStats.getDefaultInstance());
}
/**
* repeated .cz.proto.OperatorStats operator_stats = 2;
*/
public java.util.List
getOperatorStatsBuilderList() {
return getOperatorStatsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.OperatorStats, cz.proto.OperatorStats.Builder, cz.proto.OperatorStatsOrBuilder>
getOperatorStatsFieldBuilder() {
if (operatorStatsBuilder_ == null) {
operatorStatsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.OperatorStats, cz.proto.OperatorStats.Builder, cz.proto.OperatorStatsOrBuilder>(
operatorStats_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
operatorStats_ = null;
}
return operatorStatsBuilder_;
}
private cz.proto.TimeRangeOuterClass.TimeRange timeRangeMs_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TimeRangeOuterClass.TimeRange, cz.proto.TimeRangeOuterClass.TimeRange.Builder, cz.proto.TimeRangeOuterClass.TimeRangeOrBuilder> timeRangeMsBuilder_;
/**
* .cz.proto.TimeRange time_range_ms = 3;
* @return Whether the timeRangeMs field is set.
*/
public boolean hasTimeRangeMs() {
return timeRangeMsBuilder_ != null || timeRangeMs_ != null;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
* @return The timeRangeMs.
*/
public cz.proto.TimeRangeOuterClass.TimeRange getTimeRangeMs() {
if (timeRangeMsBuilder_ == null) {
return timeRangeMs_ == null ? cz.proto.TimeRangeOuterClass.TimeRange.getDefaultInstance() : timeRangeMs_;
} else {
return timeRangeMsBuilder_.getMessage();
}
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public Builder setTimeRangeMs(cz.proto.TimeRangeOuterClass.TimeRange value) {
if (timeRangeMsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timeRangeMs_ = value;
onChanged();
} else {
timeRangeMsBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public Builder setTimeRangeMs(
cz.proto.TimeRangeOuterClass.TimeRange.Builder builderForValue) {
if (timeRangeMsBuilder_ == null) {
timeRangeMs_ = builderForValue.build();
onChanged();
} else {
timeRangeMsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public Builder mergeTimeRangeMs(cz.proto.TimeRangeOuterClass.TimeRange value) {
if (timeRangeMsBuilder_ == null) {
if (timeRangeMs_ != null) {
timeRangeMs_ =
cz.proto.TimeRangeOuterClass.TimeRange.newBuilder(timeRangeMs_).mergeFrom(value).buildPartial();
} else {
timeRangeMs_ = value;
}
onChanged();
} else {
timeRangeMsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public Builder clearTimeRangeMs() {
if (timeRangeMsBuilder_ == null) {
timeRangeMs_ = null;
onChanged();
} else {
timeRangeMs_ = null;
timeRangeMsBuilder_ = null;
}
return this;
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public cz.proto.TimeRangeOuterClass.TimeRange.Builder getTimeRangeMsBuilder() {
onChanged();
return getTimeRangeMsFieldBuilder().getBuilder();
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
public cz.proto.TimeRangeOuterClass.TimeRangeOrBuilder getTimeRangeMsOrBuilder() {
if (timeRangeMsBuilder_ != null) {
return timeRangeMsBuilder_.getMessageOrBuilder();
} else {
return timeRangeMs_ == null ?
cz.proto.TimeRangeOuterClass.TimeRange.getDefaultInstance() : timeRangeMs_;
}
}
/**
* .cz.proto.TimeRange time_range_ms = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TimeRangeOuterClass.TimeRange, cz.proto.TimeRangeOuterClass.TimeRange.Builder, cz.proto.TimeRangeOuterClass.TimeRangeOrBuilder>
getTimeRangeMsFieldBuilder() {
if (timeRangeMsBuilder_ == null) {
timeRangeMsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.TimeRangeOuterClass.TimeRange, cz.proto.TimeRangeOuterClass.TimeRange.Builder, cz.proto.TimeRangeOuterClass.TimeRangeOrBuilder>(
getTimeRangeMs(),
getParentForChildren(),
isClean());
timeRangeMs_ = null;
}
return timeRangeMsBuilder_;
}
private long peakMemory_ ;
/**
* uint64 peak_memory = 4;
* @return The peakMemory.
*/
@java.lang.Override
public long getPeakMemory() {
return peakMemory_;
}
/**
* uint64 peak_memory = 4;
* @param value The peakMemory to set.
* @return This builder for chaining.
*/
public Builder setPeakMemory(long value) {
peakMemory_ = value;
onChanged();
return this;
}
/**
* uint64 peak_memory = 4;
* @return This builder for chaining.
*/
public Builder clearPeakMemory() {
peakMemory_ = 0L;
onChanged();
return this;
}
private java.util.List driverStats_ =
java.util.Collections.emptyList();
private void ensureDriverStatsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
driverStats_ = new java.util.ArrayList(driverStats_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimeDriverStats, cz.proto.RuntimeDriverStats.Builder, cz.proto.RuntimeDriverStatsOrBuilder> driverStatsBuilder_;
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public java.util.List getDriverStatsList() {
if (driverStatsBuilder_ == null) {
return java.util.Collections.unmodifiableList(driverStats_);
} else {
return driverStatsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public int getDriverStatsCount() {
if (driverStatsBuilder_ == null) {
return driverStats_.size();
} else {
return driverStatsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public cz.proto.RuntimeDriverStats getDriverStats(int index) {
if (driverStatsBuilder_ == null) {
return driverStats_.get(index);
} else {
return driverStatsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder setDriverStats(
int index, cz.proto.RuntimeDriverStats value) {
if (driverStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDriverStatsIsMutable();
driverStats_.set(index, value);
onChanged();
} else {
driverStatsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder setDriverStats(
int index, cz.proto.RuntimeDriverStats.Builder builderForValue) {
if (driverStatsBuilder_ == null) {
ensureDriverStatsIsMutable();
driverStats_.set(index, builderForValue.build());
onChanged();
} else {
driverStatsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder addDriverStats(cz.proto.RuntimeDriverStats value) {
if (driverStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDriverStatsIsMutable();
driverStats_.add(value);
onChanged();
} else {
driverStatsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder addDriverStats(
int index, cz.proto.RuntimeDriverStats value) {
if (driverStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDriverStatsIsMutable();
driverStats_.add(index, value);
onChanged();
} else {
driverStatsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder addDriverStats(
cz.proto.RuntimeDriverStats.Builder builderForValue) {
if (driverStatsBuilder_ == null) {
ensureDriverStatsIsMutable();
driverStats_.add(builderForValue.build());
onChanged();
} else {
driverStatsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder addDriverStats(
int index, cz.proto.RuntimeDriverStats.Builder builderForValue) {
if (driverStatsBuilder_ == null) {
ensureDriverStatsIsMutable();
driverStats_.add(index, builderForValue.build());
onChanged();
} else {
driverStatsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder addAllDriverStats(
java.lang.Iterable extends cz.proto.RuntimeDriverStats> values) {
if (driverStatsBuilder_ == null) {
ensureDriverStatsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, driverStats_);
onChanged();
} else {
driverStatsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder clearDriverStats() {
if (driverStatsBuilder_ == null) {
driverStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
driverStatsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public Builder removeDriverStats(int index) {
if (driverStatsBuilder_ == null) {
ensureDriverStatsIsMutable();
driverStats_.remove(index);
onChanged();
} else {
driverStatsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public cz.proto.RuntimeDriverStats.Builder getDriverStatsBuilder(
int index) {
return getDriverStatsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public cz.proto.RuntimeDriverStatsOrBuilder getDriverStatsOrBuilder(
int index) {
if (driverStatsBuilder_ == null) {
return driverStats_.get(index); } else {
return driverStatsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public java.util.List extends cz.proto.RuntimeDriverStatsOrBuilder>
getDriverStatsOrBuilderList() {
if (driverStatsBuilder_ != null) {
return driverStatsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(driverStats_);
}
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public cz.proto.RuntimeDriverStats.Builder addDriverStatsBuilder() {
return getDriverStatsFieldBuilder().addBuilder(
cz.proto.RuntimeDriverStats.getDefaultInstance());
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public cz.proto.RuntimeDriverStats.Builder addDriverStatsBuilder(
int index) {
return getDriverStatsFieldBuilder().addBuilder(
index, cz.proto.RuntimeDriverStats.getDefaultInstance());
}
/**
* repeated .cz.proto.RuntimeDriverStats driver_stats = 5;
*/
public java.util.List
getDriverStatsBuilderList() {
return getDriverStatsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimeDriverStats, cz.proto.RuntimeDriverStats.Builder, cz.proto.RuntimeDriverStatsOrBuilder>
getDriverStatsFieldBuilder() {
if (driverStatsBuilder_ == null) {
driverStatsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimeDriverStats, cz.proto.RuntimeDriverStats.Builder, cz.proto.RuntimeDriverStatsOrBuilder>(
driverStats_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
driverStats_ = null;
}
return driverStatsBuilder_;
}
private long runnerId_ ;
/**
* uint64 runner_id = 6;
* @return The runnerId.
*/
@java.lang.Override
public long getRunnerId() {
return runnerId_;
}
/**
* uint64 runner_id = 6;
* @param value The runnerId to set.
* @return This builder for chaining.
*/
public Builder setRunnerId(long value) {
runnerId_ = value;
onChanged();
return this;
}
/**
* uint64 runner_id = 6;
* @return This builder for chaining.
*/
public Builder clearRunnerId() {
runnerId_ = 0L;
onChanged();
return this;
}
private java.util.List pipelineStats_ =
java.util.Collections.emptyList();
private void ensurePipelineStatsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
pipelineStats_ = new java.util.ArrayList(pipelineStats_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimePipelineStats, cz.proto.RuntimePipelineStats.Builder, cz.proto.RuntimePipelineStatsOrBuilder> pipelineStatsBuilder_;
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public java.util.List getPipelineStatsList() {
if (pipelineStatsBuilder_ == null) {
return java.util.Collections.unmodifiableList(pipelineStats_);
} else {
return pipelineStatsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public int getPipelineStatsCount() {
if (pipelineStatsBuilder_ == null) {
return pipelineStats_.size();
} else {
return pipelineStatsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public cz.proto.RuntimePipelineStats getPipelineStats(int index) {
if (pipelineStatsBuilder_ == null) {
return pipelineStats_.get(index);
} else {
return pipelineStatsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder setPipelineStats(
int index, cz.proto.RuntimePipelineStats value) {
if (pipelineStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePipelineStatsIsMutable();
pipelineStats_.set(index, value);
onChanged();
} else {
pipelineStatsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder setPipelineStats(
int index, cz.proto.RuntimePipelineStats.Builder builderForValue) {
if (pipelineStatsBuilder_ == null) {
ensurePipelineStatsIsMutable();
pipelineStats_.set(index, builderForValue.build());
onChanged();
} else {
pipelineStatsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder addPipelineStats(cz.proto.RuntimePipelineStats value) {
if (pipelineStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePipelineStatsIsMutable();
pipelineStats_.add(value);
onChanged();
} else {
pipelineStatsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder addPipelineStats(
int index, cz.proto.RuntimePipelineStats value) {
if (pipelineStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePipelineStatsIsMutable();
pipelineStats_.add(index, value);
onChanged();
} else {
pipelineStatsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder addPipelineStats(
cz.proto.RuntimePipelineStats.Builder builderForValue) {
if (pipelineStatsBuilder_ == null) {
ensurePipelineStatsIsMutable();
pipelineStats_.add(builderForValue.build());
onChanged();
} else {
pipelineStatsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder addPipelineStats(
int index, cz.proto.RuntimePipelineStats.Builder builderForValue) {
if (pipelineStatsBuilder_ == null) {
ensurePipelineStatsIsMutable();
pipelineStats_.add(index, builderForValue.build());
onChanged();
} else {
pipelineStatsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder addAllPipelineStats(
java.lang.Iterable extends cz.proto.RuntimePipelineStats> values) {
if (pipelineStatsBuilder_ == null) {
ensurePipelineStatsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pipelineStats_);
onChanged();
} else {
pipelineStatsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder clearPipelineStats() {
if (pipelineStatsBuilder_ == null) {
pipelineStats_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
pipelineStatsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public Builder removePipelineStats(int index) {
if (pipelineStatsBuilder_ == null) {
ensurePipelineStatsIsMutable();
pipelineStats_.remove(index);
onChanged();
} else {
pipelineStatsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public cz.proto.RuntimePipelineStats.Builder getPipelineStatsBuilder(
int index) {
return getPipelineStatsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public cz.proto.RuntimePipelineStatsOrBuilder getPipelineStatsOrBuilder(
int index) {
if (pipelineStatsBuilder_ == null) {
return pipelineStats_.get(index); } else {
return pipelineStatsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public java.util.List extends cz.proto.RuntimePipelineStatsOrBuilder>
getPipelineStatsOrBuilderList() {
if (pipelineStatsBuilder_ != null) {
return pipelineStatsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(pipelineStats_);
}
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public cz.proto.RuntimePipelineStats.Builder addPipelineStatsBuilder() {
return getPipelineStatsFieldBuilder().addBuilder(
cz.proto.RuntimePipelineStats.getDefaultInstance());
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public cz.proto.RuntimePipelineStats.Builder addPipelineStatsBuilder(
int index) {
return getPipelineStatsFieldBuilder().addBuilder(
index, cz.proto.RuntimePipelineStats.getDefaultInstance());
}
/**
* repeated .cz.proto.RuntimePipelineStats pipeline_stats = 7;
*/
public java.util.List
getPipelineStatsBuilderList() {
return getPipelineStatsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimePipelineStats, cz.proto.RuntimePipelineStats.Builder, cz.proto.RuntimePipelineStatsOrBuilder>
getPipelineStatsFieldBuilder() {
if (pipelineStatsBuilder_ == null) {
pipelineStatsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.RuntimePipelineStats, cz.proto.RuntimePipelineStats.Builder, cz.proto.RuntimePipelineStatsOrBuilder>(
pipelineStats_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
pipelineStats_ = null;
}
return pipelineStatsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.WorkerStats)
}
// @@protoc_insertion_point(class_scope:cz.proto.WorkerStats)
private static final cz.proto.WorkerStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.WorkerStats();
}
public static cz.proto.WorkerStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WorkerStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WorkerStats(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.WorkerStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy