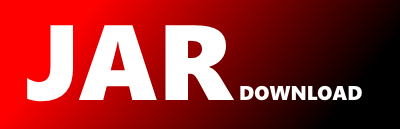
cz.proto.access.CheckPrivileges Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: privilege.proto
package cz.proto.access;
/**
* Protobuf type {@code cz.proto.access.CheckPrivileges}
*/
public final class CheckPrivileges extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.access.CheckPrivileges)
CheckPrivilegesOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckPrivileges.newBuilder() to construct.
private CheckPrivileges(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckPrivileges() {
accessToken_ = "";
content_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckPrivileges();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckPrivileges(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.AccountOuterClass.UserIdentifier.Builder subBuilder = null;
if (principal_ != null) {
subBuilder = principal_.toBuilder();
}
principal_ = input.readMessage(cz.proto.AccountOuterClass.UserIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(principal_);
principal_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
accessToken_ = s;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
content_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
content_.add(
input.readMessage(cz.proto.access.CheckPrivileges.Content.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
content_ = java.util.Collections.unmodifiableList(content_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.access.CheckPrivileges.class, cz.proto.access.CheckPrivileges.Builder.class);
}
public interface ContentOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.access.CheckPrivileges.Content)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
int getModeValue();
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The mode.
*/
cz.proto.access.EffectMode getMode();
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the action.
*/
java.util.List getActionList();
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return The count of action.
*/
int getActionCount();
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the element to return.
* @return The action at the given index.
*/
cz.proto.access.ActionType getAction(int index);
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the enum numeric values on the wire for action.
*/
java.util.List
getActionValueList();
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of action at the given index.
*/
int getActionValue(int index);
/**
*
* true means that we check grant_action privileges for grant/revoke query
*
*
* bool with_grant_option = 3;
* @return The withGrantOption.
*/
boolean getWithGrantOption();
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return Whether the object field is set.
*/
boolean hasObject();
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return The object.
*/
cz.proto.ObjectIdentifier getObject();
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
cz.proto.ObjectIdentifierOrBuilder getObjectOrBuilder();
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The enum numeric value on the wire for grantedType.
*/
int getGrantedTypeValue();
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The grantedType.
*/
cz.proto.access.GrantedType.Type getGrantedType();
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The enum numeric value on the wire for subObjectType.
*/
int getSubObjectTypeValue();
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The subObjectType.
*/
cz.proto.ObjectType getSubObjectType();
}
/**
* Protobuf type {@code cz.proto.access.CheckPrivileges.Content}
*/
public static final class Content extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.access.CheckPrivileges.Content)
ContentOrBuilder {
private static final long serialVersionUID = 0L;
// Use Content.newBuilder() to construct.
private Content(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Content() {
mode_ = 0;
action_ = java.util.Collections.emptyList();
grantedType_ = 0;
subObjectType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Content();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Content(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
mode_ = rawValue;
break;
}
case 16: {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
action_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
action_.add(rawValue);
break;
}
case 18: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int rawValue = input.readEnum();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
action_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
action_.add(rawValue);
}
input.popLimit(oldLimit);
break;
}
case 24: {
withGrantOption_ = input.readBool();
break;
}
case 34: {
cz.proto.ObjectIdentifier.Builder subBuilder = null;
if (object_ != null) {
subBuilder = object_.toBuilder();
}
object_ = input.readMessage(cz.proto.ObjectIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(object_);
object_ = subBuilder.buildPartial();
}
break;
}
case 40: {
int rawValue = input.readEnum();
grantedType_ = rawValue;
break;
}
case 48: {
int rawValue = input.readEnum();
subObjectType_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
action_ = java.util.Collections.unmodifiableList(action_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_Content_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_Content_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.access.CheckPrivileges.Content.class, cz.proto.access.CheckPrivileges.Content.Builder.class);
}
public static final int MODE_FIELD_NUMBER = 1;
private int mode_;
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The mode.
*/
@java.lang.Override public cz.proto.access.EffectMode getMode() {
@SuppressWarnings("deprecation")
cz.proto.access.EffectMode result = cz.proto.access.EffectMode.valueOf(mode_);
return result == null ? cz.proto.access.EffectMode.UNRECOGNIZED : result;
}
public static final int ACTION_FIELD_NUMBER = 2;
private java.util.List action_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, cz.proto.access.ActionType> action_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, cz.proto.access.ActionType>() {
public cz.proto.access.ActionType convert(java.lang.Integer from) {
@SuppressWarnings("deprecation")
cz.proto.access.ActionType result = cz.proto.access.ActionType.valueOf(from);
return result == null ? cz.proto.access.ActionType.UNRECOGNIZED : result;
}
};
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the action.
*/
@java.lang.Override
public java.util.List getActionList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, cz.proto.access.ActionType>(action_, action_converter_);
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return The count of action.
*/
@java.lang.Override
public int getActionCount() {
return action_.size();
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the element to return.
* @return The action at the given index.
*/
@java.lang.Override
public cz.proto.access.ActionType getAction(int index) {
return action_converter_.convert(action_.get(index));
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the enum numeric values on the wire for action.
*/
@java.lang.Override
public java.util.List
getActionValueList() {
return action_;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of action at the given index.
*/
@java.lang.Override
public int getActionValue(int index) {
return action_.get(index);
}
private int actionMemoizedSerializedSize;
public static final int WITH_GRANT_OPTION_FIELD_NUMBER = 3;
private boolean withGrantOption_;
/**
*
* true means that we check grant_action privileges for grant/revoke query
*
*
* bool with_grant_option = 3;
* @return The withGrantOption.
*/
@java.lang.Override
public boolean getWithGrantOption() {
return withGrantOption_;
}
public static final int OBJECT_FIELD_NUMBER = 4;
private cz.proto.ObjectIdentifier object_;
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return Whether the object field is set.
*/
@java.lang.Override
public boolean hasObject() {
return object_ != null;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return The object.
*/
@java.lang.Override
public cz.proto.ObjectIdentifier getObject() {
return object_ == null ? cz.proto.ObjectIdentifier.getDefaultInstance() : object_;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
@java.lang.Override
public cz.proto.ObjectIdentifierOrBuilder getObjectOrBuilder() {
return getObject();
}
public static final int GRANTED_TYPE_FIELD_NUMBER = 5;
private int grantedType_;
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The enum numeric value on the wire for grantedType.
*/
@java.lang.Override public int getGrantedTypeValue() {
return grantedType_;
}
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The grantedType.
*/
@java.lang.Override public cz.proto.access.GrantedType.Type getGrantedType() {
@SuppressWarnings("deprecation")
cz.proto.access.GrantedType.Type result = cz.proto.access.GrantedType.Type.valueOf(grantedType_);
return result == null ? cz.proto.access.GrantedType.Type.UNRECOGNIZED : result;
}
public static final int SUB_OBJECT_TYPE_FIELD_NUMBER = 6;
private int subObjectType_;
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The enum numeric value on the wire for subObjectType.
*/
@java.lang.Override public int getSubObjectTypeValue() {
return subObjectType_;
}
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The subObjectType.
*/
@java.lang.Override public cz.proto.ObjectType getSubObjectType() {
@SuppressWarnings("deprecation")
cz.proto.ObjectType result = cz.proto.ObjectType.valueOf(subObjectType_);
return result == null ? cz.proto.ObjectType.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (mode_ != cz.proto.access.EffectMode.DENY_OVERRIDE.getNumber()) {
output.writeEnum(1, mode_);
}
if (getActionList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(actionMemoizedSerializedSize);
}
for (int i = 0; i < action_.size(); i++) {
output.writeEnumNoTag(action_.get(i));
}
if (withGrantOption_ != false) {
output.writeBool(3, withGrantOption_);
}
if (object_ != null) {
output.writeMessage(4, getObject());
}
if (grantedType_ != cz.proto.access.GrantedType.Type.PRIVILEGE.getNumber()) {
output.writeEnum(5, grantedType_);
}
if (subObjectType_ != cz.proto.ObjectType.UNKNOWN.getNumber()) {
output.writeEnum(6, subObjectType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (mode_ != cz.proto.access.EffectMode.DENY_OVERRIDE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, mode_);
}
{
int dataSize = 0;
for (int i = 0; i < action_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(action_.get(i));
}
size += dataSize;
if (!getActionList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}actionMemoizedSerializedSize = dataSize;
}
if (withGrantOption_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, withGrantOption_);
}
if (object_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getObject());
}
if (grantedType_ != cz.proto.access.GrantedType.Type.PRIVILEGE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, grantedType_);
}
if (subObjectType_ != cz.proto.ObjectType.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, subObjectType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.access.CheckPrivileges.Content)) {
return super.equals(obj);
}
cz.proto.access.CheckPrivileges.Content other = (cz.proto.access.CheckPrivileges.Content) obj;
if (mode_ != other.mode_) return false;
if (!action_.equals(other.action_)) return false;
if (getWithGrantOption()
!= other.getWithGrantOption()) return false;
if (hasObject() != other.hasObject()) return false;
if (hasObject()) {
if (!getObject()
.equals(other.getObject())) return false;
}
if (grantedType_ != other.grantedType_) return false;
if (subObjectType_ != other.subObjectType_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
if (getActionCount() > 0) {
hash = (37 * hash) + ACTION_FIELD_NUMBER;
hash = (53 * hash) + action_.hashCode();
}
hash = (37 * hash) + WITH_GRANT_OPTION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWithGrantOption());
if (hasObject()) {
hash = (37 * hash) + OBJECT_FIELD_NUMBER;
hash = (53 * hash) + getObject().hashCode();
}
hash = (37 * hash) + GRANTED_TYPE_FIELD_NUMBER;
hash = (53 * hash) + grantedType_;
hash = (37 * hash) + SUB_OBJECT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + subObjectType_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges.Content parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges.Content parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges.Content parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.access.CheckPrivileges.Content prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.access.CheckPrivileges.Content}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.access.CheckPrivileges.Content)
cz.proto.access.CheckPrivileges.ContentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_Content_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_Content_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.access.CheckPrivileges.Content.class, cz.proto.access.CheckPrivileges.Content.Builder.class);
}
// Construct using cz.proto.access.CheckPrivileges.Content.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
mode_ = 0;
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
withGrantOption_ = false;
if (objectBuilder_ == null) {
object_ = null;
} else {
object_ = null;
objectBuilder_ = null;
}
grantedType_ = 0;
subObjectType_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_Content_descriptor;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges.Content getDefaultInstanceForType() {
return cz.proto.access.CheckPrivileges.Content.getDefaultInstance();
}
@java.lang.Override
public cz.proto.access.CheckPrivileges.Content build() {
cz.proto.access.CheckPrivileges.Content result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges.Content buildPartial() {
cz.proto.access.CheckPrivileges.Content result = new cz.proto.access.CheckPrivileges.Content(this);
int from_bitField0_ = bitField0_;
result.mode_ = mode_;
if (((bitField0_ & 0x00000001) != 0)) {
action_ = java.util.Collections.unmodifiableList(action_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.action_ = action_;
result.withGrantOption_ = withGrantOption_;
if (objectBuilder_ == null) {
result.object_ = object_;
} else {
result.object_ = objectBuilder_.build();
}
result.grantedType_ = grantedType_;
result.subObjectType_ = subObjectType_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.access.CheckPrivileges.Content) {
return mergeFrom((cz.proto.access.CheckPrivileges.Content)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.access.CheckPrivileges.Content other) {
if (other == cz.proto.access.CheckPrivileges.Content.getDefaultInstance()) return this;
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
if (!other.action_.isEmpty()) {
if (action_.isEmpty()) {
action_ = other.action_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureActionIsMutable();
action_.addAll(other.action_);
}
onChanged();
}
if (other.getWithGrantOption() != false) {
setWithGrantOption(other.getWithGrantOption());
}
if (other.hasObject()) {
mergeObject(other.getObject());
}
if (other.grantedType_ != 0) {
setGrantedTypeValue(other.getGrantedTypeValue());
}
if (other.subObjectType_ != 0) {
setSubObjectTypeValue(other.getSubObjectTypeValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.access.CheckPrivileges.Content parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.access.CheckPrivileges.Content) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int mode_ = 0;
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The enum numeric value on the wire for mode.
*/
@java.lang.Override public int getModeValue() {
return mode_;
}
/**
* .cz.proto.access.EffectMode mode = 1;
* @param value The enum numeric value on the wire for mode to set.
* @return This builder for chaining.
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
* .cz.proto.access.EffectMode mode = 1;
* @return The mode.
*/
@java.lang.Override
public cz.proto.access.EffectMode getMode() {
@SuppressWarnings("deprecation")
cz.proto.access.EffectMode result = cz.proto.access.EffectMode.valueOf(mode_);
return result == null ? cz.proto.access.EffectMode.UNRECOGNIZED : result;
}
/**
* .cz.proto.access.EffectMode mode = 1;
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(cz.proto.access.EffectMode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.access.EffectMode mode = 1;
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
private java.util.List action_ =
java.util.Collections.emptyList();
private void ensureActionIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
action_ = new java.util.ArrayList(action_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the action.
*/
public java.util.List getActionList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, cz.proto.access.ActionType>(action_, action_converter_);
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return The count of action.
*/
public int getActionCount() {
return action_.size();
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the element to return.
* @return The action at the given index.
*/
public cz.proto.access.ActionType getAction(int index) {
return action_converter_.convert(action_.get(index));
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index to set the value at.
* @param value The action to set.
* @return This builder for chaining.
*/
public Builder setAction(
int index, cz.proto.access.ActionType value) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.set(index, value.getNumber());
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param value The action to add.
* @return This builder for chaining.
*/
public Builder addAction(cz.proto.access.ActionType value) {
if (value == null) {
throw new NullPointerException();
}
ensureActionIsMutable();
action_.add(value.getNumber());
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param values The action to add.
* @return This builder for chaining.
*/
public Builder addAllAction(
java.lang.Iterable extends cz.proto.access.ActionType> values) {
ensureActionIsMutable();
for (cz.proto.access.ActionType value : values) {
action_.add(value.getNumber());
}
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return This builder for chaining.
*/
public Builder clearAction() {
action_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @return A list containing the enum numeric values on the wire for action.
*/
public java.util.List
getActionValueList() {
return java.util.Collections.unmodifiableList(action_);
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of action at the given index.
*/
public int getActionValue(int index) {
return action_.get(index);
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of action at the given index.
* @return This builder for chaining.
*/
public Builder setActionValue(
int index, int value) {
ensureActionIsMutable();
action_.set(index, value);
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param value The enum numeric value on the wire for action to add.
* @return This builder for chaining.
*/
public Builder addActionValue(int value) {
ensureActionIsMutable();
action_.add(value);
onChanged();
return this;
}
/**
* repeated .cz.proto.access.ActionType action = 2;
* @param values The enum numeric values on the wire for action to add.
* @return This builder for chaining.
*/
public Builder addAllActionValue(
java.lang.Iterable values) {
ensureActionIsMutable();
for (int value : values) {
action_.add(value);
}
onChanged();
return this;
}
private boolean withGrantOption_ ;
/**
*
* true means that we check grant_action privileges for grant/revoke query
*
*
* bool with_grant_option = 3;
* @return The withGrantOption.
*/
@java.lang.Override
public boolean getWithGrantOption() {
return withGrantOption_;
}
/**
*
* true means that we check grant_action privileges for grant/revoke query
*
*
* bool with_grant_option = 3;
* @param value The withGrantOption to set.
* @return This builder for chaining.
*/
public Builder setWithGrantOption(boolean value) {
withGrantOption_ = value;
onChanged();
return this;
}
/**
*
* true means that we check grant_action privileges for grant/revoke query
*
*
* bool with_grant_option = 3;
* @return This builder for chaining.
*/
public Builder clearWithGrantOption() {
withGrantOption_ = false;
onChanged();
return this;
}
private cz.proto.ObjectIdentifier object_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ObjectIdentifier, cz.proto.ObjectIdentifier.Builder, cz.proto.ObjectIdentifierOrBuilder> objectBuilder_;
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return Whether the object field is set.
*/
public boolean hasObject() {
return objectBuilder_ != null || object_ != null;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
* @return The object.
*/
public cz.proto.ObjectIdentifier getObject() {
if (objectBuilder_ == null) {
return object_ == null ? cz.proto.ObjectIdentifier.getDefaultInstance() : object_;
} else {
return objectBuilder_.getMessage();
}
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public Builder setObject(cz.proto.ObjectIdentifier value) {
if (objectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
object_ = value;
onChanged();
} else {
objectBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public Builder setObject(
cz.proto.ObjectIdentifier.Builder builderForValue) {
if (objectBuilder_ == null) {
object_ = builderForValue.build();
onChanged();
} else {
objectBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public Builder mergeObject(cz.proto.ObjectIdentifier value) {
if (objectBuilder_ == null) {
if (object_ != null) {
object_ =
cz.proto.ObjectIdentifier.newBuilder(object_).mergeFrom(value).buildPartial();
} else {
object_ = value;
}
onChanged();
} else {
objectBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public Builder clearObject() {
if (objectBuilder_ == null) {
object_ = null;
onChanged();
} else {
object_ = null;
objectBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public cz.proto.ObjectIdentifier.Builder getObjectBuilder() {
onChanged();
return getObjectFieldBuilder().getBuilder();
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
public cz.proto.ObjectIdentifierOrBuilder getObjectOrBuilder() {
if (objectBuilder_ != null) {
return objectBuilder_.getMessageOrBuilder();
} else {
return object_ == null ?
cz.proto.ObjectIdentifier.getDefaultInstance() : object_;
}
}
/**
* .cz.proto.ObjectIdentifier object = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ObjectIdentifier, cz.proto.ObjectIdentifier.Builder, cz.proto.ObjectIdentifierOrBuilder>
getObjectFieldBuilder() {
if (objectBuilder_ == null) {
objectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ObjectIdentifier, cz.proto.ObjectIdentifier.Builder, cz.proto.ObjectIdentifierOrBuilder>(
getObject(),
getParentForChildren(),
isClean());
object_ = null;
}
return objectBuilder_;
}
private int grantedType_ = 0;
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The enum numeric value on the wire for grantedType.
*/
@java.lang.Override public int getGrantedTypeValue() {
return grantedType_;
}
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @param value The enum numeric value on the wire for grantedType to set.
* @return This builder for chaining.
*/
public Builder setGrantedTypeValue(int value) {
grantedType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return The grantedType.
*/
@java.lang.Override
public cz.proto.access.GrantedType.Type getGrantedType() {
@SuppressWarnings("deprecation")
cz.proto.access.GrantedType.Type result = cz.proto.access.GrantedType.Type.valueOf(grantedType_);
return result == null ? cz.proto.access.GrantedType.Type.UNRECOGNIZED : result;
}
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @param value The grantedType to set.
* @return This builder for chaining.
*/
public Builder setGrantedType(cz.proto.access.GrantedType.Type value) {
if (value == null) {
throw new NullPointerException();
}
grantedType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.access.GrantedType.Type granted_type = 5;
* @return This builder for chaining.
*/
public Builder clearGrantedType() {
grantedType_ = 0;
onChanged();
return this;
}
private int subObjectType_ = 0;
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The enum numeric value on the wire for subObjectType.
*/
@java.lang.Override public int getSubObjectTypeValue() {
return subObjectType_;
}
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @param value The enum numeric value on the wire for subObjectType to set.
* @return This builder for chaining.
*/
public Builder setSubObjectTypeValue(int value) {
subObjectType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return The subObjectType.
*/
@java.lang.Override
public cz.proto.ObjectType getSubObjectType() {
@SuppressWarnings("deprecation")
cz.proto.ObjectType result = cz.proto.ObjectType.valueOf(subObjectType_);
return result == null ? cz.proto.ObjectType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @param value The subObjectType to set.
* @return This builder for chaining.
*/
public Builder setSubObjectType(cz.proto.ObjectType value) {
if (value == null) {
throw new NullPointerException();
}
subObjectType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ObjectType sub_object_type = 6;
* @return This builder for chaining.
*/
public Builder clearSubObjectType() {
subObjectType_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.access.CheckPrivileges.Content)
}
// @@protoc_insertion_point(class_scope:cz.proto.access.CheckPrivileges.Content)
private static final cz.proto.access.CheckPrivileges.Content DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.access.CheckPrivileges.Content();
}
public static cz.proto.access.CheckPrivileges.Content getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Content parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Content(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges.Content getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int PRINCIPAL_FIELD_NUMBER = 1;
private cz.proto.AccountOuterClass.UserIdentifier principal_;
/**
* .cz.proto.UserIdentifier principal = 1;
* @return Whether the principal field is set.
*/
@java.lang.Override
public boolean hasPrincipal() {
return principal_ != null;
}
/**
* .cz.proto.UserIdentifier principal = 1;
* @return The principal.
*/
@java.lang.Override
public cz.proto.AccountOuterClass.UserIdentifier getPrincipal() {
return principal_ == null ? cz.proto.AccountOuterClass.UserIdentifier.getDefaultInstance() : principal_;
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
@java.lang.Override
public cz.proto.AccountOuterClass.UserIdentifierOrBuilder getPrincipalOrBuilder() {
return getPrincipal();
}
public static final int ACCESS_TOKEN_FIELD_NUMBER = 2;
private volatile java.lang.Object accessToken_;
/**
* string access_token = 2;
* @return The accessToken.
*/
@java.lang.Override
public java.lang.String getAccessToken() {
java.lang.Object ref = accessToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessToken_ = s;
return s;
}
}
/**
* string access_token = 2;
* @return The bytes for accessToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccessTokenBytes() {
java.lang.Object ref = accessToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTENT_FIELD_NUMBER = 4;
private java.util.List content_;
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
@java.lang.Override
public java.util.List getContentList() {
return content_;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
@java.lang.Override
public java.util.List extends cz.proto.access.CheckPrivileges.ContentOrBuilder>
getContentOrBuilderList() {
return content_;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
@java.lang.Override
public int getContentCount() {
return content_.size();
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
@java.lang.Override
public cz.proto.access.CheckPrivileges.Content getContent(int index) {
return content_.get(index);
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
@java.lang.Override
public cz.proto.access.CheckPrivileges.ContentOrBuilder getContentOrBuilder(
int index) {
return content_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (principal_ != null) {
output.writeMessage(1, getPrincipal());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(accessToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, accessToken_);
}
for (int i = 0; i < content_.size(); i++) {
output.writeMessage(4, content_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (principal_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPrincipal());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(accessToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, accessToken_);
}
for (int i = 0; i < content_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, content_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.access.CheckPrivileges)) {
return super.equals(obj);
}
cz.proto.access.CheckPrivileges other = (cz.proto.access.CheckPrivileges) obj;
if (hasPrincipal() != other.hasPrincipal()) return false;
if (hasPrincipal()) {
if (!getPrincipal()
.equals(other.getPrincipal())) return false;
}
if (!getAccessToken()
.equals(other.getAccessToken())) return false;
if (!getContentList()
.equals(other.getContentList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPrincipal()) {
hash = (37 * hash) + PRINCIPAL_FIELD_NUMBER;
hash = (53 * hash) + getPrincipal().hashCode();
}
hash = (37 * hash) + ACCESS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getAccessToken().hashCode();
if (getContentCount() > 0) {
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContentList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.access.CheckPrivileges parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.access.CheckPrivileges parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.access.CheckPrivileges parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.access.CheckPrivileges parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.access.CheckPrivileges prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.access.CheckPrivileges}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.access.CheckPrivileges)
cz.proto.access.CheckPrivilegesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.access.CheckPrivileges.class, cz.proto.access.CheckPrivileges.Builder.class);
}
// Construct using cz.proto.access.CheckPrivileges.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getContentFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (principalBuilder_ == null) {
principal_ = null;
} else {
principal_ = null;
principalBuilder_ = null;
}
accessToken_ = "";
if (contentBuilder_ == null) {
content_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
contentBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.access.PrivilegeOuterClass.internal_static_cz_proto_access_CheckPrivileges_descriptor;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges getDefaultInstanceForType() {
return cz.proto.access.CheckPrivileges.getDefaultInstance();
}
@java.lang.Override
public cz.proto.access.CheckPrivileges build() {
cz.proto.access.CheckPrivileges result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges buildPartial() {
cz.proto.access.CheckPrivileges result = new cz.proto.access.CheckPrivileges(this);
int from_bitField0_ = bitField0_;
if (principalBuilder_ == null) {
result.principal_ = principal_;
} else {
result.principal_ = principalBuilder_.build();
}
result.accessToken_ = accessToken_;
if (contentBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
content_ = java.util.Collections.unmodifiableList(content_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.content_ = content_;
} else {
result.content_ = contentBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.access.CheckPrivileges) {
return mergeFrom((cz.proto.access.CheckPrivileges)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.access.CheckPrivileges other) {
if (other == cz.proto.access.CheckPrivileges.getDefaultInstance()) return this;
if (other.hasPrincipal()) {
mergePrincipal(other.getPrincipal());
}
if (!other.getAccessToken().isEmpty()) {
accessToken_ = other.accessToken_;
onChanged();
}
if (contentBuilder_ == null) {
if (!other.content_.isEmpty()) {
if (content_.isEmpty()) {
content_ = other.content_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureContentIsMutable();
content_.addAll(other.content_);
}
onChanged();
}
} else {
if (!other.content_.isEmpty()) {
if (contentBuilder_.isEmpty()) {
contentBuilder_.dispose();
contentBuilder_ = null;
content_ = other.content_;
bitField0_ = (bitField0_ & ~0x00000001);
contentBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getContentFieldBuilder() : null;
} else {
contentBuilder_.addAllMessages(other.content_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.access.CheckPrivileges parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.access.CheckPrivileges) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.AccountOuterClass.UserIdentifier principal_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.AccountOuterClass.UserIdentifier, cz.proto.AccountOuterClass.UserIdentifier.Builder, cz.proto.AccountOuterClass.UserIdentifierOrBuilder> principalBuilder_;
/**
* .cz.proto.UserIdentifier principal = 1;
* @return Whether the principal field is set.
*/
public boolean hasPrincipal() {
return principalBuilder_ != null || principal_ != null;
}
/**
* .cz.proto.UserIdentifier principal = 1;
* @return The principal.
*/
public cz.proto.AccountOuterClass.UserIdentifier getPrincipal() {
if (principalBuilder_ == null) {
return principal_ == null ? cz.proto.AccountOuterClass.UserIdentifier.getDefaultInstance() : principal_;
} else {
return principalBuilder_.getMessage();
}
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public Builder setPrincipal(cz.proto.AccountOuterClass.UserIdentifier value) {
if (principalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
principal_ = value;
onChanged();
} else {
principalBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public Builder setPrincipal(
cz.proto.AccountOuterClass.UserIdentifier.Builder builderForValue) {
if (principalBuilder_ == null) {
principal_ = builderForValue.build();
onChanged();
} else {
principalBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public Builder mergePrincipal(cz.proto.AccountOuterClass.UserIdentifier value) {
if (principalBuilder_ == null) {
if (principal_ != null) {
principal_ =
cz.proto.AccountOuterClass.UserIdentifier.newBuilder(principal_).mergeFrom(value).buildPartial();
} else {
principal_ = value;
}
onChanged();
} else {
principalBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public Builder clearPrincipal() {
if (principalBuilder_ == null) {
principal_ = null;
onChanged();
} else {
principal_ = null;
principalBuilder_ = null;
}
return this;
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public cz.proto.AccountOuterClass.UserIdentifier.Builder getPrincipalBuilder() {
onChanged();
return getPrincipalFieldBuilder().getBuilder();
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
public cz.proto.AccountOuterClass.UserIdentifierOrBuilder getPrincipalOrBuilder() {
if (principalBuilder_ != null) {
return principalBuilder_.getMessageOrBuilder();
} else {
return principal_ == null ?
cz.proto.AccountOuterClass.UserIdentifier.getDefaultInstance() : principal_;
}
}
/**
* .cz.proto.UserIdentifier principal = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.AccountOuterClass.UserIdentifier, cz.proto.AccountOuterClass.UserIdentifier.Builder, cz.proto.AccountOuterClass.UserIdentifierOrBuilder>
getPrincipalFieldBuilder() {
if (principalBuilder_ == null) {
principalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.AccountOuterClass.UserIdentifier, cz.proto.AccountOuterClass.UserIdentifier.Builder, cz.proto.AccountOuterClass.UserIdentifierOrBuilder>(
getPrincipal(),
getParentForChildren(),
isClean());
principal_ = null;
}
return principalBuilder_;
}
private java.lang.Object accessToken_ = "";
/**
* string access_token = 2;
* @return The accessToken.
*/
public java.lang.String getAccessToken() {
java.lang.Object ref = accessToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string access_token = 2;
* @return The bytes for accessToken.
*/
public com.google.protobuf.ByteString
getAccessTokenBytes() {
java.lang.Object ref = accessToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string access_token = 2;
* @param value The accessToken to set.
* @return This builder for chaining.
*/
public Builder setAccessToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accessToken_ = value;
onChanged();
return this;
}
/**
* string access_token = 2;
* @return This builder for chaining.
*/
public Builder clearAccessToken() {
accessToken_ = getDefaultInstance().getAccessToken();
onChanged();
return this;
}
/**
* string access_token = 2;
* @param value The bytes for accessToken to set.
* @return This builder for chaining.
*/
public Builder setAccessTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accessToken_ = value;
onChanged();
return this;
}
private java.util.List content_ =
java.util.Collections.emptyList();
private void ensureContentIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
content_ = new java.util.ArrayList(content_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.access.CheckPrivileges.Content, cz.proto.access.CheckPrivileges.Content.Builder, cz.proto.access.CheckPrivileges.ContentOrBuilder> contentBuilder_;
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public java.util.List getContentList() {
if (contentBuilder_ == null) {
return java.util.Collections.unmodifiableList(content_);
} else {
return contentBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public int getContentCount() {
if (contentBuilder_ == null) {
return content_.size();
} else {
return contentBuilder_.getCount();
}
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public cz.proto.access.CheckPrivileges.Content getContent(int index) {
if (contentBuilder_ == null) {
return content_.get(index);
} else {
return contentBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder setContent(
int index, cz.proto.access.CheckPrivileges.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.set(index, value);
onChanged();
} else {
contentBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder setContent(
int index, cz.proto.access.CheckPrivileges.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.set(index, builderForValue.build());
onChanged();
} else {
contentBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder addContent(cz.proto.access.CheckPrivileges.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.add(value);
onChanged();
} else {
contentBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder addContent(
int index, cz.proto.access.CheckPrivileges.Content value) {
if (contentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContentIsMutable();
content_.add(index, value);
onChanged();
} else {
contentBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder addContent(
cz.proto.access.CheckPrivileges.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.add(builderForValue.build());
onChanged();
} else {
contentBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder addContent(
int index, cz.proto.access.CheckPrivileges.Content.Builder builderForValue) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.add(index, builderForValue.build());
onChanged();
} else {
contentBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder addAllContent(
java.lang.Iterable extends cz.proto.access.CheckPrivileges.Content> values) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, content_);
onChanged();
} else {
contentBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder clearContent() {
if (contentBuilder_ == null) {
content_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
contentBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public Builder removeContent(int index) {
if (contentBuilder_ == null) {
ensureContentIsMutable();
content_.remove(index);
onChanged();
} else {
contentBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public cz.proto.access.CheckPrivileges.Content.Builder getContentBuilder(
int index) {
return getContentFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public cz.proto.access.CheckPrivileges.ContentOrBuilder getContentOrBuilder(
int index) {
if (contentBuilder_ == null) {
return content_.get(index); } else {
return contentBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public java.util.List extends cz.proto.access.CheckPrivileges.ContentOrBuilder>
getContentOrBuilderList() {
if (contentBuilder_ != null) {
return contentBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(content_);
}
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public cz.proto.access.CheckPrivileges.Content.Builder addContentBuilder() {
return getContentFieldBuilder().addBuilder(
cz.proto.access.CheckPrivileges.Content.getDefaultInstance());
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public cz.proto.access.CheckPrivileges.Content.Builder addContentBuilder(
int index) {
return getContentFieldBuilder().addBuilder(
index, cz.proto.access.CheckPrivileges.Content.getDefaultInstance());
}
/**
* repeated .cz.proto.access.CheckPrivileges.Content content = 4;
*/
public java.util.List
getContentBuilderList() {
return getContentFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.access.CheckPrivileges.Content, cz.proto.access.CheckPrivileges.Content.Builder, cz.proto.access.CheckPrivileges.ContentOrBuilder>
getContentFieldBuilder() {
if (contentBuilder_ == null) {
contentBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.access.CheckPrivileges.Content, cz.proto.access.CheckPrivileges.Content.Builder, cz.proto.access.CheckPrivileges.ContentOrBuilder>(
content_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
content_ = null;
}
return contentBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.access.CheckPrivileges)
}
// @@protoc_insertion_point(class_scope:cz.proto.access.CheckPrivileges)
private static final cz.proto.access.CheckPrivileges DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.access.CheckPrivileges();
}
public static cz.proto.access.CheckPrivileges getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckPrivileges parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckPrivileges(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.access.CheckPrivileges getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy