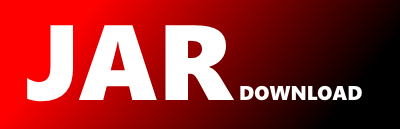
cz.proto.coordinator.CoordinatorServiceOuterClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: coordinator_service.proto
package cz.proto.coordinator;
public final class CoordinatorServiceOuterClass {
private CoordinatorServiceOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code cz.proto.coordinator.JobRequestMode}
*/
public enum JobRequestMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
*
* polling for timeout, if not terminated, then fallback to async
*
*
* HYBRID = 1;
*/
HYBRID(1),
/**
*
* purely async
*
*
* ASYNC = 2;
*/
ASYNC(2),
/**
*
* purely sync, only for debug/test
*
*
* SYNC = 3;
*/
SYNC(3),
UNRECOGNIZED(-1),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
*
* polling for timeout, if not terminated, then fallback to async
*
*
* HYBRID = 1;
*/
public static final int HYBRID_VALUE = 1;
/**
*
* purely async
*
*
* ASYNC = 2;
*/
public static final int ASYNC_VALUE = 2;
/**
*
* purely sync, only for debug/test
*
*
* SYNC = 3;
*/
public static final int SYNC_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static JobRequestMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static JobRequestMode forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return HYBRID;
case 2: return ASYNC;
case 3: return SYNC;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
JobRequestMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public JobRequestMode findValueByNumber(int number) {
return JobRequestMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.getDescriptor().getEnumTypes().get(0);
}
private static final JobRequestMode[] VALUES = values();
public static JobRequestMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private JobRequestMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.JobRequestMode)
}
/**
* Protobuf enum {@code cz.proto.coordinator.ResultFormat}
*/
public enum ResultFormat
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TEXT = 0;
*/
TEXT(0),
/**
* CSV = 1;
*/
CSV(1),
/**
* JSON = 2;
*/
JSON(2),
/**
* PARQUET = 3;
*/
PARQUET(3),
/**
* HIVE_RESULT = 4;
*/
HIVE_RESULT(4),
/**
* ARROW = 5;
*/
ARROW(5),
UNRECOGNIZED(-1),
;
/**
* TEXT = 0;
*/
public static final int TEXT_VALUE = 0;
/**
* CSV = 1;
*/
public static final int CSV_VALUE = 1;
/**
* JSON = 2;
*/
public static final int JSON_VALUE = 2;
/**
* PARQUET = 3;
*/
public static final int PARQUET_VALUE = 3;
/**
* HIVE_RESULT = 4;
*/
public static final int HIVE_RESULT_VALUE = 4;
/**
* ARROW = 5;
*/
public static final int ARROW_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultFormat valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ResultFormat forNumber(int value) {
switch (value) {
case 0: return TEXT;
case 1: return CSV;
case 2: return JSON;
case 3: return PARQUET;
case 4: return HIVE_RESULT;
case 5: return ARROW;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ResultFormat> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ResultFormat findValueByNumber(int number) {
return ResultFormat.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.getDescriptor().getEnumTypes().get(1);
}
private static final ResultFormat[] VALUES = values();
public static ResultFormat valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ResultFormat(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.ResultFormat)
}
/**
* Protobuf enum {@code cz.proto.coordinator.ResultType}
*/
public enum ResultType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* BUFFERED_STREAM = 0;
*/
BUFFERED_STREAM(0),
/**
* FILE_SYSTEM = 1;
*/
FILE_SYSTEM(1),
UNRECOGNIZED(-1),
;
/**
* BUFFERED_STREAM = 0;
*/
public static final int BUFFERED_STREAM_VALUE = 0;
/**
* FILE_SYSTEM = 1;
*/
public static final int FILE_SYSTEM_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ResultType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ResultType forNumber(int value) {
switch (value) {
case 0: return BUFFERED_STREAM;
case 1: return FILE_SYSTEM;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ResultType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ResultType findValueByNumber(int number) {
return ResultType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.getDescriptor().getEnumTypes().get(2);
}
private static final ResultType[] VALUES = values();
public static ResultType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ResultType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.ResultType)
}
/**
* Protobuf enum {@code cz.proto.coordinator.JobScheduleStrategy}
*/
public enum JobScheduleStrategy
implements com.google.protobuf.ProtocolMessageEnum {
/**
* JOB_AFFINITY = 0;
*/
JOB_AFFINITY(0),
/**
* JOB_AFFINITY_BASED_LOAD_BALANCE = 1;
*/
JOB_AFFINITY_BASED_LOAD_BALANCE(1),
/**
* JOB_AFFINITY_BASED_MEMORY = 2;
*/
JOB_AFFINITY_BASED_MEMORY(2),
/**
* RANDOM = 10;
*/
RANDOM(10),
UNRECOGNIZED(-1),
;
/**
* JOB_AFFINITY = 0;
*/
public static final int JOB_AFFINITY_VALUE = 0;
/**
* JOB_AFFINITY_BASED_LOAD_BALANCE = 1;
*/
public static final int JOB_AFFINITY_BASED_LOAD_BALANCE_VALUE = 1;
/**
* JOB_AFFINITY_BASED_MEMORY = 2;
*/
public static final int JOB_AFFINITY_BASED_MEMORY_VALUE = 2;
/**
* RANDOM = 10;
*/
public static final int RANDOM_VALUE = 10;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static JobScheduleStrategy valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static JobScheduleStrategy forNumber(int value) {
switch (value) {
case 0: return JOB_AFFINITY;
case 1: return JOB_AFFINITY_BASED_LOAD_BALANCE;
case 2: return JOB_AFFINITY_BASED_MEMORY;
case 10: return RANDOM;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
JobScheduleStrategy> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public JobScheduleStrategy findValueByNumber(int number) {
return JobScheduleStrategy.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.getDescriptor().getEnumTypes().get(3);
}
private static final JobScheduleStrategy[] VALUES = values();
public static JobScheduleStrategy valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private JobScheduleStrategy(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.JobScheduleStrategy)
}
/**
* Protobuf enum {@code cz.proto.coordinator.UpgradeActionType}
*/
public enum UpgradeActionType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* START_UPGRADE = 0;
*/
START_UPGRADE(0),
/**
* FINISH_UPGRADE = 1;
*/
FINISH_UPGRADE(1),
/**
* ABORT_UPGRADE = 2;
*/
ABORT_UPGRADE(2),
UNRECOGNIZED(-1),
;
/**
* START_UPGRADE = 0;
*/
public static final int START_UPGRADE_VALUE = 0;
/**
* FINISH_UPGRADE = 1;
*/
public static final int FINISH_UPGRADE_VALUE = 1;
/**
* ABORT_UPGRADE = 2;
*/
public static final int ABORT_UPGRADE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static UpgradeActionType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static UpgradeActionType forNumber(int value) {
switch (value) {
case 0: return START_UPGRADE;
case 1: return FINISH_UPGRADE;
case 2: return ABORT_UPGRADE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
UpgradeActionType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public UpgradeActionType findValueByNumber(int number) {
return UpgradeActionType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.getDescriptor().getEnumTypes().get(4);
}
private static final UpgradeActionType[] VALUES = values();
public static UpgradeActionType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private UpgradeActionType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.UpgradeActionType)
}
public interface JobIDOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobID)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
long getInstanceId();
}
/**
* Protobuf type {@code cz.proto.coordinator.JobID}
*/
public static final class JobID extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobID)
JobIDOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobID.newBuilder() to construct.
private JobID(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobID() {
id_ = "";
workspace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobID();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobID(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 24: {
instanceId_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobID_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_ID_FIELD_NUMBER = 3;
private long instanceId_;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (instanceId_ != 0L) {
output.writeInt64(3, instanceId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, instanceId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobID)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobID) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobID}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobID)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobID_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobID_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
workspace_ = "";
instanceId_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobID_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobID(this);
result.id_ = id_;
result.workspace_ = workspace_;
result.instanceId_ = instanceId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobID) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobID)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobID) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 3;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 3;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobID)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobID)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobID DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobID();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobID parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobID(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.Account)
com.google.protobuf.MessageOrBuilder {
/**
* int64 user_id = 1;
* @return The userId.
*/
long getUserId();
/**
* string access_token = 2;
* @return The accessToken.
*/
java.lang.String getAccessToken();
/**
* string access_token = 2;
* @return The bytes for accessToken.
*/
com.google.protobuf.ByteString
getAccessTokenBytes();
}
/**
*
* Coordinator Service API
*
*
* Protobuf type {@code cz.proto.coordinator.Account}
*/
public static final class Account extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.Account)
AccountOrBuilder {
private static final long serialVersionUID = 0L;
// Use Account.newBuilder() to construct.
private Account(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Account() {
accessToken_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Account();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Account(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
userId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
accessToken_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_Account_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_Account_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.class, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder.class);
}
public static final int USER_ID_FIELD_NUMBER = 1;
private long userId_;
/**
* int64 user_id = 1;
* @return The userId.
*/
@java.lang.Override
public long getUserId() {
return userId_;
}
public static final int ACCESS_TOKEN_FIELD_NUMBER = 2;
private volatile java.lang.Object accessToken_;
/**
* string access_token = 2;
* @return The accessToken.
*/
@java.lang.Override
public java.lang.String getAccessToken() {
java.lang.Object ref = accessToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessToken_ = s;
return s;
}
}
/**
* string access_token = 2;
* @return The bytes for accessToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccessTokenBytes() {
java.lang.Object ref = accessToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (userId_ != 0L) {
output.writeInt64(1, userId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(accessToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, accessToken_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (userId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, userId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(accessToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, accessToken_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.Account)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.Account other = (cz.proto.coordinator.CoordinatorServiceOuterClass.Account) obj;
if (getUserId()
!= other.getUserId()) return false;
if (!getAccessToken()
.equals(other.getAccessToken())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + USER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUserId());
hash = (37 * hash) + ACCESS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getAccessToken().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.Account prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Coordinator Service API
*
*
* Protobuf type {@code cz.proto.coordinator.Account}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.Account)
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_Account_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_Account_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.class, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
userId_ = 0L;
accessToken_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_Account_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account result = new cz.proto.coordinator.CoordinatorServiceOuterClass.Account(this);
result.userId_ = userId_;
result.accessToken_ = accessToken_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.Account) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.Account)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.Account other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance()) return this;
if (other.getUserId() != 0L) {
setUserId(other.getUserId());
}
if (!other.getAccessToken().isEmpty()) {
accessToken_ = other.accessToken_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.Account) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long userId_ ;
/**
* int64 user_id = 1;
* @return The userId.
*/
@java.lang.Override
public long getUserId() {
return userId_;
}
/**
* int64 user_id = 1;
* @param value The userId to set.
* @return This builder for chaining.
*/
public Builder setUserId(long value) {
userId_ = value;
onChanged();
return this;
}
/**
* int64 user_id = 1;
* @return This builder for chaining.
*/
public Builder clearUserId() {
userId_ = 0L;
onChanged();
return this;
}
private java.lang.Object accessToken_ = "";
/**
* string access_token = 2;
* @return The accessToken.
*/
public java.lang.String getAccessToken() {
java.lang.Object ref = accessToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string access_token = 2;
* @return The bytes for accessToken.
*/
public com.google.protobuf.ByteString
getAccessTokenBytes() {
java.lang.Object ref = accessToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string access_token = 2;
* @param value The accessToken to set.
* @return This builder for chaining.
*/
public Builder setAccessToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accessToken_ = value;
onChanged();
return this;
}
/**
* string access_token = 2;
* @return This builder for chaining.
*/
public Builder clearAccessToken() {
accessToken_ = getDefaultInstance().getAccessToken();
onChanged();
return this;
}
/**
* string access_token = 2;
* @param value The bytes for accessToken to set.
* @return This builder for chaining.
*/
public Builder setAccessTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accessToken_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.Account)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.Account)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.Account DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.Account();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.Account getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Account parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Account(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ClientContextInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.ClientContextInfo)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string config_statements = 1;
* @return A list containing the configStatements.
*/
java.util.List
getConfigStatementsList();
/**
* repeated string config_statements = 1;
* @return The count of configStatements.
*/
int getConfigStatementsCount();
/**
* repeated string config_statements = 1;
* @param index The index of the element to return.
* @return The configStatements at the given index.
*/
java.lang.String getConfigStatements(int index);
/**
* repeated string config_statements = 1;
* @param index The index of the value to return.
* @return The bytes of the configStatements at the given index.
*/
com.google.protobuf.ByteString
getConfigStatementsBytes(int index);
/**
* string context_json = 2;
* @return The contextJson.
*/
java.lang.String getContextJson();
/**
* string context_json = 2;
* @return The bytes for contextJson.
*/
com.google.protobuf.ByteString
getContextJsonBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.ClientContextInfo}
*/
public static final class ClientContextInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.ClientContextInfo)
ClientContextInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ClientContextInfo.newBuilder() to construct.
private ClientContextInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ClientContextInfo() {
configStatements_ = com.google.protobuf.LazyStringArrayList.EMPTY;
contextJson_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ClientContextInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ClientContextInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
configStatements_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
configStatements_.add(s);
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
contextJson_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
configStatements_ = configStatements_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ClientContextInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ClientContextInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder.class);
}
public static final int CONFIG_STATEMENTS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList configStatements_;
/**
* repeated string config_statements = 1;
* @return A list containing the configStatements.
*/
public com.google.protobuf.ProtocolStringList
getConfigStatementsList() {
return configStatements_;
}
/**
* repeated string config_statements = 1;
* @return The count of configStatements.
*/
public int getConfigStatementsCount() {
return configStatements_.size();
}
/**
* repeated string config_statements = 1;
* @param index The index of the element to return.
* @return The configStatements at the given index.
*/
public java.lang.String getConfigStatements(int index) {
return configStatements_.get(index);
}
/**
* repeated string config_statements = 1;
* @param index The index of the value to return.
* @return The bytes of the configStatements at the given index.
*/
public com.google.protobuf.ByteString
getConfigStatementsBytes(int index) {
return configStatements_.getByteString(index);
}
public static final int CONTEXT_JSON_FIELD_NUMBER = 2;
private volatile java.lang.Object contextJson_;
/**
* string context_json = 2;
* @return The contextJson.
*/
@java.lang.Override
public java.lang.String getContextJson() {
java.lang.Object ref = contextJson_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextJson_ = s;
return s;
}
}
/**
* string context_json = 2;
* @return The bytes for contextJson.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getContextJsonBytes() {
java.lang.Object ref = contextJson_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextJson_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < configStatements_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, configStatements_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(contextJson_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, contextJson_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < configStatements_.size(); i++) {
dataSize += computeStringSizeNoTag(configStatements_.getRaw(i));
}
size += dataSize;
size += 1 * getConfigStatementsList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(contextJson_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, contextJson_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo other = (cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo) obj;
if (!getConfigStatementsList()
.equals(other.getConfigStatementsList())) return false;
if (!getContextJson()
.equals(other.getContextJson())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConfigStatementsCount() > 0) {
hash = (37 * hash) + CONFIG_STATEMENTS_FIELD_NUMBER;
hash = (53 * hash) + getConfigStatementsList().hashCode();
}
hash = (37 * hash) + CONTEXT_JSON_FIELD_NUMBER;
hash = (53 * hash) + getContextJson().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.ClientContextInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.ClientContextInfo)
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ClientContextInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ClientContextInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
configStatements_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
contextJson_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ClientContextInfo_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo result = new cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
configStatements_ = configStatements_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.configStatements_ = configStatements_;
result.contextJson_ = contextJson_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.getDefaultInstance()) return this;
if (!other.configStatements_.isEmpty()) {
if (configStatements_.isEmpty()) {
configStatements_ = other.configStatements_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConfigStatementsIsMutable();
configStatements_.addAll(other.configStatements_);
}
onChanged();
}
if (!other.getContextJson().isEmpty()) {
contextJson_ = other.contextJson_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList configStatements_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureConfigStatementsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
configStatements_ = new com.google.protobuf.LazyStringArrayList(configStatements_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string config_statements = 1;
* @return A list containing the configStatements.
*/
public com.google.protobuf.ProtocolStringList
getConfigStatementsList() {
return configStatements_.getUnmodifiableView();
}
/**
* repeated string config_statements = 1;
* @return The count of configStatements.
*/
public int getConfigStatementsCount() {
return configStatements_.size();
}
/**
* repeated string config_statements = 1;
* @param index The index of the element to return.
* @return The configStatements at the given index.
*/
public java.lang.String getConfigStatements(int index) {
return configStatements_.get(index);
}
/**
* repeated string config_statements = 1;
* @param index The index of the value to return.
* @return The bytes of the configStatements at the given index.
*/
public com.google.protobuf.ByteString
getConfigStatementsBytes(int index) {
return configStatements_.getByteString(index);
}
/**
* repeated string config_statements = 1;
* @param index The index to set the value at.
* @param value The configStatements to set.
* @return This builder for chaining.
*/
public Builder setConfigStatements(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConfigStatementsIsMutable();
configStatements_.set(index, value);
onChanged();
return this;
}
/**
* repeated string config_statements = 1;
* @param value The configStatements to add.
* @return This builder for chaining.
*/
public Builder addConfigStatements(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConfigStatementsIsMutable();
configStatements_.add(value);
onChanged();
return this;
}
/**
* repeated string config_statements = 1;
* @param values The configStatements to add.
* @return This builder for chaining.
*/
public Builder addAllConfigStatements(
java.lang.Iterable values) {
ensureConfigStatementsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, configStatements_);
onChanged();
return this;
}
/**
* repeated string config_statements = 1;
* @return This builder for chaining.
*/
public Builder clearConfigStatements() {
configStatements_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string config_statements = 1;
* @param value The bytes of the configStatements to add.
* @return This builder for chaining.
*/
public Builder addConfigStatementsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureConfigStatementsIsMutable();
configStatements_.add(value);
onChanged();
return this;
}
private java.lang.Object contextJson_ = "";
/**
* string context_json = 2;
* @return The contextJson.
*/
public java.lang.String getContextJson() {
java.lang.Object ref = contextJson_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextJson_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string context_json = 2;
* @return The bytes for contextJson.
*/
public com.google.protobuf.ByteString
getContextJsonBytes() {
java.lang.Object ref = contextJson_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextJson_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string context_json = 2;
* @param value The contextJson to set.
* @return This builder for chaining.
*/
public Builder setContextJson(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contextJson_ = value;
onChanged();
return this;
}
/**
* string context_json = 2;
* @return This builder for chaining.
*/
public Builder clearContextJson() {
contextJson_ = getDefaultInstance().getContextJson();
onChanged();
return this;
}
/**
* string context_json = 2;
* @param value The bytes for contextJson to set.
* @return This builder for chaining.
*/
public Builder setContextJsonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contextJson_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.ClientContextInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.ClientContextInfo)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ClientContextInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ClientContextInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobDescOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobDesc)
com.google.protobuf.MessageOrBuilder {
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The virtualCluster.
*/
java.lang.String getVirtualCluster();
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The bytes for virtualCluster.
*/
com.google.protobuf.ByteString
getVirtualClusterBytes();
/**
* .cz.proto.JobType type = 2;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .cz.proto.JobType type = 2;
* @return The type.
*/
cz.proto.JobType getType();
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string job_name = 4;
* @return The jobName.
*/
java.lang.String getJobName();
/**
* string job_name = 4;
* @return The bytes for jobName.
*/
com.google.protobuf.ByteString
getJobNameBytes();
/**
* .cz.proto.coordinator.Account account = 5;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 5;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 5;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The enum numeric value on the wire for requestMode.
*/
int getRequestModeValue();
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The requestMode.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode getRequestMode();
/**
* int32 hybrid_polling_timeout = 7;
* @return The hybridPollingTimeout.
*/
int getHybridPollingTimeout();
/**
* map<string, string> job_config = 8;
*/
int getJobConfigCount();
/**
* map<string, string> job_config = 8;
*/
boolean containsJobConfig(
java.lang.String key);
/**
* Use {@link #getJobConfigMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getJobConfig();
/**
* map<string, string> job_config = 8;
*/
java.util.Map
getJobConfigMap();
/**
* map<string, string> job_config = 8;
*/
java.lang.String getJobConfigOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
* map<string, string> job_config = 8;
*/
java.lang.String getJobConfigOrThrow(
java.lang.String key);
/**
* .cz.proto.SQLJob sql_job = 9;
* @return Whether the sqlJob field is set.
*/
boolean hasSqlJob();
/**
* .cz.proto.SQLJob sql_job = 9;
* @return The sqlJob.
*/
cz.proto.SQLJob getSqlJob();
/**
* .cz.proto.SQLJob sql_job = 9;
*/
cz.proto.SQLJobOrBuilder getSqlJobOrBuilder();
/**
* uint64 job_timeout_ms = 15;
* @return The jobTimeoutMs.
*/
long getJobTimeoutMs();
/**
* string user_agent = 30;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
/**
* uint32 priority = 31;
* @return The priority.
*/
int getPriority();
/**
* string priority_string = 32;
* @return The priorityString.
*/
java.lang.String getPriorityString();
/**
* string priority_string = 32;
* @return The bytes for priorityString.
*/
com.google.protobuf.ByteString
getPriorityStringBytes();
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return Whether the clientContext field is set.
*/
boolean hasClientContext();
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return The clientContext.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getClientContext();
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder getClientContextOrBuilder();
/**
* string query_tag = 34;
* @return The queryTag.
*/
java.lang.String getQueryTag();
/**
* string query_tag = 34;
* @return The bytes for queryTag.
*/
com.google.protobuf.ByteString
getQueryTagBytes();
/**
* optional string jdbc_domain = 35;
* @return Whether the jdbcDomain field is set.
*/
boolean hasJdbcDomain();
/**
* optional string jdbc_domain = 35;
* @return The jdbcDomain.
*/
java.lang.String getJdbcDomain();
/**
* optional string jdbc_domain = 35;
* @return The bytes for jdbcDomain.
*/
com.google.protobuf.ByteString
getJdbcDomainBytes();
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return Whether the clientProfiling field is set.
*/
boolean hasClientProfiling();
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return The clientProfiling.
*/
cz.proto.JobProfiling getClientProfiling();
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
cz.proto.JobProfilingOrBuilder getClientProfilingOrBuilder();
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.JobCase getJobCase();
}
/**
* Protobuf type {@code cz.proto.coordinator.JobDesc}
*/
public static final class JobDesc extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobDesc)
JobDescOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobDesc.newBuilder() to construct.
private JobDesc(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobDesc() {
virtualCluster_ = "";
type_ = 0;
jobName_ = "";
requestMode_ = 0;
userAgent_ = "";
priorityString_ = "";
queryTag_ = "";
jdbcDomain_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobDesc();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobDesc(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
virtualCluster_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
jobName_ = s;
break;
}
case 42: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 48: {
int rawValue = input.readEnum();
requestMode_ = rawValue;
break;
}
case 56: {
hybridPollingTimeout_ = input.readInt32();
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
jobConfig_ = com.google.protobuf.MapField.newMapField(
JobConfigDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
jobConfig__ = input.readMessage(
JobConfigDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
jobConfig_.getMutableMap().put(
jobConfig__.getKey(), jobConfig__.getValue());
break;
}
case 74: {
cz.proto.SQLJob.Builder subBuilder = null;
if (jobCase_ == 9) {
subBuilder = ((cz.proto.SQLJob) job_).toBuilder();
}
job_ =
input.readMessage(cz.proto.SQLJob.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.SQLJob) job_);
job_ = subBuilder.buildPartial();
}
jobCase_ = 9;
break;
}
case 120: {
jobTimeoutMs_ = input.readUInt64();
break;
}
case 242: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
case 248: {
priority_ = input.readUInt32();
break;
}
case 258: {
java.lang.String s = input.readStringRequireUtf8();
priorityString_ = s;
break;
}
case 266: {
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder subBuilder = null;
if (clientContext_ != null) {
subBuilder = clientContext_.toBuilder();
}
clientContext_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientContext_);
clientContext_ = subBuilder.buildPartial();
}
break;
}
case 274: {
java.lang.String s = input.readStringRequireUtf8();
queryTag_ = s;
break;
}
case 282: {
java.lang.String s = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
jdbcDomain_ = s;
break;
}
case 290: {
cz.proto.JobProfiling.Builder subBuilder = null;
if (clientProfiling_ != null) {
subBuilder = clientProfiling_.toBuilder();
}
clientProfiling_ = input.readMessage(cz.proto.JobProfiling.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(clientProfiling_);
clientProfiling_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 8:
return internalGetJobConfig();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder.class);
}
private int bitField0_;
private int jobCase_ = 0;
private java.lang.Object job_;
public enum JobCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SQL_JOB(9),
JOB_NOT_SET(0);
private final int value;
private JobCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static JobCase valueOf(int value) {
return forNumber(value);
}
public static JobCase forNumber(int value) {
switch (value) {
case 9: return SQL_JOB;
case 0: return JOB_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public JobCase
getJobCase() {
return JobCase.forNumber(
jobCase_);
}
public static final int VIRTUAL_CLUSTER_FIELD_NUMBER = 1;
private volatile java.lang.Object virtualCluster_;
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The virtualCluster.
*/
@java.lang.Override
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
}
}
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The bytes for virtualCluster.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private int type_;
/**
* .cz.proto.JobType type = 2;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.JobType type = 2;
* @return The type.
*/
@java.lang.Override public cz.proto.JobType getType() {
@SuppressWarnings("deprecation")
cz.proto.JobType result = cz.proto.JobType.valueOf(type_);
return result == null ? cz.proto.JobType.UNRECOGNIZED : result;
}
public static final int JOB_ID_FIELD_NUMBER = 3;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int JOB_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object jobName_;
/**
* string job_name = 4;
* @return The jobName.
*/
@java.lang.Override
public java.lang.String getJobName() {
java.lang.Object ref = jobName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobName_ = s;
return s;
}
}
/**
* string job_name = 4;
* @return The bytes for jobName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobNameBytes() {
java.lang.Object ref = jobName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_FIELD_NUMBER = 5;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 5;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 5;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int REQUEST_MODE_FIELD_NUMBER = 6;
private int requestMode_;
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The enum numeric value on the wire for requestMode.
*/
@java.lang.Override public int getRequestModeValue() {
return requestMode_;
}
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The requestMode.
*/
@java.lang.Override public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode getRequestMode() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode result = cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.valueOf(requestMode_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.UNRECOGNIZED : result;
}
public static final int HYBRID_POLLING_TIMEOUT_FIELD_NUMBER = 7;
private int hybridPollingTimeout_;
/**
* int32 hybrid_polling_timeout = 7;
* @return The hybridPollingTimeout.
*/
@java.lang.Override
public int getHybridPollingTimeout() {
return hybridPollingTimeout_;
}
public static final int JOB_CONFIG_FIELD_NUMBER = 8;
private static final class JobConfigDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_JobConfigEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> jobConfig_;
private com.google.protobuf.MapField
internalGetJobConfig() {
if (jobConfig_ == null) {
return com.google.protobuf.MapField.emptyMapField(
JobConfigDefaultEntryHolder.defaultEntry);
}
return jobConfig_;
}
public int getJobConfigCount() {
return internalGetJobConfig().getMap().size();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public boolean containsJobConfig(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetJobConfig().getMap().containsKey(key);
}
/**
* Use {@link #getJobConfigMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getJobConfig() {
return getJobConfigMap();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.util.Map getJobConfigMap() {
return internalGetJobConfig().getMap();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.lang.String getJobConfigOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetJobConfig().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.lang.String getJobConfigOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetJobConfig().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SQL_JOB_FIELD_NUMBER = 9;
/**
* .cz.proto.SQLJob sql_job = 9;
* @return Whether the sqlJob field is set.
*/
@java.lang.Override
public boolean hasSqlJob() {
return jobCase_ == 9;
}
/**
* .cz.proto.SQLJob sql_job = 9;
* @return The sqlJob.
*/
@java.lang.Override
public cz.proto.SQLJob getSqlJob() {
if (jobCase_ == 9) {
return (cz.proto.SQLJob) job_;
}
return cz.proto.SQLJob.getDefaultInstance();
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
@java.lang.Override
public cz.proto.SQLJobOrBuilder getSqlJobOrBuilder() {
if (jobCase_ == 9) {
return (cz.proto.SQLJob) job_;
}
return cz.proto.SQLJob.getDefaultInstance();
}
public static final int JOB_TIMEOUT_MS_FIELD_NUMBER = 15;
private long jobTimeoutMs_;
/**
* uint64 job_timeout_ms = 15;
* @return The jobTimeoutMs.
*/
@java.lang.Override
public long getJobTimeoutMs() {
return jobTimeoutMs_;
}
public static final int USER_AGENT_FIELD_NUMBER = 30;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 30;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRIORITY_FIELD_NUMBER = 31;
private int priority_;
/**
* uint32 priority = 31;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
public static final int PRIORITY_STRING_FIELD_NUMBER = 32;
private volatile java.lang.Object priorityString_;
/**
* string priority_string = 32;
* @return The priorityString.
*/
@java.lang.Override
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
}
}
/**
* string priority_string = 32;
* @return The bytes for priorityString.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_CONTEXT_FIELD_NUMBER = 33;
private cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo clientContext_;
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return Whether the clientContext field is set.
*/
@java.lang.Override
public boolean hasClientContext() {
return clientContext_ != null;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return The clientContext.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getClientContext() {
return clientContext_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.getDefaultInstance() : clientContext_;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder getClientContextOrBuilder() {
return getClientContext();
}
public static final int QUERY_TAG_FIELD_NUMBER = 34;
private volatile java.lang.Object queryTag_;
/**
* string query_tag = 34;
* @return The queryTag.
*/
@java.lang.Override
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
}
}
/**
* string query_tag = 34;
* @return The bytes for queryTag.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JDBC_DOMAIN_FIELD_NUMBER = 35;
private volatile java.lang.Object jdbcDomain_;
/**
* optional string jdbc_domain = 35;
* @return Whether the jdbcDomain field is set.
*/
@java.lang.Override
public boolean hasJdbcDomain() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string jdbc_domain = 35;
* @return The jdbcDomain.
*/
@java.lang.Override
public java.lang.String getJdbcDomain() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jdbcDomain_ = s;
return s;
}
}
/**
* optional string jdbc_domain = 35;
* @return The bytes for jdbcDomain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJdbcDomainBytes() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jdbcDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_PROFILING_FIELD_NUMBER = 36;
private cz.proto.JobProfiling clientProfiling_;
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return Whether the clientProfiling field is set.
*/
@java.lang.Override
public boolean hasClientProfiling() {
return clientProfiling_ != null;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return The clientProfiling.
*/
@java.lang.Override
public cz.proto.JobProfiling getClientProfiling() {
return clientProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : clientProfiling_;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
@java.lang.Override
public cz.proto.JobProfilingOrBuilder getClientProfilingOrBuilder() {
return getClientProfiling();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, virtualCluster_);
}
if (type_ != cz.proto.JobType.SQL_JOB.getNumber()) {
output.writeEnum(2, type_);
}
if (jobId_ != null) {
output.writeMessage(3, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, jobName_);
}
if (account_ != null) {
output.writeMessage(5, getAccount());
}
if (requestMode_ != cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.UNKNOWN.getNumber()) {
output.writeEnum(6, requestMode_);
}
if (hybridPollingTimeout_ != 0) {
output.writeInt32(7, hybridPollingTimeout_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetJobConfig(),
JobConfigDefaultEntryHolder.defaultEntry,
8);
if (jobCase_ == 9) {
output.writeMessage(9, (cz.proto.SQLJob) job_);
}
if (jobTimeoutMs_ != 0L) {
output.writeUInt64(15, jobTimeoutMs_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, userAgent_);
}
if (priority_ != 0) {
output.writeUInt32(31, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 32, priorityString_);
}
if (clientContext_ != null) {
output.writeMessage(33, getClientContext());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 34, queryTag_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, jdbcDomain_);
}
if (clientProfiling_ != null) {
output.writeMessage(36, getClientProfiling());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, virtualCluster_);
}
if (type_ != cz.proto.JobType.SQL_JOB.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_);
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, jobName_);
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getAccount());
}
if (requestMode_ != cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, requestMode_);
}
if (hybridPollingTimeout_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, hybridPollingTimeout_);
}
for (java.util.Map.Entry entry
: internalGetJobConfig().getMap().entrySet()) {
com.google.protobuf.MapEntry
jobConfig__ = JobConfigDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, jobConfig__);
}
if (jobCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (cz.proto.SQLJob) job_);
}
if (jobTimeoutMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(15, jobTimeoutMs_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, userAgent_);
}
if (priority_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(31, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(32, priorityString_);
}
if (clientContext_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(33, getClientContext());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(34, queryTag_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, jdbcDomain_);
}
if (clientProfiling_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(36, getClientProfiling());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc) obj;
if (!getVirtualCluster()
.equals(other.getVirtualCluster())) return false;
if (type_ != other.type_) return false;
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getJobName()
.equals(other.getJobName())) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (requestMode_ != other.requestMode_) return false;
if (getHybridPollingTimeout()
!= other.getHybridPollingTimeout()) return false;
if (!internalGetJobConfig().equals(
other.internalGetJobConfig())) return false;
if (getJobTimeoutMs()
!= other.getJobTimeoutMs()) return false;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (getPriority()
!= other.getPriority()) return false;
if (!getPriorityString()
.equals(other.getPriorityString())) return false;
if (hasClientContext() != other.hasClientContext()) return false;
if (hasClientContext()) {
if (!getClientContext()
.equals(other.getClientContext())) return false;
}
if (!getQueryTag()
.equals(other.getQueryTag())) return false;
if (hasJdbcDomain() != other.hasJdbcDomain()) return false;
if (hasJdbcDomain()) {
if (!getJdbcDomain()
.equals(other.getJdbcDomain())) return false;
}
if (hasClientProfiling() != other.hasClientProfiling()) return false;
if (hasClientProfiling()) {
if (!getClientProfiling()
.equals(other.getClientProfiling())) return false;
}
if (!getJobCase().equals(other.getJobCase())) return false;
switch (jobCase_) {
case 9:
if (!getSqlJob()
.equals(other.getSqlJob())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VIRTUAL_CLUSTER_FIELD_NUMBER;
hash = (53 * hash) + getVirtualCluster().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + JOB_NAME_FIELD_NUMBER;
hash = (53 * hash) + getJobName().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (37 * hash) + REQUEST_MODE_FIELD_NUMBER;
hash = (53 * hash) + requestMode_;
hash = (37 * hash) + HYBRID_POLLING_TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + getHybridPollingTimeout();
if (!internalGetJobConfig().getMap().isEmpty()) {
hash = (37 * hash) + JOB_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + internalGetJobConfig().hashCode();
}
hash = (37 * hash) + JOB_TIMEOUT_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getJobTimeoutMs());
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (37 * hash) + PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getPriority();
hash = (37 * hash) + PRIORITY_STRING_FIELD_NUMBER;
hash = (53 * hash) + getPriorityString().hashCode();
if (hasClientContext()) {
hash = (37 * hash) + CLIENT_CONTEXT_FIELD_NUMBER;
hash = (53 * hash) + getClientContext().hashCode();
}
hash = (37 * hash) + QUERY_TAG_FIELD_NUMBER;
hash = (53 * hash) + getQueryTag().hashCode();
if (hasJdbcDomain()) {
hash = (37 * hash) + JDBC_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getJdbcDomain().hashCode();
}
if (hasClientProfiling()) {
hash = (37 * hash) + CLIENT_PROFILING_FIELD_NUMBER;
hash = (53 * hash) + getClientProfiling().hashCode();
}
switch (jobCase_) {
case 9:
hash = (37 * hash) + SQL_JOB_FIELD_NUMBER;
hash = (53 * hash) + getSqlJob().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobDesc}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobDesc)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 8:
return internalGetJobConfig();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 8:
return internalGetMutableJobConfig();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
virtualCluster_ = "";
type_ = 0;
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
jobName_ = "";
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
requestMode_ = 0;
hybridPollingTimeout_ = 0;
internalGetMutableJobConfig().clear();
jobTimeoutMs_ = 0L;
userAgent_ = "";
priority_ = 0;
priorityString_ = "";
if (clientContextBuilder_ == null) {
clientContext_ = null;
} else {
clientContext_ = null;
clientContextBuilder_ = null;
}
queryTag_ = "";
jdbcDomain_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
if (clientProfilingBuilder_ == null) {
clientProfiling_ = null;
} else {
clientProfiling_ = null;
clientProfilingBuilder_ = null;
}
jobCase_ = 0;
job_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobDesc_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.virtualCluster_ = virtualCluster_;
result.type_ = type_;
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.jobName_ = jobName_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
result.requestMode_ = requestMode_;
result.hybridPollingTimeout_ = hybridPollingTimeout_;
result.jobConfig_ = internalGetJobConfig();
result.jobConfig_.makeImmutable();
if (jobCase_ == 9) {
if (sqlJobBuilder_ == null) {
result.job_ = job_;
} else {
result.job_ = sqlJobBuilder_.build();
}
}
result.jobTimeoutMs_ = jobTimeoutMs_;
result.userAgent_ = userAgent_;
result.priority_ = priority_;
result.priorityString_ = priorityString_;
if (clientContextBuilder_ == null) {
result.clientContext_ = clientContext_;
} else {
result.clientContext_ = clientContextBuilder_.build();
}
result.queryTag_ = queryTag_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.jdbcDomain_ = jdbcDomain_;
if (clientProfilingBuilder_ == null) {
result.clientProfiling_ = clientProfiling_;
} else {
result.clientProfiling_ = clientProfilingBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.jobCase_ = jobCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance()) return this;
if (!other.getVirtualCluster().isEmpty()) {
virtualCluster_ = other.virtualCluster_;
onChanged();
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getJobName().isEmpty()) {
jobName_ = other.jobName_;
onChanged();
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.requestMode_ != 0) {
setRequestModeValue(other.getRequestModeValue());
}
if (other.getHybridPollingTimeout() != 0) {
setHybridPollingTimeout(other.getHybridPollingTimeout());
}
internalGetMutableJobConfig().mergeFrom(
other.internalGetJobConfig());
if (other.getJobTimeoutMs() != 0L) {
setJobTimeoutMs(other.getJobTimeoutMs());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
if (other.getPriority() != 0) {
setPriority(other.getPriority());
}
if (!other.getPriorityString().isEmpty()) {
priorityString_ = other.priorityString_;
onChanged();
}
if (other.hasClientContext()) {
mergeClientContext(other.getClientContext());
}
if (!other.getQueryTag().isEmpty()) {
queryTag_ = other.queryTag_;
onChanged();
}
if (other.hasJdbcDomain()) {
bitField0_ |= 0x00000002;
jdbcDomain_ = other.jdbcDomain_;
onChanged();
}
if (other.hasClientProfiling()) {
mergeClientProfiling(other.getClientProfiling());
}
switch (other.getJobCase()) {
case SQL_JOB: {
mergeSqlJob(other.getSqlJob());
break;
}
case JOB_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int jobCase_ = 0;
private java.lang.Object job_;
public JobCase
getJobCase() {
return JobCase.forNumber(
jobCase_);
}
public Builder clearJob() {
jobCase_ = 0;
job_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object virtualCluster_ = "";
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The virtualCluster.
*/
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return The bytes for virtualCluster.
*/
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @param value The virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualCluster(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualCluster_ = value;
onChanged();
return this;
}
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @return This builder for chaining.
*/
public Builder clearVirtualCluster() {
virtualCluster_ = getDefaultInstance().getVirtualCluster();
onChanged();
return this;
}
/**
*
* optional, use default_vc if not set
*
*
* string virtual_cluster = 1;
* @param value The bytes for virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualCluster_ = value;
onChanged();
return this;
}
private int type_ = 0;
/**
* .cz.proto.JobType type = 2;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.JobType type = 2;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .cz.proto.JobType type = 2;
* @return The type.
*/
@java.lang.Override
public cz.proto.JobType getType() {
@SuppressWarnings("deprecation")
cz.proto.JobType result = cz.proto.JobType.valueOf(type_);
return result == null ? cz.proto.JobType.UNRECOGNIZED : result;
}
/**
* .cz.proto.JobType type = 2;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(cz.proto.JobType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.JobType type = 2;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object jobName_ = "";
/**
* string job_name = 4;
* @return The jobName.
*/
public java.lang.String getJobName() {
java.lang.Object ref = jobName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_name = 4;
* @return The bytes for jobName.
*/
public com.google.protobuf.ByteString
getJobNameBytes() {
java.lang.Object ref = jobName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_name = 4;
* @param value The jobName to set.
* @return This builder for chaining.
*/
public Builder setJobName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobName_ = value;
onChanged();
return this;
}
/**
* string job_name = 4;
* @return This builder for chaining.
*/
public Builder clearJobName() {
jobName_ = getDefaultInstance().getJobName();
onChanged();
return this;
}
/**
* string job_name = 4;
* @param value The bytes for jobName to set.
* @return This builder for chaining.
*/
public Builder setJobNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobName_ = value;
onChanged();
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 5;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 5;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private int requestMode_ = 0;
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The enum numeric value on the wire for requestMode.
*/
@java.lang.Override public int getRequestModeValue() {
return requestMode_;
}
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @param value The enum numeric value on the wire for requestMode to set.
* @return This builder for chaining.
*/
public Builder setRequestModeValue(int value) {
requestMode_ = value;
onChanged();
return this;
}
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return The requestMode.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode getRequestMode() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode result = cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.valueOf(requestMode_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode.UNRECOGNIZED : result;
}
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @param value The requestMode to set.
* @return This builder for chaining.
*/
public Builder setRequestMode(cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestMode value) {
if (value == null) {
throw new NullPointerException();
}
requestMode_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.coordinator.JobRequestMode request_mode = 6;
* @return This builder for chaining.
*/
public Builder clearRequestMode() {
requestMode_ = 0;
onChanged();
return this;
}
private int hybridPollingTimeout_ ;
/**
* int32 hybrid_polling_timeout = 7;
* @return The hybridPollingTimeout.
*/
@java.lang.Override
public int getHybridPollingTimeout() {
return hybridPollingTimeout_;
}
/**
* int32 hybrid_polling_timeout = 7;
* @param value The hybridPollingTimeout to set.
* @return This builder for chaining.
*/
public Builder setHybridPollingTimeout(int value) {
hybridPollingTimeout_ = value;
onChanged();
return this;
}
/**
* int32 hybrid_polling_timeout = 7;
* @return This builder for chaining.
*/
public Builder clearHybridPollingTimeout() {
hybridPollingTimeout_ = 0;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> jobConfig_;
private com.google.protobuf.MapField
internalGetJobConfig() {
if (jobConfig_ == null) {
return com.google.protobuf.MapField.emptyMapField(
JobConfigDefaultEntryHolder.defaultEntry);
}
return jobConfig_;
}
private com.google.protobuf.MapField
internalGetMutableJobConfig() {
onChanged();;
if (jobConfig_ == null) {
jobConfig_ = com.google.protobuf.MapField.newMapField(
JobConfigDefaultEntryHolder.defaultEntry);
}
if (!jobConfig_.isMutable()) {
jobConfig_ = jobConfig_.copy();
}
return jobConfig_;
}
public int getJobConfigCount() {
return internalGetJobConfig().getMap().size();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public boolean containsJobConfig(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetJobConfig().getMap().containsKey(key);
}
/**
* Use {@link #getJobConfigMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getJobConfig() {
return getJobConfigMap();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.util.Map getJobConfigMap() {
return internalGetJobConfig().getMap();
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.lang.String getJobConfigOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetJobConfig().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, string> job_config = 8;
*/
@java.lang.Override
public java.lang.String getJobConfigOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetJobConfig().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearJobConfig() {
internalGetMutableJobConfig().getMutableMap()
.clear();
return this;
}
/**
* map<string, string> job_config = 8;
*/
public Builder removeJobConfig(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableJobConfig().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableJobConfig() {
return internalGetMutableJobConfig().getMutableMap();
}
/**
* map<string, string> job_config = 8;
*/
public Builder putJobConfig(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableJobConfig().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, string> job_config = 8;
*/
public Builder putAllJobConfig(
java.util.Map values) {
internalGetMutableJobConfig().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SQLJob, cz.proto.SQLJob.Builder, cz.proto.SQLJobOrBuilder> sqlJobBuilder_;
/**
* .cz.proto.SQLJob sql_job = 9;
* @return Whether the sqlJob field is set.
*/
@java.lang.Override
public boolean hasSqlJob() {
return jobCase_ == 9;
}
/**
* .cz.proto.SQLJob sql_job = 9;
* @return The sqlJob.
*/
@java.lang.Override
public cz.proto.SQLJob getSqlJob() {
if (sqlJobBuilder_ == null) {
if (jobCase_ == 9) {
return (cz.proto.SQLJob) job_;
}
return cz.proto.SQLJob.getDefaultInstance();
} else {
if (jobCase_ == 9) {
return sqlJobBuilder_.getMessage();
}
return cz.proto.SQLJob.getDefaultInstance();
}
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
public Builder setSqlJob(cz.proto.SQLJob value) {
if (sqlJobBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
job_ = value;
onChanged();
} else {
sqlJobBuilder_.setMessage(value);
}
jobCase_ = 9;
return this;
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
public Builder setSqlJob(
cz.proto.SQLJob.Builder builderForValue) {
if (sqlJobBuilder_ == null) {
job_ = builderForValue.build();
onChanged();
} else {
sqlJobBuilder_.setMessage(builderForValue.build());
}
jobCase_ = 9;
return this;
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
public Builder mergeSqlJob(cz.proto.SQLJob value) {
if (sqlJobBuilder_ == null) {
if (jobCase_ == 9 &&
job_ != cz.proto.SQLJob.getDefaultInstance()) {
job_ = cz.proto.SQLJob.newBuilder((cz.proto.SQLJob) job_)
.mergeFrom(value).buildPartial();
} else {
job_ = value;
}
onChanged();
} else {
if (jobCase_ == 9) {
sqlJobBuilder_.mergeFrom(value);
}
sqlJobBuilder_.setMessage(value);
}
jobCase_ = 9;
return this;
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
public Builder clearSqlJob() {
if (sqlJobBuilder_ == null) {
if (jobCase_ == 9) {
jobCase_ = 0;
job_ = null;
onChanged();
}
} else {
if (jobCase_ == 9) {
jobCase_ = 0;
job_ = null;
}
sqlJobBuilder_.clear();
}
return this;
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
public cz.proto.SQLJob.Builder getSqlJobBuilder() {
return getSqlJobFieldBuilder().getBuilder();
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
@java.lang.Override
public cz.proto.SQLJobOrBuilder getSqlJobOrBuilder() {
if ((jobCase_ == 9) && (sqlJobBuilder_ != null)) {
return sqlJobBuilder_.getMessageOrBuilder();
} else {
if (jobCase_ == 9) {
return (cz.proto.SQLJob) job_;
}
return cz.proto.SQLJob.getDefaultInstance();
}
}
/**
* .cz.proto.SQLJob sql_job = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SQLJob, cz.proto.SQLJob.Builder, cz.proto.SQLJobOrBuilder>
getSqlJobFieldBuilder() {
if (sqlJobBuilder_ == null) {
if (!(jobCase_ == 9)) {
job_ = cz.proto.SQLJob.getDefaultInstance();
}
sqlJobBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SQLJob, cz.proto.SQLJob.Builder, cz.proto.SQLJobOrBuilder>(
(cz.proto.SQLJob) job_,
getParentForChildren(),
isClean());
job_ = null;
}
jobCase_ = 9;
onChanged();;
return sqlJobBuilder_;
}
private long jobTimeoutMs_ ;
/**
* uint64 job_timeout_ms = 15;
* @return The jobTimeoutMs.
*/
@java.lang.Override
public long getJobTimeoutMs() {
return jobTimeoutMs_;
}
/**
* uint64 job_timeout_ms = 15;
* @param value The jobTimeoutMs to set.
* @return This builder for chaining.
*/
public Builder setJobTimeoutMs(long value) {
jobTimeoutMs_ = value;
onChanged();
return this;
}
/**
* uint64 job_timeout_ms = 15;
* @return This builder for chaining.
*/
public Builder clearJobTimeoutMs() {
jobTimeoutMs_ = 0L;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 30;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 30;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 30;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 30;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
private int priority_ ;
/**
* uint32 priority = 31;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
/**
* uint32 priority = 31;
* @param value The priority to set.
* @return This builder for chaining.
*/
public Builder setPriority(int value) {
priority_ = value;
onChanged();
return this;
}
/**
* uint32 priority = 31;
* @return This builder for chaining.
*/
public Builder clearPriority() {
priority_ = 0;
onChanged();
return this;
}
private java.lang.Object priorityString_ = "";
/**
* string priority_string = 32;
* @return The priorityString.
*/
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string priority_string = 32;
* @return The bytes for priorityString.
*/
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string priority_string = 32;
* @param value The priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
priorityString_ = value;
onChanged();
return this;
}
/**
* string priority_string = 32;
* @return This builder for chaining.
*/
public Builder clearPriorityString() {
priorityString_ = getDefaultInstance().getPriorityString();
onChanged();
return this;
}
/**
* string priority_string = 32;
* @param value The bytes for priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
priorityString_ = value;
onChanged();
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo clientContext_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder> clientContextBuilder_;
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return Whether the clientContext field is set.
*/
public boolean hasClientContext() {
return clientContextBuilder_ != null || clientContext_ != null;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
* @return The clientContext.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo getClientContext() {
if (clientContextBuilder_ == null) {
return clientContext_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.getDefaultInstance() : clientContext_;
} else {
return clientContextBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public Builder setClientContext(cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo value) {
if (clientContextBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientContext_ = value;
onChanged();
} else {
clientContextBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public Builder setClientContext(
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder builderForValue) {
if (clientContextBuilder_ == null) {
clientContext_ = builderForValue.build();
onChanged();
} else {
clientContextBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public Builder mergeClientContext(cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo value) {
if (clientContextBuilder_ == null) {
if (clientContext_ != null) {
clientContext_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.newBuilder(clientContext_).mergeFrom(value).buildPartial();
} else {
clientContext_ = value;
}
onChanged();
} else {
clientContextBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public Builder clearClientContext() {
if (clientContextBuilder_ == null) {
clientContext_ = null;
onChanged();
} else {
clientContext_ = null;
clientContextBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder getClientContextBuilder() {
onChanged();
return getClientContextFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder getClientContextOrBuilder() {
if (clientContextBuilder_ != null) {
return clientContextBuilder_.getMessageOrBuilder();
} else {
return clientContext_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.getDefaultInstance() : clientContext_;
}
}
/**
* .cz.proto.coordinator.ClientContextInfo client_context = 33;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder>
getClientContextFieldBuilder() {
if (clientContextBuilder_ == null) {
clientContextBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ClientContextInfoOrBuilder>(
getClientContext(),
getParentForChildren(),
isClean());
clientContext_ = null;
}
return clientContextBuilder_;
}
private java.lang.Object queryTag_ = "";
/**
* string query_tag = 34;
* @return The queryTag.
*/
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query_tag = 34;
* @return The bytes for queryTag.
*/
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query_tag = 34;
* @param value The queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
queryTag_ = value;
onChanged();
return this;
}
/**
* string query_tag = 34;
* @return This builder for chaining.
*/
public Builder clearQueryTag() {
queryTag_ = getDefaultInstance().getQueryTag();
onChanged();
return this;
}
/**
* string query_tag = 34;
* @param value The bytes for queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
queryTag_ = value;
onChanged();
return this;
}
private java.lang.Object jdbcDomain_ = "";
/**
* optional string jdbc_domain = 35;
* @return Whether the jdbcDomain field is set.
*/
public boolean hasJdbcDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string jdbc_domain = 35;
* @return The jdbcDomain.
*/
public java.lang.String getJdbcDomain() {
java.lang.Object ref = jdbcDomain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jdbcDomain_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string jdbc_domain = 35;
* @return The bytes for jdbcDomain.
*/
public com.google.protobuf.ByteString
getJdbcDomainBytes() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jdbcDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string jdbc_domain = 35;
* @param value The jdbcDomain to set.
* @return This builder for chaining.
*/
public Builder setJdbcDomain(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
jdbcDomain_ = value;
onChanged();
return this;
}
/**
* optional string jdbc_domain = 35;
* @return This builder for chaining.
*/
public Builder clearJdbcDomain() {
bitField0_ = (bitField0_ & ~0x00000002);
jdbcDomain_ = getDefaultInstance().getJdbcDomain();
onChanged();
return this;
}
/**
* optional string jdbc_domain = 35;
* @param value The bytes for jdbcDomain to set.
* @return This builder for chaining.
*/
public Builder setJdbcDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000002;
jdbcDomain_ = value;
onChanged();
return this;
}
private cz.proto.JobProfiling clientProfiling_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder> clientProfilingBuilder_;
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return Whether the clientProfiling field is set.
*/
public boolean hasClientProfiling() {
return clientProfilingBuilder_ != null || clientProfiling_ != null;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
* @return The clientProfiling.
*/
public cz.proto.JobProfiling getClientProfiling() {
if (clientProfilingBuilder_ == null) {
return clientProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : clientProfiling_;
} else {
return clientProfilingBuilder_.getMessage();
}
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public Builder setClientProfiling(cz.proto.JobProfiling value) {
if (clientProfilingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clientProfiling_ = value;
onChanged();
} else {
clientProfilingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public Builder setClientProfiling(
cz.proto.JobProfiling.Builder builderForValue) {
if (clientProfilingBuilder_ == null) {
clientProfiling_ = builderForValue.build();
onChanged();
} else {
clientProfilingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public Builder mergeClientProfiling(cz.proto.JobProfiling value) {
if (clientProfilingBuilder_ == null) {
if (clientProfiling_ != null) {
clientProfiling_ =
cz.proto.JobProfiling.newBuilder(clientProfiling_).mergeFrom(value).buildPartial();
} else {
clientProfiling_ = value;
}
onChanged();
} else {
clientProfilingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public Builder clearClientProfiling() {
if (clientProfilingBuilder_ == null) {
clientProfiling_ = null;
onChanged();
} else {
clientProfiling_ = null;
clientProfilingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public cz.proto.JobProfiling.Builder getClientProfilingBuilder() {
onChanged();
return getClientProfilingFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
public cz.proto.JobProfilingOrBuilder getClientProfilingOrBuilder() {
if (clientProfilingBuilder_ != null) {
return clientProfilingBuilder_.getMessageOrBuilder();
} else {
return clientProfiling_ == null ?
cz.proto.JobProfiling.getDefaultInstance() : clientProfiling_;
}
}
/**
* .cz.proto.JobProfiling client_profiling = 36;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>
getClientProfilingFieldBuilder() {
if (clientProfilingBuilder_ == null) {
clientProfilingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>(
getClientProfiling(),
getParentForChildren(),
isClean());
clientProfiling_ = null;
}
return clientProfilingBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobDesc)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobDesc)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobDesc parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobDesc(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobStatusOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobStatus)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string state = 2;
* @return The state.
*/
java.lang.String getState();
/**
* string state = 2;
* @return The bytes for state.
*/
com.google.protobuf.ByteString
getStateBytes();
/**
* string message = 3;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 3;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* int64 submit_time = 4;
* @return The submitTime.
*/
long getSubmitTime();
/**
* int64 start_time = 5;
* @return The startTime.
*/
long getStartTime();
/**
* int64 end_time = 6;
* @return The endTime.
*/
long getEndTime();
/**
* int64 pending_time = 7;
* @return The pendingTime.
*/
long getPendingTime();
/**
* int64 running_time = 8;
* @return The runningTime.
*/
long getRunningTime();
/**
* .cz.proto.JobStatus status = 9;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* .cz.proto.JobStatus status = 9;
* @return The status.
*/
cz.proto.JobStatus getStatus();
/**
* repeated string execution_log = 10;
* @return A list containing the executionLog.
*/
java.util.List
getExecutionLogList();
/**
* repeated string execution_log = 10;
* @return The count of executionLog.
*/
int getExecutionLogCount();
/**
* repeated string execution_log = 10;
* @param index The index of the element to return.
* @return The executionLog at the given index.
*/
java.lang.String getExecutionLog(int index);
/**
* repeated string execution_log = 10;
* @param index The index of the value to return.
* @return The bytes of the executionLog at the given index.
*/
com.google.protobuf.ByteString
getExecutionLogBytes(int index);
/**
* string error_code = 11;
* @return The errorCode.
*/
java.lang.String getErrorCode();
/**
* string error_code = 11;
* @return The bytes for errorCode.
*/
com.google.protobuf.ByteString
getErrorCodeBytes();
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return Whether the jobProfiling field is set.
*/
boolean hasJobProfiling();
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return The jobProfiling.
*/
cz.proto.JobProfiling getJobProfiling();
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.JobStatus}
*/
public static final class JobStatus extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobStatus)
JobStatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobStatus.newBuilder() to construct.
private JobStatus(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobStatus() {
state_ = "";
message_ = "";
status_ = 0;
executionLog_ = com.google.protobuf.LazyStringArrayList.EMPTY;
errorCode_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobStatus();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobStatus(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
state_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 32: {
submitTime_ = input.readInt64();
break;
}
case 40: {
startTime_ = input.readInt64();
break;
}
case 48: {
endTime_ = input.readInt64();
break;
}
case 56: {
pendingTime_ = input.readInt64();
break;
}
case 64: {
runningTime_ = input.readInt64();
break;
}
case 72: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
executionLog_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
executionLog_.add(s);
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
errorCode_ = s;
break;
}
case 98: {
cz.proto.JobProfiling.Builder subBuilder = null;
if (jobProfiling_ != null) {
subBuilder = jobProfiling_.toBuilder();
}
jobProfiling_ = input.readMessage(cz.proto.JobProfiling.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobProfiling_);
jobProfiling_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
executionLog_ = executionLog_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder.class);
}
public static final int JOB_ID_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int STATE_FIELD_NUMBER = 2;
private volatile java.lang.Object state_;
/**
* string state = 2;
* @return The state.
*/
@java.lang.Override
public java.lang.String getState() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
}
}
/**
* string state = 2;
* @return The bytes for state.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MESSAGE_FIELD_NUMBER = 3;
private volatile java.lang.Object message_;
/**
* string message = 3;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 3;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBMIT_TIME_FIELD_NUMBER = 4;
private long submitTime_;
/**
* int64 submit_time = 4;
* @return The submitTime.
*/
@java.lang.Override
public long getSubmitTime() {
return submitTime_;
}
public static final int START_TIME_FIELD_NUMBER = 5;
private long startTime_;
/**
* int64 start_time = 5;
* @return The startTime.
*/
@java.lang.Override
public long getStartTime() {
return startTime_;
}
public static final int END_TIME_FIELD_NUMBER = 6;
private long endTime_;
/**
* int64 end_time = 6;
* @return The endTime.
*/
@java.lang.Override
public long getEndTime() {
return endTime_;
}
public static final int PENDING_TIME_FIELD_NUMBER = 7;
private long pendingTime_;
/**
* int64 pending_time = 7;
* @return The pendingTime.
*/
@java.lang.Override
public long getPendingTime() {
return pendingTime_;
}
public static final int RUNNING_TIME_FIELD_NUMBER = 8;
private long runningTime_;
/**
* int64 running_time = 8;
* @return The runningTime.
*/
@java.lang.Override
public long getRunningTime() {
return runningTime_;
}
public static final int STATUS_FIELD_NUMBER = 9;
private int status_;
/**
* .cz.proto.JobStatus status = 9;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .cz.proto.JobStatus status = 9;
* @return The status.
*/
@java.lang.Override public cz.proto.JobStatus getStatus() {
@SuppressWarnings("deprecation")
cz.proto.JobStatus result = cz.proto.JobStatus.valueOf(status_);
return result == null ? cz.proto.JobStatus.UNRECOGNIZED : result;
}
public static final int EXECUTION_LOG_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList executionLog_;
/**
* repeated string execution_log = 10;
* @return A list containing the executionLog.
*/
public com.google.protobuf.ProtocolStringList
getExecutionLogList() {
return executionLog_;
}
/**
* repeated string execution_log = 10;
* @return The count of executionLog.
*/
public int getExecutionLogCount() {
return executionLog_.size();
}
/**
* repeated string execution_log = 10;
* @param index The index of the element to return.
* @return The executionLog at the given index.
*/
public java.lang.String getExecutionLog(int index) {
return executionLog_.get(index);
}
/**
* repeated string execution_log = 10;
* @param index The index of the value to return.
* @return The bytes of the executionLog at the given index.
*/
public com.google.protobuf.ByteString
getExecutionLogBytes(int index) {
return executionLog_.getByteString(index);
}
public static final int ERROR_CODE_FIELD_NUMBER = 11;
private volatile java.lang.Object errorCode_;
/**
* string error_code = 11;
* @return The errorCode.
*/
@java.lang.Override
public java.lang.String getErrorCode() {
java.lang.Object ref = errorCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorCode_ = s;
return s;
}
}
/**
* string error_code = 11;
* @return The bytes for errorCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorCodeBytes() {
java.lang.Object ref = errorCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_PROFILING_FIELD_NUMBER = 12;
private cz.proto.JobProfiling jobProfiling_;
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return Whether the jobProfiling field is set.
*/
@java.lang.Override
public boolean hasJobProfiling() {
return jobProfiling_ != null;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return The jobProfiling.
*/
@java.lang.Override
public cz.proto.JobProfiling getJobProfiling() {
return jobProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
@java.lang.Override
public cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder() {
return getJobProfiling();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (jobId_ != null) {
output.writeMessage(1, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(state_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, message_);
}
if (submitTime_ != 0L) {
output.writeInt64(4, submitTime_);
}
if (startTime_ != 0L) {
output.writeInt64(5, startTime_);
}
if (endTime_ != 0L) {
output.writeInt64(6, endTime_);
}
if (pendingTime_ != 0L) {
output.writeInt64(7, pendingTime_);
}
if (runningTime_ != 0L) {
output.writeInt64(8, runningTime_);
}
if (status_ != cz.proto.JobStatus.SETUP.getNumber()) {
output.writeEnum(9, status_);
}
for (int i = 0; i < executionLog_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, executionLog_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(errorCode_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, errorCode_);
}
if (jobProfiling_ != null) {
output.writeMessage(12, getJobProfiling());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(state_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, message_);
}
if (submitTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, submitTime_);
}
if (startTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, startTime_);
}
if (endTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, endTime_);
}
if (pendingTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, pendingTime_);
}
if (runningTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, runningTime_);
}
if (status_ != cz.proto.JobStatus.SETUP.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, status_);
}
{
int dataSize = 0;
for (int i = 0; i < executionLog_.size(); i++) {
dataSize += computeStringSizeNoTag(executionLog_.getRaw(i));
}
size += dataSize;
size += 1 * getExecutionLogList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(errorCode_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, errorCode_);
}
if (jobProfiling_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getJobProfiling());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus) obj;
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getState()
.equals(other.getState())) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (getSubmitTime()
!= other.getSubmitTime()) return false;
if (getStartTime()
!= other.getStartTime()) return false;
if (getEndTime()
!= other.getEndTime()) return false;
if (getPendingTime()
!= other.getPendingTime()) return false;
if (getRunningTime()
!= other.getRunningTime()) return false;
if (status_ != other.status_) return false;
if (!getExecutionLogList()
.equals(other.getExecutionLogList())) return false;
if (!getErrorCode()
.equals(other.getErrorCode())) return false;
if (hasJobProfiling() != other.hasJobProfiling()) return false;
if (hasJobProfiling()) {
if (!getJobProfiling()
.equals(other.getJobProfiling())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + getState().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (37 * hash) + SUBMIT_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSubmitTime());
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartTime());
hash = (37 * hash) + END_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEndTime());
hash = (37 * hash) + PENDING_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPendingTime());
hash = (37 * hash) + RUNNING_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRunningTime());
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (getExecutionLogCount() > 0) {
hash = (37 * hash) + EXECUTION_LOG_FIELD_NUMBER;
hash = (53 * hash) + getExecutionLogList().hashCode();
}
hash = (37 * hash) + ERROR_CODE_FIELD_NUMBER;
hash = (53 * hash) + getErrorCode().hashCode();
if (hasJobProfiling()) {
hash = (37 * hash) + JOB_PROFILING_FIELD_NUMBER;
hash = (53 * hash) + getJobProfiling().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobStatus}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobStatus)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
state_ = "";
message_ = "";
submitTime_ = 0L;
startTime_ = 0L;
endTime_ = 0L;
pendingTime_ = 0L;
runningTime_ = 0L;
status_ = 0;
executionLog_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
errorCode_ = "";
if (jobProfilingBuilder_ == null) {
jobProfiling_ = null;
} else {
jobProfiling_ = null;
jobProfilingBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobStatus_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus(this);
int from_bitField0_ = bitField0_;
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.state_ = state_;
result.message_ = message_;
result.submitTime_ = submitTime_;
result.startTime_ = startTime_;
result.endTime_ = endTime_;
result.pendingTime_ = pendingTime_;
result.runningTime_ = runningTime_;
result.status_ = status_;
if (((bitField0_ & 0x00000001) != 0)) {
executionLog_ = executionLog_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.executionLog_ = executionLog_;
result.errorCode_ = errorCode_;
if (jobProfilingBuilder_ == null) {
result.jobProfiling_ = jobProfiling_;
} else {
result.jobProfiling_ = jobProfilingBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance()) return this;
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getState().isEmpty()) {
state_ = other.state_;
onChanged();
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (other.getSubmitTime() != 0L) {
setSubmitTime(other.getSubmitTime());
}
if (other.getStartTime() != 0L) {
setStartTime(other.getStartTime());
}
if (other.getEndTime() != 0L) {
setEndTime(other.getEndTime());
}
if (other.getPendingTime() != 0L) {
setPendingTime(other.getPendingTime());
}
if (other.getRunningTime() != 0L) {
setRunningTime(other.getRunningTime());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.executionLog_.isEmpty()) {
if (executionLog_.isEmpty()) {
executionLog_ = other.executionLog_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureExecutionLogIsMutable();
executionLog_.addAll(other.executionLog_);
}
onChanged();
}
if (!other.getErrorCode().isEmpty()) {
errorCode_ = other.errorCode_;
onChanged();
}
if (other.hasJobProfiling()) {
mergeJobProfiling(other.getJobProfiling());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object state_ = "";
/**
* string state = 2;
* @return The state.
*/
public java.lang.String getState() {
java.lang.Object ref = state_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
state_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string state = 2;
* @return The bytes for state.
*/
public com.google.protobuf.ByteString
getStateBytes() {
java.lang.Object ref = state_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
state_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string state = 2;
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
state_ = value;
onChanged();
return this;
}
/**
* string state = 2;
* @return This builder for chaining.
*/
public Builder clearState() {
state_ = getDefaultInstance().getState();
onChanged();
return this;
}
/**
* string state = 2;
* @param value The bytes for state to set.
* @return This builder for chaining.
*/
public Builder setStateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
state_ = value;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 3;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 3;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 3;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 3;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 3;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private long submitTime_ ;
/**
* int64 submit_time = 4;
* @return The submitTime.
*/
@java.lang.Override
public long getSubmitTime() {
return submitTime_;
}
/**
* int64 submit_time = 4;
* @param value The submitTime to set.
* @return This builder for chaining.
*/
public Builder setSubmitTime(long value) {
submitTime_ = value;
onChanged();
return this;
}
/**
* int64 submit_time = 4;
* @return This builder for chaining.
*/
public Builder clearSubmitTime() {
submitTime_ = 0L;
onChanged();
return this;
}
private long startTime_ ;
/**
* int64 start_time = 5;
* @return The startTime.
*/
@java.lang.Override
public long getStartTime() {
return startTime_;
}
/**
* int64 start_time = 5;
* @param value The startTime to set.
* @return This builder for chaining.
*/
public Builder setStartTime(long value) {
startTime_ = value;
onChanged();
return this;
}
/**
* int64 start_time = 5;
* @return This builder for chaining.
*/
public Builder clearStartTime() {
startTime_ = 0L;
onChanged();
return this;
}
private long endTime_ ;
/**
* int64 end_time = 6;
* @return The endTime.
*/
@java.lang.Override
public long getEndTime() {
return endTime_;
}
/**
* int64 end_time = 6;
* @param value The endTime to set.
* @return This builder for chaining.
*/
public Builder setEndTime(long value) {
endTime_ = value;
onChanged();
return this;
}
/**
* int64 end_time = 6;
* @return This builder for chaining.
*/
public Builder clearEndTime() {
endTime_ = 0L;
onChanged();
return this;
}
private long pendingTime_ ;
/**
* int64 pending_time = 7;
* @return The pendingTime.
*/
@java.lang.Override
public long getPendingTime() {
return pendingTime_;
}
/**
* int64 pending_time = 7;
* @param value The pendingTime to set.
* @return This builder for chaining.
*/
public Builder setPendingTime(long value) {
pendingTime_ = value;
onChanged();
return this;
}
/**
* int64 pending_time = 7;
* @return This builder for chaining.
*/
public Builder clearPendingTime() {
pendingTime_ = 0L;
onChanged();
return this;
}
private long runningTime_ ;
/**
* int64 running_time = 8;
* @return The runningTime.
*/
@java.lang.Override
public long getRunningTime() {
return runningTime_;
}
/**
* int64 running_time = 8;
* @param value The runningTime to set.
* @return This builder for chaining.
*/
public Builder setRunningTime(long value) {
runningTime_ = value;
onChanged();
return this;
}
/**
* int64 running_time = 8;
* @return This builder for chaining.
*/
public Builder clearRunningTime() {
runningTime_ = 0L;
onChanged();
return this;
}
private int status_ = 0;
/**
* .cz.proto.JobStatus status = 9;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .cz.proto.JobStatus status = 9;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
* .cz.proto.JobStatus status = 9;
* @return The status.
*/
@java.lang.Override
public cz.proto.JobStatus getStatus() {
@SuppressWarnings("deprecation")
cz.proto.JobStatus result = cz.proto.JobStatus.valueOf(status_);
return result == null ? cz.proto.JobStatus.UNRECOGNIZED : result;
}
/**
* .cz.proto.JobStatus status = 9;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(cz.proto.JobStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.JobStatus status = 9;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList executionLog_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureExecutionLogIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
executionLog_ = new com.google.protobuf.LazyStringArrayList(executionLog_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string execution_log = 10;
* @return A list containing the executionLog.
*/
public com.google.protobuf.ProtocolStringList
getExecutionLogList() {
return executionLog_.getUnmodifiableView();
}
/**
* repeated string execution_log = 10;
* @return The count of executionLog.
*/
public int getExecutionLogCount() {
return executionLog_.size();
}
/**
* repeated string execution_log = 10;
* @param index The index of the element to return.
* @return The executionLog at the given index.
*/
public java.lang.String getExecutionLog(int index) {
return executionLog_.get(index);
}
/**
* repeated string execution_log = 10;
* @param index The index of the value to return.
* @return The bytes of the executionLog at the given index.
*/
public com.google.protobuf.ByteString
getExecutionLogBytes(int index) {
return executionLog_.getByteString(index);
}
/**
* repeated string execution_log = 10;
* @param index The index to set the value at.
* @param value The executionLog to set.
* @return This builder for chaining.
*/
public Builder setExecutionLog(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureExecutionLogIsMutable();
executionLog_.set(index, value);
onChanged();
return this;
}
/**
* repeated string execution_log = 10;
* @param value The executionLog to add.
* @return This builder for chaining.
*/
public Builder addExecutionLog(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureExecutionLogIsMutable();
executionLog_.add(value);
onChanged();
return this;
}
/**
* repeated string execution_log = 10;
* @param values The executionLog to add.
* @return This builder for chaining.
*/
public Builder addAllExecutionLog(
java.lang.Iterable values) {
ensureExecutionLogIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, executionLog_);
onChanged();
return this;
}
/**
* repeated string execution_log = 10;
* @return This builder for chaining.
*/
public Builder clearExecutionLog() {
executionLog_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string execution_log = 10;
* @param value The bytes of the executionLog to add.
* @return This builder for chaining.
*/
public Builder addExecutionLogBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureExecutionLogIsMutable();
executionLog_.add(value);
onChanged();
return this;
}
private java.lang.Object errorCode_ = "";
/**
* string error_code = 11;
* @return The errorCode.
*/
public java.lang.String getErrorCode() {
java.lang.Object ref = errorCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string error_code = 11;
* @return The bytes for errorCode.
*/
public com.google.protobuf.ByteString
getErrorCodeBytes() {
java.lang.Object ref = errorCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string error_code = 11;
* @param value The errorCode to set.
* @return This builder for chaining.
*/
public Builder setErrorCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorCode_ = value;
onChanged();
return this;
}
/**
* string error_code = 11;
* @return This builder for chaining.
*/
public Builder clearErrorCode() {
errorCode_ = getDefaultInstance().getErrorCode();
onChanged();
return this;
}
/**
* string error_code = 11;
* @param value The bytes for errorCode to set.
* @return This builder for chaining.
*/
public Builder setErrorCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorCode_ = value;
onChanged();
return this;
}
private cz.proto.JobProfiling jobProfiling_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder> jobProfilingBuilder_;
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return Whether the jobProfiling field is set.
*/
public boolean hasJobProfiling() {
return jobProfilingBuilder_ != null || jobProfiling_ != null;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
* @return The jobProfiling.
*/
public cz.proto.JobProfiling getJobProfiling() {
if (jobProfilingBuilder_ == null) {
return jobProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
} else {
return jobProfilingBuilder_.getMessage();
}
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public Builder setJobProfiling(cz.proto.JobProfiling value) {
if (jobProfilingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobProfiling_ = value;
onChanged();
} else {
jobProfilingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public Builder setJobProfiling(
cz.proto.JobProfiling.Builder builderForValue) {
if (jobProfilingBuilder_ == null) {
jobProfiling_ = builderForValue.build();
onChanged();
} else {
jobProfilingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public Builder mergeJobProfiling(cz.proto.JobProfiling value) {
if (jobProfilingBuilder_ == null) {
if (jobProfiling_ != null) {
jobProfiling_ =
cz.proto.JobProfiling.newBuilder(jobProfiling_).mergeFrom(value).buildPartial();
} else {
jobProfiling_ = value;
}
onChanged();
} else {
jobProfilingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public Builder clearJobProfiling() {
if (jobProfilingBuilder_ == null) {
jobProfiling_ = null;
onChanged();
} else {
jobProfiling_ = null;
jobProfilingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public cz.proto.JobProfiling.Builder getJobProfilingBuilder() {
onChanged();
return getJobProfilingFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
public cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder() {
if (jobProfilingBuilder_ != null) {
return jobProfilingBuilder_.getMessageOrBuilder();
} else {
return jobProfiling_ == null ?
cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
}
}
/**
* .cz.proto.JobProfiling job_profiling = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>
getJobProfilingFieldBuilder() {
if (jobProfilingBuilder_ == null) {
jobProfilingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>(
getJobProfiling(),
getParentForChildren(),
isClean());
jobProfiling_ = null;
}
return jobProfilingBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobStatus)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobStatus)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobStatus(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobResultDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobResultData)
com.google.protobuf.MessageOrBuilder {
/**
* repeated bytes data = 1;
* @return A list containing the data.
*/
java.util.List getDataList();
/**
* repeated bytes data = 1;
* @return The count of data.
*/
int getDataCount();
/**
* repeated bytes data = 1;
* @param index The index of the element to return.
* @return The data at the given index.
*/
com.google.protobuf.ByteString getData(int index);
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultData}
*/
public static final class JobResultData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobResultData)
JobResultDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobResultData.newBuilder() to construct.
private JobResultData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobResultData() {
data_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobResultData();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobResultData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
data_.add(input.readBytes());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = java.util.Collections.unmodifiableList(data_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder.class);
}
public static final int DATA_FIELD_NUMBER = 1;
private java.util.List data_;
/**
* repeated bytes data = 1;
* @return A list containing the data.
*/
@java.lang.Override
public java.util.List
getDataList() {
return data_;
}
/**
* repeated bytes data = 1;
* @return The count of data.
*/
public int getDataCount() {
return data_.size();
}
/**
* repeated bytes data = 1;
* @param index The index of the element to return.
* @return The data at the given index.
*/
public com.google.protobuf.ByteString getData(int index) {
return data_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < data_.size(); i++) {
output.writeBytes(1, data_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < data_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(data_.get(i));
}
size += dataSize;
size += 1 * getDataList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData) obj;
if (!getDataList()
.equals(other.getDataList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getDataCount() > 0) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getDataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobResultData)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
data_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultData_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
data_ = java.util.Collections.unmodifiableList(data_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.data_ = data_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.getDefaultInstance()) return this;
if (!other.data_.isEmpty()) {
if (data_.isEmpty()) {
data_ = other.data_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDataIsMutable();
data_.addAll(other.data_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List data_ = java.util.Collections.emptyList();
private void ensureDataIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
data_ = new java.util.ArrayList(data_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes data = 1;
* @return A list containing the data.
*/
public java.util.List
getDataList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(data_) : data_;
}
/**
* repeated bytes data = 1;
* @return The count of data.
*/
public int getDataCount() {
return data_.size();
}
/**
* repeated bytes data = 1;
* @param index The index of the element to return.
* @return The data at the given index.
*/
public com.google.protobuf.ByteString getData(int index) {
return data_.get(index);
}
/**
* repeated bytes data = 1;
* @param index The index to set the value at.
* @param value The data to set.
* @return This builder for chaining.
*/
public Builder setData(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDataIsMutable();
data_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes data = 1;
* @param value The data to add.
* @return This builder for chaining.
*/
public Builder addData(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureDataIsMutable();
data_.add(value);
onChanged();
return this;
}
/**
* repeated bytes data = 1;
* @param values The data to add.
* @return This builder for chaining.
*/
public Builder addAllData(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureDataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, data_);
onChanged();
return this;
}
/**
* repeated bytes data = 1;
* @return This builder for chaining.
*/
public Builder clearData() {
data_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobResultData)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobResultData)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobResultData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobResultData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LocationFileInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.LocationFileInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string file_path = 1;
* @return The filePath.
*/
java.lang.String getFilePath();
/**
* string file_path = 1;
* @return The bytes for filePath.
*/
com.google.protobuf.ByteString
getFilePathBytes();
/**
* int64 file_size = 2;
* @return The fileSize.
*/
long getFileSize();
}
/**
* Protobuf type {@code cz.proto.coordinator.LocationFileInfo}
*/
public static final class LocationFileInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.LocationFileInfo)
LocationFileInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocationFileInfo.newBuilder() to construct.
private LocationFileInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocationFileInfo() {
filePath_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LocationFileInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocationFileInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
filePath_ = s;
break;
}
case 16: {
fileSize_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_LocationFileInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_LocationFileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.class, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder.class);
}
public static final int FILE_PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object filePath_;
/**
* string file_path = 1;
* @return The filePath.
*/
@java.lang.Override
public java.lang.String getFilePath() {
java.lang.Object ref = filePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filePath_ = s;
return s;
}
}
/**
* string file_path = 1;
* @return The bytes for filePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFilePathBytes() {
java.lang.Object ref = filePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILE_SIZE_FIELD_NUMBER = 2;
private long fileSize_;
/**
* int64 file_size = 2;
* @return The fileSize.
*/
@java.lang.Override
public long getFileSize() {
return fileSize_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filePath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, filePath_);
}
if (fileSize_ != 0L) {
output.writeInt64(2, fileSize_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filePath_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, filePath_);
}
if (fileSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, fileSize_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo other = (cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo) obj;
if (!getFilePath()
.equals(other.getFilePath())) return false;
if (getFileSize()
!= other.getFileSize()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getFilePath().hashCode();
hash = (37 * hash) + FILE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFileSize());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.LocationFileInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.LocationFileInfo)
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_LocationFileInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_LocationFileInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.class, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
filePath_ = "";
fileSize_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_LocationFileInfo_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo result = new cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo(this);
result.filePath_ = filePath_;
result.fileSize_ = fileSize_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.getDefaultInstance()) return this;
if (!other.getFilePath().isEmpty()) {
filePath_ = other.filePath_;
onChanged();
}
if (other.getFileSize() != 0L) {
setFileSize(other.getFileSize());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object filePath_ = "";
/**
* string file_path = 1;
* @return The filePath.
*/
public java.lang.String getFilePath() {
java.lang.Object ref = filePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string file_path = 1;
* @return The bytes for filePath.
*/
public com.google.protobuf.ByteString
getFilePathBytes() {
java.lang.Object ref = filePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string file_path = 1;
* @param value The filePath to set.
* @return This builder for chaining.
*/
public Builder setFilePath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
filePath_ = value;
onChanged();
return this;
}
/**
* string file_path = 1;
* @return This builder for chaining.
*/
public Builder clearFilePath() {
filePath_ = getDefaultInstance().getFilePath();
onChanged();
return this;
}
/**
* string file_path = 1;
* @param value The bytes for filePath to set.
* @return This builder for chaining.
*/
public Builder setFilePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
filePath_ = value;
onChanged();
return this;
}
private long fileSize_ ;
/**
* int64 file_size = 2;
* @return The fileSize.
*/
@java.lang.Override
public long getFileSize() {
return fileSize_;
}
/**
* int64 file_size = 2;
* @param value The fileSize to set.
* @return This builder for chaining.
*/
public Builder setFileSize(long value) {
fileSize_ = value;
onChanged();
return this;
}
/**
* int64 file_size = 2;
* @return This builder for chaining.
*/
public Builder clearFileSize() {
fileSize_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.LocationFileInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.LocationFileInfo)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocationFileInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocationFileInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobResultLocationOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobResultLocation)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string location = 1;
* @return A list containing the location.
*/
java.util.List
getLocationList();
/**
* repeated string location = 1;
* @return The count of location.
*/
int getLocationCount();
/**
* repeated string location = 1;
* @param index The index of the element to return.
* @return The location at the given index.
*/
java.lang.String getLocation(int index);
/**
* repeated string location = 1;
* @param index The index of the value to return.
* @return The bytes of the location at the given index.
*/
com.google.protobuf.ByteString
getLocationBytes(int index);
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The enum numeric value on the wire for fileSystem.
*/
int getFileSystemValue();
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The fileSystem.
*/
cz.proto.FileSystem.FileSystemType getFileSystem();
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
java.lang.String getStsAkId();
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
com.google.protobuf.ByteString
getStsAkIdBytes();
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
java.lang.String getStsAkSecret();
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
com.google.protobuf.ByteString
getStsAkSecretBytes();
/**
* string sts_token = 5;
* @return The stsToken.
*/
java.lang.String getStsToken();
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
com.google.protobuf.ByteString
getStsTokenBytes();
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
java.lang.String getOssEndpoint();
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
com.google.protobuf.ByteString
getOssEndpointBytes();
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
java.lang.String getOssInternalEndpoint();
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
com.google.protobuf.ByteString
getOssInternalEndpointBytes();
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
java.util.List
getLocationFilesList();
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getLocationFiles(int index);
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
int getLocationFilesCount();
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder>
getLocationFilesOrBuilderList();
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder getLocationFilesOrBuilder(
int index);
/**
* string object_storage_region = 9;
* @return The objectStorageRegion.
*/
java.lang.String getObjectStorageRegion();
/**
* string object_storage_region = 9;
* @return The bytes for objectStorageRegion.
*/
com.google.protobuf.ByteString
getObjectStorageRegionBytes();
/**
* repeated string presigned_urls = 10;
* @return A list containing the presignedUrls.
*/
java.util.List
getPresignedUrlsList();
/**
* repeated string presigned_urls = 10;
* @return The count of presignedUrls.
*/
int getPresignedUrlsCount();
/**
* repeated string presigned_urls = 10;
* @param index The index of the element to return.
* @return The presignedUrls at the given index.
*/
java.lang.String getPresignedUrls(int index);
/**
* repeated string presigned_urls = 10;
* @param index The index of the value to return.
* @return The bytes of the presignedUrls at the given index.
*/
com.google.protobuf.ByteString
getPresignedUrlsBytes(int index);
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultLocation}
*/
public static final class JobResultLocation extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobResultLocation)
JobResultLocationOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobResultLocation.newBuilder() to construct.
private JobResultLocation(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobResultLocation() {
location_ = com.google.protobuf.LazyStringArrayList.EMPTY;
fileSystem_ = 0;
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
ossEndpoint_ = "";
ossInternalEndpoint_ = "";
locationFiles_ = java.util.Collections.emptyList();
objectStorageRegion_ = "";
presignedUrls_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobResultLocation();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobResultLocation(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
location_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
location_.add(s);
break;
}
case 16: {
int rawValue = input.readEnum();
fileSystem_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
stsAkId_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stsAkSecret_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
stsToken_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
ossEndpoint_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
ossInternalEndpoint_ = s;
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
locationFiles_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
locationFiles_.add(
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.parser(), extensionRegistry));
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
objectStorageRegion_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
presignedUrls_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
presignedUrls_.add(s);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
location_ = location_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
locationFiles_ = java.util.Collections.unmodifiableList(locationFiles_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
presignedUrls_ = presignedUrls_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultLocation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder.class);
}
public static final int LOCATION_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList location_;
/**
* repeated string location = 1;
* @return A list containing the location.
*/
public com.google.protobuf.ProtocolStringList
getLocationList() {
return location_;
}
/**
* repeated string location = 1;
* @return The count of location.
*/
public int getLocationCount() {
return location_.size();
}
/**
* repeated string location = 1;
* @param index The index of the element to return.
* @return The location at the given index.
*/
public java.lang.String getLocation(int index) {
return location_.get(index);
}
/**
* repeated string location = 1;
* @param index The index of the value to return.
* @return The bytes of the location at the given index.
*/
public com.google.protobuf.ByteString
getLocationBytes(int index) {
return location_.getByteString(index);
}
public static final int FILE_SYSTEM_FIELD_NUMBER = 2;
private int fileSystem_;
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The enum numeric value on the wire for fileSystem.
*/
@java.lang.Override public int getFileSystemValue() {
return fileSystem_;
}
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The fileSystem.
*/
@java.lang.Override public cz.proto.FileSystem.FileSystemType getFileSystem() {
@SuppressWarnings("deprecation")
cz.proto.FileSystem.FileSystemType result = cz.proto.FileSystem.FileSystemType.valueOf(fileSystem_);
return result == null ? cz.proto.FileSystem.FileSystemType.UNRECOGNIZED : result;
}
public static final int STS_AK_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object stsAkId_;
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
@java.lang.Override
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
}
}
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_SECRET_FIELD_NUMBER = 4;
private volatile java.lang.Object stsAkSecret_;
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
@java.lang.Override
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
}
}
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_TOKEN_FIELD_NUMBER = 5;
private volatile java.lang.Object stsToken_;
/**
* string sts_token = 5;
* @return The stsToken.
*/
@java.lang.Override
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
}
}
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSS_ENDPOINT_FIELD_NUMBER = 6;
private volatile java.lang.Object ossEndpoint_;
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
@java.lang.Override
public java.lang.String getOssEndpoint() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossEndpoint_ = s;
return s;
}
}
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOssEndpointBytes() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSS_INTERNAL_ENDPOINT_FIELD_NUMBER = 7;
private volatile java.lang.Object ossInternalEndpoint_;
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
@java.lang.Override
public java.lang.String getOssInternalEndpoint() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossInternalEndpoint_ = s;
return s;
}
}
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOssInternalEndpointBytes() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossInternalEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCATION_FILES_FIELD_NUMBER = 8;
private java.util.List locationFiles_;
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
@java.lang.Override
public java.util.List getLocationFilesList() {
return locationFiles_;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
@java.lang.Override
public java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder>
getLocationFilesOrBuilderList() {
return locationFiles_;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
@java.lang.Override
public int getLocationFilesCount() {
return locationFiles_.size();
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getLocationFiles(int index) {
return locationFiles_.get(index);
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder getLocationFilesOrBuilder(
int index) {
return locationFiles_.get(index);
}
public static final int OBJECT_STORAGE_REGION_FIELD_NUMBER = 9;
private volatile java.lang.Object objectStorageRegion_;
/**
* string object_storage_region = 9;
* @return The objectStorageRegion.
*/
@java.lang.Override
public java.lang.String getObjectStorageRegion() {
java.lang.Object ref = objectStorageRegion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
objectStorageRegion_ = s;
return s;
}
}
/**
* string object_storage_region = 9;
* @return The bytes for objectStorageRegion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getObjectStorageRegionBytes() {
java.lang.Object ref = objectStorageRegion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
objectStorageRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRESIGNED_URLS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList presignedUrls_;
/**
* repeated string presigned_urls = 10;
* @return A list containing the presignedUrls.
*/
public com.google.protobuf.ProtocolStringList
getPresignedUrlsList() {
return presignedUrls_;
}
/**
* repeated string presigned_urls = 10;
* @return The count of presignedUrls.
*/
public int getPresignedUrlsCount() {
return presignedUrls_.size();
}
/**
* repeated string presigned_urls = 10;
* @param index The index of the element to return.
* @return The presignedUrls at the given index.
*/
public java.lang.String getPresignedUrls(int index) {
return presignedUrls_.get(index);
}
/**
* repeated string presigned_urls = 10;
* @param index The index of the value to return.
* @return The bytes of the presignedUrls at the given index.
*/
public com.google.protobuf.ByteString
getPresignedUrlsBytes(int index) {
return presignedUrls_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < location_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, location_.getRaw(i));
}
if (fileSystem_ != cz.proto.FileSystem.FileSystemType.LOCAL.getNumber()) {
output.writeEnum(2, fileSystem_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossEndpoint_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, ossEndpoint_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossInternalEndpoint_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, ossInternalEndpoint_);
}
for (int i = 0; i < locationFiles_.size(); i++) {
output.writeMessage(8, locationFiles_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(objectStorageRegion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, objectStorageRegion_);
}
for (int i = 0; i < presignedUrls_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, presignedUrls_.getRaw(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < location_.size(); i++) {
dataSize += computeStringSizeNoTag(location_.getRaw(i));
}
size += dataSize;
size += 1 * getLocationList().size();
}
if (fileSystem_ != cz.proto.FileSystem.FileSystemType.LOCAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, fileSystem_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossEndpoint_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, ossEndpoint_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossInternalEndpoint_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, ossInternalEndpoint_);
}
for (int i = 0; i < locationFiles_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, locationFiles_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(objectStorageRegion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, objectStorageRegion_);
}
{
int dataSize = 0;
for (int i = 0; i < presignedUrls_.size(); i++) {
dataSize += computeStringSizeNoTag(presignedUrls_.getRaw(i));
}
size += dataSize;
size += 1 * getPresignedUrlsList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation) obj;
if (!getLocationList()
.equals(other.getLocationList())) return false;
if (fileSystem_ != other.fileSystem_) return false;
if (!getStsAkId()
.equals(other.getStsAkId())) return false;
if (!getStsAkSecret()
.equals(other.getStsAkSecret())) return false;
if (!getStsToken()
.equals(other.getStsToken())) return false;
if (!getOssEndpoint()
.equals(other.getOssEndpoint())) return false;
if (!getOssInternalEndpoint()
.equals(other.getOssInternalEndpoint())) return false;
if (!getLocationFilesList()
.equals(other.getLocationFilesList())) return false;
if (!getObjectStorageRegion()
.equals(other.getObjectStorageRegion())) return false;
if (!getPresignedUrlsList()
.equals(other.getPresignedUrlsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getLocationCount() > 0) {
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocationList().hashCode();
}
hash = (37 * hash) + FILE_SYSTEM_FIELD_NUMBER;
hash = (53 * hash) + fileSystem_;
hash = (37 * hash) + STS_AK_ID_FIELD_NUMBER;
hash = (53 * hash) + getStsAkId().hashCode();
hash = (37 * hash) + STS_AK_SECRET_FIELD_NUMBER;
hash = (53 * hash) + getStsAkSecret().hashCode();
hash = (37 * hash) + STS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getStsToken().hashCode();
hash = (37 * hash) + OSS_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + getOssEndpoint().hashCode();
hash = (37 * hash) + OSS_INTERNAL_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + getOssInternalEndpoint().hashCode();
if (getLocationFilesCount() > 0) {
hash = (37 * hash) + LOCATION_FILES_FIELD_NUMBER;
hash = (53 * hash) + getLocationFilesList().hashCode();
}
hash = (37 * hash) + OBJECT_STORAGE_REGION_FIELD_NUMBER;
hash = (53 * hash) + getObjectStorageRegion().hashCode();
if (getPresignedUrlsCount() > 0) {
hash = (37 * hash) + PRESIGNED_URLS_FIELD_NUMBER;
hash = (53 * hash) + getPresignedUrlsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultLocation}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobResultLocation)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultLocation_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultLocation_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLocationFilesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
location_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
fileSystem_ = 0;
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
ossEndpoint_ = "";
ossInternalEndpoint_ = "";
if (locationFilesBuilder_ == null) {
locationFiles_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
locationFilesBuilder_.clear();
}
objectStorageRegion_ = "";
presignedUrls_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultLocation_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
location_ = location_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.location_ = location_;
result.fileSystem_ = fileSystem_;
result.stsAkId_ = stsAkId_;
result.stsAkSecret_ = stsAkSecret_;
result.stsToken_ = stsToken_;
result.ossEndpoint_ = ossEndpoint_;
result.ossInternalEndpoint_ = ossInternalEndpoint_;
if (locationFilesBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
locationFiles_ = java.util.Collections.unmodifiableList(locationFiles_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.locationFiles_ = locationFiles_;
} else {
result.locationFiles_ = locationFilesBuilder_.build();
}
result.objectStorageRegion_ = objectStorageRegion_;
if (((bitField0_ & 0x00000004) != 0)) {
presignedUrls_ = presignedUrls_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.presignedUrls_ = presignedUrls_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.getDefaultInstance()) return this;
if (!other.location_.isEmpty()) {
if (location_.isEmpty()) {
location_ = other.location_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLocationIsMutable();
location_.addAll(other.location_);
}
onChanged();
}
if (other.fileSystem_ != 0) {
setFileSystemValue(other.getFileSystemValue());
}
if (!other.getStsAkId().isEmpty()) {
stsAkId_ = other.stsAkId_;
onChanged();
}
if (!other.getStsAkSecret().isEmpty()) {
stsAkSecret_ = other.stsAkSecret_;
onChanged();
}
if (!other.getStsToken().isEmpty()) {
stsToken_ = other.stsToken_;
onChanged();
}
if (!other.getOssEndpoint().isEmpty()) {
ossEndpoint_ = other.ossEndpoint_;
onChanged();
}
if (!other.getOssInternalEndpoint().isEmpty()) {
ossInternalEndpoint_ = other.ossInternalEndpoint_;
onChanged();
}
if (locationFilesBuilder_ == null) {
if (!other.locationFiles_.isEmpty()) {
if (locationFiles_.isEmpty()) {
locationFiles_ = other.locationFiles_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureLocationFilesIsMutable();
locationFiles_.addAll(other.locationFiles_);
}
onChanged();
}
} else {
if (!other.locationFiles_.isEmpty()) {
if (locationFilesBuilder_.isEmpty()) {
locationFilesBuilder_.dispose();
locationFilesBuilder_ = null;
locationFiles_ = other.locationFiles_;
bitField0_ = (bitField0_ & ~0x00000002);
locationFilesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLocationFilesFieldBuilder() : null;
} else {
locationFilesBuilder_.addAllMessages(other.locationFiles_);
}
}
}
if (!other.getObjectStorageRegion().isEmpty()) {
objectStorageRegion_ = other.objectStorageRegion_;
onChanged();
}
if (!other.presignedUrls_.isEmpty()) {
if (presignedUrls_.isEmpty()) {
presignedUrls_ = other.presignedUrls_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePresignedUrlsIsMutable();
presignedUrls_.addAll(other.presignedUrls_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList location_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureLocationIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
location_ = new com.google.protobuf.LazyStringArrayList(location_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string location = 1;
* @return A list containing the location.
*/
public com.google.protobuf.ProtocolStringList
getLocationList() {
return location_.getUnmodifiableView();
}
/**
* repeated string location = 1;
* @return The count of location.
*/
public int getLocationCount() {
return location_.size();
}
/**
* repeated string location = 1;
* @param index The index of the element to return.
* @return The location at the given index.
*/
public java.lang.String getLocation(int index) {
return location_.get(index);
}
/**
* repeated string location = 1;
* @param index The index of the value to return.
* @return The bytes of the location at the given index.
*/
public com.google.protobuf.ByteString
getLocationBytes(int index) {
return location_.getByteString(index);
}
/**
* repeated string location = 1;
* @param index The index to set the value at.
* @param value The location to set.
* @return This builder for chaining.
*/
public Builder setLocation(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationIsMutable();
location_.set(index, value);
onChanged();
return this;
}
/**
* repeated string location = 1;
* @param value The location to add.
* @return This builder for chaining.
*/
public Builder addLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationIsMutable();
location_.add(value);
onChanged();
return this;
}
/**
* repeated string location = 1;
* @param values The location to add.
* @return This builder for chaining.
*/
public Builder addAllLocation(
java.lang.Iterable values) {
ensureLocationIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, location_);
onChanged();
return this;
}
/**
* repeated string location = 1;
* @return This builder for chaining.
*/
public Builder clearLocation() {
location_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string location = 1;
* @param value The bytes of the location to add.
* @return This builder for chaining.
*/
public Builder addLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureLocationIsMutable();
location_.add(value);
onChanged();
return this;
}
private int fileSystem_ = 0;
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The enum numeric value on the wire for fileSystem.
*/
@java.lang.Override public int getFileSystemValue() {
return fileSystem_;
}
/**
* .cz.proto.FileSystemType file_system = 2;
* @param value The enum numeric value on the wire for fileSystem to set.
* @return This builder for chaining.
*/
public Builder setFileSystemValue(int value) {
fileSystem_ = value;
onChanged();
return this;
}
/**
* .cz.proto.FileSystemType file_system = 2;
* @return The fileSystem.
*/
@java.lang.Override
public cz.proto.FileSystem.FileSystemType getFileSystem() {
@SuppressWarnings("deprecation")
cz.proto.FileSystem.FileSystemType result = cz.proto.FileSystem.FileSystemType.valueOf(fileSystem_);
return result == null ? cz.proto.FileSystem.FileSystemType.UNRECOGNIZED : result;
}
/**
* .cz.proto.FileSystemType file_system = 2;
* @param value The fileSystem to set.
* @return This builder for chaining.
*/
public Builder setFileSystem(cz.proto.FileSystem.FileSystemType value) {
if (value == null) {
throw new NullPointerException();
}
fileSystem_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.FileSystemType file_system = 2;
* @return This builder for chaining.
*/
public Builder clearFileSystem() {
fileSystem_ = 0;
onChanged();
return this;
}
private java.lang.Object stsAkId_ = "";
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_id = 3;
* @param value The stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkId_ = value;
onChanged();
return this;
}
/**
* string sts_ak_id = 3;
* @return This builder for chaining.
*/
public Builder clearStsAkId() {
stsAkId_ = getDefaultInstance().getStsAkId();
onChanged();
return this;
}
/**
* string sts_ak_id = 3;
* @param value The bytes for stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkId_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkSecret_ = "";
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_secret = 4;
* @param value The stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkSecret_ = value;
onChanged();
return this;
}
/**
* string sts_ak_secret = 4;
* @return This builder for chaining.
*/
public Builder clearStsAkSecret() {
stsAkSecret_ = getDefaultInstance().getStsAkSecret();
onChanged();
return this;
}
/**
* string sts_ak_secret = 4;
* @param value The bytes for stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkSecret_ = value;
onChanged();
return this;
}
private java.lang.Object stsToken_ = "";
/**
* string sts_token = 5;
* @return The stsToken.
*/
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_token = 5;
* @param value The stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsToken_ = value;
onChanged();
return this;
}
/**
* string sts_token = 5;
* @return This builder for chaining.
*/
public Builder clearStsToken() {
stsToken_ = getDefaultInstance().getStsToken();
onChanged();
return this;
}
/**
* string sts_token = 5;
* @param value The bytes for stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsToken_ = value;
onChanged();
return this;
}
private java.lang.Object ossEndpoint_ = "";
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
public java.lang.String getOssEndpoint() {
java.lang.Object ref = ossEndpoint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossEndpoint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
public com.google.protobuf.ByteString
getOssEndpointBytes() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string oss_endpoint = 6;
* @param value The ossEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssEndpoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ossEndpoint_ = value;
onChanged();
return this;
}
/**
* string oss_endpoint = 6;
* @return This builder for chaining.
*/
public Builder clearOssEndpoint() {
ossEndpoint_ = getDefaultInstance().getOssEndpoint();
onChanged();
return this;
}
/**
* string oss_endpoint = 6;
* @param value The bytes for ossEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssEndpointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ossEndpoint_ = value;
onChanged();
return this;
}
private java.lang.Object ossInternalEndpoint_ = "";
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
public java.lang.String getOssInternalEndpoint() {
java.lang.Object ref = ossInternalEndpoint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossInternalEndpoint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
public com.google.protobuf.ByteString
getOssInternalEndpointBytes() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossInternalEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string oss_internal_endpoint = 7;
* @param value The ossInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssInternalEndpoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ossInternalEndpoint_ = value;
onChanged();
return this;
}
/**
* string oss_internal_endpoint = 7;
* @return This builder for chaining.
*/
public Builder clearOssInternalEndpoint() {
ossInternalEndpoint_ = getDefaultInstance().getOssInternalEndpoint();
onChanged();
return this;
}
/**
* string oss_internal_endpoint = 7;
* @param value The bytes for ossInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssInternalEndpointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ossInternalEndpoint_ = value;
onChanged();
return this;
}
private java.util.List locationFiles_ =
java.util.Collections.emptyList();
private void ensureLocationFilesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
locationFiles_ = new java.util.ArrayList(locationFiles_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder> locationFilesBuilder_;
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public java.util.List getLocationFilesList() {
if (locationFilesBuilder_ == null) {
return java.util.Collections.unmodifiableList(locationFiles_);
} else {
return locationFilesBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public int getLocationFilesCount() {
if (locationFilesBuilder_ == null) {
return locationFiles_.size();
} else {
return locationFilesBuilder_.getCount();
}
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo getLocationFiles(int index) {
if (locationFilesBuilder_ == null) {
return locationFiles_.get(index);
} else {
return locationFilesBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder setLocationFiles(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo value) {
if (locationFilesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationFilesIsMutable();
locationFiles_.set(index, value);
onChanged();
} else {
locationFilesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder setLocationFiles(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder builderForValue) {
if (locationFilesBuilder_ == null) {
ensureLocationFilesIsMutable();
locationFiles_.set(index, builderForValue.build());
onChanged();
} else {
locationFilesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder addLocationFiles(cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo value) {
if (locationFilesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationFilesIsMutable();
locationFiles_.add(value);
onChanged();
} else {
locationFilesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder addLocationFiles(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo value) {
if (locationFilesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLocationFilesIsMutable();
locationFiles_.add(index, value);
onChanged();
} else {
locationFilesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder addLocationFiles(
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder builderForValue) {
if (locationFilesBuilder_ == null) {
ensureLocationFilesIsMutable();
locationFiles_.add(builderForValue.build());
onChanged();
} else {
locationFilesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder addLocationFiles(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder builderForValue) {
if (locationFilesBuilder_ == null) {
ensureLocationFilesIsMutable();
locationFiles_.add(index, builderForValue.build());
onChanged();
} else {
locationFilesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder addAllLocationFiles(
java.lang.Iterable extends cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo> values) {
if (locationFilesBuilder_ == null) {
ensureLocationFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, locationFiles_);
onChanged();
} else {
locationFilesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder clearLocationFiles() {
if (locationFilesBuilder_ == null) {
locationFiles_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
locationFilesBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public Builder removeLocationFiles(int index) {
if (locationFilesBuilder_ == null) {
ensureLocationFilesIsMutable();
locationFiles_.remove(index);
onChanged();
} else {
locationFilesBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder getLocationFilesBuilder(
int index) {
return getLocationFilesFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder getLocationFilesOrBuilder(
int index) {
if (locationFilesBuilder_ == null) {
return locationFiles_.get(index); } else {
return locationFilesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder>
getLocationFilesOrBuilderList() {
if (locationFilesBuilder_ != null) {
return locationFilesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(locationFiles_);
}
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder addLocationFilesBuilder() {
return getLocationFilesFieldBuilder().addBuilder(
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder addLocationFilesBuilder(
int index) {
return getLocationFilesFieldBuilder().addBuilder(
index, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.coordinator.LocationFileInfo location_files = 8;
*/
public java.util.List
getLocationFilesBuilderList() {
return getLocationFilesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder>
getLocationFilesFieldBuilder() {
if (locationFilesBuilder_ == null) {
locationFilesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfo.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.LocationFileInfoOrBuilder>(
locationFiles_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
locationFiles_ = null;
}
return locationFilesBuilder_;
}
private java.lang.Object objectStorageRegion_ = "";
/**
* string object_storage_region = 9;
* @return The objectStorageRegion.
*/
public java.lang.String getObjectStorageRegion() {
java.lang.Object ref = objectStorageRegion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
objectStorageRegion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string object_storage_region = 9;
* @return The bytes for objectStorageRegion.
*/
public com.google.protobuf.ByteString
getObjectStorageRegionBytes() {
java.lang.Object ref = objectStorageRegion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
objectStorageRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string object_storage_region = 9;
* @param value The objectStorageRegion to set.
* @return This builder for chaining.
*/
public Builder setObjectStorageRegion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
objectStorageRegion_ = value;
onChanged();
return this;
}
/**
* string object_storage_region = 9;
* @return This builder for chaining.
*/
public Builder clearObjectStorageRegion() {
objectStorageRegion_ = getDefaultInstance().getObjectStorageRegion();
onChanged();
return this;
}
/**
* string object_storage_region = 9;
* @param value The bytes for objectStorageRegion to set.
* @return This builder for chaining.
*/
public Builder setObjectStorageRegionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
objectStorageRegion_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList presignedUrls_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensurePresignedUrlsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
presignedUrls_ = new com.google.protobuf.LazyStringArrayList(presignedUrls_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated string presigned_urls = 10;
* @return A list containing the presignedUrls.
*/
public com.google.protobuf.ProtocolStringList
getPresignedUrlsList() {
return presignedUrls_.getUnmodifiableView();
}
/**
* repeated string presigned_urls = 10;
* @return The count of presignedUrls.
*/
public int getPresignedUrlsCount() {
return presignedUrls_.size();
}
/**
* repeated string presigned_urls = 10;
* @param index The index of the element to return.
* @return The presignedUrls at the given index.
*/
public java.lang.String getPresignedUrls(int index) {
return presignedUrls_.get(index);
}
/**
* repeated string presigned_urls = 10;
* @param index The index of the value to return.
* @return The bytes of the presignedUrls at the given index.
*/
public com.google.protobuf.ByteString
getPresignedUrlsBytes(int index) {
return presignedUrls_.getByteString(index);
}
/**
* repeated string presigned_urls = 10;
* @param index The index to set the value at.
* @param value The presignedUrls to set.
* @return This builder for chaining.
*/
public Builder setPresignedUrls(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePresignedUrlsIsMutable();
presignedUrls_.set(index, value);
onChanged();
return this;
}
/**
* repeated string presigned_urls = 10;
* @param value The presignedUrls to add.
* @return This builder for chaining.
*/
public Builder addPresignedUrls(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePresignedUrlsIsMutable();
presignedUrls_.add(value);
onChanged();
return this;
}
/**
* repeated string presigned_urls = 10;
* @param values The presignedUrls to add.
* @return This builder for chaining.
*/
public Builder addAllPresignedUrls(
java.lang.Iterable values) {
ensurePresignedUrlsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, presignedUrls_);
onChanged();
return this;
}
/**
* repeated string presigned_urls = 10;
* @return This builder for chaining.
*/
public Builder clearPresignedUrls() {
presignedUrls_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* repeated string presigned_urls = 10;
* @param value The bytes of the presignedUrls to add.
* @return This builder for chaining.
*/
public Builder addPresignedUrlsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensurePresignedUrlsIsMutable();
presignedUrls_.add(value);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobResultLocation)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobResultLocation)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobResultLocation parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobResultLocation(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobResultSetMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobResultSetMetadata)
com.google.protobuf.MessageOrBuilder {
/**
* int64 num_rows = 1;
* @return The numRows.
*/
long getNumRows();
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The enum numeric value on the wire for format.
*/
int getFormatValue();
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The format.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat getFormat();
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The type.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType getType();
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
java.util.List
getFieldsList();
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
cz.proto.FieldSchema getFields(int index);
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
int getFieldsCount();
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
java.util.List extends cz.proto.FieldSchemaOrBuilder>
getFieldsOrBuilderList();
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
cz.proto.FieldSchemaOrBuilder getFieldsOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultSetMetadata}
*/
public static final class JobResultSetMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobResultSetMetadata)
JobResultSetMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobResultSetMetadata.newBuilder() to construct.
private JobResultSetMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobResultSetMetadata() {
format_ = 0;
type_ = 0;
fields_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobResultSetMetadata();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobResultSetMetadata(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
numRows_ = input.readInt64();
break;
}
case 16: {
int rawValue = input.readEnum();
format_ = rawValue;
break;
}
case 24: {
int rawValue = input.readEnum();
type_ = rawValue;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
fields_.add(
input.readMessage(cz.proto.FieldSchema.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSetMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSetMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder.class);
}
public static final int NUM_ROWS_FIELD_NUMBER = 1;
private long numRows_;
/**
* int64 num_rows = 1;
* @return The numRows.
*/
@java.lang.Override
public long getNumRows() {
return numRows_;
}
public static final int FORMAT_FIELD_NUMBER = 2;
private int format_;
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The enum numeric value on the wire for format.
*/
@java.lang.Override public int getFormatValue() {
return format_;
}
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The format.
*/
@java.lang.Override public cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat getFormat() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat result = cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.valueOf(format_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.UNRECOGNIZED : result;
}
public static final int TYPE_FIELD_NUMBER = 3;
private int type_;
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The type.
*/
@java.lang.Override public cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType getType() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType result = cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.valueOf(type_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.UNRECOGNIZED : result;
}
public static final int FIELDS_FIELD_NUMBER = 4;
private java.util.List fields_;
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
@java.lang.Override
public java.util.List getFieldsList() {
return fields_;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
@java.lang.Override
public java.util.List extends cz.proto.FieldSchemaOrBuilder>
getFieldsOrBuilderList() {
return fields_;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
@java.lang.Override
public int getFieldsCount() {
return fields_.size();
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
@java.lang.Override
public cz.proto.FieldSchema getFields(int index) {
return fields_.get(index);
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
@java.lang.Override
public cz.proto.FieldSchemaOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (numRows_ != 0L) {
output.writeInt64(1, numRows_);
}
if (format_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.TEXT.getNumber()) {
output.writeEnum(2, format_);
}
if (type_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.BUFFERED_STREAM.getNumber()) {
output.writeEnum(3, type_);
}
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(4, fields_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (numRows_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, numRows_);
}
if (format_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.TEXT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, format_);
}
if (type_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.BUFFERED_STREAM.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, type_);
}
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, fields_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata) obj;
if (getNumRows()
!= other.getNumRows()) return false;
if (format_ != other.format_) return false;
if (type_ != other.type_) return false;
if (!getFieldsList()
.equals(other.getFieldsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NUM_ROWS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumRows());
hash = (37 * hash) + FORMAT_FIELD_NUMBER;
hash = (53 * hash) + format_;
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
if (getFieldsCount() > 0) {
hash = (37 * hash) + FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultSetMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobResultSetMetadata)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSetMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSetMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFieldsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
numRows_ = 0L;
format_ = 0;
type_ = 0;
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
fieldsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSetMetadata_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata(this);
int from_bitField0_ = bitField0_;
result.numRows_ = numRows_;
result.format_ = format_;
result.type_ = type_;
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.getDefaultInstance()) return this;
if (other.getNumRows() != 0L) {
setNumRows(other.getNumRows());
}
if (other.format_ != 0) {
setFormatValue(other.getFormatValue());
}
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFieldsFieldBuilder() : null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long numRows_ ;
/**
* int64 num_rows = 1;
* @return The numRows.
*/
@java.lang.Override
public long getNumRows() {
return numRows_;
}
/**
* int64 num_rows = 1;
* @param value The numRows to set.
* @return This builder for chaining.
*/
public Builder setNumRows(long value) {
numRows_ = value;
onChanged();
return this;
}
/**
* int64 num_rows = 1;
* @return This builder for chaining.
*/
public Builder clearNumRows() {
numRows_ = 0L;
onChanged();
return this;
}
private int format_ = 0;
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The enum numeric value on the wire for format.
*/
@java.lang.Override public int getFormatValue() {
return format_;
}
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @param value The enum numeric value on the wire for format to set.
* @return This builder for chaining.
*/
public Builder setFormatValue(int value) {
format_ = value;
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return The format.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat getFormat() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat result = cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.valueOf(format_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat.UNRECOGNIZED : result;
}
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @param value The format to set.
* @return This builder for chaining.
*/
public Builder setFormat(cz.proto.coordinator.CoordinatorServiceOuterClass.ResultFormat value) {
if (value == null) {
throw new NullPointerException();
}
format_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ResultFormat format = 2;
* @return This builder for chaining.
*/
public Builder clearFormat() {
format_ = 0;
onChanged();
return this;
}
private int type_ = 0;
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .cz.proto.coordinator.ResultType type = 3;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return The type.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType getType() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType result = cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.valueOf(type_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType.UNRECOGNIZED : result;
}
/**
* .cz.proto.coordinator.ResultType type = 3;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(cz.proto.coordinator.CoordinatorServiceOuterClass.ResultType value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ResultType type = 3;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = 0;
onChanged();
return this;
}
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fields_ = new java.util.ArrayList(fields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.FieldSchema, cz.proto.FieldSchema.Builder, cz.proto.FieldSchemaOrBuilder> fieldsBuilder_;
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public java.util.List getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public cz.proto.FieldSchema getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder setFields(
int index, cz.proto.FieldSchema value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder setFields(
int index, cz.proto.FieldSchema.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder addFields(cz.proto.FieldSchema value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder addFields(
int index, cz.proto.FieldSchema value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder addFields(
cz.proto.FieldSchema.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder addFields(
int index, cz.proto.FieldSchema.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder addAllFields(
java.lang.Iterable extends cz.proto.FieldSchema> values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public cz.proto.FieldSchema.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public cz.proto.FieldSchemaOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index); } else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public java.util.List extends cz.proto.FieldSchemaOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public cz.proto.FieldSchema.Builder addFieldsBuilder() {
return getFieldsFieldBuilder().addBuilder(
cz.proto.FieldSchema.getDefaultInstance());
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public cz.proto.FieldSchema.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder().addBuilder(
index, cz.proto.FieldSchema.getDefaultInstance());
}
/**
*
* schema for result
*
*
* repeated .cz.proto.FieldSchema fields = 4;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.FieldSchema, cz.proto.FieldSchema.Builder, cz.proto.FieldSchemaOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.FieldSchema, cz.proto.FieldSchema.Builder, cz.proto.FieldSchemaOrBuilder>(
fields_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
fields_ = null;
}
return fieldsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobResultSetMetadata)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobResultSetMetadata)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobResultSetMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobResultSetMetadata(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobResultSetOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobResultSet)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return Whether the metadata field is set.
*/
boolean hasMetadata();
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return The metadata.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getMetadata();
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder getMetadataOrBuilder();
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return Whether the data field is set.
*/
boolean hasData();
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return The data.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getData();
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder getDataOrBuilder();
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return Whether the location field is set.
*/
boolean hasLocation();
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return The location.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getLocation();
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder getLocationOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultSet}
*/
public static final class JobResultSet extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobResultSet)
JobResultSetOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobResultSet.newBuilder() to construct.
private JobResultSet(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobResultSet() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobResultSet();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobResultSet(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder subBuilder = null;
if (metadata_ != null) {
subBuilder = metadata_.toBuilder();
}
metadata_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(metadata_);
metadata_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder subBuilder = null;
if (data_ != null) {
subBuilder = data_.toBuilder();
}
data_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(data_);
data_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder subBuilder = null;
if (location_ != null) {
subBuilder = location_.toBuilder();
}
location_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(location_);
location_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder.class);
}
public static final int METADATA_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata metadata_;
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return Whether the metadata field is set.
*/
@java.lang.Override
public boolean hasMetadata() {
return metadata_ != null;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return The metadata.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getMetadata() {
return metadata_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.getDefaultInstance() : metadata_;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder getMetadataOrBuilder() {
return getMetadata();
}
public static final int DATA_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData data_;
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return Whether the data field is set.
*/
@java.lang.Override
public boolean hasData() {
return data_ != null;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return The data.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getData() {
return data_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.getDefaultInstance() : data_;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder getDataOrBuilder() {
return getData();
}
public static final int LOCATION_FIELD_NUMBER = 3;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation location_;
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return Whether the location field is set.
*/
@java.lang.Override
public boolean hasLocation() {
return location_ != null;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return The location.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getLocation() {
return location_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.getDefaultInstance() : location_;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder getLocationOrBuilder() {
return getLocation();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (metadata_ != null) {
output.writeMessage(1, getMetadata());
}
if (data_ != null) {
output.writeMessage(2, getData());
}
if (location_ != null) {
output.writeMessage(3, getLocation());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (metadata_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMetadata());
}
if (data_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getData());
}
if (location_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getLocation());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet) obj;
if (hasMetadata() != other.hasMetadata()) return false;
if (hasMetadata()) {
if (!getMetadata()
.equals(other.getMetadata())) return false;
}
if (hasData() != other.hasData()) return false;
if (hasData()) {
if (!getData()
.equals(other.getData())) return false;
}
if (hasLocation() != other.hasLocation()) return false;
if (hasLocation()) {
if (!getLocation()
.equals(other.getLocation())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMetadata()) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + getMetadata().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (hasLocation()) {
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobResultSet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobResultSet)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (metadataBuilder_ == null) {
metadata_ = null;
} else {
metadata_ = null;
metadataBuilder_ = null;
}
if (dataBuilder_ == null) {
data_ = null;
} else {
data_ = null;
dataBuilder_ = null;
}
if (locationBuilder_ == null) {
location_ = null;
} else {
location_ = null;
locationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobResultSet_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet(this);
if (metadataBuilder_ == null) {
result.metadata_ = metadata_;
} else {
result.metadata_ = metadataBuilder_.build();
}
if (dataBuilder_ == null) {
result.data_ = data_;
} else {
result.data_ = dataBuilder_.build();
}
if (locationBuilder_ == null) {
result.location_ = location_;
} else {
result.location_ = locationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance()) return this;
if (other.hasMetadata()) {
mergeMetadata(other.getMetadata());
}
if (other.hasData()) {
mergeData(other.getData());
}
if (other.hasLocation()) {
mergeLocation(other.getLocation());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata metadata_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder> metadataBuilder_;
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return Whether the metadata field is set.
*/
public boolean hasMetadata() {
return metadataBuilder_ != null || metadata_ != null;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
* @return The metadata.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata getMetadata() {
if (metadataBuilder_ == null) {
return metadata_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.getDefaultInstance() : metadata_;
} else {
return metadataBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public Builder setMetadata(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metadata_ = value;
onChanged();
} else {
metadataBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public Builder setMetadata(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder builderForValue) {
if (metadataBuilder_ == null) {
metadata_ = builderForValue.build();
onChanged();
} else {
metadataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public Builder mergeMetadata(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata value) {
if (metadataBuilder_ == null) {
if (metadata_ != null) {
metadata_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.newBuilder(metadata_).mergeFrom(value).buildPartial();
} else {
metadata_ = value;
}
onChanged();
} else {
metadataBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public Builder clearMetadata() {
if (metadataBuilder_ == null) {
metadata_ = null;
onChanged();
} else {
metadata_ = null;
metadataBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder getMetadataBuilder() {
onChanged();
return getMetadataFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder getMetadataOrBuilder() {
if (metadataBuilder_ != null) {
return metadataBuilder_.getMessageOrBuilder();
} else {
return metadata_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.getDefaultInstance() : metadata_;
}
}
/**
* .cz.proto.coordinator.JobResultSetMetadata metadata = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder>
getMetadataFieldBuilder() {
if (metadataBuilder_ == null) {
metadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadata.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetMetadataOrBuilder>(
getMetadata(),
getParentForChildren(),
isClean());
metadata_ = null;
}
return metadataBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData data_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder> dataBuilder_;
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return Whether the data field is set.
*/
public boolean hasData() {
return dataBuilder_ != null || data_ != null;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
* @return The data.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData getData() {
if (dataBuilder_ == null) {
return data_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.getDefaultInstance() : data_;
} else {
return dataBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public Builder setData(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData value) {
if (dataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
} else {
dataBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public Builder setData(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder builderForValue) {
if (dataBuilder_ == null) {
data_ = builderForValue.build();
onChanged();
} else {
dataBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public Builder mergeData(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData value) {
if (dataBuilder_ == null) {
if (data_ != null) {
data_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.newBuilder(data_).mergeFrom(value).buildPartial();
} else {
data_ = value;
}
onChanged();
} else {
dataBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public Builder clearData() {
if (dataBuilder_ == null) {
data_ = null;
onChanged();
} else {
data_ = null;
dataBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder getDataBuilder() {
onChanged();
return getDataFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder getDataOrBuilder() {
if (dataBuilder_ != null) {
return dataBuilder_.getMessageOrBuilder();
} else {
return data_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.getDefaultInstance() : data_;
}
}
/**
* .cz.proto.coordinator.JobResultData data = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder>
getDataFieldBuilder() {
if (dataBuilder_ == null) {
dataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultData.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultDataOrBuilder>(
getData(),
getParentForChildren(),
isClean());
data_ = null;
}
return dataBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation location_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder> locationBuilder_;
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return Whether the location field is set.
*/
public boolean hasLocation() {
return locationBuilder_ != null || location_ != null;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
* @return The location.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation getLocation() {
if (locationBuilder_ == null) {
return location_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.getDefaultInstance() : location_;
} else {
return locationBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public Builder setLocation(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation value) {
if (locationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
location_ = value;
onChanged();
} else {
locationBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public Builder setLocation(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder builderForValue) {
if (locationBuilder_ == null) {
location_ = builderForValue.build();
onChanged();
} else {
locationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public Builder mergeLocation(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation value) {
if (locationBuilder_ == null) {
if (location_ != null) {
location_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.newBuilder(location_).mergeFrom(value).buildPartial();
} else {
location_ = value;
}
onChanged();
} else {
locationBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public Builder clearLocation() {
if (locationBuilder_ == null) {
location_ = null;
onChanged();
} else {
location_ = null;
locationBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder getLocationBuilder() {
onChanged();
return getLocationFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder getLocationOrBuilder() {
if (locationBuilder_ != null) {
return locationBuilder_.getMessageOrBuilder();
} else {
return location_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.getDefaultInstance() : location_;
}
}
/**
* .cz.proto.coordinator.JobResultLocation location = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder>
getLocationFieldBuilder() {
if (locationBuilder_ == null) {
locationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocation.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultLocationOrBuilder>(
getLocation(),
getParentForChildren(),
isClean());
location_ = null;
}
return locationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobResultSet)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobResultSet)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobResultSet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobResultSet(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubmitJobRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.SubmitJobRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return Whether the jobDesc field is set.
*/
boolean hasJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return The jobDesc.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.SubmitJobRequest}
*/
public static final class SubmitJobRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.SubmitJobRequest)
SubmitJobRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubmitJobRequest.newBuilder() to construct.
private SubmitJobRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubmitJobRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubmitJobRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubmitJobRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder subBuilder = null;
if (jobDesc_ != null) {
subBuilder = jobDesc_.toBuilder();
}
jobDesc_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobDesc_);
jobDesc_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.Builder.class);
}
public static final int JOB_DESC_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return Whether the jobDesc field is set.
*/
@java.lang.Override
public boolean hasJobDesc() {
return jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return The jobDesc.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
return getJobDesc();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (jobDesc_ != null) {
output.writeMessage(1, getJobDesc());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (jobDesc_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getJobDesc());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest) obj;
if (hasJobDesc() != other.hasJobDesc()) return false;
if (hasJobDesc()) {
if (!getJobDesc()
.equals(other.getJobDesc())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasJobDesc()) {
hash = (37 * hash) + JOB_DESC_FIELD_NUMBER;
hash = (53 * hash) + getJobDesc().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.SubmitJobRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.SubmitJobRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (jobDescBuilder_ == null) {
jobDesc_ = null;
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest(this);
if (jobDescBuilder_ == null) {
result.jobDesc_ = jobDesc_;
} else {
result.jobDesc_ = jobDescBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest.getDefaultInstance()) return this;
if (other.hasJobDesc()) {
mergeJobDesc(other.getJobDesc());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder> jobDescBuilder_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return Whether the jobDesc field is set.
*/
public boolean hasJobDesc() {
return jobDescBuilder_ != null || jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
* @return The jobDesc.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
if (jobDescBuilder_ == null) {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
} else {
return jobDescBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public Builder setJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobDesc_ = value;
onChanged();
} else {
jobDescBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public Builder setJobDesc(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder builderForValue) {
if (jobDescBuilder_ == null) {
jobDesc_ = builderForValue.build();
onChanged();
} else {
jobDescBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public Builder mergeJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (jobDesc_ != null) {
jobDesc_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.newBuilder(jobDesc_).mergeFrom(value).buildPartial();
} else {
jobDesc_ = value;
}
onChanged();
} else {
jobDescBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public Builder clearJobDesc() {
if (jobDescBuilder_ == null) {
jobDesc_ = null;
onChanged();
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder getJobDescBuilder() {
onChanged();
return getJobDescFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
if (jobDescBuilder_ != null) {
return jobDescBuilder_.getMessageOrBuilder();
} else {
return jobDesc_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>
getJobDescFieldBuilder() {
if (jobDescBuilder_ == null) {
jobDescBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>(
getJobDesc(),
getParentForChildren(),
isClean());
jobDesc_ = null;
}
return jobDescBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.SubmitJobRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.SubmitJobRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubmitJobRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubmitJobRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SubmitJobResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.SubmitJobResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder();
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
boolean hasResultSet();
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet();
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.SubmitJobResponse}
*/
public static final class SubmitJobResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.SubmitJobResponse)
SubmitJobResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use SubmitJobResponse.newBuilder() to construct.
private SubmitJobResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SubmitJobResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SubmitJobResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SubmitJobResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder subBuilder = null;
if (resultSet_ != null) {
subBuilder = resultSet_.toBuilder();
}
resultSet_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resultSet_);
resultSet_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int STATUS_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int RESULT_SET_FIELD_NUMBER = 3;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet resultSet_;
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
@java.lang.Override
public boolean hasResultSet() {
return resultSet_ != null;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet() {
return resultSet_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder() {
return getResultSet();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (status_ != null) {
output.writeMessage(2, getStatus());
}
if (resultSet_ != null) {
output.writeMessage(3, getResultSet());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStatus());
}
if (resultSet_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getResultSet());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasResultSet() != other.hasResultSet()) return false;
if (hasResultSet()) {
if (!getResultSet()
.equals(other.getResultSet())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasResultSet()) {
hash = (37 * hash) + RESULT_SET_FIELD_NUMBER;
hash = (53 * hash) + getResultSet().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.SubmitJobResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.SubmitJobResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (resultSetBuilder_ == null) {
resultSet_ = null;
} else {
resultSet_ = null;
resultSetBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_SubmitJobResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (resultSetBuilder_ == null) {
result.resultSet_ = resultSet_;
} else {
result.resultSet_ = resultSetBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasResultSet()) {
mergeResultSet(other.getResultSet());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder mergeStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet resultSet_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder> resultSetBuilder_;
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
public boolean hasResultSet() {
return resultSetBuilder_ != null || resultSet_ != null;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet() {
if (resultSetBuilder_ == null) {
return resultSet_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
} else {
return resultSetBuilder_.getMessage();
}
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder setResultSet(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet value) {
if (resultSetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resultSet_ = value;
onChanged();
} else {
resultSetBuilder_.setMessage(value);
}
return this;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder setResultSet(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder builderForValue) {
if (resultSetBuilder_ == null) {
resultSet_ = builderForValue.build();
onChanged();
} else {
resultSetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder mergeResultSet(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet value) {
if (resultSetBuilder_ == null) {
if (resultSet_ != null) {
resultSet_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.newBuilder(resultSet_).mergeFrom(value).buildPartial();
} else {
resultSet_ = value;
}
onChanged();
} else {
resultSetBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder clearResultSet() {
if (resultSetBuilder_ == null) {
resultSet_ = null;
onChanged();
} else {
resultSet_ = null;
resultSetBuilder_ = null;
}
return this;
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder getResultSetBuilder() {
onChanged();
return getResultSetFieldBuilder().getBuilder();
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder() {
if (resultSetBuilder_ != null) {
return resultSetBuilder_.getMessageOrBuilder();
} else {
return resultSet_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
}
}
/**
*
* for sync/hybrid requests, do not support paging
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder>
getResultSetFieldBuilder() {
if (resultSetBuilder_ == null) {
resultSetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder>(
getResultSet(),
getParentForChildren(),
isClean());
resultSet_ = null;
}
return resultSetBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.SubmitJobResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.SubmitJobResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubmitJobResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SubmitJobResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.SubmitJobResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListJobsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.ListJobsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
long getInstanceId();
/**
* string virtual_cluster = 4;
* @return The virtualCluster.
*/
java.lang.String getVirtualCluster();
/**
* string virtual_cluster = 4;
* @return The bytes for virtualCluster.
*/
com.google.protobuf.ByteString
getVirtualClusterBytes();
/**
* string job_status = 5;
* @return The jobStatus.
*/
java.lang.String getJobStatus();
/**
* string job_status = 5;
* @return The bytes for jobStatus.
*/
com.google.protobuf.ByteString
getJobStatusBytes();
/**
* int64 min_start_time = 6;
* @return The minStartTime.
*/
long getMinStartTime();
/**
* int64 max_start_time = 7;
* @return The maxStartTime.
*/
long getMaxStartTime();
/**
* int64 max_size = 9;
* @return The maxSize.
*/
long getMaxSize();
/**
* bool list_in_meta = 10;
* @return The listInMeta.
*/
boolean getListInMeta();
/**
* int64 offset = 11;
* @return The offset.
*/
long getOffset();
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The enum numeric value on the wire for orderBy.
*/
int getOrderByValue();
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The orderBy.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby getOrderBy();
/**
* bool ascending = 13;
* @return The ascending.
*/
boolean getAscending();
/**
* string priority_string = 14;
* @return The priorityString.
*/
java.lang.String getPriorityString();
/**
* string priority_string = 14;
* @return The bytes for priorityString.
*/
com.google.protobuf.ByteString
getPriorityStringBytes();
/**
* string job_id = 15;
* @return The jobId.
*/
java.lang.String getJobId();
/**
* string job_id = 15;
* @return The bytes for jobId.
*/
com.google.protobuf.ByteString
getJobIdBytes();
/**
* int64 owner_id = 16;
* @return The ownerId.
*/
long getOwnerId();
/**
* int64 min_running_time_ms = 17;
* @return The minRunningTimeMs.
*/
long getMinRunningTimeMs();
/**
* int64 max_running_time_ms = 18;
* @return The maxRunningTimeMs.
*/
long getMaxRunningTimeMs();
/**
* string query_tag = 19;
* @return The queryTag.
*/
java.lang.String getQueryTag();
/**
* string query_tag = 19;
* @return The bytes for queryTag.
*/
com.google.protobuf.ByteString
getQueryTagBytes();
/**
* bool detail = 28;
* @return The detail.
*/
boolean getDetail();
/**
* bool force = 29;
* @return The force.
*/
boolean getForce();
/**
* string user_agent = 30;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsRequest}
*/
public static final class ListJobsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.ListJobsRequest)
ListJobsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListJobsRequest.newBuilder() to construct.
private ListJobsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListJobsRequest() {
workspace_ = "";
virtualCluster_ = "";
jobStatus_ = "";
orderBy_ = 0;
priorityString_ = "";
jobId_ = "";
queryTag_ = "";
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListJobsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListJobsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 24: {
instanceId_ = input.readInt64();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
virtualCluster_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
jobStatus_ = s;
break;
}
case 48: {
minStartTime_ = input.readInt64();
break;
}
case 56: {
maxStartTime_ = input.readInt64();
break;
}
case 72: {
maxSize_ = input.readInt64();
break;
}
case 80: {
listInMeta_ = input.readBool();
break;
}
case 88: {
offset_ = input.readInt64();
break;
}
case 96: {
int rawValue = input.readEnum();
orderBy_ = rawValue;
break;
}
case 104: {
ascending_ = input.readBool();
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
priorityString_ = s;
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
jobId_ = s;
break;
}
case 128: {
ownerId_ = input.readInt64();
break;
}
case 136: {
minRunningTimeMs_ = input.readInt64();
break;
}
case 144: {
maxRunningTimeMs_ = input.readInt64();
break;
}
case 154: {
java.lang.String s = input.readStringRequireUtf8();
queryTag_ = s;
break;
}
case 224: {
detail_ = input.readBool();
break;
}
case 232: {
force_ = input.readBool();
break;
}
case 242: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.Builder.class);
}
/**
* Protobuf enum {@code cz.proto.coordinator.ListJobsRequest.ListOrderby}
*/
public enum ListOrderby
implements com.google.protobuf.ProtocolMessageEnum {
/**
* ORDER_BY_START_TIME = 0;
*/
ORDER_BY_START_TIME(0),
/**
* ORDER_BY_RUNNING_TIME = 1;
*/
ORDER_BY_RUNNING_TIME(1),
/**
* ORDER_BY_JOB_PRIORITY = 2;
*/
ORDER_BY_JOB_PRIORITY(2),
/**
* ORDER_BY_JOB_STATUS = 3;
*/
ORDER_BY_JOB_STATUS(3),
UNRECOGNIZED(-1),
;
/**
* ORDER_BY_START_TIME = 0;
*/
public static final int ORDER_BY_START_TIME_VALUE = 0;
/**
* ORDER_BY_RUNNING_TIME = 1;
*/
public static final int ORDER_BY_RUNNING_TIME_VALUE = 1;
/**
* ORDER_BY_JOB_PRIORITY = 2;
*/
public static final int ORDER_BY_JOB_PRIORITY_VALUE = 2;
/**
* ORDER_BY_JOB_STATUS = 3;
*/
public static final int ORDER_BY_JOB_STATUS_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ListOrderby valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ListOrderby forNumber(int value) {
switch (value) {
case 0: return ORDER_BY_START_TIME;
case 1: return ORDER_BY_RUNNING_TIME;
case 2: return ORDER_BY_JOB_PRIORITY;
case 3: return ORDER_BY_JOB_STATUS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ListOrderby> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ListOrderby findValueByNumber(int number) {
return ListOrderby.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.getDescriptor().getEnumTypes().get(0);
}
private static final ListOrderby[] VALUES = values();
public static ListOrderby valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ListOrderby(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.coordinator.ListJobsRequest.ListOrderby)
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_ID_FIELD_NUMBER = 3;
private long instanceId_;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int VIRTUAL_CLUSTER_FIELD_NUMBER = 4;
private volatile java.lang.Object virtualCluster_;
/**
* string virtual_cluster = 4;
* @return The virtualCluster.
*/
@java.lang.Override
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
}
}
/**
* string virtual_cluster = 4;
* @return The bytes for virtualCluster.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_STATUS_FIELD_NUMBER = 5;
private volatile java.lang.Object jobStatus_;
/**
* string job_status = 5;
* @return The jobStatus.
*/
@java.lang.Override
public java.lang.String getJobStatus() {
java.lang.Object ref = jobStatus_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobStatus_ = s;
return s;
}
}
/**
* string job_status = 5;
* @return The bytes for jobStatus.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobStatusBytes() {
java.lang.Object ref = jobStatus_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MIN_START_TIME_FIELD_NUMBER = 6;
private long minStartTime_;
/**
* int64 min_start_time = 6;
* @return The minStartTime.
*/
@java.lang.Override
public long getMinStartTime() {
return minStartTime_;
}
public static final int MAX_START_TIME_FIELD_NUMBER = 7;
private long maxStartTime_;
/**
* int64 max_start_time = 7;
* @return The maxStartTime.
*/
@java.lang.Override
public long getMaxStartTime() {
return maxStartTime_;
}
public static final int MAX_SIZE_FIELD_NUMBER = 9;
private long maxSize_;
/**
* int64 max_size = 9;
* @return The maxSize.
*/
@java.lang.Override
public long getMaxSize() {
return maxSize_;
}
public static final int LIST_IN_META_FIELD_NUMBER = 10;
private boolean listInMeta_;
/**
* bool list_in_meta = 10;
* @return The listInMeta.
*/
@java.lang.Override
public boolean getListInMeta() {
return listInMeta_;
}
public static final int OFFSET_FIELD_NUMBER = 11;
private long offset_;
/**
* int64 offset = 11;
* @return The offset.
*/
@java.lang.Override
public long getOffset() {
return offset_;
}
public static final int ORDER_BY_FIELD_NUMBER = 12;
private int orderBy_;
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The enum numeric value on the wire for orderBy.
*/
@java.lang.Override public int getOrderByValue() {
return orderBy_;
}
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The orderBy.
*/
@java.lang.Override public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby getOrderBy() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby result = cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.valueOf(orderBy_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.UNRECOGNIZED : result;
}
public static final int ASCENDING_FIELD_NUMBER = 13;
private boolean ascending_;
/**
* bool ascending = 13;
* @return The ascending.
*/
@java.lang.Override
public boolean getAscending() {
return ascending_;
}
public static final int PRIORITY_STRING_FIELD_NUMBER = 14;
private volatile java.lang.Object priorityString_;
/**
* string priority_string = 14;
* @return The priorityString.
*/
@java.lang.Override
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
}
}
/**
* string priority_string = 14;
* @return The bytes for priorityString.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_ID_FIELD_NUMBER = 15;
private volatile java.lang.Object jobId_;
/**
* string job_id = 15;
* @return The jobId.
*/
@java.lang.Override
public java.lang.String getJobId() {
java.lang.Object ref = jobId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobId_ = s;
return s;
}
}
/**
* string job_id = 15;
* @return The bytes for jobId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobIdBytes() {
java.lang.Object ref = jobId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OWNER_ID_FIELD_NUMBER = 16;
private long ownerId_;
/**
* int64 owner_id = 16;
* @return The ownerId.
*/
@java.lang.Override
public long getOwnerId() {
return ownerId_;
}
public static final int MIN_RUNNING_TIME_MS_FIELD_NUMBER = 17;
private long minRunningTimeMs_;
/**
* int64 min_running_time_ms = 17;
* @return The minRunningTimeMs.
*/
@java.lang.Override
public long getMinRunningTimeMs() {
return minRunningTimeMs_;
}
public static final int MAX_RUNNING_TIME_MS_FIELD_NUMBER = 18;
private long maxRunningTimeMs_;
/**
* int64 max_running_time_ms = 18;
* @return The maxRunningTimeMs.
*/
@java.lang.Override
public long getMaxRunningTimeMs() {
return maxRunningTimeMs_;
}
public static final int QUERY_TAG_FIELD_NUMBER = 19;
private volatile java.lang.Object queryTag_;
/**
* string query_tag = 19;
* @return The queryTag.
*/
@java.lang.Override
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
}
}
/**
* string query_tag = 19;
* @return The bytes for queryTag.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DETAIL_FIELD_NUMBER = 28;
private boolean detail_;
/**
* bool detail = 28;
* @return The detail.
*/
@java.lang.Override
public boolean getDetail() {
return detail_;
}
public static final int FORCE_FIELD_NUMBER = 29;
private boolean force_;
/**
* bool force = 29;
* @return The force.
*/
@java.lang.Override
public boolean getForce() {
return force_;
}
public static final int USER_AGENT_FIELD_NUMBER = 30;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 30;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (instanceId_ != 0L) {
output.writeInt64(3, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, virtualCluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobStatus_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, jobStatus_);
}
if (minStartTime_ != 0L) {
output.writeInt64(6, minStartTime_);
}
if (maxStartTime_ != 0L) {
output.writeInt64(7, maxStartTime_);
}
if (maxSize_ != 0L) {
output.writeInt64(9, maxSize_);
}
if (listInMeta_ != false) {
output.writeBool(10, listInMeta_);
}
if (offset_ != 0L) {
output.writeInt64(11, offset_);
}
if (orderBy_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.ORDER_BY_START_TIME.getNumber()) {
output.writeEnum(12, orderBy_);
}
if (ascending_ != false) {
output.writeBool(13, ascending_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, priorityString_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, jobId_);
}
if (ownerId_ != 0L) {
output.writeInt64(16, ownerId_);
}
if (minRunningTimeMs_ != 0L) {
output.writeInt64(17, minRunningTimeMs_);
}
if (maxRunningTimeMs_ != 0L) {
output.writeInt64(18, maxRunningTimeMs_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, queryTag_);
}
if (detail_ != false) {
output.writeBool(28, detail_);
}
if (force_ != false) {
output.writeBool(29, force_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, virtualCluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobStatus_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, jobStatus_);
}
if (minStartTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, minStartTime_);
}
if (maxStartTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, maxStartTime_);
}
if (maxSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, maxSize_);
}
if (listInMeta_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, listInMeta_);
}
if (offset_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, offset_);
}
if (orderBy_ != cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.ORDER_BY_START_TIME.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, orderBy_);
}
if (ascending_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, ascending_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, priorityString_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, jobId_);
}
if (ownerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(16, ownerId_);
}
if (minRunningTimeMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, minRunningTimeMs_);
}
if (maxRunningTimeMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, maxRunningTimeMs_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, queryTag_);
}
if (detail_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(28, detail_);
}
if (force_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(29, force_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getVirtualCluster()
.equals(other.getVirtualCluster())) return false;
if (!getJobStatus()
.equals(other.getJobStatus())) return false;
if (getMinStartTime()
!= other.getMinStartTime()) return false;
if (getMaxStartTime()
!= other.getMaxStartTime()) return false;
if (getMaxSize()
!= other.getMaxSize()) return false;
if (getListInMeta()
!= other.getListInMeta()) return false;
if (getOffset()
!= other.getOffset()) return false;
if (orderBy_ != other.orderBy_) return false;
if (getAscending()
!= other.getAscending()) return false;
if (!getPriorityString()
.equals(other.getPriorityString())) return false;
if (!getJobId()
.equals(other.getJobId())) return false;
if (getOwnerId()
!= other.getOwnerId()) return false;
if (getMinRunningTimeMs()
!= other.getMinRunningTimeMs()) return false;
if (getMaxRunningTimeMs()
!= other.getMaxRunningTimeMs()) return false;
if (!getQueryTag()
.equals(other.getQueryTag())) return false;
if (getDetail()
!= other.getDetail()) return false;
if (getForce()
!= other.getForce()) return false;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + VIRTUAL_CLUSTER_FIELD_NUMBER;
hash = (53 * hash) + getVirtualCluster().hashCode();
hash = (37 * hash) + JOB_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getJobStatus().hashCode();
hash = (37 * hash) + MIN_START_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinStartTime());
hash = (37 * hash) + MAX_START_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxStartTime());
hash = (37 * hash) + MAX_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxSize());
hash = (37 * hash) + LIST_IN_META_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getListInMeta());
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOffset());
hash = (37 * hash) + ORDER_BY_FIELD_NUMBER;
hash = (53 * hash) + orderBy_;
hash = (37 * hash) + ASCENDING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAscending());
hash = (37 * hash) + PRIORITY_STRING_FIELD_NUMBER;
hash = (53 * hash) + getPriorityString().hashCode();
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
hash = (37 * hash) + OWNER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOwnerId());
hash = (37 * hash) + MIN_RUNNING_TIME_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMinRunningTimeMs());
hash = (37 * hash) + MAX_RUNNING_TIME_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxRunningTimeMs());
hash = (37 * hash) + QUERY_TAG_FIELD_NUMBER;
hash = (53 * hash) + getQueryTag().hashCode();
hash = (37 * hash) + DETAIL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDetail());
hash = (37 * hash) + FORCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getForce());
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.ListJobsRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
workspace_ = "";
instanceId_ = 0L;
virtualCluster_ = "";
jobStatus_ = "";
minStartTime_ = 0L;
maxStartTime_ = 0L;
maxSize_ = 0L;
listInMeta_ = false;
offset_ = 0L;
orderBy_ = 0;
ascending_ = false;
priorityString_ = "";
jobId_ = "";
ownerId_ = 0L;
minRunningTimeMs_ = 0L;
maxRunningTimeMs_ = 0L;
queryTag_ = "";
detail_ = false;
force_ = false;
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
result.workspace_ = workspace_;
result.instanceId_ = instanceId_;
result.virtualCluster_ = virtualCluster_;
result.jobStatus_ = jobStatus_;
result.minStartTime_ = minStartTime_;
result.maxStartTime_ = maxStartTime_;
result.maxSize_ = maxSize_;
result.listInMeta_ = listInMeta_;
result.offset_ = offset_;
result.orderBy_ = orderBy_;
result.ascending_ = ascending_;
result.priorityString_ = priorityString_;
result.jobId_ = jobId_;
result.ownerId_ = ownerId_;
result.minRunningTimeMs_ = minRunningTimeMs_;
result.maxRunningTimeMs_ = maxRunningTimeMs_;
result.queryTag_ = queryTag_;
result.detail_ = detail_;
result.force_ = force_;
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getVirtualCluster().isEmpty()) {
virtualCluster_ = other.virtualCluster_;
onChanged();
}
if (!other.getJobStatus().isEmpty()) {
jobStatus_ = other.jobStatus_;
onChanged();
}
if (other.getMinStartTime() != 0L) {
setMinStartTime(other.getMinStartTime());
}
if (other.getMaxStartTime() != 0L) {
setMaxStartTime(other.getMaxStartTime());
}
if (other.getMaxSize() != 0L) {
setMaxSize(other.getMaxSize());
}
if (other.getListInMeta() != false) {
setListInMeta(other.getListInMeta());
}
if (other.getOffset() != 0L) {
setOffset(other.getOffset());
}
if (other.orderBy_ != 0) {
setOrderByValue(other.getOrderByValue());
}
if (other.getAscending() != false) {
setAscending(other.getAscending());
}
if (!other.getPriorityString().isEmpty()) {
priorityString_ = other.priorityString_;
onChanged();
}
if (!other.getJobId().isEmpty()) {
jobId_ = other.jobId_;
onChanged();
}
if (other.getOwnerId() != 0L) {
setOwnerId(other.getOwnerId());
}
if (other.getMinRunningTimeMs() != 0L) {
setMinRunningTimeMs(other.getMinRunningTimeMs());
}
if (other.getMaxRunningTimeMs() != 0L) {
setMaxRunningTimeMs(other.getMaxRunningTimeMs());
}
if (!other.getQueryTag().isEmpty()) {
queryTag_ = other.queryTag_;
onChanged();
}
if (other.getDetail() != false) {
setDetail(other.getDetail());
}
if (other.getForce() != false) {
setForce(other.getForce());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 3;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 3;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object virtualCluster_ = "";
/**
* string virtual_cluster = 4;
* @return The virtualCluster.
*/
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string virtual_cluster = 4;
* @return The bytes for virtualCluster.
*/
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string virtual_cluster = 4;
* @param value The virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualCluster(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualCluster_ = value;
onChanged();
return this;
}
/**
* string virtual_cluster = 4;
* @return This builder for chaining.
*/
public Builder clearVirtualCluster() {
virtualCluster_ = getDefaultInstance().getVirtualCluster();
onChanged();
return this;
}
/**
* string virtual_cluster = 4;
* @param value The bytes for virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualCluster_ = value;
onChanged();
return this;
}
private java.lang.Object jobStatus_ = "";
/**
* string job_status = 5;
* @return The jobStatus.
*/
public java.lang.String getJobStatus() {
java.lang.Object ref = jobStatus_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobStatus_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_status = 5;
* @return The bytes for jobStatus.
*/
public com.google.protobuf.ByteString
getJobStatusBytes() {
java.lang.Object ref = jobStatus_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_status = 5;
* @param value The jobStatus to set.
* @return This builder for chaining.
*/
public Builder setJobStatus(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobStatus_ = value;
onChanged();
return this;
}
/**
* string job_status = 5;
* @return This builder for chaining.
*/
public Builder clearJobStatus() {
jobStatus_ = getDefaultInstance().getJobStatus();
onChanged();
return this;
}
/**
* string job_status = 5;
* @param value The bytes for jobStatus to set.
* @return This builder for chaining.
*/
public Builder setJobStatusBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobStatus_ = value;
onChanged();
return this;
}
private long minStartTime_ ;
/**
* int64 min_start_time = 6;
* @return The minStartTime.
*/
@java.lang.Override
public long getMinStartTime() {
return minStartTime_;
}
/**
* int64 min_start_time = 6;
* @param value The minStartTime to set.
* @return This builder for chaining.
*/
public Builder setMinStartTime(long value) {
minStartTime_ = value;
onChanged();
return this;
}
/**
* int64 min_start_time = 6;
* @return This builder for chaining.
*/
public Builder clearMinStartTime() {
minStartTime_ = 0L;
onChanged();
return this;
}
private long maxStartTime_ ;
/**
* int64 max_start_time = 7;
* @return The maxStartTime.
*/
@java.lang.Override
public long getMaxStartTime() {
return maxStartTime_;
}
/**
* int64 max_start_time = 7;
* @param value The maxStartTime to set.
* @return This builder for chaining.
*/
public Builder setMaxStartTime(long value) {
maxStartTime_ = value;
onChanged();
return this;
}
/**
* int64 max_start_time = 7;
* @return This builder for chaining.
*/
public Builder clearMaxStartTime() {
maxStartTime_ = 0L;
onChanged();
return this;
}
private long maxSize_ ;
/**
* int64 max_size = 9;
* @return The maxSize.
*/
@java.lang.Override
public long getMaxSize() {
return maxSize_;
}
/**
* int64 max_size = 9;
* @param value The maxSize to set.
* @return This builder for chaining.
*/
public Builder setMaxSize(long value) {
maxSize_ = value;
onChanged();
return this;
}
/**
* int64 max_size = 9;
* @return This builder for chaining.
*/
public Builder clearMaxSize() {
maxSize_ = 0L;
onChanged();
return this;
}
private boolean listInMeta_ ;
/**
* bool list_in_meta = 10;
* @return The listInMeta.
*/
@java.lang.Override
public boolean getListInMeta() {
return listInMeta_;
}
/**
* bool list_in_meta = 10;
* @param value The listInMeta to set.
* @return This builder for chaining.
*/
public Builder setListInMeta(boolean value) {
listInMeta_ = value;
onChanged();
return this;
}
/**
* bool list_in_meta = 10;
* @return This builder for chaining.
*/
public Builder clearListInMeta() {
listInMeta_ = false;
onChanged();
return this;
}
private long offset_ ;
/**
* int64 offset = 11;
* @return The offset.
*/
@java.lang.Override
public long getOffset() {
return offset_;
}
/**
* int64 offset = 11;
* @param value The offset to set.
* @return This builder for chaining.
*/
public Builder setOffset(long value) {
offset_ = value;
onChanged();
return this;
}
/**
* int64 offset = 11;
* @return This builder for chaining.
*/
public Builder clearOffset() {
offset_ = 0L;
onChanged();
return this;
}
private int orderBy_ = 0;
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The enum numeric value on the wire for orderBy.
*/
@java.lang.Override public int getOrderByValue() {
return orderBy_;
}
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @param value The enum numeric value on the wire for orderBy to set.
* @return This builder for chaining.
*/
public Builder setOrderByValue(int value) {
orderBy_ = value;
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return The orderBy.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby getOrderBy() {
@SuppressWarnings("deprecation")
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby result = cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.valueOf(orderBy_);
return result == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby.UNRECOGNIZED : result;
}
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @param value The orderBy to set.
* @return This builder for chaining.
*/
public Builder setOrderBy(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest.ListOrderby value) {
if (value == null) {
throw new NullPointerException();
}
orderBy_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.coordinator.ListJobsRequest.ListOrderby order_by = 12;
* @return This builder for chaining.
*/
public Builder clearOrderBy() {
orderBy_ = 0;
onChanged();
return this;
}
private boolean ascending_ ;
/**
* bool ascending = 13;
* @return The ascending.
*/
@java.lang.Override
public boolean getAscending() {
return ascending_;
}
/**
* bool ascending = 13;
* @param value The ascending to set.
* @return This builder for chaining.
*/
public Builder setAscending(boolean value) {
ascending_ = value;
onChanged();
return this;
}
/**
* bool ascending = 13;
* @return This builder for chaining.
*/
public Builder clearAscending() {
ascending_ = false;
onChanged();
return this;
}
private java.lang.Object priorityString_ = "";
/**
* string priority_string = 14;
* @return The priorityString.
*/
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string priority_string = 14;
* @return The bytes for priorityString.
*/
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string priority_string = 14;
* @param value The priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
priorityString_ = value;
onChanged();
return this;
}
/**
* string priority_string = 14;
* @return This builder for chaining.
*/
public Builder clearPriorityString() {
priorityString_ = getDefaultInstance().getPriorityString();
onChanged();
return this;
}
/**
* string priority_string = 14;
* @param value The bytes for priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
priorityString_ = value;
onChanged();
return this;
}
private java.lang.Object jobId_ = "";
/**
* string job_id = 15;
* @return The jobId.
*/
public java.lang.String getJobId() {
java.lang.Object ref = jobId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_id = 15;
* @return The bytes for jobId.
*/
public com.google.protobuf.ByteString
getJobIdBytes() {
java.lang.Object ref = jobId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_id = 15;
* @param value The jobId to set.
* @return This builder for chaining.
*/
public Builder setJobId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
return this;
}
/**
* string job_id = 15;
* @return This builder for chaining.
*/
public Builder clearJobId() {
jobId_ = getDefaultInstance().getJobId();
onChanged();
return this;
}
/**
* string job_id = 15;
* @param value The bytes for jobId to set.
* @return This builder for chaining.
*/
public Builder setJobIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobId_ = value;
onChanged();
return this;
}
private long ownerId_ ;
/**
* int64 owner_id = 16;
* @return The ownerId.
*/
@java.lang.Override
public long getOwnerId() {
return ownerId_;
}
/**
* int64 owner_id = 16;
* @param value The ownerId to set.
* @return This builder for chaining.
*/
public Builder setOwnerId(long value) {
ownerId_ = value;
onChanged();
return this;
}
/**
* int64 owner_id = 16;
* @return This builder for chaining.
*/
public Builder clearOwnerId() {
ownerId_ = 0L;
onChanged();
return this;
}
private long minRunningTimeMs_ ;
/**
* int64 min_running_time_ms = 17;
* @return The minRunningTimeMs.
*/
@java.lang.Override
public long getMinRunningTimeMs() {
return minRunningTimeMs_;
}
/**
* int64 min_running_time_ms = 17;
* @param value The minRunningTimeMs to set.
* @return This builder for chaining.
*/
public Builder setMinRunningTimeMs(long value) {
minRunningTimeMs_ = value;
onChanged();
return this;
}
/**
* int64 min_running_time_ms = 17;
* @return This builder for chaining.
*/
public Builder clearMinRunningTimeMs() {
minRunningTimeMs_ = 0L;
onChanged();
return this;
}
private long maxRunningTimeMs_ ;
/**
* int64 max_running_time_ms = 18;
* @return The maxRunningTimeMs.
*/
@java.lang.Override
public long getMaxRunningTimeMs() {
return maxRunningTimeMs_;
}
/**
* int64 max_running_time_ms = 18;
* @param value The maxRunningTimeMs to set.
* @return This builder for chaining.
*/
public Builder setMaxRunningTimeMs(long value) {
maxRunningTimeMs_ = value;
onChanged();
return this;
}
/**
* int64 max_running_time_ms = 18;
* @return This builder for chaining.
*/
public Builder clearMaxRunningTimeMs() {
maxRunningTimeMs_ = 0L;
onChanged();
return this;
}
private java.lang.Object queryTag_ = "";
/**
* string query_tag = 19;
* @return The queryTag.
*/
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query_tag = 19;
* @return The bytes for queryTag.
*/
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query_tag = 19;
* @param value The queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
queryTag_ = value;
onChanged();
return this;
}
/**
* string query_tag = 19;
* @return This builder for chaining.
*/
public Builder clearQueryTag() {
queryTag_ = getDefaultInstance().getQueryTag();
onChanged();
return this;
}
/**
* string query_tag = 19;
* @param value The bytes for queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
queryTag_ = value;
onChanged();
return this;
}
private boolean detail_ ;
/**
* bool detail = 28;
* @return The detail.
*/
@java.lang.Override
public boolean getDetail() {
return detail_;
}
/**
* bool detail = 28;
* @param value The detail to set.
* @return This builder for chaining.
*/
public Builder setDetail(boolean value) {
detail_ = value;
onChanged();
return this;
}
/**
* bool detail = 28;
* @return This builder for chaining.
*/
public Builder clearDetail() {
detail_ = false;
onChanged();
return this;
}
private boolean force_ ;
/**
* bool force = 29;
* @return The force.
*/
@java.lang.Override
public boolean getForce() {
return force_;
}
/**
* bool force = 29;
* @param value The force to set.
* @return This builder for chaining.
*/
public Builder setForce(boolean value) {
force_ = value;
onChanged();
return this;
}
/**
* bool force = 29;
* @return This builder for chaining.
*/
public Builder clearForce() {
force_ = false;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 30;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 30;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 30;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 30;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.ListJobsRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.ListJobsRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListJobsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListJobsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ListJobsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.ListJobsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
java.util.List
getJobDetailsList();
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getJobDetails(int index);
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
int getJobDetailsCount();
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder>
getJobDetailsOrBuilderList();
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder getJobDetailsOrBuilder(
int index);
/**
* int64 total_job_count = 3;
* @return The totalJobCount.
*/
long getTotalJobCount();
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsResponse}
*/
public static final class ListJobsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.ListJobsResponse)
ListJobsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use ListJobsResponse.newBuilder() to construct.
private ListJobsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ListJobsResponse() {
jobDetails_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ListJobsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ListJobsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
jobDetails_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
jobDetails_.add(
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.parser(), extensionRegistry));
break;
}
case 24: {
totalJobCount_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
jobDetails_ = java.util.Collections.unmodifiableList(jobDetails_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.Builder.class);
}
public interface JobDetailOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.ListJobsResponse.JobDetail)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return The status.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus();
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder();
/**
* string job_name = 2;
* @return The jobName.
*/
java.lang.String getJobName();
/**
* string job_name = 2;
* @return The bytes for jobName.
*/
com.google.protobuf.ByteString
getJobNameBytes();
/**
* uint64 owner_id = 3;
* @return The ownerId.
*/
long getOwnerId();
/**
* uint32 priority = 4;
* @return The priority.
*/
int getPriority();
/**
* string job_type = 5;
* @return The jobType.
*/
java.lang.String getJobType();
/**
* string job_type = 5;
* @return The bytes for jobType.
*/
com.google.protobuf.ByteString
getJobTypeBytes();
/**
* repeated string statement = 6;
* @return A list containing the statement.
*/
java.util.List
getStatementList();
/**
* repeated string statement = 6;
* @return The count of statement.
*/
int getStatementCount();
/**
* repeated string statement = 6;
* @param index The index of the element to return.
* @return The statement at the given index.
*/
java.lang.String getStatement(int index);
/**
* repeated string statement = 6;
* @param index The index of the value to return.
* @return The bytes of the statement at the given index.
*/
com.google.protobuf.ByteString
getStatementBytes(int index);
/**
* uint64 input_bytes = 7;
* @return The inputBytes.
*/
long getInputBytes();
/**
* uint64 rows_produced = 8;
* @return The rowsProduced.
*/
long getRowsProduced();
/**
* string virtual_cluster = 9;
* @return The virtualCluster.
*/
java.lang.String getVirtualCluster();
/**
* string virtual_cluster = 9;
* @return The bytes for virtualCluster.
*/
com.google.protobuf.ByteString
getVirtualClusterBytes();
/**
* string priority_string = 10;
* @return The priorityString.
*/
java.lang.String getPriorityString();
/**
* string priority_string = 10;
* @return The bytes for priorityString.
*/
com.google.protobuf.ByteString
getPriorityStringBytes();
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
java.util.List
getJobHistoryList();
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
cz.proto.JobHistory getJobHistory(int index);
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
int getJobHistoryCount();
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoryOrBuilderList();
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
cz.proto.JobHistoryOrBuilder getJobHistoryOrBuilder(
int index);
/**
* string virtual_cluster_type = 14;
* @return The virtualClusterType.
*/
java.lang.String getVirtualClusterType();
/**
* string virtual_cluster_type = 14;
* @return The bytes for virtualClusterType.
*/
com.google.protobuf.ByteString
getVirtualClusterTypeBytes();
/**
* string virtual_cluster_size = 15;
* @return The virtualClusterSize.
*/
java.lang.String getVirtualClusterSize();
/**
* string virtual_cluster_size = 15;
* @return The bytes for virtualClusterSize.
*/
com.google.protobuf.ByteString
getVirtualClusterSizeBytes();
/**
* string query_tag = 16;
* @return The queryTag.
*/
java.lang.String getQueryTag();
/**
* string query_tag = 16;
* @return The bytes for queryTag.
*/
com.google.protobuf.ByteString
getQueryTagBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsResponse.JobDetail}
*/
public static final class JobDetail extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.ListJobsResponse.JobDetail)
JobDetailOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobDetail.newBuilder() to construct.
private JobDetail(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobDetail() {
jobName_ = "";
jobType_ = "";
statement_ = com.google.protobuf.LazyStringArrayList.EMPTY;
virtualCluster_ = "";
priorityString_ = "";
jobHistory_ = java.util.Collections.emptyList();
virtualClusterType_ = "";
virtualClusterSize_ = "";
queryTag_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobDetail();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobDetail(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
jobName_ = s;
break;
}
case 24: {
ownerId_ = input.readUInt64();
break;
}
case 32: {
priority_ = input.readUInt32();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
jobType_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
statement_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
statement_.add(s);
break;
}
case 56: {
inputBytes_ = input.readUInt64();
break;
}
case 64: {
rowsProduced_ = input.readUInt64();
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
virtualCluster_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
priorityString_ = s;
break;
}
case 98: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
jobHistory_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
jobHistory_.add(
input.readMessage(cz.proto.JobHistory.parser(), extensionRegistry));
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
virtualClusterType_ = s;
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
virtualClusterSize_ = s;
break;
}
case 130: {
java.lang.String s = input.readStringRequireUtf8();
queryTag_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
statement_ = statement_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
jobHistory_ = java.util.Collections.unmodifiableList(jobHistory_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_JobDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_JobDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int JOB_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object jobName_;
/**
* string job_name = 2;
* @return The jobName.
*/
@java.lang.Override
public java.lang.String getJobName() {
java.lang.Object ref = jobName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobName_ = s;
return s;
}
}
/**
* string job_name = 2;
* @return The bytes for jobName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobNameBytes() {
java.lang.Object ref = jobName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OWNER_ID_FIELD_NUMBER = 3;
private long ownerId_;
/**
* uint64 owner_id = 3;
* @return The ownerId.
*/
@java.lang.Override
public long getOwnerId() {
return ownerId_;
}
public static final int PRIORITY_FIELD_NUMBER = 4;
private int priority_;
/**
* uint32 priority = 4;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
public static final int JOB_TYPE_FIELD_NUMBER = 5;
private volatile java.lang.Object jobType_;
/**
* string job_type = 5;
* @return The jobType.
*/
@java.lang.Override
public java.lang.String getJobType() {
java.lang.Object ref = jobType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobType_ = s;
return s;
}
}
/**
* string job_type = 5;
* @return The bytes for jobType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobTypeBytes() {
java.lang.Object ref = jobType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATEMENT_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList statement_;
/**
* repeated string statement = 6;
* @return A list containing the statement.
*/
public com.google.protobuf.ProtocolStringList
getStatementList() {
return statement_;
}
/**
* repeated string statement = 6;
* @return The count of statement.
*/
public int getStatementCount() {
return statement_.size();
}
/**
* repeated string statement = 6;
* @param index The index of the element to return.
* @return The statement at the given index.
*/
public java.lang.String getStatement(int index) {
return statement_.get(index);
}
/**
* repeated string statement = 6;
* @param index The index of the value to return.
* @return The bytes of the statement at the given index.
*/
public com.google.protobuf.ByteString
getStatementBytes(int index) {
return statement_.getByteString(index);
}
public static final int INPUT_BYTES_FIELD_NUMBER = 7;
private long inputBytes_;
/**
* uint64 input_bytes = 7;
* @return The inputBytes.
*/
@java.lang.Override
public long getInputBytes() {
return inputBytes_;
}
public static final int ROWS_PRODUCED_FIELD_NUMBER = 8;
private long rowsProduced_;
/**
* uint64 rows_produced = 8;
* @return The rowsProduced.
*/
@java.lang.Override
public long getRowsProduced() {
return rowsProduced_;
}
public static final int VIRTUAL_CLUSTER_FIELD_NUMBER = 9;
private volatile java.lang.Object virtualCluster_;
/**
* string virtual_cluster = 9;
* @return The virtualCluster.
*/
@java.lang.Override
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
}
}
/**
* string virtual_cluster = 9;
* @return The bytes for virtualCluster.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRIORITY_STRING_FIELD_NUMBER = 10;
private volatile java.lang.Object priorityString_;
/**
* string priority_string = 10;
* @return The priorityString.
*/
@java.lang.Override
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
}
}
/**
* string priority_string = 10;
* @return The bytes for priorityString.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_HISTORY_FIELD_NUMBER = 12;
private java.util.List jobHistory_;
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
@java.lang.Override
public java.util.List getJobHistoryList() {
return jobHistory_;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
@java.lang.Override
public java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoryOrBuilderList() {
return jobHistory_;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
@java.lang.Override
public int getJobHistoryCount() {
return jobHistory_.size();
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
@java.lang.Override
public cz.proto.JobHistory getJobHistory(int index) {
return jobHistory_.get(index);
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
@java.lang.Override
public cz.proto.JobHistoryOrBuilder getJobHistoryOrBuilder(
int index) {
return jobHistory_.get(index);
}
public static final int VIRTUAL_CLUSTER_TYPE_FIELD_NUMBER = 14;
private volatile java.lang.Object virtualClusterType_;
/**
* string virtual_cluster_type = 14;
* @return The virtualClusterType.
*/
@java.lang.Override
public java.lang.String getVirtualClusterType() {
java.lang.Object ref = virtualClusterType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualClusterType_ = s;
return s;
}
}
/**
* string virtual_cluster_type = 14;
* @return The bytes for virtualClusterType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualClusterTypeBytes() {
java.lang.Object ref = virtualClusterType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualClusterType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VIRTUAL_CLUSTER_SIZE_FIELD_NUMBER = 15;
private volatile java.lang.Object virtualClusterSize_;
/**
* string virtual_cluster_size = 15;
* @return The virtualClusterSize.
*/
@java.lang.Override
public java.lang.String getVirtualClusterSize() {
java.lang.Object ref = virtualClusterSize_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualClusterSize_ = s;
return s;
}
}
/**
* string virtual_cluster_size = 15;
* @return The bytes for virtualClusterSize.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualClusterSizeBytes() {
java.lang.Object ref = virtualClusterSize_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualClusterSize_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUERY_TAG_FIELD_NUMBER = 16;
private volatile java.lang.Object queryTag_;
/**
* string query_tag = 16;
* @return The queryTag.
*/
@java.lang.Override
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
}
}
/**
* string query_tag = 16;
* @return The bytes for queryTag.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, jobName_);
}
if (ownerId_ != 0L) {
output.writeUInt64(3, ownerId_);
}
if (priority_ != 0) {
output.writeUInt32(4, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, jobType_);
}
for (int i = 0; i < statement_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, statement_.getRaw(i));
}
if (inputBytes_ != 0L) {
output.writeUInt64(7, inputBytes_);
}
if (rowsProduced_ != 0L) {
output.writeUInt64(8, rowsProduced_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, virtualCluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, priorityString_);
}
for (int i = 0; i < jobHistory_.size(); i++) {
output.writeMessage(12, jobHistory_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualClusterType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, virtualClusterType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualClusterSize_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, virtualClusterSize_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, queryTag_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, jobName_);
}
if (ownerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, ownerId_);
}
if (priority_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, jobType_);
}
{
int dataSize = 0;
for (int i = 0; i < statement_.size(); i++) {
dataSize += computeStringSizeNoTag(statement_.getRaw(i));
}
size += dataSize;
size += 1 * getStatementList().size();
}
if (inputBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, inputBytes_);
}
if (rowsProduced_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(8, rowsProduced_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualCluster_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, virtualCluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(priorityString_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, priorityString_);
}
for (int i = 0; i < jobHistory_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, jobHistory_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualClusterType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, virtualClusterType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualClusterSize_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, virtualClusterSize_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(queryTag_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, queryTag_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail other = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!getJobName()
.equals(other.getJobName())) return false;
if (getOwnerId()
!= other.getOwnerId()) return false;
if (getPriority()
!= other.getPriority()) return false;
if (!getJobType()
.equals(other.getJobType())) return false;
if (!getStatementList()
.equals(other.getStatementList())) return false;
if (getInputBytes()
!= other.getInputBytes()) return false;
if (getRowsProduced()
!= other.getRowsProduced()) return false;
if (!getVirtualCluster()
.equals(other.getVirtualCluster())) return false;
if (!getPriorityString()
.equals(other.getPriorityString())) return false;
if (!getJobHistoryList()
.equals(other.getJobHistoryList())) return false;
if (!getVirtualClusterType()
.equals(other.getVirtualClusterType())) return false;
if (!getVirtualClusterSize()
.equals(other.getVirtualClusterSize())) return false;
if (!getQueryTag()
.equals(other.getQueryTag())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (37 * hash) + JOB_NAME_FIELD_NUMBER;
hash = (53 * hash) + getJobName().hashCode();
hash = (37 * hash) + OWNER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOwnerId());
hash = (37 * hash) + PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getPriority();
hash = (37 * hash) + JOB_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getJobType().hashCode();
if (getStatementCount() > 0) {
hash = (37 * hash) + STATEMENT_FIELD_NUMBER;
hash = (53 * hash) + getStatementList().hashCode();
}
hash = (37 * hash) + INPUT_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInputBytes());
hash = (37 * hash) + ROWS_PRODUCED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRowsProduced());
hash = (37 * hash) + VIRTUAL_CLUSTER_FIELD_NUMBER;
hash = (53 * hash) + getVirtualCluster().hashCode();
hash = (37 * hash) + PRIORITY_STRING_FIELD_NUMBER;
hash = (53 * hash) + getPriorityString().hashCode();
if (getJobHistoryCount() > 0) {
hash = (37 * hash) + JOB_HISTORY_FIELD_NUMBER;
hash = (53 * hash) + getJobHistoryList().hashCode();
}
hash = (37 * hash) + VIRTUAL_CLUSTER_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getVirtualClusterType().hashCode();
hash = (37 * hash) + VIRTUAL_CLUSTER_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getVirtualClusterSize().hashCode();
hash = (37 * hash) + QUERY_TAG_FIELD_NUMBER;
hash = (53 * hash) + getQueryTag().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsResponse.JobDetail}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.ListJobsResponse.JobDetail)
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_JobDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_JobDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getJobHistoryFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
jobName_ = "";
ownerId_ = 0L;
priority_ = 0;
jobType_ = "";
statement_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
inputBytes_ = 0L;
rowsProduced_ = 0L;
virtualCluster_ = "";
priorityString_ = "";
if (jobHistoryBuilder_ == null) {
jobHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
jobHistoryBuilder_.clear();
}
virtualClusterType_ = "";
virtualClusterSize_ = "";
queryTag_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_JobDetail_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail result = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail(this);
int from_bitField0_ = bitField0_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.jobName_ = jobName_;
result.ownerId_ = ownerId_;
result.priority_ = priority_;
result.jobType_ = jobType_;
if (((bitField0_ & 0x00000001) != 0)) {
statement_ = statement_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.statement_ = statement_;
result.inputBytes_ = inputBytes_;
result.rowsProduced_ = rowsProduced_;
result.virtualCluster_ = virtualCluster_;
result.priorityString_ = priorityString_;
if (jobHistoryBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
jobHistory_ = java.util.Collections.unmodifiableList(jobHistory_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.jobHistory_ = jobHistory_;
} else {
result.jobHistory_ = jobHistoryBuilder_.build();
}
result.virtualClusterType_ = virtualClusterType_;
result.virtualClusterSize_ = virtualClusterSize_;
result.queryTag_ = queryTag_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (!other.getJobName().isEmpty()) {
jobName_ = other.jobName_;
onChanged();
}
if (other.getOwnerId() != 0L) {
setOwnerId(other.getOwnerId());
}
if (other.getPriority() != 0) {
setPriority(other.getPriority());
}
if (!other.getJobType().isEmpty()) {
jobType_ = other.jobType_;
onChanged();
}
if (!other.statement_.isEmpty()) {
if (statement_.isEmpty()) {
statement_ = other.statement_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureStatementIsMutable();
statement_.addAll(other.statement_);
}
onChanged();
}
if (other.getInputBytes() != 0L) {
setInputBytes(other.getInputBytes());
}
if (other.getRowsProduced() != 0L) {
setRowsProduced(other.getRowsProduced());
}
if (!other.getVirtualCluster().isEmpty()) {
virtualCluster_ = other.virtualCluster_;
onChanged();
}
if (!other.getPriorityString().isEmpty()) {
priorityString_ = other.priorityString_;
onChanged();
}
if (jobHistoryBuilder_ == null) {
if (!other.jobHistory_.isEmpty()) {
if (jobHistory_.isEmpty()) {
jobHistory_ = other.jobHistory_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureJobHistoryIsMutable();
jobHistory_.addAll(other.jobHistory_);
}
onChanged();
}
} else {
if (!other.jobHistory_.isEmpty()) {
if (jobHistoryBuilder_.isEmpty()) {
jobHistoryBuilder_.dispose();
jobHistoryBuilder_ = null;
jobHistory_ = other.jobHistory_;
bitField0_ = (bitField0_ & ~0x00000002);
jobHistoryBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getJobHistoryFieldBuilder() : null;
} else {
jobHistoryBuilder_.addAllMessages(other.jobHistory_);
}
}
}
if (!other.getVirtualClusterType().isEmpty()) {
virtualClusterType_ = other.virtualClusterType_;
onChanged();
}
if (!other.getVirtualClusterSize().isEmpty()) {
virtualClusterSize_ = other.virtualClusterSize_;
onChanged();
}
if (!other.getQueryTag().isEmpty()) {
queryTag_ = other.queryTag_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
* @return The status.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public Builder setStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public Builder setStatus(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public Builder mergeStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.coordinator.JobStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private java.lang.Object jobName_ = "";
/**
* string job_name = 2;
* @return The jobName.
*/
public java.lang.String getJobName() {
java.lang.Object ref = jobName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_name = 2;
* @return The bytes for jobName.
*/
public com.google.protobuf.ByteString
getJobNameBytes() {
java.lang.Object ref = jobName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_name = 2;
* @param value The jobName to set.
* @return This builder for chaining.
*/
public Builder setJobName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobName_ = value;
onChanged();
return this;
}
/**
* string job_name = 2;
* @return This builder for chaining.
*/
public Builder clearJobName() {
jobName_ = getDefaultInstance().getJobName();
onChanged();
return this;
}
/**
* string job_name = 2;
* @param value The bytes for jobName to set.
* @return This builder for chaining.
*/
public Builder setJobNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobName_ = value;
onChanged();
return this;
}
private long ownerId_ ;
/**
* uint64 owner_id = 3;
* @return The ownerId.
*/
@java.lang.Override
public long getOwnerId() {
return ownerId_;
}
/**
* uint64 owner_id = 3;
* @param value The ownerId to set.
* @return This builder for chaining.
*/
public Builder setOwnerId(long value) {
ownerId_ = value;
onChanged();
return this;
}
/**
* uint64 owner_id = 3;
* @return This builder for chaining.
*/
public Builder clearOwnerId() {
ownerId_ = 0L;
onChanged();
return this;
}
private int priority_ ;
/**
* uint32 priority = 4;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
/**
* uint32 priority = 4;
* @param value The priority to set.
* @return This builder for chaining.
*/
public Builder setPriority(int value) {
priority_ = value;
onChanged();
return this;
}
/**
* uint32 priority = 4;
* @return This builder for chaining.
*/
public Builder clearPriority() {
priority_ = 0;
onChanged();
return this;
}
private java.lang.Object jobType_ = "";
/**
* string job_type = 5;
* @return The jobType.
*/
public java.lang.String getJobType() {
java.lang.Object ref = jobType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_type = 5;
* @return The bytes for jobType.
*/
public com.google.protobuf.ByteString
getJobTypeBytes() {
java.lang.Object ref = jobType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_type = 5;
* @param value The jobType to set.
* @return This builder for chaining.
*/
public Builder setJobType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobType_ = value;
onChanged();
return this;
}
/**
* string job_type = 5;
* @return This builder for chaining.
*/
public Builder clearJobType() {
jobType_ = getDefaultInstance().getJobType();
onChanged();
return this;
}
/**
* string job_type = 5;
* @param value The bytes for jobType to set.
* @return This builder for chaining.
*/
public Builder setJobTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobType_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList statement_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureStatementIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
statement_ = new com.google.protobuf.LazyStringArrayList(statement_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string statement = 6;
* @return A list containing the statement.
*/
public com.google.protobuf.ProtocolStringList
getStatementList() {
return statement_.getUnmodifiableView();
}
/**
* repeated string statement = 6;
* @return The count of statement.
*/
public int getStatementCount() {
return statement_.size();
}
/**
* repeated string statement = 6;
* @param index The index of the element to return.
* @return The statement at the given index.
*/
public java.lang.String getStatement(int index) {
return statement_.get(index);
}
/**
* repeated string statement = 6;
* @param index The index of the value to return.
* @return The bytes of the statement at the given index.
*/
public com.google.protobuf.ByteString
getStatementBytes(int index) {
return statement_.getByteString(index);
}
/**
* repeated string statement = 6;
* @param index The index to set the value at.
* @param value The statement to set.
* @return This builder for chaining.
*/
public Builder setStatement(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStatementIsMutable();
statement_.set(index, value);
onChanged();
return this;
}
/**
* repeated string statement = 6;
* @param value The statement to add.
* @return This builder for chaining.
*/
public Builder addStatement(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureStatementIsMutable();
statement_.add(value);
onChanged();
return this;
}
/**
* repeated string statement = 6;
* @param values The statement to add.
* @return This builder for chaining.
*/
public Builder addAllStatement(
java.lang.Iterable values) {
ensureStatementIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, statement_);
onChanged();
return this;
}
/**
* repeated string statement = 6;
* @return This builder for chaining.
*/
public Builder clearStatement() {
statement_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string statement = 6;
* @param value The bytes of the statement to add.
* @return This builder for chaining.
*/
public Builder addStatementBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureStatementIsMutable();
statement_.add(value);
onChanged();
return this;
}
private long inputBytes_ ;
/**
* uint64 input_bytes = 7;
* @return The inputBytes.
*/
@java.lang.Override
public long getInputBytes() {
return inputBytes_;
}
/**
* uint64 input_bytes = 7;
* @param value The inputBytes to set.
* @return This builder for chaining.
*/
public Builder setInputBytes(long value) {
inputBytes_ = value;
onChanged();
return this;
}
/**
* uint64 input_bytes = 7;
* @return This builder for chaining.
*/
public Builder clearInputBytes() {
inputBytes_ = 0L;
onChanged();
return this;
}
private long rowsProduced_ ;
/**
* uint64 rows_produced = 8;
* @return The rowsProduced.
*/
@java.lang.Override
public long getRowsProduced() {
return rowsProduced_;
}
/**
* uint64 rows_produced = 8;
* @param value The rowsProduced to set.
* @return This builder for chaining.
*/
public Builder setRowsProduced(long value) {
rowsProduced_ = value;
onChanged();
return this;
}
/**
* uint64 rows_produced = 8;
* @return This builder for chaining.
*/
public Builder clearRowsProduced() {
rowsProduced_ = 0L;
onChanged();
return this;
}
private java.lang.Object virtualCluster_ = "";
/**
* string virtual_cluster = 9;
* @return The virtualCluster.
*/
public java.lang.String getVirtualCluster() {
java.lang.Object ref = virtualCluster_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualCluster_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string virtual_cluster = 9;
* @return The bytes for virtualCluster.
*/
public com.google.protobuf.ByteString
getVirtualClusterBytes() {
java.lang.Object ref = virtualCluster_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualCluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string virtual_cluster = 9;
* @param value The virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualCluster(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualCluster_ = value;
onChanged();
return this;
}
/**
* string virtual_cluster = 9;
* @return This builder for chaining.
*/
public Builder clearVirtualCluster() {
virtualCluster_ = getDefaultInstance().getVirtualCluster();
onChanged();
return this;
}
/**
* string virtual_cluster = 9;
* @param value The bytes for virtualCluster to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualCluster_ = value;
onChanged();
return this;
}
private java.lang.Object priorityString_ = "";
/**
* string priority_string = 10;
* @return The priorityString.
*/
public java.lang.String getPriorityString() {
java.lang.Object ref = priorityString_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
priorityString_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string priority_string = 10;
* @return The bytes for priorityString.
*/
public com.google.protobuf.ByteString
getPriorityStringBytes() {
java.lang.Object ref = priorityString_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
priorityString_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string priority_string = 10;
* @param value The priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityString(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
priorityString_ = value;
onChanged();
return this;
}
/**
* string priority_string = 10;
* @return This builder for chaining.
*/
public Builder clearPriorityString() {
priorityString_ = getDefaultInstance().getPriorityString();
onChanged();
return this;
}
/**
* string priority_string = 10;
* @param value The bytes for priorityString to set.
* @return This builder for chaining.
*/
public Builder setPriorityStringBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
priorityString_ = value;
onChanged();
return this;
}
private java.util.List jobHistory_ =
java.util.Collections.emptyList();
private void ensureJobHistoryIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
jobHistory_ = new java.util.ArrayList(jobHistory_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder> jobHistoryBuilder_;
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public java.util.List getJobHistoryList() {
if (jobHistoryBuilder_ == null) {
return java.util.Collections.unmodifiableList(jobHistory_);
} else {
return jobHistoryBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public int getJobHistoryCount() {
if (jobHistoryBuilder_ == null) {
return jobHistory_.size();
} else {
return jobHistoryBuilder_.getCount();
}
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public cz.proto.JobHistory getJobHistory(int index) {
if (jobHistoryBuilder_ == null) {
return jobHistory_.get(index);
} else {
return jobHistoryBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder setJobHistory(
int index, cz.proto.JobHistory value) {
if (jobHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoryIsMutable();
jobHistory_.set(index, value);
onChanged();
} else {
jobHistoryBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder setJobHistory(
int index, cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoryBuilder_ == null) {
ensureJobHistoryIsMutable();
jobHistory_.set(index, builderForValue.build());
onChanged();
} else {
jobHistoryBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder addJobHistory(cz.proto.JobHistory value) {
if (jobHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoryIsMutable();
jobHistory_.add(value);
onChanged();
} else {
jobHistoryBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder addJobHistory(
int index, cz.proto.JobHistory value) {
if (jobHistoryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoryIsMutable();
jobHistory_.add(index, value);
onChanged();
} else {
jobHistoryBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder addJobHistory(
cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoryBuilder_ == null) {
ensureJobHistoryIsMutable();
jobHistory_.add(builderForValue.build());
onChanged();
} else {
jobHistoryBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder addJobHistory(
int index, cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoryBuilder_ == null) {
ensureJobHistoryIsMutable();
jobHistory_.add(index, builderForValue.build());
onChanged();
} else {
jobHistoryBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder addAllJobHistory(
java.lang.Iterable extends cz.proto.JobHistory> values) {
if (jobHistoryBuilder_ == null) {
ensureJobHistoryIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jobHistory_);
onChanged();
} else {
jobHistoryBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder clearJobHistory() {
if (jobHistoryBuilder_ == null) {
jobHistory_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
jobHistoryBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public Builder removeJobHistory(int index) {
if (jobHistoryBuilder_ == null) {
ensureJobHistoryIsMutable();
jobHistory_.remove(index);
onChanged();
} else {
jobHistoryBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public cz.proto.JobHistory.Builder getJobHistoryBuilder(
int index) {
return getJobHistoryFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public cz.proto.JobHistoryOrBuilder getJobHistoryOrBuilder(
int index) {
if (jobHistoryBuilder_ == null) {
return jobHistory_.get(index); } else {
return jobHistoryBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoryOrBuilderList() {
if (jobHistoryBuilder_ != null) {
return jobHistoryBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(jobHistory_);
}
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public cz.proto.JobHistory.Builder addJobHistoryBuilder() {
return getJobHistoryFieldBuilder().addBuilder(
cz.proto.JobHistory.getDefaultInstance());
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public cz.proto.JobHistory.Builder addJobHistoryBuilder(
int index) {
return getJobHistoryFieldBuilder().addBuilder(
index, cz.proto.JobHistory.getDefaultInstance());
}
/**
* repeated .cz.proto.JobHistory job_history = 12;
*/
public java.util.List
getJobHistoryBuilderList() {
return getJobHistoryFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder>
getJobHistoryFieldBuilder() {
if (jobHistoryBuilder_ == null) {
jobHistoryBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder>(
jobHistory_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
jobHistory_ = null;
}
return jobHistoryBuilder_;
}
private java.lang.Object virtualClusterType_ = "";
/**
* string virtual_cluster_type = 14;
* @return The virtualClusterType.
*/
public java.lang.String getVirtualClusterType() {
java.lang.Object ref = virtualClusterType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualClusterType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string virtual_cluster_type = 14;
* @return The bytes for virtualClusterType.
*/
public com.google.protobuf.ByteString
getVirtualClusterTypeBytes() {
java.lang.Object ref = virtualClusterType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualClusterType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string virtual_cluster_type = 14;
* @param value The virtualClusterType to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualClusterType_ = value;
onChanged();
return this;
}
/**
* string virtual_cluster_type = 14;
* @return This builder for chaining.
*/
public Builder clearVirtualClusterType() {
virtualClusterType_ = getDefaultInstance().getVirtualClusterType();
onChanged();
return this;
}
/**
* string virtual_cluster_type = 14;
* @param value The bytes for virtualClusterType to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualClusterType_ = value;
onChanged();
return this;
}
private java.lang.Object virtualClusterSize_ = "";
/**
* string virtual_cluster_size = 15;
* @return The virtualClusterSize.
*/
public java.lang.String getVirtualClusterSize() {
java.lang.Object ref = virtualClusterSize_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualClusterSize_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string virtual_cluster_size = 15;
* @return The bytes for virtualClusterSize.
*/
public com.google.protobuf.ByteString
getVirtualClusterSizeBytes() {
java.lang.Object ref = virtualClusterSize_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualClusterSize_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string virtual_cluster_size = 15;
* @param value The virtualClusterSize to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterSize(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualClusterSize_ = value;
onChanged();
return this;
}
/**
* string virtual_cluster_size = 15;
* @return This builder for chaining.
*/
public Builder clearVirtualClusterSize() {
virtualClusterSize_ = getDefaultInstance().getVirtualClusterSize();
onChanged();
return this;
}
/**
* string virtual_cluster_size = 15;
* @param value The bytes for virtualClusterSize to set.
* @return This builder for chaining.
*/
public Builder setVirtualClusterSizeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualClusterSize_ = value;
onChanged();
return this;
}
private java.lang.Object queryTag_ = "";
/**
* string query_tag = 16;
* @return The queryTag.
*/
public java.lang.String getQueryTag() {
java.lang.Object ref = queryTag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
queryTag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string query_tag = 16;
* @return The bytes for queryTag.
*/
public com.google.protobuf.ByteString
getQueryTagBytes() {
java.lang.Object ref = queryTag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
queryTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string query_tag = 16;
* @param value The queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
queryTag_ = value;
onChanged();
return this;
}
/**
* string query_tag = 16;
* @return This builder for chaining.
*/
public Builder clearQueryTag() {
queryTag_ = getDefaultInstance().getQueryTag();
onChanged();
return this;
}
/**
* string query_tag = 16;
* @param value The bytes for queryTag to set.
* @return This builder for chaining.
*/
public Builder setQueryTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
queryTag_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.ListJobsResponse.JobDetail)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.ListJobsResponse.JobDetail)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobDetail parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobDetail(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int JOB_DETAILS_FIELD_NUMBER = 2;
private java.util.List jobDetails_;
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
@java.lang.Override
public java.util.List getJobDetailsList() {
return jobDetails_;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
@java.lang.Override
public java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder>
getJobDetailsOrBuilderList() {
return jobDetails_;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
@java.lang.Override
public int getJobDetailsCount() {
return jobDetails_.size();
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getJobDetails(int index) {
return jobDetails_.get(index);
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder getJobDetailsOrBuilder(
int index) {
return jobDetails_.get(index);
}
public static final int TOTAL_JOB_COUNT_FIELD_NUMBER = 3;
private long totalJobCount_;
/**
* int64 total_job_count = 3;
* @return The totalJobCount.
*/
@java.lang.Override
public long getTotalJobCount() {
return totalJobCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
for (int i = 0; i < jobDetails_.size(); i++) {
output.writeMessage(2, jobDetails_.get(i));
}
if (totalJobCount_ != 0L) {
output.writeInt64(3, totalJobCount_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
for (int i = 0; i < jobDetails_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, jobDetails_.get(i));
}
if (totalJobCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, totalJobCount_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (!getJobDetailsList()
.equals(other.getJobDetailsList())) return false;
if (getTotalJobCount()
!= other.getTotalJobCount()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (getJobDetailsCount() > 0) {
hash = (37 * hash) + JOB_DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getJobDetailsList().hashCode();
}
hash = (37 * hash) + TOTAL_JOB_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTotalJobCount());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.ListJobsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.ListJobsResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getJobDetailsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (jobDetailsBuilder_ == null) {
jobDetails_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
jobDetailsBuilder_.clear();
}
totalJobCount_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_ListJobsResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse(this);
int from_bitField0_ = bitField0_;
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (jobDetailsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
jobDetails_ = java.util.Collections.unmodifiableList(jobDetails_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.jobDetails_ = jobDetails_;
} else {
result.jobDetails_ = jobDetailsBuilder_.build();
}
result.totalJobCount_ = totalJobCount_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (jobDetailsBuilder_ == null) {
if (!other.jobDetails_.isEmpty()) {
if (jobDetails_.isEmpty()) {
jobDetails_ = other.jobDetails_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureJobDetailsIsMutable();
jobDetails_.addAll(other.jobDetails_);
}
onChanged();
}
} else {
if (!other.jobDetails_.isEmpty()) {
if (jobDetailsBuilder_.isEmpty()) {
jobDetailsBuilder_.dispose();
jobDetailsBuilder_ = null;
jobDetails_ = other.jobDetails_;
bitField0_ = (bitField0_ & ~0x00000001);
jobDetailsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getJobDetailsFieldBuilder() : null;
} else {
jobDetailsBuilder_.addAllMessages(other.jobDetails_);
}
}
}
if (other.getTotalJobCount() != 0L) {
setTotalJobCount(other.getTotalJobCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private java.util.List jobDetails_ =
java.util.Collections.emptyList();
private void ensureJobDetailsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
jobDetails_ = new java.util.ArrayList(jobDetails_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder> jobDetailsBuilder_;
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public java.util.List getJobDetailsList() {
if (jobDetailsBuilder_ == null) {
return java.util.Collections.unmodifiableList(jobDetails_);
} else {
return jobDetailsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public int getJobDetailsCount() {
if (jobDetailsBuilder_ == null) {
return jobDetails_.size();
} else {
return jobDetailsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail getJobDetails(int index) {
if (jobDetailsBuilder_ == null) {
return jobDetails_.get(index);
} else {
return jobDetailsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder setJobDetails(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail value) {
if (jobDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobDetailsIsMutable();
jobDetails_.set(index, value);
onChanged();
} else {
jobDetailsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder setJobDetails(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder builderForValue) {
if (jobDetailsBuilder_ == null) {
ensureJobDetailsIsMutable();
jobDetails_.set(index, builderForValue.build());
onChanged();
} else {
jobDetailsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder addJobDetails(cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail value) {
if (jobDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobDetailsIsMutable();
jobDetails_.add(value);
onChanged();
} else {
jobDetailsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder addJobDetails(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail value) {
if (jobDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobDetailsIsMutable();
jobDetails_.add(index, value);
onChanged();
} else {
jobDetailsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder addJobDetails(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder builderForValue) {
if (jobDetailsBuilder_ == null) {
ensureJobDetailsIsMutable();
jobDetails_.add(builderForValue.build());
onChanged();
} else {
jobDetailsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder addJobDetails(
int index, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder builderForValue) {
if (jobDetailsBuilder_ == null) {
ensureJobDetailsIsMutable();
jobDetails_.add(index, builderForValue.build());
onChanged();
} else {
jobDetailsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder addAllJobDetails(
java.lang.Iterable extends cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail> values) {
if (jobDetailsBuilder_ == null) {
ensureJobDetailsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jobDetails_);
onChanged();
} else {
jobDetailsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder clearJobDetails() {
if (jobDetailsBuilder_ == null) {
jobDetails_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
jobDetailsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public Builder removeJobDetails(int index) {
if (jobDetailsBuilder_ == null) {
ensureJobDetailsIsMutable();
jobDetails_.remove(index);
onChanged();
} else {
jobDetailsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder getJobDetailsBuilder(
int index) {
return getJobDetailsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder getJobDetailsOrBuilder(
int index) {
if (jobDetailsBuilder_ == null) {
return jobDetails_.get(index); } else {
return jobDetailsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder>
getJobDetailsOrBuilderList() {
if (jobDetailsBuilder_ != null) {
return jobDetailsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(jobDetails_);
}
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder addJobDetailsBuilder() {
return getJobDetailsFieldBuilder().addBuilder(
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.getDefaultInstance());
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder addJobDetailsBuilder(
int index) {
return getJobDetailsFieldBuilder().addBuilder(
index, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.getDefaultInstance());
}
/**
* repeated .cz.proto.coordinator.ListJobsResponse.JobDetail job_details = 2;
*/
public java.util.List
getJobDetailsBuilderList() {
return getJobDetailsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder>
getJobDetailsFieldBuilder() {
if (jobDetailsBuilder_ == null) {
jobDetailsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetail.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse.JobDetailOrBuilder>(
jobDetails_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
jobDetails_ = null;
}
return jobDetailsBuilder_;
}
private long totalJobCount_ ;
/**
* int64 total_job_count = 3;
* @return The totalJobCount.
*/
@java.lang.Override
public long getTotalJobCount() {
return totalJobCount_;
}
/**
* int64 total_job_count = 3;
* @param value The totalJobCount to set.
* @return This builder for chaining.
*/
public Builder setTotalJobCount(long value) {
totalJobCount_ = value;
onChanged();
return this;
}
/**
* int64 total_job_count = 3;
* @return This builder for chaining.
*/
public Builder clearTotalJobCount() {
totalJobCount_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.ListJobsResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.ListJobsResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ListJobsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ListJobsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.ListJobsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CancelJobRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.CancelJobRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
*
* TODO(LYF): make it better
*
*
* bool force = 3;
* @return The force.
*/
boolean getForce();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.CancelJobRequest}
*/
public static final class CancelJobRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.CancelJobRequest)
CancelJobRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CancelJobRequest.newBuilder() to construct.
private CancelJobRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CancelJobRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CancelJobRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CancelJobRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 24: {
force_ = input.readBool();
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int FORCE_FIELD_NUMBER = 3;
private boolean force_;
/**
*
* TODO(LYF): make it better
*
*
* bool force = 3;
* @return The force.
*/
@java.lang.Override
public boolean getForce() {
return force_;
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (force_ != false) {
output.writeBool(3, force_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (force_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, force_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (getForce()
!= other.getForce()) return false;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + FORCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getForce());
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.CancelJobRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.CancelJobRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
force_ = false;
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.force_ = force_;
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (other.getForce() != false) {
setForce(other.getForce());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private boolean force_ ;
/**
*
* TODO(LYF): make it better
*
*
* bool force = 3;
* @return The force.
*/
@java.lang.Override
public boolean getForce() {
return force_;
}
/**
*
* TODO(LYF): make it better
*
*
* bool force = 3;
* @param value The force to set.
* @return This builder for chaining.
*/
public Builder setForce(boolean value) {
force_ = value;
onChanged();
return this;
}
/**
*
* TODO(LYF): make it better
*
*
* bool force = 3;
* @return This builder for chaining.
*/
public Builder clearForce() {
force_ = false;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.CancelJobRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.CancelJobRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CancelJobRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CancelJobRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CancelJobResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.CancelJobResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.CancelJobResponse}
*/
public static final class CancelJobResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.CancelJobResponse)
CancelJobResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CancelJobResponse.newBuilder() to construct.
private CancelJobResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CancelJobResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CancelJobResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CancelJobResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.CancelJobResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.CancelJobResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_CancelJobResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.CancelJobResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.CancelJobResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CancelJobResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CancelJobResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.CancelJobResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobRequest}
*/
public static final class GetJobRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobRequest)
GetJobRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobRequest.newBuilder() to construct.
private GetJobRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
boolean hasJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResponse}
*/
public static final class GetJobResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobResponse)
GetJobResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobResponse.newBuilder() to construct.
private GetJobResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder subBuilder = null;
if (jobDesc_ != null) {
subBuilder = jobDesc_.toBuilder();
}
jobDesc_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobDesc_);
jobDesc_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int JOB_DESC_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
@java.lang.Override
public boolean hasJobDesc() {
return jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
return getJobDesc();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (jobDesc_ != null) {
output.writeMessage(2, getJobDesc());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (jobDesc_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobDesc());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasJobDesc() != other.hasJobDesc()) return false;
if (hasJobDesc()) {
if (!getJobDesc()
.equals(other.getJobDesc())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasJobDesc()) {
hash = (37 * hash) + JOB_DESC_FIELD_NUMBER;
hash = (53 * hash) + getJobDesc().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (jobDescBuilder_ == null) {
jobDesc_ = null;
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (jobDescBuilder_ == null) {
result.jobDesc_ = jobDesc_;
} else {
result.jobDesc_ = jobDescBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasJobDesc()) {
mergeJobDesc(other.getJobDesc());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder> jobDescBuilder_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
public boolean hasJobDesc() {
return jobDescBuilder_ != null || jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
if (jobDescBuilder_ == null) {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
} else {
return jobDescBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder setJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobDesc_ = value;
onChanged();
} else {
jobDescBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder setJobDesc(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder builderForValue) {
if (jobDescBuilder_ == null) {
jobDesc_ = builderForValue.build();
onChanged();
} else {
jobDescBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder mergeJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (jobDesc_ != null) {
jobDesc_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.newBuilder(jobDesc_).mergeFrom(value).buildPartial();
} else {
jobDesc_ = value;
}
onChanged();
} else {
jobDescBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder clearJobDesc() {
if (jobDescBuilder_ == null) {
jobDesc_ = null;
onChanged();
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder getJobDescBuilder() {
onChanged();
return getJobDescFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
if (jobDescBuilder_ != null) {
return jobDescBuilder_.getMessageOrBuilder();
} else {
return jobDesc_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>
getJobDescFieldBuilder() {
if (jobDescBuilder_ == null) {
jobDescBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>(
getJobDesc(),
getParentForChildren(),
isClean());
jobDesc_ = null;
}
return jobDescBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobStatusRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobStatusRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobStatusRequest}
*/
public static final class GetJobStatusRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobStatusRequest)
GetJobStatusRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobStatusRequest.newBuilder() to construct.
private GetJobStatusRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobStatusRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobStatusRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobStatusRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobStatusRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobStatusRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobStatusRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobStatusRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobStatusRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobStatusRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobStatusResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobStatusResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobStatusResponse}
*/
public static final class GetJobStatusResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobStatusResponse)
GetJobStatusResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobStatusResponse.newBuilder() to construct.
private GetJobStatusResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobStatusResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobStatusResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobStatusResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int STATUS_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (status_ != null) {
output.writeMessage(2, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobStatusResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobStatusResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobStatusResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder mergeStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobStatusResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobStatusResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobStatusResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobStatusResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobStatusResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobResultRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobResultRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
*
* buffered result row offset
*
*
* int32 offset = 3;
* @return The offset.
*/
int getOffset();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
/**
* optional string jdbc_domain = 11;
* @return Whether the jdbcDomain field is set.
*/
boolean hasJdbcDomain();
/**
* optional string jdbc_domain = 11;
* @return The jdbcDomain.
*/
java.lang.String getJdbcDomain();
/**
* optional string jdbc_domain = 11;
* @return The bytes for jdbcDomain.
*/
com.google.protobuf.ByteString
getJdbcDomainBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResultRequest}
*/
public static final class GetJobResultRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobResultRequest)
GetJobResultRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobResultRequest.newBuilder() to construct.
private GetJobResultRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobResultRequest() {
userAgent_ = "";
jdbcDomain_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobResultRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobResultRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 24: {
offset_ = input.readInt32();
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
jdbcDomain_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder.class);
}
private int bitField0_;
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int OFFSET_FIELD_NUMBER = 3;
private int offset_;
/**
*
* buffered result row offset
*
*
* int32 offset = 3;
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JDBC_DOMAIN_FIELD_NUMBER = 11;
private volatile java.lang.Object jdbcDomain_;
/**
* optional string jdbc_domain = 11;
* @return Whether the jdbcDomain field is set.
*/
@java.lang.Override
public boolean hasJdbcDomain() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string jdbc_domain = 11;
* @return The jdbcDomain.
*/
@java.lang.Override
public java.lang.String getJdbcDomain() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jdbcDomain_ = s;
return s;
}
}
/**
* optional string jdbc_domain = 11;
* @return The bytes for jdbcDomain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJdbcDomainBytes() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jdbcDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (offset_ != 0) {
output.writeInt32(3, offset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, jdbcDomain_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (offset_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, offset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, jdbcDomain_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (getOffset()
!= other.getOffset()) return false;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (hasJdbcDomain() != other.hasJdbcDomain()) return false;
if (hasJdbcDomain()) {
if (!getJdbcDomain()
.equals(other.getJdbcDomain())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + getOffset();
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
if (hasJdbcDomain()) {
hash = (37 * hash) + JDBC_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getJdbcDomain().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResultRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobResultRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
offset_ = 0;
userAgent_ = "";
jdbcDomain_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.offset_ = offset_;
result.userAgent_ = userAgent_;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.jdbcDomain_ = jdbcDomain_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (other.getOffset() != 0) {
setOffset(other.getOffset());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
if (other.hasJdbcDomain()) {
bitField0_ |= 0x00000001;
jdbcDomain_ = other.jdbcDomain_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private int offset_ ;
/**
*
* buffered result row offset
*
*
* int32 offset = 3;
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
/**
*
* buffered result row offset
*
*
* int32 offset = 3;
* @param value The offset to set.
* @return This builder for chaining.
*/
public Builder setOffset(int value) {
offset_ = value;
onChanged();
return this;
}
/**
*
* buffered result row offset
*
*
* int32 offset = 3;
* @return This builder for chaining.
*/
public Builder clearOffset() {
offset_ = 0;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
private java.lang.Object jdbcDomain_ = "";
/**
* optional string jdbc_domain = 11;
* @return Whether the jdbcDomain field is set.
*/
public boolean hasJdbcDomain() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string jdbc_domain = 11;
* @return The jdbcDomain.
*/
public java.lang.String getJdbcDomain() {
java.lang.Object ref = jdbcDomain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jdbcDomain_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string jdbc_domain = 11;
* @return The bytes for jdbcDomain.
*/
public com.google.protobuf.ByteString
getJdbcDomainBytes() {
java.lang.Object ref = jdbcDomain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jdbcDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string jdbc_domain = 11;
* @param value The jdbcDomain to set.
* @return This builder for chaining.
*/
public Builder setJdbcDomain(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
jdbcDomain_ = value;
onChanged();
return this;
}
/**
* optional string jdbc_domain = 11;
* @return This builder for chaining.
*/
public Builder clearJdbcDomain() {
bitField0_ = (bitField0_ & ~0x00000001);
jdbcDomain_ = getDefaultInstance().getJdbcDomain();
onChanged();
return this;
}
/**
* optional string jdbc_domain = 11;
* @param value The bytes for jdbcDomain to set.
* @return This builder for chaining.
*/
public Builder setJdbcDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
bitField0_ |= 0x00000001;
jdbcDomain_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobResultRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobResultRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobResultRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobResultRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobResultResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobResultResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus();
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder();
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
boolean hasResultSet();
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet();
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResultResponse}
*/
public static final class GetJobResultResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobResultResponse)
GetJobResultResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobResultResponse.newBuilder() to construct.
private GetJobResultResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobResultResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobResultResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobResultResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder subBuilder = null;
if (resultSet_ != null) {
subBuilder = resultSet_.toBuilder();
}
resultSet_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(resultSet_);
resultSet_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int STATUS_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int RESULT_SET_FIELD_NUMBER = 3;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet resultSet_;
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
@java.lang.Override
public boolean hasResultSet() {
return resultSet_ != null;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet() {
return resultSet_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder() {
return getResultSet();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (status_ != null) {
output.writeMessage(2, getStatus());
}
if (resultSet_ != null) {
output.writeMessage(3, getResultSet());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStatus());
}
if (resultSet_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getResultSet());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasResultSet() != other.hasResultSet()) return false;
if (hasResultSet()) {
if (!getResultSet()
.equals(other.getResultSet())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasResultSet()) {
hash = (37 * hash) + RESULT_SET_FIELD_NUMBER;
hash = (53 * hash) + getResultSet().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobResultResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobResultResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (resultSetBuilder_ == null) {
resultSet_ = null;
} else {
resultSet_ = null;
resultSetBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobResultResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (resultSetBuilder_ == null) {
result.resultSet_ = resultSet_;
} else {
result.resultSet_ = resultSetBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasResultSet()) {
mergeResultSet(other.getResultSet());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
* @return The status.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder setStatus(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder mergeStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.coordinator.JobStatus status = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet resultSet_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder> resultSetBuilder_;
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return Whether the resultSet field is set.
*/
public boolean hasResultSet() {
return resultSetBuilder_ != null || resultSet_ != null;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
* @return The resultSet.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet getResultSet() {
if (resultSetBuilder_ == null) {
return resultSet_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
} else {
return resultSetBuilder_.getMessage();
}
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder setResultSet(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet value) {
if (resultSetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
resultSet_ = value;
onChanged();
} else {
resultSetBuilder_.setMessage(value);
}
return this;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder setResultSet(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder builderForValue) {
if (resultSetBuilder_ == null) {
resultSet_ = builderForValue.build();
onChanged();
} else {
resultSetBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder mergeResultSet(cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet value) {
if (resultSetBuilder_ == null) {
if (resultSet_ != null) {
resultSet_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.newBuilder(resultSet_).mergeFrom(value).buildPartial();
} else {
resultSet_ = value;
}
onChanged();
} else {
resultSetBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public Builder clearResultSet() {
if (resultSetBuilder_ == null) {
resultSet_ = null;
onChanged();
} else {
resultSet_ = null;
resultSetBuilder_ = null;
}
return this;
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder getResultSetBuilder() {
onChanged();
return getResultSetFieldBuilder().getBuilder();
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder getResultSetOrBuilder() {
if (resultSetBuilder_ != null) {
return resultSetBuilder_.getMessageOrBuilder();
} else {
return resultSet_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.getDefaultInstance() : resultSet_;
}
}
/**
*
* return result set after query terminated
*
*
* .cz.proto.coordinator.JobResultSet result_set = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder>
getResultSetFieldBuilder() {
if (resultSetBuilder_ == null) {
resultSetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSet.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobResultSetOrBuilder>(
getResultSet(),
getParentForChildren(),
isClean());
resultSet_ = null;
}
return resultSetBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobResultResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobResultResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobResultResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobResultResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobProfileRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobProfileRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProfileRequest}
*/
public static final class GetJobProfileRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobProfileRequest)
GetJobProfileRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobProfileRequest.newBuilder() to construct.
private GetJobProfileRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobProfileRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobProfileRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobProfileRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProfileRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobProfileRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobProfileRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobProfileRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobProfileRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobProfileRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobProfileResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobProfileResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
boolean hasJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc();
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder();
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return Whether the jobStatus field is set.
*/
boolean hasJobStatus();
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return The jobStatus.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getJobStatus();
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getJobStatusOrBuilder();
/**
* .cz.proto.JobSummary job_summary = 4;
* @return Whether the jobSummary field is set.
*/
boolean hasJobSummary();
/**
* .cz.proto.JobSummary job_summary = 4;
* @return The jobSummary.
*/
cz.proto.JobSummary getJobSummary();
/**
* .cz.proto.JobSummary job_summary = 4;
*/
cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder();
/**
* string job_client = 5;
* @return The jobClient.
*/
java.lang.String getJobClient();
/**
* string job_client = 5;
* @return The bytes for jobClient.
*/
com.google.protobuf.ByteString
getJobClientBytes();
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return Whether the jobProfiling field is set.
*/
boolean hasJobProfiling();
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return The jobProfiling.
*/
cz.proto.JobProfiling getJobProfiling();
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder();
/**
* string stats_download_url = 7;
* @return The statsDownloadUrl.
*/
java.lang.String getStatsDownloadUrl();
/**
* string stats_download_url = 7;
* @return The bytes for statsDownloadUrl.
*/
com.google.protobuf.ByteString
getStatsDownloadUrlBytes();
/**
* string plan_download_url = 8;
* @return The planDownloadUrl.
*/
java.lang.String getPlanDownloadUrl();
/**
* string plan_download_url = 8;
* @return The bytes for planDownloadUrl.
*/
com.google.protobuf.ByteString
getPlanDownloadUrlBytes();
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
java.util.List
getJobHistoriesList();
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
cz.proto.JobHistory getJobHistories(int index);
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
int getJobHistoriesCount();
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoriesOrBuilderList();
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
cz.proto.JobHistoryOrBuilder getJobHistoriesOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProfileResponse}
*/
public static final class GetJobProfileResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobProfileResponse)
GetJobProfileResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobProfileResponse.newBuilder() to construct.
private GetJobProfileResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobProfileResponse() {
jobClient_ = "";
statsDownloadUrl_ = "";
planDownloadUrl_ = "";
jobHistories_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobProfileResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobProfileResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder subBuilder = null;
if (jobDesc_ != null) {
subBuilder = jobDesc_.toBuilder();
}
jobDesc_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobDesc_);
jobDesc_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder subBuilder = null;
if (jobStatus_ != null) {
subBuilder = jobStatus_.toBuilder();
}
jobStatus_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobStatus_);
jobStatus_ = subBuilder.buildPartial();
}
break;
}
case 34: {
cz.proto.JobSummary.Builder subBuilder = null;
if (jobSummary_ != null) {
subBuilder = jobSummary_.toBuilder();
}
jobSummary_ = input.readMessage(cz.proto.JobSummary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobSummary_);
jobSummary_ = subBuilder.buildPartial();
}
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
jobClient_ = s;
break;
}
case 50: {
cz.proto.JobProfiling.Builder subBuilder = null;
if (jobProfiling_ != null) {
subBuilder = jobProfiling_.toBuilder();
}
jobProfiling_ = input.readMessage(cz.proto.JobProfiling.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobProfiling_);
jobProfiling_ = subBuilder.buildPartial();
}
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
statsDownloadUrl_ = s;
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
planDownloadUrl_ = s;
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
jobHistories_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
jobHistories_.add(
input.readMessage(cz.proto.JobHistory.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
jobHistories_ = java.util.Collections.unmodifiableList(jobHistories_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int JOB_DESC_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
@java.lang.Override
public boolean hasJobDesc() {
return jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
return getJobDesc();
}
public static final int JOB_STATUS_FIELD_NUMBER = 3;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus jobStatus_;
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return Whether the jobStatus field is set.
*/
@java.lang.Override
public boolean hasJobStatus() {
return jobStatus_ != null;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return The jobStatus.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getJobStatus() {
return jobStatus_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : jobStatus_;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getJobStatusOrBuilder() {
return getJobStatus();
}
public static final int JOB_SUMMARY_FIELD_NUMBER = 4;
private cz.proto.JobSummary jobSummary_;
/**
* .cz.proto.JobSummary job_summary = 4;
* @return Whether the jobSummary field is set.
*/
@java.lang.Override
public boolean hasJobSummary() {
return jobSummary_ != null;
}
/**
* .cz.proto.JobSummary job_summary = 4;
* @return The jobSummary.
*/
@java.lang.Override
public cz.proto.JobSummary getJobSummary() {
return jobSummary_ == null ? cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
@java.lang.Override
public cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder() {
return getJobSummary();
}
public static final int JOB_CLIENT_FIELD_NUMBER = 5;
private volatile java.lang.Object jobClient_;
/**
* string job_client = 5;
* @return The jobClient.
*/
@java.lang.Override
public java.lang.String getJobClient() {
java.lang.Object ref = jobClient_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobClient_ = s;
return s;
}
}
/**
* string job_client = 5;
* @return The bytes for jobClient.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobClientBytes() {
java.lang.Object ref = jobClient_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobClient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_PROFILING_FIELD_NUMBER = 6;
private cz.proto.JobProfiling jobProfiling_;
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return Whether the jobProfiling field is set.
*/
@java.lang.Override
public boolean hasJobProfiling() {
return jobProfiling_ != null;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return The jobProfiling.
*/
@java.lang.Override
public cz.proto.JobProfiling getJobProfiling() {
return jobProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
@java.lang.Override
public cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder() {
return getJobProfiling();
}
public static final int STATS_DOWNLOAD_URL_FIELD_NUMBER = 7;
private volatile java.lang.Object statsDownloadUrl_;
/**
* string stats_download_url = 7;
* @return The statsDownloadUrl.
*/
@java.lang.Override
public java.lang.String getStatsDownloadUrl() {
java.lang.Object ref = statsDownloadUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
statsDownloadUrl_ = s;
return s;
}
}
/**
* string stats_download_url = 7;
* @return The bytes for statsDownloadUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStatsDownloadUrlBytes() {
java.lang.Object ref = statsDownloadUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statsDownloadUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PLAN_DOWNLOAD_URL_FIELD_NUMBER = 8;
private volatile java.lang.Object planDownloadUrl_;
/**
* string plan_download_url = 8;
* @return The planDownloadUrl.
*/
@java.lang.Override
public java.lang.String getPlanDownloadUrl() {
java.lang.Object ref = planDownloadUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
planDownloadUrl_ = s;
return s;
}
}
/**
* string plan_download_url = 8;
* @return The bytes for planDownloadUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPlanDownloadUrlBytes() {
java.lang.Object ref = planDownloadUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
planDownloadUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOB_HISTORIES_FIELD_NUMBER = 9;
private java.util.List jobHistories_;
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
@java.lang.Override
public java.util.List getJobHistoriesList() {
return jobHistories_;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
@java.lang.Override
public java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoriesOrBuilderList() {
return jobHistories_;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
@java.lang.Override
public int getJobHistoriesCount() {
return jobHistories_.size();
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
@java.lang.Override
public cz.proto.JobHistory getJobHistories(int index) {
return jobHistories_.get(index);
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
@java.lang.Override
public cz.proto.JobHistoryOrBuilder getJobHistoriesOrBuilder(
int index) {
return jobHistories_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (jobDesc_ != null) {
output.writeMessage(2, getJobDesc());
}
if (jobStatus_ != null) {
output.writeMessage(3, getJobStatus());
}
if (jobSummary_ != null) {
output.writeMessage(4, getJobSummary());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobClient_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, jobClient_);
}
if (jobProfiling_ != null) {
output.writeMessage(6, getJobProfiling());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(statsDownloadUrl_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, statsDownloadUrl_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(planDownloadUrl_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, planDownloadUrl_);
}
for (int i = 0; i < jobHistories_.size(); i++) {
output.writeMessage(9, jobHistories_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (jobDesc_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobDesc());
}
if (jobStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getJobStatus());
}
if (jobSummary_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getJobSummary());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobClient_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, jobClient_);
}
if (jobProfiling_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getJobProfiling());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(statsDownloadUrl_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, statsDownloadUrl_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(planDownloadUrl_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, planDownloadUrl_);
}
for (int i = 0; i < jobHistories_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, jobHistories_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasJobDesc() != other.hasJobDesc()) return false;
if (hasJobDesc()) {
if (!getJobDesc()
.equals(other.getJobDesc())) return false;
}
if (hasJobStatus() != other.hasJobStatus()) return false;
if (hasJobStatus()) {
if (!getJobStatus()
.equals(other.getJobStatus())) return false;
}
if (hasJobSummary() != other.hasJobSummary()) return false;
if (hasJobSummary()) {
if (!getJobSummary()
.equals(other.getJobSummary())) return false;
}
if (!getJobClient()
.equals(other.getJobClient())) return false;
if (hasJobProfiling() != other.hasJobProfiling()) return false;
if (hasJobProfiling()) {
if (!getJobProfiling()
.equals(other.getJobProfiling())) return false;
}
if (!getStatsDownloadUrl()
.equals(other.getStatsDownloadUrl())) return false;
if (!getPlanDownloadUrl()
.equals(other.getPlanDownloadUrl())) return false;
if (!getJobHistoriesList()
.equals(other.getJobHistoriesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasJobDesc()) {
hash = (37 * hash) + JOB_DESC_FIELD_NUMBER;
hash = (53 * hash) + getJobDesc().hashCode();
}
if (hasJobStatus()) {
hash = (37 * hash) + JOB_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getJobStatus().hashCode();
}
if (hasJobSummary()) {
hash = (37 * hash) + JOB_SUMMARY_FIELD_NUMBER;
hash = (53 * hash) + getJobSummary().hashCode();
}
hash = (37 * hash) + JOB_CLIENT_FIELD_NUMBER;
hash = (53 * hash) + getJobClient().hashCode();
if (hasJobProfiling()) {
hash = (37 * hash) + JOB_PROFILING_FIELD_NUMBER;
hash = (53 * hash) + getJobProfiling().hashCode();
}
hash = (37 * hash) + STATS_DOWNLOAD_URL_FIELD_NUMBER;
hash = (53 * hash) + getStatsDownloadUrl().hashCode();
hash = (37 * hash) + PLAN_DOWNLOAD_URL_FIELD_NUMBER;
hash = (53 * hash) + getPlanDownloadUrl().hashCode();
if (getJobHistoriesCount() > 0) {
hash = (37 * hash) + JOB_HISTORIES_FIELD_NUMBER;
hash = (53 * hash) + getJobHistoriesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProfileResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobProfileResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getJobHistoriesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (jobDescBuilder_ == null) {
jobDesc_ = null;
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
if (jobStatusBuilder_ == null) {
jobStatus_ = null;
} else {
jobStatus_ = null;
jobStatusBuilder_ = null;
}
if (jobSummaryBuilder_ == null) {
jobSummary_ = null;
} else {
jobSummary_ = null;
jobSummaryBuilder_ = null;
}
jobClient_ = "";
if (jobProfilingBuilder_ == null) {
jobProfiling_ = null;
} else {
jobProfiling_ = null;
jobProfilingBuilder_ = null;
}
statsDownloadUrl_ = "";
planDownloadUrl_ = "";
if (jobHistoriesBuilder_ == null) {
jobHistories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
jobHistoriesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProfileResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse(this);
int from_bitField0_ = bitField0_;
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (jobDescBuilder_ == null) {
result.jobDesc_ = jobDesc_;
} else {
result.jobDesc_ = jobDescBuilder_.build();
}
if (jobStatusBuilder_ == null) {
result.jobStatus_ = jobStatus_;
} else {
result.jobStatus_ = jobStatusBuilder_.build();
}
if (jobSummaryBuilder_ == null) {
result.jobSummary_ = jobSummary_;
} else {
result.jobSummary_ = jobSummaryBuilder_.build();
}
result.jobClient_ = jobClient_;
if (jobProfilingBuilder_ == null) {
result.jobProfiling_ = jobProfiling_;
} else {
result.jobProfiling_ = jobProfilingBuilder_.build();
}
result.statsDownloadUrl_ = statsDownloadUrl_;
result.planDownloadUrl_ = planDownloadUrl_;
if (jobHistoriesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
jobHistories_ = java.util.Collections.unmodifiableList(jobHistories_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.jobHistories_ = jobHistories_;
} else {
result.jobHistories_ = jobHistoriesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasJobDesc()) {
mergeJobDesc(other.getJobDesc());
}
if (other.hasJobStatus()) {
mergeJobStatus(other.getJobStatus());
}
if (other.hasJobSummary()) {
mergeJobSummary(other.getJobSummary());
}
if (!other.getJobClient().isEmpty()) {
jobClient_ = other.jobClient_;
onChanged();
}
if (other.hasJobProfiling()) {
mergeJobProfiling(other.getJobProfiling());
}
if (!other.getStatsDownloadUrl().isEmpty()) {
statsDownloadUrl_ = other.statsDownloadUrl_;
onChanged();
}
if (!other.getPlanDownloadUrl().isEmpty()) {
planDownloadUrl_ = other.planDownloadUrl_;
onChanged();
}
if (jobHistoriesBuilder_ == null) {
if (!other.jobHistories_.isEmpty()) {
if (jobHistories_.isEmpty()) {
jobHistories_ = other.jobHistories_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureJobHistoriesIsMutable();
jobHistories_.addAll(other.jobHistories_);
}
onChanged();
}
} else {
if (!other.jobHistories_.isEmpty()) {
if (jobHistoriesBuilder_.isEmpty()) {
jobHistoriesBuilder_.dispose();
jobHistoriesBuilder_ = null;
jobHistories_ = other.jobHistories_;
bitField0_ = (bitField0_ & ~0x00000001);
jobHistoriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getJobHistoriesFieldBuilder() : null;
} else {
jobHistoriesBuilder_.addAllMessages(other.jobHistories_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc jobDesc_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder> jobDescBuilder_;
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return Whether the jobDesc field is set.
*/
public boolean hasJobDesc() {
return jobDescBuilder_ != null || jobDesc_ != null;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
* @return The jobDesc.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc getJobDesc() {
if (jobDescBuilder_ == null) {
return jobDesc_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
} else {
return jobDescBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder setJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobDesc_ = value;
onChanged();
} else {
jobDescBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder setJobDesc(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder builderForValue) {
if (jobDescBuilder_ == null) {
jobDesc_ = builderForValue.build();
onChanged();
} else {
jobDescBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder mergeJobDesc(cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc value) {
if (jobDescBuilder_ == null) {
if (jobDesc_ != null) {
jobDesc_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.newBuilder(jobDesc_).mergeFrom(value).buildPartial();
} else {
jobDesc_ = value;
}
onChanged();
} else {
jobDescBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public Builder clearJobDesc() {
if (jobDescBuilder_ == null) {
jobDesc_ = null;
onChanged();
} else {
jobDesc_ = null;
jobDescBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder getJobDescBuilder() {
onChanged();
return getJobDescFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder getJobDescOrBuilder() {
if (jobDescBuilder_ != null) {
return jobDescBuilder_.getMessageOrBuilder();
} else {
return jobDesc_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.getDefaultInstance() : jobDesc_;
}
}
/**
* .cz.proto.coordinator.JobDesc job_desc = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>
getJobDescFieldBuilder() {
if (jobDescBuilder_ == null) {
jobDescBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDesc.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobDescOrBuilder>(
getJobDesc(),
getParentForChildren(),
isClean());
jobDesc_ = null;
}
return jobDescBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus jobStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder> jobStatusBuilder_;
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return Whether the jobStatus field is set.
*/
public boolean hasJobStatus() {
return jobStatusBuilder_ != null || jobStatus_ != null;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
* @return The jobStatus.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus getJobStatus() {
if (jobStatusBuilder_ == null) {
return jobStatus_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : jobStatus_;
} else {
return jobStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public Builder setJobStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (jobStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobStatus_ = value;
onChanged();
} else {
jobStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public Builder setJobStatus(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder builderForValue) {
if (jobStatusBuilder_ == null) {
jobStatus_ = builderForValue.build();
onChanged();
} else {
jobStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public Builder mergeJobStatus(cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus value) {
if (jobStatusBuilder_ == null) {
if (jobStatus_ != null) {
jobStatus_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.newBuilder(jobStatus_).mergeFrom(value).buildPartial();
} else {
jobStatus_ = value;
}
onChanged();
} else {
jobStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public Builder clearJobStatus() {
if (jobStatusBuilder_ == null) {
jobStatus_ = null;
onChanged();
} else {
jobStatus_ = null;
jobStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder getJobStatusBuilder() {
onChanged();
return getJobStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder getJobStatusOrBuilder() {
if (jobStatusBuilder_ != null) {
return jobStatusBuilder_.getMessageOrBuilder();
} else {
return jobStatus_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.getDefaultInstance() : jobStatus_;
}
}
/**
* .cz.proto.coordinator.JobStatus job_status = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>
getJobStatusFieldBuilder() {
if (jobStatusBuilder_ == null) {
jobStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatus.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobStatusOrBuilder>(
getJobStatus(),
getParentForChildren(),
isClean());
jobStatus_ = null;
}
return jobStatusBuilder_;
}
private cz.proto.JobSummary jobSummary_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder> jobSummaryBuilder_;
/**
* .cz.proto.JobSummary job_summary = 4;
* @return Whether the jobSummary field is set.
*/
public boolean hasJobSummary() {
return jobSummaryBuilder_ != null || jobSummary_ != null;
}
/**
* .cz.proto.JobSummary job_summary = 4;
* @return The jobSummary.
*/
public cz.proto.JobSummary getJobSummary() {
if (jobSummaryBuilder_ == null) {
return jobSummary_ == null ? cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
} else {
return jobSummaryBuilder_.getMessage();
}
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public Builder setJobSummary(cz.proto.JobSummary value) {
if (jobSummaryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobSummary_ = value;
onChanged();
} else {
jobSummaryBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public Builder setJobSummary(
cz.proto.JobSummary.Builder builderForValue) {
if (jobSummaryBuilder_ == null) {
jobSummary_ = builderForValue.build();
onChanged();
} else {
jobSummaryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public Builder mergeJobSummary(cz.proto.JobSummary value) {
if (jobSummaryBuilder_ == null) {
if (jobSummary_ != null) {
jobSummary_ =
cz.proto.JobSummary.newBuilder(jobSummary_).mergeFrom(value).buildPartial();
} else {
jobSummary_ = value;
}
onChanged();
} else {
jobSummaryBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public Builder clearJobSummary() {
if (jobSummaryBuilder_ == null) {
jobSummary_ = null;
onChanged();
} else {
jobSummary_ = null;
jobSummaryBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public cz.proto.JobSummary.Builder getJobSummaryBuilder() {
onChanged();
return getJobSummaryFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
public cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder() {
if (jobSummaryBuilder_ != null) {
return jobSummaryBuilder_.getMessageOrBuilder();
} else {
return jobSummary_ == null ?
cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
}
}
/**
* .cz.proto.JobSummary job_summary = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder>
getJobSummaryFieldBuilder() {
if (jobSummaryBuilder_ == null) {
jobSummaryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder>(
getJobSummary(),
getParentForChildren(),
isClean());
jobSummary_ = null;
}
return jobSummaryBuilder_;
}
private java.lang.Object jobClient_ = "";
/**
* string job_client = 5;
* @return The jobClient.
*/
public java.lang.String getJobClient() {
java.lang.Object ref = jobClient_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobClient_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string job_client = 5;
* @return The bytes for jobClient.
*/
public com.google.protobuf.ByteString
getJobClientBytes() {
java.lang.Object ref = jobClient_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobClient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string job_client = 5;
* @param value The jobClient to set.
* @return This builder for chaining.
*/
public Builder setJobClient(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobClient_ = value;
onChanged();
return this;
}
/**
* string job_client = 5;
* @return This builder for chaining.
*/
public Builder clearJobClient() {
jobClient_ = getDefaultInstance().getJobClient();
onChanged();
return this;
}
/**
* string job_client = 5;
* @param value The bytes for jobClient to set.
* @return This builder for chaining.
*/
public Builder setJobClientBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobClient_ = value;
onChanged();
return this;
}
private cz.proto.JobProfiling jobProfiling_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder> jobProfilingBuilder_;
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return Whether the jobProfiling field is set.
*/
public boolean hasJobProfiling() {
return jobProfilingBuilder_ != null || jobProfiling_ != null;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
* @return The jobProfiling.
*/
public cz.proto.JobProfiling getJobProfiling() {
if (jobProfilingBuilder_ == null) {
return jobProfiling_ == null ? cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
} else {
return jobProfilingBuilder_.getMessage();
}
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public Builder setJobProfiling(cz.proto.JobProfiling value) {
if (jobProfilingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobProfiling_ = value;
onChanged();
} else {
jobProfilingBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public Builder setJobProfiling(
cz.proto.JobProfiling.Builder builderForValue) {
if (jobProfilingBuilder_ == null) {
jobProfiling_ = builderForValue.build();
onChanged();
} else {
jobProfilingBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public Builder mergeJobProfiling(cz.proto.JobProfiling value) {
if (jobProfilingBuilder_ == null) {
if (jobProfiling_ != null) {
jobProfiling_ =
cz.proto.JobProfiling.newBuilder(jobProfiling_).mergeFrom(value).buildPartial();
} else {
jobProfiling_ = value;
}
onChanged();
} else {
jobProfilingBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public Builder clearJobProfiling() {
if (jobProfilingBuilder_ == null) {
jobProfiling_ = null;
onChanged();
} else {
jobProfiling_ = null;
jobProfilingBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public cz.proto.JobProfiling.Builder getJobProfilingBuilder() {
onChanged();
return getJobProfilingFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
public cz.proto.JobProfilingOrBuilder getJobProfilingOrBuilder() {
if (jobProfilingBuilder_ != null) {
return jobProfilingBuilder_.getMessageOrBuilder();
} else {
return jobProfiling_ == null ?
cz.proto.JobProfiling.getDefaultInstance() : jobProfiling_;
}
}
/**
* .cz.proto.JobProfiling job_profiling = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>
getJobProfilingFieldBuilder() {
if (jobProfilingBuilder_ == null) {
jobProfilingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProfiling, cz.proto.JobProfiling.Builder, cz.proto.JobProfilingOrBuilder>(
getJobProfiling(),
getParentForChildren(),
isClean());
jobProfiling_ = null;
}
return jobProfilingBuilder_;
}
private java.lang.Object statsDownloadUrl_ = "";
/**
* string stats_download_url = 7;
* @return The statsDownloadUrl.
*/
public java.lang.String getStatsDownloadUrl() {
java.lang.Object ref = statsDownloadUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
statsDownloadUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stats_download_url = 7;
* @return The bytes for statsDownloadUrl.
*/
public com.google.protobuf.ByteString
getStatsDownloadUrlBytes() {
java.lang.Object ref = statsDownloadUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
statsDownloadUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stats_download_url = 7;
* @param value The statsDownloadUrl to set.
* @return This builder for chaining.
*/
public Builder setStatsDownloadUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
statsDownloadUrl_ = value;
onChanged();
return this;
}
/**
* string stats_download_url = 7;
* @return This builder for chaining.
*/
public Builder clearStatsDownloadUrl() {
statsDownloadUrl_ = getDefaultInstance().getStatsDownloadUrl();
onChanged();
return this;
}
/**
* string stats_download_url = 7;
* @param value The bytes for statsDownloadUrl to set.
* @return This builder for chaining.
*/
public Builder setStatsDownloadUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
statsDownloadUrl_ = value;
onChanged();
return this;
}
private java.lang.Object planDownloadUrl_ = "";
/**
* string plan_download_url = 8;
* @return The planDownloadUrl.
*/
public java.lang.String getPlanDownloadUrl() {
java.lang.Object ref = planDownloadUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
planDownloadUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string plan_download_url = 8;
* @return The bytes for planDownloadUrl.
*/
public com.google.protobuf.ByteString
getPlanDownloadUrlBytes() {
java.lang.Object ref = planDownloadUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
planDownloadUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string plan_download_url = 8;
* @param value The planDownloadUrl to set.
* @return This builder for chaining.
*/
public Builder setPlanDownloadUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
planDownloadUrl_ = value;
onChanged();
return this;
}
/**
* string plan_download_url = 8;
* @return This builder for chaining.
*/
public Builder clearPlanDownloadUrl() {
planDownloadUrl_ = getDefaultInstance().getPlanDownloadUrl();
onChanged();
return this;
}
/**
* string plan_download_url = 8;
* @param value The bytes for planDownloadUrl to set.
* @return This builder for chaining.
*/
public Builder setPlanDownloadUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
planDownloadUrl_ = value;
onChanged();
return this;
}
private java.util.List jobHistories_ =
java.util.Collections.emptyList();
private void ensureJobHistoriesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
jobHistories_ = new java.util.ArrayList(jobHistories_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder> jobHistoriesBuilder_;
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public java.util.List getJobHistoriesList() {
if (jobHistoriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(jobHistories_);
} else {
return jobHistoriesBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public int getJobHistoriesCount() {
if (jobHistoriesBuilder_ == null) {
return jobHistories_.size();
} else {
return jobHistoriesBuilder_.getCount();
}
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public cz.proto.JobHistory getJobHistories(int index) {
if (jobHistoriesBuilder_ == null) {
return jobHistories_.get(index);
} else {
return jobHistoriesBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder setJobHistories(
int index, cz.proto.JobHistory value) {
if (jobHistoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoriesIsMutable();
jobHistories_.set(index, value);
onChanged();
} else {
jobHistoriesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder setJobHistories(
int index, cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoriesBuilder_ == null) {
ensureJobHistoriesIsMutable();
jobHistories_.set(index, builderForValue.build());
onChanged();
} else {
jobHistoriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder addJobHistories(cz.proto.JobHistory value) {
if (jobHistoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoriesIsMutable();
jobHistories_.add(value);
onChanged();
} else {
jobHistoriesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder addJobHistories(
int index, cz.proto.JobHistory value) {
if (jobHistoriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureJobHistoriesIsMutable();
jobHistories_.add(index, value);
onChanged();
} else {
jobHistoriesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder addJobHistories(
cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoriesBuilder_ == null) {
ensureJobHistoriesIsMutable();
jobHistories_.add(builderForValue.build());
onChanged();
} else {
jobHistoriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder addJobHistories(
int index, cz.proto.JobHistory.Builder builderForValue) {
if (jobHistoriesBuilder_ == null) {
ensureJobHistoriesIsMutable();
jobHistories_.add(index, builderForValue.build());
onChanged();
} else {
jobHistoriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder addAllJobHistories(
java.lang.Iterable extends cz.proto.JobHistory> values) {
if (jobHistoriesBuilder_ == null) {
ensureJobHistoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, jobHistories_);
onChanged();
} else {
jobHistoriesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder clearJobHistories() {
if (jobHistoriesBuilder_ == null) {
jobHistories_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
jobHistoriesBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public Builder removeJobHistories(int index) {
if (jobHistoriesBuilder_ == null) {
ensureJobHistoriesIsMutable();
jobHistories_.remove(index);
onChanged();
} else {
jobHistoriesBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public cz.proto.JobHistory.Builder getJobHistoriesBuilder(
int index) {
return getJobHistoriesFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public cz.proto.JobHistoryOrBuilder getJobHistoriesOrBuilder(
int index) {
if (jobHistoriesBuilder_ == null) {
return jobHistories_.get(index); } else {
return jobHistoriesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public java.util.List extends cz.proto.JobHistoryOrBuilder>
getJobHistoriesOrBuilderList() {
if (jobHistoriesBuilder_ != null) {
return jobHistoriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(jobHistories_);
}
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public cz.proto.JobHistory.Builder addJobHistoriesBuilder() {
return getJobHistoriesFieldBuilder().addBuilder(
cz.proto.JobHistory.getDefaultInstance());
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public cz.proto.JobHistory.Builder addJobHistoriesBuilder(
int index) {
return getJobHistoriesFieldBuilder().addBuilder(
index, cz.proto.JobHistory.getDefaultInstance());
}
/**
* repeated .cz.proto.JobHistory job_histories = 9;
*/
public java.util.List
getJobHistoriesBuilderList() {
return getJobHistoriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder>
getJobHistoriesFieldBuilder() {
if (jobHistoriesBuilder_ == null) {
jobHistoriesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.JobHistory, cz.proto.JobHistory.Builder, cz.proto.JobHistoryOrBuilder>(
jobHistories_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
jobHistories_ = null;
}
return jobHistoriesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobProfileResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobProfileResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobProfileResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobProfileResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobSummaryRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobSummaryRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobSummaryRequest}
*/
public static final class GetJobSummaryRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobSummaryRequest)
GetJobSummaryRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobSummaryRequest.newBuilder() to construct.
private GetJobSummaryRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobSummaryRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobSummaryRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobSummaryRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobSummaryRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobSummaryRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobSummaryRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobSummaryRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobSummaryRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobSummaryRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobSummaryResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobSummaryResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.JobSummary job_summary = 2;
* @return Whether the jobSummary field is set.
*/
boolean hasJobSummary();
/**
* .cz.proto.JobSummary job_summary = 2;
* @return The jobSummary.
*/
cz.proto.JobSummary getJobSummary();
/**
* .cz.proto.JobSummary job_summary = 2;
*/
cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobSummaryResponse}
*/
public static final class GetJobSummaryResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobSummaryResponse)
GetJobSummaryResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobSummaryResponse.newBuilder() to construct.
private GetJobSummaryResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobSummaryResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobSummaryResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobSummaryResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.JobSummary.Builder subBuilder = null;
if (jobSummary_ != null) {
subBuilder = jobSummary_.toBuilder();
}
jobSummary_ = input.readMessage(cz.proto.JobSummary.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobSummary_);
jobSummary_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int JOB_SUMMARY_FIELD_NUMBER = 2;
private cz.proto.JobSummary jobSummary_;
/**
* .cz.proto.JobSummary job_summary = 2;
* @return Whether the jobSummary field is set.
*/
@java.lang.Override
public boolean hasJobSummary() {
return jobSummary_ != null;
}
/**
* .cz.proto.JobSummary job_summary = 2;
* @return The jobSummary.
*/
@java.lang.Override
public cz.proto.JobSummary getJobSummary() {
return jobSummary_ == null ? cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
@java.lang.Override
public cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder() {
return getJobSummary();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (jobSummary_ != null) {
output.writeMessage(2, getJobSummary());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (jobSummary_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobSummary());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasJobSummary() != other.hasJobSummary()) return false;
if (hasJobSummary()) {
if (!getJobSummary()
.equals(other.getJobSummary())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasJobSummary()) {
hash = (37 * hash) + JOB_SUMMARY_FIELD_NUMBER;
hash = (53 * hash) + getJobSummary().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobSummaryResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobSummaryResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (jobSummaryBuilder_ == null) {
jobSummary_ = null;
} else {
jobSummary_ = null;
jobSummaryBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobSummaryResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (jobSummaryBuilder_ == null) {
result.jobSummary_ = jobSummary_;
} else {
result.jobSummary_ = jobSummaryBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasJobSummary()) {
mergeJobSummary(other.getJobSummary());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.JobSummary jobSummary_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder> jobSummaryBuilder_;
/**
* .cz.proto.JobSummary job_summary = 2;
* @return Whether the jobSummary field is set.
*/
public boolean hasJobSummary() {
return jobSummaryBuilder_ != null || jobSummary_ != null;
}
/**
* .cz.proto.JobSummary job_summary = 2;
* @return The jobSummary.
*/
public cz.proto.JobSummary getJobSummary() {
if (jobSummaryBuilder_ == null) {
return jobSummary_ == null ? cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
} else {
return jobSummaryBuilder_.getMessage();
}
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public Builder setJobSummary(cz.proto.JobSummary value) {
if (jobSummaryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobSummary_ = value;
onChanged();
} else {
jobSummaryBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public Builder setJobSummary(
cz.proto.JobSummary.Builder builderForValue) {
if (jobSummaryBuilder_ == null) {
jobSummary_ = builderForValue.build();
onChanged();
} else {
jobSummaryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public Builder mergeJobSummary(cz.proto.JobSummary value) {
if (jobSummaryBuilder_ == null) {
if (jobSummary_ != null) {
jobSummary_ =
cz.proto.JobSummary.newBuilder(jobSummary_).mergeFrom(value).buildPartial();
} else {
jobSummary_ = value;
}
onChanged();
} else {
jobSummaryBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public Builder clearJobSummary() {
if (jobSummaryBuilder_ == null) {
jobSummary_ = null;
onChanged();
} else {
jobSummary_ = null;
jobSummaryBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public cz.proto.JobSummary.Builder getJobSummaryBuilder() {
onChanged();
return getJobSummaryFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
public cz.proto.JobSummaryOrBuilder getJobSummaryOrBuilder() {
if (jobSummaryBuilder_ != null) {
return jobSummaryBuilder_.getMessageOrBuilder();
} else {
return jobSummary_ == null ?
cz.proto.JobSummary.getDefaultInstance() : jobSummary_;
}
}
/**
* .cz.proto.JobSummary job_summary = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder>
getJobSummaryFieldBuilder() {
if (jobSummaryBuilder_ == null) {
jobSummaryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobSummary, cz.proto.JobSummary.Builder, cz.proto.JobSummaryOrBuilder>(
getJobSummary(),
getParentForChildren(),
isClean());
jobSummary_ = null;
}
return jobSummaryBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobSummaryResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobSummaryResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobSummaryResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobSummaryResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobProgressRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobProgressRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* bool verbose = 3;
* @return The verbose.
*/
boolean getVerbose();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProgressRequest}
*/
public static final class GetJobProgressRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobProgressRequest)
GetJobProgressRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobProgressRequest.newBuilder() to construct.
private GetJobProgressRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobProgressRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobProgressRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobProgressRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 24: {
verbose_ = input.readBool();
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int VERBOSE_FIELD_NUMBER = 3;
private boolean verbose_;
/**
* bool verbose = 3;
* @return The verbose.
*/
@java.lang.Override
public boolean getVerbose() {
return verbose_;
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (verbose_ != false) {
output.writeBool(3, verbose_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (verbose_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, verbose_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (getVerbose()
!= other.getVerbose()) return false;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + VERBOSE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getVerbose());
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProgressRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobProgressRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
verbose_ = false;
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.verbose_ = verbose_;
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (other.getVerbose() != false) {
setVerbose(other.getVerbose());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private boolean verbose_ ;
/**
* bool verbose = 3;
* @return The verbose.
*/
@java.lang.Override
public boolean getVerbose() {
return verbose_;
}
/**
* bool verbose = 3;
* @param value The verbose to set.
* @return This builder for chaining.
*/
public Builder setVerbose(boolean value) {
verbose_ = value;
onChanged();
return this;
}
/**
* bool verbose = 3;
* @return This builder for chaining.
*/
public Builder clearVerbose() {
verbose_ = false;
onChanged();
return this;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobProgressRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobProgressRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobProgressRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobProgressRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobProgressResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobProgressResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.JobProgress progress = 2;
* @return Whether the progress field is set.
*/
boolean hasProgress();
/**
* .cz.proto.JobProgress progress = 2;
* @return The progress.
*/
cz.proto.JobProgress getProgress();
/**
* .cz.proto.JobProgress progress = 2;
*/
cz.proto.JobProgressOrBuilder getProgressOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProgressResponse}
*/
public static final class GetJobProgressResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobProgressResponse)
GetJobProgressResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobProgressResponse.newBuilder() to construct.
private GetJobProgressResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobProgressResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobProgressResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobProgressResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.JobProgress.Builder subBuilder = null;
if (progress_ != null) {
subBuilder = progress_.toBuilder();
}
progress_ = input.readMessage(cz.proto.JobProgress.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(progress_);
progress_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int PROGRESS_FIELD_NUMBER = 2;
private cz.proto.JobProgress progress_;
/**
* .cz.proto.JobProgress progress = 2;
* @return Whether the progress field is set.
*/
@java.lang.Override
public boolean hasProgress() {
return progress_ != null;
}
/**
* .cz.proto.JobProgress progress = 2;
* @return The progress.
*/
@java.lang.Override
public cz.proto.JobProgress getProgress() {
return progress_ == null ? cz.proto.JobProgress.getDefaultInstance() : progress_;
}
/**
* .cz.proto.JobProgress progress = 2;
*/
@java.lang.Override
public cz.proto.JobProgressOrBuilder getProgressOrBuilder() {
return getProgress();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (progress_ != null) {
output.writeMessage(2, getProgress());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (progress_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getProgress());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasProgress() != other.hasProgress()) return false;
if (hasProgress()) {
if (!getProgress()
.equals(other.getProgress())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasProgress()) {
hash = (37 * hash) + PROGRESS_FIELD_NUMBER;
hash = (53 * hash) + getProgress().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobProgressResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobProgressResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (progressBuilder_ == null) {
progress_ = null;
} else {
progress_ = null;
progressBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobProgressResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (progressBuilder_ == null) {
result.progress_ = progress_;
} else {
result.progress_ = progressBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasProgress()) {
mergeProgress(other.getProgress());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.JobProgress progress_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProgress, cz.proto.JobProgress.Builder, cz.proto.JobProgressOrBuilder> progressBuilder_;
/**
* .cz.proto.JobProgress progress = 2;
* @return Whether the progress field is set.
*/
public boolean hasProgress() {
return progressBuilder_ != null || progress_ != null;
}
/**
* .cz.proto.JobProgress progress = 2;
* @return The progress.
*/
public cz.proto.JobProgress getProgress() {
if (progressBuilder_ == null) {
return progress_ == null ? cz.proto.JobProgress.getDefaultInstance() : progress_;
} else {
return progressBuilder_.getMessage();
}
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public Builder setProgress(cz.proto.JobProgress value) {
if (progressBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
progress_ = value;
onChanged();
} else {
progressBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public Builder setProgress(
cz.proto.JobProgress.Builder builderForValue) {
if (progressBuilder_ == null) {
progress_ = builderForValue.build();
onChanged();
} else {
progressBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public Builder mergeProgress(cz.proto.JobProgress value) {
if (progressBuilder_ == null) {
if (progress_ != null) {
progress_ =
cz.proto.JobProgress.newBuilder(progress_).mergeFrom(value).buildPartial();
} else {
progress_ = value;
}
onChanged();
} else {
progressBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public Builder clearProgress() {
if (progressBuilder_ == null) {
progress_ = null;
onChanged();
} else {
progress_ = null;
progressBuilder_ = null;
}
return this;
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public cz.proto.JobProgress.Builder getProgressBuilder() {
onChanged();
return getProgressFieldBuilder().getBuilder();
}
/**
* .cz.proto.JobProgress progress = 2;
*/
public cz.proto.JobProgressOrBuilder getProgressOrBuilder() {
if (progressBuilder_ != null) {
return progressBuilder_.getMessageOrBuilder();
} else {
return progress_ == null ?
cz.proto.JobProgress.getDefaultInstance() : progress_;
}
}
/**
* .cz.proto.JobProgress progress = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProgress, cz.proto.JobProgress.Builder, cz.proto.JobProgressOrBuilder>
getProgressFieldBuilder() {
if (progressBuilder_ == null) {
progressBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.JobProgress, cz.proto.JobProgress.Builder, cz.proto.JobProgressOrBuilder>(
getProgress(),
getParentForChildren(),
isClean());
progress_ = null;
}
return progressBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobProgressResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobProgressResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobProgressResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobProgressResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobPlanRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobPlanRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount();
/**
* .cz.proto.coordinator.Account account = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
boolean hasJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId();
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder();
/**
* string user_agent = 10;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobPlanRequest}
*/
public static final class GetJobPlanRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobPlanRequest)
GetJobPlanRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobPlanRequest.newBuilder() to construct.
private GetJobPlanRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobPlanRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobPlanRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobPlanRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder subBuilder = null;
if (jobId_ != null) {
subBuilder = jobId_.toBuilder();
}
jobId_ = input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobId_);
jobId_ = subBuilder.buildPartial();
}
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int JOB_ID_FIELD_NUMBER = 2;
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
@java.lang.Override
public boolean hasJobId() {
return jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
return getJobId();
}
public static final int USER_AGENT_FIELD_NUMBER = 10;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 10;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (jobId_ != null) {
output.writeMessage(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (jobId_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobId());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasJobId() != other.hasJobId()) return false;
if (hasJobId()) {
if (!getJobId()
.equals(other.getJobId())) return false;
}
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasJobId()) {
hash = (37 * hash) + JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
}
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobPlanRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobPlanRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (jobIdBuilder_ == null) {
jobId_ = null;
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
userAgent_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (jobIdBuilder_ == null) {
result.jobId_ = jobId_;
} else {
result.jobId_ = jobIdBuilder_.build();
}
result.userAgent_ = userAgent_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasJobId()) {
mergeJobId(other.getJobId());
}
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.coordinator.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.coordinator.Account account = 1;
* @return The account.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder setAccount(
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder mergeAccount(cz.proto.coordinator.CoordinatorServiceOuterClass.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.coordinator.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.Account, cz.proto.coordinator.CoordinatorServiceOuterClass.Account.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.coordinator.CoordinatorServiceOuterClass.JobID jobId_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder> jobIdBuilder_;
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return Whether the jobId field is set.
*/
public boolean hasJobId() {
return jobIdBuilder_ != null || jobId_ != null;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
* @return The jobId.
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID getJobId() {
if (jobIdBuilder_ == null) {
return jobId_ == null ? cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
} else {
return jobIdBuilder_.getMessage();
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
} else {
jobIdBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder setJobId(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder builderForValue) {
if (jobIdBuilder_ == null) {
jobId_ = builderForValue.build();
onChanged();
} else {
jobIdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder mergeJobId(cz.proto.coordinator.CoordinatorServiceOuterClass.JobID value) {
if (jobIdBuilder_ == null) {
if (jobId_ != null) {
jobId_ =
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.newBuilder(jobId_).mergeFrom(value).buildPartial();
} else {
jobId_ = value;
}
onChanged();
} else {
jobIdBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public Builder clearJobId() {
if (jobIdBuilder_ == null) {
jobId_ = null;
onChanged();
} else {
jobId_ = null;
jobIdBuilder_ = null;
}
return this;
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder getJobIdBuilder() {
onChanged();
return getJobIdFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder getJobIdOrBuilder() {
if (jobIdBuilder_ != null) {
return jobIdBuilder_.getMessageOrBuilder();
} else {
return jobId_ == null ?
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.getDefaultInstance() : jobId_;
}
}
/**
* .cz.proto.coordinator.JobID job_id = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>
getJobIdFieldBuilder() {
if (jobIdBuilder_ == null) {
jobIdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.JobID, cz.proto.coordinator.CoordinatorServiceOuterClass.JobID.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.JobIDOrBuilder>(
getJobId(),
getParentForChildren(),
isClean());
jobId_ = null;
}
return jobIdBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 10;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 10;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 10;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 10;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 10;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobPlanRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobPlanRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobPlanRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobPlanRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetJobPlanResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.GetJobPlanResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
boolean hasRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
cz.proto.ServiceCommon.ResponseStatus getRespStatus();
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder();
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return Whether the jobPlan field is set.
*/
boolean hasJobPlan();
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return The jobPlan.
*/
cz.proto.SimplifyDag getJobPlan();
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
cz.proto.SimplifyDagOrBuilder getJobPlanOrBuilder();
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobPlanResponse}
*/
public static final class GetJobPlanResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.GetJobPlanResponse)
GetJobPlanResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetJobPlanResponse.newBuilder() to construct.
private GetJobPlanResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetJobPlanResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetJobPlanResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetJobPlanResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ServiceCommon.ResponseStatus.Builder subBuilder = null;
if (respStatus_ != null) {
subBuilder = respStatus_.toBuilder();
}
respStatus_ = input.readMessage(cz.proto.ServiceCommon.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(respStatus_);
respStatus_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.SimplifyDag.Builder subBuilder = null;
if (jobPlan_ != null) {
subBuilder = jobPlan_.toBuilder();
}
jobPlan_ = input.readMessage(cz.proto.SimplifyDag.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(jobPlan_);
jobPlan_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.Builder.class);
}
public static final int RESP_STATUS_FIELD_NUMBER = 1;
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
@java.lang.Override
public boolean hasRespStatus() {
return respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
@java.lang.Override
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
return getRespStatus();
}
public static final int JOB_PLAN_FIELD_NUMBER = 2;
private cz.proto.SimplifyDag jobPlan_;
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return Whether the jobPlan field is set.
*/
@java.lang.Override
public boolean hasJobPlan() {
return jobPlan_ != null;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return The jobPlan.
*/
@java.lang.Override
public cz.proto.SimplifyDag getJobPlan() {
return jobPlan_ == null ? cz.proto.SimplifyDag.getDefaultInstance() : jobPlan_;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
@java.lang.Override
public cz.proto.SimplifyDagOrBuilder getJobPlanOrBuilder() {
return getJobPlan();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (respStatus_ != null) {
output.writeMessage(1, getRespStatus());
}
if (jobPlan_ != null) {
output.writeMessage(2, getJobPlan());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (respStatus_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRespStatus());
}
if (jobPlan_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getJobPlan());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse other = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse) obj;
if (hasRespStatus() != other.hasRespStatus()) return false;
if (hasRespStatus()) {
if (!getRespStatus()
.equals(other.getRespStatus())) return false;
}
if (hasJobPlan() != other.hasJobPlan()) return false;
if (hasJobPlan()) {
if (!getJobPlan()
.equals(other.getJobPlan())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRespStatus()) {
hash = (37 * hash) + RESP_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getRespStatus().hashCode();
}
if (hasJobPlan()) {
hash = (37 * hash) + JOB_PLAN_FIELD_NUMBER;
hash = (53 * hash) + getJobPlan().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.GetJobPlanResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.GetJobPlanResponse)
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.class, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (respStatusBuilder_ == null) {
respStatus_ = null;
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
if (jobPlanBuilder_ == null) {
jobPlan_ = null;
} else {
jobPlan_ = null;
jobPlanBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_GetJobPlanResponse_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse result = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse(this);
if (respStatusBuilder_ == null) {
result.respStatus_ = respStatus_;
} else {
result.respStatus_ = respStatusBuilder_.build();
}
if (jobPlanBuilder_ == null) {
result.jobPlan_ = jobPlan_;
} else {
result.jobPlan_ = jobPlanBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse.getDefaultInstance()) return this;
if (other.hasRespStatus()) {
mergeRespStatus(other.getRespStatus());
}
if (other.hasJobPlan()) {
mergeJobPlan(other.getJobPlan());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ServiceCommon.ResponseStatus respStatus_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder> respStatusBuilder_;
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return Whether the respStatus field is set.
*/
public boolean hasRespStatus() {
return respStatusBuilder_ != null || respStatus_ != null;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
* @return The respStatus.
*/
public cz.proto.ServiceCommon.ResponseStatus getRespStatus() {
if (respStatusBuilder_ == null) {
return respStatus_ == null ? cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
} else {
return respStatusBuilder_.getMessage();
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
respStatus_ = value;
onChanged();
} else {
respStatusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder setRespStatus(
cz.proto.ServiceCommon.ResponseStatus.Builder builderForValue) {
if (respStatusBuilder_ == null) {
respStatus_ = builderForValue.build();
onChanged();
} else {
respStatusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder mergeRespStatus(cz.proto.ServiceCommon.ResponseStatus value) {
if (respStatusBuilder_ == null) {
if (respStatus_ != null) {
respStatus_ =
cz.proto.ServiceCommon.ResponseStatus.newBuilder(respStatus_).mergeFrom(value).buildPartial();
} else {
respStatus_ = value;
}
onChanged();
} else {
respStatusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public Builder clearRespStatus() {
if (respStatusBuilder_ == null) {
respStatus_ = null;
onChanged();
} else {
respStatus_ = null;
respStatusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatus.Builder getRespStatusBuilder() {
onChanged();
return getRespStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
public cz.proto.ServiceCommon.ResponseStatusOrBuilder getRespStatusOrBuilder() {
if (respStatusBuilder_ != null) {
return respStatusBuilder_.getMessageOrBuilder();
} else {
return respStatus_ == null ?
cz.proto.ServiceCommon.ResponseStatus.getDefaultInstance() : respStatus_;
}
}
/**
* .cz.proto.ResponseStatus resp_status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>
getRespStatusFieldBuilder() {
if (respStatusBuilder_ == null) {
respStatusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ServiceCommon.ResponseStatus, cz.proto.ServiceCommon.ResponseStatus.Builder, cz.proto.ServiceCommon.ResponseStatusOrBuilder>(
getRespStatus(),
getParentForChildren(),
isClean());
respStatus_ = null;
}
return respStatusBuilder_;
}
private cz.proto.SimplifyDag jobPlan_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SimplifyDag, cz.proto.SimplifyDag.Builder, cz.proto.SimplifyDagOrBuilder> jobPlanBuilder_;
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return Whether the jobPlan field is set.
*/
public boolean hasJobPlan() {
return jobPlanBuilder_ != null || jobPlan_ != null;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
* @return The jobPlan.
*/
public cz.proto.SimplifyDag getJobPlan() {
if (jobPlanBuilder_ == null) {
return jobPlan_ == null ? cz.proto.SimplifyDag.getDefaultInstance() : jobPlan_;
} else {
return jobPlanBuilder_.getMessage();
}
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public Builder setJobPlan(cz.proto.SimplifyDag value) {
if (jobPlanBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
jobPlan_ = value;
onChanged();
} else {
jobPlanBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public Builder setJobPlan(
cz.proto.SimplifyDag.Builder builderForValue) {
if (jobPlanBuilder_ == null) {
jobPlan_ = builderForValue.build();
onChanged();
} else {
jobPlanBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public Builder mergeJobPlan(cz.proto.SimplifyDag value) {
if (jobPlanBuilder_ == null) {
if (jobPlan_ != null) {
jobPlan_ =
cz.proto.SimplifyDag.newBuilder(jobPlan_).mergeFrom(value).buildPartial();
} else {
jobPlan_ = value;
}
onChanged();
} else {
jobPlanBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public Builder clearJobPlan() {
if (jobPlanBuilder_ == null) {
jobPlan_ = null;
onChanged();
} else {
jobPlan_ = null;
jobPlanBuilder_ = null;
}
return this;
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public cz.proto.SimplifyDag.Builder getJobPlanBuilder() {
onChanged();
return getJobPlanFieldBuilder().getBuilder();
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
public cz.proto.SimplifyDagOrBuilder getJobPlanOrBuilder() {
if (jobPlanBuilder_ != null) {
return jobPlanBuilder_.getMessageOrBuilder();
} else {
return jobPlan_ == null ?
cz.proto.SimplifyDag.getDefaultInstance() : jobPlan_;
}
}
/**
* .cz.proto.SimplifyDag job_plan = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SimplifyDag, cz.proto.SimplifyDag.Builder, cz.proto.SimplifyDagOrBuilder>
getJobPlanFieldBuilder() {
if (jobPlanBuilder_ == null) {
jobPlanBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.SimplifyDag, cz.proto.SimplifyDag.Builder, cz.proto.SimplifyDagOrBuilder>(
getJobPlan(),
getParentForChildren(),
isClean());
jobPlan_ = null;
}
return jobPlanBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.GetJobPlanResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.GetJobPlanResponse)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetJobPlanResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetJobPlanResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.JobRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return Whether the getResultRequest field is set.
*/
boolean hasGetResultRequest();
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return The getResultRequest.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getGetResultRequest();
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder getGetResultRequestOrBuilder();
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return Whether the getSummaryRequest field is set.
*/
boolean hasGetSummaryRequest();
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return The getSummaryRequest.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getGetSummaryRequest();
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder getGetSummaryRequestOrBuilder();
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return Whether the getPlanRequest field is set.
*/
boolean hasGetPlanRequest();
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return The getPlanRequest.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getGetPlanRequest();
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder getGetPlanRequestOrBuilder();
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return Whether the getProfileRequest field is set.
*/
boolean hasGetProfileRequest();
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return The getProfileRequest.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getGetProfileRequest();
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder getGetProfileRequestOrBuilder();
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return Whether the getProgressRequest field is set.
*/
boolean hasGetProgressRequest();
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return The getProgressRequest.
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getGetProgressRequest();
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder getGetProgressRequestOrBuilder();
/**
* string user_agent = 30;
* @return The userAgent.
*/
java.lang.String getUserAgent();
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
com.google.protobuf.ByteString
getUserAgentBytes();
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.RequestCase getRequestCase();
}
/**
* Protobuf type {@code cz.proto.coordinator.JobRequest}
*/
public static final class JobRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.JobRequest)
JobRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobRequest.newBuilder() to construct.
private JobRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobRequest() {
userAgent_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder subBuilder = null;
if (requestCase_ == 1) {
subBuilder = ((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_).toBuilder();
}
request_ =
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_);
request_ = subBuilder.buildPartial();
}
requestCase_ = 1;
break;
}
case 18: {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder subBuilder = null;
if (requestCase_ == 2) {
subBuilder = ((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_).toBuilder();
}
request_ =
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_);
request_ = subBuilder.buildPartial();
}
requestCase_ = 2;
break;
}
case 26: {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder subBuilder = null;
if (requestCase_ == 3) {
subBuilder = ((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_).toBuilder();
}
request_ =
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_);
request_ = subBuilder.buildPartial();
}
requestCase_ = 3;
break;
}
case 34: {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder subBuilder = null;
if (requestCase_ == 4) {
subBuilder = ((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_).toBuilder();
}
request_ =
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_);
request_ = subBuilder.buildPartial();
}
requestCase_ = 4;
break;
}
case 42: {
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder subBuilder = null;
if (requestCase_ == 5) {
subBuilder = ((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_).toBuilder();
}
request_ =
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_);
request_ = subBuilder.buildPartial();
}
requestCase_ = 5;
break;
}
case 242: {
java.lang.String s = input.readStringRequireUtf8();
userAgent_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.Builder.class);
}
private int requestCase_ = 0;
private java.lang.Object request_;
public enum RequestCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
GET_RESULT_REQUEST(1),
GET_SUMMARY_REQUEST(2),
GET_PLAN_REQUEST(3),
GET_PROFILE_REQUEST(4),
GET_PROGRESS_REQUEST(5),
REQUEST_NOT_SET(0);
private final int value;
private RequestCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RequestCase valueOf(int value) {
return forNumber(value);
}
public static RequestCase forNumber(int value) {
switch (value) {
case 1: return GET_RESULT_REQUEST;
case 2: return GET_SUMMARY_REQUEST;
case 3: return GET_PLAN_REQUEST;
case 4: return GET_PROFILE_REQUEST;
case 5: return GET_PROGRESS_REQUEST;
case 0: return REQUEST_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public RequestCase
getRequestCase() {
return RequestCase.forNumber(
requestCase_);
}
public static final int GET_RESULT_REQUEST_FIELD_NUMBER = 1;
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return Whether the getResultRequest field is set.
*/
@java.lang.Override
public boolean hasGetResultRequest() {
return requestCase_ == 1;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return The getResultRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getGetResultRequest() {
if (requestCase_ == 1) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder getGetResultRequestOrBuilder() {
if (requestCase_ == 1) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
public static final int GET_SUMMARY_REQUEST_FIELD_NUMBER = 2;
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return Whether the getSummaryRequest field is set.
*/
@java.lang.Override
public boolean hasGetSummaryRequest() {
return requestCase_ == 2;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return The getSummaryRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getGetSummaryRequest() {
if (requestCase_ == 2) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder getGetSummaryRequestOrBuilder() {
if (requestCase_ == 2) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
public static final int GET_PLAN_REQUEST_FIELD_NUMBER = 3;
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return Whether the getPlanRequest field is set.
*/
@java.lang.Override
public boolean hasGetPlanRequest() {
return requestCase_ == 3;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return The getPlanRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getGetPlanRequest() {
if (requestCase_ == 3) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder getGetPlanRequestOrBuilder() {
if (requestCase_ == 3) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
public static final int GET_PROFILE_REQUEST_FIELD_NUMBER = 4;
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return Whether the getProfileRequest field is set.
*/
@java.lang.Override
public boolean hasGetProfileRequest() {
return requestCase_ == 4;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return The getProfileRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getGetProfileRequest() {
if (requestCase_ == 4) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder getGetProfileRequestOrBuilder() {
if (requestCase_ == 4) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
public static final int GET_PROGRESS_REQUEST_FIELD_NUMBER = 5;
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return Whether the getProgressRequest field is set.
*/
@java.lang.Override
public boolean hasGetProgressRequest() {
return requestCase_ == 5;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return The getProgressRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getGetProgressRequest() {
if (requestCase_ == 5) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder getGetProgressRequestOrBuilder() {
if (requestCase_ == 5) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
public static final int USER_AGENT_FIELD_NUMBER = 30;
private volatile java.lang.Object userAgent_;
/**
* string user_agent = 30;
* @return The userAgent.
*/
@java.lang.Override
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (requestCase_ == 1) {
output.writeMessage(1, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_);
}
if (requestCase_ == 2) {
output.writeMessage(2, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_);
}
if (requestCase_ == 3) {
output.writeMessage(3, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_);
}
if (requestCase_ == 4) {
output.writeMessage(4, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_);
}
if (requestCase_ == 5) {
output.writeMessage(5, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, userAgent_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (requestCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_);
}
if (requestCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_);
}
if (requestCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_);
}
if (requestCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_);
}
if (requestCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userAgent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, userAgent_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest)) {
return super.equals(obj);
}
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest other = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest) obj;
if (!getUserAgent()
.equals(other.getUserAgent())) return false;
if (!getRequestCase().equals(other.getRequestCase())) return false;
switch (requestCase_) {
case 1:
if (!getGetResultRequest()
.equals(other.getGetResultRequest())) return false;
break;
case 2:
if (!getGetSummaryRequest()
.equals(other.getGetSummaryRequest())) return false;
break;
case 3:
if (!getGetPlanRequest()
.equals(other.getGetPlanRequest())) return false;
break;
case 4:
if (!getGetProfileRequest()
.equals(other.getGetProfileRequest())) return false;
break;
case 5:
if (!getGetProgressRequest()
.equals(other.getGetProgressRequest())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + USER_AGENT_FIELD_NUMBER;
hash = (53 * hash) + getUserAgent().hashCode();
switch (requestCase_) {
case 1:
hash = (37 * hash) + GET_RESULT_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getGetResultRequest().hashCode();
break;
case 2:
hash = (37 * hash) + GET_SUMMARY_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getGetSummaryRequest().hashCode();
break;
case 3:
hash = (37 * hash) + GET_PLAN_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getGetPlanRequest().hashCode();
break;
case 4:
hash = (37 * hash) + GET_PROFILE_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getGetProfileRequest().hashCode();
break;
case 5:
hash = (37 * hash) + GET_PROGRESS_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getGetProgressRequest().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.coordinator.JobRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.coordinator.JobRequest)
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.Builder.class);
}
// Construct using cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
userAgent_ = "";
requestCase_ = 0;
request_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_JobRequest_descriptor;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest getDefaultInstanceForType() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest build() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest buildPartial() {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest result = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest(this);
if (requestCase_ == 1) {
if (getResultRequestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = getResultRequestBuilder_.build();
}
}
if (requestCase_ == 2) {
if (getSummaryRequestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = getSummaryRequestBuilder_.build();
}
}
if (requestCase_ == 3) {
if (getPlanRequestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = getPlanRequestBuilder_.build();
}
}
if (requestCase_ == 4) {
if (getProfileRequestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = getProfileRequestBuilder_.build();
}
}
if (requestCase_ == 5) {
if (getProgressRequestBuilder_ == null) {
result.request_ = request_;
} else {
result.request_ = getProgressRequestBuilder_.build();
}
}
result.userAgent_ = userAgent_;
result.requestCase_ = requestCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest) {
return mergeFrom((cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest other) {
if (other == cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest.getDefaultInstance()) return this;
if (!other.getUserAgent().isEmpty()) {
userAgent_ = other.userAgent_;
onChanged();
}
switch (other.getRequestCase()) {
case GET_RESULT_REQUEST: {
mergeGetResultRequest(other.getGetResultRequest());
break;
}
case GET_SUMMARY_REQUEST: {
mergeGetSummaryRequest(other.getGetSummaryRequest());
break;
}
case GET_PLAN_REQUEST: {
mergeGetPlanRequest(other.getGetPlanRequest());
break;
}
case GET_PROFILE_REQUEST: {
mergeGetProfileRequest(other.getGetProfileRequest());
break;
}
case GET_PROGRESS_REQUEST: {
mergeGetProgressRequest(other.getGetProgressRequest());
break;
}
case REQUEST_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int requestCase_ = 0;
private java.lang.Object request_;
public RequestCase
getRequestCase() {
return RequestCase.forNumber(
requestCase_);
}
public Builder clearRequest() {
requestCase_ = 0;
request_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder> getResultRequestBuilder_;
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return Whether the getResultRequest field is set.
*/
@java.lang.Override
public boolean hasGetResultRequest() {
return requestCase_ == 1;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
* @return The getResultRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest getGetResultRequest() {
if (getResultRequestBuilder_ == null) {
if (requestCase_ == 1) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
} else {
if (requestCase_ == 1) {
return getResultRequestBuilder_.getMessage();
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
public Builder setGetResultRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest value) {
if (getResultRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
getResultRequestBuilder_.setMessage(value);
}
requestCase_ = 1;
return this;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
public Builder setGetResultRequest(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder builderForValue) {
if (getResultRequestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
getResultRequestBuilder_.setMessage(builderForValue.build());
}
requestCase_ = 1;
return this;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
public Builder mergeGetResultRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest value) {
if (getResultRequestBuilder_ == null) {
if (requestCase_ == 1 &&
request_ != cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance()) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.newBuilder((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_)
.mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
if (requestCase_ == 1) {
getResultRequestBuilder_.mergeFrom(value);
}
getResultRequestBuilder_.setMessage(value);
}
requestCase_ = 1;
return this;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
public Builder clearGetResultRequest() {
if (getResultRequestBuilder_ == null) {
if (requestCase_ == 1) {
requestCase_ = 0;
request_ = null;
onChanged();
}
} else {
if (requestCase_ == 1) {
requestCase_ = 0;
request_ = null;
}
getResultRequestBuilder_.clear();
}
return this;
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder getGetResultRequestBuilder() {
return getGetResultRequestFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder getGetResultRequestOrBuilder() {
if ((requestCase_ == 1) && (getResultRequestBuilder_ != null)) {
return getResultRequestBuilder_.getMessageOrBuilder();
} else {
if (requestCase_ == 1) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobResultRequest get_result_request = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder>
getGetResultRequestFieldBuilder() {
if (getResultRequestBuilder_ == null) {
if (!(requestCase_ == 1)) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.getDefaultInstance();
}
getResultRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequestOrBuilder>(
(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobResultRequest) request_,
getParentForChildren(),
isClean());
request_ = null;
}
requestCase_ = 1;
onChanged();;
return getResultRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder> getSummaryRequestBuilder_;
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return Whether the getSummaryRequest field is set.
*/
@java.lang.Override
public boolean hasGetSummaryRequest() {
return requestCase_ == 2;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
* @return The getSummaryRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest getGetSummaryRequest() {
if (getSummaryRequestBuilder_ == null) {
if (requestCase_ == 2) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
} else {
if (requestCase_ == 2) {
return getSummaryRequestBuilder_.getMessage();
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
public Builder setGetSummaryRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest value) {
if (getSummaryRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
getSummaryRequestBuilder_.setMessage(value);
}
requestCase_ = 2;
return this;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
public Builder setGetSummaryRequest(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder builderForValue) {
if (getSummaryRequestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
getSummaryRequestBuilder_.setMessage(builderForValue.build());
}
requestCase_ = 2;
return this;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
public Builder mergeGetSummaryRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest value) {
if (getSummaryRequestBuilder_ == null) {
if (requestCase_ == 2 &&
request_ != cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance()) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.newBuilder((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_)
.mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
if (requestCase_ == 2) {
getSummaryRequestBuilder_.mergeFrom(value);
}
getSummaryRequestBuilder_.setMessage(value);
}
requestCase_ = 2;
return this;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
public Builder clearGetSummaryRequest() {
if (getSummaryRequestBuilder_ == null) {
if (requestCase_ == 2) {
requestCase_ = 0;
request_ = null;
onChanged();
}
} else {
if (requestCase_ == 2) {
requestCase_ = 0;
request_ = null;
}
getSummaryRequestBuilder_.clear();
}
return this;
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder getGetSummaryRequestBuilder() {
return getGetSummaryRequestFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder getGetSummaryRequestOrBuilder() {
if ((requestCase_ == 2) && (getSummaryRequestBuilder_ != null)) {
return getSummaryRequestBuilder_.getMessageOrBuilder();
} else {
if (requestCase_ == 2) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobSummaryRequest get_summary_request = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder>
getGetSummaryRequestFieldBuilder() {
if (getSummaryRequestBuilder_ == null) {
if (!(requestCase_ == 2)) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.getDefaultInstance();
}
getSummaryRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequestOrBuilder>(
(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobSummaryRequest) request_,
getParentForChildren(),
isClean());
request_ = null;
}
requestCase_ = 2;
onChanged();;
return getSummaryRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder> getPlanRequestBuilder_;
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return Whether the getPlanRequest field is set.
*/
@java.lang.Override
public boolean hasGetPlanRequest() {
return requestCase_ == 3;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
* @return The getPlanRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest getGetPlanRequest() {
if (getPlanRequestBuilder_ == null) {
if (requestCase_ == 3) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
} else {
if (requestCase_ == 3) {
return getPlanRequestBuilder_.getMessage();
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
public Builder setGetPlanRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest value) {
if (getPlanRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
getPlanRequestBuilder_.setMessage(value);
}
requestCase_ = 3;
return this;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
public Builder setGetPlanRequest(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder builderForValue) {
if (getPlanRequestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
getPlanRequestBuilder_.setMessage(builderForValue.build());
}
requestCase_ = 3;
return this;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
public Builder mergeGetPlanRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest value) {
if (getPlanRequestBuilder_ == null) {
if (requestCase_ == 3 &&
request_ != cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance()) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.newBuilder((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_)
.mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
if (requestCase_ == 3) {
getPlanRequestBuilder_.mergeFrom(value);
}
getPlanRequestBuilder_.setMessage(value);
}
requestCase_ = 3;
return this;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
public Builder clearGetPlanRequest() {
if (getPlanRequestBuilder_ == null) {
if (requestCase_ == 3) {
requestCase_ = 0;
request_ = null;
onChanged();
}
} else {
if (requestCase_ == 3) {
requestCase_ = 0;
request_ = null;
}
getPlanRequestBuilder_.clear();
}
return this;
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder getGetPlanRequestBuilder() {
return getGetPlanRequestFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder getGetPlanRequestOrBuilder() {
if ((requestCase_ == 3) && (getPlanRequestBuilder_ != null)) {
return getPlanRequestBuilder_.getMessageOrBuilder();
} else {
if (requestCase_ == 3) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobPlanRequest get_plan_request = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder>
getGetPlanRequestFieldBuilder() {
if (getPlanRequestBuilder_ == null) {
if (!(requestCase_ == 3)) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.getDefaultInstance();
}
getPlanRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequestOrBuilder>(
(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobPlanRequest) request_,
getParentForChildren(),
isClean());
request_ = null;
}
requestCase_ = 3;
onChanged();;
return getPlanRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder> getProfileRequestBuilder_;
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return Whether the getProfileRequest field is set.
*/
@java.lang.Override
public boolean hasGetProfileRequest() {
return requestCase_ == 4;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
* @return The getProfileRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest getGetProfileRequest() {
if (getProfileRequestBuilder_ == null) {
if (requestCase_ == 4) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
} else {
if (requestCase_ == 4) {
return getProfileRequestBuilder_.getMessage();
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
public Builder setGetProfileRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest value) {
if (getProfileRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
getProfileRequestBuilder_.setMessage(value);
}
requestCase_ = 4;
return this;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
public Builder setGetProfileRequest(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder builderForValue) {
if (getProfileRequestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
getProfileRequestBuilder_.setMessage(builderForValue.build());
}
requestCase_ = 4;
return this;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
public Builder mergeGetProfileRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest value) {
if (getProfileRequestBuilder_ == null) {
if (requestCase_ == 4 &&
request_ != cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance()) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.newBuilder((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_)
.mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
if (requestCase_ == 4) {
getProfileRequestBuilder_.mergeFrom(value);
}
getProfileRequestBuilder_.setMessage(value);
}
requestCase_ = 4;
return this;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
public Builder clearGetProfileRequest() {
if (getProfileRequestBuilder_ == null) {
if (requestCase_ == 4) {
requestCase_ = 0;
request_ = null;
onChanged();
}
} else {
if (requestCase_ == 4) {
requestCase_ = 0;
request_ = null;
}
getProfileRequestBuilder_.clear();
}
return this;
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder getGetProfileRequestBuilder() {
return getGetProfileRequestFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder getGetProfileRequestOrBuilder() {
if ((requestCase_ == 4) && (getProfileRequestBuilder_ != null)) {
return getProfileRequestBuilder_.getMessageOrBuilder();
} else {
if (requestCase_ == 4) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobProfileRequest get_profile_request = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder>
getGetProfileRequestFieldBuilder() {
if (getProfileRequestBuilder_ == null) {
if (!(requestCase_ == 4)) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.getDefaultInstance();
}
getProfileRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequestOrBuilder>(
(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProfileRequest) request_,
getParentForChildren(),
isClean());
request_ = null;
}
requestCase_ = 4;
onChanged();;
return getProfileRequestBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder> getProgressRequestBuilder_;
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return Whether the getProgressRequest field is set.
*/
@java.lang.Override
public boolean hasGetProgressRequest() {
return requestCase_ == 5;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
* @return The getProgressRequest.
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest getGetProgressRequest() {
if (getProgressRequestBuilder_ == null) {
if (requestCase_ == 5) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
} else {
if (requestCase_ == 5) {
return getProgressRequestBuilder_.getMessage();
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
public Builder setGetProgressRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest value) {
if (getProgressRequestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
request_ = value;
onChanged();
} else {
getProgressRequestBuilder_.setMessage(value);
}
requestCase_ = 5;
return this;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
public Builder setGetProgressRequest(
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder builderForValue) {
if (getProgressRequestBuilder_ == null) {
request_ = builderForValue.build();
onChanged();
} else {
getProgressRequestBuilder_.setMessage(builderForValue.build());
}
requestCase_ = 5;
return this;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
public Builder mergeGetProgressRequest(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest value) {
if (getProgressRequestBuilder_ == null) {
if (requestCase_ == 5 &&
request_ != cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance()) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.newBuilder((cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_)
.mergeFrom(value).buildPartial();
} else {
request_ = value;
}
onChanged();
} else {
if (requestCase_ == 5) {
getProgressRequestBuilder_.mergeFrom(value);
}
getProgressRequestBuilder_.setMessage(value);
}
requestCase_ = 5;
return this;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
public Builder clearGetProgressRequest() {
if (getProgressRequestBuilder_ == null) {
if (requestCase_ == 5) {
requestCase_ = 0;
request_ = null;
onChanged();
}
} else {
if (requestCase_ == 5) {
requestCase_ = 0;
request_ = null;
}
getProgressRequestBuilder_.clear();
}
return this;
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder getGetProgressRequestBuilder() {
return getGetProgressRequestFieldBuilder().getBuilder();
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder getGetProgressRequestOrBuilder() {
if ((requestCase_ == 5) && (getProgressRequestBuilder_ != null)) {
return getProgressRequestBuilder_.getMessageOrBuilder();
} else {
if (requestCase_ == 5) {
return (cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_;
}
return cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
}
/**
* .cz.proto.coordinator.GetJobProgressRequest get_progress_request = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder>
getGetProgressRequestFieldBuilder() {
if (getProgressRequestBuilder_ == null) {
if (!(requestCase_ == 5)) {
request_ = cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.getDefaultInstance();
}
getProgressRequestBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest.Builder, cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequestOrBuilder>(
(cz.proto.coordinator.CoordinatorServiceOuterClass.GetJobProgressRequest) request_,
getParentForChildren(),
isClean());
request_ = null;
}
requestCase_ = 5;
onChanged();;
return getProgressRequestBuilder_;
}
private java.lang.Object userAgent_ = "";
/**
* string user_agent = 30;
* @return The userAgent.
*/
public java.lang.String getUserAgent() {
java.lang.Object ref = userAgent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userAgent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_agent = 30;
* @return The bytes for userAgent.
*/
public com.google.protobuf.ByteString
getUserAgentBytes() {
java.lang.Object ref = userAgent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_agent = 30;
* @param value The userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userAgent_ = value;
onChanged();
return this;
}
/**
* string user_agent = 30;
* @return This builder for chaining.
*/
public Builder clearUserAgent() {
userAgent_ = getDefaultInstance().getUserAgent();
onChanged();
return this;
}
/**
* string user_agent = 30;
* @param value The bytes for userAgent to set.
* @return This builder for chaining.
*/
public Builder setUserAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userAgent_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.coordinator.JobRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.coordinator.JobRequest)
private static final cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest();
}
public static cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.coordinator.CoordinatorServiceOuterClass.JobRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InitializeInstanceRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.coordinator.InitializeInstanceRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.coordinator.CreateWorkspaceRequest create_workspace = 1;
*/
java.util.List
getCreateWorkspaceList();
/**
* repeated .cz.proto.coordinator.CreateWorkspaceRequest create_workspace = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.CreateWorkspaceRequest getCreateWorkspace(int index);
/**
* repeated .cz.proto.coordinator.CreateWorkspaceRequest create_workspace = 1;
*/
int getCreateWorkspaceCount();
/**
* repeated .cz.proto.coordinator.CreateWorkspaceRequest create_workspace = 1;
*/
java.util.List extends cz.proto.coordinator.CoordinatorServiceOuterClass.CreateWorkspaceRequestOrBuilder>
getCreateWorkspaceOrBuilderList();
/**
* repeated .cz.proto.coordinator.CreateWorkspaceRequest create_workspace = 1;
*/
cz.proto.coordinator.CoordinatorServiceOuterClass.CreateWorkspaceRequestOrBuilder getCreateWorkspaceOrBuilder(
int index);
}
/**
* Protobuf type {@code cz.proto.coordinator.InitializeInstanceRequest}
*/
public static final class InitializeInstanceRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.coordinator.InitializeInstanceRequest)
InitializeInstanceRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use InitializeInstanceRequest.newBuilder() to construct.
private InitializeInstanceRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InitializeInstanceRequest() {
createWorkspace_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InitializeInstanceRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InitializeInstanceRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
createWorkspace_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
createWorkspace_.add(
input.readMessage(cz.proto.coordinator.CoordinatorServiceOuterClass.CreateWorkspaceRequest.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
createWorkspace_ = java.util.Collections.unmodifiableList(createWorkspace_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_InitializeInstanceRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.coordinator.CoordinatorServiceOuterClass.internal_static_cz_proto_coordinator_InitializeInstanceRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.coordinator.CoordinatorServiceOuterClass.InitializeInstanceRequest.class, cz.proto.coordinator.CoordinatorServiceOuterClass.InitializeInstanceRequest.Builder.class);
}
public static final int CREATE_WORKSPACE_FIELD_NUMBER = 1;
private java.util.List