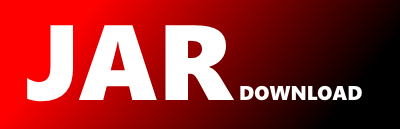
cz.proto.ingestion.Ingestion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ingestion.proto
package cz.proto.ingestion;
public final class Ingestion {
private Ingestion() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code cz.proto.ingestion.IGSTableType}
*/
public enum IGSTableType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NORMAL = 0;
*/
NORMAL(0),
/**
* CLUSTER = 1;
*/
CLUSTER(1),
/**
* ACID = 2;
*/
ACID(2),
/**
* UNKNOWN = 3;
*/
UNKNOWN(3),
UNRECOGNIZED(-1),
;
/**
* NORMAL = 0;
*/
public static final int NORMAL_VALUE = 0;
/**
* CLUSTER = 1;
*/
public static final int CLUSTER_VALUE = 1;
/**
* ACID = 2;
*/
public static final int ACID_VALUE = 2;
/**
* UNKNOWN = 3;
*/
public static final int UNKNOWN_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IGSTableType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static IGSTableType forNumber(int value) {
switch (value) {
case 0: return NORMAL;
case 1: return CLUSTER;
case 2: return ACID;
case 3: return UNKNOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
IGSTableType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public IGSTableType findValueByNumber(int number) {
return IGSTableType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.getDescriptor().getEnumTypes().get(0);
}
private static final IGSTableType[] VALUES = values();
public static IGSTableType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private IGSTableType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.IGSTableType)
}
/**
* Protobuf enum {@code cz.proto.ingestion.Code}
*/
public enum Code
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SUCCESS = 0;
*/
SUCCESS(0),
/**
* FAILED = 1;
*/
FAILED(1),
/**
* IGS_WORKER_REGISTED = 2;
*/
IGS_WORKER_REGISTED(2),
/**
* THROTTLED = 3;
*/
THROTTLED(3),
/**
* NOT_FOUND = 4;
*/
NOT_FOUND(4),
/**
* ALREADY_PRESENT = 5;
*/
ALREADY_PRESENT(5),
/**
* TABLE_EXIST = 6;
*/
TABLE_EXIST(6),
/**
* TABLE_DROPPED = 7;
*/
TABLE_DROPPED(7),
/**
* CORRUPTION = 8;
*/
CORRUPTION(8),
UNRECOGNIZED(-1),
;
/**
* SUCCESS = 0;
*/
public static final int SUCCESS_VALUE = 0;
/**
* FAILED = 1;
*/
public static final int FAILED_VALUE = 1;
/**
* IGS_WORKER_REGISTED = 2;
*/
public static final int IGS_WORKER_REGISTED_VALUE = 2;
/**
* THROTTLED = 3;
*/
public static final int THROTTLED_VALUE = 3;
/**
* NOT_FOUND = 4;
*/
public static final int NOT_FOUND_VALUE = 4;
/**
* ALREADY_PRESENT = 5;
*/
public static final int ALREADY_PRESENT_VALUE = 5;
/**
* TABLE_EXIST = 6;
*/
public static final int TABLE_EXIST_VALUE = 6;
/**
* TABLE_DROPPED = 7;
*/
public static final int TABLE_DROPPED_VALUE = 7;
/**
* CORRUPTION = 8;
*/
public static final int CORRUPTION_VALUE = 8;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Code valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Code forNumber(int value) {
switch (value) {
case 0: return SUCCESS;
case 1: return FAILED;
case 2: return IGS_WORKER_REGISTED;
case 3: return THROTTLED;
case 4: return NOT_FOUND;
case 5: return ALREADY_PRESENT;
case 6: return TABLE_EXIST;
case 7: return TABLE_DROPPED;
case 8: return CORRUPTION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Code> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Code findValueByNumber(int number) {
return Code.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.getDescriptor().getEnumTypes().get(1);
}
private static final Code[] VALUES = values();
public static Code valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Code(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.Code)
}
/**
* Protobuf enum {@code cz.proto.ingestion.ConnectMode}
*/
public enum ConnectMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DIRECT = 0;
*/
DIRECT(0),
/**
* GATEWAY = 1;
*/
GATEWAY(1),
/**
* GATEWAY_INTERNAL = 2;
*/
GATEWAY_INTERNAL(2),
/**
* GATEWAY_DIRECT = 3;
*/
GATEWAY_DIRECT(3),
UNRECOGNIZED(-1),
;
/**
* DIRECT = 0;
*/
public static final int DIRECT_VALUE = 0;
/**
* GATEWAY = 1;
*/
public static final int GATEWAY_VALUE = 1;
/**
* GATEWAY_INTERNAL = 2;
*/
public static final int GATEWAY_INTERNAL_VALUE = 2;
/**
* GATEWAY_DIRECT = 3;
*/
public static final int GATEWAY_DIRECT_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConnectMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ConnectMode forNumber(int value) {
switch (value) {
case 0: return DIRECT;
case 1: return GATEWAY;
case 2: return GATEWAY_INTERNAL;
case 3: return GATEWAY_DIRECT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ConnectMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConnectMode findValueByNumber(int number) {
return ConnectMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.getDescriptor().getEnumTypes().get(2);
}
private static final ConnectMode[] VALUES = values();
public static ConnectMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ConnectMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.ConnectMode)
}
/**
*
**
* all gateway method list.
*
*
* Protobuf enum {@code cz.proto.ingestion.MethodEnum}
*/
public enum MethodEnum
implements com.google.protobuf.ProtocolMessageEnum {
/**
* GATEWAY_RPC_CALL = 0;
*/
GATEWAY_RPC_CALL(0),
/**
* GET_TABLE_META = 1;
*/
GET_TABLE_META(1),
/**
* CREATE_TABLET = 2;
*/
CREATE_TABLET(2),
/**
* GET_MUTATE_WORKER = 3;
*/
GET_MUTATE_WORKER(3),
/**
* COMMIT_TABLET = 4;
*/
COMMIT_TABLET(4),
/**
* DROP_TABLET = 5;
*/
DROP_TABLET(5),
/**
* CHECK_TABLE_EXISTS = 6;
*/
CHECK_TABLE_EXISTS(6),
/**
*
*** BULKLOAD ENUMS **
*
*
* CREATE_BULK_LOAD_STREAM = 11;
*/
CREATE_BULK_LOAD_STREAM(11),
/**
* GET_BULK_LOAD_STREAM = 12;
*/
GET_BULK_LOAD_STREAM(12),
/**
* COMMIT_BULK_LOAD_STREAM = 13;
*/
COMMIT_BULK_LOAD_STREAM(13),
/**
* OPEN_BULK_LOAD_STREAM_WRITER = 14;
*/
OPEN_BULK_LOAD_STREAM_WRITER(14),
/**
* FINISH_BULK_LOAD_STREAM_WRITER = 15;
*/
FINISH_BULK_LOAD_STREAM_WRITER(15),
/**
*
*** V2 INTERFACE ENUMS **
*
*
* CREATE_OR_GET_STREAM_V2 = 16;
*/
CREATE_OR_GET_STREAM_V2(16),
/**
* CLOSE_STREAM_V2 = 17;
*/
CLOSE_STREAM_V2(17),
/**
* GET_ROUTE_WORKER_V2 = 18;
*/
GET_ROUTE_WORKER_V2(18),
/**
* CREATE_BULK_LOAD_STREAM_V2 = 19;
*/
CREATE_BULK_LOAD_STREAM_V2(19),
/**
* GET_BULK_LOAD_STREAM_V2 = 20;
*/
GET_BULK_LOAD_STREAM_V2(20),
/**
* COMMIT_BULK_LOAD_STREAM_V2 = 21;
*/
COMMIT_BULK_LOAD_STREAM_V2(21),
/**
* OPEN_BULK_LOAD_STREAM_WRITER_V2 = 22;
*/
OPEN_BULK_LOAD_STREAM_WRITER_V2(22),
/**
* FINISH_BULK_LOAD_STREAM_WRITER_V2 = 23;
*/
FINISH_BULK_LOAD_STREAM_WRITER_V2(23),
/**
* GET_BULK_LOAD_STREAM_STS_TOKEN_V2 = 24;
*/
GET_BULK_LOAD_STREAM_STS_TOKEN_V2(24),
/**
* COMMIT_V2 = 25;
*/
COMMIT_V2(25),
/**
* ASYNC_COMMIT_V2 = 26;
*/
ASYNC_COMMIT_V2(26),
/**
* CHECK_COMMIT_RESULT_V2 = 27;
*/
CHECK_COMMIT_RESULT_V2(27),
/**
*
*** ROUTER SERVICE INTERFACE ENUMS **
*
*
* GET_ROUTER_CONTROLLER_ADDRESS = 28;
*/
GET_ROUTER_CONTROLLER_ADDRESS(28),
/**
*
*** Schema Change **
*
*
* SCHEMA_CHANGE = 29;
*/
SCHEMA_CHANGE(29),
UNRECOGNIZED(-1),
;
/**
* GATEWAY_RPC_CALL = 0;
*/
public static final int GATEWAY_RPC_CALL_VALUE = 0;
/**
* GET_TABLE_META = 1;
*/
public static final int GET_TABLE_META_VALUE = 1;
/**
* CREATE_TABLET = 2;
*/
public static final int CREATE_TABLET_VALUE = 2;
/**
* GET_MUTATE_WORKER = 3;
*/
public static final int GET_MUTATE_WORKER_VALUE = 3;
/**
* COMMIT_TABLET = 4;
*/
public static final int COMMIT_TABLET_VALUE = 4;
/**
* DROP_TABLET = 5;
*/
public static final int DROP_TABLET_VALUE = 5;
/**
* CHECK_TABLE_EXISTS = 6;
*/
public static final int CHECK_TABLE_EXISTS_VALUE = 6;
/**
*
*** BULKLOAD ENUMS **
*
*
* CREATE_BULK_LOAD_STREAM = 11;
*/
public static final int CREATE_BULK_LOAD_STREAM_VALUE = 11;
/**
* GET_BULK_LOAD_STREAM = 12;
*/
public static final int GET_BULK_LOAD_STREAM_VALUE = 12;
/**
* COMMIT_BULK_LOAD_STREAM = 13;
*/
public static final int COMMIT_BULK_LOAD_STREAM_VALUE = 13;
/**
* OPEN_BULK_LOAD_STREAM_WRITER = 14;
*/
public static final int OPEN_BULK_LOAD_STREAM_WRITER_VALUE = 14;
/**
* FINISH_BULK_LOAD_STREAM_WRITER = 15;
*/
public static final int FINISH_BULK_LOAD_STREAM_WRITER_VALUE = 15;
/**
*
*** V2 INTERFACE ENUMS **
*
*
* CREATE_OR_GET_STREAM_V2 = 16;
*/
public static final int CREATE_OR_GET_STREAM_V2_VALUE = 16;
/**
* CLOSE_STREAM_V2 = 17;
*/
public static final int CLOSE_STREAM_V2_VALUE = 17;
/**
* GET_ROUTE_WORKER_V2 = 18;
*/
public static final int GET_ROUTE_WORKER_V2_VALUE = 18;
/**
* CREATE_BULK_LOAD_STREAM_V2 = 19;
*/
public static final int CREATE_BULK_LOAD_STREAM_V2_VALUE = 19;
/**
* GET_BULK_LOAD_STREAM_V2 = 20;
*/
public static final int GET_BULK_LOAD_STREAM_V2_VALUE = 20;
/**
* COMMIT_BULK_LOAD_STREAM_V2 = 21;
*/
public static final int COMMIT_BULK_LOAD_STREAM_V2_VALUE = 21;
/**
* OPEN_BULK_LOAD_STREAM_WRITER_V2 = 22;
*/
public static final int OPEN_BULK_LOAD_STREAM_WRITER_V2_VALUE = 22;
/**
* FINISH_BULK_LOAD_STREAM_WRITER_V2 = 23;
*/
public static final int FINISH_BULK_LOAD_STREAM_WRITER_V2_VALUE = 23;
/**
* GET_BULK_LOAD_STREAM_STS_TOKEN_V2 = 24;
*/
public static final int GET_BULK_LOAD_STREAM_STS_TOKEN_V2_VALUE = 24;
/**
* COMMIT_V2 = 25;
*/
public static final int COMMIT_V2_VALUE = 25;
/**
* ASYNC_COMMIT_V2 = 26;
*/
public static final int ASYNC_COMMIT_V2_VALUE = 26;
/**
* CHECK_COMMIT_RESULT_V2 = 27;
*/
public static final int CHECK_COMMIT_RESULT_V2_VALUE = 27;
/**
*
*** ROUTER SERVICE INTERFACE ENUMS **
*
*
* GET_ROUTER_CONTROLLER_ADDRESS = 28;
*/
public static final int GET_ROUTER_CONTROLLER_ADDRESS_VALUE = 28;
/**
*
*** Schema Change **
*
*
* SCHEMA_CHANGE = 29;
*/
public static final int SCHEMA_CHANGE_VALUE = 29;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MethodEnum valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static MethodEnum forNumber(int value) {
switch (value) {
case 0: return GATEWAY_RPC_CALL;
case 1: return GET_TABLE_META;
case 2: return CREATE_TABLET;
case 3: return GET_MUTATE_WORKER;
case 4: return COMMIT_TABLET;
case 5: return DROP_TABLET;
case 6: return CHECK_TABLE_EXISTS;
case 11: return CREATE_BULK_LOAD_STREAM;
case 12: return GET_BULK_LOAD_STREAM;
case 13: return COMMIT_BULK_LOAD_STREAM;
case 14: return OPEN_BULK_LOAD_STREAM_WRITER;
case 15: return FINISH_BULK_LOAD_STREAM_WRITER;
case 16: return CREATE_OR_GET_STREAM_V2;
case 17: return CLOSE_STREAM_V2;
case 18: return GET_ROUTE_WORKER_V2;
case 19: return CREATE_BULK_LOAD_STREAM_V2;
case 20: return GET_BULK_LOAD_STREAM_V2;
case 21: return COMMIT_BULK_LOAD_STREAM_V2;
case 22: return OPEN_BULK_LOAD_STREAM_WRITER_V2;
case 23: return FINISH_BULK_LOAD_STREAM_WRITER_V2;
case 24: return GET_BULK_LOAD_STREAM_STS_TOKEN_V2;
case 25: return COMMIT_V2;
case 26: return ASYNC_COMMIT_V2;
case 27: return CHECK_COMMIT_RESULT_V2;
case 28: return GET_ROUTER_CONTROLLER_ADDRESS;
case 29: return SCHEMA_CHANGE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MethodEnum> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MethodEnum findValueByNumber(int number) {
return MethodEnum.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.getDescriptor().getEnumTypes().get(3);
}
private static final MethodEnum[] VALUES = values();
public static MethodEnum valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private MethodEnum(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.MethodEnum)
}
public interface GetTableMetaRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTableMetaRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string client_id = 1;
* @return The clientId.
*/
java.lang.String getClientId();
/**
* string client_id = 1;
* @return The bytes for clientId.
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
* string schema_name = 2;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 3;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* string workspace = 4;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 4;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* int64 instance_id = 5;
* @return The instanceId.
*/
long getInstanceId();
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 6;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTableMetaRequest}
*/
public static final class GetTableMetaRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTableMetaRequest)
GetTableMetaRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTableMetaRequest.newBuilder() to construct.
private GetTableMetaRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTableMetaRequest() {
clientId_ = "";
schemaName_ = "";
tableName_ = "";
workspace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTableMetaRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTableMetaRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
clientId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 40: {
instanceId_ = input.readInt64();
break;
}
case 50: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTableMetaRequest.class, cz.proto.ingestion.Ingestion.GetTableMetaRequest.Builder.class);
}
public static final int CLIENT_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object clientId_;
/**
* string client_id = 1;
* @return The clientId.
*/
@java.lang.Override
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
}
}
/**
* string client_id = 1;
* @return The bytes for clientId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 2;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object tableName_;
/**
* string table_name = 3;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WORKSPACE_FIELD_NUMBER = 4;
private volatile java.lang.Object workspace_;
/**
* string workspace = 4;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 4;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_ID_FIELD_NUMBER = 5;
private long instanceId_;
/**
* int64 instance_id = 5;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int ACCOUNT_FIELD_NUMBER = 6;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(clientId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, clientId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, tableName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, workspace_);
}
if (instanceId_ != 0L) {
output.writeInt64(5, instanceId_);
}
if (account_ != null) {
output.writeMessage(6, getAccount());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(clientId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, clientId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, tableName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, workspace_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, instanceId_);
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getAccount());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTableMetaRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTableMetaRequest other = (cz.proto.ingestion.Ingestion.GetTableMetaRequest) obj;
if (!getClientId()
.equals(other.getClientId())) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTableMetaRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTableMetaRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTableMetaRequest)
cz.proto.ingestion.Ingestion.GetTableMetaRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTableMetaRequest.class, cz.proto.ingestion.Ingestion.GetTableMetaRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTableMetaRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
clientId_ = "";
schemaName_ = "";
tableName_ = "";
workspace_ = "";
instanceId_ = 0L;
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTableMetaRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaRequest build() {
cz.proto.ingestion.Ingestion.GetTableMetaRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaRequest buildPartial() {
cz.proto.ingestion.Ingestion.GetTableMetaRequest result = new cz.proto.ingestion.Ingestion.GetTableMetaRequest(this);
result.clientId_ = clientId_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
result.workspace_ = workspace_;
result.instanceId_ = instanceId_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTableMetaRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTableMetaRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTableMetaRequest other) {
if (other == cz.proto.ingestion.Ingestion.GetTableMetaRequest.getDefaultInstance()) return this;
if (!other.getClientId().isEmpty()) {
clientId_ = other.clientId_;
onChanged();
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTableMetaRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTableMetaRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object clientId_ = "";
/**
* string client_id = 1;
* @return The clientId.
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string client_id = 1;
* @return The bytes for clientId.
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string client_id = 1;
* @param value The clientId to set.
* @return This builder for chaining.
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientId_ = value;
onChanged();
return this;
}
/**
* string client_id = 1;
* @return This builder for chaining.
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
onChanged();
return this;
}
/**
* string client_id = 1;
* @param value The bytes for clientId to set.
* @return This builder for chaining.
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientId_ = value;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 2;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 2;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 2;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 2;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 3;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 3;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 3;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 3;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 4;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 4;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 4;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 4;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 4;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 5;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 5;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 5;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTableMetaRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTableMetaRequest)
private static final cz.proto.ingestion.Ingestion.GetTableMetaRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTableMetaRequest();
}
public static cz.proto.ingestion.Ingestion.GetTableMetaRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTableMetaRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTableMetaRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTableMetaResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTableMetaResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.Entity table_meta = 1;
* @return Whether the tableMeta field is set.
*/
boolean hasTableMeta();
/**
* .cz.proto.Entity table_meta = 1;
* @return The tableMeta.
*/
cz.proto.MetadataEntity.Entity getTableMeta();
/**
* .cz.proto.Entity table_meta = 1;
*/
cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder();
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The enum numeric value on the wire for tableType.
*/
int getTableTypeValue();
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The tableType.
*/
cz.proto.ingestion.Ingestion.IGSTableType getTableType();
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
long getInstanceId();
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTableMetaResponse}
*/
public static final class GetTableMetaResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTableMetaResponse)
GetTableMetaResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTableMetaResponse.newBuilder() to construct.
private GetTableMetaResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTableMetaResponse() {
tableType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTableMetaResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTableMetaResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.MetadataEntity.Entity.Builder subBuilder = null;
if (tableMeta_ != null) {
subBuilder = tableMeta_.toBuilder();
}
tableMeta_ = input.readMessage(cz.proto.MetadataEntity.Entity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableMeta_);
tableMeta_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
tableType_ = rawValue;
break;
}
case 24: {
instanceId_ = input.readInt64();
break;
}
case 34: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTableMetaResponse.class, cz.proto.ingestion.Ingestion.GetTableMetaResponse.Builder.class);
}
public static final int TABLE_META_FIELD_NUMBER = 1;
private cz.proto.MetadataEntity.Entity tableMeta_;
/**
* .cz.proto.Entity table_meta = 1;
* @return Whether the tableMeta field is set.
*/
@java.lang.Override
public boolean hasTableMeta() {
return tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 1;
* @return The tableMeta.
*/
@java.lang.Override
public cz.proto.MetadataEntity.Entity getTableMeta() {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
/**
* .cz.proto.Entity table_meta = 1;
*/
@java.lang.Override
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
return getTableMeta();
}
public static final int TABLE_TYPE_FIELD_NUMBER = 2;
private int tableType_;
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The tableType.
*/
@java.lang.Override public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
public static final int INSTANCE_ID_FIELD_NUMBER = 3;
private long instanceId_;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int STATUS_FIELD_NUMBER = 4;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tableMeta_ != null) {
output.writeMessage(1, getTableMeta());
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
output.writeEnum(2, tableType_);
}
if (instanceId_ != 0L) {
output.writeInt64(3, instanceId_);
}
if (status_ != null) {
output.writeMessage(4, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tableMeta_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTableMeta());
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, tableType_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, instanceId_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTableMetaResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTableMetaResponse other = (cz.proto.ingestion.Ingestion.GetTableMetaResponse) obj;
if (hasTableMeta() != other.hasTableMeta()) return false;
if (hasTableMeta()) {
if (!getTableMeta()
.equals(other.getTableMeta())) return false;
}
if (tableType_ != other.tableType_) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTableMeta()) {
hash = (37 * hash) + TABLE_META_FIELD_NUMBER;
hash = (53 * hash) + getTableMeta().hashCode();
}
hash = (37 * hash) + TABLE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + tableType_;
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTableMetaResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTableMetaResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTableMetaResponse)
cz.proto.ingestion.Ingestion.GetTableMetaResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTableMetaResponse.class, cz.proto.ingestion.Ingestion.GetTableMetaResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTableMetaResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
tableType_ = 0;
instanceId_ = 0L;
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTableMetaResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTableMetaResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaResponse build() {
cz.proto.ingestion.Ingestion.GetTableMetaResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaResponse buildPartial() {
cz.proto.ingestion.Ingestion.GetTableMetaResponse result = new cz.proto.ingestion.Ingestion.GetTableMetaResponse(this);
if (tableMetaBuilder_ == null) {
result.tableMeta_ = tableMeta_;
} else {
result.tableMeta_ = tableMetaBuilder_.build();
}
result.tableType_ = tableType_;
result.instanceId_ = instanceId_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTableMetaResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTableMetaResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTableMetaResponse other) {
if (other == cz.proto.ingestion.Ingestion.GetTableMetaResponse.getDefaultInstance()) return this;
if (other.hasTableMeta()) {
mergeTableMeta(other.getTableMeta());
}
if (other.tableType_ != 0) {
setTableTypeValue(other.getTableTypeValue());
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTableMetaResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTableMetaResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.MetadataEntity.Entity tableMeta_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder> tableMetaBuilder_;
/**
* .cz.proto.Entity table_meta = 1;
* @return Whether the tableMeta field is set.
*/
public boolean hasTableMeta() {
return tableMetaBuilder_ != null || tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 1;
* @return The tableMeta.
*/
public cz.proto.MetadataEntity.Entity getTableMeta() {
if (tableMetaBuilder_ == null) {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
} else {
return tableMetaBuilder_.getMessage();
}
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public Builder setTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableMeta_ = value;
onChanged();
} else {
tableMetaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public Builder setTableMeta(
cz.proto.MetadataEntity.Entity.Builder builderForValue) {
if (tableMetaBuilder_ == null) {
tableMeta_ = builderForValue.build();
onChanged();
} else {
tableMetaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public Builder mergeTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (tableMeta_ != null) {
tableMeta_ =
cz.proto.MetadataEntity.Entity.newBuilder(tableMeta_).mergeFrom(value).buildPartial();
} else {
tableMeta_ = value;
}
onChanged();
} else {
tableMetaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public Builder clearTableMeta() {
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
onChanged();
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public cz.proto.MetadataEntity.Entity.Builder getTableMetaBuilder() {
onChanged();
return getTableMetaFieldBuilder().getBuilder();
}
/**
* .cz.proto.Entity table_meta = 1;
*/
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
if (tableMetaBuilder_ != null) {
return tableMetaBuilder_.getMessageOrBuilder();
} else {
return tableMeta_ == null ?
cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
}
/**
* .cz.proto.Entity table_meta = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>
getTableMetaFieldBuilder() {
if (tableMetaBuilder_ == null) {
tableMetaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>(
getTableMeta(),
getParentForChildren(),
isClean());
tableMeta_ = null;
}
return tableMetaBuilder_;
}
private int tableType_ = 0;
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @param value The enum numeric value on the wire for tableType to set.
* @return This builder for chaining.
*/
public Builder setTableTypeValue(int value) {
tableType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return The tableType.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @param value The tableType to set.
* @return This builder for chaining.
*/
public Builder setTableType(cz.proto.ingestion.Ingestion.IGSTableType value) {
if (value == null) {
throw new NullPointerException();
}
tableType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 2;
* @return This builder for chaining.
*/
public Builder clearTableType() {
tableType_ = 0;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 3;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 3;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 3;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTableMetaResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTableMetaResponse)
private static final cz.proto.ingestion.Ingestion.GetTableMetaResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTableMetaResponse();
}
public static cz.proto.ingestion.Ingestion.GetTableMetaResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTableMetaResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTableMetaResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTableMetaResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ControllerCreateTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.ControllerCreateTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string schema_name = 1;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 1;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 2;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 2;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* .cz.proto.Entity table_meta = 3;
* @return Whether the tableMeta field is set.
*/
boolean hasTableMeta();
/**
* .cz.proto.Entity table_meta = 3;
* @return The tableMeta.
*/
cz.proto.MetadataEntity.Entity getTableMeta();
/**
* .cz.proto.Entity table_meta = 3;
*/
cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder();
/**
* int64 tablet_nums = 4;
* @return The tabletNums.
*/
long getTabletNums();
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The enum numeric value on the wire for tableType.
*/
int getTableTypeValue();
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The tableType.
*/
cz.proto.ingestion.Ingestion.IGSTableType getTableType();
/**
* repeated string key_names = 6;
* @return A list containing the keyNames.
*/
java.util.List
getKeyNamesList();
/**
* repeated string key_names = 6;
* @return The count of keyNames.
*/
int getKeyNamesCount();
/**
* repeated string key_names = 6;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
java.lang.String getKeyNames(int index);
/**
* repeated string key_names = 6;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
com.google.protobuf.ByteString
getKeyNamesBytes(int index);
/**
* uint64 buckets_count = 7;
* @return The bucketsCount.
*/
long getBucketsCount();
/**
* repeated string sort_names = 8;
* @return A list containing the sortNames.
*/
java.util.List
getSortNamesList();
/**
* repeated string sort_names = 8;
* @return The count of sortNames.
*/
int getSortNamesCount();
/**
* repeated string sort_names = 8;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
java.lang.String getSortNames(int index);
/**
* repeated string sort_names = 8;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
com.google.protobuf.ByteString
getSortNamesBytes(int index);
/**
* string workspace = 9;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 9;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* int64 instance_id = 10;
* @return The instanceId.
*/
long getInstanceId();
/**
* .cz.proto.ingestion.Account account = 11;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 11;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 11;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.ControllerCreateTabletRequest}
*/
public static final class ControllerCreateTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.ControllerCreateTabletRequest)
ControllerCreateTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use ControllerCreateTabletRequest.newBuilder() to construct.
private ControllerCreateTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ControllerCreateTabletRequest() {
schemaName_ = "";
tableName_ = "";
tableType_ = 0;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
workspace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ControllerCreateTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ControllerCreateTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 26: {
cz.proto.MetadataEntity.Entity.Builder subBuilder = null;
if (tableMeta_ != null) {
subBuilder = tableMeta_.toBuilder();
}
tableMeta_ = input.readMessage(cz.proto.MetadataEntity.Entity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableMeta_);
tableMeta_ = subBuilder.buildPartial();
}
break;
}
case 32: {
tabletNums_ = input.readInt64();
break;
}
case 40: {
int rawValue = input.readEnum();
tableType_ = rawValue;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
keyNames_.add(s);
break;
}
case 56: {
bucketsCount_ = input.readUInt64();
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
sortNames_.add(s);
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 80: {
instanceId_ = input.readInt64();
break;
}
case 90: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_ControllerCreateTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_ControllerCreateTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.class, cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.Builder.class);
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 1;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 1;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object tableName_;
/**
* string table_name = 2;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 2;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_META_FIELD_NUMBER = 3;
private cz.proto.MetadataEntity.Entity tableMeta_;
/**
* .cz.proto.Entity table_meta = 3;
* @return Whether the tableMeta field is set.
*/
@java.lang.Override
public boolean hasTableMeta() {
return tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 3;
* @return The tableMeta.
*/
@java.lang.Override
public cz.proto.MetadataEntity.Entity getTableMeta() {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
/**
* .cz.proto.Entity table_meta = 3;
*/
@java.lang.Override
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
return getTableMeta();
}
public static final int TABLET_NUMS_FIELD_NUMBER = 4;
private long tabletNums_;
/**
* int64 tablet_nums = 4;
* @return The tabletNums.
*/
@java.lang.Override
public long getTabletNums() {
return tabletNums_;
}
public static final int TABLE_TYPE_FIELD_NUMBER = 5;
private int tableType_;
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The tableType.
*/
@java.lang.Override public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
public static final int KEY_NAMES_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList keyNames_;
/**
* repeated string key_names = 6;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_;
}
/**
* repeated string key_names = 6;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 6;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 6;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
public static final int BUCKETS_COUNT_FIELD_NUMBER = 7;
private long bucketsCount_;
/**
* uint64 buckets_count = 7;
* @return The bucketsCount.
*/
@java.lang.Override
public long getBucketsCount() {
return bucketsCount_;
}
public static final int SORT_NAMES_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList sortNames_;
/**
* repeated string sort_names = 8;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_;
}
/**
* repeated string sort_names = 8;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 8;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 8;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
public static final int WORKSPACE_FIELD_NUMBER = 9;
private volatile java.lang.Object workspace_;
/**
* string workspace = 9;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 9;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_ID_FIELD_NUMBER = 10;
private long instanceId_;
/**
* int64 instance_id = 10;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int ACCOUNT_FIELD_NUMBER = 11;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 11;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 11;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, tableName_);
}
if (tableMeta_ != null) {
output.writeMessage(3, getTableMeta());
}
if (tabletNums_ != 0L) {
output.writeInt64(4, tabletNums_);
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
output.writeEnum(5, tableType_);
}
for (int i = 0; i < keyNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, keyNames_.getRaw(i));
}
if (bucketsCount_ != 0L) {
output.writeUInt64(7, bucketsCount_);
}
for (int i = 0; i < sortNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, sortNames_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, workspace_);
}
if (instanceId_ != 0L) {
output.writeInt64(10, instanceId_);
}
if (account_ != null) {
output.writeMessage(11, getAccount());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, tableName_);
}
if (tableMeta_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTableMeta());
}
if (tabletNums_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, tabletNums_);
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, tableType_);
}
{
int dataSize = 0;
for (int i = 0; i < keyNames_.size(); i++) {
dataSize += computeStringSizeNoTag(keyNames_.getRaw(i));
}
size += dataSize;
size += 1 * getKeyNamesList().size();
}
if (bucketsCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(7, bucketsCount_);
}
{
int dataSize = 0;
for (int i = 0; i < sortNames_.size(); i++) {
dataSize += computeStringSizeNoTag(sortNames_.getRaw(i));
}
size += dataSize;
size += 1 * getSortNamesList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, workspace_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, instanceId_);
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getAccount());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest other = (cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest) obj;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (hasTableMeta() != other.hasTableMeta()) return false;
if (hasTableMeta()) {
if (!getTableMeta()
.equals(other.getTableMeta())) return false;
}
if (getTabletNums()
!= other.getTabletNums()) return false;
if (tableType_ != other.tableType_) return false;
if (!getKeyNamesList()
.equals(other.getKeyNamesList())) return false;
if (getBucketsCount()
!= other.getBucketsCount()) return false;
if (!getSortNamesList()
.equals(other.getSortNamesList())) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
if (hasTableMeta()) {
hash = (37 * hash) + TABLE_META_FIELD_NUMBER;
hash = (53 * hash) + getTableMeta().hashCode();
}
hash = (37 * hash) + TABLET_NUMS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletNums());
hash = (37 * hash) + TABLE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + tableType_;
if (getKeyNamesCount() > 0) {
hash = (37 * hash) + KEY_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getKeyNamesList().hashCode();
}
hash = (37 * hash) + BUCKETS_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBucketsCount());
if (getSortNamesCount() > 0) {
hash = (37 * hash) + SORT_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getSortNamesList().hashCode();
}
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.ControllerCreateTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.ControllerCreateTabletRequest)
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_ControllerCreateTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_ControllerCreateTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.class, cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
schemaName_ = "";
tableName_ = "";
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
tabletNums_ = 0L;
tableType_ = 0;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
bucketsCount_ = 0L;
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
workspace_ = "";
instanceId_ = 0L;
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_ControllerCreateTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest build() {
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest result = new cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest(this);
int from_bitField0_ = bitField0_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
if (tableMetaBuilder_ == null) {
result.tableMeta_ = tableMeta_;
} else {
result.tableMeta_ = tableMetaBuilder_.build();
}
result.tabletNums_ = tabletNums_;
result.tableType_ = tableType_;
if (((bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keyNames_ = keyNames_;
result.bucketsCount_ = bucketsCount_;
if (((bitField0_ & 0x00000002) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.sortNames_ = sortNames_;
result.workspace_ = workspace_;
result.instanceId_ = instanceId_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest.getDefaultInstance()) return this;
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.hasTableMeta()) {
mergeTableMeta(other.getTableMeta());
}
if (other.getTabletNums() != 0L) {
setTabletNums(other.getTabletNums());
}
if (other.tableType_ != 0) {
setTableTypeValue(other.getTableTypeValue());
}
if (!other.keyNames_.isEmpty()) {
if (keyNames_.isEmpty()) {
keyNames_ = other.keyNames_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeyNamesIsMutable();
keyNames_.addAll(other.keyNames_);
}
onChanged();
}
if (other.getBucketsCount() != 0L) {
setBucketsCount(other.getBucketsCount());
}
if (!other.sortNames_.isEmpty()) {
if (sortNames_.isEmpty()) {
sortNames_ = other.sortNames_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSortNamesIsMutable();
sortNames_.addAll(other.sortNames_);
}
onChanged();
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 1;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 1;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 1;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 1;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 1;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 2;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 2;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 2;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 2;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 2;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private cz.proto.MetadataEntity.Entity tableMeta_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder> tableMetaBuilder_;
/**
* .cz.proto.Entity table_meta = 3;
* @return Whether the tableMeta field is set.
*/
public boolean hasTableMeta() {
return tableMetaBuilder_ != null || tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 3;
* @return The tableMeta.
*/
public cz.proto.MetadataEntity.Entity getTableMeta() {
if (tableMetaBuilder_ == null) {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
} else {
return tableMetaBuilder_.getMessage();
}
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public Builder setTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableMeta_ = value;
onChanged();
} else {
tableMetaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public Builder setTableMeta(
cz.proto.MetadataEntity.Entity.Builder builderForValue) {
if (tableMetaBuilder_ == null) {
tableMeta_ = builderForValue.build();
onChanged();
} else {
tableMetaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public Builder mergeTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (tableMeta_ != null) {
tableMeta_ =
cz.proto.MetadataEntity.Entity.newBuilder(tableMeta_).mergeFrom(value).buildPartial();
} else {
tableMeta_ = value;
}
onChanged();
} else {
tableMetaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public Builder clearTableMeta() {
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
onChanged();
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public cz.proto.MetadataEntity.Entity.Builder getTableMetaBuilder() {
onChanged();
return getTableMetaFieldBuilder().getBuilder();
}
/**
* .cz.proto.Entity table_meta = 3;
*/
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
if (tableMetaBuilder_ != null) {
return tableMetaBuilder_.getMessageOrBuilder();
} else {
return tableMeta_ == null ?
cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
}
/**
* .cz.proto.Entity table_meta = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>
getTableMetaFieldBuilder() {
if (tableMetaBuilder_ == null) {
tableMetaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>(
getTableMeta(),
getParentForChildren(),
isClean());
tableMeta_ = null;
}
return tableMetaBuilder_;
}
private long tabletNums_ ;
/**
* int64 tablet_nums = 4;
* @return The tabletNums.
*/
@java.lang.Override
public long getTabletNums() {
return tabletNums_;
}
/**
* int64 tablet_nums = 4;
* @param value The tabletNums to set.
* @return This builder for chaining.
*/
public Builder setTabletNums(long value) {
tabletNums_ = value;
onChanged();
return this;
}
/**
* int64 tablet_nums = 4;
* @return This builder for chaining.
*/
public Builder clearTabletNums() {
tabletNums_ = 0L;
onChanged();
return this;
}
private int tableType_ = 0;
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @param value The enum numeric value on the wire for tableType to set.
* @return This builder for chaining.
*/
public Builder setTableTypeValue(int value) {
tableType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return The tableType.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @param value The tableType to set.
* @return This builder for chaining.
*/
public Builder setTableType(cz.proto.ingestion.Ingestion.IGSTableType value) {
if (value == null) {
throw new NullPointerException();
}
tableType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 5;
* @return This builder for chaining.
*/
public Builder clearTableType() {
tableType_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureKeyNamesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList(keyNames_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string key_names = 6;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_.getUnmodifiableView();
}
/**
* repeated string key_names = 6;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 6;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 6;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
/**
* repeated string key_names = 6;
* @param index The index to set the value at.
* @param value The keyNames to set.
* @return This builder for chaining.
*/
public Builder setKeyNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string key_names = 6;
* @param value The keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
/**
* repeated string key_names = 6;
* @param values The keyNames to add.
* @return This builder for chaining.
*/
public Builder addAllKeyNames(
java.lang.Iterable values) {
ensureKeyNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keyNames_);
onChanged();
return this;
}
/**
* repeated string key_names = 6;
* @return This builder for chaining.
*/
public Builder clearKeyNames() {
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string key_names = 6;
* @param value The bytes of the keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
private long bucketsCount_ ;
/**
* uint64 buckets_count = 7;
* @return The bucketsCount.
*/
@java.lang.Override
public long getBucketsCount() {
return bucketsCount_;
}
/**
* uint64 buckets_count = 7;
* @param value The bucketsCount to set.
* @return This builder for chaining.
*/
public Builder setBucketsCount(long value) {
bucketsCount_ = value;
onChanged();
return this;
}
/**
* uint64 buckets_count = 7;
* @return This builder for chaining.
*/
public Builder clearBucketsCount() {
bucketsCount_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSortNamesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList(sortNames_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string sort_names = 8;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_.getUnmodifiableView();
}
/**
* repeated string sort_names = 8;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 8;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 8;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
/**
* repeated string sort_names = 8;
* @param index The index to set the value at.
* @param value The sortNames to set.
* @return This builder for chaining.
*/
public Builder setSortNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sort_names = 8;
* @param value The sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
/**
* repeated string sort_names = 8;
* @param values The sortNames to add.
* @return This builder for chaining.
*/
public Builder addAllSortNames(
java.lang.Iterable values) {
ensureSortNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sortNames_);
onChanged();
return this;
}
/**
* repeated string sort_names = 8;
* @return This builder for chaining.
*/
public Builder clearSortNames() {
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string sort_names = 8;
* @param value The bytes of the sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 9;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 9;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 9;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 9;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 9;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 10;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 10;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 10;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 11;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 11;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.ControllerCreateTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.ControllerCreateTabletRequest)
private static final cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest();
}
public static cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ControllerCreateTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ControllerCreateTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.ControllerCreateTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WorkerCreateTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.WorkerCreateTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string schema_name = 2;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 3;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return Whether the tabletMeta field is set.
*/
boolean hasTabletMeta();
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return The tabletMeta.
*/
org.apache.kudu.KuduCommon.SchemaPB getTabletMeta();
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
org.apache.kudu.KuduCommon.SchemaPBOrBuilder getTabletMetaOrBuilder();
/**
* int64 worker_id = 5;
* @return The workerId.
*/
long getWorkerId();
/**
* .cz.proto.Entity table_meta = 6;
* @return Whether the tableMeta field is set.
*/
boolean hasTableMeta();
/**
* .cz.proto.Entity table_meta = 6;
* @return The tableMeta.
*/
cz.proto.MetadataEntity.Entity getTableMeta();
/**
* .cz.proto.Entity table_meta = 6;
*/
cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder();
/**
* string table_path = 7;
* @return The tablePath.
*/
java.lang.String getTablePath();
/**
* string table_path = 7;
* @return The bytes for tablePath.
*/
com.google.protobuf.ByteString
getTablePathBytes();
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
int getTableTypeValue();
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
cz.proto.ingestion.Ingestion.IGSTableType getTableType();
/**
* repeated string key_names = 9;
* @return A list containing the keyNames.
*/
java.util.List
getKeyNamesList();
/**
* repeated string key_names = 9;
* @return The count of keyNames.
*/
int getKeyNamesCount();
/**
* repeated string key_names = 9;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
java.lang.String getKeyNames(int index);
/**
* repeated string key_names = 9;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
com.google.protobuf.ByteString
getKeyNamesBytes(int index);
/**
* repeated uint64 hash_range_list = 10;
* @return A list containing the hashRangeList.
*/
java.util.List getHashRangeListList();
/**
* repeated uint64 hash_range_list = 10;
* @return The count of hashRangeList.
*/
int getHashRangeListCount();
/**
* repeated uint64 hash_range_list = 10;
* @param index The index of the element to return.
* @return The hashRangeList at the given index.
*/
long getHashRangeList(int index);
/**
* repeated uint32 buckets_list = 11;
* @return A list containing the bucketsList.
*/
java.util.List getBucketsListList();
/**
* repeated uint32 buckets_list = 11;
* @return The count of bucketsList.
*/
int getBucketsListCount();
/**
* repeated uint32 buckets_list = 11;
* @param index The index of the element to return.
* @return The bucketsList at the given index.
*/
int getBucketsList(int index);
/**
* repeated string sort_names = 12;
* @return A list containing the sortNames.
*/
java.util.List
getSortNamesList();
/**
* repeated string sort_names = 12;
* @return The count of sortNames.
*/
int getSortNamesCount();
/**
* repeated string sort_names = 12;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
java.lang.String getSortNames(int index);
/**
* repeated string sort_names = 12;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
com.google.protobuf.ByteString
getSortNamesBytes(int index);
/**
* string workspace = 13;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 13;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* int64 instance_id = 14;
* @return The instanceId.
*/
long getInstanceId();
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
java.util.List
getPartitionInfosList();
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
cz.proto.ingestion.Ingestion.PartitionColumnInfo getPartitionInfos(int index);
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
int getPartitionInfosCount();
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
java.util.List extends cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder>
getPartitionInfosOrBuilderList();
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder getPartitionInfosOrBuilder(
int index);
/**
* .cz.proto.Entity table_full_meta = 16;
* @return Whether the tableFullMeta field is set.
*/
boolean hasTableFullMeta();
/**
* .cz.proto.Entity table_full_meta = 16;
* @return The tableFullMeta.
*/
cz.proto.MetadataEntity.Entity getTableFullMeta();
/**
* .cz.proto.Entity table_full_meta = 16;
*/
cz.proto.MetadataEntity.EntityOrBuilder getTableFullMetaOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerCreateTabletRequest}
*/
public static final class WorkerCreateTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.WorkerCreateTabletRequest)
WorkerCreateTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use WorkerCreateTabletRequest.newBuilder() to construct.
private WorkerCreateTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WorkerCreateTabletRequest() {
schemaName_ = "";
tableName_ = "";
tablePath_ = "";
tableType_ = 0;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
hashRangeList_ = emptyLongList();
bucketsList_ = emptyIntList();
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
workspace_ = "";
partitionInfos_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WorkerCreateTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WorkerCreateTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 34: {
org.apache.kudu.KuduCommon.SchemaPB.Builder subBuilder = null;
if (tabletMeta_ != null) {
subBuilder = tabletMeta_.toBuilder();
}
tabletMeta_ = input.readMessage(org.apache.kudu.KuduCommon.SchemaPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tabletMeta_);
tabletMeta_ = subBuilder.buildPartial();
}
break;
}
case 40: {
workerId_ = input.readInt64();
break;
}
case 50: {
cz.proto.MetadataEntity.Entity.Builder subBuilder = null;
if (tableMeta_ != null) {
subBuilder = tableMeta_.toBuilder();
}
tableMeta_ = input.readMessage(cz.proto.MetadataEntity.Entity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableMeta_);
tableMeta_ = subBuilder.buildPartial();
}
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
tablePath_ = s;
break;
}
case 64: {
int rawValue = input.readEnum();
tableType_ = rawValue;
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
keyNames_.add(s);
break;
}
case 80: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
hashRangeList_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
hashRangeList_.addLong(input.readUInt64());
break;
}
case 82: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
hashRangeList_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
hashRangeList_.addLong(input.readUInt64());
}
input.popLimit(limit);
break;
}
case 88: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
bucketsList_ = newIntList();
mutable_bitField0_ |= 0x00000004;
}
bucketsList_.addInt(input.readUInt32());
break;
}
case 90: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) != 0) && input.getBytesUntilLimit() > 0) {
bucketsList_ = newIntList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
bucketsList_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000008;
}
sortNames_.add(s);
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 112: {
instanceId_ = input.readInt64();
break;
}
case 122: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
partitionInfos_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
partitionInfos_.add(
input.readMessage(cz.proto.ingestion.Ingestion.PartitionColumnInfo.parser(), extensionRegistry));
break;
}
case 130: {
cz.proto.MetadataEntity.Entity.Builder subBuilder = null;
if (tableFullMeta_ != null) {
subBuilder = tableFullMeta_.toBuilder();
}
tableFullMeta_ = input.readMessage(cz.proto.MetadataEntity.Entity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableFullMeta_);
tableFullMeta_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
hashRangeList_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
bucketsList_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
partitionInfos_ = java.util.Collections.unmodifiableList(partitionInfos_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerCreateTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerCreateTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.class, cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 2;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object tableName_;
/**
* string table_name = 3;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLET_META_FIELD_NUMBER = 4;
private org.apache.kudu.KuduCommon.SchemaPB tabletMeta_;
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return Whether the tabletMeta field is set.
*/
@java.lang.Override
public boolean hasTabletMeta() {
return tabletMeta_ != null;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return The tabletMeta.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB getTabletMeta() {
return tabletMeta_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : tabletMeta_;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getTabletMetaOrBuilder() {
return getTabletMeta();
}
public static final int WORKER_ID_FIELD_NUMBER = 5;
private long workerId_;
/**
* int64 worker_id = 5;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
public static final int TABLE_META_FIELD_NUMBER = 6;
private cz.proto.MetadataEntity.Entity tableMeta_;
/**
* .cz.proto.Entity table_meta = 6;
* @return Whether the tableMeta field is set.
*/
@java.lang.Override
public boolean hasTableMeta() {
return tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 6;
* @return The tableMeta.
*/
@java.lang.Override
public cz.proto.MetadataEntity.Entity getTableMeta() {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
/**
* .cz.proto.Entity table_meta = 6;
*/
@java.lang.Override
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
return getTableMeta();
}
public static final int TABLE_PATH_FIELD_NUMBER = 7;
private volatile java.lang.Object tablePath_;
/**
* string table_path = 7;
* @return The tablePath.
*/
@java.lang.Override
public java.lang.String getTablePath() {
java.lang.Object ref = tablePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tablePath_ = s;
return s;
}
}
/**
* string table_path = 7;
* @return The bytes for tablePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTablePathBytes() {
java.lang.Object ref = tablePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tablePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_TYPE_FIELD_NUMBER = 8;
private int tableType_;
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
@java.lang.Override public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
public static final int KEY_NAMES_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList keyNames_;
/**
* repeated string key_names = 9;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_;
}
/**
* repeated string key_names = 9;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 9;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 9;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
public static final int HASH_RANGE_LIST_FIELD_NUMBER = 10;
private com.google.protobuf.Internal.LongList hashRangeList_;
/**
* repeated uint64 hash_range_list = 10;
* @return A list containing the hashRangeList.
*/
@java.lang.Override
public java.util.List
getHashRangeListList() {
return hashRangeList_;
}
/**
* repeated uint64 hash_range_list = 10;
* @return The count of hashRangeList.
*/
public int getHashRangeListCount() {
return hashRangeList_.size();
}
/**
* repeated uint64 hash_range_list = 10;
* @param index The index of the element to return.
* @return The hashRangeList at the given index.
*/
public long getHashRangeList(int index) {
return hashRangeList_.getLong(index);
}
private int hashRangeListMemoizedSerializedSize = -1;
public static final int BUCKETS_LIST_FIELD_NUMBER = 11;
private com.google.protobuf.Internal.IntList bucketsList_;
/**
* repeated uint32 buckets_list = 11;
* @return A list containing the bucketsList.
*/
@java.lang.Override
public java.util.List
getBucketsListList() {
return bucketsList_;
}
/**
* repeated uint32 buckets_list = 11;
* @return The count of bucketsList.
*/
public int getBucketsListCount() {
return bucketsList_.size();
}
/**
* repeated uint32 buckets_list = 11;
* @param index The index of the element to return.
* @return The bucketsList at the given index.
*/
public int getBucketsList(int index) {
return bucketsList_.getInt(index);
}
private int bucketsListMemoizedSerializedSize = -1;
public static final int SORT_NAMES_FIELD_NUMBER = 12;
private com.google.protobuf.LazyStringList sortNames_;
/**
* repeated string sort_names = 12;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_;
}
/**
* repeated string sort_names = 12;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 12;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 12;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
public static final int WORKSPACE_FIELD_NUMBER = 13;
private volatile java.lang.Object workspace_;
/**
* string workspace = 13;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 13;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_ID_FIELD_NUMBER = 14;
private long instanceId_;
/**
* int64 instance_id = 14;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int PARTITION_INFOS_FIELD_NUMBER = 15;
private java.util.List partitionInfos_;
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
@java.lang.Override
public java.util.List getPartitionInfosList() {
return partitionInfos_;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
@java.lang.Override
public java.util.List extends cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder>
getPartitionInfosOrBuilderList() {
return partitionInfos_;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
@java.lang.Override
public int getPartitionInfosCount() {
return partitionInfos_.size();
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfo getPartitionInfos(int index) {
return partitionInfos_.get(index);
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder getPartitionInfosOrBuilder(
int index) {
return partitionInfos_.get(index);
}
public static final int TABLE_FULL_META_FIELD_NUMBER = 16;
private cz.proto.MetadataEntity.Entity tableFullMeta_;
/**
* .cz.proto.Entity table_full_meta = 16;
* @return Whether the tableFullMeta field is set.
*/
@java.lang.Override
public boolean hasTableFullMeta() {
return tableFullMeta_ != null;
}
/**
* .cz.proto.Entity table_full_meta = 16;
* @return The tableFullMeta.
*/
@java.lang.Override
public cz.proto.MetadataEntity.Entity getTableFullMeta() {
return tableFullMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableFullMeta_;
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
@java.lang.Override
public cz.proto.MetadataEntity.EntityOrBuilder getTableFullMetaOrBuilder() {
return getTableFullMeta();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasTabletMeta()) {
if (!getTabletMeta().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, tableName_);
}
if (tabletMeta_ != null) {
output.writeMessage(4, getTabletMeta());
}
if (workerId_ != 0L) {
output.writeInt64(5, workerId_);
}
if (tableMeta_ != null) {
output.writeMessage(6, getTableMeta());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tablePath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, tablePath_);
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
output.writeEnum(8, tableType_);
}
for (int i = 0; i < keyNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, keyNames_.getRaw(i));
}
if (getHashRangeListList().size() > 0) {
output.writeUInt32NoTag(82);
output.writeUInt32NoTag(hashRangeListMemoizedSerializedSize);
}
for (int i = 0; i < hashRangeList_.size(); i++) {
output.writeUInt64NoTag(hashRangeList_.getLong(i));
}
if (getBucketsListList().size() > 0) {
output.writeUInt32NoTag(90);
output.writeUInt32NoTag(bucketsListMemoizedSerializedSize);
}
for (int i = 0; i < bucketsList_.size(); i++) {
output.writeUInt32NoTag(bucketsList_.getInt(i));
}
for (int i = 0; i < sortNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, sortNames_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, workspace_);
}
if (instanceId_ != 0L) {
output.writeInt64(14, instanceId_);
}
for (int i = 0; i < partitionInfos_.size(); i++) {
output.writeMessage(15, partitionInfos_.get(i));
}
if (tableFullMeta_ != null) {
output.writeMessage(16, getTableFullMeta());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, tableName_);
}
if (tabletMeta_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getTabletMeta());
}
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, workerId_);
}
if (tableMeta_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getTableMeta());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tablePath_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, tablePath_);
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, tableType_);
}
{
int dataSize = 0;
for (int i = 0; i < keyNames_.size(); i++) {
dataSize += computeStringSizeNoTag(keyNames_.getRaw(i));
}
size += dataSize;
size += 1 * getKeyNamesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < hashRangeList_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(hashRangeList_.getLong(i));
}
size += dataSize;
if (!getHashRangeListList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
hashRangeListMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < bucketsList_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(bucketsList_.getInt(i));
}
size += dataSize;
if (!getBucketsListList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
bucketsListMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < sortNames_.size(); i++) {
dataSize += computeStringSizeNoTag(sortNames_.getRaw(i));
}
size += dataSize;
size += 1 * getSortNamesList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, workspace_);
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, instanceId_);
}
for (int i = 0; i < partitionInfos_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, partitionInfos_.get(i));
}
if (tableFullMeta_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getTableFullMeta());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest other = (cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (hasTabletMeta() != other.hasTabletMeta()) return false;
if (hasTabletMeta()) {
if (!getTabletMeta()
.equals(other.getTabletMeta())) return false;
}
if (getWorkerId()
!= other.getWorkerId()) return false;
if (hasTableMeta() != other.hasTableMeta()) return false;
if (hasTableMeta()) {
if (!getTableMeta()
.equals(other.getTableMeta())) return false;
}
if (!getTablePath()
.equals(other.getTablePath())) return false;
if (tableType_ != other.tableType_) return false;
if (!getKeyNamesList()
.equals(other.getKeyNamesList())) return false;
if (!getHashRangeListList()
.equals(other.getHashRangeListList())) return false;
if (!getBucketsListList()
.equals(other.getBucketsListList())) return false;
if (!getSortNamesList()
.equals(other.getSortNamesList())) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getPartitionInfosList()
.equals(other.getPartitionInfosList())) return false;
if (hasTableFullMeta() != other.hasTableFullMeta()) return false;
if (hasTableFullMeta()) {
if (!getTableFullMeta()
.equals(other.getTableFullMeta())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
if (hasTabletMeta()) {
hash = (37 * hash) + TABLET_META_FIELD_NUMBER;
hash = (53 * hash) + getTabletMeta().hashCode();
}
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
if (hasTableMeta()) {
hash = (37 * hash) + TABLE_META_FIELD_NUMBER;
hash = (53 * hash) + getTableMeta().hashCode();
}
hash = (37 * hash) + TABLE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getTablePath().hashCode();
hash = (37 * hash) + TABLE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + tableType_;
if (getKeyNamesCount() > 0) {
hash = (37 * hash) + KEY_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getKeyNamesList().hashCode();
}
if (getHashRangeListCount() > 0) {
hash = (37 * hash) + HASH_RANGE_LIST_FIELD_NUMBER;
hash = (53 * hash) + getHashRangeListList().hashCode();
}
if (getBucketsListCount() > 0) {
hash = (37 * hash) + BUCKETS_LIST_FIELD_NUMBER;
hash = (53 * hash) + getBucketsListList().hashCode();
}
if (getSortNamesCount() > 0) {
hash = (37 * hash) + SORT_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getSortNamesList().hashCode();
}
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
if (getPartitionInfosCount() > 0) {
hash = (37 * hash) + PARTITION_INFOS_FIELD_NUMBER;
hash = (53 * hash) + getPartitionInfosList().hashCode();
}
if (hasTableFullMeta()) {
hash = (37 * hash) + TABLE_FULL_META_FIELD_NUMBER;
hash = (53 * hash) + getTableFullMeta().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerCreateTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.WorkerCreateTabletRequest)
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerCreateTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerCreateTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.class, cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPartitionInfosFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
schemaName_ = "";
tableName_ = "";
if (tabletMetaBuilder_ == null) {
tabletMeta_ = null;
} else {
tabletMeta_ = null;
tabletMetaBuilder_ = null;
}
workerId_ = 0L;
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
tablePath_ = "";
tableType_ = 0;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
hashRangeList_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
bucketsList_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
workspace_ = "";
instanceId_ = 0L;
if (partitionInfosBuilder_ == null) {
partitionInfos_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
partitionInfosBuilder_.clear();
}
if (tableFullMetaBuilder_ == null) {
tableFullMeta_ = null;
} else {
tableFullMeta_ = null;
tableFullMetaBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerCreateTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest build() {
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest result = new cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest(this);
int from_bitField0_ = bitField0_;
result.tabletId_ = tabletId_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
if (tabletMetaBuilder_ == null) {
result.tabletMeta_ = tabletMeta_;
} else {
result.tabletMeta_ = tabletMetaBuilder_.build();
}
result.workerId_ = workerId_;
if (tableMetaBuilder_ == null) {
result.tableMeta_ = tableMeta_;
} else {
result.tableMeta_ = tableMetaBuilder_.build();
}
result.tablePath_ = tablePath_;
result.tableType_ = tableType_;
if (((bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keyNames_ = keyNames_;
if (((bitField0_ & 0x00000002) != 0)) {
hashRangeList_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.hashRangeList_ = hashRangeList_;
if (((bitField0_ & 0x00000004) != 0)) {
bucketsList_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.bucketsList_ = bucketsList_;
if (((bitField0_ & 0x00000008) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000008);
}
result.sortNames_ = sortNames_;
result.workspace_ = workspace_;
result.instanceId_ = instanceId_;
if (partitionInfosBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
partitionInfos_ = java.util.Collections.unmodifiableList(partitionInfos_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.partitionInfos_ = partitionInfos_;
} else {
result.partitionInfos_ = partitionInfosBuilder_.build();
}
if (tableFullMetaBuilder_ == null) {
result.tableFullMeta_ = tableFullMeta_;
} else {
result.tableFullMeta_ = tableFullMetaBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.hasTabletMeta()) {
mergeTabletMeta(other.getTabletMeta());
}
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
if (other.hasTableMeta()) {
mergeTableMeta(other.getTableMeta());
}
if (!other.getTablePath().isEmpty()) {
tablePath_ = other.tablePath_;
onChanged();
}
if (other.tableType_ != 0) {
setTableTypeValue(other.getTableTypeValue());
}
if (!other.keyNames_.isEmpty()) {
if (keyNames_.isEmpty()) {
keyNames_ = other.keyNames_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeyNamesIsMutable();
keyNames_.addAll(other.keyNames_);
}
onChanged();
}
if (!other.hashRangeList_.isEmpty()) {
if (hashRangeList_.isEmpty()) {
hashRangeList_ = other.hashRangeList_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureHashRangeListIsMutable();
hashRangeList_.addAll(other.hashRangeList_);
}
onChanged();
}
if (!other.bucketsList_.isEmpty()) {
if (bucketsList_.isEmpty()) {
bucketsList_ = other.bucketsList_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureBucketsListIsMutable();
bucketsList_.addAll(other.bucketsList_);
}
onChanged();
}
if (!other.sortNames_.isEmpty()) {
if (sortNames_.isEmpty()) {
sortNames_ = other.sortNames_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureSortNamesIsMutable();
sortNames_.addAll(other.sortNames_);
}
onChanged();
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (partitionInfosBuilder_ == null) {
if (!other.partitionInfos_.isEmpty()) {
if (partitionInfos_.isEmpty()) {
partitionInfos_ = other.partitionInfos_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensurePartitionInfosIsMutable();
partitionInfos_.addAll(other.partitionInfos_);
}
onChanged();
}
} else {
if (!other.partitionInfos_.isEmpty()) {
if (partitionInfosBuilder_.isEmpty()) {
partitionInfosBuilder_.dispose();
partitionInfosBuilder_ = null;
partitionInfos_ = other.partitionInfos_;
bitField0_ = (bitField0_ & ~0x00000010);
partitionInfosBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPartitionInfosFieldBuilder() : null;
} else {
partitionInfosBuilder_.addAllMessages(other.partitionInfos_);
}
}
}
if (other.hasTableFullMeta()) {
mergeTableFullMeta(other.getTableFullMeta());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasTabletMeta()) {
if (!getTabletMeta().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 2;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 2;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 2;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 2;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 3;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 3;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 3;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 3;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private org.apache.kudu.KuduCommon.SchemaPB tabletMeta_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder> tabletMetaBuilder_;
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return Whether the tabletMeta field is set.
*/
public boolean hasTabletMeta() {
return tabletMetaBuilder_ != null || tabletMeta_ != null;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
* @return The tabletMeta.
*/
public org.apache.kudu.KuduCommon.SchemaPB getTabletMeta() {
if (tabletMetaBuilder_ == null) {
return tabletMeta_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : tabletMeta_;
} else {
return tabletMetaBuilder_.getMessage();
}
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public Builder setTabletMeta(org.apache.kudu.KuduCommon.SchemaPB value) {
if (tabletMetaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tabletMeta_ = value;
onChanged();
} else {
tabletMetaBuilder_.setMessage(value);
}
return this;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public Builder setTabletMeta(
org.apache.kudu.KuduCommon.SchemaPB.Builder builderForValue) {
if (tabletMetaBuilder_ == null) {
tabletMeta_ = builderForValue.build();
onChanged();
} else {
tabletMetaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public Builder mergeTabletMeta(org.apache.kudu.KuduCommon.SchemaPB value) {
if (tabletMetaBuilder_ == null) {
if (tabletMeta_ != null) {
tabletMeta_ =
org.apache.kudu.KuduCommon.SchemaPB.newBuilder(tabletMeta_).mergeFrom(value).buildPartial();
} else {
tabletMeta_ = value;
}
onChanged();
} else {
tabletMetaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public Builder clearTabletMeta() {
if (tabletMetaBuilder_ == null) {
tabletMeta_ = null;
onChanged();
} else {
tabletMeta_ = null;
tabletMetaBuilder_ = null;
}
return this;
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public org.apache.kudu.KuduCommon.SchemaPB.Builder getTabletMetaBuilder() {
onChanged();
return getTabletMetaFieldBuilder().getBuilder();
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getTabletMetaOrBuilder() {
if (tabletMetaBuilder_ != null) {
return tabletMetaBuilder_.getMessageOrBuilder();
} else {
return tabletMeta_ == null ?
org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : tabletMeta_;
}
}
/**
* .kudu.SchemaPB tablet_meta = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>
getTabletMetaFieldBuilder() {
if (tabletMetaBuilder_ == null) {
tabletMetaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>(
getTabletMeta(),
getParentForChildren(),
isClean());
tabletMeta_ = null;
}
return tabletMetaBuilder_;
}
private long workerId_ ;
/**
* int64 worker_id = 5;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 5;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 5;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
private cz.proto.MetadataEntity.Entity tableMeta_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder> tableMetaBuilder_;
/**
* .cz.proto.Entity table_meta = 6;
* @return Whether the tableMeta field is set.
*/
public boolean hasTableMeta() {
return tableMetaBuilder_ != null || tableMeta_ != null;
}
/**
* .cz.proto.Entity table_meta = 6;
* @return The tableMeta.
*/
public cz.proto.MetadataEntity.Entity getTableMeta() {
if (tableMetaBuilder_ == null) {
return tableMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
} else {
return tableMetaBuilder_.getMessage();
}
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public Builder setTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableMeta_ = value;
onChanged();
} else {
tableMetaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public Builder setTableMeta(
cz.proto.MetadataEntity.Entity.Builder builderForValue) {
if (tableMetaBuilder_ == null) {
tableMeta_ = builderForValue.build();
onChanged();
} else {
tableMetaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public Builder mergeTableMeta(cz.proto.MetadataEntity.Entity value) {
if (tableMetaBuilder_ == null) {
if (tableMeta_ != null) {
tableMeta_ =
cz.proto.MetadataEntity.Entity.newBuilder(tableMeta_).mergeFrom(value).buildPartial();
} else {
tableMeta_ = value;
}
onChanged();
} else {
tableMetaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public Builder clearTableMeta() {
if (tableMetaBuilder_ == null) {
tableMeta_ = null;
onChanged();
} else {
tableMeta_ = null;
tableMetaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public cz.proto.MetadataEntity.Entity.Builder getTableMetaBuilder() {
onChanged();
return getTableMetaFieldBuilder().getBuilder();
}
/**
* .cz.proto.Entity table_meta = 6;
*/
public cz.proto.MetadataEntity.EntityOrBuilder getTableMetaOrBuilder() {
if (tableMetaBuilder_ != null) {
return tableMetaBuilder_.getMessageOrBuilder();
} else {
return tableMeta_ == null ?
cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableMeta_;
}
}
/**
* .cz.proto.Entity table_meta = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>
getTableMetaFieldBuilder() {
if (tableMetaBuilder_ == null) {
tableMetaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>(
getTableMeta(),
getParentForChildren(),
isClean());
tableMeta_ = null;
}
return tableMetaBuilder_;
}
private java.lang.Object tablePath_ = "";
/**
* string table_path = 7;
* @return The tablePath.
*/
public java.lang.String getTablePath() {
java.lang.Object ref = tablePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tablePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_path = 7;
* @return The bytes for tablePath.
*/
public com.google.protobuf.ByteString
getTablePathBytes() {
java.lang.Object ref = tablePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tablePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_path = 7;
* @param value The tablePath to set.
* @return This builder for chaining.
*/
public Builder setTablePath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tablePath_ = value;
onChanged();
return this;
}
/**
* string table_path = 7;
* @return This builder for chaining.
*/
public Builder clearTablePath() {
tablePath_ = getDefaultInstance().getTablePath();
onChanged();
return this;
}
/**
* string table_path = 7;
* @param value The bytes for tablePath to set.
* @return This builder for chaining.
*/
public Builder setTablePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tablePath_ = value;
onChanged();
return this;
}
private int tableType_ = 0;
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @param value The enum numeric value on the wire for tableType to set.
* @return This builder for chaining.
*/
public Builder setTableTypeValue(int value) {
tableType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @param value The tableType to set.
* @return This builder for chaining.
*/
public Builder setTableType(cz.proto.ingestion.Ingestion.IGSTableType value) {
if (value == null) {
throw new NullPointerException();
}
tableType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return This builder for chaining.
*/
public Builder clearTableType() {
tableType_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureKeyNamesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList(keyNames_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string key_names = 9;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_.getUnmodifiableView();
}
/**
* repeated string key_names = 9;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 9;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 9;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
/**
* repeated string key_names = 9;
* @param index The index to set the value at.
* @param value The keyNames to set.
* @return This builder for chaining.
*/
public Builder setKeyNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string key_names = 9;
* @param value The keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
/**
* repeated string key_names = 9;
* @param values The keyNames to add.
* @return This builder for chaining.
*/
public Builder addAllKeyNames(
java.lang.Iterable values) {
ensureKeyNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keyNames_);
onChanged();
return this;
}
/**
* repeated string key_names = 9;
* @return This builder for chaining.
*/
public Builder clearKeyNames() {
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string key_names = 9;
* @param value The bytes of the keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList hashRangeList_ = emptyLongList();
private void ensureHashRangeListIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
hashRangeList_ = mutableCopy(hashRangeList_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated uint64 hash_range_list = 10;
* @return A list containing the hashRangeList.
*/
public java.util.List
getHashRangeListList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(hashRangeList_) : hashRangeList_;
}
/**
* repeated uint64 hash_range_list = 10;
* @return The count of hashRangeList.
*/
public int getHashRangeListCount() {
return hashRangeList_.size();
}
/**
* repeated uint64 hash_range_list = 10;
* @param index The index of the element to return.
* @return The hashRangeList at the given index.
*/
public long getHashRangeList(int index) {
return hashRangeList_.getLong(index);
}
/**
* repeated uint64 hash_range_list = 10;
* @param index The index to set the value at.
* @param value The hashRangeList to set.
* @return This builder for chaining.
*/
public Builder setHashRangeList(
int index, long value) {
ensureHashRangeListIsMutable();
hashRangeList_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated uint64 hash_range_list = 10;
* @param value The hashRangeList to add.
* @return This builder for chaining.
*/
public Builder addHashRangeList(long value) {
ensureHashRangeListIsMutable();
hashRangeList_.addLong(value);
onChanged();
return this;
}
/**
* repeated uint64 hash_range_list = 10;
* @param values The hashRangeList to add.
* @return This builder for chaining.
*/
public Builder addAllHashRangeList(
java.lang.Iterable extends java.lang.Long> values) {
ensureHashRangeListIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hashRangeList_);
onChanged();
return this;
}
/**
* repeated uint64 hash_range_list = 10;
* @return This builder for chaining.
*/
public Builder clearHashRangeList() {
hashRangeList_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList bucketsList_ = emptyIntList();
private void ensureBucketsListIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
bucketsList_ = mutableCopy(bucketsList_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated uint32 buckets_list = 11;
* @return A list containing the bucketsList.
*/
public java.util.List
getBucketsListList() {
return ((bitField0_ & 0x00000004) != 0) ?
java.util.Collections.unmodifiableList(bucketsList_) : bucketsList_;
}
/**
* repeated uint32 buckets_list = 11;
* @return The count of bucketsList.
*/
public int getBucketsListCount() {
return bucketsList_.size();
}
/**
* repeated uint32 buckets_list = 11;
* @param index The index of the element to return.
* @return The bucketsList at the given index.
*/
public int getBucketsList(int index) {
return bucketsList_.getInt(index);
}
/**
* repeated uint32 buckets_list = 11;
* @param index The index to set the value at.
* @param value The bucketsList to set.
* @return This builder for chaining.
*/
public Builder setBucketsList(
int index, int value) {
ensureBucketsListIsMutable();
bucketsList_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated uint32 buckets_list = 11;
* @param value The bucketsList to add.
* @return This builder for chaining.
*/
public Builder addBucketsList(int value) {
ensureBucketsListIsMutable();
bucketsList_.addInt(value);
onChanged();
return this;
}
/**
* repeated uint32 buckets_list = 11;
* @param values The bucketsList to add.
* @return This builder for chaining.
*/
public Builder addAllBucketsList(
java.lang.Iterable extends java.lang.Integer> values) {
ensureBucketsListIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bucketsList_);
onChanged();
return this;
}
/**
* repeated uint32 buckets_list = 11;
* @return This builder for chaining.
*/
public Builder clearBucketsList() {
bucketsList_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSortNamesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList(sortNames_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated string sort_names = 12;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_.getUnmodifiableView();
}
/**
* repeated string sort_names = 12;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 12;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 12;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
/**
* repeated string sort_names = 12;
* @param index The index to set the value at.
* @param value The sortNames to set.
* @return This builder for chaining.
*/
public Builder setSortNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sort_names = 12;
* @param value The sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
/**
* repeated string sort_names = 12;
* @param values The sortNames to add.
* @return This builder for chaining.
*/
public Builder addAllSortNames(
java.lang.Iterable values) {
ensureSortNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sortNames_);
onChanged();
return this;
}
/**
* repeated string sort_names = 12;
* @return This builder for chaining.
*/
public Builder clearSortNames() {
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* repeated string sort_names = 12;
* @param value The bytes of the sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 13;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 13;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 13;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 13;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 13;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 14;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 14;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 14;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.util.List partitionInfos_ =
java.util.Collections.emptyList();
private void ensurePartitionInfosIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
partitionInfos_ = new java.util.ArrayList(partitionInfos_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.PartitionColumnInfo, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder, cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder> partitionInfosBuilder_;
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public java.util.List getPartitionInfosList() {
if (partitionInfosBuilder_ == null) {
return java.util.Collections.unmodifiableList(partitionInfos_);
} else {
return partitionInfosBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public int getPartitionInfosCount() {
if (partitionInfosBuilder_ == null) {
return partitionInfos_.size();
} else {
return partitionInfosBuilder_.getCount();
}
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public cz.proto.ingestion.Ingestion.PartitionColumnInfo getPartitionInfos(int index) {
if (partitionInfosBuilder_ == null) {
return partitionInfos_.get(index);
} else {
return partitionInfosBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder setPartitionInfos(
int index, cz.proto.ingestion.Ingestion.PartitionColumnInfo value) {
if (partitionInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionInfosIsMutable();
partitionInfos_.set(index, value);
onChanged();
} else {
partitionInfosBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder setPartitionInfos(
int index, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder builderForValue) {
if (partitionInfosBuilder_ == null) {
ensurePartitionInfosIsMutable();
partitionInfos_.set(index, builderForValue.build());
onChanged();
} else {
partitionInfosBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder addPartitionInfos(cz.proto.ingestion.Ingestion.PartitionColumnInfo value) {
if (partitionInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionInfosIsMutable();
partitionInfos_.add(value);
onChanged();
} else {
partitionInfosBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder addPartitionInfos(
int index, cz.proto.ingestion.Ingestion.PartitionColumnInfo value) {
if (partitionInfosBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePartitionInfosIsMutable();
partitionInfos_.add(index, value);
onChanged();
} else {
partitionInfosBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder addPartitionInfos(
cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder builderForValue) {
if (partitionInfosBuilder_ == null) {
ensurePartitionInfosIsMutable();
partitionInfos_.add(builderForValue.build());
onChanged();
} else {
partitionInfosBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder addPartitionInfos(
int index, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder builderForValue) {
if (partitionInfosBuilder_ == null) {
ensurePartitionInfosIsMutable();
partitionInfos_.add(index, builderForValue.build());
onChanged();
} else {
partitionInfosBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder addAllPartitionInfos(
java.lang.Iterable extends cz.proto.ingestion.Ingestion.PartitionColumnInfo> values) {
if (partitionInfosBuilder_ == null) {
ensurePartitionInfosIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, partitionInfos_);
onChanged();
} else {
partitionInfosBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder clearPartitionInfos() {
if (partitionInfosBuilder_ == null) {
partitionInfos_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
partitionInfosBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public Builder removePartitionInfos(int index) {
if (partitionInfosBuilder_ == null) {
ensurePartitionInfosIsMutable();
partitionInfos_.remove(index);
onChanged();
} else {
partitionInfosBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder getPartitionInfosBuilder(
int index) {
return getPartitionInfosFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder getPartitionInfosOrBuilder(
int index) {
if (partitionInfosBuilder_ == null) {
return partitionInfos_.get(index); } else {
return partitionInfosBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public java.util.List extends cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder>
getPartitionInfosOrBuilderList() {
if (partitionInfosBuilder_ != null) {
return partitionInfosBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(partitionInfos_);
}
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder addPartitionInfosBuilder() {
return getPartitionInfosFieldBuilder().addBuilder(
cz.proto.ingestion.Ingestion.PartitionColumnInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder addPartitionInfosBuilder(
int index) {
return getPartitionInfosFieldBuilder().addBuilder(
index, cz.proto.ingestion.Ingestion.PartitionColumnInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.PartitionColumnInfo partition_infos = 15;
*/
public java.util.List
getPartitionInfosBuilderList() {
return getPartitionInfosFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.PartitionColumnInfo, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder, cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder>
getPartitionInfosFieldBuilder() {
if (partitionInfosBuilder_ == null) {
partitionInfosBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.PartitionColumnInfo, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder, cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder>(
partitionInfos_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
partitionInfos_ = null;
}
return partitionInfosBuilder_;
}
private cz.proto.MetadataEntity.Entity tableFullMeta_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder> tableFullMetaBuilder_;
/**
* .cz.proto.Entity table_full_meta = 16;
* @return Whether the tableFullMeta field is set.
*/
public boolean hasTableFullMeta() {
return tableFullMetaBuilder_ != null || tableFullMeta_ != null;
}
/**
* .cz.proto.Entity table_full_meta = 16;
* @return The tableFullMeta.
*/
public cz.proto.MetadataEntity.Entity getTableFullMeta() {
if (tableFullMetaBuilder_ == null) {
return tableFullMeta_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableFullMeta_;
} else {
return tableFullMetaBuilder_.getMessage();
}
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public Builder setTableFullMeta(cz.proto.MetadataEntity.Entity value) {
if (tableFullMetaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableFullMeta_ = value;
onChanged();
} else {
tableFullMetaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public Builder setTableFullMeta(
cz.proto.MetadataEntity.Entity.Builder builderForValue) {
if (tableFullMetaBuilder_ == null) {
tableFullMeta_ = builderForValue.build();
onChanged();
} else {
tableFullMetaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public Builder mergeTableFullMeta(cz.proto.MetadataEntity.Entity value) {
if (tableFullMetaBuilder_ == null) {
if (tableFullMeta_ != null) {
tableFullMeta_ =
cz.proto.MetadataEntity.Entity.newBuilder(tableFullMeta_).mergeFrom(value).buildPartial();
} else {
tableFullMeta_ = value;
}
onChanged();
} else {
tableFullMetaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public Builder clearTableFullMeta() {
if (tableFullMetaBuilder_ == null) {
tableFullMeta_ = null;
onChanged();
} else {
tableFullMeta_ = null;
tableFullMetaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public cz.proto.MetadataEntity.Entity.Builder getTableFullMetaBuilder() {
onChanged();
return getTableFullMetaFieldBuilder().getBuilder();
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
public cz.proto.MetadataEntity.EntityOrBuilder getTableFullMetaOrBuilder() {
if (tableFullMetaBuilder_ != null) {
return tableFullMetaBuilder_.getMessageOrBuilder();
} else {
return tableFullMeta_ == null ?
cz.proto.MetadataEntity.Entity.getDefaultInstance() : tableFullMeta_;
}
}
/**
* .cz.proto.Entity table_full_meta = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>
getTableFullMetaFieldBuilder() {
if (tableFullMetaBuilder_ == null) {
tableFullMetaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>(
getTableFullMeta(),
getParentForChildren(),
isClean());
tableFullMeta_ = null;
}
return tableFullMetaBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.WorkerCreateTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.WorkerCreateTabletRequest)
private static final cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest();
}
public static cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WorkerCreateTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WorkerCreateTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerCreateTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PartitionColumnInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.PartitionColumnInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string virtual_partition_column_name = 1;
* @return The virtualPartitionColumnName.
*/
java.lang.String getVirtualPartitionColumnName();
/**
* string virtual_partition_column_name = 1;
* @return The bytes for virtualPartitionColumnName.
*/
com.google.protobuf.ByteString
getVirtualPartitionColumnNameBytes();
/**
* uint32 virtual_partition_column_id = 2;
* @return The virtualPartitionColumnId.
*/
int getVirtualPartitionColumnId();
/**
* string source_partition_column_name = 3;
* @return The sourcePartitionColumnName.
*/
java.lang.String getSourcePartitionColumnName();
/**
* string source_partition_column_name = 3;
* @return The bytes for sourcePartitionColumnName.
*/
com.google.protobuf.ByteString
getSourcePartitionColumnNameBytes();
/**
* uint32 source_partition_column_id = 4;
* @return The sourcePartitionColumnId.
*/
int getSourcePartitionColumnId();
}
/**
* Protobuf type {@code cz.proto.ingestion.PartitionColumnInfo}
*/
public static final class PartitionColumnInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.PartitionColumnInfo)
PartitionColumnInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use PartitionColumnInfo.newBuilder() to construct.
private PartitionColumnInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PartitionColumnInfo() {
virtualPartitionColumnName_ = "";
sourcePartitionColumnName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PartitionColumnInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PartitionColumnInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
virtualPartitionColumnName_ = s;
break;
}
case 16: {
virtualPartitionColumnId_ = input.readUInt32();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
sourcePartitionColumnName_ = s;
break;
}
case 32: {
sourcePartitionColumnId_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_PartitionColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_PartitionColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.PartitionColumnInfo.class, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder.class);
}
public static final int VIRTUAL_PARTITION_COLUMN_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object virtualPartitionColumnName_;
/**
* string virtual_partition_column_name = 1;
* @return The virtualPartitionColumnName.
*/
@java.lang.Override
public java.lang.String getVirtualPartitionColumnName() {
java.lang.Object ref = virtualPartitionColumnName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualPartitionColumnName_ = s;
return s;
}
}
/**
* string virtual_partition_column_name = 1;
* @return The bytes for virtualPartitionColumnName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVirtualPartitionColumnNameBytes() {
java.lang.Object ref = virtualPartitionColumnName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualPartitionColumnName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VIRTUAL_PARTITION_COLUMN_ID_FIELD_NUMBER = 2;
private int virtualPartitionColumnId_;
/**
* uint32 virtual_partition_column_id = 2;
* @return The virtualPartitionColumnId.
*/
@java.lang.Override
public int getVirtualPartitionColumnId() {
return virtualPartitionColumnId_;
}
public static final int SOURCE_PARTITION_COLUMN_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object sourcePartitionColumnName_;
/**
* string source_partition_column_name = 3;
* @return The sourcePartitionColumnName.
*/
@java.lang.Override
public java.lang.String getSourcePartitionColumnName() {
java.lang.Object ref = sourcePartitionColumnName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourcePartitionColumnName_ = s;
return s;
}
}
/**
* string source_partition_column_name = 3;
* @return The bytes for sourcePartitionColumnName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourcePartitionColumnNameBytes() {
java.lang.Object ref = sourcePartitionColumnName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourcePartitionColumnName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_PARTITION_COLUMN_ID_FIELD_NUMBER = 4;
private int sourcePartitionColumnId_;
/**
* uint32 source_partition_column_id = 4;
* @return The sourcePartitionColumnId.
*/
@java.lang.Override
public int getSourcePartitionColumnId() {
return sourcePartitionColumnId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualPartitionColumnName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, virtualPartitionColumnName_);
}
if (virtualPartitionColumnId_ != 0) {
output.writeUInt32(2, virtualPartitionColumnId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sourcePartitionColumnName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, sourcePartitionColumnName_);
}
if (sourcePartitionColumnId_ != 0) {
output.writeUInt32(4, sourcePartitionColumnId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(virtualPartitionColumnName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, virtualPartitionColumnName_);
}
if (virtualPartitionColumnId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, virtualPartitionColumnId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sourcePartitionColumnName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, sourcePartitionColumnName_);
}
if (sourcePartitionColumnId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, sourcePartitionColumnId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.PartitionColumnInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.PartitionColumnInfo other = (cz.proto.ingestion.Ingestion.PartitionColumnInfo) obj;
if (!getVirtualPartitionColumnName()
.equals(other.getVirtualPartitionColumnName())) return false;
if (getVirtualPartitionColumnId()
!= other.getVirtualPartitionColumnId()) return false;
if (!getSourcePartitionColumnName()
.equals(other.getSourcePartitionColumnName())) return false;
if (getSourcePartitionColumnId()
!= other.getSourcePartitionColumnId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VIRTUAL_PARTITION_COLUMN_NAME_FIELD_NUMBER;
hash = (53 * hash) + getVirtualPartitionColumnName().hashCode();
hash = (37 * hash) + VIRTUAL_PARTITION_COLUMN_ID_FIELD_NUMBER;
hash = (53 * hash) + getVirtualPartitionColumnId();
hash = (37 * hash) + SOURCE_PARTITION_COLUMN_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSourcePartitionColumnName().hashCode();
hash = (37 * hash) + SOURCE_PARTITION_COLUMN_ID_FIELD_NUMBER;
hash = (53 * hash) + getSourcePartitionColumnId();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.PartitionColumnInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.PartitionColumnInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.PartitionColumnInfo)
cz.proto.ingestion.Ingestion.PartitionColumnInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_PartitionColumnInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_PartitionColumnInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.PartitionColumnInfo.class, cz.proto.ingestion.Ingestion.PartitionColumnInfo.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.PartitionColumnInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
virtualPartitionColumnName_ = "";
virtualPartitionColumnId_ = 0;
sourcePartitionColumnName_ = "";
sourcePartitionColumnId_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_PartitionColumnInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfo getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.PartitionColumnInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfo build() {
cz.proto.ingestion.Ingestion.PartitionColumnInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfo buildPartial() {
cz.proto.ingestion.Ingestion.PartitionColumnInfo result = new cz.proto.ingestion.Ingestion.PartitionColumnInfo(this);
result.virtualPartitionColumnName_ = virtualPartitionColumnName_;
result.virtualPartitionColumnId_ = virtualPartitionColumnId_;
result.sourcePartitionColumnName_ = sourcePartitionColumnName_;
result.sourcePartitionColumnId_ = sourcePartitionColumnId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.PartitionColumnInfo) {
return mergeFrom((cz.proto.ingestion.Ingestion.PartitionColumnInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.PartitionColumnInfo other) {
if (other == cz.proto.ingestion.Ingestion.PartitionColumnInfo.getDefaultInstance()) return this;
if (!other.getVirtualPartitionColumnName().isEmpty()) {
virtualPartitionColumnName_ = other.virtualPartitionColumnName_;
onChanged();
}
if (other.getVirtualPartitionColumnId() != 0) {
setVirtualPartitionColumnId(other.getVirtualPartitionColumnId());
}
if (!other.getSourcePartitionColumnName().isEmpty()) {
sourcePartitionColumnName_ = other.sourcePartitionColumnName_;
onChanged();
}
if (other.getSourcePartitionColumnId() != 0) {
setSourcePartitionColumnId(other.getSourcePartitionColumnId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.PartitionColumnInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.PartitionColumnInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object virtualPartitionColumnName_ = "";
/**
* string virtual_partition_column_name = 1;
* @return The virtualPartitionColumnName.
*/
public java.lang.String getVirtualPartitionColumnName() {
java.lang.Object ref = virtualPartitionColumnName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
virtualPartitionColumnName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string virtual_partition_column_name = 1;
* @return The bytes for virtualPartitionColumnName.
*/
public com.google.protobuf.ByteString
getVirtualPartitionColumnNameBytes() {
java.lang.Object ref = virtualPartitionColumnName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
virtualPartitionColumnName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string virtual_partition_column_name = 1;
* @param value The virtualPartitionColumnName to set.
* @return This builder for chaining.
*/
public Builder setVirtualPartitionColumnName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
virtualPartitionColumnName_ = value;
onChanged();
return this;
}
/**
* string virtual_partition_column_name = 1;
* @return This builder for chaining.
*/
public Builder clearVirtualPartitionColumnName() {
virtualPartitionColumnName_ = getDefaultInstance().getVirtualPartitionColumnName();
onChanged();
return this;
}
/**
* string virtual_partition_column_name = 1;
* @param value The bytes for virtualPartitionColumnName to set.
* @return This builder for chaining.
*/
public Builder setVirtualPartitionColumnNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
virtualPartitionColumnName_ = value;
onChanged();
return this;
}
private int virtualPartitionColumnId_ ;
/**
* uint32 virtual_partition_column_id = 2;
* @return The virtualPartitionColumnId.
*/
@java.lang.Override
public int getVirtualPartitionColumnId() {
return virtualPartitionColumnId_;
}
/**
* uint32 virtual_partition_column_id = 2;
* @param value The virtualPartitionColumnId to set.
* @return This builder for chaining.
*/
public Builder setVirtualPartitionColumnId(int value) {
virtualPartitionColumnId_ = value;
onChanged();
return this;
}
/**
* uint32 virtual_partition_column_id = 2;
* @return This builder for chaining.
*/
public Builder clearVirtualPartitionColumnId() {
virtualPartitionColumnId_ = 0;
onChanged();
return this;
}
private java.lang.Object sourcePartitionColumnName_ = "";
/**
* string source_partition_column_name = 3;
* @return The sourcePartitionColumnName.
*/
public java.lang.String getSourcePartitionColumnName() {
java.lang.Object ref = sourcePartitionColumnName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourcePartitionColumnName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string source_partition_column_name = 3;
* @return The bytes for sourcePartitionColumnName.
*/
public com.google.protobuf.ByteString
getSourcePartitionColumnNameBytes() {
java.lang.Object ref = sourcePartitionColumnName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourcePartitionColumnName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string source_partition_column_name = 3;
* @param value The sourcePartitionColumnName to set.
* @return This builder for chaining.
*/
public Builder setSourcePartitionColumnName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourcePartitionColumnName_ = value;
onChanged();
return this;
}
/**
* string source_partition_column_name = 3;
* @return This builder for chaining.
*/
public Builder clearSourcePartitionColumnName() {
sourcePartitionColumnName_ = getDefaultInstance().getSourcePartitionColumnName();
onChanged();
return this;
}
/**
* string source_partition_column_name = 3;
* @param value The bytes for sourcePartitionColumnName to set.
* @return This builder for chaining.
*/
public Builder setSourcePartitionColumnNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourcePartitionColumnName_ = value;
onChanged();
return this;
}
private int sourcePartitionColumnId_ ;
/**
* uint32 source_partition_column_id = 4;
* @return The sourcePartitionColumnId.
*/
@java.lang.Override
public int getSourcePartitionColumnId() {
return sourcePartitionColumnId_;
}
/**
* uint32 source_partition_column_id = 4;
* @param value The sourcePartitionColumnId to set.
* @return This builder for chaining.
*/
public Builder setSourcePartitionColumnId(int value) {
sourcePartitionColumnId_ = value;
onChanged();
return this;
}
/**
* uint32 source_partition_column_id = 4;
* @return This builder for chaining.
*/
public Builder clearSourcePartitionColumnId() {
sourcePartitionColumnId_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.PartitionColumnInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.PartitionColumnInfo)
private static final cz.proto.ingestion.Ingestion.PartitionColumnInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.PartitionColumnInfo();
}
public static cz.proto.ingestion.Ingestion.PartitionColumnInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PartitionColumnInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PartitionColumnInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.PartitionColumnInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateTabletResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.CreateTabletResponse)
com.google.protobuf.MessageOrBuilder {
/**
* int64 created_time = 1;
* @return The createdTime.
*/
long getCreatedTime();
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.CreateTabletResponse}
*/
public static final class CreateTabletResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.CreateTabletResponse)
CreateTabletResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateTabletResponse.newBuilder() to construct.
private CreateTabletResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateTabletResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateTabletResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CreateTabletResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
createdTime_ = input.readInt64();
break;
}
case 18: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CreateTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CreateTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CreateTabletResponse.class, cz.proto.ingestion.Ingestion.CreateTabletResponse.Builder.class);
}
public static final int CREATED_TIME_FIELD_NUMBER = 1;
private long createdTime_;
/**
* int64 created_time = 1;
* @return The createdTime.
*/
@java.lang.Override
public long getCreatedTime() {
return createdTime_;
}
public static final int STATUS_FIELD_NUMBER = 2;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (createdTime_ != 0L) {
output.writeInt64(1, createdTime_);
}
if (status_ != null) {
output.writeMessage(2, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (createdTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, createdTime_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.CreateTabletResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.CreateTabletResponse other = (cz.proto.ingestion.Ingestion.CreateTabletResponse) obj;
if (getCreatedTime()
!= other.getCreatedTime()) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CREATED_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreatedTime());
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.CreateTabletResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.CreateTabletResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.CreateTabletResponse)
cz.proto.ingestion.Ingestion.CreateTabletResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CreateTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CreateTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CreateTabletResponse.class, cz.proto.ingestion.Ingestion.CreateTabletResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.CreateTabletResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
createdTime_ = 0L;
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CreateTabletResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CreateTabletResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.CreateTabletResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CreateTabletResponse build() {
cz.proto.ingestion.Ingestion.CreateTabletResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CreateTabletResponse buildPartial() {
cz.proto.ingestion.Ingestion.CreateTabletResponse result = new cz.proto.ingestion.Ingestion.CreateTabletResponse(this);
result.createdTime_ = createdTime_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.CreateTabletResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.CreateTabletResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.CreateTabletResponse other) {
if (other == cz.proto.ingestion.Ingestion.CreateTabletResponse.getDefaultInstance()) return this;
if (other.getCreatedTime() != 0L) {
setCreatedTime(other.getCreatedTime());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.CreateTabletResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.CreateTabletResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long createdTime_ ;
/**
* int64 created_time = 1;
* @return The createdTime.
*/
@java.lang.Override
public long getCreatedTime() {
return createdTime_;
}
/**
* int64 created_time = 1;
* @param value The createdTime to set.
* @return This builder for chaining.
*/
public Builder setCreatedTime(long value) {
createdTime_ = value;
onChanged();
return this;
}
/**
* int64 created_time = 1;
* @return This builder for chaining.
*/
public Builder clearCreatedTime() {
createdTime_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.CreateTabletResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.CreateTabletResponse)
private static final cz.proto.ingestion.Ingestion.CreateTabletResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.CreateTabletResponse();
}
public static cz.proto.ingestion.Ingestion.CreateTabletResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateTabletResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateTabletResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CreateTabletResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommitTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.CommitTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string schema_name = 3;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 4;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* int64 tableId = 5;
* @return The tableId.
*/
long getTableId();
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 7;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.CommitTabletRequest}
*/
public static final class CommitTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.CommitTabletRequest)
CommitTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommitTabletRequest.newBuilder() to construct.
private CommitTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommitTabletRequest() {
workspace_ = "";
schemaName_ = "";
tableName_ = "";
tabletId_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommitTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommitTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 40: {
tableId_ = input.readInt64();
break;
}
case 48: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
tabletId_.addLong(input.readInt64());
break;
}
case 50: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 58: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CommitTabletRequest.class, cz.proto.ingestion.Ingestion.CommitTabletRequest.Builder.class);
}
public static final int INSTANCE_ID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 3;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object tableName_;
/**
* string table_name = 4;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLEID_FIELD_NUMBER = 5;
private long tableId_;
/**
* int64 tableId = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
public static final int TABLET_ID_FIELD_NUMBER = 6;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
public static final int ACCOUNT_FIELD_NUMBER = 7;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tableName_);
}
if (tableId_ != 0L) {
output.writeInt64(5, tableId_);
}
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
if (account_ != null) {
output.writeMessage(7, getAccount());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tableName_);
}
if (tableId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, tableId_);
}
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getAccount());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.CommitTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.CommitTabletRequest other = (cz.proto.ingestion.Ingestion.CommitTabletRequest) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (getTableId()
!= other.getTableId()) return false;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (37 * hash) + TABLEID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTableId());
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.CommitTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.CommitTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.CommitTabletRequest)
cz.proto.ingestion.Ingestion.CommitTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CommitTabletRequest.class, cz.proto.ingestion.Ingestion.CommitTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.CommitTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
workspace_ = "";
schemaName_ = "";
tableName_ = "";
tableId_ = 0L;
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.CommitTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletRequest build() {
cz.proto.ingestion.Ingestion.CommitTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.CommitTabletRequest result = new cz.proto.ingestion.Ingestion.CommitTabletRequest(this);
int from_bitField0_ = bitField0_;
result.instanceId_ = instanceId_;
result.workspace_ = workspace_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
result.tableId_ = tableId_;
if (((bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tabletId_ = tabletId_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.CommitTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.CommitTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.CommitTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.CommitTabletRequest.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.getTableId() != 0L) {
setTableId(other.getTableId());
}
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.CommitTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.CommitTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long instanceId_ ;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 3;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 3;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 3;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 3;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 4;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 4;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 4;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 4;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private long tableId_ ;
/**
* int64 tableId = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
/**
* int64 tableId = 5;
* @param value The tableId to set.
* @return This builder for chaining.
*/
public Builder setTableId(long value) {
tableId_ = value;
onChanged();
return this;
}
/**
* int64 tableId = 5;
* @return This builder for chaining.
*/
public Builder clearTableId() {
tableId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList tabletId_ = emptyLongList();
private void ensureTabletIdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tabletId_ = mutableCopy(tabletId_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
public java.util.List
getTabletIdList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(tabletId_) : tabletId_;
}
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
/**
* repeated int64 tablet_id = 6;
* @param index The index to set the value at.
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(
int index, long value) {
ensureTabletIdIsMutable();
tabletId_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @param value The tabletId to add.
* @return This builder for chaining.
*/
public Builder addTabletId(long value) {
ensureTabletIdIsMutable();
tabletId_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @param values The tabletId to add.
* @return This builder for chaining.
*/
public Builder addAllTabletId(
java.lang.Iterable extends java.lang.Long> values) {
ensureTabletIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tabletId_);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.CommitTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.CommitTabletRequest)
private static final cz.proto.ingestion.Ingestion.CommitTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.CommitTabletRequest();
}
public static cz.proto.ingestion.Ingestion.CommitTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommitTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommitTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommitTabletResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.CommitTabletResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.CommitTabletResponse}
*/
public static final class CommitTabletResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.CommitTabletResponse)
CommitTabletResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommitTabletResponse.newBuilder() to construct.
private CommitTabletResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommitTabletResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommitTabletResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommitTabletResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CommitTabletResponse.class, cz.proto.ingestion.Ingestion.CommitTabletResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.CommitTabletResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.CommitTabletResponse other = (cz.proto.ingestion.Ingestion.CommitTabletResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.CommitTabletResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.CommitTabletResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.CommitTabletResponse)
cz.proto.ingestion.Ingestion.CommitTabletResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CommitTabletResponse.class, cz.proto.ingestion.Ingestion.CommitTabletResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.CommitTabletResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CommitTabletResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.CommitTabletResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletResponse build() {
cz.proto.ingestion.Ingestion.CommitTabletResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletResponse buildPartial() {
cz.proto.ingestion.Ingestion.CommitTabletResponse result = new cz.proto.ingestion.Ingestion.CommitTabletResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.CommitTabletResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.CommitTabletResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.CommitTabletResponse other) {
if (other == cz.proto.ingestion.Ingestion.CommitTabletResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.CommitTabletResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.CommitTabletResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.CommitTabletResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.CommitTabletResponse)
private static final cz.proto.ingestion.Ingestion.CommitTabletResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.CommitTabletResponse();
}
public static cz.proto.ingestion.Ingestion.CommitTabletResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommitTabletResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommitTabletResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CommitTabletResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DropTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DropTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string schema_name = 3;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 4;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* int64 tableId = 5;
* @return The tableId.
*/
long getTableId();
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 7;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
/**
* string request_id = 8;
* @return The requestId.
*/
java.lang.String getRequestId();
/**
* string request_id = 8;
* @return The bytes for requestId.
*/
com.google.protobuf.ByteString
getRequestIdBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.DropTabletRequest}
*/
public static final class DropTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.DropTabletRequest)
DropTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use DropTabletRequest.newBuilder() to construct.
private DropTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DropTabletRequest() {
workspace_ = "";
schemaName_ = "";
tableName_ = "";
tabletId_ = emptyLongList();
requestId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DropTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DropTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 40: {
tableId_ = input.readInt64();
break;
}
case 48: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
tabletId_.addLong(input.readInt64());
break;
}
case 50: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 58: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
requestId_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DropTabletRequest.class, cz.proto.ingestion.Ingestion.DropTabletRequest.Builder.class);
}
public static final int INSTANCE_ID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 3;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object tableName_;
/**
* string table_name = 4;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLEID_FIELD_NUMBER = 5;
private long tableId_;
/**
* int64 tableId = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
public static final int TABLET_ID_FIELD_NUMBER = 6;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
public static final int ACCOUNT_FIELD_NUMBER = 7;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int REQUEST_ID_FIELD_NUMBER = 8;
private volatile java.lang.Object requestId_;
/**
* string request_id = 8;
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
* string request_id = 8;
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tableName_);
}
if (tableId_ != 0L) {
output.writeInt64(5, tableId_);
}
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
if (account_ != null) {
output.writeMessage(7, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, requestId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tableName_);
}
if (tableId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, tableId_);
}
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, requestId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.DropTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.DropTabletRequest other = (cz.proto.ingestion.Ingestion.DropTabletRequest) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (getTableId()
!= other.getTableId()) return false;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!getRequestId()
.equals(other.getRequestId())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (37 * hash) + TABLEID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTableId());
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.DropTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.DropTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.DropTabletRequest)
cz.proto.ingestion.Ingestion.DropTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DropTabletRequest.class, cz.proto.ingestion.Ingestion.DropTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.DropTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
workspace_ = "";
schemaName_ = "";
tableName_ = "";
tableId_ = 0L;
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
requestId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.DropTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletRequest build() {
cz.proto.ingestion.Ingestion.DropTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.DropTabletRequest result = new cz.proto.ingestion.Ingestion.DropTabletRequest(this);
int from_bitField0_ = bitField0_;
result.instanceId_ = instanceId_;
result.workspace_ = workspace_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
result.tableId_ = tableId_;
if (((bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tabletId_ = tabletId_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
result.requestId_ = requestId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.DropTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.DropTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.DropTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.DropTabletRequest.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.getTableId() != 0L) {
setTableId(other.getTableId());
}
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (!other.getRequestId().isEmpty()) {
requestId_ = other.requestId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.DropTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.DropTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long instanceId_ ;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 3;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 3;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 3;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 3;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 4;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 4;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 4;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 4;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private long tableId_ ;
/**
* int64 tableId = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
/**
* int64 tableId = 5;
* @param value The tableId to set.
* @return This builder for chaining.
*/
public Builder setTableId(long value) {
tableId_ = value;
onChanged();
return this;
}
/**
* int64 tableId = 5;
* @return This builder for chaining.
*/
public Builder clearTableId() {
tableId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList tabletId_ = emptyLongList();
private void ensureTabletIdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tabletId_ = mutableCopy(tabletId_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 tablet_id = 6;
* @return A list containing the tabletId.
*/
public java.util.List
getTabletIdList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(tabletId_) : tabletId_;
}
/**
* repeated int64 tablet_id = 6;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 6;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
/**
* repeated int64 tablet_id = 6;
* @param index The index to set the value at.
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(
int index, long value) {
ensureTabletIdIsMutable();
tabletId_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @param value The tabletId to add.
* @return This builder for chaining.
*/
public Builder addTabletId(long value) {
ensureTabletIdIsMutable();
tabletId_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @param values The tabletId to add.
* @return This builder for chaining.
*/
public Builder addAllTabletId(
java.lang.Iterable extends java.lang.Long> values) {
ensureTabletIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tabletId_);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 6;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 7;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 7;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private java.lang.Object requestId_ = "";
/**
* string request_id = 8;
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string request_id = 8;
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string request_id = 8;
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
onChanged();
return this;
}
/**
* string request_id = 8;
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
onChanged();
return this;
}
/**
* string request_id = 8;
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.DropTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.DropTabletRequest)
private static final cz.proto.ingestion.Ingestion.DropTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.DropTabletRequest();
}
public static cz.proto.ingestion.Ingestion.DropTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DropTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DropTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DropTabletResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DropTabletResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.DropTabletResponse}
*/
public static final class DropTabletResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.DropTabletResponse)
DropTabletResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use DropTabletResponse.newBuilder() to construct.
private DropTabletResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DropTabletResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DropTabletResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DropTabletResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DropTabletResponse.class, cz.proto.ingestion.Ingestion.DropTabletResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.DropTabletResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.DropTabletResponse other = (cz.proto.ingestion.Ingestion.DropTabletResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.DropTabletResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.DropTabletResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.DropTabletResponse)
cz.proto.ingestion.Ingestion.DropTabletResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DropTabletResponse.class, cz.proto.ingestion.Ingestion.DropTabletResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.DropTabletResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DropTabletResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.DropTabletResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletResponse build() {
cz.proto.ingestion.Ingestion.DropTabletResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletResponse buildPartial() {
cz.proto.ingestion.Ingestion.DropTabletResponse result = new cz.proto.ingestion.Ingestion.DropTabletResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.DropTabletResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.DropTabletResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.DropTabletResponse other) {
if (other == cz.proto.ingestion.Ingestion.DropTabletResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.DropTabletResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.DropTabletResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.DropTabletResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.DropTabletResponse)
private static final cz.proto.ingestion.Ingestion.DropTabletResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.DropTabletResponse();
}
public static cz.proto.ingestion.Ingestion.DropTabletResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DropTabletResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DropTabletResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DropTabletResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RestartTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.RestartTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string message = 2;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 2;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.RestartTabletRequest}
*/
public static final class RestartTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.RestartTabletRequest)
RestartTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use RestartTabletRequest.newBuilder() to construct.
private RestartTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RestartTabletRequest() {
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RestartTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RestartTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.RestartTabletRequest.class, cz.proto.ingestion.Ingestion.RestartTabletRequest.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
* string message = 2;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.RestartTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.RestartTabletRequest other = (cz.proto.ingestion.Ingestion.RestartTabletRequest) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.RestartTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.RestartTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.RestartTabletRequest)
cz.proto.ingestion.Ingestion.RestartTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.RestartTabletRequest.class, cz.proto.ingestion.Ingestion.RestartTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.RestartTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
message_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.RestartTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletRequest build() {
cz.proto.ingestion.Ingestion.RestartTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.RestartTabletRequest result = new cz.proto.ingestion.Ingestion.RestartTabletRequest(this);
result.tabletId_ = tabletId_;
result.message_ = message_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.RestartTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.RestartTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.RestartTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.RestartTabletRequest.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.RestartTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.RestartTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 2;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 2;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 2;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 2;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.RestartTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.RestartTabletRequest)
private static final cz.proto.ingestion.Ingestion.RestartTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.RestartTabletRequest();
}
public static cz.proto.ingestion.Ingestion.RestartTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RestartTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RestartTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RestartTabletResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.RestartTabletResponse)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string message = 2;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 2;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.RestartTabletResponse}
*/
public static final class RestartTabletResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.RestartTabletResponse)
RestartTabletResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use RestartTabletResponse.newBuilder() to construct.
private RestartTabletResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RestartTabletResponse() {
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RestartTabletResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RestartTabletResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 26: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.RestartTabletResponse.class, cz.proto.ingestion.Ingestion.RestartTabletResponse.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
* string message = 2;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 3;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
if (status_ != null) {
output.writeMessage(3, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.RestartTabletResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.RestartTabletResponse other = (cz.proto.ingestion.Ingestion.RestartTabletResponse) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.RestartTabletResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.RestartTabletResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.RestartTabletResponse)
cz.proto.ingestion.Ingestion.RestartTabletResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.RestartTabletResponse.class, cz.proto.ingestion.Ingestion.RestartTabletResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.RestartTabletResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
message_ = "";
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_RestartTabletResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.RestartTabletResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletResponse build() {
cz.proto.ingestion.Ingestion.RestartTabletResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletResponse buildPartial() {
cz.proto.ingestion.Ingestion.RestartTabletResponse result = new cz.proto.ingestion.Ingestion.RestartTabletResponse(this);
result.tabletId_ = tabletId_;
result.message_ = message_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.RestartTabletResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.RestartTabletResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.RestartTabletResponse other) {
if (other == cz.proto.ingestion.Ingestion.RestartTabletResponse.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.RestartTabletResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.RestartTabletResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 2;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 2;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 2;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 2;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.RestartTabletResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.RestartTabletResponse)
private static final cz.proto.ingestion.Ingestion.RestartTabletResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.RestartTabletResponse();
}
public static cz.proto.ingestion.Ingestion.RestartTabletResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RestartTabletResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RestartTabletResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.RestartTabletResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelTabletRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DelTabletRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string message = 2;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 2;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.DelTabletRequest}
*/
public static final class DelTabletRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.DelTabletRequest)
DelTabletRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use DelTabletRequest.newBuilder() to construct.
private DelTabletRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DelTabletRequest() {
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DelTabletRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DelTabletRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DelTabletRequest.class, cz.proto.ingestion.Ingestion.DelTabletRequest.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
* string message = 2;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.DelTabletRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.DelTabletRequest other = (cz.proto.ingestion.Ingestion.DelTabletRequest) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.DelTabletRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.DelTabletRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.DelTabletRequest)
cz.proto.ingestion.Ingestion.DelTabletRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DelTabletRequest.class, cz.proto.ingestion.Ingestion.DelTabletRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.DelTabletRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
message_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.DelTabletRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletRequest build() {
cz.proto.ingestion.Ingestion.DelTabletRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletRequest buildPartial() {
cz.proto.ingestion.Ingestion.DelTabletRequest result = new cz.proto.ingestion.Ingestion.DelTabletRequest(this);
result.tabletId_ = tabletId_;
result.message_ = message_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.DelTabletRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.DelTabletRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.DelTabletRequest other) {
if (other == cz.proto.ingestion.Ingestion.DelTabletRequest.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.DelTabletRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.DelTabletRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 2;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 2;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 2;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 2;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.DelTabletRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.DelTabletRequest)
private static final cz.proto.ingestion.Ingestion.DelTabletRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.DelTabletRequest();
}
public static cz.proto.ingestion.Ingestion.DelTabletRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DelTabletRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DelTabletRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DelTabletResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DelTabletResponse)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string message = 2;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 2;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.DelTabletResponse}
*/
public static final class DelTabletResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.DelTabletResponse)
DelTabletResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use DelTabletResponse.newBuilder() to construct.
private DelTabletResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DelTabletResponse() {
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DelTabletResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DelTabletResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 26: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DelTabletResponse.class, cz.proto.ingestion.Ingestion.DelTabletResponse.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
* string message = 2;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 3;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
if (status_ != null) {
output.writeMessage(3, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.DelTabletResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.DelTabletResponse other = (cz.proto.ingestion.Ingestion.DelTabletResponse) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.DelTabletResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.DelTabletResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.DelTabletResponse)
cz.proto.ingestion.Ingestion.DelTabletResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DelTabletResponse.class, cz.proto.ingestion.Ingestion.DelTabletResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.DelTabletResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
message_ = "";
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DelTabletResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.DelTabletResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletResponse build() {
cz.proto.ingestion.Ingestion.DelTabletResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletResponse buildPartial() {
cz.proto.ingestion.Ingestion.DelTabletResponse result = new cz.proto.ingestion.Ingestion.DelTabletResponse(this);
result.tabletId_ = tabletId_;
result.message_ = message_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.DelTabletResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.DelTabletResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.DelTabletResponse other) {
if (other == cz.proto.ingestion.Ingestion.DelTabletResponse.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.DelTabletResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.DelTabletResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 2;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 2;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 2;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 2;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.DelTabletResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.DelTabletResponse)
private static final cz.proto.ingestion.Ingestion.DelTabletResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.DelTabletResponse();
}
public static cz.proto.ingestion.Ingestion.DelTabletResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DelTabletResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DelTabletResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DelTabletResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BroadcastRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.BroadcastRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* int64 table_id = 2;
* @return The tableId.
*/
long getTableId();
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
/**
* bool clear_cache = 4;
* @return The clearCache.
*/
boolean getClearCache();
}
/**
* Protobuf type {@code cz.proto.ingestion.BroadcastRequest}
*/
public static final class BroadcastRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.BroadcastRequest)
BroadcastRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use BroadcastRequest.newBuilder() to construct.
private BroadcastRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BroadcastRequest() {
tabletId_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BroadcastRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BroadcastRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 16: {
tableId_ = input.readInt64();
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
tabletId_.addLong(input.readInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 32: {
clearCache_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.BroadcastRequest.class, cz.proto.ingestion.Ingestion.BroadcastRequest.Builder.class);
}
public static final int INSTANCE_ID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int TABLE_ID_FIELD_NUMBER = 2;
private long tableId_;
/**
* int64 table_id = 2;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
public static final int TABLET_ID_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
public static final int CLEAR_CACHE_FIELD_NUMBER = 4;
private boolean clearCache_;
/**
* bool clear_cache = 4;
* @return The clearCache.
*/
@java.lang.Override
public boolean getClearCache() {
return clearCache_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
if (tableId_ != 0L) {
output.writeInt64(2, tableId_);
}
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
if (clearCache_ != false) {
output.writeBool(4, clearCache_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
if (tableId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, tableId_);
}
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
if (clearCache_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, clearCache_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.BroadcastRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.BroadcastRequest other = (cz.proto.ingestion.Ingestion.BroadcastRequest) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (getTableId()
!= other.getTableId()) return false;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (getClearCache()
!= other.getClearCache()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + TABLE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTableId());
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
hash = (37 * hash) + CLEAR_CACHE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getClearCache());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.BroadcastRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.BroadcastRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.BroadcastRequest)
cz.proto.ingestion.Ingestion.BroadcastRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.BroadcastRequest.class, cz.proto.ingestion.Ingestion.BroadcastRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.BroadcastRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
tableId_ = 0L;
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
clearCache_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.BroadcastRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastRequest build() {
cz.proto.ingestion.Ingestion.BroadcastRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastRequest buildPartial() {
cz.proto.ingestion.Ingestion.BroadcastRequest result = new cz.proto.ingestion.Ingestion.BroadcastRequest(this);
int from_bitField0_ = bitField0_;
result.instanceId_ = instanceId_;
result.tableId_ = tableId_;
if (((bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tabletId_ = tabletId_;
result.clearCache_ = clearCache_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.BroadcastRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.BroadcastRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.BroadcastRequest other) {
if (other == cz.proto.ingestion.Ingestion.BroadcastRequest.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (other.getTableId() != 0L) {
setTableId(other.getTableId());
}
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
if (other.getClearCache() != false) {
setClearCache(other.getClearCache());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.BroadcastRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.BroadcastRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long instanceId_ ;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private long tableId_ ;
/**
* int64 table_id = 2;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
/**
* int64 table_id = 2;
* @param value The tableId to set.
* @return This builder for chaining.
*/
public Builder setTableId(long value) {
tableId_ = value;
onChanged();
return this;
}
/**
* int64 table_id = 2;
* @return This builder for chaining.
*/
public Builder clearTableId() {
tableId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList tabletId_ = emptyLongList();
private void ensureTabletIdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tabletId_ = mutableCopy(tabletId_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
public java.util.List
getTabletIdList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(tabletId_) : tabletId_;
}
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
/**
* repeated int64 tablet_id = 3;
* @param index The index to set the value at.
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(
int index, long value) {
ensureTabletIdIsMutable();
tabletId_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @param value The tabletId to add.
* @return This builder for chaining.
*/
public Builder addTabletId(long value) {
ensureTabletIdIsMutable();
tabletId_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @param values The tabletId to add.
* @return This builder for chaining.
*/
public Builder addAllTabletId(
java.lang.Iterable extends java.lang.Long> values) {
ensureTabletIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tabletId_);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private boolean clearCache_ ;
/**
* bool clear_cache = 4;
* @return The clearCache.
*/
@java.lang.Override
public boolean getClearCache() {
return clearCache_;
}
/**
* bool clear_cache = 4;
* @param value The clearCache to set.
* @return This builder for chaining.
*/
public Builder setClearCache(boolean value) {
clearCache_ = value;
onChanged();
return this;
}
/**
* bool clear_cache = 4;
* @return This builder for chaining.
*/
public Builder clearClearCache() {
clearCache_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.BroadcastRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.BroadcastRequest)
private static final cz.proto.ingestion.Ingestion.BroadcastRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.BroadcastRequest();
}
public static cz.proto.ingestion.Ingestion.BroadcastRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BroadcastRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BroadcastRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BroadcastResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.BroadcastResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.BroadcastResponse}
*/
public static final class BroadcastResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.BroadcastResponse)
BroadcastResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use BroadcastResponse.newBuilder() to construct.
private BroadcastResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BroadcastResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BroadcastResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BroadcastResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.BroadcastResponse.class, cz.proto.ingestion.Ingestion.BroadcastResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.BroadcastResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.BroadcastResponse other = (cz.proto.ingestion.Ingestion.BroadcastResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.BroadcastResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.BroadcastResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.BroadcastResponse)
cz.proto.ingestion.Ingestion.BroadcastResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.BroadcastResponse.class, cz.proto.ingestion.Ingestion.BroadcastResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.BroadcastResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_BroadcastResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.BroadcastResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastResponse build() {
cz.proto.ingestion.Ingestion.BroadcastResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastResponse buildPartial() {
cz.proto.ingestion.Ingestion.BroadcastResponse result = new cz.proto.ingestion.Ingestion.BroadcastResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.BroadcastResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.BroadcastResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.BroadcastResponse other) {
if (other == cz.proto.ingestion.Ingestion.BroadcastResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.BroadcastResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.BroadcastResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.BroadcastResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.BroadcastResponse)
private static final cz.proto.ingestion.Ingestion.BroadcastResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.BroadcastResponse();
}
public static cz.proto.ingestion.Ingestion.BroadcastResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BroadcastResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BroadcastResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.BroadcastResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WorkerHBRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.WorkerHBRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 worker_id = 1;
* @return The workerId.
*/
long getWorkerId();
/**
* int64 worker_port = 2;
* @return The workerPort.
*/
long getWorkerPort();
/**
* string worker_host = 3;
* @return The workerHost.
*/
java.lang.String getWorkerHost();
/**
* string worker_host = 3;
* @return The bytes for workerHost.
*/
com.google.protobuf.ByteString
getWorkerHostBytes();
/**
* string message = 4;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 4;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerHBRequest}
*/
public static final class WorkerHBRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.WorkerHBRequest)
WorkerHBRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use WorkerHBRequest.newBuilder() to construct.
private WorkerHBRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WorkerHBRequest() {
workerHost_ = "";
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WorkerHBRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WorkerHBRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
workerId_ = input.readInt64();
break;
}
case 16: {
workerPort_ = input.readInt64();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
workerHost_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerHBRequest.class, cz.proto.ingestion.Ingestion.WorkerHBRequest.Builder.class);
}
public static final int WORKER_ID_FIELD_NUMBER = 1;
private long workerId_;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
public static final int WORKER_PORT_FIELD_NUMBER = 2;
private long workerPort_;
/**
* int64 worker_port = 2;
* @return The workerPort.
*/
@java.lang.Override
public long getWorkerPort() {
return workerPort_;
}
public static final int WORKER_HOST_FIELD_NUMBER = 3;
private volatile java.lang.Object workerHost_;
/**
* string worker_host = 3;
* @return The workerHost.
*/
@java.lang.Override
public java.lang.String getWorkerHost() {
java.lang.Object ref = workerHost_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workerHost_ = s;
return s;
}
}
/**
* string worker_host = 3;
* @return The bytes for workerHost.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkerHostBytes() {
java.lang.Object ref = workerHost_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MESSAGE_FIELD_NUMBER = 4;
private volatile java.lang.Object message_;
/**
* string message = 4;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 4;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (workerId_ != 0L) {
output.writeInt64(1, workerId_);
}
if (workerPort_ != 0L) {
output.writeInt64(2, workerPort_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workerHost_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, workerHost_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, message_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, workerId_);
}
if (workerPort_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, workerPort_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workerHost_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, workerHost_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, message_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.WorkerHBRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.WorkerHBRequest other = (cz.proto.ingestion.Ingestion.WorkerHBRequest) obj;
if (getWorkerId()
!= other.getWorkerId()) return false;
if (getWorkerPort()
!= other.getWorkerPort()) return false;
if (!getWorkerHost()
.equals(other.getWorkerHost())) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
hash = (37 * hash) + WORKER_PORT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerPort());
hash = (37 * hash) + WORKER_HOST_FIELD_NUMBER;
hash = (53 * hash) + getWorkerHost().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.WorkerHBRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerHBRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.WorkerHBRequest)
cz.proto.ingestion.Ingestion.WorkerHBRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerHBRequest.class, cz.proto.ingestion.Ingestion.WorkerHBRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.WorkerHBRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
workerId_ = 0L;
workerPort_ = 0L;
workerHost_ = "";
message_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.WorkerHBRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBRequest build() {
cz.proto.ingestion.Ingestion.WorkerHBRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBRequest buildPartial() {
cz.proto.ingestion.Ingestion.WorkerHBRequest result = new cz.proto.ingestion.Ingestion.WorkerHBRequest(this);
result.workerId_ = workerId_;
result.workerPort_ = workerPort_;
result.workerHost_ = workerHost_;
result.message_ = message_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.WorkerHBRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.WorkerHBRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.WorkerHBRequest other) {
if (other == cz.proto.ingestion.Ingestion.WorkerHBRequest.getDefaultInstance()) return this;
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
if (other.getWorkerPort() != 0L) {
setWorkerPort(other.getWorkerPort());
}
if (!other.getWorkerHost().isEmpty()) {
workerHost_ = other.workerHost_;
onChanged();
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.WorkerHBRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.WorkerHBRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long workerId_ ;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 1;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
private long workerPort_ ;
/**
* int64 worker_port = 2;
* @return The workerPort.
*/
@java.lang.Override
public long getWorkerPort() {
return workerPort_;
}
/**
* int64 worker_port = 2;
* @param value The workerPort to set.
* @return This builder for chaining.
*/
public Builder setWorkerPort(long value) {
workerPort_ = value;
onChanged();
return this;
}
/**
* int64 worker_port = 2;
* @return This builder for chaining.
*/
public Builder clearWorkerPort() {
workerPort_ = 0L;
onChanged();
return this;
}
private java.lang.Object workerHost_ = "";
/**
* string worker_host = 3;
* @return The workerHost.
*/
public java.lang.String getWorkerHost() {
java.lang.Object ref = workerHost_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workerHost_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string worker_host = 3;
* @return The bytes for workerHost.
*/
public com.google.protobuf.ByteString
getWorkerHostBytes() {
java.lang.Object ref = workerHost_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workerHost_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string worker_host = 3;
* @param value The workerHost to set.
* @return This builder for chaining.
*/
public Builder setWorkerHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workerHost_ = value;
onChanged();
return this;
}
/**
* string worker_host = 3;
* @return This builder for chaining.
*/
public Builder clearWorkerHost() {
workerHost_ = getDefaultInstance().getWorkerHost();
onChanged();
return this;
}
/**
* string worker_host = 3;
* @param value The bytes for workerHost to set.
* @return This builder for chaining.
*/
public Builder setWorkerHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workerHost_ = value;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 4;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 4;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 4;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 4;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 4;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.WorkerHBRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.WorkerHBRequest)
private static final cz.proto.ingestion.Ingestion.WorkerHBRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.WorkerHBRequest();
}
public static cz.proto.ingestion.Ingestion.WorkerHBRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WorkerHBRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WorkerHBRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WorkerHBResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.WorkerHBResponse)
com.google.protobuf.MessageOrBuilder {
/**
* int64 worker_id = 1;
* @return The workerId.
*/
long getWorkerId();
/**
* string message = 2;
* @return The message.
*/
java.lang.String getMessage();
/**
* string message = 2;
* @return The bytes for message.
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerHBResponse}
*/
public static final class WorkerHBResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.WorkerHBResponse)
WorkerHBResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use WorkerHBResponse.newBuilder() to construct.
private WorkerHBResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WorkerHBResponse() {
message_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WorkerHBResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WorkerHBResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
workerId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 26: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerHBResponse.class, cz.proto.ingestion.Ingestion.WorkerHBResponse.Builder.class);
}
public static final int WORKER_ID_FIELD_NUMBER = 1;
private long workerId_;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
public static final int MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object message_;
/**
* string message = 2;
* @return The message.
*/
@java.lang.Override
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 3;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (workerId_ != 0L) {
output.writeInt64(1, workerId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, message_);
}
if (status_ != null) {
output.writeMessage(3, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, workerId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(message_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, message_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.WorkerHBResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.WorkerHBResponse other = (cz.proto.ingestion.Ingestion.WorkerHBResponse) obj;
if (getWorkerId()
!= other.getWorkerId()) return false;
if (!getMessage()
.equals(other.getMessage())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.WorkerHBResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.WorkerHBResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.WorkerHBResponse)
cz.proto.ingestion.Ingestion.WorkerHBResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.WorkerHBResponse.class, cz.proto.ingestion.Ingestion.WorkerHBResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.WorkerHBResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
workerId_ = 0L;
message_ = "";
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_WorkerHBResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.WorkerHBResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBResponse build() {
cz.proto.ingestion.Ingestion.WorkerHBResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBResponse buildPartial() {
cz.proto.ingestion.Ingestion.WorkerHBResponse result = new cz.proto.ingestion.Ingestion.WorkerHBResponse(this);
result.workerId_ = workerId_;
result.message_ = message_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.WorkerHBResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.WorkerHBResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.WorkerHBResponse other) {
if (other == cz.proto.ingestion.Ingestion.WorkerHBResponse.getDefaultInstance()) return this;
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.WorkerHBResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.WorkerHBResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long workerId_ ;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 1;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
private java.lang.Object message_ = "";
/**
* string message = 2;
* @return The message.
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string message = 2;
* @return The bytes for message.
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string message = 2;
* @param value The message to set.
* @return This builder for chaining.
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
* string message = 2;
* @return This builder for chaining.
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
* string message = 2;
* @param value The bytes for message to set.
* @return This builder for chaining.
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.WorkerHBResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.WorkerHBResponse)
private static final cz.proto.ingestion.Ingestion.WorkerHBResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.WorkerHBResponse();
}
public static cz.proto.ingestion.Ingestion.WorkerHBResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WorkerHBResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WorkerHBResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.WorkerHBResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTabletsMappingRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTabletsMappingRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 worker_id = 1;
* @return The workerId.
*/
long getWorkerId();
/**
* string schema_name = 2;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 3;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletsMappingRequest}
*/
public static final class GetTabletsMappingRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTabletsMappingRequest)
GetTabletsMappingRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTabletsMappingRequest.newBuilder() to construct.
private GetTabletsMappingRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTabletsMappingRequest() {
schemaName_ = "";
tableName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTabletsMappingRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTabletsMappingRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
workerId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.class, cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.Builder.class);
}
public static final int WORKER_ID_FIELD_NUMBER = 1;
private long workerId_;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 2;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object tableName_;
/**
* string table_name = 3;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (workerId_ != 0L) {
output.writeInt64(1, workerId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, tableName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, workerId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, tableName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTabletsMappingRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest other = (cz.proto.ingestion.Ingestion.GetTabletsMappingRequest) obj;
if (getWorkerId()
!= other.getWorkerId()) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTabletsMappingRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletsMappingRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTabletsMappingRequest)
cz.proto.ingestion.Ingestion.GetTabletsMappingRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.class, cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
workerId_ = 0L;
schemaName_ = "";
tableName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingRequest build() {
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingRequest buildPartial() {
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest result = new cz.proto.ingestion.Ingestion.GetTabletsMappingRequest(this);
result.workerId_ = workerId_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTabletsMappingRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTabletsMappingRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTabletsMappingRequest other) {
if (other == cz.proto.ingestion.Ingestion.GetTabletsMappingRequest.getDefaultInstance()) return this;
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTabletsMappingRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTabletsMappingRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long workerId_ ;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 1;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 2;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 2;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 2;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 2;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 3;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 3;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 3;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 3;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTabletsMappingRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTabletsMappingRequest)
private static final cz.proto.ingestion.Ingestion.GetTabletsMappingRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTabletsMappingRequest();
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTabletsMappingRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTabletsMappingRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTabletsMappingResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTabletsMappingResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
int getTabletMappingCount();
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
boolean containsTabletMapping(
java.lang.String key);
/**
* Use {@link #getTabletMappingMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getTabletMapping();
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
java.util.Map
getTabletMappingMap();
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrDefault(
java.lang.String key,
cz.proto.ingestion.Ingestion.TabletIdList defaultValue);
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletsMappingResponse}
*/
public static final class GetTabletsMappingResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTabletsMappingResponse)
GetTabletsMappingResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTabletsMappingResponse.newBuilder() to construct.
private GetTabletsMappingResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTabletsMappingResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTabletsMappingResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTabletsMappingResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletMapping_ = com.google.protobuf.MapField.newMapField(
TabletMappingDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
tabletMapping__ = input.readMessage(
TabletMappingDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
tabletMapping_.getMutableMap().put(
tabletMapping__.getKey(), tabletMapping__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTabletMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.class, cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int TABLET_MAPPING_FIELD_NUMBER = 2;
private static final class TabletMappingDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, cz.proto.ingestion.Ingestion.TabletIdList> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_TabletMappingEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
cz.proto.ingestion.Ingestion.TabletIdList.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, cz.proto.ingestion.Ingestion.TabletIdList> tabletMapping_;
private com.google.protobuf.MapField
internalGetTabletMapping() {
if (tabletMapping_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TabletMappingDefaultEntryHolder.defaultEntry);
}
return tabletMapping_;
}
public int getTabletMappingCount() {
return internalGetTabletMapping().getMap().size();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public boolean containsTabletMapping(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTabletMapping().getMap().containsKey(key);
}
/**
* Use {@link #getTabletMappingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTabletMapping() {
return getTabletMappingMap();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public java.util.Map getTabletMappingMap() {
return internalGetTabletMapping().getMap();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrDefault(
java.lang.String key,
cz.proto.ingestion.Ingestion.TabletIdList defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTabletMapping().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTabletMapping().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetTabletMapping(),
TabletMappingDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
for (java.util.Map.Entry entry
: internalGetTabletMapping().getMap().entrySet()) {
com.google.protobuf.MapEntry
tabletMapping__ = TabletMappingDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, tabletMapping__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTabletsMappingResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse other = (cz.proto.ingestion.Ingestion.GetTabletsMappingResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!internalGetTabletMapping().equals(
other.internalGetTabletMapping())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (!internalGetTabletMapping().getMap().isEmpty()) {
hash = (37 * hash) + TABLET_MAPPING_FIELD_NUMBER;
hash = (53 * hash) + internalGetTabletMapping().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTabletsMappingResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletsMappingResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTabletsMappingResponse)
cz.proto.ingestion.Ingestion.GetTabletsMappingResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTabletMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableTabletMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.class, cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
internalGetMutableTabletMapping().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletsMappingResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingResponse build() {
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingResponse buildPartial() {
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse result = new cz.proto.ingestion.Ingestion.GetTabletsMappingResponse(this);
int from_bitField0_ = bitField0_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.tabletMapping_ = internalGetTabletMapping();
result.tabletMapping_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTabletsMappingResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTabletsMappingResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTabletsMappingResponse other) {
if (other == cz.proto.ingestion.Ingestion.GetTabletsMappingResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
internalGetMutableTabletMapping().mergeFrom(
other.internalGetTabletMapping());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTabletsMappingResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTabletsMappingResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, cz.proto.ingestion.Ingestion.TabletIdList> tabletMapping_;
private com.google.protobuf.MapField
internalGetTabletMapping() {
if (tabletMapping_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TabletMappingDefaultEntryHolder.defaultEntry);
}
return tabletMapping_;
}
private com.google.protobuf.MapField
internalGetMutableTabletMapping() {
onChanged();;
if (tabletMapping_ == null) {
tabletMapping_ = com.google.protobuf.MapField.newMapField(
TabletMappingDefaultEntryHolder.defaultEntry);
}
if (!tabletMapping_.isMutable()) {
tabletMapping_ = tabletMapping_.copy();
}
return tabletMapping_;
}
public int getTabletMappingCount() {
return internalGetTabletMapping().getMap().size();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public boolean containsTabletMapping(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetTabletMapping().getMap().containsKey(key);
}
/**
* Use {@link #getTabletMappingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getTabletMapping() {
return getTabletMappingMap();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public java.util.Map getTabletMappingMap() {
return internalGetTabletMapping().getMap();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrDefault(
java.lang.String key,
cz.proto.ingestion.Ingestion.TabletIdList defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTabletMapping().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getTabletMappingOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetTabletMapping().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearTabletMapping() {
internalGetMutableTabletMapping().getMutableMap()
.clear();
return this;
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
public Builder removeTabletMapping(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableTabletMapping().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableTabletMapping() {
return internalGetMutableTabletMapping().getMutableMap();
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
public Builder putTabletMapping(
java.lang.String key,
cz.proto.ingestion.Ingestion.TabletIdList value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableTabletMapping().getMutableMap()
.put(key, value);
return this;
}
/**
* map<string, .cz.proto.ingestion.TabletIdList> tablet_mapping = 2;
*/
public Builder putAllTabletMapping(
java.util.Map values) {
internalGetMutableTabletMapping().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTabletsMappingResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTabletsMappingResponse)
private static final cz.proto.ingestion.Ingestion.GetTabletsMappingResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTabletsMappingResponse();
}
public static cz.proto.ingestion.Ingestion.GetTabletsMappingResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTabletsMappingResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTabletsMappingResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletsMappingResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TabletIdListOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.TabletIdList)
com.google.protobuf.MessageOrBuilder {
/**
* repeated int64 tablet_id = 1;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 1;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 1;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.TabletIdList}
*/
public static final class TabletIdList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.TabletIdList)
TabletIdListOrBuilder {
private static final long serialVersionUID = 0L;
// Use TabletIdList.newBuilder() to construct.
private TabletIdList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TabletIdList() {
tabletId_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TabletIdList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TabletIdList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
tabletId_.addLong(input.readInt64());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletIdList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletIdList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.TabletIdList.class, cz.proto.ingestion.Ingestion.TabletIdList.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 1;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 1;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 1;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.TabletIdList)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.TabletIdList other = (cz.proto.ingestion.Ingestion.TabletIdList) obj;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletIdList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.TabletIdList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.TabletIdList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.TabletIdList)
cz.proto.ingestion.Ingestion.TabletIdListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletIdList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletIdList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.TabletIdList.class, cz.proto.ingestion.Ingestion.TabletIdList.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.TabletIdList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletIdList_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.TabletIdList.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList build() {
cz.proto.ingestion.Ingestion.TabletIdList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList buildPartial() {
cz.proto.ingestion.Ingestion.TabletIdList result = new cz.proto.ingestion.Ingestion.TabletIdList(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tabletId_ = tabletId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.TabletIdList) {
return mergeFrom((cz.proto.ingestion.Ingestion.TabletIdList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.TabletIdList other) {
if (other == cz.proto.ingestion.Ingestion.TabletIdList.getDefaultInstance()) return this;
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.TabletIdList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.TabletIdList) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.LongList tabletId_ = emptyLongList();
private void ensureTabletIdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tabletId_ = mutableCopy(tabletId_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 tablet_id = 1;
* @return A list containing the tabletId.
*/
public java.util.List
getTabletIdList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(tabletId_) : tabletId_;
}
/**
* repeated int64 tablet_id = 1;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 1;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
/**
* repeated int64 tablet_id = 1;
* @param index The index to set the value at.
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(
int index, long value) {
ensureTabletIdIsMutable();
tabletId_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 1;
* @param value The tabletId to add.
* @return This builder for chaining.
*/
public Builder addTabletId(long value) {
ensureTabletIdIsMutable();
tabletId_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 1;
* @param values The tabletId to add.
* @return This builder for chaining.
*/
public Builder addAllTabletId(
java.lang.Iterable extends java.lang.Long> values) {
ensureTabletIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tabletId_);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.TabletIdList)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.TabletIdList)
private static final cz.proto.ingestion.Ingestion.TabletIdList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.TabletIdList();
}
public static cz.proto.ingestion.Ingestion.TabletIdList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TabletIdList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TabletIdList(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletIdList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HostPortTupleOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.HostPortTuple)
com.google.protobuf.MessageOrBuilder {
/**
* string host = 1;
* @return The host.
*/
java.lang.String getHost();
/**
* string host = 1;
* @return The bytes for host.
*/
com.google.protobuf.ByteString
getHostBytes();
/**
* int32 port = 2;
* @return The port.
*/
int getPort();
}
/**
* Protobuf type {@code cz.proto.ingestion.HostPortTuple}
*/
public static final class HostPortTuple extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.HostPortTuple)
HostPortTupleOrBuilder {
private static final long serialVersionUID = 0L;
// Use HostPortTuple.newBuilder() to construct.
private HostPortTuple(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HostPortTuple() {
host_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HostPortTuple();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HostPortTuple(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
host_ = s;
break;
}
case 16: {
port_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_HostPortTuple_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_HostPortTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.HostPortTuple.class, cz.proto.ingestion.Ingestion.HostPortTuple.Builder.class);
}
public static final int HOST_FIELD_NUMBER = 1;
private volatile java.lang.Object host_;
/**
* string host = 1;
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
}
}
/**
* string host = 1;
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* int32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(host_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, host_);
}
if (port_ != 0) {
output.writeInt32(2, port_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(host_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, host_);
}
if (port_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, port_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.HostPortTuple)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.HostPortTuple other = (cz.proto.ingestion.Ingestion.HostPortTuple) obj;
if (!getHost()
.equals(other.getHost())) return false;
if (getPort()
!= other.getPort()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.HostPortTuple parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.HostPortTuple prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.HostPortTuple}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.HostPortTuple)
cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_HostPortTuple_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_HostPortTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.HostPortTuple.class, cz.proto.ingestion.Ingestion.HostPortTuple.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.HostPortTuple.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
host_ = "";
port_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_HostPortTuple_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple build() {
cz.proto.ingestion.Ingestion.HostPortTuple result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple buildPartial() {
cz.proto.ingestion.Ingestion.HostPortTuple result = new cz.proto.ingestion.Ingestion.HostPortTuple(this);
result.host_ = host_;
result.port_ = port_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.HostPortTuple) {
return mergeFrom((cz.proto.ingestion.Ingestion.HostPortTuple)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.HostPortTuple other) {
if (other == cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance()) return this;
if (!other.getHost().isEmpty()) {
host_ = other.host_;
onChanged();
}
if (other.getPort() != 0) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.HostPortTuple parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.HostPortTuple) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object host_ = "";
/**
* string host = 1;
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string host = 1;
* @return The bytes for host.
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string host = 1;
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
onChanged();
return this;
}
/**
* string host = 1;
* @return This builder for chaining.
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* string host = 1;
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
host_ = value;
onChanged();
return this;
}
private int port_ ;
/**
* int32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
/**
* int32 port = 2;
* @param value The port to set.
* @return This builder for chaining.
*/
public Builder setPort(int value) {
port_ = value;
onChanged();
return this;
}
/**
* int32 port = 2;
* @return This builder for chaining.
*/
public Builder clearPort() {
port_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.HostPortTuple)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.HostPortTuple)
private static final cz.proto.ingestion.Ingestion.HostPortTuple DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.HostPortTuple();
}
public static cz.proto.ingestion.Ingestion.HostPortTuple getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HostPortTuple parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HostPortTuple(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTabletPhysicsMappingRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTabletPhysicsMappingRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
long getTabletId();
/**
* string schema_name = 2;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 3;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletPhysicsMappingRequest}
*/
public static final class GetTabletPhysicsMappingRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTabletPhysicsMappingRequest)
GetTabletPhysicsMappingRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTabletPhysicsMappingRequest.newBuilder() to construct.
private GetTabletPhysicsMappingRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTabletPhysicsMappingRequest() {
schemaName_ = "";
tableName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTabletPhysicsMappingRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTabletPhysicsMappingRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
tabletId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.class, cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.Builder.class);
}
public static final int TABLET_ID_FIELD_NUMBER = 1;
private long tabletId_;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 2;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object tableName_;
/**
* string table_name = 3;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tabletId_ != 0L) {
output.writeInt64(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, tableName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, tabletId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, tableName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest other = (cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest) obj;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletPhysicsMappingRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTabletPhysicsMappingRequest)
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.class, cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tabletId_ = 0L;
schemaName_ = "";
tableName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest build() {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest buildPartial() {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest result = new cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest(this);
result.tabletId_ = tabletId_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest other) {
if (other == cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest.getDefaultInstance()) return this;
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 1;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 1;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 1;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 2;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 2;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 2;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 2;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 3;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 3;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 3;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 3;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTabletPhysicsMappingRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTabletPhysicsMappingRequest)
private static final cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest();
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTabletPhysicsMappingRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTabletPhysicsMappingRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TabletPhysicsInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.TabletPhysicsInfo)
com.google.protobuf.MessageOrBuilder {
/**
* int64 worker_id = 1;
* @return The workerId.
*/
long getWorkerId();
/**
* int64 tablet_id = 2;
* @return The tabletId.
*/
long getTabletId();
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return Whether the host field is set.
*/
boolean hasHost();
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return The host.
*/
cz.proto.ingestion.Ingestion.HostPortTuple getHost();
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder getHostOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.TabletPhysicsInfo}
*/
public static final class TabletPhysicsInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.TabletPhysicsInfo)
TabletPhysicsInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TabletPhysicsInfo.newBuilder() to construct.
private TabletPhysicsInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TabletPhysicsInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TabletPhysicsInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TabletPhysicsInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
workerId_ = input.readInt64();
break;
}
case 16: {
tabletId_ = input.readInt64();
break;
}
case 26: {
cz.proto.ingestion.Ingestion.HostPortTuple.Builder subBuilder = null;
if (host_ != null) {
subBuilder = host_.toBuilder();
}
host_ = input.readMessage(cz.proto.ingestion.Ingestion.HostPortTuple.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(host_);
host_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletPhysicsInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletPhysicsInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.TabletPhysicsInfo.class, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder.class);
}
public static final int WORKER_ID_FIELD_NUMBER = 1;
private long workerId_;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
public static final int TABLET_ID_FIELD_NUMBER = 2;
private long tabletId_;
/**
* int64 tablet_id = 2;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int HOST_FIELD_NUMBER = 3;
private cz.proto.ingestion.Ingestion.HostPortTuple host_;
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return host_ != null;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return The host.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getHost() {
return host_ == null ? cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance() : host_;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder getHostOrBuilder() {
return getHost();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (workerId_ != 0L) {
output.writeInt64(1, workerId_);
}
if (tabletId_ != 0L) {
output.writeInt64(2, tabletId_);
}
if (host_ != null) {
output.writeMessage(3, getHost());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, workerId_);
}
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, tabletId_);
}
if (host_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getHost());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.TabletPhysicsInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.TabletPhysicsInfo other = (cz.proto.ingestion.Ingestion.TabletPhysicsInfo) obj;
if (getWorkerId()
!= other.getWorkerId()) return false;
if (getTabletId()
!= other.getTabletId()) return false;
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost()
.equals(other.getHost())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.TabletPhysicsInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.TabletPhysicsInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.TabletPhysicsInfo)
cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletPhysicsInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletPhysicsInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.TabletPhysicsInfo.class, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.TabletPhysicsInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
workerId_ = 0L;
tabletId_ = 0L;
if (hostBuilder_ == null) {
host_ = null;
} else {
host_ = null;
hostBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_TabletPhysicsInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.TabletPhysicsInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo build() {
cz.proto.ingestion.Ingestion.TabletPhysicsInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo buildPartial() {
cz.proto.ingestion.Ingestion.TabletPhysicsInfo result = new cz.proto.ingestion.Ingestion.TabletPhysicsInfo(this);
result.workerId_ = workerId_;
result.tabletId_ = tabletId_;
if (hostBuilder_ == null) {
result.host_ = host_;
} else {
result.host_ = hostBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.TabletPhysicsInfo) {
return mergeFrom((cz.proto.ingestion.Ingestion.TabletPhysicsInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.TabletPhysicsInfo other) {
if (other == cz.proto.ingestion.Ingestion.TabletPhysicsInfo.getDefaultInstance()) return this;
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (other.hasHost()) {
mergeHost(other.getHost());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.TabletPhysicsInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.TabletPhysicsInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long workerId_ ;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 1;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 2;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 2;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 2;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.HostPortTuple host_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.HostPortTuple, cz.proto.ingestion.Ingestion.HostPortTuple.Builder, cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder> hostBuilder_;
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return Whether the host field is set.
*/
public boolean hasHost() {
return hostBuilder_ != null || host_ != null;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
* @return The host.
*/
public cz.proto.ingestion.Ingestion.HostPortTuple getHost() {
if (hostBuilder_ == null) {
return host_ == null ? cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance() : host_;
} else {
return hostBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public Builder setHost(cz.proto.ingestion.Ingestion.HostPortTuple value) {
if (hostBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
onChanged();
} else {
hostBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public Builder setHost(
cz.proto.ingestion.Ingestion.HostPortTuple.Builder builderForValue) {
if (hostBuilder_ == null) {
host_ = builderForValue.build();
onChanged();
} else {
hostBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public Builder mergeHost(cz.proto.ingestion.Ingestion.HostPortTuple value) {
if (hostBuilder_ == null) {
if (host_ != null) {
host_ =
cz.proto.ingestion.Ingestion.HostPortTuple.newBuilder(host_).mergeFrom(value).buildPartial();
} else {
host_ = value;
}
onChanged();
} else {
hostBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public Builder clearHost() {
if (hostBuilder_ == null) {
host_ = null;
onChanged();
} else {
host_ = null;
hostBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public cz.proto.ingestion.Ingestion.HostPortTuple.Builder getHostBuilder() {
onChanged();
return getHostFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
public cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder getHostOrBuilder() {
if (hostBuilder_ != null) {
return hostBuilder_.getMessageOrBuilder();
} else {
return host_ == null ?
cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance() : host_;
}
}
/**
* .cz.proto.ingestion.HostPortTuple host = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.HostPortTuple, cz.proto.ingestion.Ingestion.HostPortTuple.Builder, cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder>
getHostFieldBuilder() {
if (hostBuilder_ == null) {
hostBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.HostPortTuple, cz.proto.ingestion.Ingestion.HostPortTuple.Builder, cz.proto.ingestion.Ingestion.HostPortTupleOrBuilder>(
getHost(),
getParentForChildren(),
isClean());
host_ = null;
}
return hostBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.TabletPhysicsInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.TabletPhysicsInfo)
private static final cz.proto.ingestion.Ingestion.TabletPhysicsInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.TabletPhysicsInfo();
}
public static cz.proto.ingestion.Ingestion.TabletPhysicsInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TabletPhysicsInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TabletPhysicsInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetTabletPhysicsMappingResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetTabletPhysicsMappingResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
java.util.List
getTabletsList();
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
cz.proto.ingestion.Ingestion.TabletPhysicsInfo getTablets(int index);
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
int getTabletsCount();
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
java.util.List extends cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder>
getTabletsOrBuilderList();
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder getTabletsOrBuilder(
int index);
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletPhysicsMappingResponse}
*/
public static final class GetTabletPhysicsMappingResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetTabletPhysicsMappingResponse)
GetTabletPhysicsMappingResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetTabletPhysicsMappingResponse.newBuilder() to construct.
private GetTabletPhysicsMappingResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetTabletPhysicsMappingResponse() {
tablets_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetTabletPhysicsMappingResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetTabletPhysicsMappingResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tablets_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
tablets_.add(
input.readMessage(cz.proto.ingestion.Ingestion.TabletPhysicsInfo.parser(), extensionRegistry));
break;
}
case 18: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tablets_ = java.util.Collections.unmodifiableList(tablets_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.class, cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.Builder.class);
}
public static final int TABLETS_FIELD_NUMBER = 1;
private java.util.List tablets_;
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
@java.lang.Override
public java.util.List getTabletsList() {
return tablets_;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder>
getTabletsOrBuilderList() {
return tablets_;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
@java.lang.Override
public int getTabletsCount() {
return tablets_.size();
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo getTablets(int index) {
return tablets_.get(index);
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder getTabletsOrBuilder(
int index) {
return tablets_.get(index);
}
public static final int STATUS_FIELD_NUMBER = 2;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < tablets_.size(); i++) {
output.writeMessage(1, tablets_.get(i));
}
if (status_ != null) {
output.writeMessage(2, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < tablets_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, tablets_.get(i));
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse other = (cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse) obj;
if (!getTabletsList()
.equals(other.getTabletsList())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTabletsCount() > 0) {
hash = (37 * hash) + TABLETS_FIELD_NUMBER;
hash = (53 * hash) + getTabletsList().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetTabletPhysicsMappingResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetTabletPhysicsMappingResponse)
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.class, cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTabletsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tabletsBuilder_ == null) {
tablets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
tabletsBuilder_.clear();
}
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetTabletPhysicsMappingResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse build() {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse buildPartial() {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse result = new cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse(this);
int from_bitField0_ = bitField0_;
if (tabletsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
tablets_ = java.util.Collections.unmodifiableList(tablets_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tablets_ = tablets_;
} else {
result.tablets_ = tabletsBuilder_.build();
}
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse other) {
if (other == cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse.getDefaultInstance()) return this;
if (tabletsBuilder_ == null) {
if (!other.tablets_.isEmpty()) {
if (tablets_.isEmpty()) {
tablets_ = other.tablets_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletsIsMutable();
tablets_.addAll(other.tablets_);
}
onChanged();
}
} else {
if (!other.tablets_.isEmpty()) {
if (tabletsBuilder_.isEmpty()) {
tabletsBuilder_.dispose();
tabletsBuilder_ = null;
tablets_ = other.tablets_;
bitField0_ = (bitField0_ & ~0x00000001);
tabletsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTabletsFieldBuilder() : null;
} else {
tabletsBuilder_.addAllMessages(other.tablets_);
}
}
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List tablets_ =
java.util.Collections.emptyList();
private void ensureTabletsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tablets_ = new java.util.ArrayList(tablets_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.TabletPhysicsInfo, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder, cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder> tabletsBuilder_;
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public java.util.List getTabletsList() {
if (tabletsBuilder_ == null) {
return java.util.Collections.unmodifiableList(tablets_);
} else {
return tabletsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public int getTabletsCount() {
if (tabletsBuilder_ == null) {
return tablets_.size();
} else {
return tabletsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo getTablets(int index) {
if (tabletsBuilder_ == null) {
return tablets_.get(index);
} else {
return tabletsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder setTablets(
int index, cz.proto.ingestion.Ingestion.TabletPhysicsInfo value) {
if (tabletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTabletsIsMutable();
tablets_.set(index, value);
onChanged();
} else {
tabletsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder setTablets(
int index, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder builderForValue) {
if (tabletsBuilder_ == null) {
ensureTabletsIsMutable();
tablets_.set(index, builderForValue.build());
onChanged();
} else {
tabletsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder addTablets(cz.proto.ingestion.Ingestion.TabletPhysicsInfo value) {
if (tabletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTabletsIsMutable();
tablets_.add(value);
onChanged();
} else {
tabletsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder addTablets(
int index, cz.proto.ingestion.Ingestion.TabletPhysicsInfo value) {
if (tabletsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTabletsIsMutable();
tablets_.add(index, value);
onChanged();
} else {
tabletsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder addTablets(
cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder builderForValue) {
if (tabletsBuilder_ == null) {
ensureTabletsIsMutable();
tablets_.add(builderForValue.build());
onChanged();
} else {
tabletsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder addTablets(
int index, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder builderForValue) {
if (tabletsBuilder_ == null) {
ensureTabletsIsMutable();
tablets_.add(index, builderForValue.build());
onChanged();
} else {
tabletsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder addAllTablets(
java.lang.Iterable extends cz.proto.ingestion.Ingestion.TabletPhysicsInfo> values) {
if (tabletsBuilder_ == null) {
ensureTabletsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tablets_);
onChanged();
} else {
tabletsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder clearTablets() {
if (tabletsBuilder_ == null) {
tablets_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
tabletsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public Builder removeTablets(int index) {
if (tabletsBuilder_ == null) {
ensureTabletsIsMutable();
tablets_.remove(index);
onChanged();
} else {
tabletsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder getTabletsBuilder(
int index) {
return getTabletsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder getTabletsOrBuilder(
int index) {
if (tabletsBuilder_ == null) {
return tablets_.get(index); } else {
return tabletsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public java.util.List extends cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder>
getTabletsOrBuilderList() {
if (tabletsBuilder_ != null) {
return tabletsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tablets_);
}
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder addTabletsBuilder() {
return getTabletsFieldBuilder().addBuilder(
cz.proto.ingestion.Ingestion.TabletPhysicsInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder addTabletsBuilder(
int index) {
return getTabletsFieldBuilder().addBuilder(
index, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.TabletPhysicsInfo tablets = 1;
*/
public java.util.List
getTabletsBuilderList() {
return getTabletsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.TabletPhysicsInfo, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder, cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder>
getTabletsFieldBuilder() {
if (tabletsBuilder_ == null) {
tabletsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.Ingestion.TabletPhysicsInfo, cz.proto.ingestion.Ingestion.TabletPhysicsInfo.Builder, cz.proto.ingestion.Ingestion.TabletPhysicsInfoOrBuilder>(
tablets_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
tablets_ = null;
}
return tabletsBuilder_;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetTabletPhysicsMappingResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetTabletPhysicsMappingResponse)
private static final cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse();
}
public static cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetTabletPhysicsMappingResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetTabletPhysicsMappingResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetTabletPhysicsMappingResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetWorkersMappingRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetWorkersMappingRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 worker_id = 1;
* @return The workerId.
*/
long getWorkerId();
}
/**
* Protobuf type {@code cz.proto.ingestion.GetWorkersMappingRequest}
*/
public static final class GetWorkersMappingRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetWorkersMappingRequest)
GetWorkersMappingRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetWorkersMappingRequest.newBuilder() to construct.
private GetWorkersMappingRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetWorkersMappingRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetWorkersMappingRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetWorkersMappingRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
workerId_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.class, cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.Builder.class);
}
public static final int WORKER_ID_FIELD_NUMBER = 1;
private long workerId_;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (workerId_ != 0L) {
output.writeInt64(1, workerId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (workerId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, workerId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetWorkersMappingRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest other = (cz.proto.ingestion.Ingestion.GetWorkersMappingRequest) obj;
if (getWorkerId()
!= other.getWorkerId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WORKER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWorkerId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetWorkersMappingRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetWorkersMappingRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetWorkersMappingRequest)
cz.proto.ingestion.Ingestion.GetWorkersMappingRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.class, cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
workerId_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingRequest build() {
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingRequest buildPartial() {
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest result = new cz.proto.ingestion.Ingestion.GetWorkersMappingRequest(this);
result.workerId_ = workerId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetWorkersMappingRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetWorkersMappingRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetWorkersMappingRequest other) {
if (other == cz.proto.ingestion.Ingestion.GetWorkersMappingRequest.getDefaultInstance()) return this;
if (other.getWorkerId() != 0L) {
setWorkerId(other.getWorkerId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetWorkersMappingRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetWorkersMappingRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long workerId_ ;
/**
* int64 worker_id = 1;
* @return The workerId.
*/
@java.lang.Override
public long getWorkerId() {
return workerId_;
}
/**
* int64 worker_id = 1;
* @param value The workerId to set.
* @return This builder for chaining.
*/
public Builder setWorkerId(long value) {
workerId_ = value;
onChanged();
return this;
}
/**
* int64 worker_id = 1;
* @return This builder for chaining.
*/
public Builder clearWorkerId() {
workerId_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetWorkersMappingRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetWorkersMappingRequest)
private static final cz.proto.ingestion.Ingestion.GetWorkersMappingRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetWorkersMappingRequest();
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetWorkersMappingRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetWorkersMappingRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetWorkersMappingResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.GetWorkersMappingResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
int getWorkersMappingCount();
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
boolean containsWorkersMapping(
long key);
/**
* Use {@link #getWorkersMappingMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getWorkersMapping();
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
java.util.Map
getWorkersMappingMap();
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrDefault(
long key,
cz.proto.ingestion.Ingestion.HostPortTuple defaultValue);
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrThrow(
long key);
}
/**
* Protobuf type {@code cz.proto.ingestion.GetWorkersMappingResponse}
*/
public static final class GetWorkersMappingResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.GetWorkersMappingResponse)
GetWorkersMappingResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetWorkersMappingResponse.newBuilder() to construct.
private GetWorkersMappingResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetWorkersMappingResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetWorkersMappingResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetWorkersMappingResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
workersMapping_ = com.google.protobuf.MapField.newMapField(
WorkersMappingDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
workersMapping__ = input.readMessage(
WorkersMappingDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
workersMapping_.getMutableMap().put(
workersMapping__.getKey(), workersMapping__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetWorkersMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.class, cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int WORKERS_MAPPING_FIELD_NUMBER = 2;
private static final class WorkersMappingDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.Long, cz.proto.ingestion.Ingestion.HostPortTuple> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_WorkersMappingEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.INT64,
0L,
com.google.protobuf.WireFormat.FieldType.MESSAGE,
cz.proto.ingestion.Ingestion.HostPortTuple.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.Long, cz.proto.ingestion.Ingestion.HostPortTuple> workersMapping_;
private com.google.protobuf.MapField
internalGetWorkersMapping() {
if (workersMapping_ == null) {
return com.google.protobuf.MapField.emptyMapField(
WorkersMappingDefaultEntryHolder.defaultEntry);
}
return workersMapping_;
}
public int getWorkersMappingCount() {
return internalGetWorkersMapping().getMap().size();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public boolean containsWorkersMapping(
long key) {
return internalGetWorkersMapping().getMap().containsKey(key);
}
/**
* Use {@link #getWorkersMappingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getWorkersMapping() {
return getWorkersMappingMap();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public java.util.Map getWorkersMappingMap() {
return internalGetWorkersMapping().getMap();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrDefault(
long key,
cz.proto.ingestion.Ingestion.HostPortTuple defaultValue) {
java.util.Map map =
internalGetWorkersMapping().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrThrow(
long key) {
java.util.Map map =
internalGetWorkersMapping().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
com.google.protobuf.GeneratedMessageV3
.serializeLongMapTo(
output,
internalGetWorkersMapping(),
WorkersMappingDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
for (java.util.Map.Entry entry
: internalGetWorkersMapping().getMap().entrySet()) {
com.google.protobuf.MapEntry
workersMapping__ = WorkersMappingDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, workersMapping__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.GetWorkersMappingResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse other = (cz.proto.ingestion.Ingestion.GetWorkersMappingResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!internalGetWorkersMapping().equals(
other.internalGetWorkersMapping())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (!internalGetWorkersMapping().getMap().isEmpty()) {
hash = (37 * hash) + WORKERS_MAPPING_FIELD_NUMBER;
hash = (53 * hash) + internalGetWorkersMapping().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.GetWorkersMappingResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.GetWorkersMappingResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.GetWorkersMappingResponse)
cz.proto.ingestion.Ingestion.GetWorkersMappingResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetWorkersMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableWorkersMapping();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.class, cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
internalGetMutableWorkersMapping().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_GetWorkersMappingResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingResponse build() {
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingResponse buildPartial() {
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse result = new cz.proto.ingestion.Ingestion.GetWorkersMappingResponse(this);
int from_bitField0_ = bitField0_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.workersMapping_ = internalGetWorkersMapping();
result.workersMapping_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.GetWorkersMappingResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.GetWorkersMappingResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.GetWorkersMappingResponse other) {
if (other == cz.proto.ingestion.Ingestion.GetWorkersMappingResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
internalGetMutableWorkersMapping().mergeFrom(
other.internalGetWorkersMapping());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.GetWorkersMappingResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.GetWorkersMappingResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private com.google.protobuf.MapField<
java.lang.Long, cz.proto.ingestion.Ingestion.HostPortTuple> workersMapping_;
private com.google.protobuf.MapField
internalGetWorkersMapping() {
if (workersMapping_ == null) {
return com.google.protobuf.MapField.emptyMapField(
WorkersMappingDefaultEntryHolder.defaultEntry);
}
return workersMapping_;
}
private com.google.protobuf.MapField
internalGetMutableWorkersMapping() {
onChanged();;
if (workersMapping_ == null) {
workersMapping_ = com.google.protobuf.MapField.newMapField(
WorkersMappingDefaultEntryHolder.defaultEntry);
}
if (!workersMapping_.isMutable()) {
workersMapping_ = workersMapping_.copy();
}
return workersMapping_;
}
public int getWorkersMappingCount() {
return internalGetWorkersMapping().getMap().size();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public boolean containsWorkersMapping(
long key) {
return internalGetWorkersMapping().getMap().containsKey(key);
}
/**
* Use {@link #getWorkersMappingMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getWorkersMapping() {
return getWorkersMappingMap();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public java.util.Map getWorkersMappingMap() {
return internalGetWorkersMapping().getMap();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrDefault(
long key,
cz.proto.ingestion.Ingestion.HostPortTuple defaultValue) {
java.util.Map map =
internalGetWorkersMapping().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.HostPortTuple getWorkersMappingOrThrow(
long key) {
java.util.Map map =
internalGetWorkersMapping().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearWorkersMapping() {
internalGetMutableWorkersMapping().getMutableMap()
.clear();
return this;
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
public Builder removeWorkersMapping(
long key) {
internalGetMutableWorkersMapping().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableWorkersMapping() {
return internalGetMutableWorkersMapping().getMutableMap();
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
public Builder putWorkersMapping(
long key,
cz.proto.ingestion.Ingestion.HostPortTuple value) {
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableWorkersMapping().getMutableMap()
.put(key, value);
return this;
}
/**
* map<int64, .cz.proto.ingestion.HostPortTuple> workers_mapping = 2;
*/
public Builder putAllWorkersMapping(
java.util.Map values) {
internalGetMutableWorkersMapping().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.GetWorkersMappingResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.GetWorkersMappingResponse)
private static final cz.proto.ingestion.Ingestion.GetWorkersMappingResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.GetWorkersMappingResponse();
}
public static cz.proto.ingestion.Ingestion.GetWorkersMappingResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetWorkersMappingResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetWorkersMappingResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.GetWorkersMappingResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckTableExistsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.CheckTableExistsRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance = 1;
* @return The instance.
*/
long getInstance();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string schema_name = 3;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 4;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* int64 table_id = 5;
* @return The tableId.
*/
long getTableId();
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 6;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.CheckTableExistsRequest}
*/
public static final class CheckTableExistsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.CheckTableExistsRequest)
CheckTableExistsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckTableExistsRequest.newBuilder() to construct.
private CheckTableExistsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckTableExistsRequest() {
workspace_ = "";
schemaName_ = "";
tableName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckTableExistsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckTableExistsRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instance_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 40: {
tableId_ = input.readInt64();
break;
}
case 50: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CheckTableExistsRequest.class, cz.proto.ingestion.Ingestion.CheckTableExistsRequest.Builder.class);
}
public static final int INSTANCE_FIELD_NUMBER = 1;
private long instance_;
/**
* int64 instance = 1;
* @return The instance.
*/
@java.lang.Override
public long getInstance() {
return instance_;
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 3;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object tableName_;
/**
* string table_name = 4;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_ID_FIELD_NUMBER = 5;
private long tableId_;
/**
* int64 table_id = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
public static final int ACCOUNT_FIELD_NUMBER = 6;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (instance_ != 0L) {
output.writeInt64(1, instance_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tableName_);
}
if (tableId_ != 0L) {
output.writeInt64(5, tableId_);
}
if (account_ != null) {
output.writeMessage(6, getAccount());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instance_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instance_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tableName_);
}
if (tableId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, tableId_);
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getAccount());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.CheckTableExistsRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.CheckTableExistsRequest other = (cz.proto.ingestion.Ingestion.CheckTableExistsRequest) obj;
if (getInstance()
!= other.getInstance()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (getTableId()
!= other.getTableId()) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstance());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (37 * hash) + TABLE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTableId());
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.CheckTableExistsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.CheckTableExistsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.CheckTableExistsRequest)
cz.proto.ingestion.Ingestion.CheckTableExistsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CheckTableExistsRequest.class, cz.proto.ingestion.Ingestion.CheckTableExistsRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.CheckTableExistsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instance_ = 0L;
workspace_ = "";
schemaName_ = "";
tableName_ = "";
tableId_ = 0L;
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.CheckTableExistsRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsRequest build() {
cz.proto.ingestion.Ingestion.CheckTableExistsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsRequest buildPartial() {
cz.proto.ingestion.Ingestion.CheckTableExistsRequest result = new cz.proto.ingestion.Ingestion.CheckTableExistsRequest(this);
result.instance_ = instance_;
result.workspace_ = workspace_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
result.tableId_ = tableId_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.CheckTableExistsRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.CheckTableExistsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.CheckTableExistsRequest other) {
if (other == cz.proto.ingestion.Ingestion.CheckTableExistsRequest.getDefaultInstance()) return this;
if (other.getInstance() != 0L) {
setInstance(other.getInstance());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.getTableId() != 0L) {
setTableId(other.getTableId());
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.CheckTableExistsRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.CheckTableExistsRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long instance_ ;
/**
* int64 instance = 1;
* @return The instance.
*/
@java.lang.Override
public long getInstance() {
return instance_;
}
/**
* int64 instance = 1;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(long value) {
instance_ = value;
onChanged();
return this;
}
/**
* int64 instance = 1;
* @return This builder for chaining.
*/
public Builder clearInstance() {
instance_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 3;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 3;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 3;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 3;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 4;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 4;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 4;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 4;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private long tableId_ ;
/**
* int64 table_id = 5;
* @return The tableId.
*/
@java.lang.Override
public long getTableId() {
return tableId_;
}
/**
* int64 table_id = 5;
* @param value The tableId to set.
* @return This builder for chaining.
*/
public Builder setTableId(long value) {
tableId_ = value;
onChanged();
return this;
}
/**
* int64 table_id = 5;
* @return This builder for chaining.
*/
public Builder clearTableId() {
tableId_ = 0L;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 6;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 6;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.CheckTableExistsRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.CheckTableExistsRequest)
private static final cz.proto.ingestion.Ingestion.CheckTableExistsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.CheckTableExistsRequest();
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckTableExistsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckTableExistsRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CheckTableExistsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.CheckTableExistsResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.Ingestion.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.CheckTableExistsResponse}
*/
public static final class CheckTableExistsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.CheckTableExistsResponse)
CheckTableExistsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CheckTableExistsResponse.newBuilder() to construct.
private CheckTableExistsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CheckTableExistsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CheckTableExistsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CheckTableExistsResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.Ingestion.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.Ingestion.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CheckTableExistsResponse.class, cz.proto.ingestion.Ingestion.CheckTableExistsResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.CheckTableExistsResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.CheckTableExistsResponse other = (cz.proto.ingestion.Ingestion.CheckTableExistsResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.CheckTableExistsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.CheckTableExistsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.CheckTableExistsResponse)
cz.proto.ingestion.Ingestion.CheckTableExistsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.CheckTableExistsResponse.class, cz.proto.ingestion.Ingestion.CheckTableExistsResponse.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.CheckTableExistsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_CheckTableExistsResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsResponse getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.CheckTableExistsResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsResponse build() {
cz.proto.ingestion.Ingestion.CheckTableExistsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsResponse buildPartial() {
cz.proto.ingestion.Ingestion.CheckTableExistsResponse result = new cz.proto.ingestion.Ingestion.CheckTableExistsResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.CheckTableExistsResponse) {
return mergeFrom((cz.proto.ingestion.Ingestion.CheckTableExistsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.CheckTableExistsResponse other) {
if (other == cz.proto.ingestion.Ingestion.CheckTableExistsResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.CheckTableExistsResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.CheckTableExistsResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.Ingestion.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.Ingestion.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.Ingestion.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.Ingestion.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.Ingestion.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
public cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.Ingestion.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.ResponseStatus, cz.proto.ingestion.Ingestion.ResponseStatus.Builder, cz.proto.ingestion.Ingestion.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.CheckTableExistsResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.CheckTableExistsResponse)
private static final cz.proto.ingestion.Ingestion.CheckTableExistsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.CheckTableExistsResponse();
}
public static cz.proto.ingestion.Ingestion.CheckTableExistsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CheckTableExistsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CheckTableExistsResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.CheckTableExistsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataMutateRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DataMutateRequest)
com.google.protobuf.MessageOrBuilder {
/**
* int64 batch_id = 1;
* @return The batchId.
*/
long getBatchId();
/**
* int64 write_timestamp = 2;
* @return The writeTimestamp.
*/
long getWriteTimestamp();
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return Whether the rowOperations field is set.
*/
boolean hasRowOperations();
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return The rowOperations.
*/
org.apache.kudu.RowOperations.RowOperationsPB getRowOperations();
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRowOperationsOrBuilder();
/**
* string schema_name = 4;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 4;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 5;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 5;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* .cz.proto.Entity schema = 6;
* @return Whether the schema field is set.
*/
boolean hasSchema();
/**
* .cz.proto.Entity schema = 6;
* @return The schema.
*/
cz.proto.MetadataEntity.Entity getSchema();
/**
* .cz.proto.Entity schema = 6;
*/
cz.proto.MetadataEntity.EntityOrBuilder getSchemaOrBuilder();
/**
* .kudu.SchemaPB schema_pb = 7;
* @return Whether the schemaPb field is set.
*/
boolean hasSchemaPb();
/**
* .kudu.SchemaPB schema_pb = 7;
* @return The schemaPb.
*/
org.apache.kudu.KuduCommon.SchemaPB getSchemaPb();
/**
* .kudu.SchemaPB schema_pb = 7;
*/
org.apache.kudu.KuduCommon.SchemaPBOrBuilder getSchemaPbOrBuilder();
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
int getTableTypeValue();
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
cz.proto.ingestion.Ingestion.IGSTableType getTableType();
/**
* uint64 buckets_count = 9;
* @return The bucketsCount.
*/
long getBucketsCount();
/**
* bool is_dispatch = 10;
* @return The isDispatch.
*/
boolean getIsDispatch();
/**
* int64 tablet_id = 11;
* @return The tabletId.
*/
long getTabletId();
/**
* repeated string key_names = 12;
* @return A list containing the keyNames.
*/
java.util.List
getKeyNamesList();
/**
* repeated string key_names = 12;
* @return The count of keyNames.
*/
int getKeyNamesCount();
/**
* repeated string key_names = 12;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
java.lang.String getKeyNames(int index);
/**
* repeated string key_names = 12;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
com.google.protobuf.ByteString
getKeyNamesBytes(int index);
/**
* repeated string sort_names = 13;
* @return A list containing the sortNames.
*/
java.util.List
getSortNamesList();
/**
* repeated string sort_names = 13;
* @return The count of sortNames.
*/
int getSortNamesCount();
/**
* repeated string sort_names = 13;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
java.lang.String getSortNames(int index);
/**
* repeated string sort_names = 13;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
com.google.protobuf.ByteString
getSortNamesBytes(int index);
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return Whether the keyRowOperations field is set.
*/
boolean hasKeyRowOperations();
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return The keyRowOperations.
*/
org.apache.kudu.RowOperations.RowOperationsPB getKeyRowOperations();
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getKeyRowOperationsOrBuilder();
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return Whether the keySchemaPb field is set.
*/
boolean hasKeySchemaPb();
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return The keySchemaPb.
*/
org.apache.kudu.KuduCommon.SchemaPB getKeySchemaPb();
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
org.apache.kudu.KuduCommon.SchemaPBOrBuilder getKeySchemaPbOrBuilder();
/**
* repeated uint32 bucket_ids = 16;
* @return A list containing the bucketIds.
*/
java.util.List getBucketIdsList();
/**
* repeated uint32 bucket_ids = 16;
* @return The count of bucketIds.
*/
int getBucketIdsCount();
/**
* repeated uint32 bucket_ids = 16;
* @param index The index of the element to return.
* @return The bucketIds at the given index.
*/
int getBucketIds(int index);
/**
* repeated int32 indexes = 17;
* @return A list containing the indexes.
*/
java.util.List getIndexesList();
/**
* repeated int32 indexes = 17;
* @return The count of indexes.
*/
int getIndexesCount();
/**
* repeated int32 indexes = 17;
* @param index The index of the element to return.
* @return The indexes at the given index.
*/
int getIndexes(int index);
/**
* int64 instance_id = 18;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 19;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 19;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* .cz.proto.ingestion.Account account = 20;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.Account account = 20;
* @return The account.
*/
cz.proto.ingestion.Ingestion.Account getAccount();
/**
* .cz.proto.ingestion.Account account = 20;
*/
cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder();
/**
* string token = 21;
* @return The token.
*/
java.lang.String getToken();
/**
* string token = 21;
* @return The bytes for token.
*/
com.google.protobuf.ByteString
getTokenBytes();
/**
* string request_id = 22;
* @return The requestId.
*/
java.lang.String getRequestId();
/**
* string request_id = 22;
* @return The bytes for requestId.
*/
com.google.protobuf.ByteString
getRequestIdBytes();
/**
* int32 batch_count = 23;
* @return The batchCount.
*/
int getBatchCount();
}
/**
* Protobuf type {@code cz.proto.ingestion.DataMutateRequest}
*/
public static final class DataMutateRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.DataMutateRequest)
DataMutateRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataMutateRequest.newBuilder() to construct.
private DataMutateRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataMutateRequest() {
schemaName_ = "";
tableName_ = "";
tableType_ = 0;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bucketIds_ = emptyIntList();
indexes_ = emptyIntList();
workspace_ = "";
token_ = "";
requestId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DataMutateRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataMutateRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
batchId_ = input.readInt64();
break;
}
case 16: {
writeTimestamp_ = input.readInt64();
break;
}
case 26: {
org.apache.kudu.RowOperations.RowOperationsPB.Builder subBuilder = null;
if (rowOperations_ != null) {
subBuilder = rowOperations_.toBuilder();
}
rowOperations_ = input.readMessage(org.apache.kudu.RowOperations.RowOperationsPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rowOperations_);
rowOperations_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
case 50: {
cz.proto.MetadataEntity.Entity.Builder subBuilder = null;
if (schema_ != null) {
subBuilder = schema_.toBuilder();
}
schema_ = input.readMessage(cz.proto.MetadataEntity.Entity.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(schema_);
schema_ = subBuilder.buildPartial();
}
break;
}
case 58: {
org.apache.kudu.KuduCommon.SchemaPB.Builder subBuilder = null;
if (schemaPb_ != null) {
subBuilder = schemaPb_.toBuilder();
}
schemaPb_ = input.readMessage(org.apache.kudu.KuduCommon.SchemaPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(schemaPb_);
schemaPb_ = subBuilder.buildPartial();
}
break;
}
case 64: {
int rawValue = input.readEnum();
tableType_ = rawValue;
break;
}
case 72: {
bucketsCount_ = input.readUInt64();
break;
}
case 80: {
isDispatch_ = input.readBool();
break;
}
case 88: {
tabletId_ = input.readInt64();
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
keyNames_.add(s);
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
sortNames_.add(s);
break;
}
case 114: {
org.apache.kudu.RowOperations.RowOperationsPB.Builder subBuilder = null;
if (keyRowOperations_ != null) {
subBuilder = keyRowOperations_.toBuilder();
}
keyRowOperations_ = input.readMessage(org.apache.kudu.RowOperations.RowOperationsPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(keyRowOperations_);
keyRowOperations_ = subBuilder.buildPartial();
}
break;
}
case 122: {
org.apache.kudu.KuduCommon.SchemaPB.Builder subBuilder = null;
if (keySchemaPb_ != null) {
subBuilder = keySchemaPb_.toBuilder();
}
keySchemaPb_ = input.readMessage(org.apache.kudu.KuduCommon.SchemaPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(keySchemaPb_);
keySchemaPb_ = subBuilder.buildPartial();
}
break;
}
case 128: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
bucketIds_ = newIntList();
mutable_bitField0_ |= 0x00000004;
}
bucketIds_.addInt(input.readUInt32());
break;
}
case 130: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) != 0) && input.getBytesUntilLimit() > 0) {
bucketIds_ = newIntList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
bucketIds_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 136: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
indexes_ = newIntList();
mutable_bitField0_ |= 0x00000008;
}
indexes_.addInt(input.readInt32());
break;
}
case 138: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000008) != 0) && input.getBytesUntilLimit() > 0) {
indexes_ = newIntList();
mutable_bitField0_ |= 0x00000008;
}
while (input.getBytesUntilLimit() > 0) {
indexes_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
case 144: {
instanceId_ = input.readInt64();
break;
}
case 154: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 162: {
cz.proto.ingestion.Ingestion.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.Ingestion.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 170: {
java.lang.String s = input.readStringRequireUtf8();
token_ = s;
break;
}
case 178: {
java.lang.String s = input.readStringRequireUtf8();
requestId_ = s;
break;
}
case 184: {
batchCount_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
bucketIds_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
indexes_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DataMutateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DataMutateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DataMutateRequest.class, cz.proto.ingestion.Ingestion.DataMutateRequest.Builder.class);
}
public static final int BATCH_ID_FIELD_NUMBER = 1;
private long batchId_;
/**
* int64 batch_id = 1;
* @return The batchId.
*/
@java.lang.Override
public long getBatchId() {
return batchId_;
}
public static final int WRITE_TIMESTAMP_FIELD_NUMBER = 2;
private long writeTimestamp_;
/**
* int64 write_timestamp = 2;
* @return The writeTimestamp.
*/
@java.lang.Override
public long getWriteTimestamp() {
return writeTimestamp_;
}
public static final int ROW_OPERATIONS_FIELD_NUMBER = 3;
private org.apache.kudu.RowOperations.RowOperationsPB rowOperations_;
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return Whether the rowOperations field is set.
*/
@java.lang.Override
public boolean hasRowOperations() {
return rowOperations_ != null;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return The rowOperations.
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPB getRowOperations() {
return rowOperations_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rowOperations_;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRowOperationsOrBuilder() {
return getRowOperations();
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 4;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 4;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object tableName_;
/**
* string table_name = 5;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 5;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_FIELD_NUMBER = 6;
private cz.proto.MetadataEntity.Entity schema_;
/**
* .cz.proto.Entity schema = 6;
* @return Whether the schema field is set.
*/
@java.lang.Override
public boolean hasSchema() {
return schema_ != null;
}
/**
* .cz.proto.Entity schema = 6;
* @return The schema.
*/
@java.lang.Override
public cz.proto.MetadataEntity.Entity getSchema() {
return schema_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : schema_;
}
/**
* .cz.proto.Entity schema = 6;
*/
@java.lang.Override
public cz.proto.MetadataEntity.EntityOrBuilder getSchemaOrBuilder() {
return getSchema();
}
public static final int SCHEMA_PB_FIELD_NUMBER = 7;
private org.apache.kudu.KuduCommon.SchemaPB schemaPb_;
/**
* .kudu.SchemaPB schema_pb = 7;
* @return Whether the schemaPb field is set.
*/
@java.lang.Override
public boolean hasSchemaPb() {
return schemaPb_ != null;
}
/**
* .kudu.SchemaPB schema_pb = 7;
* @return The schemaPb.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB getSchemaPb() {
return schemaPb_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : schemaPb_;
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getSchemaPbOrBuilder() {
return getSchemaPb();
}
public static final int TABLE_TYPE_FIELD_NUMBER = 8;
private int tableType_;
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
@java.lang.Override public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
public static final int BUCKETS_COUNT_FIELD_NUMBER = 9;
private long bucketsCount_;
/**
* uint64 buckets_count = 9;
* @return The bucketsCount.
*/
@java.lang.Override
public long getBucketsCount() {
return bucketsCount_;
}
public static final int IS_DISPATCH_FIELD_NUMBER = 10;
private boolean isDispatch_;
/**
* bool is_dispatch = 10;
* @return The isDispatch.
*/
@java.lang.Override
public boolean getIsDispatch() {
return isDispatch_;
}
public static final int TABLET_ID_FIELD_NUMBER = 11;
private long tabletId_;
/**
* int64 tablet_id = 11;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
public static final int KEY_NAMES_FIELD_NUMBER = 12;
private com.google.protobuf.LazyStringList keyNames_;
/**
* repeated string key_names = 12;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_;
}
/**
* repeated string key_names = 12;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 12;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 12;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
public static final int SORT_NAMES_FIELD_NUMBER = 13;
private com.google.protobuf.LazyStringList sortNames_;
/**
* repeated string sort_names = 13;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_;
}
/**
* repeated string sort_names = 13;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 13;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 13;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
public static final int KEY_ROW_OPERATIONS_FIELD_NUMBER = 14;
private org.apache.kudu.RowOperations.RowOperationsPB keyRowOperations_;
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return Whether the keyRowOperations field is set.
*/
@java.lang.Override
public boolean hasKeyRowOperations() {
return keyRowOperations_ != null;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return The keyRowOperations.
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPB getKeyRowOperations() {
return keyRowOperations_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : keyRowOperations_;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getKeyRowOperationsOrBuilder() {
return getKeyRowOperations();
}
public static final int KEY_SCHEMA_PB_FIELD_NUMBER = 15;
private org.apache.kudu.KuduCommon.SchemaPB keySchemaPb_;
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return Whether the keySchemaPb field is set.
*/
@java.lang.Override
public boolean hasKeySchemaPb() {
return keySchemaPb_ != null;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return The keySchemaPb.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB getKeySchemaPb() {
return keySchemaPb_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : keySchemaPb_;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getKeySchemaPbOrBuilder() {
return getKeySchemaPb();
}
public static final int BUCKET_IDS_FIELD_NUMBER = 16;
private com.google.protobuf.Internal.IntList bucketIds_;
/**
* repeated uint32 bucket_ids = 16;
* @return A list containing the bucketIds.
*/
@java.lang.Override
public java.util.List
getBucketIdsList() {
return bucketIds_;
}
/**
* repeated uint32 bucket_ids = 16;
* @return The count of bucketIds.
*/
public int getBucketIdsCount() {
return bucketIds_.size();
}
/**
* repeated uint32 bucket_ids = 16;
* @param index The index of the element to return.
* @return The bucketIds at the given index.
*/
public int getBucketIds(int index) {
return bucketIds_.getInt(index);
}
private int bucketIdsMemoizedSerializedSize = -1;
public static final int INDEXES_FIELD_NUMBER = 17;
private com.google.protobuf.Internal.IntList indexes_;
/**
* repeated int32 indexes = 17;
* @return A list containing the indexes.
*/
@java.lang.Override
public java.util.List
getIndexesList() {
return indexes_;
}
/**
* repeated int32 indexes = 17;
* @return The count of indexes.
*/
public int getIndexesCount() {
return indexes_.size();
}
/**
* repeated int32 indexes = 17;
* @param index The index of the element to return.
* @return The indexes at the given index.
*/
public int getIndexes(int index) {
return indexes_.getInt(index);
}
private int indexesMemoizedSerializedSize = -1;
public static final int INSTANCE_ID_FIELD_NUMBER = 18;
private long instanceId_;
/**
* int64 instance_id = 18;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int WORKSPACE_FIELD_NUMBER = 19;
private volatile java.lang.Object workspace_;
/**
* string workspace = 19;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 19;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACCOUNT_FIELD_NUMBER = 20;
private cz.proto.ingestion.Ingestion.Account account_;
/**
* .cz.proto.ingestion.Account account = 20;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 20;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.Account getAccount() {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int TOKEN_FIELD_NUMBER = 21;
private volatile java.lang.Object token_;
/**
* string token = 21;
* @return The token.
*/
@java.lang.Override
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
}
}
/**
* string token = 21;
* @return The bytes for token.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_ID_FIELD_NUMBER = 22;
private volatile java.lang.Object requestId_;
/**
* string request_id = 22;
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
* string request_id = 22;
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BATCH_COUNT_FIELD_NUMBER = 23;
private int batchCount_;
/**
* int32 batch_count = 23;
* @return The batchCount.
*/
@java.lang.Override
public int getBatchCount() {
return batchCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasSchemaPb()) {
if (!getSchemaPb().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasKeySchemaPb()) {
if (!getKeySchemaPb().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (batchId_ != 0L) {
output.writeInt64(1, batchId_);
}
if (writeTimestamp_ != 0L) {
output.writeInt64(2, writeTimestamp_);
}
if (rowOperations_ != null) {
output.writeMessage(3, getRowOperations());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, tableName_);
}
if (schema_ != null) {
output.writeMessage(6, getSchema());
}
if (schemaPb_ != null) {
output.writeMessage(7, getSchemaPb());
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
output.writeEnum(8, tableType_);
}
if (bucketsCount_ != 0L) {
output.writeUInt64(9, bucketsCount_);
}
if (isDispatch_ != false) {
output.writeBool(10, isDispatch_);
}
if (tabletId_ != 0L) {
output.writeInt64(11, tabletId_);
}
for (int i = 0; i < keyNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, keyNames_.getRaw(i));
}
for (int i = 0; i < sortNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, sortNames_.getRaw(i));
}
if (keyRowOperations_ != null) {
output.writeMessage(14, getKeyRowOperations());
}
if (keySchemaPb_ != null) {
output.writeMessage(15, getKeySchemaPb());
}
if (getBucketIdsList().size() > 0) {
output.writeUInt32NoTag(130);
output.writeUInt32NoTag(bucketIdsMemoizedSerializedSize);
}
for (int i = 0; i < bucketIds_.size(); i++) {
output.writeUInt32NoTag(bucketIds_.getInt(i));
}
if (getIndexesList().size() > 0) {
output.writeUInt32NoTag(138);
output.writeUInt32NoTag(indexesMemoizedSerializedSize);
}
for (int i = 0; i < indexes_.size(); i++) {
output.writeInt32NoTag(indexes_.getInt(i));
}
if (instanceId_ != 0L) {
output.writeInt64(18, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, workspace_);
}
if (account_ != null) {
output.writeMessage(20, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(token_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, token_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 22, requestId_);
}
if (batchCount_ != 0) {
output.writeInt32(23, batchCount_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (batchId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, batchId_);
}
if (writeTimestamp_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, writeTimestamp_);
}
if (rowOperations_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getRowOperations());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, tableName_);
}
if (schema_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSchema());
}
if (schemaPb_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getSchemaPb());
}
if (tableType_ != cz.proto.ingestion.Ingestion.IGSTableType.NORMAL.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, tableType_);
}
if (bucketsCount_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(9, bucketsCount_);
}
if (isDispatch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, isDispatch_);
}
if (tabletId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, tabletId_);
}
{
int dataSize = 0;
for (int i = 0; i < keyNames_.size(); i++) {
dataSize += computeStringSizeNoTag(keyNames_.getRaw(i));
}
size += dataSize;
size += 1 * getKeyNamesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < sortNames_.size(); i++) {
dataSize += computeStringSizeNoTag(sortNames_.getRaw(i));
}
size += dataSize;
size += 1 * getSortNamesList().size();
}
if (keyRowOperations_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getKeyRowOperations());
}
if (keySchemaPb_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getKeySchemaPb());
}
{
int dataSize = 0;
for (int i = 0; i < bucketIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(bucketIds_.getInt(i));
}
size += dataSize;
if (!getBucketIdsList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
bucketIdsMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < indexes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(indexes_.getInt(i));
}
size += dataSize;
if (!getIndexesList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
indexesMemoizedSerializedSize = dataSize;
}
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, workspace_);
}
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getAccount());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(token_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, token_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(22, requestId_);
}
if (batchCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(23, batchCount_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.Ingestion.DataMutateRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.Ingestion.DataMutateRequest other = (cz.proto.ingestion.Ingestion.DataMutateRequest) obj;
if (getBatchId()
!= other.getBatchId()) return false;
if (getWriteTimestamp()
!= other.getWriteTimestamp()) return false;
if (hasRowOperations() != other.hasRowOperations()) return false;
if (hasRowOperations()) {
if (!getRowOperations()
.equals(other.getRowOperations())) return false;
}
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (hasSchema() != other.hasSchema()) return false;
if (hasSchema()) {
if (!getSchema()
.equals(other.getSchema())) return false;
}
if (hasSchemaPb() != other.hasSchemaPb()) return false;
if (hasSchemaPb()) {
if (!getSchemaPb()
.equals(other.getSchemaPb())) return false;
}
if (tableType_ != other.tableType_) return false;
if (getBucketsCount()
!= other.getBucketsCount()) return false;
if (getIsDispatch()
!= other.getIsDispatch()) return false;
if (getTabletId()
!= other.getTabletId()) return false;
if (!getKeyNamesList()
.equals(other.getKeyNamesList())) return false;
if (!getSortNamesList()
.equals(other.getSortNamesList())) return false;
if (hasKeyRowOperations() != other.hasKeyRowOperations()) return false;
if (hasKeyRowOperations()) {
if (!getKeyRowOperations()
.equals(other.getKeyRowOperations())) return false;
}
if (hasKeySchemaPb() != other.hasKeySchemaPb()) return false;
if (hasKeySchemaPb()) {
if (!getKeySchemaPb()
.equals(other.getKeySchemaPb())) return false;
}
if (!getBucketIdsList()
.equals(other.getBucketIdsList())) return false;
if (!getIndexesList()
.equals(other.getIndexesList())) return false;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (!getToken()
.equals(other.getToken())) return false;
if (!getRequestId()
.equals(other.getRequestId())) return false;
if (getBatchCount()
!= other.getBatchCount()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BATCH_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBatchId());
hash = (37 * hash) + WRITE_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWriteTimestamp());
if (hasRowOperations()) {
hash = (37 * hash) + ROW_OPERATIONS_FIELD_NUMBER;
hash = (53 * hash) + getRowOperations().hashCode();
}
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
if (hasSchema()) {
hash = (37 * hash) + SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getSchema().hashCode();
}
if (hasSchemaPb()) {
hash = (37 * hash) + SCHEMA_PB_FIELD_NUMBER;
hash = (53 * hash) + getSchemaPb().hashCode();
}
hash = (37 * hash) + TABLE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + tableType_;
hash = (37 * hash) + BUCKETS_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBucketsCount());
hash = (37 * hash) + IS_DISPATCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsDispatch());
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTabletId());
if (getKeyNamesCount() > 0) {
hash = (37 * hash) + KEY_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getKeyNamesList().hashCode();
}
if (getSortNamesCount() > 0) {
hash = (37 * hash) + SORT_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getSortNamesList().hashCode();
}
if (hasKeyRowOperations()) {
hash = (37 * hash) + KEY_ROW_OPERATIONS_FIELD_NUMBER;
hash = (53 * hash) + getKeyRowOperations().hashCode();
}
if (hasKeySchemaPb()) {
hash = (37 * hash) + KEY_SCHEMA_PB_FIELD_NUMBER;
hash = (53 * hash) + getKeySchemaPb().hashCode();
}
if (getBucketIdsCount() > 0) {
hash = (37 * hash) + BUCKET_IDS_FIELD_NUMBER;
hash = (53 * hash) + getBucketIdsList().hashCode();
}
if (getIndexesCount() > 0) {
hash = (37 * hash) + INDEXES_FIELD_NUMBER;
hash = (53 * hash) + getIndexesList().hashCode();
}
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
hash = (37 * hash) + BATCH_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getBatchCount();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.Ingestion.DataMutateRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.DataMutateRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.DataMutateRequest)
cz.proto.ingestion.Ingestion.DataMutateRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DataMutateRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DataMutateRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.Ingestion.DataMutateRequest.class, cz.proto.ingestion.Ingestion.DataMutateRequest.Builder.class);
}
// Construct using cz.proto.ingestion.Ingestion.DataMutateRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
batchId_ = 0L;
writeTimestamp_ = 0L;
if (rowOperationsBuilder_ == null) {
rowOperations_ = null;
} else {
rowOperations_ = null;
rowOperationsBuilder_ = null;
}
schemaName_ = "";
tableName_ = "";
if (schemaBuilder_ == null) {
schema_ = null;
} else {
schema_ = null;
schemaBuilder_ = null;
}
if (schemaPbBuilder_ == null) {
schemaPb_ = null;
} else {
schemaPb_ = null;
schemaPbBuilder_ = null;
}
tableType_ = 0;
bucketsCount_ = 0L;
isDispatch_ = false;
tabletId_ = 0L;
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
if (keyRowOperationsBuilder_ == null) {
keyRowOperations_ = null;
} else {
keyRowOperations_ = null;
keyRowOperationsBuilder_ = null;
}
if (keySchemaPbBuilder_ == null) {
keySchemaPb_ = null;
} else {
keySchemaPb_ = null;
keySchemaPbBuilder_ = null;
}
bucketIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
indexes_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000008);
instanceId_ = 0L;
workspace_ = "";
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
token_ = "";
requestId_ = "";
batchCount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.Ingestion.internal_static_cz_proto_ingestion_DataMutateRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DataMutateRequest getDefaultInstanceForType() {
return cz.proto.ingestion.Ingestion.DataMutateRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DataMutateRequest build() {
cz.proto.ingestion.Ingestion.DataMutateRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DataMutateRequest buildPartial() {
cz.proto.ingestion.Ingestion.DataMutateRequest result = new cz.proto.ingestion.Ingestion.DataMutateRequest(this);
int from_bitField0_ = bitField0_;
result.batchId_ = batchId_;
result.writeTimestamp_ = writeTimestamp_;
if (rowOperationsBuilder_ == null) {
result.rowOperations_ = rowOperations_;
} else {
result.rowOperations_ = rowOperationsBuilder_.build();
}
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
if (schemaBuilder_ == null) {
result.schema_ = schema_;
} else {
result.schema_ = schemaBuilder_.build();
}
if (schemaPbBuilder_ == null) {
result.schemaPb_ = schemaPb_;
} else {
result.schemaPb_ = schemaPbBuilder_.build();
}
result.tableType_ = tableType_;
result.bucketsCount_ = bucketsCount_;
result.isDispatch_ = isDispatch_;
result.tabletId_ = tabletId_;
if (((bitField0_ & 0x00000001) != 0)) {
keyNames_ = keyNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.keyNames_ = keyNames_;
if (((bitField0_ & 0x00000002) != 0)) {
sortNames_ = sortNames_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.sortNames_ = sortNames_;
if (keyRowOperationsBuilder_ == null) {
result.keyRowOperations_ = keyRowOperations_;
} else {
result.keyRowOperations_ = keyRowOperationsBuilder_.build();
}
if (keySchemaPbBuilder_ == null) {
result.keySchemaPb_ = keySchemaPb_;
} else {
result.keySchemaPb_ = keySchemaPbBuilder_.build();
}
if (((bitField0_ & 0x00000004) != 0)) {
bucketIds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.bucketIds_ = bucketIds_;
if (((bitField0_ & 0x00000008) != 0)) {
indexes_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000008);
}
result.indexes_ = indexes_;
result.instanceId_ = instanceId_;
result.workspace_ = workspace_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
result.token_ = token_;
result.requestId_ = requestId_;
result.batchCount_ = batchCount_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.Ingestion.DataMutateRequest) {
return mergeFrom((cz.proto.ingestion.Ingestion.DataMutateRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.Ingestion.DataMutateRequest other) {
if (other == cz.proto.ingestion.Ingestion.DataMutateRequest.getDefaultInstance()) return this;
if (other.getBatchId() != 0L) {
setBatchId(other.getBatchId());
}
if (other.getWriteTimestamp() != 0L) {
setWriteTimestamp(other.getWriteTimestamp());
}
if (other.hasRowOperations()) {
mergeRowOperations(other.getRowOperations());
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
if (other.hasSchema()) {
mergeSchema(other.getSchema());
}
if (other.hasSchemaPb()) {
mergeSchemaPb(other.getSchemaPb());
}
if (other.tableType_ != 0) {
setTableTypeValue(other.getTableTypeValue());
}
if (other.getBucketsCount() != 0L) {
setBucketsCount(other.getBucketsCount());
}
if (other.getIsDispatch() != false) {
setIsDispatch(other.getIsDispatch());
}
if (other.getTabletId() != 0L) {
setTabletId(other.getTabletId());
}
if (!other.keyNames_.isEmpty()) {
if (keyNames_.isEmpty()) {
keyNames_ = other.keyNames_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeyNamesIsMutable();
keyNames_.addAll(other.keyNames_);
}
onChanged();
}
if (!other.sortNames_.isEmpty()) {
if (sortNames_.isEmpty()) {
sortNames_ = other.sortNames_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSortNamesIsMutable();
sortNames_.addAll(other.sortNames_);
}
onChanged();
}
if (other.hasKeyRowOperations()) {
mergeKeyRowOperations(other.getKeyRowOperations());
}
if (other.hasKeySchemaPb()) {
mergeKeySchemaPb(other.getKeySchemaPb());
}
if (!other.bucketIds_.isEmpty()) {
if (bucketIds_.isEmpty()) {
bucketIds_ = other.bucketIds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureBucketIdsIsMutable();
bucketIds_.addAll(other.bucketIds_);
}
onChanged();
}
if (!other.indexes_.isEmpty()) {
if (indexes_.isEmpty()) {
indexes_ = other.indexes_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureIndexesIsMutable();
indexes_.addAll(other.indexes_);
}
onChanged();
}
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (!other.getToken().isEmpty()) {
token_ = other.token_;
onChanged();
}
if (!other.getRequestId().isEmpty()) {
requestId_ = other.requestId_;
onChanged();
}
if (other.getBatchCount() != 0) {
setBatchCount(other.getBatchCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasSchemaPb()) {
if (!getSchemaPb().isInitialized()) {
return false;
}
}
if (hasKeySchemaPb()) {
if (!getKeySchemaPb().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.Ingestion.DataMutateRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.Ingestion.DataMutateRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long batchId_ ;
/**
* int64 batch_id = 1;
* @return The batchId.
*/
@java.lang.Override
public long getBatchId() {
return batchId_;
}
/**
* int64 batch_id = 1;
* @param value The batchId to set.
* @return This builder for chaining.
*/
public Builder setBatchId(long value) {
batchId_ = value;
onChanged();
return this;
}
/**
* int64 batch_id = 1;
* @return This builder for chaining.
*/
public Builder clearBatchId() {
batchId_ = 0L;
onChanged();
return this;
}
private long writeTimestamp_ ;
/**
* int64 write_timestamp = 2;
* @return The writeTimestamp.
*/
@java.lang.Override
public long getWriteTimestamp() {
return writeTimestamp_;
}
/**
* int64 write_timestamp = 2;
* @param value The writeTimestamp to set.
* @return This builder for chaining.
*/
public Builder setWriteTimestamp(long value) {
writeTimestamp_ = value;
onChanged();
return this;
}
/**
* int64 write_timestamp = 2;
* @return This builder for chaining.
*/
public Builder clearWriteTimestamp() {
writeTimestamp_ = 0L;
onChanged();
return this;
}
private org.apache.kudu.RowOperations.RowOperationsPB rowOperations_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder> rowOperationsBuilder_;
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return Whether the rowOperations field is set.
*/
public boolean hasRowOperations() {
return rowOperationsBuilder_ != null || rowOperations_ != null;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
* @return The rowOperations.
*/
public org.apache.kudu.RowOperations.RowOperationsPB getRowOperations() {
if (rowOperationsBuilder_ == null) {
return rowOperations_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rowOperations_;
} else {
return rowOperationsBuilder_.getMessage();
}
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public Builder setRowOperations(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (rowOperationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rowOperations_ = value;
onChanged();
} else {
rowOperationsBuilder_.setMessage(value);
}
return this;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public Builder setRowOperations(
org.apache.kudu.RowOperations.RowOperationsPB.Builder builderForValue) {
if (rowOperationsBuilder_ == null) {
rowOperations_ = builderForValue.build();
onChanged();
} else {
rowOperationsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public Builder mergeRowOperations(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (rowOperationsBuilder_ == null) {
if (rowOperations_ != null) {
rowOperations_ =
org.apache.kudu.RowOperations.RowOperationsPB.newBuilder(rowOperations_).mergeFrom(value).buildPartial();
} else {
rowOperations_ = value;
}
onChanged();
} else {
rowOperationsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public Builder clearRowOperations() {
if (rowOperationsBuilder_ == null) {
rowOperations_ = null;
onChanged();
} else {
rowOperations_ = null;
rowOperationsBuilder_ = null;
}
return this;
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public org.apache.kudu.RowOperations.RowOperationsPB.Builder getRowOperationsBuilder() {
onChanged();
return getRowOperationsFieldBuilder().getBuilder();
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRowOperationsOrBuilder() {
if (rowOperationsBuilder_ != null) {
return rowOperationsBuilder_.getMessageOrBuilder();
} else {
return rowOperations_ == null ?
org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rowOperations_;
}
}
/**
* .kudu.RowOperationsPB row_operations = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>
getRowOperationsFieldBuilder() {
if (rowOperationsBuilder_ == null) {
rowOperationsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>(
getRowOperations(),
getParentForChildren(),
isClean());
rowOperations_ = null;
}
return rowOperationsBuilder_;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 4;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 4;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 4;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 4;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 4;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 5;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 5;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 5;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 5;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 5;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
private cz.proto.MetadataEntity.Entity schema_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder> schemaBuilder_;
/**
* .cz.proto.Entity schema = 6;
* @return Whether the schema field is set.
*/
public boolean hasSchema() {
return schemaBuilder_ != null || schema_ != null;
}
/**
* .cz.proto.Entity schema = 6;
* @return The schema.
*/
public cz.proto.MetadataEntity.Entity getSchema() {
if (schemaBuilder_ == null) {
return schema_ == null ? cz.proto.MetadataEntity.Entity.getDefaultInstance() : schema_;
} else {
return schemaBuilder_.getMessage();
}
}
/**
* .cz.proto.Entity schema = 6;
*/
public Builder setSchema(cz.proto.MetadataEntity.Entity value) {
if (schemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
schema_ = value;
onChanged();
} else {
schemaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.Entity schema = 6;
*/
public Builder setSchema(
cz.proto.MetadataEntity.Entity.Builder builderForValue) {
if (schemaBuilder_ == null) {
schema_ = builderForValue.build();
onChanged();
} else {
schemaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.Entity schema = 6;
*/
public Builder mergeSchema(cz.proto.MetadataEntity.Entity value) {
if (schemaBuilder_ == null) {
if (schema_ != null) {
schema_ =
cz.proto.MetadataEntity.Entity.newBuilder(schema_).mergeFrom(value).buildPartial();
} else {
schema_ = value;
}
onChanged();
} else {
schemaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.Entity schema = 6;
*/
public Builder clearSchema() {
if (schemaBuilder_ == null) {
schema_ = null;
onChanged();
} else {
schema_ = null;
schemaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.Entity schema = 6;
*/
public cz.proto.MetadataEntity.Entity.Builder getSchemaBuilder() {
onChanged();
return getSchemaFieldBuilder().getBuilder();
}
/**
* .cz.proto.Entity schema = 6;
*/
public cz.proto.MetadataEntity.EntityOrBuilder getSchemaOrBuilder() {
if (schemaBuilder_ != null) {
return schemaBuilder_.getMessageOrBuilder();
} else {
return schema_ == null ?
cz.proto.MetadataEntity.Entity.getDefaultInstance() : schema_;
}
}
/**
* .cz.proto.Entity schema = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>
getSchemaFieldBuilder() {
if (schemaBuilder_ == null) {
schemaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.MetadataEntity.Entity, cz.proto.MetadataEntity.Entity.Builder, cz.proto.MetadataEntity.EntityOrBuilder>(
getSchema(),
getParentForChildren(),
isClean());
schema_ = null;
}
return schemaBuilder_;
}
private org.apache.kudu.KuduCommon.SchemaPB schemaPb_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder> schemaPbBuilder_;
/**
* .kudu.SchemaPB schema_pb = 7;
* @return Whether the schemaPb field is set.
*/
public boolean hasSchemaPb() {
return schemaPbBuilder_ != null || schemaPb_ != null;
}
/**
* .kudu.SchemaPB schema_pb = 7;
* @return The schemaPb.
*/
public org.apache.kudu.KuduCommon.SchemaPB getSchemaPb() {
if (schemaPbBuilder_ == null) {
return schemaPb_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : schemaPb_;
} else {
return schemaPbBuilder_.getMessage();
}
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public Builder setSchemaPb(org.apache.kudu.KuduCommon.SchemaPB value) {
if (schemaPbBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
schemaPb_ = value;
onChanged();
} else {
schemaPbBuilder_.setMessage(value);
}
return this;
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public Builder setSchemaPb(
org.apache.kudu.KuduCommon.SchemaPB.Builder builderForValue) {
if (schemaPbBuilder_ == null) {
schemaPb_ = builderForValue.build();
onChanged();
} else {
schemaPbBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public Builder mergeSchemaPb(org.apache.kudu.KuduCommon.SchemaPB value) {
if (schemaPbBuilder_ == null) {
if (schemaPb_ != null) {
schemaPb_ =
org.apache.kudu.KuduCommon.SchemaPB.newBuilder(schemaPb_).mergeFrom(value).buildPartial();
} else {
schemaPb_ = value;
}
onChanged();
} else {
schemaPbBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public Builder clearSchemaPb() {
if (schemaPbBuilder_ == null) {
schemaPb_ = null;
onChanged();
} else {
schemaPb_ = null;
schemaPbBuilder_ = null;
}
return this;
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public org.apache.kudu.KuduCommon.SchemaPB.Builder getSchemaPbBuilder() {
onChanged();
return getSchemaPbFieldBuilder().getBuilder();
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getSchemaPbOrBuilder() {
if (schemaPbBuilder_ != null) {
return schemaPbBuilder_.getMessageOrBuilder();
} else {
return schemaPb_ == null ?
org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : schemaPb_;
}
}
/**
* .kudu.SchemaPB schema_pb = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>
getSchemaPbFieldBuilder() {
if (schemaPbBuilder_ == null) {
schemaPbBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>(
getSchemaPb(),
getParentForChildren(),
isClean());
schemaPb_ = null;
}
return schemaPbBuilder_;
}
private int tableType_ = 0;
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The enum numeric value on the wire for tableType.
*/
@java.lang.Override public int getTableTypeValue() {
return tableType_;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @param value The enum numeric value on the wire for tableType to set.
* @return This builder for chaining.
*/
public Builder setTableTypeValue(int value) {
tableType_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return The tableType.
*/
@java.lang.Override
public cz.proto.ingestion.Ingestion.IGSTableType getTableType() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.Ingestion.IGSTableType result = cz.proto.ingestion.Ingestion.IGSTableType.valueOf(tableType_);
return result == null ? cz.proto.ingestion.Ingestion.IGSTableType.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @param value The tableType to set.
* @return This builder for chaining.
*/
public Builder setTableType(cz.proto.ingestion.Ingestion.IGSTableType value) {
if (value == null) {
throw new NullPointerException();
}
tableType_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.IGSTableType table_type = 8;
* @return This builder for chaining.
*/
public Builder clearTableType() {
tableType_ = 0;
onChanged();
return this;
}
private long bucketsCount_ ;
/**
* uint64 buckets_count = 9;
* @return The bucketsCount.
*/
@java.lang.Override
public long getBucketsCount() {
return bucketsCount_;
}
/**
* uint64 buckets_count = 9;
* @param value The bucketsCount to set.
* @return This builder for chaining.
*/
public Builder setBucketsCount(long value) {
bucketsCount_ = value;
onChanged();
return this;
}
/**
* uint64 buckets_count = 9;
* @return This builder for chaining.
*/
public Builder clearBucketsCount() {
bucketsCount_ = 0L;
onChanged();
return this;
}
private boolean isDispatch_ ;
/**
* bool is_dispatch = 10;
* @return The isDispatch.
*/
@java.lang.Override
public boolean getIsDispatch() {
return isDispatch_;
}
/**
* bool is_dispatch = 10;
* @param value The isDispatch to set.
* @return This builder for chaining.
*/
public Builder setIsDispatch(boolean value) {
isDispatch_ = value;
onChanged();
return this;
}
/**
* bool is_dispatch = 10;
* @return This builder for chaining.
*/
public Builder clearIsDispatch() {
isDispatch_ = false;
onChanged();
return this;
}
private long tabletId_ ;
/**
* int64 tablet_id = 11;
* @return The tabletId.
*/
@java.lang.Override
public long getTabletId() {
return tabletId_;
}
/**
* int64 tablet_id = 11;
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(long value) {
tabletId_ = value;
onChanged();
return this;
}
/**
* int64 tablet_id = 11;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureKeyNamesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
keyNames_ = new com.google.protobuf.LazyStringArrayList(keyNames_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string key_names = 12;
* @return A list containing the keyNames.
*/
public com.google.protobuf.ProtocolStringList
getKeyNamesList() {
return keyNames_.getUnmodifiableView();
}
/**
* repeated string key_names = 12;
* @return The count of keyNames.
*/
public int getKeyNamesCount() {
return keyNames_.size();
}
/**
* repeated string key_names = 12;
* @param index The index of the element to return.
* @return The keyNames at the given index.
*/
public java.lang.String getKeyNames(int index) {
return keyNames_.get(index);
}
/**
* repeated string key_names = 12;
* @param index The index of the value to return.
* @return The bytes of the keyNames at the given index.
*/
public com.google.protobuf.ByteString
getKeyNamesBytes(int index) {
return keyNames_.getByteString(index);
}
/**
* repeated string key_names = 12;
* @param index The index to set the value at.
* @param value The keyNames to set.
* @return This builder for chaining.
*/
public Builder setKeyNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string key_names = 12;
* @param value The keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
/**
* repeated string key_names = 12;
* @param values The keyNames to add.
* @return This builder for chaining.
*/
public Builder addAllKeyNames(
java.lang.Iterable values) {
ensureKeyNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, keyNames_);
onChanged();
return this;
}
/**
* repeated string key_names = 12;
* @return This builder for chaining.
*/
public Builder clearKeyNames() {
keyNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string key_names = 12;
* @param value The bytes of the keyNames to add.
* @return This builder for chaining.
*/
public Builder addKeyNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureKeyNamesIsMutable();
keyNames_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSortNamesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
sortNames_ = new com.google.protobuf.LazyStringArrayList(sortNames_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string sort_names = 13;
* @return A list containing the sortNames.
*/
public com.google.protobuf.ProtocolStringList
getSortNamesList() {
return sortNames_.getUnmodifiableView();
}
/**
* repeated string sort_names = 13;
* @return The count of sortNames.
*/
public int getSortNamesCount() {
return sortNames_.size();
}
/**
* repeated string sort_names = 13;
* @param index The index of the element to return.
* @return The sortNames at the given index.
*/
public java.lang.String getSortNames(int index) {
return sortNames_.get(index);
}
/**
* repeated string sort_names = 13;
* @param index The index of the value to return.
* @return The bytes of the sortNames at the given index.
*/
public com.google.protobuf.ByteString
getSortNamesBytes(int index) {
return sortNames_.getByteString(index);
}
/**
* repeated string sort_names = 13;
* @param index The index to set the value at.
* @param value The sortNames to set.
* @return This builder for chaining.
*/
public Builder setSortNames(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sort_names = 13;
* @param value The sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNames(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
/**
* repeated string sort_names = 13;
* @param values The sortNames to add.
* @return This builder for chaining.
*/
public Builder addAllSortNames(
java.lang.Iterable values) {
ensureSortNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sortNames_);
onChanged();
return this;
}
/**
* repeated string sort_names = 13;
* @return This builder for chaining.
*/
public Builder clearSortNames() {
sortNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string sort_names = 13;
* @param value The bytes of the sortNames to add.
* @return This builder for chaining.
*/
public Builder addSortNamesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSortNamesIsMutable();
sortNames_.add(value);
onChanged();
return this;
}
private org.apache.kudu.RowOperations.RowOperationsPB keyRowOperations_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder> keyRowOperationsBuilder_;
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return Whether the keyRowOperations field is set.
*/
public boolean hasKeyRowOperations() {
return keyRowOperationsBuilder_ != null || keyRowOperations_ != null;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
* @return The keyRowOperations.
*/
public org.apache.kudu.RowOperations.RowOperationsPB getKeyRowOperations() {
if (keyRowOperationsBuilder_ == null) {
return keyRowOperations_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : keyRowOperations_;
} else {
return keyRowOperationsBuilder_.getMessage();
}
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public Builder setKeyRowOperations(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (keyRowOperationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
keyRowOperations_ = value;
onChanged();
} else {
keyRowOperationsBuilder_.setMessage(value);
}
return this;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public Builder setKeyRowOperations(
org.apache.kudu.RowOperations.RowOperationsPB.Builder builderForValue) {
if (keyRowOperationsBuilder_ == null) {
keyRowOperations_ = builderForValue.build();
onChanged();
} else {
keyRowOperationsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public Builder mergeKeyRowOperations(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (keyRowOperationsBuilder_ == null) {
if (keyRowOperations_ != null) {
keyRowOperations_ =
org.apache.kudu.RowOperations.RowOperationsPB.newBuilder(keyRowOperations_).mergeFrom(value).buildPartial();
} else {
keyRowOperations_ = value;
}
onChanged();
} else {
keyRowOperationsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public Builder clearKeyRowOperations() {
if (keyRowOperationsBuilder_ == null) {
keyRowOperations_ = null;
onChanged();
} else {
keyRowOperations_ = null;
keyRowOperationsBuilder_ = null;
}
return this;
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public org.apache.kudu.RowOperations.RowOperationsPB.Builder getKeyRowOperationsBuilder() {
onChanged();
return getKeyRowOperationsFieldBuilder().getBuilder();
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getKeyRowOperationsOrBuilder() {
if (keyRowOperationsBuilder_ != null) {
return keyRowOperationsBuilder_.getMessageOrBuilder();
} else {
return keyRowOperations_ == null ?
org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : keyRowOperations_;
}
}
/**
* .kudu.RowOperationsPB key_row_operations = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>
getKeyRowOperationsFieldBuilder() {
if (keyRowOperationsBuilder_ == null) {
keyRowOperationsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>(
getKeyRowOperations(),
getParentForChildren(),
isClean());
keyRowOperations_ = null;
}
return keyRowOperationsBuilder_;
}
private org.apache.kudu.KuduCommon.SchemaPB keySchemaPb_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder> keySchemaPbBuilder_;
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return Whether the keySchemaPb field is set.
*/
public boolean hasKeySchemaPb() {
return keySchemaPbBuilder_ != null || keySchemaPb_ != null;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
* @return The keySchemaPb.
*/
public org.apache.kudu.KuduCommon.SchemaPB getKeySchemaPb() {
if (keySchemaPbBuilder_ == null) {
return keySchemaPb_ == null ? org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : keySchemaPb_;
} else {
return keySchemaPbBuilder_.getMessage();
}
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public Builder setKeySchemaPb(org.apache.kudu.KuduCommon.SchemaPB value) {
if (keySchemaPbBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
keySchemaPb_ = value;
onChanged();
} else {
keySchemaPbBuilder_.setMessage(value);
}
return this;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public Builder setKeySchemaPb(
org.apache.kudu.KuduCommon.SchemaPB.Builder builderForValue) {
if (keySchemaPbBuilder_ == null) {
keySchemaPb_ = builderForValue.build();
onChanged();
} else {
keySchemaPbBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public Builder mergeKeySchemaPb(org.apache.kudu.KuduCommon.SchemaPB value) {
if (keySchemaPbBuilder_ == null) {
if (keySchemaPb_ != null) {
keySchemaPb_ =
org.apache.kudu.KuduCommon.SchemaPB.newBuilder(keySchemaPb_).mergeFrom(value).buildPartial();
} else {
keySchemaPb_ = value;
}
onChanged();
} else {
keySchemaPbBuilder_.mergeFrom(value);
}
return this;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public Builder clearKeySchemaPb() {
if (keySchemaPbBuilder_ == null) {
keySchemaPb_ = null;
onChanged();
} else {
keySchemaPb_ = null;
keySchemaPbBuilder_ = null;
}
return this;
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public org.apache.kudu.KuduCommon.SchemaPB.Builder getKeySchemaPbBuilder() {
onChanged();
return getKeySchemaPbFieldBuilder().getBuilder();
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
public org.apache.kudu.KuduCommon.SchemaPBOrBuilder getKeySchemaPbOrBuilder() {
if (keySchemaPbBuilder_ != null) {
return keySchemaPbBuilder_.getMessageOrBuilder();
} else {
return keySchemaPb_ == null ?
org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance() : keySchemaPb_;
}
}
/**
* .kudu.SchemaPB key_schema_pb = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>
getKeySchemaPbFieldBuilder() {
if (keySchemaPbBuilder_ == null) {
keySchemaPbBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.SchemaPB, org.apache.kudu.KuduCommon.SchemaPB.Builder, org.apache.kudu.KuduCommon.SchemaPBOrBuilder>(
getKeySchemaPb(),
getParentForChildren(),
isClean());
keySchemaPb_ = null;
}
return keySchemaPbBuilder_;
}
private com.google.protobuf.Internal.IntList bucketIds_ = emptyIntList();
private void ensureBucketIdsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
bucketIds_ = mutableCopy(bucketIds_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated uint32 bucket_ids = 16;
* @return A list containing the bucketIds.
*/
public java.util.List
getBucketIdsList() {
return ((bitField0_ & 0x00000004) != 0) ?
java.util.Collections.unmodifiableList(bucketIds_) : bucketIds_;
}
/**
* repeated uint32 bucket_ids = 16;
* @return The count of bucketIds.
*/
public int getBucketIdsCount() {
return bucketIds_.size();
}
/**
* repeated uint32 bucket_ids = 16;
* @param index The index of the element to return.
* @return The bucketIds at the given index.
*/
public int getBucketIds(int index) {
return bucketIds_.getInt(index);
}
/**
* repeated uint32 bucket_ids = 16;
* @param index The index to set the value at.
* @param value The bucketIds to set.
* @return This builder for chaining.
*/
public Builder setBucketIds(
int index, int value) {
ensureBucketIdsIsMutable();
bucketIds_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated uint32 bucket_ids = 16;
* @param value The bucketIds to add.
* @return This builder for chaining.
*/
public Builder addBucketIds(int value) {
ensureBucketIdsIsMutable();
bucketIds_.addInt(value);
onChanged();
return this;
}
/**
* repeated uint32 bucket_ids = 16;
* @param values The bucketIds to add.
* @return This builder for chaining.
*/
public Builder addAllBucketIds(
java.lang.Iterable extends java.lang.Integer> values) {
ensureBucketIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bucketIds_);
onChanged();
return this;
}
/**
* repeated uint32 bucket_ids = 16;
* @return This builder for chaining.
*/
public Builder clearBucketIds() {
bucketIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList indexes_ = emptyIntList();
private void ensureIndexesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
indexes_ = mutableCopy(indexes_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated int32 indexes = 17;
* @return A list containing the indexes.
*/
public java.util.List
getIndexesList() {
return ((bitField0_ & 0x00000008) != 0) ?
java.util.Collections.unmodifiableList(indexes_) : indexes_;
}
/**
* repeated int32 indexes = 17;
* @return The count of indexes.
*/
public int getIndexesCount() {
return indexes_.size();
}
/**
* repeated int32 indexes = 17;
* @param index The index of the element to return.
* @return The indexes at the given index.
*/
public int getIndexes(int index) {
return indexes_.getInt(index);
}
/**
* repeated int32 indexes = 17;
* @param index The index to set the value at.
* @param value The indexes to set.
* @return This builder for chaining.
*/
public Builder setIndexes(
int index, int value) {
ensureIndexesIsMutable();
indexes_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated int32 indexes = 17;
* @param value The indexes to add.
* @return This builder for chaining.
*/
public Builder addIndexes(int value) {
ensureIndexesIsMutable();
indexes_.addInt(value);
onChanged();
return this;
}
/**
* repeated int32 indexes = 17;
* @param values The indexes to add.
* @return This builder for chaining.
*/
public Builder addAllIndexes(
java.lang.Iterable extends java.lang.Integer> values) {
ensureIndexesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, indexes_);
onChanged();
return this;
}
/**
* repeated int32 indexes = 17;
* @return This builder for chaining.
*/
public Builder clearIndexes() {
indexes_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 18;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 18;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 18;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 19;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 19;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 19;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 19;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 19;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private cz.proto.ingestion.Ingestion.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.Account account = 20;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.Account account = 20;
* @return The account.
*/
public cz.proto.ingestion.Ingestion.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public Builder setAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public Builder setAccount(
cz.proto.ingestion.Ingestion.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public Builder mergeAccount(cz.proto.ingestion.Ingestion.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.Ingestion.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public cz.proto.ingestion.Ingestion.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
public cz.proto.ingestion.Ingestion.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.Ingestion.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.Account account = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.Ingestion.Account, cz.proto.ingestion.Ingestion.Account.Builder, cz.proto.ingestion.Ingestion.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private java.lang.Object token_ = "";
/**
* string token = 21;
* @return The token.
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string token = 21;
* @return The bytes for token.
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string token = 21;
* @param value The token to set.
* @return This builder for chaining.
*/
public Builder setToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
return this;
}
/**
* string token = 21;
* @return This builder for chaining.
*/
public Builder clearToken() {
token_ = getDefaultInstance().getToken();
onChanged();
return this;
}
/**
* string token = 21;
* @param value The bytes for token to set.
* @return This builder for chaining.
*/
public Builder setTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
token_ = value;
onChanged();
return this;
}
private java.lang.Object requestId_ = "";
/**
* string request_id = 22;
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string request_id = 22;
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string request_id = 22;
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
onChanged();
return this;
}
/**
* string request_id = 22;
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
onChanged();
return this;
}
/**
* string request_id = 22;
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
onChanged();
return this;
}
private int batchCount_ ;
/**
* int32 batch_count = 23;
* @return The batchCount.
*/
@java.lang.Override
public int getBatchCount() {
return batchCount_;
}
/**
* int32 batch_count = 23;
* @param value The batchCount to set.
* @return This builder for chaining.
*/
public Builder setBatchCount(int value) {
batchCount_ = value;
onChanged();
return this;
}
/**
* int32 batch_count = 23;
* @return This builder for chaining.
*/
public Builder clearBatchCount() {
batchCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.DataMutateRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.DataMutateRequest)
private static final cz.proto.ingestion.Ingestion.DataMutateRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.Ingestion.DataMutateRequest();
}
public static cz.proto.ingestion.Ingestion.DataMutateRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DataMutateRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataMutateRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.Ingestion.DataMutateRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataMutateRequestInternalOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.DataMutateRequestInternal)
com.google.protobuf.MessageOrBuilder {
/**
* int64 batch_id = 1;
* @return The batchId.
*/
long getBatchId();
/**
* string schema_name = 2;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 2;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 3;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 3;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
/**
* .cz.proto.Entity schema = 4;
* @return Whether the schema field is set.
*/
boolean hasSchema();
/**
* .cz.proto.Entity schema = 4;
* @return The schema.
*/
cz.proto.MetadataEntity.Entity getSchema();
/**
* .cz.proto.Entity schema = 4;
*/
cz.proto.MetadataEntity.EntityOrBuilder getSchemaOrBuilder();
/**
* .kudu.SchemaPB schema_pb = 5;
* @return Whether the schemaPb field is set.
*/
boolean hasSchemaPb();
/**
* .kudu.SchemaPB schema_pb = 5;
* @return The schemaPb.
*/
org.apache.kudu.KuduCommon.SchemaPB getSchemaPb();
/**
* .kudu.SchemaPB schema_pb = 5;
*/
org.apache.kudu.KuduCommon.SchemaPBOrBuilder getSchemaPbOrBuilder();
/**
* .cz.proto.ingestion.IGSTableType table_type = 6;
* @return The enum numeric value on the wire for tableType.
*/
int getTableTypeValue();
/**
* .cz.proto.ingestion.IGSTableType table_type = 6;
* @return The tableType.
*/
cz.proto.ingestion.Ingestion.IGSTableType getTableType();
/**
* bool is_dispatch = 7;
* @return The isDispatch.
*/
boolean getIsDispatch();
/**
* repeated int64 tablet_ids = 8;
* @return A list containing the tabletIds.
*/
java.util.List getTabletIdsList();
/**
* repeated int64 tablet_ids = 8;
* @return The count of tabletIds.
*/
int getTabletIdsCount();
/**
* repeated int64 tablet_ids = 8;
* @param index The index of the element to return.
* @return The tabletIds at the given index.
*/
long getTabletIds(int index);
/**
* int64 instance_id = 9;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 10;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 10;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string request_id = 11;
* @return The requestId.
*/
java.lang.String getRequestId();
/**
* string request_id = 11;
* @return The bytes for requestId.
*/
com.google.protobuf.ByteString
getRequestIdBytes();
/**
* repeated .kudu.RowOperationsPB dispatched_row_operations = 12;
*/
java.util.List
getDispatchedRowOperationsList();
/**
*