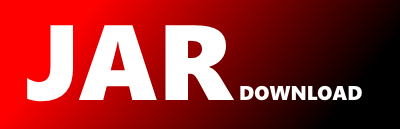
cz.proto.ingestion.v2.IngestionV2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ingestion_v2.proto
package cz.proto.ingestion.v2;
public final class IngestionV2 {
private IngestionV2() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.Code}
*/
public enum Code
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SUCCESS = 0;
*/
SUCCESS(0),
/**
* FAILED = 1;
*/
FAILED(1),
/**
* THROTTLED = 2;
*/
THROTTLED(2),
/**
* INTERNAL_ERROR = 3;
*/
INTERNAL_ERROR(3),
/**
* PRECHECK_FAILED = 4;
*/
PRECHECK_FAILED(4),
/**
* PARTIALLY_SUCCESS = 5;
*/
PARTIALLY_SUCCESS(5),
/**
* STREAM_UNAVAILABLE = 6;
*/
STREAM_UNAVAILABLE(6),
/**
* NEED_REDIRECT = 7;
*/
NEED_REDIRECT(7),
UNRECOGNIZED(-1),
;
/**
* SUCCESS = 0;
*/
public static final int SUCCESS_VALUE = 0;
/**
* FAILED = 1;
*/
public static final int FAILED_VALUE = 1;
/**
* THROTTLED = 2;
*/
public static final int THROTTLED_VALUE = 2;
/**
* INTERNAL_ERROR = 3;
*/
public static final int INTERNAL_ERROR_VALUE = 3;
/**
* PRECHECK_FAILED = 4;
*/
public static final int PRECHECK_FAILED_VALUE = 4;
/**
* PARTIALLY_SUCCESS = 5;
*/
public static final int PARTIALLY_SUCCESS_VALUE = 5;
/**
* STREAM_UNAVAILABLE = 6;
*/
public static final int STREAM_UNAVAILABLE_VALUE = 6;
/**
* NEED_REDIRECT = 7;
*/
public static final int NEED_REDIRECT_VALUE = 7;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Code valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Code forNumber(int value) {
switch (value) {
case 0: return SUCCESS;
case 1: return FAILED;
case 2: return THROTTLED;
case 3: return INTERNAL_ERROR;
case 4: return PRECHECK_FAILED;
case 5: return PARTIALLY_SUCCESS;
case 6: return STREAM_UNAVAILABLE;
case 7: return NEED_REDIRECT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Code> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Code findValueByNumber(int number) {
return Code.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.getDescriptor().getEnumTypes().get(0);
}
private static final Code[] VALUES = values();
public static Code valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Code(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.Code)
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.BulkLoadStreamState}
*/
public enum BulkLoadStreamState
implements com.google.protobuf.ProtocolMessageEnum {
/**
* BL_CREATED = 0;
*/
BL_CREATED(0),
/**
* BL_SEALED = 1;
*/
BL_SEALED(1),
/**
* BL_COMMIT_SUBMITTED = 2;
*/
BL_COMMIT_SUBMITTED(2),
/**
* BL_COMMIT_SUCCESS = 3;
*/
BL_COMMIT_SUCCESS(3),
/**
* BL_COMMIT_FAILED = 4;
*/
BL_COMMIT_FAILED(4),
/**
* BL_CANCELLED = 5;
*/
BL_CANCELLED(5),
UNRECOGNIZED(-1),
;
/**
* BL_CREATED = 0;
*/
public static final int BL_CREATED_VALUE = 0;
/**
* BL_SEALED = 1;
*/
public static final int BL_SEALED_VALUE = 1;
/**
* BL_COMMIT_SUBMITTED = 2;
*/
public static final int BL_COMMIT_SUBMITTED_VALUE = 2;
/**
* BL_COMMIT_SUCCESS = 3;
*/
public static final int BL_COMMIT_SUCCESS_VALUE = 3;
/**
* BL_COMMIT_FAILED = 4;
*/
public static final int BL_COMMIT_FAILED_VALUE = 4;
/**
* BL_CANCELLED = 5;
*/
public static final int BL_CANCELLED_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BulkLoadStreamState valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static BulkLoadStreamState forNumber(int value) {
switch (value) {
case 0: return BL_CREATED;
case 1: return BL_SEALED;
case 2: return BL_COMMIT_SUBMITTED;
case 3: return BL_COMMIT_SUCCESS;
case 4: return BL_COMMIT_FAILED;
case 5: return BL_CANCELLED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BulkLoadStreamState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BulkLoadStreamState findValueByNumber(int number) {
return BulkLoadStreamState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.getDescriptor().getEnumTypes().get(1);
}
private static final BulkLoadStreamState[] VALUES = values();
public static BulkLoadStreamState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BulkLoadStreamState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.BulkLoadStreamState)
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.BulkLoadStreamOperation}
*/
public enum BulkLoadStreamOperation
implements com.google.protobuf.ProtocolMessageEnum {
/**
* BL_APPEND = 0;
*/
BL_APPEND(0),
/**
* BL_OVERWRITE = 1;
*/
BL_OVERWRITE(1),
/**
* BL_UPSERT = 2;
*/
BL_UPSERT(2),
UNRECOGNIZED(-1),
;
/**
* BL_APPEND = 0;
*/
public static final int BL_APPEND_VALUE = 0;
/**
* BL_OVERWRITE = 1;
*/
public static final int BL_OVERWRITE_VALUE = 1;
/**
* BL_UPSERT = 2;
*/
public static final int BL_UPSERT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BulkLoadStreamOperation valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static BulkLoadStreamOperation forNumber(int value) {
switch (value) {
case 0: return BL_APPEND;
case 1: return BL_OVERWRITE;
case 2: return BL_UPSERT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BulkLoadStreamOperation> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BulkLoadStreamOperation findValueByNumber(int number) {
return BulkLoadStreamOperation.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.getDescriptor().getEnumTypes().get(2);
}
private static final BulkLoadStreamOperation[] VALUES = values();
public static BulkLoadStreamOperation valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BulkLoadStreamOperation(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.BulkLoadStreamOperation)
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.ConnectMode}
*/
public enum ConnectMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* DIRECT = 0;
*/
DIRECT(0),
/**
* GATEWAY = 1;
*/
GATEWAY(1),
/**
* GATEWAY_INTERNAL = 2;
*/
GATEWAY_INTERNAL(2),
/**
* GATEWAY_DIRECT = 3;
*/
GATEWAY_DIRECT(3),
UNRECOGNIZED(-1),
;
/**
* DIRECT = 0;
*/
public static final int DIRECT_VALUE = 0;
/**
* GATEWAY = 1;
*/
public static final int GATEWAY_VALUE = 1;
/**
* GATEWAY_INTERNAL = 2;
*/
public static final int GATEWAY_INTERNAL_VALUE = 2;
/**
* GATEWAY_DIRECT = 3;
*/
public static final int GATEWAY_DIRECT_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ConnectMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ConnectMode forNumber(int value) {
switch (value) {
case 0: return DIRECT;
case 1: return GATEWAY;
case 2: return GATEWAY_INTERNAL;
case 3: return GATEWAY_DIRECT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ConnectMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ConnectMode findValueByNumber(int number) {
return ConnectMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.getDescriptor().getEnumTypes().get(3);
}
private static final ConnectMode[] VALUES = values();
public static ConnectMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ConnectMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.ConnectMode)
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.OperationType}
*/
public enum OperationType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* INSERT = 1;
*/
INSERT(1),
/**
* UPDATE = 2;
*/
UPDATE(2),
/**
* DELETE = 3;
*/
DELETE(3),
/**
* UPSERT = 4;
*/
UPSERT(4),
/**
* INSERT_IGNORE = 5;
*/
INSERT_IGNORE(5),
/**
* UPDATE_IGNORE = 6;
*/
UPDATE_IGNORE(6),
/**
* DELETE_IGNORE = 7;
*/
DELETE_IGNORE(7),
UNRECOGNIZED(-1),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* INSERT = 1;
*/
public static final int INSERT_VALUE = 1;
/**
* UPDATE = 2;
*/
public static final int UPDATE_VALUE = 2;
/**
* DELETE = 3;
*/
public static final int DELETE_VALUE = 3;
/**
* UPSERT = 4;
*/
public static final int UPSERT_VALUE = 4;
/**
* INSERT_IGNORE = 5;
*/
public static final int INSERT_IGNORE_VALUE = 5;
/**
* UPDATE_IGNORE = 6;
*/
public static final int UPDATE_IGNORE_VALUE = 6;
/**
* DELETE_IGNORE = 7;
*/
public static final int DELETE_IGNORE_VALUE = 7;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OperationType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OperationType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return INSERT;
case 2: return UPDATE;
case 3: return DELETE;
case 4: return UPSERT;
case 5: return INSERT_IGNORE;
case 6: return UPDATE_IGNORE;
case 7: return DELETE_IGNORE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OperationType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OperationType findValueByNumber(int number) {
return OperationType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.getDescriptor().getEnumTypes().get(4);
}
private static final OperationType[] VALUES = values();
public static OperationType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OperationType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.OperationType)
}
public interface UserIdentifierOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.UserIdentifier)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string user_name = 3;
* @return The userName.
*/
java.lang.String getUserName();
/**
* string user_name = 3;
* @return The bytes for userName.
*/
com.google.protobuf.ByteString
getUserNameBytes();
/**
* int64 user_id = 4;
* @return The userId.
*/
long getUserId();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.UserIdentifier}
*/
public static final class UserIdentifier extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.UserIdentifier)
UserIdentifierOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserIdentifier.newBuilder() to construct.
private UserIdentifier(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserIdentifier() {
workspace_ = "";
userName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new UserIdentifier();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UserIdentifier(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
userName_ = s;
break;
}
case 32: {
userId_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_UserIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_UserIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.class, cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder.class);
}
public static final int INSTANCE_ID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object userName_;
/**
* string user_name = 3;
* @return The userName.
*/
@java.lang.Override
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userName_ = s;
return s;
}
}
/**
* string user_name = 3;
* @return The bytes for userName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_ID_FIELD_NUMBER = 4;
private long userId_;
/**
* int64 user_id = 4;
* @return The userId.
*/
@java.lang.Override
public long getUserId() {
return userId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, userName_);
}
if (userId_ != 0L) {
output.writeInt64(4, userId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(userName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, userName_);
}
if (userId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, userId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.UserIdentifier)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.UserIdentifier other = (cz.proto.ingestion.v2.IngestionV2.UserIdentifier) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (!getUserName()
.equals(other.getUserName())) return false;
if (getUserId()
!= other.getUserId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + USER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getUserName().hashCode();
hash = (37 * hash) + USER_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUserId());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.UserIdentifier prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.UserIdentifier}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.UserIdentifier)
cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_UserIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_UserIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.class, cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.UserIdentifier.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
workspace_ = "";
userName_ = "";
userId_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_UserIdentifier_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.UserIdentifier.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier build() {
cz.proto.ingestion.v2.IngestionV2.UserIdentifier result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier buildPartial() {
cz.proto.ingestion.v2.IngestionV2.UserIdentifier result = new cz.proto.ingestion.v2.IngestionV2.UserIdentifier(this);
result.instanceId_ = instanceId_;
result.workspace_ = workspace_;
result.userName_ = userName_;
result.userId_ = userId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.UserIdentifier) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.UserIdentifier)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.UserIdentifier other) {
if (other == cz.proto.ingestion.v2.IngestionV2.UserIdentifier.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (!other.getUserName().isEmpty()) {
userName_ = other.userName_;
onChanged();
}
if (other.getUserId() != 0L) {
setUserId(other.getUserId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.UserIdentifier parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.UserIdentifier) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private java.lang.Object userName_ = "";
/**
* string user_name = 3;
* @return The userName.
*/
public java.lang.String getUserName() {
java.lang.Object ref = userName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string user_name = 3;
* @return The bytes for userName.
*/
public com.google.protobuf.ByteString
getUserNameBytes() {
java.lang.Object ref = userName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string user_name = 3;
* @param value The userName to set.
* @return This builder for chaining.
*/
public Builder setUserName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userName_ = value;
onChanged();
return this;
}
/**
* string user_name = 3;
* @return This builder for chaining.
*/
public Builder clearUserName() {
userName_ = getDefaultInstance().getUserName();
onChanged();
return this;
}
/**
* string user_name = 3;
* @param value The bytes for userName to set.
* @return This builder for chaining.
*/
public Builder setUserNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userName_ = value;
onChanged();
return this;
}
private long userId_ ;
/**
* int64 user_id = 4;
* @return The userId.
*/
@java.lang.Override
public long getUserId() {
return userId_;
}
/**
* int64 user_id = 4;
* @param value The userId to set.
* @return This builder for chaining.
*/
public Builder setUserId(long value) {
userId_ = value;
onChanged();
return this;
}
/**
* int64 user_id = 4;
* @return This builder for chaining.
*/
public Builder clearUserId() {
userId_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.UserIdentifier)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.UserIdentifier)
private static final cz.proto.ingestion.v2.IngestionV2.UserIdentifier DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.UserIdentifier();
}
public static cz.proto.ingestion.v2.IngestionV2.UserIdentifier getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserIdentifier parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UserIdentifier(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccountOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.Account)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return Whether the userIdent field is set.
*/
boolean hasUserIdent();
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return The userIdent.
*/
cz.proto.ingestion.v2.IngestionV2.UserIdentifier getUserIdent();
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder getUserIdentOrBuilder();
/**
* string token = 2;
* @return The token.
*/
java.lang.String getToken();
/**
* string token = 2;
* @return The bytes for token.
*/
com.google.protobuf.ByteString
getTokenBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.Account}
*/
public static final class Account extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.Account)
AccountOrBuilder {
private static final long serialVersionUID = 0L;
// Use Account.newBuilder() to construct.
private Account(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Account() {
token_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Account();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Account(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder subBuilder = null;
if (userIdent_ != null) {
subBuilder = userIdent_.toBuilder();
}
userIdent_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.UserIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(userIdent_);
userIdent_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
token_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_Account_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_Account_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.Account.class, cz.proto.ingestion.v2.IngestionV2.Account.Builder.class);
}
public static final int USER_IDENT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.UserIdentifier userIdent_;
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return Whether the userIdent field is set.
*/
@java.lang.Override
public boolean hasUserIdent() {
return userIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return The userIdent.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier getUserIdent() {
return userIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.UserIdentifier.getDefaultInstance() : userIdent_;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder getUserIdentOrBuilder() {
return getUserIdent();
}
public static final int TOKEN_FIELD_NUMBER = 2;
private volatile java.lang.Object token_;
/**
* string token = 2;
* @return The token.
*/
@java.lang.Override
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
}
}
/**
* string token = 2;
* @return The bytes for token.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (userIdent_ != null) {
output.writeMessage(1, getUserIdent());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(token_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, token_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (userIdent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getUserIdent());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(token_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, token_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.Account)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.Account other = (cz.proto.ingestion.v2.IngestionV2.Account) obj;
if (hasUserIdent() != other.hasUserIdent()) return false;
if (hasUserIdent()) {
if (!getUserIdent()
.equals(other.getUserIdent())) return false;
}
if (!getToken()
.equals(other.getToken())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUserIdent()) {
hash = (37 * hash) + USER_IDENT_FIELD_NUMBER;
hash = (53 * hash) + getUserIdent().hashCode();
}
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.Account parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.Account prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.Account}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.Account)
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_Account_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_Account_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.Account.class, cz.proto.ingestion.v2.IngestionV2.Account.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.Account.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (userIdentBuilder_ == null) {
userIdent_ = null;
} else {
userIdent_ = null;
userIdentBuilder_ = null;
}
token_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_Account_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account build() {
cz.proto.ingestion.v2.IngestionV2.Account result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account buildPartial() {
cz.proto.ingestion.v2.IngestionV2.Account result = new cz.proto.ingestion.v2.IngestionV2.Account(this);
if (userIdentBuilder_ == null) {
result.userIdent_ = userIdent_;
} else {
result.userIdent_ = userIdentBuilder_.build();
}
result.token_ = token_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.Account) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.Account)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.Account other) {
if (other == cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance()) return this;
if (other.hasUserIdent()) {
mergeUserIdent(other.getUserIdent());
}
if (!other.getToken().isEmpty()) {
token_ = other.token_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.Account parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.Account) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.UserIdentifier userIdent_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.UserIdentifier, cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder> userIdentBuilder_;
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return Whether the userIdent field is set.
*/
public boolean hasUserIdent() {
return userIdentBuilder_ != null || userIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
* @return The userIdent.
*/
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier getUserIdent() {
if (userIdentBuilder_ == null) {
return userIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.UserIdentifier.getDefaultInstance() : userIdent_;
} else {
return userIdentBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public Builder setUserIdent(cz.proto.ingestion.v2.IngestionV2.UserIdentifier value) {
if (userIdentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userIdent_ = value;
onChanged();
} else {
userIdentBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public Builder setUserIdent(
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder builderForValue) {
if (userIdentBuilder_ == null) {
userIdent_ = builderForValue.build();
onChanged();
} else {
userIdentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public Builder mergeUserIdent(cz.proto.ingestion.v2.IngestionV2.UserIdentifier value) {
if (userIdentBuilder_ == null) {
if (userIdent_ != null) {
userIdent_ =
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.newBuilder(userIdent_).mergeFrom(value).buildPartial();
} else {
userIdent_ = value;
}
onChanged();
} else {
userIdentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public Builder clearUserIdent() {
if (userIdentBuilder_ == null) {
userIdent_ = null;
onChanged();
} else {
userIdent_ = null;
userIdentBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder getUserIdentBuilder() {
onChanged();
return getUserIdentFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder getUserIdentOrBuilder() {
if (userIdentBuilder_ != null) {
return userIdentBuilder_.getMessageOrBuilder();
} else {
return userIdent_ == null ?
cz.proto.ingestion.v2.IngestionV2.UserIdentifier.getDefaultInstance() : userIdent_;
}
}
/**
* .cz.proto.ingestion.v2.UserIdentifier user_ident = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.UserIdentifier, cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder>
getUserIdentFieldBuilder() {
if (userIdentBuilder_ == null) {
userIdentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.UserIdentifier, cz.proto.ingestion.v2.IngestionV2.UserIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.UserIdentifierOrBuilder>(
getUserIdent(),
getParentForChildren(),
isClean());
userIdent_ = null;
}
return userIdentBuilder_;
}
private java.lang.Object token_ = "";
/**
* string token = 2;
* @return The token.
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string token = 2;
* @return The bytes for token.
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string token = 2;
* @param value The token to set.
* @return This builder for chaining.
*/
public Builder setToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
token_ = value;
onChanged();
return this;
}
/**
* string token = 2;
* @return This builder for chaining.
*/
public Builder clearToken() {
token_ = getDefaultInstance().getToken();
onChanged();
return this;
}
/**
* string token = 2;
* @param value The bytes for token to set.
* @return This builder for chaining.
*/
public Builder setTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
token_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.Account)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.Account)
private static final cz.proto.ingestion.v2.IngestionV2.Account DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.Account();
}
public static cz.proto.ingestion.v2.IngestionV2.Account getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Account parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Account(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TableIdentifierOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.TableIdentifier)
com.google.protobuf.MessageOrBuilder {
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
long getInstanceId();
/**
* string workspace = 2;
* @return The workspace.
*/
java.lang.String getWorkspace();
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
com.google.protobuf.ByteString
getWorkspaceBytes();
/**
* string schema_name = 3;
* @return The schemaName.
*/
java.lang.String getSchemaName();
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
com.google.protobuf.ByteString
getSchemaNameBytes();
/**
* string table_name = 4;
* @return The tableName.
*/
java.lang.String getTableName();
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
com.google.protobuf.ByteString
getTableNameBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.TableIdentifier}
*/
public static final class TableIdentifier extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.TableIdentifier)
TableIdentifierOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableIdentifier.newBuilder() to construct.
private TableIdentifier(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableIdentifier() {
workspace_ = "";
schemaName_ = "";
tableName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableIdentifier();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableIdentifier(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
instanceId_ = input.readInt64();
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
workspace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
schemaName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tableName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_TableIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_TableIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.class, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder.class);
}
public static final int INSTANCE_ID_FIELD_NUMBER = 1;
private long instanceId_;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
public static final int WORKSPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object workspace_;
/**
* string workspace = 2;
* @return The workspace.
*/
@java.lang.Override
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEMA_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object schemaName_;
/**
* string schema_name = 3;
* @return The schemaName.
*/
@java.lang.Override
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TABLE_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object tableName_;
/**
* string table_name = 4;
* @return The tableName.
*/
@java.lang.Override
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (instanceId_ != 0L) {
output.writeInt64(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tableName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (instanceId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, instanceId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(workspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, workspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schemaName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, schemaName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tableName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tableName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.TableIdentifier)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.TableIdentifier other = (cz.proto.ingestion.v2.IngestionV2.TableIdentifier) obj;
if (getInstanceId()
!= other.getInstanceId()) return false;
if (!getWorkspace()
.equals(other.getWorkspace())) return false;
if (!getSchemaName()
.equals(other.getSchemaName())) return false;
if (!getTableName()
.equals(other.getTableName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + INSTANCE_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInstanceId());
hash = (37 * hash) + WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getWorkspace().hashCode();
hash = (37 * hash) + SCHEMA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSchemaName().hashCode();
hash = (37 * hash) + TABLE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getTableName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.TableIdentifier prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.TableIdentifier}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.TableIdentifier)
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_TableIdentifier_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_TableIdentifier_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.class, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
instanceId_ = 0L;
workspace_ = "";
schemaName_ = "";
tableName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_TableIdentifier_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier build() {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier buildPartial() {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier result = new cz.proto.ingestion.v2.IngestionV2.TableIdentifier(this);
result.instanceId_ = instanceId_;
result.workspace_ = workspace_;
result.schemaName_ = schemaName_;
result.tableName_ = tableName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.TableIdentifier) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.TableIdentifier)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.TableIdentifier other) {
if (other == cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance()) return this;
if (other.getInstanceId() != 0L) {
setInstanceId(other.getInstanceId());
}
if (!other.getWorkspace().isEmpty()) {
workspace_ = other.workspace_;
onChanged();
}
if (!other.getSchemaName().isEmpty()) {
schemaName_ = other.schemaName_;
onChanged();
}
if (!other.getTableName().isEmpty()) {
tableName_ = other.tableName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.TableIdentifier) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long instanceId_ ;
/**
* int64 instance_id = 1;
* @return The instanceId.
*/
@java.lang.Override
public long getInstanceId() {
return instanceId_;
}
/**
* int64 instance_id = 1;
* @param value The instanceId to set.
* @return This builder for chaining.
*/
public Builder setInstanceId(long value) {
instanceId_ = value;
onChanged();
return this;
}
/**
* int64 instance_id = 1;
* @return This builder for chaining.
*/
public Builder clearInstanceId() {
instanceId_ = 0L;
onChanged();
return this;
}
private java.lang.Object workspace_ = "";
/**
* string workspace = 2;
* @return The workspace.
*/
public java.lang.String getWorkspace() {
java.lang.Object ref = workspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
workspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string workspace = 2;
* @return The bytes for workspace.
*/
public com.google.protobuf.ByteString
getWorkspaceBytes() {
java.lang.Object ref = workspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
workspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string workspace = 2;
* @param value The workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
workspace_ = value;
onChanged();
return this;
}
/**
* string workspace = 2;
* @return This builder for chaining.
*/
public Builder clearWorkspace() {
workspace_ = getDefaultInstance().getWorkspace();
onChanged();
return this;
}
/**
* string workspace = 2;
* @param value The bytes for workspace to set.
* @return This builder for chaining.
*/
public Builder setWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
workspace_ = value;
onChanged();
return this;
}
private java.lang.Object schemaName_ = "";
/**
* string schema_name = 3;
* @return The schemaName.
*/
public java.lang.String getSchemaName() {
java.lang.Object ref = schemaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schemaName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string schema_name = 3;
* @return The bytes for schemaName.
*/
public com.google.protobuf.ByteString
getSchemaNameBytes() {
java.lang.Object ref = schemaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schemaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string schema_name = 3;
* @param value The schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schemaName_ = value;
onChanged();
return this;
}
/**
* string schema_name = 3;
* @return This builder for chaining.
*/
public Builder clearSchemaName() {
schemaName_ = getDefaultInstance().getSchemaName();
onChanged();
return this;
}
/**
* string schema_name = 3;
* @param value The bytes for schemaName to set.
* @return This builder for chaining.
*/
public Builder setSchemaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schemaName_ = value;
onChanged();
return this;
}
private java.lang.Object tableName_ = "";
/**
* string table_name = 4;
* @return The tableName.
*/
public java.lang.String getTableName() {
java.lang.Object ref = tableName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tableName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string table_name = 4;
* @return The bytes for tableName.
*/
public com.google.protobuf.ByteString
getTableNameBytes() {
java.lang.Object ref = tableName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tableName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string table_name = 4;
* @param value The tableName to set.
* @return This builder for chaining.
*/
public Builder setTableName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tableName_ = value;
onChanged();
return this;
}
/**
* string table_name = 4;
* @return This builder for chaining.
*/
public Builder clearTableName() {
tableName_ = getDefaultInstance().getTableName();
onChanged();
return this;
}
/**
* string table_name = 4;
* @param value The bytes for tableName to set.
* @return This builder for chaining.
*/
public Builder setTableNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tableName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.TableIdentifier)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.TableIdentifier)
private static final cz.proto.ingestion.v2.IngestionV2.TableIdentifier DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.TableIdentifier();
}
public static cz.proto.ingestion.v2.IngestionV2.TableIdentifier getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableIdentifier parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableIdentifier(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ResponseStatusOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.ResponseStatus)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The enum numeric value on the wire for code.
*/
int getCodeValue();
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The code.
*/
cz.proto.ingestion.v2.IngestionV2.Code getCode();
/**
* string error_message = 2;
* @return The errorMessage.
*/
java.lang.String getErrorMessage();
/**
* string error_message = 2;
* @return The bytes for errorMessage.
*/
com.google.protobuf.ByteString
getErrorMessageBytes();
/**
* string request_id = 3;
* @return The requestId.
*/
java.lang.String getRequestId();
/**
* string request_id = 3;
* @return The bytes for requestId.
*/
com.google.protobuf.ByteString
getRequestIdBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.ResponseStatus}
*/
public static final class ResponseStatus extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.ResponseStatus)
ResponseStatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use ResponseStatus.newBuilder() to construct.
private ResponseStatus(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ResponseStatus() {
code_ = 0;
errorMessage_ = "";
requestId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ResponseStatus();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResponseStatus(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
code_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
errorMessage_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
requestId_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_ResponseStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_ResponseStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.class, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder.class);
}
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The enum numeric value on the wire for code.
*/
@java.lang.Override public int getCodeValue() {
return code_;
}
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The code.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.Code getCode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.Code result = cz.proto.ingestion.v2.IngestionV2.Code.valueOf(code_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.Code.UNRECOGNIZED : result;
}
public static final int ERROR_MESSAGE_FIELD_NUMBER = 2;
private volatile java.lang.Object errorMessage_;
/**
* string error_message = 2;
* @return The errorMessage.
*/
@java.lang.Override
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
}
}
/**
* string error_message = 2;
* @return The bytes for errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object requestId_;
/**
* string request_id = 3;
* @return The requestId.
*/
@java.lang.Override
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
}
}
/**
* string request_id = 3;
* @return The bytes for requestId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (code_ != cz.proto.ingestion.v2.IngestionV2.Code.SUCCESS.getNumber()) {
output.writeEnum(1, code_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(errorMessage_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, errorMessage_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, requestId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (code_ != cz.proto.ingestion.v2.IngestionV2.Code.SUCCESS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, code_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(errorMessage_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, errorMessage_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(requestId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, requestId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.ResponseStatus)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.ResponseStatus other = (cz.proto.ingestion.v2.IngestionV2.ResponseStatus) obj;
if (code_ != other.code_) return false;
if (!getErrorMessage()
.equals(other.getErrorMessage())) return false;
if (!getRequestId()
.equals(other.getRequestId())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + code_;
hash = (37 * hash) + ERROR_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
hash = (37 * hash) + REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.ResponseStatus prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.ResponseStatus}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.ResponseStatus)
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_ResponseStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_ResponseStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.class, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
code_ = 0;
errorMessage_ = "";
requestId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_ResponseStatus_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus build() {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus buildPartial() {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus result = new cz.proto.ingestion.v2.IngestionV2.ResponseStatus(this);
result.code_ = code_;
result.errorMessage_ = errorMessage_;
result.requestId_ = requestId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.ResponseStatus) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.ResponseStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.ResponseStatus other) {
if (other == cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance()) return this;
if (other.code_ != 0) {
setCodeValue(other.getCodeValue());
}
if (!other.getErrorMessage().isEmpty()) {
errorMessage_ = other.errorMessage_;
onChanged();
}
if (!other.getRequestId().isEmpty()) {
requestId_ = other.requestId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.ResponseStatus) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int code_ = 0;
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The enum numeric value on the wire for code.
*/
@java.lang.Override public int getCodeValue() {
return code_;
}
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @param value The enum numeric value on the wire for code to set.
* @return This builder for chaining.
*/
public Builder setCodeValue(int value) {
code_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return The code.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Code getCode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.Code result = cz.proto.ingestion.v2.IngestionV2.Code.valueOf(code_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.Code.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @param value The code to set.
* @return This builder for chaining.
*/
public Builder setCode(cz.proto.ingestion.v2.IngestionV2.Code value) {
if (value == null) {
throw new NullPointerException();
}
code_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.Code code = 1;
* @return This builder for chaining.
*/
public Builder clearCode() {
code_ = 0;
onChanged();
return this;
}
private java.lang.Object errorMessage_ = "";
/**
* string error_message = 2;
* @return The errorMessage.
*/
public java.lang.String getErrorMessage() {
java.lang.Object ref = errorMessage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorMessage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string error_message = 2;
* @return The bytes for errorMessage.
*/
public com.google.protobuf.ByteString
getErrorMessageBytes() {
java.lang.Object ref = errorMessage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorMessage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string error_message = 2;
* @param value The errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorMessage_ = value;
onChanged();
return this;
}
/**
* string error_message = 2;
* @return This builder for chaining.
*/
public Builder clearErrorMessage() {
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
/**
* string error_message = 2;
* @param value The bytes for errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorMessage_ = value;
onChanged();
return this;
}
private java.lang.Object requestId_ = "";
/**
* string request_id = 3;
* @return The requestId.
*/
public java.lang.String getRequestId() {
java.lang.Object ref = requestId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string request_id = 3;
* @return The bytes for requestId.
*/
public com.google.protobuf.ByteString
getRequestIdBytes() {
java.lang.Object ref = requestId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string request_id = 3;
* @param value The requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestId_ = value;
onChanged();
return this;
}
/**
* string request_id = 3;
* @return This builder for chaining.
*/
public Builder clearRequestId() {
requestId_ = getDefaultInstance().getRequestId();
onChanged();
return this;
}
/**
* string request_id = 3;
* @param value The bytes for requestId to set.
* @return This builder for chaining.
*/
public Builder setRequestIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.ResponseStatus)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.ResponseStatus)
private static final cz.proto.ingestion.v2.IngestionV2.ResponseStatus DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.ResponseStatus();
}
public static cz.proto.ingestion.v2.IngestionV2.ResponseStatus getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ResponseStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResponseStatus(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataFieldOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.DataField)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* .cz.proto.DataType type = 2;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* .cz.proto.DataType type = 2;
* @return The type.
*/
cz.proto.DataType getType();
/**
* .cz.proto.DataType type = 2;
*/
cz.proto.DataTypeOrBuilder getTypeOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.DataField}
*/
public static final class DataField extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.DataField)
DataFieldOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataField.newBuilder() to construct.
private DataField(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataField() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DataField();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataField(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
cz.proto.DataType.Builder subBuilder = null;
if (type_ != null) {
subBuilder = type_.toBuilder();
}
type_ = input.readMessage(cz.proto.DataType.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(type_);
type_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DataField_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DataField_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.DataField.class, cz.proto.ingestion.v2.IngestionV2.DataField.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
private cz.proto.DataType type_;
/**
* .cz.proto.DataType type = 2;
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return type_ != null;
}
/**
* .cz.proto.DataType type = 2;
* @return The type.
*/
@java.lang.Override
public cz.proto.DataType getType() {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
}
/**
* .cz.proto.DataType type = 2;
*/
@java.lang.Override
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
return getType();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (type_ != null) {
output.writeMessage(2, getType());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (type_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getType());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.DataField)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.DataField other = (cz.proto.ingestion.v2.IngestionV2.DataField) obj;
if (!getName()
.equals(other.getName())) return false;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType()
.equals(other.getType())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DataField parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.DataField prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.DataField}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.DataField)
cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DataField_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DataField_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.DataField.class, cz.proto.ingestion.v2.IngestionV2.DataField.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.DataField.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
if (typeBuilder_ == null) {
type_ = null;
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DataField_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataField getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.DataField.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataField build() {
cz.proto.ingestion.v2.IngestionV2.DataField result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataField buildPartial() {
cz.proto.ingestion.v2.IngestionV2.DataField result = new cz.proto.ingestion.v2.IngestionV2.DataField(this);
result.name_ = name_;
if (typeBuilder_ == null) {
result.type_ = type_;
} else {
result.type_ = typeBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.DataField) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.DataField)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.DataField other) {
if (other == cz.proto.ingestion.v2.IngestionV2.DataField.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
mergeType(other.getType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.DataField parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.DataField) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private cz.proto.DataType type_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder> typeBuilder_;
/**
* .cz.proto.DataType type = 2;
* @return Whether the type field is set.
*/
public boolean hasType() {
return typeBuilder_ != null || type_ != null;
}
/**
* .cz.proto.DataType type = 2;
* @return The type.
*/
public cz.proto.DataType getType() {
if (typeBuilder_ == null) {
return type_ == null ? cz.proto.DataType.getDefaultInstance() : type_;
} else {
return typeBuilder_.getMessage();
}
}
/**
* .cz.proto.DataType type = 2;
*/
public Builder setType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
} else {
typeBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.DataType type = 2;
*/
public Builder setType(
cz.proto.DataType.Builder builderForValue) {
if (typeBuilder_ == null) {
type_ = builderForValue.build();
onChanged();
} else {
typeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.DataType type = 2;
*/
public Builder mergeType(cz.proto.DataType value) {
if (typeBuilder_ == null) {
if (type_ != null) {
type_ =
cz.proto.DataType.newBuilder(type_).mergeFrom(value).buildPartial();
} else {
type_ = value;
}
onChanged();
} else {
typeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.DataType type = 2;
*/
public Builder clearType() {
if (typeBuilder_ == null) {
type_ = null;
onChanged();
} else {
type_ = null;
typeBuilder_ = null;
}
return this;
}
/**
* .cz.proto.DataType type = 2;
*/
public cz.proto.DataType.Builder getTypeBuilder() {
onChanged();
return getTypeFieldBuilder().getBuilder();
}
/**
* .cz.proto.DataType type = 2;
*/
public cz.proto.DataTypeOrBuilder getTypeOrBuilder() {
if (typeBuilder_ != null) {
return typeBuilder_.getMessageOrBuilder();
} else {
return type_ == null ?
cz.proto.DataType.getDefaultInstance() : type_;
}
}
/**
* .cz.proto.DataType type = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>
getTypeFieldBuilder() {
if (typeBuilder_ == null) {
typeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.DataType, cz.proto.DataType.Builder, cz.proto.DataTypeOrBuilder>(
getType(),
getParentForChildren(),
isClean());
type_ = null;
}
return typeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.DataField)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.DataField)
private static final cz.proto.ingestion.v2.IngestionV2.DataField DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.DataField();
}
public static cz.proto.ingestion.v2.IngestionV2.DataField getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DataField parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataField(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataField getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DistributionSpecOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.DistributionSpec)
com.google.protobuf.MessageOrBuilder {
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
java.util.List getFieldIdsList();
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
int getFieldIdsCount();
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
int getFieldIds(int index);
/**
* repeated string hash_functions = 2;
* @return A list containing the hashFunctions.
*/
java.util.List
getHashFunctionsList();
/**
* repeated string hash_functions = 2;
* @return The count of hashFunctions.
*/
int getHashFunctionsCount();
/**
* repeated string hash_functions = 2;
* @param index The index of the element to return.
* @return The hashFunctions at the given index.
*/
java.lang.String getHashFunctions(int index);
/**
* repeated string hash_functions = 2;
* @param index The index of the value to return.
* @return The bytes of the hashFunctions at the given index.
*/
com.google.protobuf.ByteString
getHashFunctionsBytes(int index);
/**
* uint32 num_buckets = 3;
* @return The numBuckets.
*/
int getNumBuckets();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.DistributionSpec}
*/
public static final class DistributionSpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.DistributionSpec)
DistributionSpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use DistributionSpec.newBuilder() to construct.
private DistributionSpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DistributionSpec() {
fieldIds_ = emptyIntList();
hashFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DistributionSpec();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DistributionSpec(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
fieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
fieldIds_.addInt(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
fieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
fieldIds_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
hashFunctions_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
hashFunctions_.add(s);
break;
}
case 24: {
numBuckets_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
fieldIds_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
hashFunctions_ = hashFunctions_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DistributionSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DistributionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.class, cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder.class);
}
public static final int FIELD_IDS_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.IntList fieldIds_;
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
@java.lang.Override
public java.util.List
getFieldIdsList() {
return fieldIds_;
}
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
public int getFieldIdsCount() {
return fieldIds_.size();
}
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
public int getFieldIds(int index) {
return fieldIds_.getInt(index);
}
private int fieldIdsMemoizedSerializedSize = -1;
public static final int HASH_FUNCTIONS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList hashFunctions_;
/**
* repeated string hash_functions = 2;
* @return A list containing the hashFunctions.
*/
public com.google.protobuf.ProtocolStringList
getHashFunctionsList() {
return hashFunctions_;
}
/**
* repeated string hash_functions = 2;
* @return The count of hashFunctions.
*/
public int getHashFunctionsCount() {
return hashFunctions_.size();
}
/**
* repeated string hash_functions = 2;
* @param index The index of the element to return.
* @return The hashFunctions at the given index.
*/
public java.lang.String getHashFunctions(int index) {
return hashFunctions_.get(index);
}
/**
* repeated string hash_functions = 2;
* @param index The index of the value to return.
* @return The bytes of the hashFunctions at the given index.
*/
public com.google.protobuf.ByteString
getHashFunctionsBytes(int index) {
return hashFunctions_.getByteString(index);
}
public static final int NUM_BUCKETS_FIELD_NUMBER = 3;
private int numBuckets_;
/**
* uint32 num_buckets = 3;
* @return The numBuckets.
*/
@java.lang.Override
public int getNumBuckets() {
return numBuckets_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getFieldIdsList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(fieldIdsMemoizedSerializedSize);
}
for (int i = 0; i < fieldIds_.size(); i++) {
output.writeUInt32NoTag(fieldIds_.getInt(i));
}
for (int i = 0; i < hashFunctions_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, hashFunctions_.getRaw(i));
}
if (numBuckets_ != 0) {
output.writeUInt32(3, numBuckets_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < fieldIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(fieldIds_.getInt(i));
}
size += dataSize;
if (!getFieldIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
fieldIdsMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < hashFunctions_.size(); i++) {
dataSize += computeStringSizeNoTag(hashFunctions_.getRaw(i));
}
size += dataSize;
size += 1 * getHashFunctionsList().size();
}
if (numBuckets_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, numBuckets_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.DistributionSpec)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.DistributionSpec other = (cz.proto.ingestion.v2.IngestionV2.DistributionSpec) obj;
if (!getFieldIdsList()
.equals(other.getFieldIdsList())) return false;
if (!getHashFunctionsList()
.equals(other.getHashFunctionsList())) return false;
if (getNumBuckets()
!= other.getNumBuckets()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFieldIdsCount() > 0) {
hash = (37 * hash) + FIELD_IDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldIdsList().hashCode();
}
if (getHashFunctionsCount() > 0) {
hash = (37 * hash) + HASH_FUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + getHashFunctionsList().hashCode();
}
hash = (37 * hash) + NUM_BUCKETS_FIELD_NUMBER;
hash = (53 * hash) + getNumBuckets();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.DistributionSpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.DistributionSpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.DistributionSpec)
cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DistributionSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DistributionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.class, cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.DistributionSpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
fieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
hashFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
numBuckets_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_DistributionSpec_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.DistributionSpec.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec build() {
cz.proto.ingestion.v2.IngestionV2.DistributionSpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec buildPartial() {
cz.proto.ingestion.v2.IngestionV2.DistributionSpec result = new cz.proto.ingestion.v2.IngestionV2.DistributionSpec(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
fieldIds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fieldIds_ = fieldIds_;
if (((bitField0_ & 0x00000002) != 0)) {
hashFunctions_ = hashFunctions_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.hashFunctions_ = hashFunctions_;
result.numBuckets_ = numBuckets_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.DistributionSpec) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.DistributionSpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.DistributionSpec other) {
if (other == cz.proto.ingestion.v2.IngestionV2.DistributionSpec.getDefaultInstance()) return this;
if (!other.fieldIds_.isEmpty()) {
if (fieldIds_.isEmpty()) {
fieldIds_ = other.fieldIds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldIdsIsMutable();
fieldIds_.addAll(other.fieldIds_);
}
onChanged();
}
if (!other.hashFunctions_.isEmpty()) {
if (hashFunctions_.isEmpty()) {
hashFunctions_ = other.hashFunctions_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureHashFunctionsIsMutable();
hashFunctions_.addAll(other.hashFunctions_);
}
onChanged();
}
if (other.getNumBuckets() != 0) {
setNumBuckets(other.getNumBuckets());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.DistributionSpec parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.DistributionSpec) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList fieldIds_ = emptyIntList();
private void ensureFieldIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fieldIds_ = mutableCopy(fieldIds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
public java.util.List
getFieldIdsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(fieldIds_) : fieldIds_;
}
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
public int getFieldIdsCount() {
return fieldIds_.size();
}
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
public int getFieldIds(int index) {
return fieldIds_.getInt(index);
}
/**
* repeated uint32 field_ids = 1;
* @param index The index to set the value at.
* @param value The fieldIds to set.
* @return This builder for chaining.
*/
public Builder setFieldIds(
int index, int value) {
ensureFieldIdsIsMutable();
fieldIds_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @param value The fieldIds to add.
* @return This builder for chaining.
*/
public Builder addFieldIds(int value) {
ensureFieldIdsIsMutable();
fieldIds_.addInt(value);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @param values The fieldIds to add.
* @return This builder for chaining.
*/
public Builder addAllFieldIds(
java.lang.Iterable extends java.lang.Integer> values) {
ensureFieldIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fieldIds_);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @return This builder for chaining.
*/
public Builder clearFieldIds() {
fieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList hashFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureHashFunctionsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
hashFunctions_ = new com.google.protobuf.LazyStringArrayList(hashFunctions_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string hash_functions = 2;
* @return A list containing the hashFunctions.
*/
public com.google.protobuf.ProtocolStringList
getHashFunctionsList() {
return hashFunctions_.getUnmodifiableView();
}
/**
* repeated string hash_functions = 2;
* @return The count of hashFunctions.
*/
public int getHashFunctionsCount() {
return hashFunctions_.size();
}
/**
* repeated string hash_functions = 2;
* @param index The index of the element to return.
* @return The hashFunctions at the given index.
*/
public java.lang.String getHashFunctions(int index) {
return hashFunctions_.get(index);
}
/**
* repeated string hash_functions = 2;
* @param index The index of the value to return.
* @return The bytes of the hashFunctions at the given index.
*/
public com.google.protobuf.ByteString
getHashFunctionsBytes(int index) {
return hashFunctions_.getByteString(index);
}
/**
* repeated string hash_functions = 2;
* @param index The index to set the value at.
* @param value The hashFunctions to set.
* @return This builder for chaining.
*/
public Builder setHashFunctions(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHashFunctionsIsMutable();
hashFunctions_.set(index, value);
onChanged();
return this;
}
/**
* repeated string hash_functions = 2;
* @param value The hashFunctions to add.
* @return This builder for chaining.
*/
public Builder addHashFunctions(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureHashFunctionsIsMutable();
hashFunctions_.add(value);
onChanged();
return this;
}
/**
* repeated string hash_functions = 2;
* @param values The hashFunctions to add.
* @return This builder for chaining.
*/
public Builder addAllHashFunctions(
java.lang.Iterable values) {
ensureHashFunctionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hashFunctions_);
onChanged();
return this;
}
/**
* repeated string hash_functions = 2;
* @return This builder for chaining.
*/
public Builder clearHashFunctions() {
hashFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string hash_functions = 2;
* @param value The bytes of the hashFunctions to add.
* @return This builder for chaining.
*/
public Builder addHashFunctionsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureHashFunctionsIsMutable();
hashFunctions_.add(value);
onChanged();
return this;
}
private int numBuckets_ ;
/**
* uint32 num_buckets = 3;
* @return The numBuckets.
*/
@java.lang.Override
public int getNumBuckets() {
return numBuckets_;
}
/**
* uint32 num_buckets = 3;
* @param value The numBuckets to set.
* @return This builder for chaining.
*/
public Builder setNumBuckets(int value) {
numBuckets_ = value;
onChanged();
return this;
}
/**
* uint32 num_buckets = 3;
* @return This builder for chaining.
*/
public Builder clearNumBuckets() {
numBuckets_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.DistributionSpec)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.DistributionSpec)
private static final cz.proto.ingestion.v2.IngestionV2.DistributionSpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.DistributionSpec();
}
public static cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DistributionSpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DistributionSpec(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PrimaryKeySpecOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.PrimaryKeySpec)
com.google.protobuf.MessageOrBuilder {
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
java.util.List getFieldIdsList();
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
int getFieldIdsCount();
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
int getFieldIds(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.PrimaryKeySpec}
*/
public static final class PrimaryKeySpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.PrimaryKeySpec)
PrimaryKeySpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use PrimaryKeySpec.newBuilder() to construct.
private PrimaryKeySpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PrimaryKeySpec() {
fieldIds_ = emptyIntList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PrimaryKeySpec();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PrimaryKeySpec(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
fieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
fieldIds_.addInt(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
fieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
fieldIds_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
fieldIds_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PrimaryKeySpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PrimaryKeySpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.class, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder.class);
}
public static final int FIELD_IDS_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.IntList fieldIds_;
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
@java.lang.Override
public java.util.List
getFieldIdsList() {
return fieldIds_;
}
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
public int getFieldIdsCount() {
return fieldIds_.size();
}
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
public int getFieldIds(int index) {
return fieldIds_.getInt(index);
}
private int fieldIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getFieldIdsList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(fieldIdsMemoizedSerializedSize);
}
for (int i = 0; i < fieldIds_.size(); i++) {
output.writeUInt32NoTag(fieldIds_.getInt(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < fieldIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(fieldIds_.getInt(i));
}
size += dataSize;
if (!getFieldIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
fieldIdsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec other = (cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec) obj;
if (!getFieldIdsList()
.equals(other.getFieldIdsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFieldIdsCount() > 0) {
hash = (37 * hash) + FIELD_IDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.PrimaryKeySpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.PrimaryKeySpec)
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PrimaryKeySpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PrimaryKeySpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.class, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
fieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PrimaryKeySpec_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec build() {
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec buildPartial() {
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec result = new cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
fieldIds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fieldIds_ = fieldIds_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec other) {
if (other == cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.getDefaultInstance()) return this;
if (!other.fieldIds_.isEmpty()) {
if (fieldIds_.isEmpty()) {
fieldIds_ = other.fieldIds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldIdsIsMutable();
fieldIds_.addAll(other.fieldIds_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList fieldIds_ = emptyIntList();
private void ensureFieldIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fieldIds_ = mutableCopy(fieldIds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint32 field_ids = 1;
* @return A list containing the fieldIds.
*/
public java.util.List
getFieldIdsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(fieldIds_) : fieldIds_;
}
/**
* repeated uint32 field_ids = 1;
* @return The count of fieldIds.
*/
public int getFieldIdsCount() {
return fieldIds_.size();
}
/**
* repeated uint32 field_ids = 1;
* @param index The index of the element to return.
* @return The fieldIds at the given index.
*/
public int getFieldIds(int index) {
return fieldIds_.getInt(index);
}
/**
* repeated uint32 field_ids = 1;
* @param index The index to set the value at.
* @param value The fieldIds to set.
* @return This builder for chaining.
*/
public Builder setFieldIds(
int index, int value) {
ensureFieldIdsIsMutable();
fieldIds_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @param value The fieldIds to add.
* @return This builder for chaining.
*/
public Builder addFieldIds(int value) {
ensureFieldIdsIsMutable();
fieldIds_.addInt(value);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @param values The fieldIds to add.
* @return This builder for chaining.
*/
public Builder addAllFieldIds(
java.lang.Iterable extends java.lang.Integer> values) {
ensureFieldIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fieldIds_);
onChanged();
return this;
}
/**
* repeated uint32 field_ids = 1;
* @return This builder for chaining.
*/
public Builder clearFieldIds() {
fieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.PrimaryKeySpec)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.PrimaryKeySpec)
private static final cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec();
}
public static cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PrimaryKeySpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PrimaryKeySpec(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PartitionSpecOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.PartitionSpec)
com.google.protobuf.MessageOrBuilder {
/**
* repeated uint32 src_field_ids = 1;
* @return A list containing the srcFieldIds.
*/
java.util.List getSrcFieldIdsList();
/**
* repeated uint32 src_field_ids = 1;
* @return The count of srcFieldIds.
*/
int getSrcFieldIdsCount();
/**
* repeated uint32 src_field_ids = 1;
* @param index The index of the element to return.
* @return The srcFieldIds at the given index.
*/
int getSrcFieldIds(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.PartitionSpec}
*/
public static final class PartitionSpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.PartitionSpec)
PartitionSpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use PartitionSpec.newBuilder() to construct.
private PartitionSpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PartitionSpec() {
srcFieldIds_ = emptyIntList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PartitionSpec();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PartitionSpec(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
srcFieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
srcFieldIds_.addInt(input.readUInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
srcFieldIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
srcFieldIds_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
srcFieldIds_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PartitionSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PartitionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.class, cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder.class);
}
public static final int SRC_FIELD_IDS_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.IntList srcFieldIds_;
/**
* repeated uint32 src_field_ids = 1;
* @return A list containing the srcFieldIds.
*/
@java.lang.Override
public java.util.List
getSrcFieldIdsList() {
return srcFieldIds_;
}
/**
* repeated uint32 src_field_ids = 1;
* @return The count of srcFieldIds.
*/
public int getSrcFieldIdsCount() {
return srcFieldIds_.size();
}
/**
* repeated uint32 src_field_ids = 1;
* @param index The index of the element to return.
* @return The srcFieldIds at the given index.
*/
public int getSrcFieldIds(int index) {
return srcFieldIds_.getInt(index);
}
private int srcFieldIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getSrcFieldIdsList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(srcFieldIdsMemoizedSerializedSize);
}
for (int i = 0; i < srcFieldIds_.size(); i++) {
output.writeUInt32NoTag(srcFieldIds_.getInt(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < srcFieldIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(srcFieldIds_.getInt(i));
}
size += dataSize;
if (!getSrcFieldIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
srcFieldIdsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.PartitionSpec)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.PartitionSpec other = (cz.proto.ingestion.v2.IngestionV2.PartitionSpec) obj;
if (!getSrcFieldIdsList()
.equals(other.getSrcFieldIdsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSrcFieldIdsCount() > 0) {
hash = (37 * hash) + SRC_FIELD_IDS_FIELD_NUMBER;
hash = (53 * hash) + getSrcFieldIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.PartitionSpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.PartitionSpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.PartitionSpec)
cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PartitionSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PartitionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.class, cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.PartitionSpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
srcFieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_PartitionSpec_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.PartitionSpec.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec build() {
cz.proto.ingestion.v2.IngestionV2.PartitionSpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec buildPartial() {
cz.proto.ingestion.v2.IngestionV2.PartitionSpec result = new cz.proto.ingestion.v2.IngestionV2.PartitionSpec(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
srcFieldIds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.srcFieldIds_ = srcFieldIds_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.PartitionSpec) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.PartitionSpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.PartitionSpec other) {
if (other == cz.proto.ingestion.v2.IngestionV2.PartitionSpec.getDefaultInstance()) return this;
if (!other.srcFieldIds_.isEmpty()) {
if (srcFieldIds_.isEmpty()) {
srcFieldIds_ = other.srcFieldIds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSrcFieldIdsIsMutable();
srcFieldIds_.addAll(other.srcFieldIds_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.PartitionSpec parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.PartitionSpec) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList srcFieldIds_ = emptyIntList();
private void ensureSrcFieldIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
srcFieldIds_ = mutableCopy(srcFieldIds_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated uint32 src_field_ids = 1;
* @return A list containing the srcFieldIds.
*/
public java.util.List
getSrcFieldIdsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(srcFieldIds_) : srcFieldIds_;
}
/**
* repeated uint32 src_field_ids = 1;
* @return The count of srcFieldIds.
*/
public int getSrcFieldIdsCount() {
return srcFieldIds_.size();
}
/**
* repeated uint32 src_field_ids = 1;
* @param index The index of the element to return.
* @return The srcFieldIds at the given index.
*/
public int getSrcFieldIds(int index) {
return srcFieldIds_.getInt(index);
}
/**
* repeated uint32 src_field_ids = 1;
* @param index The index to set the value at.
* @param value The srcFieldIds to set.
* @return This builder for chaining.
*/
public Builder setSrcFieldIds(
int index, int value) {
ensureSrcFieldIdsIsMutable();
srcFieldIds_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated uint32 src_field_ids = 1;
* @param value The srcFieldIds to add.
* @return This builder for chaining.
*/
public Builder addSrcFieldIds(int value) {
ensureSrcFieldIdsIsMutable();
srcFieldIds_.addInt(value);
onChanged();
return this;
}
/**
* repeated uint32 src_field_ids = 1;
* @param values The srcFieldIds to add.
* @return This builder for chaining.
*/
public Builder addAllSrcFieldIds(
java.lang.Iterable extends java.lang.Integer> values) {
ensureSrcFieldIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, srcFieldIds_);
onChanged();
return this;
}
/**
* repeated uint32 src_field_ids = 1;
* @return This builder for chaining.
*/
public Builder clearSrcFieldIds() {
srcFieldIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.PartitionSpec)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.PartitionSpec)
private static final cz.proto.ingestion.v2.IngestionV2.PartitionSpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.PartitionSpec();
}
public static cz.proto.ingestion.v2.IngestionV2.PartitionSpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PartitionSpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PartitionSpec(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StreamSchemaOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.StreamSchema)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
java.util.List
getDataFieldsList();
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
cz.proto.ingestion.v2.IngestionV2.DataField getDataFields(int index);
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
int getDataFieldsCount();
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
java.util.List extends cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder>
getDataFieldsOrBuilderList();
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder getDataFieldsOrBuilder(
int index);
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return Whether the distSpec field is set.
*/
boolean hasDistSpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return The distSpec.
*/
cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDistSpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder getDistSpecOrBuilder();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return Whether the primaryKeySpec field is set.
*/
boolean hasPrimaryKeySpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return The primaryKeySpec.
*/
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getPrimaryKeySpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder getPrimaryKeySpecOrBuilder();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return Whether the partitionSpec field is set.
*/
boolean hasPartitionSpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return The partitionSpec.
*/
cz.proto.ingestion.v2.IngestionV2.PartitionSpec getPartitionSpec();
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder getPartitionSpecOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.StreamSchema}
*/
public static final class StreamSchema extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.StreamSchema)
StreamSchemaOrBuilder {
private static final long serialVersionUID = 0L;
// Use StreamSchema.newBuilder() to construct.
private StreamSchema(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StreamSchema() {
dataFields_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StreamSchema();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StreamSchema(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
dataFields_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
dataFields_.add(
input.readMessage(cz.proto.ingestion.v2.IngestionV2.DataField.parser(), extensionRegistry));
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder subBuilder = null;
if (distSpec_ != null) {
subBuilder = distSpec_.toBuilder();
}
distSpec_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.DistributionSpec.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(distSpec_);
distSpec_ = subBuilder.buildPartial();
}
break;
}
case 26: {
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder subBuilder = null;
if (primaryKeySpec_ != null) {
subBuilder = primaryKeySpec_.toBuilder();
}
primaryKeySpec_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(primaryKeySpec_);
primaryKeySpec_ = subBuilder.buildPartial();
}
break;
}
case 34: {
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder subBuilder = null;
if (partitionSpec_ != null) {
subBuilder = partitionSpec_.toBuilder();
}
partitionSpec_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.PartitionSpec.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(partitionSpec_);
partitionSpec_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
dataFields_ = java.util.Collections.unmodifiableList(dataFields_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StreamSchema_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StreamSchema_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.StreamSchema.class, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder.class);
}
public static final int DATA_FIELDS_FIELD_NUMBER = 1;
private java.util.List dataFields_;
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
@java.lang.Override
public java.util.List getDataFieldsList() {
return dataFields_;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder>
getDataFieldsOrBuilderList() {
return dataFields_;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
@java.lang.Override
public int getDataFieldsCount() {
return dataFields_.size();
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataField getDataFields(int index) {
return dataFields_.get(index);
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder getDataFieldsOrBuilder(
int index) {
return dataFields_.get(index);
}
public static final int DIST_SPEC_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.DistributionSpec distSpec_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return Whether the distSpec field is set.
*/
@java.lang.Override
public boolean hasDistSpec() {
return distSpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return The distSpec.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDistSpec() {
return distSpec_ == null ? cz.proto.ingestion.v2.IngestionV2.DistributionSpec.getDefaultInstance() : distSpec_;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder getDistSpecOrBuilder() {
return getDistSpec();
}
public static final int PRIMARY_KEY_SPEC_FIELD_NUMBER = 3;
private cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec primaryKeySpec_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return Whether the primaryKeySpec field is set.
*/
@java.lang.Override
public boolean hasPrimaryKeySpec() {
return primaryKeySpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return The primaryKeySpec.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getPrimaryKeySpec() {
return primaryKeySpec_ == null ? cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.getDefaultInstance() : primaryKeySpec_;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder getPrimaryKeySpecOrBuilder() {
return getPrimaryKeySpec();
}
public static final int PARTITION_SPEC_FIELD_NUMBER = 4;
private cz.proto.ingestion.v2.IngestionV2.PartitionSpec partitionSpec_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return Whether the partitionSpec field is set.
*/
@java.lang.Override
public boolean hasPartitionSpec() {
return partitionSpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return The partitionSpec.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec getPartitionSpec() {
return partitionSpec_ == null ? cz.proto.ingestion.v2.IngestionV2.PartitionSpec.getDefaultInstance() : partitionSpec_;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder getPartitionSpecOrBuilder() {
return getPartitionSpec();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < dataFields_.size(); i++) {
output.writeMessage(1, dataFields_.get(i));
}
if (distSpec_ != null) {
output.writeMessage(2, getDistSpec());
}
if (primaryKeySpec_ != null) {
output.writeMessage(3, getPrimaryKeySpec());
}
if (partitionSpec_ != null) {
output.writeMessage(4, getPartitionSpec());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < dataFields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, dataFields_.get(i));
}
if (distSpec_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDistSpec());
}
if (primaryKeySpec_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPrimaryKeySpec());
}
if (partitionSpec_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPartitionSpec());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.StreamSchema)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.StreamSchema other = (cz.proto.ingestion.v2.IngestionV2.StreamSchema) obj;
if (!getDataFieldsList()
.equals(other.getDataFieldsList())) return false;
if (hasDistSpec() != other.hasDistSpec()) return false;
if (hasDistSpec()) {
if (!getDistSpec()
.equals(other.getDistSpec())) return false;
}
if (hasPrimaryKeySpec() != other.hasPrimaryKeySpec()) return false;
if (hasPrimaryKeySpec()) {
if (!getPrimaryKeySpec()
.equals(other.getPrimaryKeySpec())) return false;
}
if (hasPartitionSpec() != other.hasPartitionSpec()) return false;
if (hasPartitionSpec()) {
if (!getPartitionSpec()
.equals(other.getPartitionSpec())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getDataFieldsCount() > 0) {
hash = (37 * hash) + DATA_FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getDataFieldsList().hashCode();
}
if (hasDistSpec()) {
hash = (37 * hash) + DIST_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getDistSpec().hashCode();
}
if (hasPrimaryKeySpec()) {
hash = (37 * hash) + PRIMARY_KEY_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getPrimaryKeySpec().hashCode();
}
if (hasPartitionSpec()) {
hash = (37 * hash) + PARTITION_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getPartitionSpec().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.StreamSchema prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.StreamSchema}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.StreamSchema)
cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StreamSchema_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StreamSchema_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.StreamSchema.class, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.StreamSchema.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDataFieldsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (dataFieldsBuilder_ == null) {
dataFields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
dataFieldsBuilder_.clear();
}
if (distSpecBuilder_ == null) {
distSpec_ = null;
} else {
distSpec_ = null;
distSpecBuilder_ = null;
}
if (primaryKeySpecBuilder_ == null) {
primaryKeySpec_ = null;
} else {
primaryKeySpec_ = null;
primaryKeySpecBuilder_ = null;
}
if (partitionSpecBuilder_ == null) {
partitionSpec_ = null;
} else {
partitionSpec_ = null;
partitionSpecBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StreamSchema_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema build() {
cz.proto.ingestion.v2.IngestionV2.StreamSchema result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema buildPartial() {
cz.proto.ingestion.v2.IngestionV2.StreamSchema result = new cz.proto.ingestion.v2.IngestionV2.StreamSchema(this);
int from_bitField0_ = bitField0_;
if (dataFieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
dataFields_ = java.util.Collections.unmodifiableList(dataFields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.dataFields_ = dataFields_;
} else {
result.dataFields_ = dataFieldsBuilder_.build();
}
if (distSpecBuilder_ == null) {
result.distSpec_ = distSpec_;
} else {
result.distSpec_ = distSpecBuilder_.build();
}
if (primaryKeySpecBuilder_ == null) {
result.primaryKeySpec_ = primaryKeySpec_;
} else {
result.primaryKeySpec_ = primaryKeySpecBuilder_.build();
}
if (partitionSpecBuilder_ == null) {
result.partitionSpec_ = partitionSpec_;
} else {
result.partitionSpec_ = partitionSpecBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.StreamSchema) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.StreamSchema)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.StreamSchema other) {
if (other == cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance()) return this;
if (dataFieldsBuilder_ == null) {
if (!other.dataFields_.isEmpty()) {
if (dataFields_.isEmpty()) {
dataFields_ = other.dataFields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDataFieldsIsMutable();
dataFields_.addAll(other.dataFields_);
}
onChanged();
}
} else {
if (!other.dataFields_.isEmpty()) {
if (dataFieldsBuilder_.isEmpty()) {
dataFieldsBuilder_.dispose();
dataFieldsBuilder_ = null;
dataFields_ = other.dataFields_;
bitField0_ = (bitField0_ & ~0x00000001);
dataFieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDataFieldsFieldBuilder() : null;
} else {
dataFieldsBuilder_.addAllMessages(other.dataFields_);
}
}
}
if (other.hasDistSpec()) {
mergeDistSpec(other.getDistSpec());
}
if (other.hasPrimaryKeySpec()) {
mergePrimaryKeySpec(other.getPrimaryKeySpec());
}
if (other.hasPartitionSpec()) {
mergePartitionSpec(other.getPartitionSpec());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.StreamSchema parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.StreamSchema) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List dataFields_ =
java.util.Collections.emptyList();
private void ensureDataFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
dataFields_ = new java.util.ArrayList(dataFields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DataField, cz.proto.ingestion.v2.IngestionV2.DataField.Builder, cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder> dataFieldsBuilder_;
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public java.util.List getDataFieldsList() {
if (dataFieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(dataFields_);
} else {
return dataFieldsBuilder_.getMessageList();
}
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public int getDataFieldsCount() {
if (dataFieldsBuilder_ == null) {
return dataFields_.size();
} else {
return dataFieldsBuilder_.getCount();
}
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.DataField getDataFields(int index) {
if (dataFieldsBuilder_ == null) {
return dataFields_.get(index);
} else {
return dataFieldsBuilder_.getMessage(index);
}
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder setDataFields(
int index, cz.proto.ingestion.v2.IngestionV2.DataField value) {
if (dataFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDataFieldsIsMutable();
dataFields_.set(index, value);
onChanged();
} else {
dataFieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder setDataFields(
int index, cz.proto.ingestion.v2.IngestionV2.DataField.Builder builderForValue) {
if (dataFieldsBuilder_ == null) {
ensureDataFieldsIsMutable();
dataFields_.set(index, builderForValue.build());
onChanged();
} else {
dataFieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder addDataFields(cz.proto.ingestion.v2.IngestionV2.DataField value) {
if (dataFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDataFieldsIsMutable();
dataFields_.add(value);
onChanged();
} else {
dataFieldsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder addDataFields(
int index, cz.proto.ingestion.v2.IngestionV2.DataField value) {
if (dataFieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDataFieldsIsMutable();
dataFields_.add(index, value);
onChanged();
} else {
dataFieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder addDataFields(
cz.proto.ingestion.v2.IngestionV2.DataField.Builder builderForValue) {
if (dataFieldsBuilder_ == null) {
ensureDataFieldsIsMutable();
dataFields_.add(builderForValue.build());
onChanged();
} else {
dataFieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder addDataFields(
int index, cz.proto.ingestion.v2.IngestionV2.DataField.Builder builderForValue) {
if (dataFieldsBuilder_ == null) {
ensureDataFieldsIsMutable();
dataFields_.add(index, builderForValue.build());
onChanged();
} else {
dataFieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder addAllDataFields(
java.lang.Iterable extends cz.proto.ingestion.v2.IngestionV2.DataField> values) {
if (dataFieldsBuilder_ == null) {
ensureDataFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, dataFields_);
onChanged();
} else {
dataFieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder clearDataFields() {
if (dataFieldsBuilder_ == null) {
dataFields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
dataFieldsBuilder_.clear();
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public Builder removeDataFields(int index) {
if (dataFieldsBuilder_ == null) {
ensureDataFieldsIsMutable();
dataFields_.remove(index);
onChanged();
} else {
dataFieldsBuilder_.remove(index);
}
return this;
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.DataField.Builder getDataFieldsBuilder(
int index) {
return getDataFieldsFieldBuilder().getBuilder(index);
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder getDataFieldsOrBuilder(
int index) {
if (dataFieldsBuilder_ == null) {
return dataFields_.get(index); } else {
return dataFieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public java.util.List extends cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder>
getDataFieldsOrBuilderList() {
if (dataFieldsBuilder_ != null) {
return dataFieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(dataFields_);
}
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.DataField.Builder addDataFieldsBuilder() {
return getDataFieldsFieldBuilder().addBuilder(
cz.proto.ingestion.v2.IngestionV2.DataField.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.DataField.Builder addDataFieldsBuilder(
int index) {
return getDataFieldsFieldBuilder().addBuilder(
index, cz.proto.ingestion.v2.IngestionV2.DataField.getDefaultInstance());
}
/**
* repeated .cz.proto.ingestion.v2.DataField data_fields = 1;
*/
public java.util.List
getDataFieldsBuilderList() {
return getDataFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DataField, cz.proto.ingestion.v2.IngestionV2.DataField.Builder, cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder>
getDataFieldsFieldBuilder() {
if (dataFieldsBuilder_ == null) {
dataFieldsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DataField, cz.proto.ingestion.v2.IngestionV2.DataField.Builder, cz.proto.ingestion.v2.IngestionV2.DataFieldOrBuilder>(
dataFields_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
dataFields_ = null;
}
return dataFieldsBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.DistributionSpec distSpec_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DistributionSpec, cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder> distSpecBuilder_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return Whether the distSpec field is set.
*/
public boolean hasDistSpec() {
return distSpecBuilder_ != null || distSpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
* @return The distSpec.
*/
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec getDistSpec() {
if (distSpecBuilder_ == null) {
return distSpec_ == null ? cz.proto.ingestion.v2.IngestionV2.DistributionSpec.getDefaultInstance() : distSpec_;
} else {
return distSpecBuilder_.getMessage();
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public Builder setDistSpec(cz.proto.ingestion.v2.IngestionV2.DistributionSpec value) {
if (distSpecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
distSpec_ = value;
onChanged();
} else {
distSpecBuilder_.setMessage(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public Builder setDistSpec(
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder builderForValue) {
if (distSpecBuilder_ == null) {
distSpec_ = builderForValue.build();
onChanged();
} else {
distSpecBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public Builder mergeDistSpec(cz.proto.ingestion.v2.IngestionV2.DistributionSpec value) {
if (distSpecBuilder_ == null) {
if (distSpec_ != null) {
distSpec_ =
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.newBuilder(distSpec_).mergeFrom(value).buildPartial();
} else {
distSpec_ = value;
}
onChanged();
} else {
distSpecBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public Builder clearDistSpec() {
if (distSpecBuilder_ == null) {
distSpec_ = null;
onChanged();
} else {
distSpec_ = null;
distSpecBuilder_ = null;
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder getDistSpecBuilder() {
onChanged();
return getDistSpecFieldBuilder().getBuilder();
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder getDistSpecOrBuilder() {
if (distSpecBuilder_ != null) {
return distSpecBuilder_.getMessageOrBuilder();
} else {
return distSpec_ == null ?
cz.proto.ingestion.v2.IngestionV2.DistributionSpec.getDefaultInstance() : distSpec_;
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.DistributionSpec dist_spec = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DistributionSpec, cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder>
getDistSpecFieldBuilder() {
if (distSpecBuilder_ == null) {
distSpecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.DistributionSpec, cz.proto.ingestion.v2.IngestionV2.DistributionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.DistributionSpecOrBuilder>(
getDistSpec(),
getParentForChildren(),
isClean());
distSpec_ = null;
}
return distSpecBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec primaryKeySpec_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder> primaryKeySpecBuilder_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return Whether the primaryKeySpec field is set.
*/
public boolean hasPrimaryKeySpec() {
return primaryKeySpecBuilder_ != null || primaryKeySpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
* @return The primaryKeySpec.
*/
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec getPrimaryKeySpec() {
if (primaryKeySpecBuilder_ == null) {
return primaryKeySpec_ == null ? cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.getDefaultInstance() : primaryKeySpec_;
} else {
return primaryKeySpecBuilder_.getMessage();
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public Builder setPrimaryKeySpec(cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec value) {
if (primaryKeySpecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
primaryKeySpec_ = value;
onChanged();
} else {
primaryKeySpecBuilder_.setMessage(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public Builder setPrimaryKeySpec(
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder builderForValue) {
if (primaryKeySpecBuilder_ == null) {
primaryKeySpec_ = builderForValue.build();
onChanged();
} else {
primaryKeySpecBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public Builder mergePrimaryKeySpec(cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec value) {
if (primaryKeySpecBuilder_ == null) {
if (primaryKeySpec_ != null) {
primaryKeySpec_ =
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.newBuilder(primaryKeySpec_).mergeFrom(value).buildPartial();
} else {
primaryKeySpec_ = value;
}
onChanged();
} else {
primaryKeySpecBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public Builder clearPrimaryKeySpec() {
if (primaryKeySpecBuilder_ == null) {
primaryKeySpec_ = null;
onChanged();
} else {
primaryKeySpec_ = null;
primaryKeySpecBuilder_ = null;
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder getPrimaryKeySpecBuilder() {
onChanged();
return getPrimaryKeySpecFieldBuilder().getBuilder();
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
public cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder getPrimaryKeySpecOrBuilder() {
if (primaryKeySpecBuilder_ != null) {
return primaryKeySpecBuilder_.getMessageOrBuilder();
} else {
return primaryKeySpec_ == null ?
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.getDefaultInstance() : primaryKeySpec_;
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PrimaryKeySpec primary_key_spec = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder>
getPrimaryKeySpecFieldBuilder() {
if (primaryKeySpecBuilder_ == null) {
primaryKeySpecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpec.Builder, cz.proto.ingestion.v2.IngestionV2.PrimaryKeySpecOrBuilder>(
getPrimaryKeySpec(),
getParentForChildren(),
isClean());
primaryKeySpec_ = null;
}
return primaryKeySpecBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.PartitionSpec partitionSpec_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PartitionSpec, cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder> partitionSpecBuilder_;
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return Whether the partitionSpec field is set.
*/
public boolean hasPartitionSpec() {
return partitionSpecBuilder_ != null || partitionSpec_ != null;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
* @return The partitionSpec.
*/
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec getPartitionSpec() {
if (partitionSpecBuilder_ == null) {
return partitionSpec_ == null ? cz.proto.ingestion.v2.IngestionV2.PartitionSpec.getDefaultInstance() : partitionSpec_;
} else {
return partitionSpecBuilder_.getMessage();
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public Builder setPartitionSpec(cz.proto.ingestion.v2.IngestionV2.PartitionSpec value) {
if (partitionSpecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
partitionSpec_ = value;
onChanged();
} else {
partitionSpecBuilder_.setMessage(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public Builder setPartitionSpec(
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder builderForValue) {
if (partitionSpecBuilder_ == null) {
partitionSpec_ = builderForValue.build();
onChanged();
} else {
partitionSpecBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public Builder mergePartitionSpec(cz.proto.ingestion.v2.IngestionV2.PartitionSpec value) {
if (partitionSpecBuilder_ == null) {
if (partitionSpec_ != null) {
partitionSpec_ =
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.newBuilder(partitionSpec_).mergeFrom(value).buildPartial();
} else {
partitionSpec_ = value;
}
onChanged();
} else {
partitionSpecBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public Builder clearPartitionSpec() {
if (partitionSpecBuilder_ == null) {
partitionSpec_ = null;
onChanged();
} else {
partitionSpec_ = null;
partitionSpecBuilder_ = null;
}
return this;
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder getPartitionSpecBuilder() {
onChanged();
return getPartitionSpecFieldBuilder().getBuilder();
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder getPartitionSpecOrBuilder() {
if (partitionSpecBuilder_ != null) {
return partitionSpecBuilder_.getMessageOrBuilder();
} else {
return partitionSpec_ == null ?
cz.proto.ingestion.v2.IngestionV2.PartitionSpec.getDefaultInstance() : partitionSpec_;
}
}
/**
*
* optional
*
*
* .cz.proto.ingestion.v2.PartitionSpec partition_spec = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PartitionSpec, cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder>
getPartitionSpecFieldBuilder() {
if (partitionSpecBuilder_ == null) {
partitionSpecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.PartitionSpec, cz.proto.ingestion.v2.IngestionV2.PartitionSpec.Builder, cz.proto.ingestion.v2.IngestionV2.PartitionSpecOrBuilder>(
getPartitionSpec(),
getParentForChildren(),
isClean());
partitionSpec_ = null;
}
return partitionSpecBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.StreamSchema)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.StreamSchema)
private static final cz.proto.ingestion.v2.IngestionV2.StreamSchema DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.StreamSchema();
}
public static cz.proto.ingestion.v2.IngestionV2.StreamSchema getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StreamSchema parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StreamSchema(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateOrGetStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CreateOrGetStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
boolean hasTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder();
/**
* uint32 num_tablets = 3;
* @return The numTablets.
*/
int getNumTablets();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateOrGetStreamRequest}
*/
public static final class CreateOrGetStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CreateOrGetStreamRequest)
CreateOrGetStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateOrGetStreamRequest.newBuilder() to construct.
private CreateOrGetStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateOrGetStreamRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateOrGetStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CreateOrGetStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (tableIdent_ != null) {
subBuilder = tableIdent_.toBuilder();
}
tableIdent_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableIdent_);
tableIdent_ = subBuilder.buildPartial();
}
break;
}
case 24: {
numTablets_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int TABLE_IDENT_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
@java.lang.Override
public boolean hasTableIdent() {
return tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
return getTableIdent();
}
public static final int NUM_TABLETS_FIELD_NUMBER = 3;
private int numTablets_;
/**
* uint32 num_tablets = 3;
* @return The numTablets.
*/
@java.lang.Override
public int getNumTablets() {
return numTablets_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (tableIdent_ != null) {
output.writeMessage(2, getTableIdent());
}
if (numTablets_ != 0) {
output.writeUInt32(3, numTablets_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (tableIdent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTableIdent());
}
if (numTablets_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, numTablets_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest other = (cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasTableIdent() != other.hasTableIdent()) return false;
if (hasTableIdent()) {
if (!getTableIdent()
.equals(other.getTableIdent())) return false;
}
if (getNumTablets()
!= other.getNumTablets()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasTableIdent()) {
hash = (37 * hash) + TABLE_IDENT_FIELD_NUMBER;
hash = (53 * hash) + getTableIdent().hashCode();
}
hash = (37 * hash) + NUM_TABLETS_FIELD_NUMBER;
hash = (53 * hash) + getNumTablets();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateOrGetStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CreateOrGetStreamRequest)
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
numTablets_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest build() {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest result = new cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (tableIdentBuilder_ == null) {
result.tableIdent_ = tableIdent_;
} else {
result.tableIdent_ = tableIdentBuilder_.build();
}
result.numTablets_ = numTablets_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasTableIdent()) {
mergeTableIdent(other.getTableIdent());
}
if (other.getNumTablets() != 0) {
setNumTablets(other.getNumTablets());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> tableIdentBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
public boolean hasTableIdent() {
return tableIdentBuilder_ != null || tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
if (tableIdentBuilder_ == null) {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
} else {
return tableIdentBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder setTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableIdent_ = value;
onChanged();
} else {
tableIdentBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder setTableIdent(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (tableIdentBuilder_ == null) {
tableIdent_ = builderForValue.build();
onChanged();
} else {
tableIdentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder mergeTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (tableIdent_ != null) {
tableIdent_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(tableIdent_).mergeFrom(value).buildPartial();
} else {
tableIdent_ = value;
}
onChanged();
} else {
tableIdentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder clearTableIdent() {
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
onChanged();
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getTableIdentBuilder() {
onChanged();
return getTableIdentFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
if (tableIdentBuilder_ != null) {
return tableIdentBuilder_.getMessageOrBuilder();
} else {
return tableIdent_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getTableIdentFieldBuilder() {
if (tableIdentBuilder_ == null) {
tableIdentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getTableIdent(),
getParentForChildren(),
isClean());
tableIdent_ = null;
}
return tableIdentBuilder_;
}
private int numTablets_ ;
/**
* uint32 num_tablets = 3;
* @return The numTablets.
*/
@java.lang.Override
public int getNumTablets() {
return numTablets_;
}
/**
* uint32 num_tablets = 3;
* @param value The numTablets to set.
* @return This builder for chaining.
*/
public Builder setNumTablets(int value) {
numTablets_ = value;
onChanged();
return this;
}
/**
* uint32 num_tablets = 3;
* @return This builder for chaining.
*/
public Builder clearNumTablets() {
numTablets_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CreateOrGetStreamRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CreateOrGetStreamRequest)
private static final cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateOrGetStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateOrGetStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateOrGetStreamResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CreateOrGetStreamResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
boolean hasTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder();
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return Whether the dataSchema field is set.
*/
boolean hasDataSchema();
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return The dataSchema.
*/
cz.proto.ingestion.v2.IngestionV2.StreamSchema getDataSchema();
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getDataSchemaOrBuilder();
/**
* bool already_exists = 3;
* @return The alreadyExists.
*/
boolean getAlreadyExists();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* bool require_commit = 5;
* @return The requireCommit.
*/
boolean getRequireCommit();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateOrGetStreamResponse}
*/
public static final class CreateOrGetStreamResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CreateOrGetStreamResponse)
CreateOrGetStreamResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateOrGetStreamResponse.newBuilder() to construct.
private CreateOrGetStreamResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateOrGetStreamResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateOrGetStreamResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CreateOrGetStreamResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (tableIdent_ != null) {
subBuilder = tableIdent_.toBuilder();
}
tableIdent_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableIdent_);
tableIdent_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder subBuilder = null;
if (dataSchema_ != null) {
subBuilder = dataSchema_.toBuilder();
}
dataSchema_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.StreamSchema.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dataSchema_);
dataSchema_ = subBuilder.buildPartial();
}
break;
}
case 24: {
alreadyExists_ = input.readBool();
break;
}
case 34: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 40: {
requireCommit_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.Builder.class);
}
public static final int TABLE_IDENT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
@java.lang.Override
public boolean hasTableIdent() {
return tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
return getTableIdent();
}
public static final int DATA_SCHEMA_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.StreamSchema dataSchema_;
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return Whether the dataSchema field is set.
*/
@java.lang.Override
public boolean hasDataSchema() {
return dataSchema_ != null;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return The dataSchema.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getDataSchema() {
return dataSchema_ == null ? cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : dataSchema_;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getDataSchemaOrBuilder() {
return getDataSchema();
}
public static final int ALREADY_EXISTS_FIELD_NUMBER = 3;
private boolean alreadyExists_;
/**
* bool already_exists = 3;
* @return The alreadyExists.
*/
@java.lang.Override
public boolean getAlreadyExists() {
return alreadyExists_;
}
public static final int STATUS_FIELD_NUMBER = 4;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int REQUIRE_COMMIT_FIELD_NUMBER = 5;
private boolean requireCommit_;
/**
* bool require_commit = 5;
* @return The requireCommit.
*/
@java.lang.Override
public boolean getRequireCommit() {
return requireCommit_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (tableIdent_ != null) {
output.writeMessage(1, getTableIdent());
}
if (dataSchema_ != null) {
output.writeMessage(2, getDataSchema());
}
if (alreadyExists_ != false) {
output.writeBool(3, alreadyExists_);
}
if (status_ != null) {
output.writeMessage(4, getStatus());
}
if (requireCommit_ != false) {
output.writeBool(5, requireCommit_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tableIdent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTableIdent());
}
if (dataSchema_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getDataSchema());
}
if (alreadyExists_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, alreadyExists_);
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getStatus());
}
if (requireCommit_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, requireCommit_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse other = (cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse) obj;
if (hasTableIdent() != other.hasTableIdent()) return false;
if (hasTableIdent()) {
if (!getTableIdent()
.equals(other.getTableIdent())) return false;
}
if (hasDataSchema() != other.hasDataSchema()) return false;
if (hasDataSchema()) {
if (!getDataSchema()
.equals(other.getDataSchema())) return false;
}
if (getAlreadyExists()
!= other.getAlreadyExists()) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (getRequireCommit()
!= other.getRequireCommit()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTableIdent()) {
hash = (37 * hash) + TABLE_IDENT_FIELD_NUMBER;
hash = (53 * hash) + getTableIdent().hashCode();
}
if (hasDataSchema()) {
hash = (37 * hash) + DATA_SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getDataSchema().hashCode();
}
hash = (37 * hash) + ALREADY_EXISTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAlreadyExists());
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (37 * hash) + REQUIRE_COMMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRequireCommit());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateOrGetStreamResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CreateOrGetStreamResponse)
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
if (dataSchemaBuilder_ == null) {
dataSchema_ = null;
} else {
dataSchema_ = null;
dataSchemaBuilder_ = null;
}
alreadyExists_ = false;
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
requireCommit_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateOrGetStreamResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse build() {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse result = new cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse(this);
if (tableIdentBuilder_ == null) {
result.tableIdent_ = tableIdent_;
} else {
result.tableIdent_ = tableIdentBuilder_.build();
}
if (dataSchemaBuilder_ == null) {
result.dataSchema_ = dataSchema_;
} else {
result.dataSchema_ = dataSchemaBuilder_.build();
}
result.alreadyExists_ = alreadyExists_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
result.requireCommit_ = requireCommit_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse.getDefaultInstance()) return this;
if (other.hasTableIdent()) {
mergeTableIdent(other.getTableIdent());
}
if (other.hasDataSchema()) {
mergeDataSchema(other.getDataSchema());
}
if (other.getAlreadyExists() != false) {
setAlreadyExists(other.getAlreadyExists());
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.getRequireCommit() != false) {
setRequireCommit(other.getRequireCommit());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> tableIdentBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
public boolean hasTableIdent() {
return tableIdentBuilder_ != null || tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
if (tableIdentBuilder_ == null) {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
} else {
return tableIdentBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder setTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableIdent_ = value;
onChanged();
} else {
tableIdentBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder setTableIdent(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (tableIdentBuilder_ == null) {
tableIdent_ = builderForValue.build();
onChanged();
} else {
tableIdentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder mergeTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (tableIdent_ != null) {
tableIdent_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(tableIdent_).mergeFrom(value).buildPartial();
} else {
tableIdent_ = value;
}
onChanged();
} else {
tableIdentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder clearTableIdent() {
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
onChanged();
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getTableIdentBuilder() {
onChanged();
return getTableIdentFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
if (tableIdentBuilder_ != null) {
return tableIdentBuilder_.getMessageOrBuilder();
} else {
return tableIdent_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getTableIdentFieldBuilder() {
if (tableIdentBuilder_ == null) {
tableIdentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getTableIdent(),
getParentForChildren(),
isClean());
tableIdent_ = null;
}
return tableIdentBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.StreamSchema dataSchema_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder> dataSchemaBuilder_;
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return Whether the dataSchema field is set.
*/
public boolean hasDataSchema() {
return dataSchemaBuilder_ != null || dataSchema_ != null;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
* @return The dataSchema.
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getDataSchema() {
if (dataSchemaBuilder_ == null) {
return dataSchema_ == null ? cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : dataSchema_;
} else {
return dataSchemaBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public Builder setDataSchema(cz.proto.ingestion.v2.IngestionV2.StreamSchema value) {
if (dataSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dataSchema_ = value;
onChanged();
} else {
dataSchemaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public Builder setDataSchema(
cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder builderForValue) {
if (dataSchemaBuilder_ == null) {
dataSchema_ = builderForValue.build();
onChanged();
} else {
dataSchemaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public Builder mergeDataSchema(cz.proto.ingestion.v2.IngestionV2.StreamSchema value) {
if (dataSchemaBuilder_ == null) {
if (dataSchema_ != null) {
dataSchema_ =
cz.proto.ingestion.v2.IngestionV2.StreamSchema.newBuilder(dataSchema_).mergeFrom(value).buildPartial();
} else {
dataSchema_ = value;
}
onChanged();
} else {
dataSchemaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public Builder clearDataSchema() {
if (dataSchemaBuilder_ == null) {
dataSchema_ = null;
onChanged();
} else {
dataSchema_ = null;
dataSchemaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder getDataSchemaBuilder() {
onChanged();
return getDataSchemaFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getDataSchemaOrBuilder() {
if (dataSchemaBuilder_ != null) {
return dataSchemaBuilder_.getMessageOrBuilder();
} else {
return dataSchema_ == null ?
cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : dataSchema_;
}
}
/**
* .cz.proto.ingestion.v2.StreamSchema data_schema = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder>
getDataSchemaFieldBuilder() {
if (dataSchemaBuilder_ == null) {
dataSchemaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder>(
getDataSchema(),
getParentForChildren(),
isClean());
dataSchema_ = null;
}
return dataSchemaBuilder_;
}
private boolean alreadyExists_ ;
/**
* bool already_exists = 3;
* @return The alreadyExists.
*/
@java.lang.Override
public boolean getAlreadyExists() {
return alreadyExists_;
}
/**
* bool already_exists = 3;
* @param value The alreadyExists to set.
* @return This builder for chaining.
*/
public Builder setAlreadyExists(boolean value) {
alreadyExists_ = value;
onChanged();
return this;
}
/**
* bool already_exists = 3;
* @return This builder for chaining.
*/
public Builder clearAlreadyExists() {
alreadyExists_ = false;
onChanged();
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private boolean requireCommit_ ;
/**
* bool require_commit = 5;
* @return The requireCommit.
*/
@java.lang.Override
public boolean getRequireCommit() {
return requireCommit_;
}
/**
* bool require_commit = 5;
* @param value The requireCommit to set.
* @return This builder for chaining.
*/
public Builder setRequireCommit(boolean value) {
requireCommit_ = value;
onChanged();
return this;
}
/**
* bool require_commit = 5;
* @return This builder for chaining.
*/
public Builder clearRequireCommit() {
requireCommit_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CreateOrGetStreamResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CreateOrGetStreamResponse)
private static final cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateOrGetStreamResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateOrGetStreamResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateOrGetStreamResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CloseStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CloseStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
boolean hasTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CloseStreamRequest}
*/
public static final class CloseStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CloseStreamRequest)
CloseStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CloseStreamRequest.newBuilder() to construct.
private CloseStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CloseStreamRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CloseStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CloseStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (tableIdent_ != null) {
subBuilder = tableIdent_.toBuilder();
}
tableIdent_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableIdent_);
tableIdent_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int TABLE_IDENT_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
@java.lang.Override
public boolean hasTableIdent() {
return tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
return getTableIdent();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (tableIdent_ != null) {
output.writeMessage(2, getTableIdent());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (tableIdent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getTableIdent());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest other = (cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasTableIdent() != other.hasTableIdent()) return false;
if (hasTableIdent()) {
if (!getTableIdent()
.equals(other.getTableIdent())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasTableIdent()) {
hash = (37 * hash) + TABLE_IDENT_FIELD_NUMBER;
hash = (53 * hash) + getTableIdent().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CloseStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CloseStreamRequest)
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest build() {
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest result = new cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (tableIdentBuilder_ == null) {
result.tableIdent_ = tableIdent_;
} else {
result.tableIdent_ = tableIdentBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasTableIdent()) {
mergeTableIdent(other.getTableIdent());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> tableIdentBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return Whether the tableIdent field is set.
*/
public boolean hasTableIdent() {
return tableIdentBuilder_ != null || tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
* @return The tableIdent.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
if (tableIdentBuilder_ == null) {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
} else {
return tableIdentBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder setTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableIdent_ = value;
onChanged();
} else {
tableIdentBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder setTableIdent(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (tableIdentBuilder_ == null) {
tableIdent_ = builderForValue.build();
onChanged();
} else {
tableIdentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder mergeTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (tableIdent_ != null) {
tableIdent_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(tableIdent_).mergeFrom(value).buildPartial();
} else {
tableIdent_ = value;
}
onChanged();
} else {
tableIdentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public Builder clearTableIdent() {
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
onChanged();
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getTableIdentBuilder() {
onChanged();
return getTableIdentFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
if (tableIdentBuilder_ != null) {
return tableIdentBuilder_.getMessageOrBuilder();
} else {
return tableIdent_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getTableIdentFieldBuilder() {
if (tableIdentBuilder_ == null) {
tableIdentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getTableIdent(),
getParentForChildren(),
isClean());
tableIdent_ = null;
}
return tableIdentBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CloseStreamRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CloseStreamRequest)
private static final cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CloseStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CloseStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CloseStreamResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CloseStreamResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CloseStreamResponse}
*/
public static final class CloseStreamResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CloseStreamResponse)
CloseStreamResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CloseStreamResponse.newBuilder() to construct.
private CloseStreamResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CloseStreamResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CloseStreamResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CloseStreamResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse other = (cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CloseStreamResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CloseStreamResponse)
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CloseStreamResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse build() {
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse result = new cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CloseStreamResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CloseStreamResponse)
private static final cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CloseStreamResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CloseStreamResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CloseStreamResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OssStagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.OssStagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
java.lang.String getStsAkId();
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
com.google.protobuf.ByteString
getStsAkIdBytes();
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
java.lang.String getStsAkSecret();
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
com.google.protobuf.ByteString
getStsAkSecretBytes();
/**
* string sts_token = 5;
* @return The stsToken.
*/
java.lang.String getStsToken();
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
com.google.protobuf.ByteString
getStsTokenBytes();
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
java.lang.String getOssEndpoint();
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
com.google.protobuf.ByteString
getOssEndpointBytes();
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
java.lang.String getOssInternalEndpoint();
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
com.google.protobuf.ByteString
getOssInternalEndpointBytes();
/**
* uint64 oss_expire_time = 8;
* @return The ossExpireTime.
*/
long getOssExpireTime();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OssStagingPathInfo}
*/
public static final class OssStagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.OssStagingPathInfo)
OssStagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use OssStagingPathInfo.newBuilder() to construct.
private OssStagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OssStagingPathInfo() {
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
ossEndpoint_ = "";
ossInternalEndpoint_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OssStagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OssStagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
stsAkId_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stsAkSecret_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
stsToken_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
ossEndpoint_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
ossInternalEndpoint_ = s;
break;
}
case 64: {
ossExpireTime_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OssStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OssStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object stsAkId_;
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
@java.lang.Override
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
}
}
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_SECRET_FIELD_NUMBER = 4;
private volatile java.lang.Object stsAkSecret_;
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
@java.lang.Override
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
}
}
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_TOKEN_FIELD_NUMBER = 5;
private volatile java.lang.Object stsToken_;
/**
* string sts_token = 5;
* @return The stsToken.
*/
@java.lang.Override
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
}
}
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSS_ENDPOINT_FIELD_NUMBER = 6;
private volatile java.lang.Object ossEndpoint_;
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
@java.lang.Override
public java.lang.String getOssEndpoint() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossEndpoint_ = s;
return s;
}
}
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOssEndpointBytes() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSS_INTERNAL_ENDPOINT_FIELD_NUMBER = 7;
private volatile java.lang.Object ossInternalEndpoint_;
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
@java.lang.Override
public java.lang.String getOssInternalEndpoint() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossInternalEndpoint_ = s;
return s;
}
}
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOssInternalEndpointBytes() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossInternalEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OSS_EXPIRE_TIME_FIELD_NUMBER = 8;
private long ossExpireTime_;
/**
* uint64 oss_expire_time = 8;
* @return The ossExpireTime.
*/
@java.lang.Override
public long getOssExpireTime() {
return ossExpireTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossEndpoint_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, ossEndpoint_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossInternalEndpoint_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, ossInternalEndpoint_);
}
if (ossExpireTime_ != 0L) {
output.writeUInt64(8, ossExpireTime_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossEndpoint_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, ossEndpoint_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(ossInternalEndpoint_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, ossInternalEndpoint_);
}
if (ossExpireTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(8, ossExpireTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) obj;
if (!getPath()
.equals(other.getPath())) return false;
if (!getStsAkId()
.equals(other.getStsAkId())) return false;
if (!getStsAkSecret()
.equals(other.getStsAkSecret())) return false;
if (!getStsToken()
.equals(other.getStsToken())) return false;
if (!getOssEndpoint()
.equals(other.getOssEndpoint())) return false;
if (!getOssInternalEndpoint()
.equals(other.getOssInternalEndpoint())) return false;
if (getOssExpireTime()
!= other.getOssExpireTime()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + STS_AK_ID_FIELD_NUMBER;
hash = (53 * hash) + getStsAkId().hashCode();
hash = (37 * hash) + STS_AK_SECRET_FIELD_NUMBER;
hash = (53 * hash) + getStsAkSecret().hashCode();
hash = (37 * hash) + STS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getStsToken().hashCode();
hash = (37 * hash) + OSS_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + getOssEndpoint().hashCode();
hash = (37 * hash) + OSS_INTERNAL_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + getOssInternalEndpoint().hashCode();
hash = (37 * hash) + OSS_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOssExpireTime());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OssStagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.OssStagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OssStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OssStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
ossEndpoint_ = "";
ossInternalEndpoint_ = "";
ossExpireTime_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OssStagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo(this);
result.path_ = path_;
result.stsAkId_ = stsAkId_;
result.stsAkSecret_ = stsAkSecret_;
result.stsToken_ = stsToken_;
result.ossEndpoint_ = ossEndpoint_;
result.ossInternalEndpoint_ = ossInternalEndpoint_;
result.ossExpireTime_ = ossExpireTime_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getStsAkId().isEmpty()) {
stsAkId_ = other.stsAkId_;
onChanged();
}
if (!other.getStsAkSecret().isEmpty()) {
stsAkSecret_ = other.stsAkSecret_;
onChanged();
}
if (!other.getStsToken().isEmpty()) {
stsToken_ = other.stsToken_;
onChanged();
}
if (!other.getOssEndpoint().isEmpty()) {
ossEndpoint_ = other.ossEndpoint_;
onChanged();
}
if (!other.getOssInternalEndpoint().isEmpty()) {
ossInternalEndpoint_ = other.ossInternalEndpoint_;
onChanged();
}
if (other.getOssExpireTime() != 0L) {
setOssExpireTime(other.getOssExpireTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkId_ = "";
/**
* string sts_ak_id = 3;
* @return The stsAkId.
*/
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_id = 3;
* @return The bytes for stsAkId.
*/
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_id = 3;
* @param value The stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkId_ = value;
onChanged();
return this;
}
/**
* string sts_ak_id = 3;
* @return This builder for chaining.
*/
public Builder clearStsAkId() {
stsAkId_ = getDefaultInstance().getStsAkId();
onChanged();
return this;
}
/**
* string sts_ak_id = 3;
* @param value The bytes for stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkId_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkSecret_ = "";
/**
* string sts_ak_secret = 4;
* @return The stsAkSecret.
*/
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_secret = 4;
* @return The bytes for stsAkSecret.
*/
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_secret = 4;
* @param value The stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkSecret_ = value;
onChanged();
return this;
}
/**
* string sts_ak_secret = 4;
* @return This builder for chaining.
*/
public Builder clearStsAkSecret() {
stsAkSecret_ = getDefaultInstance().getStsAkSecret();
onChanged();
return this;
}
/**
* string sts_ak_secret = 4;
* @param value The bytes for stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkSecret_ = value;
onChanged();
return this;
}
private java.lang.Object stsToken_ = "";
/**
* string sts_token = 5;
* @return The stsToken.
*/
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_token = 5;
* @return The bytes for stsToken.
*/
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_token = 5;
* @param value The stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsToken_ = value;
onChanged();
return this;
}
/**
* string sts_token = 5;
* @return This builder for chaining.
*/
public Builder clearStsToken() {
stsToken_ = getDefaultInstance().getStsToken();
onChanged();
return this;
}
/**
* string sts_token = 5;
* @param value The bytes for stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsToken_ = value;
onChanged();
return this;
}
private java.lang.Object ossEndpoint_ = "";
/**
* string oss_endpoint = 6;
* @return The ossEndpoint.
*/
public java.lang.String getOssEndpoint() {
java.lang.Object ref = ossEndpoint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossEndpoint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string oss_endpoint = 6;
* @return The bytes for ossEndpoint.
*/
public com.google.protobuf.ByteString
getOssEndpointBytes() {
java.lang.Object ref = ossEndpoint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string oss_endpoint = 6;
* @param value The ossEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssEndpoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ossEndpoint_ = value;
onChanged();
return this;
}
/**
* string oss_endpoint = 6;
* @return This builder for chaining.
*/
public Builder clearOssEndpoint() {
ossEndpoint_ = getDefaultInstance().getOssEndpoint();
onChanged();
return this;
}
/**
* string oss_endpoint = 6;
* @param value The bytes for ossEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssEndpointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ossEndpoint_ = value;
onChanged();
return this;
}
private java.lang.Object ossInternalEndpoint_ = "";
/**
* string oss_internal_endpoint = 7;
* @return The ossInternalEndpoint.
*/
public java.lang.String getOssInternalEndpoint() {
java.lang.Object ref = ossInternalEndpoint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ossInternalEndpoint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string oss_internal_endpoint = 7;
* @return The bytes for ossInternalEndpoint.
*/
public com.google.protobuf.ByteString
getOssInternalEndpointBytes() {
java.lang.Object ref = ossInternalEndpoint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ossInternalEndpoint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string oss_internal_endpoint = 7;
* @param value The ossInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssInternalEndpoint(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ossInternalEndpoint_ = value;
onChanged();
return this;
}
/**
* string oss_internal_endpoint = 7;
* @return This builder for chaining.
*/
public Builder clearOssInternalEndpoint() {
ossInternalEndpoint_ = getDefaultInstance().getOssInternalEndpoint();
onChanged();
return this;
}
/**
* string oss_internal_endpoint = 7;
* @param value The bytes for ossInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setOssInternalEndpointBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ossInternalEndpoint_ = value;
onChanged();
return this;
}
private long ossExpireTime_ ;
/**
* uint64 oss_expire_time = 8;
* @return The ossExpireTime.
*/
@java.lang.Override
public long getOssExpireTime() {
return ossExpireTime_;
}
/**
* uint64 oss_expire_time = 8;
* @param value The ossExpireTime to set.
* @return This builder for chaining.
*/
public Builder setOssExpireTime(long value) {
ossExpireTime_ = value;
onChanged();
return this;
}
/**
* uint64 oss_expire_time = 8;
* @return This builder for chaining.
*/
public Builder clearOssExpireTime() {
ossExpireTime_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.OssStagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.OssStagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OssStagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OssStagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CosStagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CosStagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
java.lang.String getStsAkId();
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
com.google.protobuf.ByteString
getStsAkIdBytes();
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
java.lang.String getStsAkSecret();
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
com.google.protobuf.ByteString
getStsAkSecretBytes();
/**
* string sts_token = 4;
* @return The stsToken.
*/
java.lang.String getStsToken();
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
com.google.protobuf.ByteString
getStsTokenBytes();
/**
* string cos_region = 5;
* @return The cosRegion.
*/
java.lang.String getCosRegion();
/**
* string cos_region = 5;
* @return The bytes for cosRegion.
*/
com.google.protobuf.ByteString
getCosRegionBytes();
/**
* uint64 cos_expire_time = 6;
* @return The cosExpireTime.
*/
long getCosExpireTime();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CosStagingPathInfo}
*/
public static final class CosStagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CosStagingPathInfo)
CosStagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use CosStagingPathInfo.newBuilder() to construct.
private CosStagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CosStagingPathInfo() {
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
cosRegion_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CosStagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CosStagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
stsAkId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
stsAkSecret_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stsToken_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
cosRegion_ = s;
break;
}
case 48: {
cosExpireTime_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CosStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CosStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object stsAkId_;
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
@java.lang.Override
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_SECRET_FIELD_NUMBER = 3;
private volatile java.lang.Object stsAkSecret_;
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
@java.lang.Override
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_TOKEN_FIELD_NUMBER = 4;
private volatile java.lang.Object stsToken_;
/**
* string sts_token = 4;
* @return The stsToken.
*/
@java.lang.Override
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COS_REGION_FIELD_NUMBER = 5;
private volatile java.lang.Object cosRegion_;
/**
* string cos_region = 5;
* @return The cosRegion.
*/
@java.lang.Override
public java.lang.String getCosRegion() {
java.lang.Object ref = cosRegion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cosRegion_ = s;
return s;
}
}
/**
* string cos_region = 5;
* @return The bytes for cosRegion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCosRegionBytes() {
java.lang.Object ref = cosRegion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cosRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COS_EXPIRE_TIME_FIELD_NUMBER = 6;
private long cosExpireTime_;
/**
* uint64 cos_expire_time = 6;
* @return The cosExpireTime.
*/
@java.lang.Override
public long getCosExpireTime() {
return cosExpireTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cosRegion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, cosRegion_);
}
if (cosExpireTime_ != 0L) {
output.writeUInt64(6, cosExpireTime_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cosRegion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, cosRegion_);
}
if (cosExpireTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, cosExpireTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) obj;
if (!getPath()
.equals(other.getPath())) return false;
if (!getStsAkId()
.equals(other.getStsAkId())) return false;
if (!getStsAkSecret()
.equals(other.getStsAkSecret())) return false;
if (!getStsToken()
.equals(other.getStsToken())) return false;
if (!getCosRegion()
.equals(other.getCosRegion())) return false;
if (getCosExpireTime()
!= other.getCosExpireTime()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + STS_AK_ID_FIELD_NUMBER;
hash = (53 * hash) + getStsAkId().hashCode();
hash = (37 * hash) + STS_AK_SECRET_FIELD_NUMBER;
hash = (53 * hash) + getStsAkSecret().hashCode();
hash = (37 * hash) + STS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getStsToken().hashCode();
hash = (37 * hash) + COS_REGION_FIELD_NUMBER;
hash = (53 * hash) + getCosRegion().hashCode();
hash = (37 * hash) + COS_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCosExpireTime());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CosStagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CosStagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CosStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CosStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
cosRegion_ = "";
cosExpireTime_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CosStagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo(this);
result.path_ = path_;
result.stsAkId_ = stsAkId_;
result.stsAkSecret_ = stsAkSecret_;
result.stsToken_ = stsToken_;
result.cosRegion_ = cosRegion_;
result.cosExpireTime_ = cosExpireTime_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getStsAkId().isEmpty()) {
stsAkId_ = other.stsAkId_;
onChanged();
}
if (!other.getStsAkSecret().isEmpty()) {
stsAkSecret_ = other.stsAkSecret_;
onChanged();
}
if (!other.getStsToken().isEmpty()) {
stsToken_ = other.stsToken_;
onChanged();
}
if (!other.getCosRegion().isEmpty()) {
cosRegion_ = other.cosRegion_;
onChanged();
}
if (other.getCosExpireTime() != 0L) {
setCosExpireTime(other.getCosExpireTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkId_ = "";
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_id = 2;
* @param value The stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkId_ = value;
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @return This builder for chaining.
*/
public Builder clearStsAkId() {
stsAkId_ = getDefaultInstance().getStsAkId();
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @param value The bytes for stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkId_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkSecret_ = "";
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_secret = 3;
* @param value The stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkSecret_ = value;
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @return This builder for chaining.
*/
public Builder clearStsAkSecret() {
stsAkSecret_ = getDefaultInstance().getStsAkSecret();
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @param value The bytes for stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkSecret_ = value;
onChanged();
return this;
}
private java.lang.Object stsToken_ = "";
/**
* string sts_token = 4;
* @return The stsToken.
*/
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_token = 4;
* @param value The stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsToken_ = value;
onChanged();
return this;
}
/**
* string sts_token = 4;
* @return This builder for chaining.
*/
public Builder clearStsToken() {
stsToken_ = getDefaultInstance().getStsToken();
onChanged();
return this;
}
/**
* string sts_token = 4;
* @param value The bytes for stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsToken_ = value;
onChanged();
return this;
}
private java.lang.Object cosRegion_ = "";
/**
* string cos_region = 5;
* @return The cosRegion.
*/
public java.lang.String getCosRegion() {
java.lang.Object ref = cosRegion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cosRegion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string cos_region = 5;
* @return The bytes for cosRegion.
*/
public com.google.protobuf.ByteString
getCosRegionBytes() {
java.lang.Object ref = cosRegion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cosRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string cos_region = 5;
* @param value The cosRegion to set.
* @return This builder for chaining.
*/
public Builder setCosRegion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cosRegion_ = value;
onChanged();
return this;
}
/**
* string cos_region = 5;
* @return This builder for chaining.
*/
public Builder clearCosRegion() {
cosRegion_ = getDefaultInstance().getCosRegion();
onChanged();
return this;
}
/**
* string cos_region = 5;
* @param value The bytes for cosRegion to set.
* @return This builder for chaining.
*/
public Builder setCosRegionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cosRegion_ = value;
onChanged();
return this;
}
private long cosExpireTime_ ;
/**
* uint64 cos_expire_time = 6;
* @return The cosExpireTime.
*/
@java.lang.Override
public long getCosExpireTime() {
return cosExpireTime_;
}
/**
* uint64 cos_expire_time = 6;
* @param value The cosExpireTime to set.
* @return This builder for chaining.
*/
public Builder setCosExpireTime(long value) {
cosExpireTime_ = value;
onChanged();
return this;
}
/**
* uint64 cos_expire_time = 6;
* @return This builder for chaining.
*/
public Builder clearCosExpireTime() {
cosExpireTime_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CosStagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CosStagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CosStagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CosStagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface S3StagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.S3StagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
java.lang.String getStsAkId();
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
com.google.protobuf.ByteString
getStsAkIdBytes();
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
java.lang.String getStsAkSecret();
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
com.google.protobuf.ByteString
getStsAkSecretBytes();
/**
* string sts_token = 4;
* @return The stsToken.
*/
java.lang.String getStsToken();
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
com.google.protobuf.ByteString
getStsTokenBytes();
/**
* string s3_region = 5;
* @return The s3Region.
*/
java.lang.String getS3Region();
/**
* string s3_region = 5;
* @return The bytes for s3Region.
*/
com.google.protobuf.ByteString
getS3RegionBytes();
/**
* uint64 s3_expire_time = 6;
* @return The s3ExpireTime.
*/
long getS3ExpireTime();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.S3StagingPathInfo}
*/
public static final class S3StagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.S3StagingPathInfo)
S3StagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use S3StagingPathInfo.newBuilder() to construct.
private S3StagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private S3StagingPathInfo() {
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
s3Region_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new S3StagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private S3StagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
stsAkId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
stsAkSecret_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stsToken_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
s3Region_ = s;
break;
}
case 48: {
s3ExpireTime_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_S3StagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_S3StagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object stsAkId_;
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
@java.lang.Override
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_SECRET_FIELD_NUMBER = 3;
private volatile java.lang.Object stsAkSecret_;
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
@java.lang.Override
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_TOKEN_FIELD_NUMBER = 4;
private volatile java.lang.Object stsToken_;
/**
* string sts_token = 4;
* @return The stsToken.
*/
@java.lang.Override
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S3_REGION_FIELD_NUMBER = 5;
private volatile java.lang.Object s3Region_;
/**
* string s3_region = 5;
* @return The s3Region.
*/
@java.lang.Override
public java.lang.String getS3Region() {
java.lang.Object ref = s3Region_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
s3Region_ = s;
return s;
}
}
/**
* string s3_region = 5;
* @return The bytes for s3Region.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getS3RegionBytes() {
java.lang.Object ref = s3Region_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s3Region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S3_EXPIRE_TIME_FIELD_NUMBER = 6;
private long s3ExpireTime_;
/**
* uint64 s3_expire_time = 6;
* @return The s3ExpireTime.
*/
@java.lang.Override
public long getS3ExpireTime() {
return s3ExpireTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(s3Region_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, s3Region_);
}
if (s3ExpireTime_ != 0L) {
output.writeUInt64(6, s3ExpireTime_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(s3Region_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, s3Region_);
}
if (s3ExpireTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, s3ExpireTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) obj;
if (!getPath()
.equals(other.getPath())) return false;
if (!getStsAkId()
.equals(other.getStsAkId())) return false;
if (!getStsAkSecret()
.equals(other.getStsAkSecret())) return false;
if (!getStsToken()
.equals(other.getStsToken())) return false;
if (!getS3Region()
.equals(other.getS3Region())) return false;
if (getS3ExpireTime()
!= other.getS3ExpireTime()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + STS_AK_ID_FIELD_NUMBER;
hash = (53 * hash) + getStsAkId().hashCode();
hash = (37 * hash) + STS_AK_SECRET_FIELD_NUMBER;
hash = (53 * hash) + getStsAkSecret().hashCode();
hash = (37 * hash) + STS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getStsToken().hashCode();
hash = (37 * hash) + S3_REGION_FIELD_NUMBER;
hash = (53 * hash) + getS3Region().hashCode();
hash = (37 * hash) + S3_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getS3ExpireTime());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.S3StagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.S3StagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_S3StagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_S3StagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
s3Region_ = "";
s3ExpireTime_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_S3StagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo(this);
result.path_ = path_;
result.stsAkId_ = stsAkId_;
result.stsAkSecret_ = stsAkSecret_;
result.stsToken_ = stsToken_;
result.s3Region_ = s3Region_;
result.s3ExpireTime_ = s3ExpireTime_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getStsAkId().isEmpty()) {
stsAkId_ = other.stsAkId_;
onChanged();
}
if (!other.getStsAkSecret().isEmpty()) {
stsAkSecret_ = other.stsAkSecret_;
onChanged();
}
if (!other.getStsToken().isEmpty()) {
stsToken_ = other.stsToken_;
onChanged();
}
if (!other.getS3Region().isEmpty()) {
s3Region_ = other.s3Region_;
onChanged();
}
if (other.getS3ExpireTime() != 0L) {
setS3ExpireTime(other.getS3ExpireTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkId_ = "";
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_id = 2;
* @param value The stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkId_ = value;
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @return This builder for chaining.
*/
public Builder clearStsAkId() {
stsAkId_ = getDefaultInstance().getStsAkId();
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @param value The bytes for stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkId_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkSecret_ = "";
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_secret = 3;
* @param value The stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkSecret_ = value;
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @return This builder for chaining.
*/
public Builder clearStsAkSecret() {
stsAkSecret_ = getDefaultInstance().getStsAkSecret();
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @param value The bytes for stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkSecret_ = value;
onChanged();
return this;
}
private java.lang.Object stsToken_ = "";
/**
* string sts_token = 4;
* @return The stsToken.
*/
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_token = 4;
* @param value The stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsToken_ = value;
onChanged();
return this;
}
/**
* string sts_token = 4;
* @return This builder for chaining.
*/
public Builder clearStsToken() {
stsToken_ = getDefaultInstance().getStsToken();
onChanged();
return this;
}
/**
* string sts_token = 4;
* @param value The bytes for stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsToken_ = value;
onChanged();
return this;
}
private java.lang.Object s3Region_ = "";
/**
* string s3_region = 5;
* @return The s3Region.
*/
public java.lang.String getS3Region() {
java.lang.Object ref = s3Region_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
s3Region_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string s3_region = 5;
* @return The bytes for s3Region.
*/
public com.google.protobuf.ByteString
getS3RegionBytes() {
java.lang.Object ref = s3Region_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s3Region_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string s3_region = 5;
* @param value The s3Region to set.
* @return This builder for chaining.
*/
public Builder setS3Region(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
s3Region_ = value;
onChanged();
return this;
}
/**
* string s3_region = 5;
* @return This builder for chaining.
*/
public Builder clearS3Region() {
s3Region_ = getDefaultInstance().getS3Region();
onChanged();
return this;
}
/**
* string s3_region = 5;
* @param value The bytes for s3Region to set.
* @return This builder for chaining.
*/
public Builder setS3RegionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
s3Region_ = value;
onChanged();
return this;
}
private long s3ExpireTime_ ;
/**
* uint64 s3_expire_time = 6;
* @return The s3ExpireTime.
*/
@java.lang.Override
public long getS3ExpireTime() {
return s3ExpireTime_;
}
/**
* uint64 s3_expire_time = 6;
* @param value The s3ExpireTime to set.
* @return This builder for chaining.
*/
public Builder setS3ExpireTime(long value) {
s3ExpireTime_ = value;
onChanged();
return this;
}
/**
* uint64 s3_expire_time = 6;
* @return This builder for chaining.
*/
public Builder clearS3ExpireTime() {
s3ExpireTime_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.S3StagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.S3StagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public S3StagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new S3StagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GcsStagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GcsStagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
java.lang.String getStsAkId();
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
com.google.protobuf.ByteString
getStsAkIdBytes();
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
java.lang.String getStsAkSecret();
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
com.google.protobuf.ByteString
getStsAkSecretBytes();
/**
* string sts_token = 4;
* @return The stsToken.
*/
java.lang.String getStsToken();
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
com.google.protobuf.ByteString
getStsTokenBytes();
/**
* string gcs_region = 5;
* @return The gcsRegion.
*/
java.lang.String getGcsRegion();
/**
* string gcs_region = 5;
* @return The bytes for gcsRegion.
*/
com.google.protobuf.ByteString
getGcsRegionBytes();
/**
* uint64 gcs_expire_time = 6;
* @return The gcsExpireTime.
*/
long getGcsExpireTime();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GcsStagingPathInfo}
*/
public static final class GcsStagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GcsStagingPathInfo)
GcsStagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use GcsStagingPathInfo.newBuilder() to construct.
private GcsStagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GcsStagingPathInfo() {
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
gcsRegion_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GcsStagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GcsStagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
stsAkId_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
stsAkSecret_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
stsToken_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
gcsRegion_ = s;
break;
}
case 48: {
gcsExpireTime_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GcsStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GcsStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_ID_FIELD_NUMBER = 2;
private volatile java.lang.Object stsAkId_;
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
@java.lang.Override
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_AK_SECRET_FIELD_NUMBER = 3;
private volatile java.lang.Object stsAkSecret_;
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
@java.lang.Override
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STS_TOKEN_FIELD_NUMBER = 4;
private volatile java.lang.Object stsToken_;
/**
* string sts_token = 4;
* @return The stsToken.
*/
@java.lang.Override
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GCS_REGION_FIELD_NUMBER = 5;
private volatile java.lang.Object gcsRegion_;
/**
* string gcs_region = 5;
* @return The gcsRegion.
*/
@java.lang.Override
public java.lang.String getGcsRegion() {
java.lang.Object ref = gcsRegion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
gcsRegion_ = s;
return s;
}
}
/**
* string gcs_region = 5;
* @return The bytes for gcsRegion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getGcsRegionBytes() {
java.lang.Object ref = gcsRegion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gcsRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GCS_EXPIRE_TIME_FIELD_NUMBER = 6;
private long gcsExpireTime_;
/**
* uint64 gcs_expire_time = 6;
* @return The gcsExpireTime.
*/
@java.lang.Override
public long getGcsExpireTime() {
return gcsExpireTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(gcsRegion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, gcsRegion_);
}
if (gcsExpireTime_ != 0L) {
output.writeUInt64(6, gcsExpireTime_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, stsAkId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsAkSecret_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stsAkSecret_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stsToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, stsToken_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(gcsRegion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, gcsRegion_);
}
if (gcsExpireTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(6, gcsExpireTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) obj;
if (!getPath()
.equals(other.getPath())) return false;
if (!getStsAkId()
.equals(other.getStsAkId())) return false;
if (!getStsAkSecret()
.equals(other.getStsAkSecret())) return false;
if (!getStsToken()
.equals(other.getStsToken())) return false;
if (!getGcsRegion()
.equals(other.getGcsRegion())) return false;
if (getGcsExpireTime()
!= other.getGcsExpireTime()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (37 * hash) + STS_AK_ID_FIELD_NUMBER;
hash = (53 * hash) + getStsAkId().hashCode();
hash = (37 * hash) + STS_AK_SECRET_FIELD_NUMBER;
hash = (53 * hash) + getStsAkSecret().hashCode();
hash = (37 * hash) + STS_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getStsToken().hashCode();
hash = (37 * hash) + GCS_REGION_FIELD_NUMBER;
hash = (53 * hash) + getGcsRegion().hashCode();
hash = (37 * hash) + GCS_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getGcsExpireTime());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GcsStagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GcsStagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GcsStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GcsStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
path_ = "";
stsAkId_ = "";
stsAkSecret_ = "";
stsToken_ = "";
gcsRegion_ = "";
gcsExpireTime_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GcsStagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo(this);
result.path_ = path_;
result.stsAkId_ = stsAkId_;
result.stsAkSecret_ = stsAkSecret_;
result.stsToken_ = stsToken_;
result.gcsRegion_ = gcsRegion_;
result.gcsExpireTime_ = gcsExpireTime_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
if (!other.getStsAkId().isEmpty()) {
stsAkId_ = other.stsAkId_;
onChanged();
}
if (!other.getStsAkSecret().isEmpty()) {
stsAkSecret_ = other.stsAkSecret_;
onChanged();
}
if (!other.getStsToken().isEmpty()) {
stsToken_ = other.stsToken_;
onChanged();
}
if (!other.getGcsRegion().isEmpty()) {
gcsRegion_ = other.gcsRegion_;
onChanged();
}
if (other.getGcsExpireTime() != 0L) {
setGcsExpireTime(other.getGcsExpireTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkId_ = "";
/**
* string sts_ak_id = 2;
* @return The stsAkId.
*/
public java.lang.String getStsAkId() {
java.lang.Object ref = stsAkId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_id = 2;
* @return The bytes for stsAkId.
*/
public com.google.protobuf.ByteString
getStsAkIdBytes() {
java.lang.Object ref = stsAkId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_id = 2;
* @param value The stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkId_ = value;
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @return This builder for chaining.
*/
public Builder clearStsAkId() {
stsAkId_ = getDefaultInstance().getStsAkId();
onChanged();
return this;
}
/**
* string sts_ak_id = 2;
* @param value The bytes for stsAkId to set.
* @return This builder for chaining.
*/
public Builder setStsAkIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkId_ = value;
onChanged();
return this;
}
private java.lang.Object stsAkSecret_ = "";
/**
* string sts_ak_secret = 3;
* @return The stsAkSecret.
*/
public java.lang.String getStsAkSecret() {
java.lang.Object ref = stsAkSecret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsAkSecret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_ak_secret = 3;
* @return The bytes for stsAkSecret.
*/
public com.google.protobuf.ByteString
getStsAkSecretBytes() {
java.lang.Object ref = stsAkSecret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsAkSecret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_ak_secret = 3;
* @param value The stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsAkSecret_ = value;
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @return This builder for chaining.
*/
public Builder clearStsAkSecret() {
stsAkSecret_ = getDefaultInstance().getStsAkSecret();
onChanged();
return this;
}
/**
* string sts_ak_secret = 3;
* @param value The bytes for stsAkSecret to set.
* @return This builder for chaining.
*/
public Builder setStsAkSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsAkSecret_ = value;
onChanged();
return this;
}
private java.lang.Object stsToken_ = "";
/**
* string sts_token = 4;
* @return The stsToken.
*/
public java.lang.String getStsToken() {
java.lang.Object ref = stsToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stsToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sts_token = 4;
* @return The bytes for stsToken.
*/
public com.google.protobuf.ByteString
getStsTokenBytes() {
java.lang.Object ref = stsToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stsToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sts_token = 4;
* @param value The stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stsToken_ = value;
onChanged();
return this;
}
/**
* string sts_token = 4;
* @return This builder for chaining.
*/
public Builder clearStsToken() {
stsToken_ = getDefaultInstance().getStsToken();
onChanged();
return this;
}
/**
* string sts_token = 4;
* @param value The bytes for stsToken to set.
* @return This builder for chaining.
*/
public Builder setStsTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stsToken_ = value;
onChanged();
return this;
}
private java.lang.Object gcsRegion_ = "";
/**
* string gcs_region = 5;
* @return The gcsRegion.
*/
public java.lang.String getGcsRegion() {
java.lang.Object ref = gcsRegion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
gcsRegion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string gcs_region = 5;
* @return The bytes for gcsRegion.
*/
public com.google.protobuf.ByteString
getGcsRegionBytes() {
java.lang.Object ref = gcsRegion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gcsRegion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string gcs_region = 5;
* @param value The gcsRegion to set.
* @return This builder for chaining.
*/
public Builder setGcsRegion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
gcsRegion_ = value;
onChanged();
return this;
}
/**
* string gcs_region = 5;
* @return This builder for chaining.
*/
public Builder clearGcsRegion() {
gcsRegion_ = getDefaultInstance().getGcsRegion();
onChanged();
return this;
}
/**
* string gcs_region = 5;
* @param value The bytes for gcsRegion to set.
* @return This builder for chaining.
*/
public Builder setGcsRegionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
gcsRegion_ = value;
onChanged();
return this;
}
private long gcsExpireTime_ ;
/**
* uint64 gcs_expire_time = 6;
* @return The gcsExpireTime.
*/
@java.lang.Override
public long getGcsExpireTime() {
return gcsExpireTime_;
}
/**
* uint64 gcs_expire_time = 6;
* @param value The gcsExpireTime to set.
* @return This builder for chaining.
*/
public Builder setGcsExpireTime(long value) {
gcsExpireTime_ = value;
onChanged();
return this;
}
/**
* uint64 gcs_expire_time = 6;
* @return This builder for chaining.
*/
public Builder clearGcsExpireTime() {
gcsExpireTime_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GcsStagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GcsStagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GcsStagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GcsStagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LocalStagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.LocalStagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string path = 1;
* @return The path.
*/
java.lang.String getPath();
/**
* string path = 1;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.LocalStagingPathInfo}
*/
public static final class LocalStagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.LocalStagingPathInfo)
LocalStagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocalStagingPathInfo.newBuilder() to construct.
private LocalStagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocalStagingPathInfo() {
path_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LocalStagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocalStagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
path_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_LocalStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_LocalStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder.class);
}
public static final int PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object path_;
/**
* string path = 1;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, path_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(path_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, path_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) obj;
if (!getPath()
.equals(other.getPath())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.LocalStagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.LocalStagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_LocalStagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_LocalStagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
path_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_LocalStagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo(this);
result.path_ = path_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance()) return this;
if (!other.getPath().isEmpty()) {
path_ = other.path_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object path_ = "";
/**
* string path = 1;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string path = 1;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string path = 1;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
onChanged();
return this;
}
/**
* string path = 1;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* string path = 1;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
path_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.LocalStagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.LocalStagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocalStagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocalStagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StagingPathInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.StagingPathInfo)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return Whether the ossPath field is set.
*/
boolean hasOssPath();
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return The ossPath.
*/
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getOssPath();
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder getOssPathOrBuilder();
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return Whether the cosPath field is set.
*/
boolean hasCosPath();
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return The cosPath.
*/
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getCosPath();
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder getCosPathOrBuilder();
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return Whether the localPath field is set.
*/
boolean hasLocalPath();
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return The localPath.
*/
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getLocalPath();
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder getLocalPathOrBuilder();
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return Whether the s3Path field is set.
*/
boolean hasS3Path();
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return The s3Path.
*/
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getS3Path();
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder getS3PathOrBuilder();
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return Whether the gcsPath field is set.
*/
boolean hasGcsPath();
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return The gcsPath.
*/
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getGcsPath();
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder getGcsPathOrBuilder();
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.PathInfoCase getPathInfoCase();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.StagingPathInfo}
*/
public static final class StagingPathInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.StagingPathInfo)
StagingPathInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use StagingPathInfo.newBuilder() to construct.
private StagingPathInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StagingPathInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StagingPathInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StagingPathInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder subBuilder = null;
if (pathInfoCase_ == 1) {
subBuilder = ((cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_).toBuilder();
}
pathInfo_ =
input.readMessage(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_);
pathInfo_ = subBuilder.buildPartial();
}
pathInfoCase_ = 1;
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder subBuilder = null;
if (pathInfoCase_ == 2) {
subBuilder = ((cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_).toBuilder();
}
pathInfo_ =
input.readMessage(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_);
pathInfo_ = subBuilder.buildPartial();
}
pathInfoCase_ = 2;
break;
}
case 26: {
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder subBuilder = null;
if (pathInfoCase_ == 3) {
subBuilder = ((cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_).toBuilder();
}
pathInfo_ =
input.readMessage(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_);
pathInfo_ = subBuilder.buildPartial();
}
pathInfoCase_ = 3;
break;
}
case 34: {
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder subBuilder = null;
if (pathInfoCase_ == 4) {
subBuilder = ((cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_).toBuilder();
}
pathInfo_ =
input.readMessage(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_);
pathInfo_ = subBuilder.buildPartial();
}
pathInfoCase_ = 4;
break;
}
case 42: {
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder subBuilder = null;
if (pathInfoCase_ == 5) {
subBuilder = ((cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_).toBuilder();
}
pathInfo_ =
input.readMessage(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_);
pathInfo_ = subBuilder.buildPartial();
}
pathInfoCase_ = 5;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder.class);
}
private int pathInfoCase_ = 0;
private java.lang.Object pathInfo_;
public enum PathInfoCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
OSS_PATH(1),
COS_PATH(2),
LOCAL_PATH(3),
S3_PATH(4),
GCS_PATH(5),
PATHINFO_NOT_SET(0);
private final int value;
private PathInfoCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PathInfoCase valueOf(int value) {
return forNumber(value);
}
public static PathInfoCase forNumber(int value) {
switch (value) {
case 1: return OSS_PATH;
case 2: return COS_PATH;
case 3: return LOCAL_PATH;
case 4: return S3_PATH;
case 5: return GCS_PATH;
case 0: return PATHINFO_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PathInfoCase
getPathInfoCase() {
return PathInfoCase.forNumber(
pathInfoCase_);
}
public static final int OSS_PATH_FIELD_NUMBER = 1;
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return Whether the ossPath field is set.
*/
@java.lang.Override
public boolean hasOssPath() {
return pathInfoCase_ == 1;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return The ossPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getOssPath() {
if (pathInfoCase_ == 1) {
return (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder getOssPathOrBuilder() {
if (pathInfoCase_ == 1) {
return (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
public static final int COS_PATH_FIELD_NUMBER = 2;
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return Whether the cosPath field is set.
*/
@java.lang.Override
public boolean hasCosPath() {
return pathInfoCase_ == 2;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return The cosPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getCosPath() {
if (pathInfoCase_ == 2) {
return (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder getCosPathOrBuilder() {
if (pathInfoCase_ == 2) {
return (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
public static final int LOCAL_PATH_FIELD_NUMBER = 3;
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return Whether the localPath field is set.
*/
@java.lang.Override
public boolean hasLocalPath() {
return pathInfoCase_ == 3;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return The localPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getLocalPath() {
if (pathInfoCase_ == 3) {
return (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder getLocalPathOrBuilder() {
if (pathInfoCase_ == 3) {
return (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
public static final int S3_PATH_FIELD_NUMBER = 4;
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return Whether the s3Path field is set.
*/
@java.lang.Override
public boolean hasS3Path() {
return pathInfoCase_ == 4;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return The s3Path.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getS3Path() {
if (pathInfoCase_ == 4) {
return (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder getS3PathOrBuilder() {
if (pathInfoCase_ == 4) {
return (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
public static final int GCS_PATH_FIELD_NUMBER = 5;
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return Whether the gcsPath field is set.
*/
@java.lang.Override
public boolean hasGcsPath() {
return pathInfoCase_ == 5;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return The gcsPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getGcsPath() {
if (pathInfoCase_ == 5) {
return (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder getGcsPathOrBuilder() {
if (pathInfoCase_ == 5) {
return (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (pathInfoCase_ == 1) {
output.writeMessage(1, (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 2) {
output.writeMessage(2, (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 3) {
output.writeMessage(3, (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 4) {
output.writeMessage(4, (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 5) {
output.writeMessage(5, (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (pathInfoCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_);
}
if (pathInfoCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.StagingPathInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo other = (cz.proto.ingestion.v2.IngestionV2.StagingPathInfo) obj;
if (!getPathInfoCase().equals(other.getPathInfoCase())) return false;
switch (pathInfoCase_) {
case 1:
if (!getOssPath()
.equals(other.getOssPath())) return false;
break;
case 2:
if (!getCosPath()
.equals(other.getCosPath())) return false;
break;
case 3:
if (!getLocalPath()
.equals(other.getLocalPath())) return false;
break;
case 4:
if (!getS3Path()
.equals(other.getS3Path())) return false;
break;
case 5:
if (!getGcsPath()
.equals(other.getGcsPath())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (pathInfoCase_) {
case 1:
hash = (37 * hash) + OSS_PATH_FIELD_NUMBER;
hash = (53 * hash) + getOssPath().hashCode();
break;
case 2:
hash = (37 * hash) + COS_PATH_FIELD_NUMBER;
hash = (53 * hash) + getCosPath().hashCode();
break;
case 3:
hash = (37 * hash) + LOCAL_PATH_FIELD_NUMBER;
hash = (53 * hash) + getLocalPath().hashCode();
break;
case 4:
hash = (37 * hash) + S3_PATH_FIELD_NUMBER;
hash = (53 * hash) + getS3Path().hashCode();
break;
case 5:
hash = (37 * hash) + GCS_PATH_FIELD_NUMBER;
hash = (53 * hash) + getGcsPath().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.StagingPathInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.StagingPathInfo)
cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StagingPathInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StagingPathInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.class, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
pathInfoCase_ = 0;
pathInfo_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_StagingPathInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo build() {
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo result = new cz.proto.ingestion.v2.IngestionV2.StagingPathInfo(this);
if (pathInfoCase_ == 1) {
if (ossPathBuilder_ == null) {
result.pathInfo_ = pathInfo_;
} else {
result.pathInfo_ = ossPathBuilder_.build();
}
}
if (pathInfoCase_ == 2) {
if (cosPathBuilder_ == null) {
result.pathInfo_ = pathInfo_;
} else {
result.pathInfo_ = cosPathBuilder_.build();
}
}
if (pathInfoCase_ == 3) {
if (localPathBuilder_ == null) {
result.pathInfo_ = pathInfo_;
} else {
result.pathInfo_ = localPathBuilder_.build();
}
}
if (pathInfoCase_ == 4) {
if (s3PathBuilder_ == null) {
result.pathInfo_ = pathInfo_;
} else {
result.pathInfo_ = s3PathBuilder_.build();
}
}
if (pathInfoCase_ == 5) {
if (gcsPathBuilder_ == null) {
result.pathInfo_ = pathInfo_;
} else {
result.pathInfo_ = gcsPathBuilder_.build();
}
}
result.pathInfoCase_ = pathInfoCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.StagingPathInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.StagingPathInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance()) return this;
switch (other.getPathInfoCase()) {
case OSS_PATH: {
mergeOssPath(other.getOssPath());
break;
}
case COS_PATH: {
mergeCosPath(other.getCosPath());
break;
}
case LOCAL_PATH: {
mergeLocalPath(other.getLocalPath());
break;
}
case S3_PATH: {
mergeS3Path(other.getS3Path());
break;
}
case GCS_PATH: {
mergeGcsPath(other.getGcsPath());
break;
}
case PATHINFO_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.StagingPathInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int pathInfoCase_ = 0;
private java.lang.Object pathInfo_;
public PathInfoCase
getPathInfoCase() {
return PathInfoCase.forNumber(
pathInfoCase_);
}
public Builder clearPathInfo() {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder> ossPathBuilder_;
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return Whether the ossPath field is set.
*/
@java.lang.Override
public boolean hasOssPath() {
return pathInfoCase_ == 1;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
* @return The ossPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo getOssPath() {
if (ossPathBuilder_ == null) {
if (pathInfoCase_ == 1) {
return (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
} else {
if (pathInfoCase_ == 1) {
return ossPathBuilder_.getMessage();
}
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
public Builder setOssPath(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo value) {
if (ossPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pathInfo_ = value;
onChanged();
} else {
ossPathBuilder_.setMessage(value);
}
pathInfoCase_ = 1;
return this;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
public Builder setOssPath(
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder builderForValue) {
if (ossPathBuilder_ == null) {
pathInfo_ = builderForValue.build();
onChanged();
} else {
ossPathBuilder_.setMessage(builderForValue.build());
}
pathInfoCase_ = 1;
return this;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
public Builder mergeOssPath(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo value) {
if (ossPathBuilder_ == null) {
if (pathInfoCase_ == 1 &&
pathInfo_ != cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance()) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.newBuilder((cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_)
.mergeFrom(value).buildPartial();
} else {
pathInfo_ = value;
}
onChanged();
} else {
if (pathInfoCase_ == 1) {
ossPathBuilder_.mergeFrom(value);
}
ossPathBuilder_.setMessage(value);
}
pathInfoCase_ = 1;
return this;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
public Builder clearOssPath() {
if (ossPathBuilder_ == null) {
if (pathInfoCase_ == 1) {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
}
} else {
if (pathInfoCase_ == 1) {
pathInfoCase_ = 0;
pathInfo_ = null;
}
ossPathBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder getOssPathBuilder() {
return getOssPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder getOssPathOrBuilder() {
if ((pathInfoCase_ == 1) && (ossPathBuilder_ != null)) {
return ossPathBuilder_.getMessageOrBuilder();
} else {
if (pathInfoCase_ == 1) {
return (cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.OssStagingPathInfo oss_path = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder>
getOssPathFieldBuilder() {
if (ossPathBuilder_ == null) {
if (!(pathInfoCase_ == 1)) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.getDefaultInstance();
}
ossPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfoOrBuilder>(
(cz.proto.ingestion.v2.IngestionV2.OssStagingPathInfo) pathInfo_,
getParentForChildren(),
isClean());
pathInfo_ = null;
}
pathInfoCase_ = 1;
onChanged();;
return ossPathBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder> cosPathBuilder_;
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return Whether the cosPath field is set.
*/
@java.lang.Override
public boolean hasCosPath() {
return pathInfoCase_ == 2;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
* @return The cosPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo getCosPath() {
if (cosPathBuilder_ == null) {
if (pathInfoCase_ == 2) {
return (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
} else {
if (pathInfoCase_ == 2) {
return cosPathBuilder_.getMessage();
}
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
public Builder setCosPath(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo value) {
if (cosPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pathInfo_ = value;
onChanged();
} else {
cosPathBuilder_.setMessage(value);
}
pathInfoCase_ = 2;
return this;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
public Builder setCosPath(
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder builderForValue) {
if (cosPathBuilder_ == null) {
pathInfo_ = builderForValue.build();
onChanged();
} else {
cosPathBuilder_.setMessage(builderForValue.build());
}
pathInfoCase_ = 2;
return this;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
public Builder mergeCosPath(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo value) {
if (cosPathBuilder_ == null) {
if (pathInfoCase_ == 2 &&
pathInfo_ != cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance()) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.newBuilder((cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_)
.mergeFrom(value).buildPartial();
} else {
pathInfo_ = value;
}
onChanged();
} else {
if (pathInfoCase_ == 2) {
cosPathBuilder_.mergeFrom(value);
}
cosPathBuilder_.setMessage(value);
}
pathInfoCase_ = 2;
return this;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
public Builder clearCosPath() {
if (cosPathBuilder_ == null) {
if (pathInfoCase_ == 2) {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
}
} else {
if (pathInfoCase_ == 2) {
pathInfoCase_ = 0;
pathInfo_ = null;
}
cosPathBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder getCosPathBuilder() {
return getCosPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder getCosPathOrBuilder() {
if ((pathInfoCase_ == 2) && (cosPathBuilder_ != null)) {
return cosPathBuilder_.getMessageOrBuilder();
} else {
if (pathInfoCase_ == 2) {
return (cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.CosStagingPathInfo cos_path = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder>
getCosPathFieldBuilder() {
if (cosPathBuilder_ == null) {
if (!(pathInfoCase_ == 2)) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.getDefaultInstance();
}
cosPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfoOrBuilder>(
(cz.proto.ingestion.v2.IngestionV2.CosStagingPathInfo) pathInfo_,
getParentForChildren(),
isClean());
pathInfo_ = null;
}
pathInfoCase_ = 2;
onChanged();;
return cosPathBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder> localPathBuilder_;
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return Whether the localPath field is set.
*/
@java.lang.Override
public boolean hasLocalPath() {
return pathInfoCase_ == 3;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
* @return The localPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo getLocalPath() {
if (localPathBuilder_ == null) {
if (pathInfoCase_ == 3) {
return (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
} else {
if (pathInfoCase_ == 3) {
return localPathBuilder_.getMessage();
}
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
public Builder setLocalPath(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo value) {
if (localPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pathInfo_ = value;
onChanged();
} else {
localPathBuilder_.setMessage(value);
}
pathInfoCase_ = 3;
return this;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
public Builder setLocalPath(
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder builderForValue) {
if (localPathBuilder_ == null) {
pathInfo_ = builderForValue.build();
onChanged();
} else {
localPathBuilder_.setMessage(builderForValue.build());
}
pathInfoCase_ = 3;
return this;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
public Builder mergeLocalPath(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo value) {
if (localPathBuilder_ == null) {
if (pathInfoCase_ == 3 &&
pathInfo_ != cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance()) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.newBuilder((cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_)
.mergeFrom(value).buildPartial();
} else {
pathInfo_ = value;
}
onChanged();
} else {
if (pathInfoCase_ == 3) {
localPathBuilder_.mergeFrom(value);
}
localPathBuilder_.setMessage(value);
}
pathInfoCase_ = 3;
return this;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
public Builder clearLocalPath() {
if (localPathBuilder_ == null) {
if (pathInfoCase_ == 3) {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
}
} else {
if (pathInfoCase_ == 3) {
pathInfoCase_ = 0;
pathInfo_ = null;
}
localPathBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder getLocalPathBuilder() {
return getLocalPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder getLocalPathOrBuilder() {
if ((pathInfoCase_ == 3) && (localPathBuilder_ != null)) {
return localPathBuilder_.getMessageOrBuilder();
} else {
if (pathInfoCase_ == 3) {
return (cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.LocalStagingPathInfo local_path = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder>
getLocalPathFieldBuilder() {
if (localPathBuilder_ == null) {
if (!(pathInfoCase_ == 3)) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.getDefaultInstance();
}
localPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfoOrBuilder>(
(cz.proto.ingestion.v2.IngestionV2.LocalStagingPathInfo) pathInfo_,
getParentForChildren(),
isClean());
pathInfo_ = null;
}
pathInfoCase_ = 3;
onChanged();;
return localPathBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder> s3PathBuilder_;
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return Whether the s3Path field is set.
*/
@java.lang.Override
public boolean hasS3Path() {
return pathInfoCase_ == 4;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
* @return The s3Path.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo getS3Path() {
if (s3PathBuilder_ == null) {
if (pathInfoCase_ == 4) {
return (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
} else {
if (pathInfoCase_ == 4) {
return s3PathBuilder_.getMessage();
}
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
public Builder setS3Path(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo value) {
if (s3PathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pathInfo_ = value;
onChanged();
} else {
s3PathBuilder_.setMessage(value);
}
pathInfoCase_ = 4;
return this;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
public Builder setS3Path(
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder builderForValue) {
if (s3PathBuilder_ == null) {
pathInfo_ = builderForValue.build();
onChanged();
} else {
s3PathBuilder_.setMessage(builderForValue.build());
}
pathInfoCase_ = 4;
return this;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
public Builder mergeS3Path(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo value) {
if (s3PathBuilder_ == null) {
if (pathInfoCase_ == 4 &&
pathInfo_ != cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance()) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.newBuilder((cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_)
.mergeFrom(value).buildPartial();
} else {
pathInfo_ = value;
}
onChanged();
} else {
if (pathInfoCase_ == 4) {
s3PathBuilder_.mergeFrom(value);
}
s3PathBuilder_.setMessage(value);
}
pathInfoCase_ = 4;
return this;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
public Builder clearS3Path() {
if (s3PathBuilder_ == null) {
if (pathInfoCase_ == 4) {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
}
} else {
if (pathInfoCase_ == 4) {
pathInfoCase_ = 0;
pathInfo_ = null;
}
s3PathBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder getS3PathBuilder() {
return getS3PathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder getS3PathOrBuilder() {
if ((pathInfoCase_ == 4) && (s3PathBuilder_ != null)) {
return s3PathBuilder_.getMessageOrBuilder();
} else {
if (pathInfoCase_ == 4) {
return (cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.S3StagingPathInfo s3_path = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder>
getS3PathFieldBuilder() {
if (s3PathBuilder_ == null) {
if (!(pathInfoCase_ == 4)) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.getDefaultInstance();
}
s3PathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfoOrBuilder>(
(cz.proto.ingestion.v2.IngestionV2.S3StagingPathInfo) pathInfo_,
getParentForChildren(),
isClean());
pathInfo_ = null;
}
pathInfoCase_ = 4;
onChanged();;
return s3PathBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder> gcsPathBuilder_;
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return Whether the gcsPath field is set.
*/
@java.lang.Override
public boolean hasGcsPath() {
return pathInfoCase_ == 5;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
* @return The gcsPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo getGcsPath() {
if (gcsPathBuilder_ == null) {
if (pathInfoCase_ == 5) {
return (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
} else {
if (pathInfoCase_ == 5) {
return gcsPathBuilder_.getMessage();
}
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
public Builder setGcsPath(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo value) {
if (gcsPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pathInfo_ = value;
onChanged();
} else {
gcsPathBuilder_.setMessage(value);
}
pathInfoCase_ = 5;
return this;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
public Builder setGcsPath(
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder builderForValue) {
if (gcsPathBuilder_ == null) {
pathInfo_ = builderForValue.build();
onChanged();
} else {
gcsPathBuilder_.setMessage(builderForValue.build());
}
pathInfoCase_ = 5;
return this;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
public Builder mergeGcsPath(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo value) {
if (gcsPathBuilder_ == null) {
if (pathInfoCase_ == 5 &&
pathInfo_ != cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance()) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.newBuilder((cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_)
.mergeFrom(value).buildPartial();
} else {
pathInfo_ = value;
}
onChanged();
} else {
if (pathInfoCase_ == 5) {
gcsPathBuilder_.mergeFrom(value);
}
gcsPathBuilder_.setMessage(value);
}
pathInfoCase_ = 5;
return this;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
public Builder clearGcsPath() {
if (gcsPathBuilder_ == null) {
if (pathInfoCase_ == 5) {
pathInfoCase_ = 0;
pathInfo_ = null;
onChanged();
}
} else {
if (pathInfoCase_ == 5) {
pathInfoCase_ = 0;
pathInfo_ = null;
}
gcsPathBuilder_.clear();
}
return this;
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder getGcsPathBuilder() {
return getGcsPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder getGcsPathOrBuilder() {
if ((pathInfoCase_ == 5) && (gcsPathBuilder_ != null)) {
return gcsPathBuilder_.getMessageOrBuilder();
} else {
if (pathInfoCase_ == 5) {
return (cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_;
}
return cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
}
/**
* .cz.proto.ingestion.v2.GcsStagingPathInfo gcs_path = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder>
getGcsPathFieldBuilder() {
if (gcsPathBuilder_ == null) {
if (!(pathInfoCase_ == 5)) {
pathInfo_ = cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.getDefaultInstance();
}
gcsPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfoOrBuilder>(
(cz.proto.ingestion.v2.IngestionV2.GcsStagingPathInfo) pathInfo_,
getParentForChildren(),
isClean());
pathInfo_ = null;
}
pathInfoCase_ = 5;
onChanged();;
return gcsPathBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.StagingPathInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.StagingPathInfo)
private static final cz.proto.ingestion.v2.IngestionV2.StagingPathInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.StagingPathInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StagingPathInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StagingPathInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BulkLoadStreamInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.BulkLoadStreamInfo)
com.google.protobuf.MessageOrBuilder {
/**
* string stream_id = 1;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 1;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The enum numeric value on the wire for streamState.
*/
int getStreamStateValue();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The streamState.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState getStreamState();
/**
* string sql_job_id = 3;
* @return The sqlJobId.
*/
java.lang.String getSqlJobId();
/**
* string sql_job_id = 3;
* @return The bytes for sqlJobId.
*/
com.google.protobuf.ByteString
getSqlJobIdBytes();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The enum numeric value on the wire for operation.
*/
int getOperationValue();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The operation.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation();
/**
* string partition_spec = 6;
* @return The partitionSpec.
*/
java.lang.String getPartitionSpec();
/**
* string partition_spec = 6;
* @return The bytes for partitionSpec.
*/
com.google.protobuf.ByteString
getPartitionSpecBytes();
/**
* repeated string record_keys = 7;
* @return A list containing the recordKeys.
*/
java.util.List
getRecordKeysList();
/**
* repeated string record_keys = 7;
* @return The count of recordKeys.
*/
int getRecordKeysCount();
/**
* repeated string record_keys = 7;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
java.lang.String getRecordKeys(int index);
/**
* repeated string record_keys = 7;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
com.google.protobuf.ByteString
getRecordKeysBytes(int index);
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return Whether the streamSchema field is set.
*/
boolean hasStreamSchema();
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return The streamSchema.
*/
cz.proto.ingestion.v2.IngestionV2.StreamSchema getStreamSchema();
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getStreamSchemaOrBuilder();
/**
* string sql_error_msg = 9;
* @return The sqlErrorMsg.
*/
java.lang.String getSqlErrorMsg();
/**
* string sql_error_msg = 9;
* @return The bytes for sqlErrorMsg.
*/
com.google.protobuf.ByteString
getSqlErrorMsgBytes();
/**
* bool prefer_internal_endpoint = 10;
* @return The preferInternalEndpoint.
*/
boolean getPreferInternalEndpoint();
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
int getEncryptionOptionsCount();
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
boolean containsEncryptionOptions(
java.lang.String key);
/**
* Use {@link #getEncryptionOptionsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getEncryptionOptions();
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
java.util.Map
getEncryptionOptionsMap();
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
java.lang.String getEncryptionOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
java.lang.String getEncryptionOptionsOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.BulkLoadStreamInfo}
*/
public static final class BulkLoadStreamInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.BulkLoadStreamInfo)
BulkLoadStreamInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use BulkLoadStreamInfo.newBuilder() to construct.
private BulkLoadStreamInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BulkLoadStreamInfo() {
streamId_ = "";
streamState_ = 0;
sqlJobId_ = "";
operation_ = 0;
partitionSpec_ = "";
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
sqlErrorMsg_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BulkLoadStreamInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BulkLoadStreamInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
streamState_ = rawValue;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
sqlJobId_ = s;
break;
}
case 34: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 40: {
int rawValue = input.readEnum();
operation_ = rawValue;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
partitionSpec_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
recordKeys_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
recordKeys_.add(s);
break;
}
case 66: {
cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder subBuilder = null;
if (streamSchema_ != null) {
subBuilder = streamSchema_.toBuilder();
}
streamSchema_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.StreamSchema.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(streamSchema_);
streamSchema_ = subBuilder.buildPartial();
}
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
sqlErrorMsg_ = s;
break;
}
case 80: {
preferInternalEndpoint_ = input.readBool();
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
encryptionOptions_ = com.google.protobuf.MapField.newMapField(
EncryptionOptionsDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
encryptionOptions__ = input.readMessage(
EncryptionOptionsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
encryptionOptions_.getMutableMap().put(
encryptionOptions__.getKey(), encryptionOptions__.getValue());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
recordKeys_ = recordKeys_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 11:
return internalGetEncryptionOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.class, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder.class);
}
public static final int STREAM_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 1;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 1;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STREAM_STATE_FIELD_NUMBER = 2;
private int streamState_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The enum numeric value on the wire for streamState.
*/
@java.lang.Override public int getStreamStateValue() {
return streamState_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The streamState.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState getStreamState() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.valueOf(streamState_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.UNRECOGNIZED : result;
}
public static final int SQL_JOB_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object sqlJobId_;
/**
* string sql_job_id = 3;
* @return The sqlJobId.
*/
@java.lang.Override
public java.lang.String getSqlJobId() {
java.lang.Object ref = sqlJobId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sqlJobId_ = s;
return s;
}
}
/**
* string sql_job_id = 3;
* @return The bytes for sqlJobId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSqlJobIdBytes() {
java.lang.Object ref = sqlJobId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sqlJobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IDENTIFIER_FIELD_NUMBER = 4;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int OPERATION_FIELD_NUMBER = 5;
private int operation_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The operation.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.valueOf(operation_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.UNRECOGNIZED : result;
}
public static final int PARTITION_SPEC_FIELD_NUMBER = 6;
private volatile java.lang.Object partitionSpec_;
/**
* string partition_spec = 6;
* @return The partitionSpec.
*/
@java.lang.Override
public java.lang.String getPartitionSpec() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partitionSpec_ = s;
return s;
}
}
/**
* string partition_spec = 6;
* @return The bytes for partitionSpec.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPartitionSpecBytes() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partitionSpec_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RECORD_KEYS_FIELD_NUMBER = 7;
private com.google.protobuf.LazyStringList recordKeys_;
/**
* repeated string record_keys = 7;
* @return A list containing the recordKeys.
*/
public com.google.protobuf.ProtocolStringList
getRecordKeysList() {
return recordKeys_;
}
/**
* repeated string record_keys = 7;
* @return The count of recordKeys.
*/
public int getRecordKeysCount() {
return recordKeys_.size();
}
/**
* repeated string record_keys = 7;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
public java.lang.String getRecordKeys(int index) {
return recordKeys_.get(index);
}
/**
* repeated string record_keys = 7;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
public com.google.protobuf.ByteString
getRecordKeysBytes(int index) {
return recordKeys_.getByteString(index);
}
public static final int STREAM_SCHEMA_FIELD_NUMBER = 8;
private cz.proto.ingestion.v2.IngestionV2.StreamSchema streamSchema_;
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return Whether the streamSchema field is set.
*/
@java.lang.Override
public boolean hasStreamSchema() {
return streamSchema_ != null;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return The streamSchema.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getStreamSchema() {
return streamSchema_ == null ? cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : streamSchema_;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getStreamSchemaOrBuilder() {
return getStreamSchema();
}
public static final int SQL_ERROR_MSG_FIELD_NUMBER = 9;
private volatile java.lang.Object sqlErrorMsg_;
/**
* string sql_error_msg = 9;
* @return The sqlErrorMsg.
*/
@java.lang.Override
public java.lang.String getSqlErrorMsg() {
java.lang.Object ref = sqlErrorMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sqlErrorMsg_ = s;
return s;
}
}
/**
* string sql_error_msg = 9;
* @return The bytes for sqlErrorMsg.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSqlErrorMsgBytes() {
java.lang.Object ref = sqlErrorMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sqlErrorMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PREFER_INTERNAL_ENDPOINT_FIELD_NUMBER = 10;
private boolean preferInternalEndpoint_;
/**
* bool prefer_internal_endpoint = 10;
* @return The preferInternalEndpoint.
*/
@java.lang.Override
public boolean getPreferInternalEndpoint() {
return preferInternalEndpoint_;
}
public static final int ENCRYPTION_OPTIONS_FIELD_NUMBER = 11;
private static final class EncryptionOptionsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_EncryptionOptionsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> encryptionOptions_;
private com.google.protobuf.MapField
internalGetEncryptionOptions() {
if (encryptionOptions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EncryptionOptionsDefaultEntryHolder.defaultEntry);
}
return encryptionOptions_;
}
public int getEncryptionOptionsCount() {
return internalGetEncryptionOptions().getMap().size();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public boolean containsEncryptionOptions(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetEncryptionOptions().getMap().containsKey(key);
}
/**
* Use {@link #getEncryptionOptionsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getEncryptionOptions() {
return getEncryptionOptionsMap();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.util.Map getEncryptionOptionsMap() {
return internalGetEncryptionOptions().getMap();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.lang.String getEncryptionOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetEncryptionOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.lang.String getEncryptionOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetEncryptionOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, streamId_);
}
if (streamState_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.BL_CREATED.getNumber()) {
output.writeEnum(2, streamState_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sqlJobId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, sqlJobId_);
}
if (identifier_ != null) {
output.writeMessage(4, getIdentifier());
}
if (operation_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.BL_APPEND.getNumber()) {
output.writeEnum(5, operation_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(partitionSpec_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, partitionSpec_);
}
for (int i = 0; i < recordKeys_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, recordKeys_.getRaw(i));
}
if (streamSchema_ != null) {
output.writeMessage(8, getStreamSchema());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sqlErrorMsg_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, sqlErrorMsg_);
}
if (preferInternalEndpoint_ != false) {
output.writeBool(10, preferInternalEndpoint_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetEncryptionOptions(),
EncryptionOptionsDefaultEntryHolder.defaultEntry,
11);
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, streamId_);
}
if (streamState_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.BL_CREATED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, streamState_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sqlJobId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, sqlJobId_);
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getIdentifier());
}
if (operation_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.BL_APPEND.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, operation_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(partitionSpec_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, partitionSpec_);
}
{
int dataSize = 0;
for (int i = 0; i < recordKeys_.size(); i++) {
dataSize += computeStringSizeNoTag(recordKeys_.getRaw(i));
}
size += dataSize;
size += 1 * getRecordKeysList().size();
}
if (streamSchema_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getStreamSchema());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sqlErrorMsg_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, sqlErrorMsg_);
}
if (preferInternalEndpoint_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, preferInternalEndpoint_);
}
for (java.util.Map.Entry entry
: internalGetEncryptionOptions().getMap().entrySet()) {
com.google.protobuf.MapEntry
encryptionOptions__ = EncryptionOptionsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, encryptionOptions__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo other = (cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo) obj;
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (streamState_ != other.streamState_) return false;
if (!getSqlJobId()
.equals(other.getSqlJobId())) return false;
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (operation_ != other.operation_) return false;
if (!getPartitionSpec()
.equals(other.getPartitionSpec())) return false;
if (!getRecordKeysList()
.equals(other.getRecordKeysList())) return false;
if (hasStreamSchema() != other.hasStreamSchema()) return false;
if (hasStreamSchema()) {
if (!getStreamSchema()
.equals(other.getStreamSchema())) return false;
}
if (!getSqlErrorMsg()
.equals(other.getSqlErrorMsg())) return false;
if (getPreferInternalEndpoint()
!= other.getPreferInternalEndpoint()) return false;
if (!internalGetEncryptionOptions().equals(
other.internalGetEncryptionOptions())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (37 * hash) + STREAM_STATE_FIELD_NUMBER;
hash = (53 * hash) + streamState_;
hash = (37 * hash) + SQL_JOB_ID_FIELD_NUMBER;
hash = (53 * hash) + getSqlJobId().hashCode();
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + OPERATION_FIELD_NUMBER;
hash = (53 * hash) + operation_;
hash = (37 * hash) + PARTITION_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getPartitionSpec().hashCode();
if (getRecordKeysCount() > 0) {
hash = (37 * hash) + RECORD_KEYS_FIELD_NUMBER;
hash = (53 * hash) + getRecordKeysList().hashCode();
}
if (hasStreamSchema()) {
hash = (37 * hash) + STREAM_SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getStreamSchema().hashCode();
}
hash = (37 * hash) + SQL_ERROR_MSG_FIELD_NUMBER;
hash = (53 * hash) + getSqlErrorMsg().hashCode();
hash = (37 * hash) + PREFER_INTERNAL_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPreferInternalEndpoint());
if (!internalGetEncryptionOptions().getMap().isEmpty()) {
hash = (37 * hash) + ENCRYPTION_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + internalGetEncryptionOptions().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.BulkLoadStreamInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.BulkLoadStreamInfo)
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 11:
return internalGetEncryptionOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 11:
return internalGetMutableEncryptionOptions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.class, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
streamId_ = "";
streamState_ = 0;
sqlJobId_ = "";
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
operation_ = 0;
partitionSpec_ = "";
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (streamSchemaBuilder_ == null) {
streamSchema_ = null;
} else {
streamSchema_ = null;
streamSchemaBuilder_ = null;
}
sqlErrorMsg_ = "";
preferInternalEndpoint_ = false;
internalGetMutableEncryptionOptions().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamInfo_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo build() {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo buildPartial() {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo result = new cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo(this);
int from_bitField0_ = bitField0_;
result.streamId_ = streamId_;
result.streamState_ = streamState_;
result.sqlJobId_ = sqlJobId_;
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.operation_ = operation_;
result.partitionSpec_ = partitionSpec_;
if (((bitField0_ & 0x00000001) != 0)) {
recordKeys_ = recordKeys_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.recordKeys_ = recordKeys_;
if (streamSchemaBuilder_ == null) {
result.streamSchema_ = streamSchema_;
} else {
result.streamSchema_ = streamSchemaBuilder_.build();
}
result.sqlErrorMsg_ = sqlErrorMsg_;
result.preferInternalEndpoint_ = preferInternalEndpoint_;
result.encryptionOptions_ = internalGetEncryptionOptions();
result.encryptionOptions_.makeImmutable();
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo other) {
if (other == cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance()) return this;
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
if (other.streamState_ != 0) {
setStreamStateValue(other.getStreamStateValue());
}
if (!other.getSqlJobId().isEmpty()) {
sqlJobId_ = other.sqlJobId_;
onChanged();
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (other.operation_ != 0) {
setOperationValue(other.getOperationValue());
}
if (!other.getPartitionSpec().isEmpty()) {
partitionSpec_ = other.partitionSpec_;
onChanged();
}
if (!other.recordKeys_.isEmpty()) {
if (recordKeys_.isEmpty()) {
recordKeys_ = other.recordKeys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRecordKeysIsMutable();
recordKeys_.addAll(other.recordKeys_);
}
onChanged();
}
if (other.hasStreamSchema()) {
mergeStreamSchema(other.getStreamSchema());
}
if (!other.getSqlErrorMsg().isEmpty()) {
sqlErrorMsg_ = other.sqlErrorMsg_;
onChanged();
}
if (other.getPreferInternalEndpoint() != false) {
setPreferInternalEndpoint(other.getPreferInternalEndpoint());
}
internalGetMutableEncryptionOptions().mergeFrom(
other.internalGetEncryptionOptions());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object streamId_ = "";
/**
* string stream_id = 1;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 1;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 1;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 1;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 1;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
private int streamState_ = 0;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The enum numeric value on the wire for streamState.
*/
@java.lang.Override public int getStreamStateValue() {
return streamState_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @param value The enum numeric value on the wire for streamState to set.
* @return This builder for chaining.
*/
public Builder setStreamStateValue(int value) {
streamState_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return The streamState.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState getStreamState() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.valueOf(streamState_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @param value The streamState to set.
* @return This builder for chaining.
*/
public Builder setStreamState(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamState value) {
if (value == null) {
throw new NullPointerException();
}
streamState_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamState stream_state = 2;
* @return This builder for chaining.
*/
public Builder clearStreamState() {
streamState_ = 0;
onChanged();
return this;
}
private java.lang.Object sqlJobId_ = "";
/**
* string sql_job_id = 3;
* @return The sqlJobId.
*/
public java.lang.String getSqlJobId() {
java.lang.Object ref = sqlJobId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sqlJobId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sql_job_id = 3;
* @return The bytes for sqlJobId.
*/
public com.google.protobuf.ByteString
getSqlJobIdBytes() {
java.lang.Object ref = sqlJobId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sqlJobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sql_job_id = 3;
* @param value The sqlJobId to set.
* @return This builder for chaining.
*/
public Builder setSqlJobId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sqlJobId_ = value;
onChanged();
return this;
}
/**
* string sql_job_id = 3;
* @return This builder for chaining.
*/
public Builder clearSqlJobId() {
sqlJobId_ = getDefaultInstance().getSqlJobId();
onChanged();
return this;
}
/**
* string sql_job_id = 3;
* @param value The bytes for sqlJobId to set.
* @return This builder for chaining.
*/
public Builder setSqlJobIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sqlJobId_ = value;
onChanged();
return this;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private int operation_ = 0;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @param value The enum numeric value on the wire for operation to set.
* @return This builder for chaining.
*/
public Builder setOperationValue(int value) {
operation_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return The operation.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.valueOf(operation_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @param value The operation to set.
* @return This builder for chaining.
*/
public Builder setOperation(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation value) {
if (value == null) {
throw new NullPointerException();
}
operation_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 5;
* @return This builder for chaining.
*/
public Builder clearOperation() {
operation_ = 0;
onChanged();
return this;
}
private java.lang.Object partitionSpec_ = "";
/**
* string partition_spec = 6;
* @return The partitionSpec.
*/
public java.lang.String getPartitionSpec() {
java.lang.Object ref = partitionSpec_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partitionSpec_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string partition_spec = 6;
* @return The bytes for partitionSpec.
*/
public com.google.protobuf.ByteString
getPartitionSpecBytes() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partitionSpec_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string partition_spec = 6;
* @param value The partitionSpec to set.
* @return This builder for chaining.
*/
public Builder setPartitionSpec(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partitionSpec_ = value;
onChanged();
return this;
}
/**
* string partition_spec = 6;
* @return This builder for chaining.
*/
public Builder clearPartitionSpec() {
partitionSpec_ = getDefaultInstance().getPartitionSpec();
onChanged();
return this;
}
/**
* string partition_spec = 6;
* @param value The bytes for partitionSpec to set.
* @return This builder for chaining.
*/
public Builder setPartitionSpecBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partitionSpec_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRecordKeysIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
recordKeys_ = new com.google.protobuf.LazyStringArrayList(recordKeys_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string record_keys = 7;
* @return A list containing the recordKeys.
*/
public com.google.protobuf.ProtocolStringList
getRecordKeysList() {
return recordKeys_.getUnmodifiableView();
}
/**
* repeated string record_keys = 7;
* @return The count of recordKeys.
*/
public int getRecordKeysCount() {
return recordKeys_.size();
}
/**
* repeated string record_keys = 7;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
public java.lang.String getRecordKeys(int index) {
return recordKeys_.get(index);
}
/**
* repeated string record_keys = 7;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
public com.google.protobuf.ByteString
getRecordKeysBytes(int index) {
return recordKeys_.getByteString(index);
}
/**
* repeated string record_keys = 7;
* @param index The index to set the value at.
* @param value The recordKeys to set.
* @return This builder for chaining.
*/
public Builder setRecordKeys(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordKeysIsMutable();
recordKeys_.set(index, value);
onChanged();
return this;
}
/**
* repeated string record_keys = 7;
* @param value The recordKeys to add.
* @return This builder for chaining.
*/
public Builder addRecordKeys(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordKeysIsMutable();
recordKeys_.add(value);
onChanged();
return this;
}
/**
* repeated string record_keys = 7;
* @param values The recordKeys to add.
* @return This builder for chaining.
*/
public Builder addAllRecordKeys(
java.lang.Iterable values) {
ensureRecordKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, recordKeys_);
onChanged();
return this;
}
/**
* repeated string record_keys = 7;
* @return This builder for chaining.
*/
public Builder clearRecordKeys() {
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string record_keys = 7;
* @param value The bytes of the recordKeys to add.
* @return This builder for chaining.
*/
public Builder addRecordKeysBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureRecordKeysIsMutable();
recordKeys_.add(value);
onChanged();
return this;
}
private cz.proto.ingestion.v2.IngestionV2.StreamSchema streamSchema_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder> streamSchemaBuilder_;
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return Whether the streamSchema field is set.
*/
public boolean hasStreamSchema() {
return streamSchemaBuilder_ != null || streamSchema_ != null;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
* @return The streamSchema.
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchema getStreamSchema() {
if (streamSchemaBuilder_ == null) {
return streamSchema_ == null ? cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : streamSchema_;
} else {
return streamSchemaBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public Builder setStreamSchema(cz.proto.ingestion.v2.IngestionV2.StreamSchema value) {
if (streamSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
streamSchema_ = value;
onChanged();
} else {
streamSchemaBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public Builder setStreamSchema(
cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder builderForValue) {
if (streamSchemaBuilder_ == null) {
streamSchema_ = builderForValue.build();
onChanged();
} else {
streamSchemaBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public Builder mergeStreamSchema(cz.proto.ingestion.v2.IngestionV2.StreamSchema value) {
if (streamSchemaBuilder_ == null) {
if (streamSchema_ != null) {
streamSchema_ =
cz.proto.ingestion.v2.IngestionV2.StreamSchema.newBuilder(streamSchema_).mergeFrom(value).buildPartial();
} else {
streamSchema_ = value;
}
onChanged();
} else {
streamSchemaBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public Builder clearStreamSchema() {
if (streamSchemaBuilder_ == null) {
streamSchema_ = null;
onChanged();
} else {
streamSchema_ = null;
streamSchemaBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder getStreamSchemaBuilder() {
onChanged();
return getStreamSchemaFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
public cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder getStreamSchemaOrBuilder() {
if (streamSchemaBuilder_ != null) {
return streamSchemaBuilder_.getMessageOrBuilder();
} else {
return streamSchema_ == null ?
cz.proto.ingestion.v2.IngestionV2.StreamSchema.getDefaultInstance() : streamSchema_;
}
}
/**
* .cz.proto.ingestion.v2.StreamSchema stream_schema = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder>
getStreamSchemaFieldBuilder() {
if (streamSchemaBuilder_ == null) {
streamSchemaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StreamSchema, cz.proto.ingestion.v2.IngestionV2.StreamSchema.Builder, cz.proto.ingestion.v2.IngestionV2.StreamSchemaOrBuilder>(
getStreamSchema(),
getParentForChildren(),
isClean());
streamSchema_ = null;
}
return streamSchemaBuilder_;
}
private java.lang.Object sqlErrorMsg_ = "";
/**
* string sql_error_msg = 9;
* @return The sqlErrorMsg.
*/
public java.lang.String getSqlErrorMsg() {
java.lang.Object ref = sqlErrorMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sqlErrorMsg_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string sql_error_msg = 9;
* @return The bytes for sqlErrorMsg.
*/
public com.google.protobuf.ByteString
getSqlErrorMsgBytes() {
java.lang.Object ref = sqlErrorMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sqlErrorMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string sql_error_msg = 9;
* @param value The sqlErrorMsg to set.
* @return This builder for chaining.
*/
public Builder setSqlErrorMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sqlErrorMsg_ = value;
onChanged();
return this;
}
/**
* string sql_error_msg = 9;
* @return This builder for chaining.
*/
public Builder clearSqlErrorMsg() {
sqlErrorMsg_ = getDefaultInstance().getSqlErrorMsg();
onChanged();
return this;
}
/**
* string sql_error_msg = 9;
* @param value The bytes for sqlErrorMsg to set.
* @return This builder for chaining.
*/
public Builder setSqlErrorMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sqlErrorMsg_ = value;
onChanged();
return this;
}
private boolean preferInternalEndpoint_ ;
/**
* bool prefer_internal_endpoint = 10;
* @return The preferInternalEndpoint.
*/
@java.lang.Override
public boolean getPreferInternalEndpoint() {
return preferInternalEndpoint_;
}
/**
* bool prefer_internal_endpoint = 10;
* @param value The preferInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setPreferInternalEndpoint(boolean value) {
preferInternalEndpoint_ = value;
onChanged();
return this;
}
/**
* bool prefer_internal_endpoint = 10;
* @return This builder for chaining.
*/
public Builder clearPreferInternalEndpoint() {
preferInternalEndpoint_ = false;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> encryptionOptions_;
private com.google.protobuf.MapField
internalGetEncryptionOptions() {
if (encryptionOptions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EncryptionOptionsDefaultEntryHolder.defaultEntry);
}
return encryptionOptions_;
}
private com.google.protobuf.MapField
internalGetMutableEncryptionOptions() {
onChanged();;
if (encryptionOptions_ == null) {
encryptionOptions_ = com.google.protobuf.MapField.newMapField(
EncryptionOptionsDefaultEntryHolder.defaultEntry);
}
if (!encryptionOptions_.isMutable()) {
encryptionOptions_ = encryptionOptions_.copy();
}
return encryptionOptions_;
}
public int getEncryptionOptionsCount() {
return internalGetEncryptionOptions().getMap().size();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public boolean containsEncryptionOptions(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetEncryptionOptions().getMap().containsKey(key);
}
/**
* Use {@link #getEncryptionOptionsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getEncryptionOptions() {
return getEncryptionOptionsMap();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.util.Map getEncryptionOptionsMap() {
return internalGetEncryptionOptions().getMap();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.lang.String getEncryptionOptionsOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetEncryptionOptions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
@java.lang.Override
public java.lang.String getEncryptionOptionsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetEncryptionOptions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearEncryptionOptions() {
internalGetMutableEncryptionOptions().getMutableMap()
.clear();
return this;
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
public Builder removeEncryptionOptions(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableEncryptionOptions().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableEncryptionOptions() {
return internalGetMutableEncryptionOptions().getMutableMap();
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
public Builder putEncryptionOptions(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableEncryptionOptions().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Encryption config of cloud object stores.
* Use a map here as these options vary from cloud to cloud.
*
*
* map<string, string> encryption_options = 11;
*/
public Builder putAllEncryptionOptions(
java.util.Map values) {
internalGetMutableEncryptionOptions().getMutableMap()
.putAll(values);
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.BulkLoadStreamInfo)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.BulkLoadStreamInfo)
private static final cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo();
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BulkLoadStreamInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BulkLoadStreamInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BulkLoadStreamWriterConfigOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.BulkLoadStreamWriterConfig)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return Whether the stagingPath field is set.
*/
boolean hasStagingPath();
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return The stagingPath.
*/
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath();
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder();
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The enum numeric value on the wire for fileFormat.
*/
int getFileFormatValue();
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The fileFormat.
*/
cz.proto.FileFormatTypeOuterClass.FileFormatType getFileFormat();
/**
* int64 max_num_rows_per_file = 3;
* @return The maxNumRowsPerFile.
*/
long getMaxNumRowsPerFile();
/**
* int64 max_size_in_bytes_per_file = 4;
* @return The maxSizeInBytesPerFile.
*/
long getMaxSizeInBytesPerFile();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.BulkLoadStreamWriterConfig}
*/
public static final class BulkLoadStreamWriterConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.BulkLoadStreamWriterConfig)
BulkLoadStreamWriterConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use BulkLoadStreamWriterConfig.newBuilder() to construct.
private BulkLoadStreamWriterConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BulkLoadStreamWriterConfig() {
fileFormat_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BulkLoadStreamWriterConfig();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BulkLoadStreamWriterConfig(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder subBuilder = null;
if (stagingPath_ != null) {
subBuilder = stagingPath_.toBuilder();
}
stagingPath_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stagingPath_);
stagingPath_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
fileFormat_ = rawValue;
break;
}
case 24: {
maxNumRowsPerFile_ = input.readInt64();
break;
}
case 32: {
maxSizeInBytesPerFile_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamWriterConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamWriterConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.class, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder.class);
}
public static final int STAGING_PATH_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.StagingPathInfo stagingPath_;
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return Whether the stagingPath field is set.
*/
@java.lang.Override
public boolean hasStagingPath() {
return stagingPath_ != null;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return The stagingPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath() {
return stagingPath_ == null ? cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder() {
return getStagingPath();
}
public static final int FILE_FORMAT_FIELD_NUMBER = 2;
private int fileFormat_;
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The enum numeric value on the wire for fileFormat.
*/
@java.lang.Override public int getFileFormatValue() {
return fileFormat_;
}
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The fileFormat.
*/
@java.lang.Override public cz.proto.FileFormatTypeOuterClass.FileFormatType getFileFormat() {
@SuppressWarnings("deprecation")
cz.proto.FileFormatTypeOuterClass.FileFormatType result = cz.proto.FileFormatTypeOuterClass.FileFormatType.valueOf(fileFormat_);
return result == null ? cz.proto.FileFormatTypeOuterClass.FileFormatType.UNRECOGNIZED : result;
}
public static final int MAX_NUM_ROWS_PER_FILE_FIELD_NUMBER = 3;
private long maxNumRowsPerFile_;
/**
* int64 max_num_rows_per_file = 3;
* @return The maxNumRowsPerFile.
*/
@java.lang.Override
public long getMaxNumRowsPerFile() {
return maxNumRowsPerFile_;
}
public static final int MAX_SIZE_IN_BYTES_PER_FILE_FIELD_NUMBER = 4;
private long maxSizeInBytesPerFile_;
/**
* int64 max_size_in_bytes_per_file = 4;
* @return The maxSizeInBytesPerFile.
*/
@java.lang.Override
public long getMaxSizeInBytesPerFile() {
return maxSizeInBytesPerFile_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (stagingPath_ != null) {
output.writeMessage(1, getStagingPath());
}
if (fileFormat_ != cz.proto.FileFormatTypeOuterClass.FileFormatType.TEXT.getNumber()) {
output.writeEnum(2, fileFormat_);
}
if (maxNumRowsPerFile_ != 0L) {
output.writeInt64(3, maxNumRowsPerFile_);
}
if (maxSizeInBytesPerFile_ != 0L) {
output.writeInt64(4, maxSizeInBytesPerFile_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (stagingPath_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStagingPath());
}
if (fileFormat_ != cz.proto.FileFormatTypeOuterClass.FileFormatType.TEXT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, fileFormat_);
}
if (maxNumRowsPerFile_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, maxNumRowsPerFile_);
}
if (maxSizeInBytesPerFile_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, maxSizeInBytesPerFile_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig other = (cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig) obj;
if (hasStagingPath() != other.hasStagingPath()) return false;
if (hasStagingPath()) {
if (!getStagingPath()
.equals(other.getStagingPath())) return false;
}
if (fileFormat_ != other.fileFormat_) return false;
if (getMaxNumRowsPerFile()
!= other.getMaxNumRowsPerFile()) return false;
if (getMaxSizeInBytesPerFile()
!= other.getMaxSizeInBytesPerFile()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStagingPath()) {
hash = (37 * hash) + STAGING_PATH_FIELD_NUMBER;
hash = (53 * hash) + getStagingPath().hashCode();
}
hash = (37 * hash) + FILE_FORMAT_FIELD_NUMBER;
hash = (53 * hash) + fileFormat_;
hash = (37 * hash) + MAX_NUM_ROWS_PER_FILE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxNumRowsPerFile());
hash = (37 * hash) + MAX_SIZE_IN_BYTES_PER_FILE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxSizeInBytesPerFile());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.BulkLoadStreamWriterConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.BulkLoadStreamWriterConfig)
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamWriterConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamWriterConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.class, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (stagingPathBuilder_ == null) {
stagingPath_ = null;
} else {
stagingPath_ = null;
stagingPathBuilder_ = null;
}
fileFormat_ = 0;
maxNumRowsPerFile_ = 0L;
maxSizeInBytesPerFile_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_BulkLoadStreamWriterConfig_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig build() {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig buildPartial() {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig result = new cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig(this);
if (stagingPathBuilder_ == null) {
result.stagingPath_ = stagingPath_;
} else {
result.stagingPath_ = stagingPathBuilder_.build();
}
result.fileFormat_ = fileFormat_;
result.maxNumRowsPerFile_ = maxNumRowsPerFile_;
result.maxSizeInBytesPerFile_ = maxSizeInBytesPerFile_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig other) {
if (other == cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.getDefaultInstance()) return this;
if (other.hasStagingPath()) {
mergeStagingPath(other.getStagingPath());
}
if (other.fileFormat_ != 0) {
setFileFormatValue(other.getFileFormatValue());
}
if (other.getMaxNumRowsPerFile() != 0L) {
setMaxNumRowsPerFile(other.getMaxNumRowsPerFile());
}
if (other.getMaxSizeInBytesPerFile() != 0L) {
setMaxSizeInBytesPerFile(other.getMaxSizeInBytesPerFile());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.StagingPathInfo stagingPath_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder> stagingPathBuilder_;
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return Whether the stagingPath field is set.
*/
public boolean hasStagingPath() {
return stagingPathBuilder_ != null || stagingPath_ != null;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
* @return The stagingPath.
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath() {
if (stagingPathBuilder_ == null) {
return stagingPath_ == null ? cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
} else {
return stagingPathBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public Builder setStagingPath(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo value) {
if (stagingPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stagingPath_ = value;
onChanged();
} else {
stagingPathBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public Builder setStagingPath(
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder builderForValue) {
if (stagingPathBuilder_ == null) {
stagingPath_ = builderForValue.build();
onChanged();
} else {
stagingPathBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public Builder mergeStagingPath(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo value) {
if (stagingPathBuilder_ == null) {
if (stagingPath_ != null) {
stagingPath_ =
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.newBuilder(stagingPath_).mergeFrom(value).buildPartial();
} else {
stagingPath_ = value;
}
onChanged();
} else {
stagingPathBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public Builder clearStagingPath() {
if (stagingPathBuilder_ == null) {
stagingPath_ = null;
onChanged();
} else {
stagingPath_ = null;
stagingPathBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder getStagingPathBuilder() {
onChanged();
return getStagingPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder() {
if (stagingPathBuilder_ != null) {
return stagingPathBuilder_.getMessageOrBuilder();
} else {
return stagingPath_ == null ?
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
}
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder>
getStagingPathFieldBuilder() {
if (stagingPathBuilder_ == null) {
stagingPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder>(
getStagingPath(),
getParentForChildren(),
isClean());
stagingPath_ = null;
}
return stagingPathBuilder_;
}
private int fileFormat_ = 0;
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The enum numeric value on the wire for fileFormat.
*/
@java.lang.Override public int getFileFormatValue() {
return fileFormat_;
}
/**
* .cz.proto.FileFormatType file_format = 2;
* @param value The enum numeric value on the wire for fileFormat to set.
* @return This builder for chaining.
*/
public Builder setFileFormatValue(int value) {
fileFormat_ = value;
onChanged();
return this;
}
/**
* .cz.proto.FileFormatType file_format = 2;
* @return The fileFormat.
*/
@java.lang.Override
public cz.proto.FileFormatTypeOuterClass.FileFormatType getFileFormat() {
@SuppressWarnings("deprecation")
cz.proto.FileFormatTypeOuterClass.FileFormatType result = cz.proto.FileFormatTypeOuterClass.FileFormatType.valueOf(fileFormat_);
return result == null ? cz.proto.FileFormatTypeOuterClass.FileFormatType.UNRECOGNIZED : result;
}
/**
* .cz.proto.FileFormatType file_format = 2;
* @param value The fileFormat to set.
* @return This builder for chaining.
*/
public Builder setFileFormat(cz.proto.FileFormatTypeOuterClass.FileFormatType value) {
if (value == null) {
throw new NullPointerException();
}
fileFormat_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.FileFormatType file_format = 2;
* @return This builder for chaining.
*/
public Builder clearFileFormat() {
fileFormat_ = 0;
onChanged();
return this;
}
private long maxNumRowsPerFile_ ;
/**
* int64 max_num_rows_per_file = 3;
* @return The maxNumRowsPerFile.
*/
@java.lang.Override
public long getMaxNumRowsPerFile() {
return maxNumRowsPerFile_;
}
/**
* int64 max_num_rows_per_file = 3;
* @param value The maxNumRowsPerFile to set.
* @return This builder for chaining.
*/
public Builder setMaxNumRowsPerFile(long value) {
maxNumRowsPerFile_ = value;
onChanged();
return this;
}
/**
* int64 max_num_rows_per_file = 3;
* @return This builder for chaining.
*/
public Builder clearMaxNumRowsPerFile() {
maxNumRowsPerFile_ = 0L;
onChanged();
return this;
}
private long maxSizeInBytesPerFile_ ;
/**
* int64 max_size_in_bytes_per_file = 4;
* @return The maxSizeInBytesPerFile.
*/
@java.lang.Override
public long getMaxSizeInBytesPerFile() {
return maxSizeInBytesPerFile_;
}
/**
* int64 max_size_in_bytes_per_file = 4;
* @param value The maxSizeInBytesPerFile to set.
* @return This builder for chaining.
*/
public Builder setMaxSizeInBytesPerFile(long value) {
maxSizeInBytesPerFile_ = value;
onChanged();
return this;
}
/**
* int64 max_size_in_bytes_per_file = 4;
* @return This builder for chaining.
*/
public Builder clearMaxSizeInBytesPerFile() {
maxSizeInBytesPerFile_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.BulkLoadStreamWriterConfig)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.BulkLoadStreamWriterConfig)
private static final cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig();
}
public static cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BulkLoadStreamWriterConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BulkLoadStreamWriterConfig(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateBulkLoadStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CreateBulkLoadStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The enum numeric value on the wire for operation.
*/
int getOperationValue();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The operation.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation();
/**
* string partition_spec = 4;
* @return The partitionSpec.
*/
java.lang.String getPartitionSpec();
/**
* string partition_spec = 4;
* @return The bytes for partitionSpec.
*/
com.google.protobuf.ByteString
getPartitionSpecBytes();
/**
* repeated string record_keys = 5;
* @return A list containing the recordKeys.
*/
java.util.List
getRecordKeysList();
/**
* repeated string record_keys = 5;
* @return The count of recordKeys.
*/
int getRecordKeysCount();
/**
* repeated string record_keys = 5;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
java.lang.String getRecordKeys(int index);
/**
* repeated string record_keys = 5;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
com.google.protobuf.ByteString
getRecordKeysBytes(int index);
/**
* bool prefer_internal_endpoint = 6;
* @return The preferInternalEndpoint.
*/
boolean getPreferInternalEndpoint();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateBulkLoadStreamRequest}
*/
public static final class CreateBulkLoadStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CreateBulkLoadStreamRequest)
CreateBulkLoadStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateBulkLoadStreamRequest.newBuilder() to construct.
private CreateBulkLoadStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateBulkLoadStreamRequest() {
operation_ = 0;
partitionSpec_ = "";
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateBulkLoadStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CreateBulkLoadStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 24: {
int rawValue = input.readEnum();
operation_ = rawValue;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
partitionSpec_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
recordKeys_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
recordKeys_.add(s);
break;
}
case 48: {
preferInternalEndpoint_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
recordKeys_ = recordKeys_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int OPERATION_FIELD_NUMBER = 3;
private int operation_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The operation.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.valueOf(operation_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.UNRECOGNIZED : result;
}
public static final int PARTITION_SPEC_FIELD_NUMBER = 4;
private volatile java.lang.Object partitionSpec_;
/**
* string partition_spec = 4;
* @return The partitionSpec.
*/
@java.lang.Override
public java.lang.String getPartitionSpec() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partitionSpec_ = s;
return s;
}
}
/**
* string partition_spec = 4;
* @return The bytes for partitionSpec.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPartitionSpecBytes() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partitionSpec_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RECORD_KEYS_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList recordKeys_;
/**
* repeated string record_keys = 5;
* @return A list containing the recordKeys.
*/
public com.google.protobuf.ProtocolStringList
getRecordKeysList() {
return recordKeys_;
}
/**
* repeated string record_keys = 5;
* @return The count of recordKeys.
*/
public int getRecordKeysCount() {
return recordKeys_.size();
}
/**
* repeated string record_keys = 5;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
public java.lang.String getRecordKeys(int index) {
return recordKeys_.get(index);
}
/**
* repeated string record_keys = 5;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
public com.google.protobuf.ByteString
getRecordKeysBytes(int index) {
return recordKeys_.getByteString(index);
}
public static final int PREFER_INTERNAL_ENDPOINT_FIELD_NUMBER = 6;
private boolean preferInternalEndpoint_;
/**
* bool prefer_internal_endpoint = 6;
* @return The preferInternalEndpoint.
*/
@java.lang.Override
public boolean getPreferInternalEndpoint() {
return preferInternalEndpoint_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (operation_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.BL_APPEND.getNumber()) {
output.writeEnum(3, operation_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(partitionSpec_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, partitionSpec_);
}
for (int i = 0; i < recordKeys_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, recordKeys_.getRaw(i));
}
if (preferInternalEndpoint_ != false) {
output.writeBool(6, preferInternalEndpoint_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (operation_ != cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.BL_APPEND.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, operation_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(partitionSpec_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, partitionSpec_);
}
{
int dataSize = 0;
for (int i = 0; i < recordKeys_.size(); i++) {
dataSize += computeStringSizeNoTag(recordKeys_.getRaw(i));
}
size += dataSize;
size += 1 * getRecordKeysList().size();
}
if (preferInternalEndpoint_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, preferInternalEndpoint_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest other = (cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (operation_ != other.operation_) return false;
if (!getPartitionSpec()
.equals(other.getPartitionSpec())) return false;
if (!getRecordKeysList()
.equals(other.getRecordKeysList())) return false;
if (getPreferInternalEndpoint()
!= other.getPreferInternalEndpoint()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + OPERATION_FIELD_NUMBER;
hash = (53 * hash) + operation_;
hash = (37 * hash) + PARTITION_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getPartitionSpec().hashCode();
if (getRecordKeysCount() > 0) {
hash = (37 * hash) + RECORD_KEYS_FIELD_NUMBER;
hash = (53 * hash) + getRecordKeysList().hashCode();
}
hash = (37 * hash) + PREFER_INTERNAL_ENDPOINT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getPreferInternalEndpoint());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateBulkLoadStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CreateBulkLoadStreamRequest)
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
operation_ = 0;
partitionSpec_ = "";
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
preferInternalEndpoint_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest build() {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest result = new cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest(this);
int from_bitField0_ = bitField0_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.operation_ = operation_;
result.partitionSpec_ = partitionSpec_;
if (((bitField0_ & 0x00000001) != 0)) {
recordKeys_ = recordKeys_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.recordKeys_ = recordKeys_;
result.preferInternalEndpoint_ = preferInternalEndpoint_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (other.operation_ != 0) {
setOperationValue(other.getOperationValue());
}
if (!other.getPartitionSpec().isEmpty()) {
partitionSpec_ = other.partitionSpec_;
onChanged();
}
if (!other.recordKeys_.isEmpty()) {
if (recordKeys_.isEmpty()) {
recordKeys_ = other.recordKeys_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureRecordKeysIsMutable();
recordKeys_.addAll(other.recordKeys_);
}
onChanged();
}
if (other.getPreferInternalEndpoint() != false) {
setPreferInternalEndpoint(other.getPreferInternalEndpoint());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private int operation_ = 0;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The enum numeric value on the wire for operation.
*/
@java.lang.Override public int getOperationValue() {
return operation_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @param value The enum numeric value on the wire for operation to set.
* @return This builder for chaining.
*/
public Builder setOperationValue(int value) {
operation_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return The operation.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation getOperation() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation result = cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.valueOf(operation_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @param value The operation to set.
* @return This builder for chaining.
*/
public Builder setOperation(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamOperation value) {
if (value == null) {
throw new NullPointerException();
}
operation_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamOperation operation = 3;
* @return This builder for chaining.
*/
public Builder clearOperation() {
operation_ = 0;
onChanged();
return this;
}
private java.lang.Object partitionSpec_ = "";
/**
* string partition_spec = 4;
* @return The partitionSpec.
*/
public java.lang.String getPartitionSpec() {
java.lang.Object ref = partitionSpec_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
partitionSpec_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string partition_spec = 4;
* @return The bytes for partitionSpec.
*/
public com.google.protobuf.ByteString
getPartitionSpecBytes() {
java.lang.Object ref = partitionSpec_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
partitionSpec_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string partition_spec = 4;
* @param value The partitionSpec to set.
* @return This builder for chaining.
*/
public Builder setPartitionSpec(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
partitionSpec_ = value;
onChanged();
return this;
}
/**
* string partition_spec = 4;
* @return This builder for chaining.
*/
public Builder clearPartitionSpec() {
partitionSpec_ = getDefaultInstance().getPartitionSpec();
onChanged();
return this;
}
/**
* string partition_spec = 4;
* @param value The bytes for partitionSpec to set.
* @return This builder for chaining.
*/
public Builder setPartitionSpecBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
partitionSpec_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRecordKeysIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
recordKeys_ = new com.google.protobuf.LazyStringArrayList(recordKeys_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string record_keys = 5;
* @return A list containing the recordKeys.
*/
public com.google.protobuf.ProtocolStringList
getRecordKeysList() {
return recordKeys_.getUnmodifiableView();
}
/**
* repeated string record_keys = 5;
* @return The count of recordKeys.
*/
public int getRecordKeysCount() {
return recordKeys_.size();
}
/**
* repeated string record_keys = 5;
* @param index The index of the element to return.
* @return The recordKeys at the given index.
*/
public java.lang.String getRecordKeys(int index) {
return recordKeys_.get(index);
}
/**
* repeated string record_keys = 5;
* @param index The index of the value to return.
* @return The bytes of the recordKeys at the given index.
*/
public com.google.protobuf.ByteString
getRecordKeysBytes(int index) {
return recordKeys_.getByteString(index);
}
/**
* repeated string record_keys = 5;
* @param index The index to set the value at.
* @param value The recordKeys to set.
* @return This builder for chaining.
*/
public Builder setRecordKeys(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordKeysIsMutable();
recordKeys_.set(index, value);
onChanged();
return this;
}
/**
* repeated string record_keys = 5;
* @param value The recordKeys to add.
* @return This builder for chaining.
*/
public Builder addRecordKeys(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRecordKeysIsMutable();
recordKeys_.add(value);
onChanged();
return this;
}
/**
* repeated string record_keys = 5;
* @param values The recordKeys to add.
* @return This builder for chaining.
*/
public Builder addAllRecordKeys(
java.lang.Iterable values) {
ensureRecordKeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, recordKeys_);
onChanged();
return this;
}
/**
* repeated string record_keys = 5;
* @return This builder for chaining.
*/
public Builder clearRecordKeys() {
recordKeys_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string record_keys = 5;
* @param value The bytes of the recordKeys to add.
* @return This builder for chaining.
*/
public Builder addRecordKeysBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureRecordKeysIsMutable();
recordKeys_.add(value);
onChanged();
return this;
}
private boolean preferInternalEndpoint_ ;
/**
* bool prefer_internal_endpoint = 6;
* @return The preferInternalEndpoint.
*/
@java.lang.Override
public boolean getPreferInternalEndpoint() {
return preferInternalEndpoint_;
}
/**
* bool prefer_internal_endpoint = 6;
* @param value The preferInternalEndpoint to set.
* @return This builder for chaining.
*/
public Builder setPreferInternalEndpoint(boolean value) {
preferInternalEndpoint_ = value;
onChanged();
return this;
}
/**
* bool prefer_internal_endpoint = 6;
* @return This builder for chaining.
*/
public Builder clearPreferInternalEndpoint() {
preferInternalEndpoint_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CreateBulkLoadStreamRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CreateBulkLoadStreamRequest)
private static final cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateBulkLoadStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateBulkLoadStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateBulkLoadStreamResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CreateBulkLoadStreamResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
boolean hasInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateBulkLoadStreamResponse}
*/
public static final class CreateBulkLoadStreamResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CreateBulkLoadStreamResponse)
CreateBulkLoadStreamResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateBulkLoadStreamResponse.newBuilder() to construct.
private CreateBulkLoadStreamResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateBulkLoadStreamResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateBulkLoadStreamResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CreateBulkLoadStreamResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder subBuilder = null;
if (info_ != null) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int INFO_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
return getInfo();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (info_ != null) {
output.writeMessage(2, getInfo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (info_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse other = (cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasInfo() != other.hasInfo()) return false;
if (hasInfo()) {
if (!getInfo()
.equals(other.getInfo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CreateBulkLoadStreamResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CreateBulkLoadStreamResponse)
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (infoBuilder_ == null) {
info_ = null;
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CreateBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse build() {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse result = new cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (infoBuilder_ == null) {
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder> infoBuilder_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
public boolean hasInfo() {
return infoBuilder_ != null || info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder mergeInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (info_ != null) {
info_ =
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
onChanged();
} else {
infoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = null;
onChanged();
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder getInfoBuilder() {
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CreateBulkLoadStreamResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CreateBulkLoadStreamResponse)
private static final cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateBulkLoadStreamResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CreateBulkLoadStreamResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CreateBulkLoadStreamResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetBulkLoadStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetBulkLoadStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* string stream_id = 3;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
/**
* bool need_table_meta = 4;
* @return The needTableMeta.
*/
boolean getNeedTableMeta();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamRequest}
*/
public static final class GetBulkLoadStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetBulkLoadStreamRequest)
GetBulkLoadStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetBulkLoadStreamRequest.newBuilder() to construct.
private GetBulkLoadStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetBulkLoadStreamRequest() {
streamId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetBulkLoadStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetBulkLoadStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
case 32: {
needTableMeta_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int STREAM_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 3;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NEED_TABLE_META_FIELD_NUMBER = 4;
private boolean needTableMeta_;
/**
* bool need_table_meta = 4;
* @return The needTableMeta.
*/
@java.lang.Override
public boolean getNeedTableMeta() {
return needTableMeta_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, streamId_);
}
if (needTableMeta_ != false) {
output.writeBool(4, needTableMeta_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, streamId_);
}
if (needTableMeta_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, needTableMeta_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest other = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (getNeedTableMeta()
!= other.getNeedTableMeta()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (37 * hash) + NEED_TABLE_META_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getNeedTableMeta());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetBulkLoadStreamRequest)
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
streamId_ = "";
needTableMeta_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest build() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest result = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.streamId_ = streamId_;
result.needTableMeta_ = needTableMeta_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
if (other.getNeedTableMeta() != false) {
setNeedTableMeta(other.getNeedTableMeta());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private java.lang.Object streamId_ = "";
/**
* string stream_id = 3;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 3;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 3;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 3;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
private boolean needTableMeta_ ;
/**
* bool need_table_meta = 4;
* @return The needTableMeta.
*/
@java.lang.Override
public boolean getNeedTableMeta() {
return needTableMeta_;
}
/**
* bool need_table_meta = 4;
* @param value The needTableMeta to set.
* @return This builder for chaining.
*/
public Builder setNeedTableMeta(boolean value) {
needTableMeta_ = value;
onChanged();
return this;
}
/**
* bool need_table_meta = 4;
* @return This builder for chaining.
*/
public Builder clearNeedTableMeta() {
needTableMeta_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GetBulkLoadStreamRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GetBulkLoadStreamRequest)
private static final cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetBulkLoadStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetBulkLoadStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetBulkLoadStreamResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetBulkLoadStreamResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
boolean hasInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamResponse}
*/
public static final class GetBulkLoadStreamResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetBulkLoadStreamResponse)
GetBulkLoadStreamResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetBulkLoadStreamResponse.newBuilder() to construct.
private GetBulkLoadStreamResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetBulkLoadStreamResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetBulkLoadStreamResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetBulkLoadStreamResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder subBuilder = null;
if (info_ != null) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int INFO_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
return getInfo();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (info_ != null) {
output.writeMessage(2, getInfo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (info_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse other = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasInfo() != other.hasInfo()) return false;
if (hasInfo()) {
if (!getInfo()
.equals(other.getInfo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetBulkLoadStreamResponse)
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (infoBuilder_ == null) {
info_ = null;
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse build() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse result = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (infoBuilder_ == null) {
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder> infoBuilder_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
public boolean hasInfo() {
return infoBuilder_ != null || info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder mergeInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (info_ != null) {
info_ =
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
onChanged();
} else {
infoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = null;
onChanged();
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder getInfoBuilder() {
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GetBulkLoadStreamResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GetBulkLoadStreamResponse)
private static final cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetBulkLoadStreamResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetBulkLoadStreamResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommitBulkLoadStreamRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* string stream_id = 3;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
/**
* string execute_workspace = 4;
* @return The executeWorkspace.
*/
java.lang.String getExecuteWorkspace();
/**
* string execute_workspace = 4;
* @return The bytes for executeWorkspace.
*/
com.google.protobuf.ByteString
getExecuteWorkspaceBytes();
/**
* string execute_vc_name = 5;
* @return The executeVcName.
*/
java.lang.String getExecuteVcName();
/**
* string execute_vc_name = 5;
* @return The bytes for executeVcName.
*/
com.google.protobuf.ByteString
getExecuteVcNameBytes();
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The enum numeric value on the wire for commitMode.
*/
int getCommitModeValue();
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The commitMode.
*/
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode getCommitMode();
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return A list containing the specPartitionIds.
*/
java.util.List getSpecPartitionIdsList();
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return The count of specPartitionIds.
*/
int getSpecPartitionIdsCount();
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param index The index of the element to return.
* @return The specPartitionIds at the given index.
*/
int getSpecPartitionIds(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CommitBulkLoadStreamRequest}
*/
public static final class CommitBulkLoadStreamRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest)
CommitBulkLoadStreamRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommitBulkLoadStreamRequest.newBuilder() to construct.
private CommitBulkLoadStreamRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommitBulkLoadStreamRequest() {
streamId_ = "";
executeWorkspace_ = "";
executeVcName_ = "";
commitMode_ = 0;
specPartitionIds_ = emptyIntList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommitBulkLoadStreamRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommitBulkLoadStreamRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
executeWorkspace_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
executeVcName_ = s;
break;
}
case 48: {
int rawValue = input.readEnum();
commitMode_ = rawValue;
break;
}
case 56: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
specPartitionIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
specPartitionIds_.addInt(input.readUInt32());
break;
}
case 58: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
specPartitionIds_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
specPartitionIds_.addInt(input.readUInt32());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
specPartitionIds_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.Builder.class);
}
/**
* Protobuf enum {@code cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode}
*/
public enum CommitMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* COMMIT_STREAM = 0;
*/
COMMIT_STREAM(0),
/**
* ABORT_STREAM = 1;
*/
ABORT_STREAM(1),
UNRECOGNIZED(-1),
;
/**
* COMMIT_STREAM = 0;
*/
public static final int COMMIT_STREAM_VALUE = 0;
/**
* ABORT_STREAM = 1;
*/
public static final int ABORT_STREAM_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CommitMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static CommitMode forNumber(int value) {
switch (value) {
case 0: return COMMIT_STREAM;
case 1: return ABORT_STREAM;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CommitMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CommitMode findValueByNumber(int number) {
return CommitMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.getDescriptor().getEnumTypes().get(0);
}
private static final CommitMode[] VALUES = values();
public static CommitMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CommitMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode)
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int STREAM_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 3;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXECUTE_WORKSPACE_FIELD_NUMBER = 4;
private volatile java.lang.Object executeWorkspace_;
/**
* string execute_workspace = 4;
* @return The executeWorkspace.
*/
@java.lang.Override
public java.lang.String getExecuteWorkspace() {
java.lang.Object ref = executeWorkspace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executeWorkspace_ = s;
return s;
}
}
/**
* string execute_workspace = 4;
* @return The bytes for executeWorkspace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExecuteWorkspaceBytes() {
java.lang.Object ref = executeWorkspace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executeWorkspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXECUTE_VC_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object executeVcName_;
/**
* string execute_vc_name = 5;
* @return The executeVcName.
*/
@java.lang.Override
public java.lang.String getExecuteVcName() {
java.lang.Object ref = executeVcName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executeVcName_ = s;
return s;
}
}
/**
* string execute_vc_name = 5;
* @return The bytes for executeVcName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExecuteVcNameBytes() {
java.lang.Object ref = executeVcName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executeVcName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMMIT_MODE_FIELD_NUMBER = 6;
private int commitMode_;
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The enum numeric value on the wire for commitMode.
*/
@java.lang.Override public int getCommitModeValue() {
return commitMode_;
}
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The commitMode.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode getCommitMode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode result = cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.valueOf(commitMode_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.UNRECOGNIZED : result;
}
public static final int SPEC_PARTITION_IDS_FIELD_NUMBER = 7;
private com.google.protobuf.Internal.IntList specPartitionIds_;
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return A list containing the specPartitionIds.
*/
@java.lang.Override
public java.util.List
getSpecPartitionIdsList() {
return specPartitionIds_;
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return The count of specPartitionIds.
*/
public int getSpecPartitionIdsCount() {
return specPartitionIds_.size();
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param index The index of the element to return.
* @return The specPartitionIds at the given index.
*/
public int getSpecPartitionIds(int index) {
return specPartitionIds_.getInt(index);
}
private int specPartitionIdsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, streamId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executeWorkspace_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, executeWorkspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executeVcName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, executeVcName_);
}
if (commitMode_ != cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.COMMIT_STREAM.getNumber()) {
output.writeEnum(6, commitMode_);
}
if (getSpecPartitionIdsList().size() > 0) {
output.writeUInt32NoTag(58);
output.writeUInt32NoTag(specPartitionIdsMemoizedSerializedSize);
}
for (int i = 0; i < specPartitionIds_.size(); i++) {
output.writeUInt32NoTag(specPartitionIds_.getInt(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, streamId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executeWorkspace_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, executeWorkspace_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(executeVcName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, executeVcName_);
}
if (commitMode_ != cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.COMMIT_STREAM.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, commitMode_);
}
{
int dataSize = 0;
for (int i = 0; i < specPartitionIds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(specPartitionIds_.getInt(i));
}
size += dataSize;
if (!getSpecPartitionIdsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
specPartitionIdsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest other = (cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (!getExecuteWorkspace()
.equals(other.getExecuteWorkspace())) return false;
if (!getExecuteVcName()
.equals(other.getExecuteVcName())) return false;
if (commitMode_ != other.commitMode_) return false;
if (!getSpecPartitionIdsList()
.equals(other.getSpecPartitionIdsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (37 * hash) + EXECUTE_WORKSPACE_FIELD_NUMBER;
hash = (53 * hash) + getExecuteWorkspace().hashCode();
hash = (37 * hash) + EXECUTE_VC_NAME_FIELD_NUMBER;
hash = (53 * hash) + getExecuteVcName().hashCode();
hash = (37 * hash) + COMMIT_MODE_FIELD_NUMBER;
hash = (53 * hash) + commitMode_;
if (getSpecPartitionIdsCount() > 0) {
hash = (37 * hash) + SPEC_PARTITION_IDS_FIELD_NUMBER;
hash = (53 * hash) + getSpecPartitionIdsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CommitBulkLoadStreamRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest)
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.class, cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
streamId_ = "";
executeWorkspace_ = "";
executeVcName_ = "";
commitMode_ = 0;
specPartitionIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest build() {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest result = new cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest(this);
int from_bitField0_ = bitField0_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.streamId_ = streamId_;
result.executeWorkspace_ = executeWorkspace_;
result.executeVcName_ = executeVcName_;
result.commitMode_ = commitMode_;
if (((bitField0_ & 0x00000001) != 0)) {
specPartitionIds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.specPartitionIds_ = specPartitionIds_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
if (!other.getExecuteWorkspace().isEmpty()) {
executeWorkspace_ = other.executeWorkspace_;
onChanged();
}
if (!other.getExecuteVcName().isEmpty()) {
executeVcName_ = other.executeVcName_;
onChanged();
}
if (other.commitMode_ != 0) {
setCommitModeValue(other.getCommitModeValue());
}
if (!other.specPartitionIds_.isEmpty()) {
if (specPartitionIds_.isEmpty()) {
specPartitionIds_ = other.specPartitionIds_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSpecPartitionIdsIsMutable();
specPartitionIds_.addAll(other.specPartitionIds_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private java.lang.Object streamId_ = "";
/**
* string stream_id = 3;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 3;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 3;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 3;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
private java.lang.Object executeWorkspace_ = "";
/**
* string execute_workspace = 4;
* @return The executeWorkspace.
*/
public java.lang.String getExecuteWorkspace() {
java.lang.Object ref = executeWorkspace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executeWorkspace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string execute_workspace = 4;
* @return The bytes for executeWorkspace.
*/
public com.google.protobuf.ByteString
getExecuteWorkspaceBytes() {
java.lang.Object ref = executeWorkspace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executeWorkspace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string execute_workspace = 4;
* @param value The executeWorkspace to set.
* @return This builder for chaining.
*/
public Builder setExecuteWorkspace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
executeWorkspace_ = value;
onChanged();
return this;
}
/**
* string execute_workspace = 4;
* @return This builder for chaining.
*/
public Builder clearExecuteWorkspace() {
executeWorkspace_ = getDefaultInstance().getExecuteWorkspace();
onChanged();
return this;
}
/**
* string execute_workspace = 4;
* @param value The bytes for executeWorkspace to set.
* @return This builder for chaining.
*/
public Builder setExecuteWorkspaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
executeWorkspace_ = value;
onChanged();
return this;
}
private java.lang.Object executeVcName_ = "";
/**
* string execute_vc_name = 5;
* @return The executeVcName.
*/
public java.lang.String getExecuteVcName() {
java.lang.Object ref = executeVcName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executeVcName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string execute_vc_name = 5;
* @return The bytes for executeVcName.
*/
public com.google.protobuf.ByteString
getExecuteVcNameBytes() {
java.lang.Object ref = executeVcName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executeVcName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string execute_vc_name = 5;
* @param value The executeVcName to set.
* @return This builder for chaining.
*/
public Builder setExecuteVcName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
executeVcName_ = value;
onChanged();
return this;
}
/**
* string execute_vc_name = 5;
* @return This builder for chaining.
*/
public Builder clearExecuteVcName() {
executeVcName_ = getDefaultInstance().getExecuteVcName();
onChanged();
return this;
}
/**
* string execute_vc_name = 5;
* @param value The bytes for executeVcName to set.
* @return This builder for chaining.
*/
public Builder setExecuteVcNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
executeVcName_ = value;
onChanged();
return this;
}
private int commitMode_ = 0;
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The enum numeric value on the wire for commitMode.
*/
@java.lang.Override public int getCommitModeValue() {
return commitMode_;
}
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @param value The enum numeric value on the wire for commitMode to set.
* @return This builder for chaining.
*/
public Builder setCommitModeValue(int value) {
commitMode_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return The commitMode.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode getCommitMode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode result = cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.valueOf(commitMode_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @param value The commitMode to set.
* @return This builder for chaining.
*/
public Builder setCommitMode(cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest.CommitMode value) {
if (value == null) {
throw new NullPointerException();
}
commitMode_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.CommitBulkLoadStreamRequest.CommitMode commit_mode = 6;
* @return This builder for chaining.
*/
public Builder clearCommitMode() {
commitMode_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList specPartitionIds_ = emptyIntList();
private void ensureSpecPartitionIdsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
specPartitionIds_ = mutableCopy(specPartitionIds_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return A list containing the specPartitionIds.
*/
public java.util.List
getSpecPartitionIdsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(specPartitionIds_) : specPartitionIds_;
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return The count of specPartitionIds.
*/
public int getSpecPartitionIdsCount() {
return specPartitionIds_.size();
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param index The index of the element to return.
* @return The specPartitionIds at the given index.
*/
public int getSpecPartitionIds(int index) {
return specPartitionIds_.getInt(index);
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param index The index to set the value at.
* @param value The specPartitionIds to set.
* @return This builder for chaining.
*/
public Builder setSpecPartitionIds(
int index, int value) {
ensureSpecPartitionIdsIsMutable();
specPartitionIds_.setInt(index, value);
onChanged();
return this;
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param value The specPartitionIds to add.
* @return This builder for chaining.
*/
public Builder addSpecPartitionIds(int value) {
ensureSpecPartitionIdsIsMutable();
specPartitionIds_.addInt(value);
onChanged();
return this;
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @param values The specPartitionIds to add.
* @return This builder for chaining.
*/
public Builder addAllSpecPartitionIds(
java.lang.Iterable extends java.lang.Integer> values) {
ensureSpecPartitionIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, specPartitionIds_);
onChanged();
return this;
}
/**
*
* Is spec_partition_ids required?
*
*
* repeated uint32 spec_partition_ids = 7;
* @return This builder for chaining.
*/
public Builder clearSpecPartitionIds() {
specPartitionIds_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CommitBulkLoadStreamRequest)
private static final cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommitBulkLoadStreamRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommitBulkLoadStreamRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CommitBulkLoadStreamResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.CommitBulkLoadStreamResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
boolean hasInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CommitBulkLoadStreamResponse}
*/
public static final class CommitBulkLoadStreamResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.CommitBulkLoadStreamResponse)
CommitBulkLoadStreamResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CommitBulkLoadStreamResponse.newBuilder() to construct.
private CommitBulkLoadStreamResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommitBulkLoadStreamResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CommitBulkLoadStreamResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CommitBulkLoadStreamResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder subBuilder = null;
if (info_ != null) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int INFO_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
return getInfo();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (info_ != null) {
output.writeMessage(2, getInfo());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (info_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInfo());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse other = (cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasInfo() != other.hasInfo()) return false;
if (hasInfo()) {
if (!getInfo()
.equals(other.getInfo())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.CommitBulkLoadStreamResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.CommitBulkLoadStreamResponse)
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.class, cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (infoBuilder_ == null) {
info_ = null;
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_CommitBulkLoadStreamResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse build() {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse result = new cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (infoBuilder_ == null) {
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo info_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder> infoBuilder_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return Whether the info field is set.
*/
public boolean hasInfo() {
return infoBuilder_ != null || info_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
* @return The info.
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder setInfo(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder mergeInfo(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo value) {
if (infoBuilder_ == null) {
if (info_ != null) {
info_ =
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
onChanged();
} else {
infoBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = null;
onChanged();
} else {
info_ = null;
infoBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder getInfoBuilder() {
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.getDefaultInstance() : info_;
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamInfo info = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfo.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.CommitBulkLoadStreamResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.CommitBulkLoadStreamResponse)
private static final cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CommitBulkLoadStreamResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommitBulkLoadStreamResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.CommitBulkLoadStreamResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetBulkLoadStreamStsTokenRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* string stream_id = 3;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest}
*/
public static final class GetBulkLoadStreamStsTokenRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest)
GetBulkLoadStreamStsTokenRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetBulkLoadStreamStsTokenRequest.newBuilder() to construct.
private GetBulkLoadStreamStsTokenRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetBulkLoadStreamStsTokenRequest() {
streamId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetBulkLoadStreamStsTokenRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetBulkLoadStreamStsTokenRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int STREAM_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 3;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, streamId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, streamId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest other = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest)
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
streamId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest build() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest result = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.streamId_ = streamId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private java.lang.Object streamId_ = "";
/**
* string stream_id = 3;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 3;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 3;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 3;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenRequest)
private static final cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetBulkLoadStreamStsTokenRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetBulkLoadStreamStsTokenRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetBulkLoadStreamStsTokenResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return Whether the stagingPath field is set.
*/
boolean hasStagingPath();
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return The stagingPath.
*/
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath();
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse}
*/
public static final class GetBulkLoadStreamStsTokenResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse)
GetBulkLoadStreamStsTokenResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetBulkLoadStreamStsTokenResponse.newBuilder() to construct.
private GetBulkLoadStreamStsTokenResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetBulkLoadStreamStsTokenResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetBulkLoadStreamStsTokenResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetBulkLoadStreamStsTokenResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder subBuilder = null;
if (stagingPath_ != null) {
subBuilder = stagingPath_.toBuilder();
}
stagingPath_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stagingPath_);
stagingPath_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int STAGING_PATH_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.StagingPathInfo stagingPath_;
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return Whether the stagingPath field is set.
*/
@java.lang.Override
public boolean hasStagingPath() {
return stagingPath_ != null;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return The stagingPath.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath() {
return stagingPath_ == null ? cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder() {
return getStagingPath();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (stagingPath_ != null) {
output.writeMessage(2, getStagingPath());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (stagingPath_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getStagingPath());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse other = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasStagingPath() != other.hasStagingPath()) return false;
if (hasStagingPath()) {
if (!getStagingPath()
.equals(other.getStagingPath())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasStagingPath()) {
hash = (37 * hash) + STAGING_PATH_FIELD_NUMBER;
hash = (53 * hash) + getStagingPath().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse)
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.class, cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (stagingPathBuilder_ == null) {
stagingPath_ = null;
} else {
stagingPath_ = null;
stagingPathBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetBulkLoadStreamStsTokenResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse build() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse result = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (stagingPathBuilder_ == null) {
result.stagingPath_ = stagingPath_;
} else {
result.stagingPath_ = stagingPathBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasStagingPath()) {
mergeStagingPath(other.getStagingPath());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.StagingPathInfo stagingPath_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder> stagingPathBuilder_;
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return Whether the stagingPath field is set.
*/
public boolean hasStagingPath() {
return stagingPathBuilder_ != null || stagingPath_ != null;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
* @return The stagingPath.
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo getStagingPath() {
if (stagingPathBuilder_ == null) {
return stagingPath_ == null ? cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
} else {
return stagingPathBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public Builder setStagingPath(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo value) {
if (stagingPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stagingPath_ = value;
onChanged();
} else {
stagingPathBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public Builder setStagingPath(
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder builderForValue) {
if (stagingPathBuilder_ == null) {
stagingPath_ = builderForValue.build();
onChanged();
} else {
stagingPathBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public Builder mergeStagingPath(cz.proto.ingestion.v2.IngestionV2.StagingPathInfo value) {
if (stagingPathBuilder_ == null) {
if (stagingPath_ != null) {
stagingPath_ =
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.newBuilder(stagingPath_).mergeFrom(value).buildPartial();
} else {
stagingPath_ = value;
}
onChanged();
} else {
stagingPathBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public Builder clearStagingPath() {
if (stagingPathBuilder_ == null) {
stagingPath_ = null;
onChanged();
} else {
stagingPath_ = null;
stagingPathBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder getStagingPathBuilder() {
onChanged();
return getStagingPathFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder getStagingPathOrBuilder() {
if (stagingPathBuilder_ != null) {
return stagingPathBuilder_.getMessageOrBuilder();
} else {
return stagingPath_ == null ?
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.getDefaultInstance() : stagingPath_;
}
}
/**
* .cz.proto.ingestion.v2.StagingPathInfo staging_path = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder>
getStagingPathFieldBuilder() {
if (stagingPathBuilder_ == null) {
stagingPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.StagingPathInfo, cz.proto.ingestion.v2.IngestionV2.StagingPathInfo.Builder, cz.proto.ingestion.v2.IngestionV2.StagingPathInfoOrBuilder>(
getStagingPath(),
getParentForChildren(),
isClean());
stagingPath_ = null;
}
return stagingPathBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GetBulkLoadStreamStsTokenResponse)
private static final cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetBulkLoadStreamStsTokenResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetBulkLoadStreamStsTokenResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetBulkLoadStreamStsTokenResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OpenBulkLoadStreamWriterRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* string stream_id = 3;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
int getPartitionId();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest}
*/
public static final class OpenBulkLoadStreamWriterRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest)
OpenBulkLoadStreamWriterRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use OpenBulkLoadStreamWriterRequest.newBuilder() to construct.
private OpenBulkLoadStreamWriterRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OpenBulkLoadStreamWriterRequest() {
streamId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OpenBulkLoadStreamWriterRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OpenBulkLoadStreamWriterRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
case 32: {
partitionId_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.class, cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int STREAM_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 3;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARTITION_ID_FIELD_NUMBER = 4;
private int partitionId_;
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
@java.lang.Override
public int getPartitionId() {
return partitionId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, streamId_);
}
if (partitionId_ != 0) {
output.writeUInt32(4, partitionId_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, streamId_);
}
if (partitionId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, partitionId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest other = (cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (getPartitionId()
!= other.getPartitionId()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (37 * hash) + PARTITION_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartitionId();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest)
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.class, cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
streamId_ = "";
partitionId_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest build() {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest result = new cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest(this);
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.streamId_ = streamId_;
result.partitionId_ = partitionId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
if (other.getPartitionId() != 0) {
setPartitionId(other.getPartitionId());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private java.lang.Object streamId_ = "";
/**
* string stream_id = 3;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 3;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 3;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 3;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
private int partitionId_ ;
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
@java.lang.Override
public int getPartitionId() {
return partitionId_;
}
/**
* uint32 partition_id = 4;
* @param value The partitionId to set.
* @return This builder for chaining.
*/
public Builder setPartitionId(int value) {
partitionId_ = value;
onChanged();
return this;
}
/**
* uint32 partition_id = 4;
* @return This builder for chaining.
*/
public Builder clearPartitionId() {
partitionId_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterRequest)
private static final cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OpenBulkLoadStreamWriterRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OpenBulkLoadStreamWriterRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OpenBulkLoadStreamWriterResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return Whether the config field is set.
*/
boolean hasConfig();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return The config.
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getConfig();
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder getConfigOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse}
*/
public static final class OpenBulkLoadStreamWriterResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse)
OpenBulkLoadStreamWriterResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use OpenBulkLoadStreamWriterResponse.newBuilder() to construct.
private OpenBulkLoadStreamWriterResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OpenBulkLoadStreamWriterResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OpenBulkLoadStreamWriterResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OpenBulkLoadStreamWriterResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder subBuilder = null;
if (config_ != null) {
subBuilder = config_.toBuilder();
}
config_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(config_);
config_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.class, cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
public static final int CONFIG_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig config_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return Whether the config field is set.
*/
@java.lang.Override
public boolean hasConfig() {
return config_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return The config.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getConfig() {
return config_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.getDefaultInstance() : config_;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder getConfigOrBuilder() {
return getConfig();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
if (config_ != null) {
output.writeMessage(2, getConfig());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
if (config_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getConfig());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse other = (cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (hasConfig() != other.hasConfig()) return false;
if (hasConfig()) {
if (!getConfig()
.equals(other.getConfig())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasConfig()) {
hash = (37 * hash) + CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getConfig().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse)
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.class, cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
if (configBuilder_ == null) {
config_ = null;
} else {
config_ = null;
configBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_OpenBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse build() {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse result = new cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
if (configBuilder_ == null) {
result.config_ = config_;
} else {
result.config_ = configBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
if (other.hasConfig()) {
mergeConfig(other.getConfig());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig config_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder> configBuilder_;
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return Whether the config field is set.
*/
public boolean hasConfig() {
return configBuilder_ != null || config_ != null;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
* @return The config.
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig getConfig() {
if (configBuilder_ == null) {
return config_ == null ? cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.getDefaultInstance() : config_;
} else {
return configBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public Builder setConfig(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig value) {
if (configBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
config_ = value;
onChanged();
} else {
configBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public Builder setConfig(
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder builderForValue) {
if (configBuilder_ == null) {
config_ = builderForValue.build();
onChanged();
} else {
configBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public Builder mergeConfig(cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig value) {
if (configBuilder_ == null) {
if (config_ != null) {
config_ =
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.newBuilder(config_).mergeFrom(value).buildPartial();
} else {
config_ = value;
}
onChanged();
} else {
configBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public Builder clearConfig() {
if (configBuilder_ == null) {
config_ = null;
onChanged();
} else {
config_ = null;
configBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder getConfigBuilder() {
onChanged();
return getConfigFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder getConfigOrBuilder() {
if (configBuilder_ != null) {
return configBuilder_.getMessageOrBuilder();
} else {
return config_ == null ?
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.getDefaultInstance() : config_;
}
}
/**
* .cz.proto.ingestion.v2.BulkLoadStreamWriterConfig config = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder>
getConfigFieldBuilder() {
if (configBuilder_ == null) {
configBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfig.Builder, cz.proto.ingestion.v2.IngestionV2.BulkLoadStreamWriterConfigOrBuilder>(
getConfig(),
getParentForChildren(),
isClean());
config_ = null;
}
return configBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.OpenBulkLoadStreamWriterResponse)
private static final cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OpenBulkLoadStreamWriterResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OpenBulkLoadStreamWriterResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.OpenBulkLoadStreamWriterResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FinishBulkLoadStreamWriterRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
boolean hasAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
cz.proto.ingestion.v2.IngestionV2.Account getAccount();
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
boolean hasIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier();
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder();
/**
* string stream_id = 3;
* @return The streamId.
*/
java.lang.String getStreamId();
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
com.google.protobuf.ByteString
getStreamIdBytes();
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
int getPartitionId();
/**
* repeated string written_files = 5;
* @return A list containing the writtenFiles.
*/
java.util.List
getWrittenFilesList();
/**
* repeated string written_files = 5;
* @return The count of writtenFiles.
*/
int getWrittenFilesCount();
/**
* repeated string written_files = 5;
* @param index The index of the element to return.
* @return The writtenFiles at the given index.
*/
java.lang.String getWrittenFiles(int index);
/**
* repeated string written_files = 5;
* @param index The index of the value to return.
* @return The bytes of the writtenFiles at the given index.
*/
com.google.protobuf.ByteString
getWrittenFilesBytes(int index);
/**
* repeated uint64 written_lengths = 6;
* @return A list containing the writtenLengths.
*/
java.util.List getWrittenLengthsList();
/**
* repeated uint64 written_lengths = 6;
* @return The count of writtenLengths.
*/
int getWrittenLengthsCount();
/**
* repeated uint64 written_lengths = 6;
* @param index The index of the element to return.
* @return The writtenLengths at the given index.
*/
long getWrittenLengths(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest}
*/
public static final class FinishBulkLoadStreamWriterRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest)
FinishBulkLoadStreamWriterRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use FinishBulkLoadStreamWriterRequest.newBuilder() to construct.
private FinishBulkLoadStreamWriterRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FinishBulkLoadStreamWriterRequest() {
streamId_ = "";
writtenFiles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
writtenLengths_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FinishBulkLoadStreamWriterRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FinishBulkLoadStreamWriterRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.Account.Builder subBuilder = null;
if (account_ != null) {
subBuilder = account_.toBuilder();
}
account_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.Account.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(account_);
account_ = subBuilder.buildPartial();
}
break;
}
case 18: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (identifier_ != null) {
subBuilder = identifier_.toBuilder();
}
identifier_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identifier_);
identifier_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
streamId_ = s;
break;
}
case 32: {
partitionId_ = input.readUInt32();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
writtenFiles_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
writtenFiles_.add(s);
break;
}
case 48: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
writtenLengths_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
writtenLengths_.addLong(input.readUInt64());
break;
}
case 50: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
writtenLengths_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
writtenLengths_.addLong(input.readUInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
writtenFiles_ = writtenFiles_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
writtenLengths_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.class, cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.Builder.class);
}
public static final int ACCOUNT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
@java.lang.Override
public boolean hasAccount() {
return account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
return getAccount();
}
public static final int IDENTIFIER_FIELD_NUMBER = 2;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
@java.lang.Override
public boolean hasIdentifier() {
return identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
return getIdentifier();
}
public static final int STREAM_ID_FIELD_NUMBER = 3;
private volatile java.lang.Object streamId_;
/**
* string stream_id = 3;
* @return The streamId.
*/
@java.lang.Override
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARTITION_ID_FIELD_NUMBER = 4;
private int partitionId_;
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
@java.lang.Override
public int getPartitionId() {
return partitionId_;
}
public static final int WRITTEN_FILES_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList writtenFiles_;
/**
* repeated string written_files = 5;
* @return A list containing the writtenFiles.
*/
public com.google.protobuf.ProtocolStringList
getWrittenFilesList() {
return writtenFiles_;
}
/**
* repeated string written_files = 5;
* @return The count of writtenFiles.
*/
public int getWrittenFilesCount() {
return writtenFiles_.size();
}
/**
* repeated string written_files = 5;
* @param index The index of the element to return.
* @return The writtenFiles at the given index.
*/
public java.lang.String getWrittenFiles(int index) {
return writtenFiles_.get(index);
}
/**
* repeated string written_files = 5;
* @param index The index of the value to return.
* @return The bytes of the writtenFiles at the given index.
*/
public com.google.protobuf.ByteString
getWrittenFilesBytes(int index) {
return writtenFiles_.getByteString(index);
}
public static final int WRITTEN_LENGTHS_FIELD_NUMBER = 6;
private com.google.protobuf.Internal.LongList writtenLengths_;
/**
* repeated uint64 written_lengths = 6;
* @return A list containing the writtenLengths.
*/
@java.lang.Override
public java.util.List
getWrittenLengthsList() {
return writtenLengths_;
}
/**
* repeated uint64 written_lengths = 6;
* @return The count of writtenLengths.
*/
public int getWrittenLengthsCount() {
return writtenLengths_.size();
}
/**
* repeated uint64 written_lengths = 6;
* @param index The index of the element to return.
* @return The writtenLengths at the given index.
*/
public long getWrittenLengths(int index) {
return writtenLengths_.getLong(index);
}
private int writtenLengthsMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (account_ != null) {
output.writeMessage(1, getAccount());
}
if (identifier_ != null) {
output.writeMessage(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, streamId_);
}
if (partitionId_ != 0) {
output.writeUInt32(4, partitionId_);
}
for (int i = 0; i < writtenFiles_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, writtenFiles_.getRaw(i));
}
if (getWrittenLengthsList().size() > 0) {
output.writeUInt32NoTag(50);
output.writeUInt32NoTag(writtenLengthsMemoizedSerializedSize);
}
for (int i = 0; i < writtenLengths_.size(); i++) {
output.writeUInt64NoTag(writtenLengths_.getLong(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (account_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getAccount());
}
if (identifier_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIdentifier());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(streamId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, streamId_);
}
if (partitionId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, partitionId_);
}
{
int dataSize = 0;
for (int i = 0; i < writtenFiles_.size(); i++) {
dataSize += computeStringSizeNoTag(writtenFiles_.getRaw(i));
}
size += dataSize;
size += 1 * getWrittenFilesList().size();
}
{
int dataSize = 0;
for (int i = 0; i < writtenLengths_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt64SizeNoTag(writtenLengths_.getLong(i));
}
size += dataSize;
if (!getWrittenLengthsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
writtenLengthsMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest other = (cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest) obj;
if (hasAccount() != other.hasAccount()) return false;
if (hasAccount()) {
if (!getAccount()
.equals(other.getAccount())) return false;
}
if (hasIdentifier() != other.hasIdentifier()) return false;
if (hasIdentifier()) {
if (!getIdentifier()
.equals(other.getIdentifier())) return false;
}
if (!getStreamId()
.equals(other.getStreamId())) return false;
if (getPartitionId()
!= other.getPartitionId()) return false;
if (!getWrittenFilesList()
.equals(other.getWrittenFilesList())) return false;
if (!getWrittenLengthsList()
.equals(other.getWrittenLengthsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAccount()) {
hash = (37 * hash) + ACCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getAccount().hashCode();
}
if (hasIdentifier()) {
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
}
hash = (37 * hash) + STREAM_ID_FIELD_NUMBER;
hash = (53 * hash) + getStreamId().hashCode();
hash = (37 * hash) + PARTITION_ID_FIELD_NUMBER;
hash = (53 * hash) + getPartitionId();
if (getWrittenFilesCount() > 0) {
hash = (37 * hash) + WRITTEN_FILES_FIELD_NUMBER;
hash = (53 * hash) + getWrittenFilesList().hashCode();
}
if (getWrittenLengthsCount() > 0) {
hash = (37 * hash) + WRITTEN_LENGTHS_FIELD_NUMBER;
hash = (53 * hash) + getWrittenLengthsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest)
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.class, cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (accountBuilder_ == null) {
account_ = null;
} else {
account_ = null;
accountBuilder_ = null;
}
if (identifierBuilder_ == null) {
identifier_ = null;
} else {
identifier_ = null;
identifierBuilder_ = null;
}
streamId_ = "";
partitionId_ = 0;
writtenFiles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
writtenLengths_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest build() {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest result = new cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest(this);
int from_bitField0_ = bitField0_;
if (accountBuilder_ == null) {
result.account_ = account_;
} else {
result.account_ = accountBuilder_.build();
}
if (identifierBuilder_ == null) {
result.identifier_ = identifier_;
} else {
result.identifier_ = identifierBuilder_.build();
}
result.streamId_ = streamId_;
result.partitionId_ = partitionId_;
if (((bitField0_ & 0x00000001) != 0)) {
writtenFiles_ = writtenFiles_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.writtenFiles_ = writtenFiles_;
if (((bitField0_ & 0x00000002) != 0)) {
writtenLengths_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.writtenLengths_ = writtenLengths_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest.getDefaultInstance()) return this;
if (other.hasAccount()) {
mergeAccount(other.getAccount());
}
if (other.hasIdentifier()) {
mergeIdentifier(other.getIdentifier());
}
if (!other.getStreamId().isEmpty()) {
streamId_ = other.streamId_;
onChanged();
}
if (other.getPartitionId() != 0) {
setPartitionId(other.getPartitionId());
}
if (!other.writtenFiles_.isEmpty()) {
if (writtenFiles_.isEmpty()) {
writtenFiles_ = other.writtenFiles_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureWrittenFilesIsMutable();
writtenFiles_.addAll(other.writtenFiles_);
}
onChanged();
}
if (!other.writtenLengths_.isEmpty()) {
if (writtenLengths_.isEmpty()) {
writtenLengths_ = other.writtenLengths_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureWrittenLengthsIsMutable();
writtenLengths_.addAll(other.writtenLengths_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.v2.IngestionV2.Account account_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder> accountBuilder_;
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return Whether the account field is set.
*/
public boolean hasAccount() {
return accountBuilder_ != null || account_ != null;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
* @return The account.
*/
public cz.proto.ingestion.v2.IngestionV2.Account getAccount() {
if (accountBuilder_ == null) {
return account_ == null ? cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
} else {
return accountBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
account_ = value;
onChanged();
} else {
accountBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder setAccount(
cz.proto.ingestion.v2.IngestionV2.Account.Builder builderForValue) {
if (accountBuilder_ == null) {
account_ = builderForValue.build();
onChanged();
} else {
accountBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder mergeAccount(cz.proto.ingestion.v2.IngestionV2.Account value) {
if (accountBuilder_ == null) {
if (account_ != null) {
account_ =
cz.proto.ingestion.v2.IngestionV2.Account.newBuilder(account_).mergeFrom(value).buildPartial();
} else {
account_ = value;
}
onChanged();
} else {
accountBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public Builder clearAccount() {
if (accountBuilder_ == null) {
account_ = null;
onChanged();
} else {
account_ = null;
accountBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.Account.Builder getAccountBuilder() {
onChanged();
return getAccountFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder getAccountOrBuilder() {
if (accountBuilder_ != null) {
return accountBuilder_.getMessageOrBuilder();
} else {
return account_ == null ?
cz.proto.ingestion.v2.IngestionV2.Account.getDefaultInstance() : account_;
}
}
/**
* .cz.proto.ingestion.v2.Account account = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>
getAccountFieldBuilder() {
if (accountBuilder_ == null) {
accountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.Account, cz.proto.ingestion.v2.IngestionV2.Account.Builder, cz.proto.ingestion.v2.IngestionV2.AccountOrBuilder>(
getAccount(),
getParentForChildren(),
isClean());
account_ = null;
}
return accountBuilder_;
}
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier identifier_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> identifierBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return Whether the identifier field is set.
*/
public boolean hasIdentifier() {
return identifierBuilder_ != null || identifier_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
* @return The identifier.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getIdentifier() {
if (identifierBuilder_ == null) {
return identifier_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
} else {
return identifierBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
} else {
identifierBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder setIdentifier(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (identifierBuilder_ == null) {
identifier_ = builderForValue.build();
onChanged();
} else {
identifierBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder mergeIdentifier(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (identifierBuilder_ == null) {
if (identifier_ != null) {
identifier_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(identifier_).mergeFrom(value).buildPartial();
} else {
identifier_ = value;
}
onChanged();
} else {
identifierBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public Builder clearIdentifier() {
if (identifierBuilder_ == null) {
identifier_ = null;
onChanged();
} else {
identifier_ = null;
identifierBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getIdentifierBuilder() {
onChanged();
return getIdentifierFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getIdentifierOrBuilder() {
if (identifierBuilder_ != null) {
return identifierBuilder_.getMessageOrBuilder();
} else {
return identifier_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : identifier_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier identifier = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getIdentifierFieldBuilder() {
if (identifierBuilder_ == null) {
identifierBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getIdentifier(),
getParentForChildren(),
isClean());
identifier_ = null;
}
return identifierBuilder_;
}
private java.lang.Object streamId_ = "";
/**
* string stream_id = 3;
* @return The streamId.
*/
public java.lang.String getStreamId() {
java.lang.Object ref = streamId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
streamId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string stream_id = 3;
* @return The bytes for streamId.
*/
public com.google.protobuf.ByteString
getStreamIdBytes() {
java.lang.Object ref = streamId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
streamId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string stream_id = 3;
* @param value The streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
streamId_ = value;
onChanged();
return this;
}
/**
* string stream_id = 3;
* @return This builder for chaining.
*/
public Builder clearStreamId() {
streamId_ = getDefaultInstance().getStreamId();
onChanged();
return this;
}
/**
* string stream_id = 3;
* @param value The bytes for streamId to set.
* @return This builder for chaining.
*/
public Builder setStreamIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
streamId_ = value;
onChanged();
return this;
}
private int partitionId_ ;
/**
* uint32 partition_id = 4;
* @return The partitionId.
*/
@java.lang.Override
public int getPartitionId() {
return partitionId_;
}
/**
* uint32 partition_id = 4;
* @param value The partitionId to set.
* @return This builder for chaining.
*/
public Builder setPartitionId(int value) {
partitionId_ = value;
onChanged();
return this;
}
/**
* uint32 partition_id = 4;
* @return This builder for chaining.
*/
public Builder clearPartitionId() {
partitionId_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList writtenFiles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureWrittenFilesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
writtenFiles_ = new com.google.protobuf.LazyStringArrayList(writtenFiles_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string written_files = 5;
* @return A list containing the writtenFiles.
*/
public com.google.protobuf.ProtocolStringList
getWrittenFilesList() {
return writtenFiles_.getUnmodifiableView();
}
/**
* repeated string written_files = 5;
* @return The count of writtenFiles.
*/
public int getWrittenFilesCount() {
return writtenFiles_.size();
}
/**
* repeated string written_files = 5;
* @param index The index of the element to return.
* @return The writtenFiles at the given index.
*/
public java.lang.String getWrittenFiles(int index) {
return writtenFiles_.get(index);
}
/**
* repeated string written_files = 5;
* @param index The index of the value to return.
* @return The bytes of the writtenFiles at the given index.
*/
public com.google.protobuf.ByteString
getWrittenFilesBytes(int index) {
return writtenFiles_.getByteString(index);
}
/**
* repeated string written_files = 5;
* @param index The index to set the value at.
* @param value The writtenFiles to set.
* @return This builder for chaining.
*/
public Builder setWrittenFiles(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureWrittenFilesIsMutable();
writtenFiles_.set(index, value);
onChanged();
return this;
}
/**
* repeated string written_files = 5;
* @param value The writtenFiles to add.
* @return This builder for chaining.
*/
public Builder addWrittenFiles(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureWrittenFilesIsMutable();
writtenFiles_.add(value);
onChanged();
return this;
}
/**
* repeated string written_files = 5;
* @param values The writtenFiles to add.
* @return This builder for chaining.
*/
public Builder addAllWrittenFiles(
java.lang.Iterable values) {
ensureWrittenFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, writtenFiles_);
onChanged();
return this;
}
/**
* repeated string written_files = 5;
* @return This builder for chaining.
*/
public Builder clearWrittenFiles() {
writtenFiles_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string written_files = 5;
* @param value The bytes of the writtenFiles to add.
* @return This builder for chaining.
*/
public Builder addWrittenFilesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureWrittenFilesIsMutable();
writtenFiles_.add(value);
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList writtenLengths_ = emptyLongList();
private void ensureWrittenLengthsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
writtenLengths_ = mutableCopy(writtenLengths_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated uint64 written_lengths = 6;
* @return A list containing the writtenLengths.
*/
public java.util.List
getWrittenLengthsList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(writtenLengths_) : writtenLengths_;
}
/**
* repeated uint64 written_lengths = 6;
* @return The count of writtenLengths.
*/
public int getWrittenLengthsCount() {
return writtenLengths_.size();
}
/**
* repeated uint64 written_lengths = 6;
* @param index The index of the element to return.
* @return The writtenLengths at the given index.
*/
public long getWrittenLengths(int index) {
return writtenLengths_.getLong(index);
}
/**
* repeated uint64 written_lengths = 6;
* @param index The index to set the value at.
* @param value The writtenLengths to set.
* @return This builder for chaining.
*/
public Builder setWrittenLengths(
int index, long value) {
ensureWrittenLengthsIsMutable();
writtenLengths_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated uint64 written_lengths = 6;
* @param value The writtenLengths to add.
* @return This builder for chaining.
*/
public Builder addWrittenLengths(long value) {
ensureWrittenLengthsIsMutable();
writtenLengths_.addLong(value);
onChanged();
return this;
}
/**
* repeated uint64 written_lengths = 6;
* @param values The writtenLengths to add.
* @return This builder for chaining.
*/
public Builder addAllWrittenLengths(
java.lang.Iterable extends java.lang.Long> values) {
ensureWrittenLengthsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, writtenLengths_);
onChanged();
return this;
}
/**
* repeated uint64 written_lengths = 6;
* @return This builder for chaining.
*/
public Builder clearWrittenLengths() {
writtenLengths_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterRequest)
private static final cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FinishBulkLoadStreamWriterRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FinishBulkLoadStreamWriterRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FinishBulkLoadStreamWriterResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse}
*/
public static final class FinishBulkLoadStreamWriterResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse)
FinishBulkLoadStreamWriterResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use FinishBulkLoadStreamWriterResponse.newBuilder() to construct.
private FinishBulkLoadStreamWriterResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FinishBulkLoadStreamWriterResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FinishBulkLoadStreamWriterResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FinishBulkLoadStreamWriterResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.class, cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (status_ != null) {
output.writeMessage(1, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse other = (cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse)
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.class, cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_FinishBulkLoadStreamWriterResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse build() {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse result = new cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse(this);
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse.getDefaultInstance()) return this;
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder> statusBuilder_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return statusBuilder_ != null || status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
* @return The status.
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
if (statusBuilder_ == null) {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
} else {
return statusBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
onChanged();
} else {
statusBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder setStatus(
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder builderForValue) {
if (statusBuilder_ == null) {
status_ = builderForValue.build();
onChanged();
} else {
statusBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder mergeStatus(cz.proto.ingestion.v2.IngestionV2.ResponseStatus value) {
if (statusBuilder_ == null) {
if (status_ != null) {
status_ =
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.newBuilder(status_).mergeFrom(value).buildPartial();
} else {
status_ = value;
}
onChanged();
} else {
statusBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public Builder clearStatus() {
if (statusBuilder_ == null) {
status_ = null;
onChanged();
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder getStatusBuilder() {
onChanged();
return getStatusFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
if (statusBuilder_ != null) {
return statusBuilder_.getMessageOrBuilder();
} else {
return status_ == null ?
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>
getStatusFieldBuilder() {
if (statusBuilder_ == null) {
statusBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.ResponseStatus, cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder, cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder>(
getStatus(),
getParentForChildren(),
isClean());
status_ = null;
}
return statusBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.FinishBulkLoadStreamWriterResponse)
private static final cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse();
}
public static cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FinishBulkLoadStreamWriterResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FinishBulkLoadStreamWriterResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.FinishBulkLoadStreamWriterResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetRouteWorkersRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetRouteWorkersRequest)
com.google.protobuf.MessageOrBuilder {
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
boolean hasTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent();
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder();
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The enum numeric value on the wire for connectMode.
*/
int getConnectModeValue();
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The connectMode.
*/
cz.proto.ingestion.v2.IngestionV2.ConnectMode getConnectMode();
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetRouteWorkersRequest}
*/
public static final class GetRouteWorkersRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetRouteWorkersRequest)
GetRouteWorkersRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetRouteWorkersRequest.newBuilder() to construct.
private GetRouteWorkersRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetRouteWorkersRequest() {
connectMode_ = 0;
tabletId_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetRouteWorkersRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetRouteWorkersRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder subBuilder = null;
if (tableIdent_ != null) {
subBuilder = tableIdent_.toBuilder();
}
tableIdent_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.TableIdentifier.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(tableIdent_);
tableIdent_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
connectMode_ = rawValue;
break;
}
case 24: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
tabletId_.addLong(input.readInt64());
break;
}
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.class, cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.Builder.class);
}
public static final int TABLE_IDENT_FIELD_NUMBER = 1;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
@java.lang.Override
public boolean hasTableIdent() {
return tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
return getTableIdent();
}
public static final int CONNECT_MODE_FIELD_NUMBER = 2;
private int connectMode_;
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The enum numeric value on the wire for connectMode.
*/
@java.lang.Override public int getConnectModeValue() {
return connectMode_;
}
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The connectMode.
*/
@java.lang.Override public cz.proto.ingestion.v2.IngestionV2.ConnectMode getConnectMode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.ConnectMode result = cz.proto.ingestion.v2.IngestionV2.ConnectMode.valueOf(connectMode_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.ConnectMode.UNRECOGNIZED : result;
}
public static final int TABLET_ID_FIELD_NUMBER = 3;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (tableIdent_ != null) {
output.writeMessage(1, getTableIdent());
}
if (connectMode_ != cz.proto.ingestion.v2.IngestionV2.ConnectMode.DIRECT.getNumber()) {
output.writeEnum(2, connectMode_);
}
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (tableIdent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTableIdent());
}
if (connectMode_ != cz.proto.ingestion.v2.IngestionV2.ConnectMode.DIRECT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, connectMode_);
}
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest other = (cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest) obj;
if (hasTableIdent() != other.hasTableIdent()) return false;
if (hasTableIdent()) {
if (!getTableIdent()
.equals(other.getTableIdent())) return false;
}
if (connectMode_ != other.connectMode_) return false;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTableIdent()) {
hash = (37 * hash) + TABLE_IDENT_FIELD_NUMBER;
hash = (53 * hash) + getTableIdent().hashCode();
}
hash = (37 * hash) + CONNECT_MODE_FIELD_NUMBER;
hash = (53 * hash) + connectMode_;
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetRouteWorkersRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetRouteWorkersRequest)
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.class, cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
connectMode_ = 0;
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersRequest_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest build() {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest result = new cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest(this);
int from_bitField0_ = bitField0_;
if (tableIdentBuilder_ == null) {
result.tableIdent_ = tableIdent_;
} else {
result.tableIdent_ = tableIdentBuilder_.build();
}
result.connectMode_ = connectMode_;
if (((bitField0_ & 0x00000001) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tabletId_ = tabletId_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest.getDefaultInstance()) return this;
if (other.hasTableIdent()) {
mergeTableIdent(other.getTableIdent());
}
if (other.connectMode_ != 0) {
setConnectModeValue(other.getConnectModeValue());
}
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private cz.proto.ingestion.v2.IngestionV2.TableIdentifier tableIdent_;
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder> tableIdentBuilder_;
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return Whether the tableIdent field is set.
*/
public boolean hasTableIdent() {
return tableIdentBuilder_ != null || tableIdent_ != null;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
* @return The tableIdent.
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier getTableIdent() {
if (tableIdentBuilder_ == null) {
return tableIdent_ == null ? cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
} else {
return tableIdentBuilder_.getMessage();
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder setTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
tableIdent_ = value;
onChanged();
} else {
tableIdentBuilder_.setMessage(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder setTableIdent(
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder builderForValue) {
if (tableIdentBuilder_ == null) {
tableIdent_ = builderForValue.build();
onChanged();
} else {
tableIdentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder mergeTableIdent(cz.proto.ingestion.v2.IngestionV2.TableIdentifier value) {
if (tableIdentBuilder_ == null) {
if (tableIdent_ != null) {
tableIdent_ =
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.newBuilder(tableIdent_).mergeFrom(value).buildPartial();
} else {
tableIdent_ = value;
}
onChanged();
} else {
tableIdentBuilder_.mergeFrom(value);
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public Builder clearTableIdent() {
if (tableIdentBuilder_ == null) {
tableIdent_ = null;
onChanged();
} else {
tableIdent_ = null;
tableIdentBuilder_ = null;
}
return this;
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder getTableIdentBuilder() {
onChanged();
return getTableIdentFieldBuilder().getBuilder();
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
public cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder getTableIdentOrBuilder() {
if (tableIdentBuilder_ != null) {
return tableIdentBuilder_.getMessageOrBuilder();
} else {
return tableIdent_ == null ?
cz.proto.ingestion.v2.IngestionV2.TableIdentifier.getDefaultInstance() : tableIdent_;
}
}
/**
* .cz.proto.ingestion.v2.TableIdentifier table_ident = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>
getTableIdentFieldBuilder() {
if (tableIdentBuilder_ == null) {
tableIdentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.TableIdentifier, cz.proto.ingestion.v2.IngestionV2.TableIdentifier.Builder, cz.proto.ingestion.v2.IngestionV2.TableIdentifierOrBuilder>(
getTableIdent(),
getParentForChildren(),
isClean());
tableIdent_ = null;
}
return tableIdentBuilder_;
}
private int connectMode_ = 0;
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The enum numeric value on the wire for connectMode.
*/
@java.lang.Override public int getConnectModeValue() {
return connectMode_;
}
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @param value The enum numeric value on the wire for connectMode to set.
* @return This builder for chaining.
*/
public Builder setConnectModeValue(int value) {
connectMode_ = value;
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return The connectMode.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ConnectMode getConnectMode() {
@SuppressWarnings("deprecation")
cz.proto.ingestion.v2.IngestionV2.ConnectMode result = cz.proto.ingestion.v2.IngestionV2.ConnectMode.valueOf(connectMode_);
return result == null ? cz.proto.ingestion.v2.IngestionV2.ConnectMode.UNRECOGNIZED : result;
}
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @param value The connectMode to set.
* @return This builder for chaining.
*/
public Builder setConnectMode(cz.proto.ingestion.v2.IngestionV2.ConnectMode value) {
if (value == null) {
throw new NullPointerException();
}
connectMode_ = value.getNumber();
onChanged();
return this;
}
/**
* .cz.proto.ingestion.v2.ConnectMode connect_mode = 2;
* @return This builder for chaining.
*/
public Builder clearConnectMode() {
connectMode_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList tabletId_ = emptyLongList();
private void ensureTabletIdIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tabletId_ = mutableCopy(tabletId_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated int64 tablet_id = 3;
* @return A list containing the tabletId.
*/
public java.util.List
getTabletIdList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(tabletId_) : tabletId_;
}
/**
* repeated int64 tablet_id = 3;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 3;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
/**
* repeated int64 tablet_id = 3;
* @param index The index to set the value at.
* @param value The tabletId to set.
* @return This builder for chaining.
*/
public Builder setTabletId(
int index, long value) {
ensureTabletIdIsMutable();
tabletId_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @param value The tabletId to add.
* @return This builder for chaining.
*/
public Builder addTabletId(long value) {
ensureTabletIdIsMutable();
tabletId_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @param values The tabletId to add.
* @return This builder for chaining.
*/
public Builder addAllTabletId(
java.lang.Iterable extends java.lang.Long> values) {
ensureTabletIdIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tabletId_);
onChanged();
return this;
}
/**
* repeated int64 tablet_id = 3;
* @return This builder for chaining.
*/
public Builder clearTabletId() {
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.GetRouteWorkersRequest)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.GetRouteWorkersRequest)
private static final cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest();
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetRouteWorkersRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetRouteWorkersRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HostPortTupleOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.HostPortTuple)
com.google.protobuf.MessageOrBuilder {
/**
* string host = 1;
* @return The host.
*/
java.lang.String getHost();
/**
* string host = 1;
* @return The bytes for host.
*/
com.google.protobuf.ByteString
getHostBytes();
/**
* int32 port = 2;
* @return The port.
*/
int getPort();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.HostPortTuple}
*/
public static final class HostPortTuple extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.HostPortTuple)
HostPortTupleOrBuilder {
private static final long serialVersionUID = 0L;
// Use HostPortTuple.newBuilder() to construct.
private HostPortTuple(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HostPortTuple() {
host_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HostPortTuple();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HostPortTuple(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
host_ = s;
break;
}
case 16: {
port_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_HostPortTuple_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_HostPortTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.HostPortTuple.class, cz.proto.ingestion.v2.IngestionV2.HostPortTuple.Builder.class);
}
public static final int HOST_FIELD_NUMBER = 1;
private volatile java.lang.Object host_;
/**
* string host = 1;
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
}
}
/**
* string host = 1;
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* int32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(host_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, host_);
}
if (port_ != 0) {
output.writeInt32(2, port_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(host_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, host_);
}
if (port_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, port_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.HostPortTuple)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.HostPortTuple other = (cz.proto.ingestion.v2.IngestionV2.HostPortTuple) obj;
if (!getHost()
.equals(other.getHost())) return false;
if (getPort()
!= other.getPort()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.HostPortTuple prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.HostPortTuple}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.HostPortTuple)
cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_HostPortTuple_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_HostPortTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.HostPortTuple.class, cz.proto.ingestion.v2.IngestionV2.HostPortTuple.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.HostPortTuple.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
host_ = "";
port_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_HostPortTuple_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTuple getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.HostPortTuple.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTuple build() {
cz.proto.ingestion.v2.IngestionV2.HostPortTuple result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTuple buildPartial() {
cz.proto.ingestion.v2.IngestionV2.HostPortTuple result = new cz.proto.ingestion.v2.IngestionV2.HostPortTuple(this);
result.host_ = host_;
result.port_ = port_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.HostPortTuple) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.HostPortTuple)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.HostPortTuple other) {
if (other == cz.proto.ingestion.v2.IngestionV2.HostPortTuple.getDefaultInstance()) return this;
if (!other.getHost().isEmpty()) {
host_ = other.host_;
onChanged();
}
if (other.getPort() != 0) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.HostPortTuple parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.HostPortTuple) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object host_ = "";
/**
* string host = 1;
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
host_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string host = 1;
* @return The bytes for host.
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string host = 1;
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
host_ = value;
onChanged();
return this;
}
/**
* string host = 1;
* @return This builder for chaining.
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* string host = 1;
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
host_ = value;
onChanged();
return this;
}
private int port_ ;
/**
* int32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
/**
* int32 port = 2;
* @param value The port to set.
* @return This builder for chaining.
*/
public Builder setPort(int value) {
port_ = value;
onChanged();
return this;
}
/**
* int32 port = 2;
* @return This builder for chaining.
*/
public Builder clearPort() {
port_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:cz.proto.ingestion.v2.HostPortTuple)
}
// @@protoc_insertion_point(class_scope:cz.proto.ingestion.v2.HostPortTuple)
private static final cz.proto.ingestion.v2.IngestionV2.HostPortTuple DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new cz.proto.ingestion.v2.IngestionV2.HostPortTuple();
}
public static cz.proto.ingestion.v2.IngestionV2.HostPortTuple getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HostPortTuple parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HostPortTuple(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTuple getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetRouteWorkersResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:cz.proto.ingestion.v2.GetRouteWorkersResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
java.util.List
getTupleList();
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
cz.proto.ingestion.v2.IngestionV2.HostPortTuple getTuple(int index);
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
int getTupleCount();
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
java.util.List extends cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder>
getTupleOrBuilderList();
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder getTupleOrBuilder(
int index);
/**
* repeated int64 tablet_id = 2;
* @return A list containing the tabletId.
*/
java.util.List getTabletIdList();
/**
* repeated int64 tablet_id = 2;
* @return The count of tabletId.
*/
int getTabletIdCount();
/**
* repeated int64 tablet_id = 2;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
long getTabletId(int index);
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
* @return The status.
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus();
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
*/
cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder();
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetRouteWorkersResponse}
*/
public static final class GetRouteWorkersResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:cz.proto.ingestion.v2.GetRouteWorkersResponse)
GetRouteWorkersResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetRouteWorkersResponse.newBuilder() to construct.
private GetRouteWorkersResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetRouteWorkersResponse() {
tuple_ = java.util.Collections.emptyList();
tabletId_ = emptyLongList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetRouteWorkersResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetRouteWorkersResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tuple_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
tuple_.add(
input.readMessage(cz.proto.ingestion.v2.IngestionV2.HostPortTuple.parser(), extensionRegistry));
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
tabletId_.addLong(input.readInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
tabletId_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
tabletId_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 26: {
cz.proto.ingestion.v2.IngestionV2.ResponseStatus.Builder subBuilder = null;
if (status_ != null) {
subBuilder = status_.toBuilder();
}
status_ = input.readMessage(cz.proto.ingestion.v2.IngestionV2.ResponseStatus.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(status_);
status_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tuple_ = java.util.Collections.unmodifiableList(tuple_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
tabletId_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.class, cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.Builder.class);
}
public static final int TUPLE_FIELD_NUMBER = 1;
private java.util.List tuple_;
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
@java.lang.Override
public java.util.List getTupleList() {
return tuple_;
}
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
@java.lang.Override
public java.util.List extends cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder>
getTupleOrBuilderList() {
return tuple_;
}
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
@java.lang.Override
public int getTupleCount() {
return tuple_.size();
}
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTuple getTuple(int index) {
return tuple_.get(index);
}
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder getTupleOrBuilder(
int index) {
return tuple_.get(index);
}
public static final int TABLET_ID_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.LongList tabletId_;
/**
* repeated int64 tablet_id = 2;
* @return A list containing the tabletId.
*/
@java.lang.Override
public java.util.List
getTabletIdList() {
return tabletId_;
}
/**
* repeated int64 tablet_id = 2;
* @return The count of tabletId.
*/
public int getTabletIdCount() {
return tabletId_.size();
}
/**
* repeated int64 tablet_id = 2;
* @param index The index of the element to return.
* @return The tabletId at the given index.
*/
public long getTabletId(int index) {
return tabletId_.getLong(index);
}
private int tabletIdMemoizedSerializedSize = -1;
public static final int STATUS_FIELD_NUMBER = 3;
private cz.proto.ingestion.v2.IngestionV2.ResponseStatus status_;
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return status_ != null;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
* @return The status.
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatus getStatus() {
return status_ == null ? cz.proto.ingestion.v2.IngestionV2.ResponseStatus.getDefaultInstance() : status_;
}
/**
* .cz.proto.ingestion.v2.ResponseStatus status = 3;
*/
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.ResponseStatusOrBuilder getStatusOrBuilder() {
return getStatus();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
for (int i = 0; i < tuple_.size(); i++) {
output.writeMessage(1, tuple_.get(i));
}
if (getTabletIdList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(tabletIdMemoizedSerializedSize);
}
for (int i = 0; i < tabletId_.size(); i++) {
output.writeInt64NoTag(tabletId_.getLong(i));
}
if (status_ != null) {
output.writeMessage(3, getStatus());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < tuple_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, tuple_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < tabletId_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(tabletId_.getLong(i));
}
size += dataSize;
if (!getTabletIdList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
tabletIdMemoizedSerializedSize = dataSize;
}
if (status_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStatus());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse)) {
return super.equals(obj);
}
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse other = (cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse) obj;
if (!getTupleList()
.equals(other.getTupleList())) return false;
if (!getTabletIdList()
.equals(other.getTabletIdList())) return false;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus()
.equals(other.getStatus())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getTupleCount() > 0) {
hash = (37 * hash) + TUPLE_FIELD_NUMBER;
hash = (53 * hash) + getTupleList().hashCode();
}
if (getTabletIdCount() > 0) {
hash = (37 * hash) + TABLET_ID_FIELD_NUMBER;
hash = (53 * hash) + getTabletIdList().hashCode();
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code cz.proto.ingestion.v2.GetRouteWorkersResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:cz.proto.ingestion.v2.GetRouteWorkersResponse)
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.class, cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.Builder.class);
}
// Construct using cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTupleFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (tupleBuilder_ == null) {
tuple_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
tupleBuilder_.clear();
}
tabletId_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
if (statusBuilder_ == null) {
status_ = null;
} else {
status_ = null;
statusBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return cz.proto.ingestion.v2.IngestionV2.internal_static_cz_proto_ingestion_v2_GetRouteWorkersResponse_descriptor;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse getDefaultInstanceForType() {
return cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.getDefaultInstance();
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse build() {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse buildPartial() {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse result = new cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse(this);
int from_bitField0_ = bitField0_;
if (tupleBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
tuple_ = java.util.Collections.unmodifiableList(tuple_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tuple_ = tuple_;
} else {
result.tuple_ = tupleBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
tabletId_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.tabletId_ = tabletId_;
if (statusBuilder_ == null) {
result.status_ = status_;
} else {
result.status_ = statusBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse) {
return mergeFrom((cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse other) {
if (other == cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse.getDefaultInstance()) return this;
if (tupleBuilder_ == null) {
if (!other.tuple_.isEmpty()) {
if (tuple_.isEmpty()) {
tuple_ = other.tuple_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTupleIsMutable();
tuple_.addAll(other.tuple_);
}
onChanged();
}
} else {
if (!other.tuple_.isEmpty()) {
if (tupleBuilder_.isEmpty()) {
tupleBuilder_.dispose();
tupleBuilder_ = null;
tuple_ = other.tuple_;
bitField0_ = (bitField0_ & ~0x00000001);
tupleBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTupleFieldBuilder() : null;
} else {
tupleBuilder_.addAllMessages(other.tuple_);
}
}
}
if (!other.tabletId_.isEmpty()) {
if (tabletId_.isEmpty()) {
tabletId_ = other.tabletId_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTabletIdIsMutable();
tabletId_.addAll(other.tabletId_);
}
onChanged();
}
if (other.hasStatus()) {
mergeStatus(other.getStatus());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (cz.proto.ingestion.v2.IngestionV2.GetRouteWorkersResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List tuple_ =
java.util.Collections.emptyList();
private void ensureTupleIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tuple_ = new java.util.ArrayList(tuple_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
cz.proto.ingestion.v2.IngestionV2.HostPortTuple, cz.proto.ingestion.v2.IngestionV2.HostPortTuple.Builder, cz.proto.ingestion.v2.IngestionV2.HostPortTupleOrBuilder> tupleBuilder_;
/**
* repeated .cz.proto.ingestion.v2.HostPortTuple tuple = 1;
*/
public java.util.List getTupleList() {
if (tupleBuilder_ == null) {
return java.util.Collections.unmodifiableList(tuple_);
} else {
return tupleBuilder_.getMessageList();
}
}
/**
*