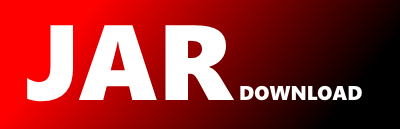
org.apache.arrow.memory.UnpooledNettyAllocationManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
package org.apache.arrow.memory;
import io.netty.buffer.UnpooledByteBufAllocatorL;
import io.netty.buffer.UnsafeDirectLittleEndian;
import io.netty.util.internal.PlatformDependent;
public class UnpooledNettyAllocationManager extends AllocationManager {
public static final AllocationManager.Factory FACTORY = new AllocationManager.Factory() {
public AllocationManager create(BufferAllocator accountingAllocator, long size) {
return new UnpooledNettyAllocationManager(accountingAllocator, size);
}
public ArrowBuf empty() {
return NettyAllocationManager.EMPTY_BUFFER;
}
};
public static final int DEFAULT_ALLOCATION_CUTOFF_VALUE = Integer.MAX_VALUE;
private static final UnpooledByteBufAllocatorL INNER_ALLOCATOR = new UnpooledByteBufAllocatorL();
static final UnsafeDirectLittleEndian EMPTY;
static final ArrowBuf EMPTY_BUFFER;
static final long CHUNK_SIZE;
private final long allocatedSize;
private final UnsafeDirectLittleEndian memoryChunk;
private final long allocatedAddress;
private final int allocationCutOffValue;
UnpooledNettyAllocationManager(BufferAllocator accountingAllocator, long requestedSize, int allocationCutOffValue) {
super(accountingAllocator);
this.allocationCutOffValue = allocationCutOffValue;
if (requestedSize > (long) allocationCutOffValue) {
this.memoryChunk = null;
this.allocatedAddress = PlatformDependent.allocateMemory(requestedSize);
this.allocatedSize = requestedSize;
} else {
this.memoryChunk = INNER_ALLOCATOR.allocate(requestedSize);
this.allocatedAddress = this.memoryChunk.memoryAddress();
this.allocatedSize = (long) this.memoryChunk.capacity();
}
}
UnpooledNettyAllocationManager(BufferAllocator accountingAllocator, long requestedSize) {
this(accountingAllocator, requestedSize, Integer.MAX_VALUE);
}
/**
* @deprecated
*/
@Deprecated
UnsafeDirectLittleEndian getMemoryChunk() {
return this.memoryChunk;
}
protected long memoryAddress() {
return this.allocatedAddress;
}
protected void release0() {
if (this.memoryChunk == null) {
PlatformDependent.freeMemory(this.allocatedAddress);
} else {
this.memoryChunk.release();
}
}
public long getSize() {
return this.allocatedSize;
}
static {
EMPTY = INNER_ALLOCATOR.empty;
EMPTY_BUFFER = new ArrowBuf(ReferenceManager.NO_OP, (BufferManager) null, 0L, EMPTY.memoryAddress());
CHUNK_SIZE = (long) INNER_ALLOCATOR.getChunkSize();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy