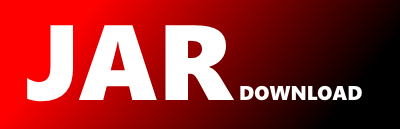
org.apache.kudu.KuduCommon Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clickzetta-java Show documentation
Show all versions of clickzetta-java Show documentation
The java SDK for clickzetta's Lakehouse
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: kudu_common.proto
package org.apache.kudu;
public final class KuduCommon {
private KuduCommon() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
*
* If you add a new type keep in mind to add it to the end
* or update AddMapping() functions like the one in key_encoder.cc
* that have a vector that maps the protobuf tag with the index.
*
*
* Protobuf enum {@code kudu.DataType}
*/
public enum DataType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_DATA = 999;
*/
UNKNOWN_DATA(999),
/**
* UINT8 = 0;
*/
UINT8(0),
/**
* INT8 = 1;
*/
INT8(1),
/**
* UINT16 = 2;
*/
UINT16(2),
/**
* INT16 = 3;
*/
INT16(3),
/**
* UINT32 = 4;
*/
UINT32(4),
/**
* INT32 = 5;
*/
INT32(5),
/**
* UINT64 = 6;
*/
UINT64(6),
/**
* INT64 = 7;
*/
INT64(7),
/**
* STRING = 8;
*/
STRING(8),
/**
* BOOL = 9;
*/
BOOL(9),
/**
* FLOAT = 10;
*/
FLOAT(10),
/**
* DOUBLE = 11;
*/
DOUBLE(11),
/**
* BINARY = 12;
*/
BINARY(12),
/**
* UNIXTIME_MICROS = 13;
*/
UNIXTIME_MICROS(13),
/**
* INT128 = 14;
*/
INT128(14),
/**
* DECIMAL32 = 15;
*/
DECIMAL32(15),
/**
* DECIMAL64 = 16;
*/
DECIMAL64(16),
/**
* DECIMAL128 = 17;
*/
DECIMAL128(17),
/**
*
* virtual column; not a real data type
*
*
* IS_DELETED = 18;
*/
IS_DELETED(18),
/**
* VARCHAR = 19;
*/
VARCHAR(19),
/**
* DATE = 20;
*/
DATE(20),
;
/**
* UNKNOWN_DATA = 999;
*/
public static final int UNKNOWN_DATA_VALUE = 999;
/**
* UINT8 = 0;
*/
public static final int UINT8_VALUE = 0;
/**
* INT8 = 1;
*/
public static final int INT8_VALUE = 1;
/**
* UINT16 = 2;
*/
public static final int UINT16_VALUE = 2;
/**
* INT16 = 3;
*/
public static final int INT16_VALUE = 3;
/**
* UINT32 = 4;
*/
public static final int UINT32_VALUE = 4;
/**
* INT32 = 5;
*/
public static final int INT32_VALUE = 5;
/**
* UINT64 = 6;
*/
public static final int UINT64_VALUE = 6;
/**
* INT64 = 7;
*/
public static final int INT64_VALUE = 7;
/**
* STRING = 8;
*/
public static final int STRING_VALUE = 8;
/**
* BOOL = 9;
*/
public static final int BOOL_VALUE = 9;
/**
* FLOAT = 10;
*/
public static final int FLOAT_VALUE = 10;
/**
* DOUBLE = 11;
*/
public static final int DOUBLE_VALUE = 11;
/**
* BINARY = 12;
*/
public static final int BINARY_VALUE = 12;
/**
* UNIXTIME_MICROS = 13;
*/
public static final int UNIXTIME_MICROS_VALUE = 13;
/**
* INT128 = 14;
*/
public static final int INT128_VALUE = 14;
/**
* DECIMAL32 = 15;
*/
public static final int DECIMAL32_VALUE = 15;
/**
* DECIMAL64 = 16;
*/
public static final int DECIMAL64_VALUE = 16;
/**
* DECIMAL128 = 17;
*/
public static final int DECIMAL128_VALUE = 17;
/**
*
* virtual column; not a real data type
*
*
* IS_DELETED = 18;
*/
public static final int IS_DELETED_VALUE = 18;
/**
* VARCHAR = 19;
*/
public static final int VARCHAR_VALUE = 19;
/**
* DATE = 20;
*/
public static final int DATE_VALUE = 20;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DataType forNumber(int value) {
switch (value) {
case 999: return UNKNOWN_DATA;
case 0: return UINT8;
case 1: return INT8;
case 2: return UINT16;
case 3: return INT16;
case 4: return UINT32;
case 5: return INT32;
case 6: return UINT64;
case 7: return INT64;
case 8: return STRING;
case 9: return BOOL;
case 10: return FLOAT;
case 11: return DOUBLE;
case 12: return BINARY;
case 13: return UNIXTIME_MICROS;
case 14: return INT128;
case 15: return DECIMAL32;
case 16: return DECIMAL64;
case 17: return DECIMAL128;
case 18: return IS_DELETED;
case 19: return VARCHAR;
case 20: return DATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DataType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DataType findValueByNumber(int number) {
return DataType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(0);
}
private static final DataType[] VALUES = values();
public static DataType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private DataType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.DataType)
}
/**
* Protobuf enum {@code kudu.EncodingType}
*/
public enum EncodingType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_ENCODING = 999;
*/
UNKNOWN_ENCODING(999),
/**
* AUTO_ENCODING = 0;
*/
AUTO_ENCODING(0),
/**
* PLAIN_ENCODING = 1;
*/
PLAIN_ENCODING(1),
/**
* PREFIX_ENCODING = 2;
*/
PREFIX_ENCODING(2),
/**
*
* GROUP_VARINT encoding is deprecated and no longer implemented.
*
*
* GROUP_VARINT = 3;
*/
GROUP_VARINT(3),
/**
* RLE = 4;
*/
RLE(4),
/**
* DICT_ENCODING = 5;
*/
DICT_ENCODING(5),
/**
* BIT_SHUFFLE = 6;
*/
BIT_SHUFFLE(6),
;
/**
* UNKNOWN_ENCODING = 999;
*/
public static final int UNKNOWN_ENCODING_VALUE = 999;
/**
* AUTO_ENCODING = 0;
*/
public static final int AUTO_ENCODING_VALUE = 0;
/**
* PLAIN_ENCODING = 1;
*/
public static final int PLAIN_ENCODING_VALUE = 1;
/**
* PREFIX_ENCODING = 2;
*/
public static final int PREFIX_ENCODING_VALUE = 2;
/**
*
* GROUP_VARINT encoding is deprecated and no longer implemented.
*
*
* GROUP_VARINT = 3;
*/
public static final int GROUP_VARINT_VALUE = 3;
/**
* RLE = 4;
*/
public static final int RLE_VALUE = 4;
/**
* DICT_ENCODING = 5;
*/
public static final int DICT_ENCODING_VALUE = 5;
/**
* BIT_SHUFFLE = 6;
*/
public static final int BIT_SHUFFLE_VALUE = 6;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EncodingType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static EncodingType forNumber(int value) {
switch (value) {
case 999: return UNKNOWN_ENCODING;
case 0: return AUTO_ENCODING;
case 1: return PLAIN_ENCODING;
case 2: return PREFIX_ENCODING;
case 3: return GROUP_VARINT;
case 4: return RLE;
case 5: return DICT_ENCODING;
case 6: return BIT_SHUFFLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EncodingType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EncodingType findValueByNumber(int number) {
return EncodingType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(1);
}
private static final EncodingType[] VALUES = values();
public static EncodingType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private EncodingType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.EncodingType)
}
/**
*
* Enums that specify the HMS-related configurations for a Kudu mini-cluster.
*
*
* Protobuf enum {@code kudu.HmsMode}
*/
public enum HmsMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* No HMS will be started.
*
*
* NONE = 0;
*/
NONE(0),
/**
*
* The HMS will be started, but will not be configured to use the Kudu
* plugin.
*
*
* DISABLE_HIVE_METASTORE = 3;
*/
DISABLE_HIVE_METASTORE(3),
/**
*
* The HMS will be started and configured to use the Kudu plugin, but the
* Kudu mini-cluster will not be configured to synchronize with it.
*
*
* ENABLE_HIVE_METASTORE = 1;
*/
ENABLE_HIVE_METASTORE(1),
/**
*
* The HMS will be started and configured to use the Kudu plugin, and the
* Kudu mini-cluster will be configured to synchronize with it.
*
*
* ENABLE_METASTORE_INTEGRATION = 2;
*/
ENABLE_METASTORE_INTEGRATION(2),
;
/**
*
* No HMS will be started.
*
*
* NONE = 0;
*/
public static final int NONE_VALUE = 0;
/**
*
* The HMS will be started, but will not be configured to use the Kudu
* plugin.
*
*
* DISABLE_HIVE_METASTORE = 3;
*/
public static final int DISABLE_HIVE_METASTORE_VALUE = 3;
/**
*
* The HMS will be started and configured to use the Kudu plugin, but the
* Kudu mini-cluster will not be configured to synchronize with it.
*
*
* ENABLE_HIVE_METASTORE = 1;
*/
public static final int ENABLE_HIVE_METASTORE_VALUE = 1;
/**
*
* The HMS will be started and configured to use the Kudu plugin, and the
* Kudu mini-cluster will be configured to synchronize with it.
*
*
* ENABLE_METASTORE_INTEGRATION = 2;
*/
public static final int ENABLE_METASTORE_INTEGRATION_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static HmsMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static HmsMode forNumber(int value) {
switch (value) {
case 0: return NONE;
case 3: return DISABLE_HIVE_METASTORE;
case 1: return ENABLE_HIVE_METASTORE;
case 2: return ENABLE_METASTORE_INTEGRATION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
HmsMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public HmsMode findValueByNumber(int number) {
return HmsMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(2);
}
private static final HmsMode[] VALUES = values();
public static HmsMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private HmsMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.HmsMode)
}
/**
*
* The external consistency mode for client requests.
* This defines how ops and/or sequences of operations that touch
* several TabletServers, in different machines, can be observed by external
* clients.
* Note that ExternalConsistencyMode makes no guarantee on atomicity, i.e.
* no sequence of operations is made atomic (or transactional) just because
* an external consistency mode is set.
* Note also that ExternalConsistencyMode has no implication on the
* consistency between replicas of the same tablet.
*
*
* Protobuf enum {@code kudu.ExternalConsistencyMode}
*/
public enum ExternalConsistencyMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_EXTERNAL_CONSISTENCY_MODE = 0;
*/
UNKNOWN_EXTERNAL_CONSISTENCY_MODE(0),
/**
*
* The response to any write will contain a timestamp.
* Any further calls from the same client to other servers will update
* those servers with that timestamp. The user will make sure that the
* timestamp is propagated through back-channels to other
* KuduClient's.
* WARNING: Failure to propagate timestamp information through
* back-channels will negate any external consistency guarantee under this
* mode.
* Example:
* 1 - Client A executes operation X in Tablet A
* 2 - Afterwards, Client A executes operation Y in Tablet B
* Client B may observe the following operation sequences:
* {}, {X}, {X Y}
* This is the default mode.
*
*
* CLIENT_PROPAGATED = 1;
*/
CLIENT_PROPAGATED(1),
/**
*
* The server will guarantee that each op is externally consistent by making
* sure that none of its results are visible until every Kudu server agrees
* that the op is in the past. The client is not obligated to forward
* timestamp information through back-channels.
* WARNING: Depending on the clock synchronization state of TabletServers
* this may imply considerable latency. Moreover operations with
* COMMIT_WAIT requested external consistency will outright fail if
* TabletServer clocks are either unsynchronized or synchronized but
* with a maximum error which surpasses a pre-configured one.
* Example:
* - Client A executes operation X in Tablet A
* - Afterwards, Client A executes operation Y in Tablet B
* Client B may observe the following operation sequences:
* {}, {X}, {X Y}
*
*
* COMMIT_WAIT = 2;
*/
COMMIT_WAIT(2),
;
/**
* UNKNOWN_EXTERNAL_CONSISTENCY_MODE = 0;
*/
public static final int UNKNOWN_EXTERNAL_CONSISTENCY_MODE_VALUE = 0;
/**
*
* The response to any write will contain a timestamp.
* Any further calls from the same client to other servers will update
* those servers with that timestamp. The user will make sure that the
* timestamp is propagated through back-channels to other
* KuduClient's.
* WARNING: Failure to propagate timestamp information through
* back-channels will negate any external consistency guarantee under this
* mode.
* Example:
* 1 - Client A executes operation X in Tablet A
* 2 - Afterwards, Client A executes operation Y in Tablet B
* Client B may observe the following operation sequences:
* {}, {X}, {X Y}
* This is the default mode.
*
*
* CLIENT_PROPAGATED = 1;
*/
public static final int CLIENT_PROPAGATED_VALUE = 1;
/**
*
* The server will guarantee that each op is externally consistent by making
* sure that none of its results are visible until every Kudu server agrees
* that the op is in the past. The client is not obligated to forward
* timestamp information through back-channels.
* WARNING: Depending on the clock synchronization state of TabletServers
* this may imply considerable latency. Moreover operations with
* COMMIT_WAIT requested external consistency will outright fail if
* TabletServer clocks are either unsynchronized or synchronized but
* with a maximum error which surpasses a pre-configured one.
* Example:
* - Client A executes operation X in Tablet A
* - Afterwards, Client A executes operation Y in Tablet B
* Client B may observe the following operation sequences:
* {}, {X}, {X Y}
*
*
* COMMIT_WAIT = 2;
*/
public static final int COMMIT_WAIT_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ExternalConsistencyMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ExternalConsistencyMode forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_EXTERNAL_CONSISTENCY_MODE;
case 1: return CLIENT_PROPAGATED;
case 2: return COMMIT_WAIT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ExternalConsistencyMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ExternalConsistencyMode findValueByNumber(int number) {
return ExternalConsistencyMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(3);
}
private static final ExternalConsistencyMode[] VALUES = values();
public static ExternalConsistencyMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ExternalConsistencyMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.ExternalConsistencyMode)
}
/**
*
* The possible read modes for clients.
* Clients set these in Scan requests.
* The server keeps 2 snapshot boundaries:
* - The earliest snapshot: this corresponds to the earliest kept undo records
* in the tablet, meaning the current state (Base) can be undone up to
* this snapshot.
* - The latest snapshot: This corresponds to the instant beyond which no op
* will have an earlier timestamp. Usually this corresponds to whatever
* clock->Now() returns, but can be higher if the client propagates a
* timestamp (see below).
*
*
* Protobuf enum {@code kudu.ReadMode}
*/
public enum ReadMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_READ_MODE = 0;
*/
UNKNOWN_READ_MODE(0),
/**
*
* When READ_LATEST is specified the server will execute the read independently
* of the clock and will always return all visible writes at the time the request
* was received. This type of read does not return a snapshot timestamp since
* it might not be repeatable, i.e. a later read executed at the same snapshot
* timestamp might yield rows that were committed by in-flight ops.
* This is the default mode.
*
*
* READ_LATEST = 1;
*/
READ_LATEST(1),
/**
*
* When READ_AT_SNAPSHOT is specified the server will attempt to perform a read
* at the required snapshot. If no snapshot is defined the server will take the
* current time as the snapshot timestamp. Snapshot reads are repeatable, i.e.
* all future reads at the same timestamp will yield the same rows. This is
* performed at the expense of waiting for in-flight ops whose timestamp
* is lower than the snapshot's timestamp to complete.
* When mixing reads and writes clients that specify COMMIT_WAIT as their
* external consistency mode and then use the returned write_timestamp
* to perform snapshot reads are guaranteed that that snapshot time is
* considered in the past by all servers and no additional action is
* necessary. Clients using CLIENT_PROPAGATED however must forcibly propagate
* the timestamps even at read time, so that the server will not generate
* any more ops before the snapshot requested by the client.
* The latter option is implemented by allowing the client to specify one or
* two timestamps, the first one obtained from the previous CLIENT_PROPAGATED
* write, directly or through back-channels, must be signed and will be
* checked by the server. The second one, if defined, is the actual snapshot
* read time. When selecting both the latter must be lower than or equal to
* the former.
* TODO implement actually signing the propagated timestamp.
*
*
* READ_AT_SNAPSHOT = 2;
*/
READ_AT_SNAPSHOT(2),
/**
*
* When READ_YOUR_WRITES is specified, the server will pick a timestamp to use
* for a server-local snapshot scan subject to the following criteria:
* (1) It will be higher than the propagated timestamp,
* (2) It will attempt to minimize latency caused by waiting for outstanding
* write ops to complete.
* More specifically, the server will choose the latest timestamp higher than
* the provided propagated timestamp bound that allows execution of the
* reads without being blocked by the in-flight ops (however the
* read can be blocked if the propagated timestamp is higher than some in-flight
* ops). If no propagated timestamp is provided the server will choose
* a timestamp such that all ops before it are committed. The chosen
* timestamp will be returned back to the client as 'snapshot timestamp'. The Kudu
* client library will use it as the propagated timestamp for subsequent reads
* to avoid unnecessarily waiting.
* Reads in this mode are not repeatable: two READ_YOUR_WRITES reads, even if
* they provide the same propagated timestamp bound, can execute at different
* timestamps and thus return different results. However, it allows
* read-your-writes and read-your-reads for each client, as the chosen
* timestamp must be higher than the one of the last write or read,
* known from the propagated timestamp.
*
*
* READ_YOUR_WRITES = 3;
*/
READ_YOUR_WRITES(3),
;
/**
* UNKNOWN_READ_MODE = 0;
*/
public static final int UNKNOWN_READ_MODE_VALUE = 0;
/**
*
* When READ_LATEST is specified the server will execute the read independently
* of the clock and will always return all visible writes at the time the request
* was received. This type of read does not return a snapshot timestamp since
* it might not be repeatable, i.e. a later read executed at the same snapshot
* timestamp might yield rows that were committed by in-flight ops.
* This is the default mode.
*
*
* READ_LATEST = 1;
*/
public static final int READ_LATEST_VALUE = 1;
/**
*
* When READ_AT_SNAPSHOT is specified the server will attempt to perform a read
* at the required snapshot. If no snapshot is defined the server will take the
* current time as the snapshot timestamp. Snapshot reads are repeatable, i.e.
* all future reads at the same timestamp will yield the same rows. This is
* performed at the expense of waiting for in-flight ops whose timestamp
* is lower than the snapshot's timestamp to complete.
* When mixing reads and writes clients that specify COMMIT_WAIT as their
* external consistency mode and then use the returned write_timestamp
* to perform snapshot reads are guaranteed that that snapshot time is
* considered in the past by all servers and no additional action is
* necessary. Clients using CLIENT_PROPAGATED however must forcibly propagate
* the timestamps even at read time, so that the server will not generate
* any more ops before the snapshot requested by the client.
* The latter option is implemented by allowing the client to specify one or
* two timestamps, the first one obtained from the previous CLIENT_PROPAGATED
* write, directly or through back-channels, must be signed and will be
* checked by the server. The second one, if defined, is the actual snapshot
* read time. When selecting both the latter must be lower than or equal to
* the former.
* TODO implement actually signing the propagated timestamp.
*
*
* READ_AT_SNAPSHOT = 2;
*/
public static final int READ_AT_SNAPSHOT_VALUE = 2;
/**
*
* When READ_YOUR_WRITES is specified, the server will pick a timestamp to use
* for a server-local snapshot scan subject to the following criteria:
* (1) It will be higher than the propagated timestamp,
* (2) It will attempt to minimize latency caused by waiting for outstanding
* write ops to complete.
* More specifically, the server will choose the latest timestamp higher than
* the provided propagated timestamp bound that allows execution of the
* reads without being blocked by the in-flight ops (however the
* read can be blocked if the propagated timestamp is higher than some in-flight
* ops). If no propagated timestamp is provided the server will choose
* a timestamp such that all ops before it are committed. The chosen
* timestamp will be returned back to the client as 'snapshot timestamp'. The Kudu
* client library will use it as the propagated timestamp for subsequent reads
* to avoid unnecessarily waiting.
* Reads in this mode are not repeatable: two READ_YOUR_WRITES reads, even if
* they provide the same propagated timestamp bound, can execute at different
* timestamps and thus return different results. However, it allows
* read-your-writes and read-your-reads for each client, as the chosen
* timestamp must be higher than the one of the last write or read,
* known from the propagated timestamp.
*
*
* READ_YOUR_WRITES = 3;
*/
public static final int READ_YOUR_WRITES_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReadMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ReadMode forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_READ_MODE;
case 1: return READ_LATEST;
case 2: return READ_AT_SNAPSHOT;
case 3: return READ_YOUR_WRITES;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReadMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReadMode findValueByNumber(int number) {
return ReadMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(4);
}
private static final ReadMode[] VALUES = values();
public static ReadMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReadMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.ReadMode)
}
/**
*
* The possible order modes for clients.
* Clients specify these in new scan requests.
* Ordered scans are fault-tolerant, and can be retried elsewhere in the case
* of tablet server failure. However, ordered scans impose additional overhead
* since the tablet server needs to sort the result rows.
*
*
* Protobuf enum {@code kudu.OrderMode}
*/
public enum OrderMode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_ORDER_MODE = 0;
*/
UNKNOWN_ORDER_MODE(0),
/**
*
* This is the default order mode.
*
*
* UNORDERED = 1;
*/
UNORDERED(1),
/**
* ORDERED = 2;
*/
ORDERED(2),
;
/**
* UNKNOWN_ORDER_MODE = 0;
*/
public static final int UNKNOWN_ORDER_MODE_VALUE = 0;
/**
*
* This is the default order mode.
*
*
* UNORDERED = 1;
*/
public static final int UNORDERED_VALUE = 1;
/**
* ORDERED = 2;
*/
public static final int ORDERED_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OrderMode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OrderMode forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_ORDER_MODE;
case 1: return UNORDERED;
case 2: return ORDERED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OrderMode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OrderMode findValueByNumber(int number) {
return OrderMode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(5);
}
private static final OrderMode[] VALUES = values();
public static OrderMode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private OrderMode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.OrderMode)
}
/**
*
* Policy with which to choose among multiple replicas.
*
*
* Protobuf enum {@code kudu.ReplicaSelection}
*/
public enum ReplicaSelection
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_REPLICA_SELECTION = 0;
*/
UNKNOWN_REPLICA_SELECTION(0),
/**
*
* Select the LEADER replica.
*
*
* LEADER_ONLY = 1;
*/
LEADER_ONLY(1),
/**
*
* Select the closest replica to the client. Replicas are classified from
* closest to furthest as follows:
* - Local replicas
* - Replicas whose tablet server has the same location as the client
* - All other replicas
*
*
* CLOSEST_REPLICA = 2;
*/
CLOSEST_REPLICA(2),
;
/**
* UNKNOWN_REPLICA_SELECTION = 0;
*/
public static final int UNKNOWN_REPLICA_SELECTION_VALUE = 0;
/**
*
* Select the LEADER replica.
*
*
* LEADER_ONLY = 1;
*/
public static final int LEADER_ONLY_VALUE = 1;
/**
*
* Select the closest replica to the client. Replicas are classified from
* closest to furthest as follows:
* - Local replicas
* - Replicas whose tablet server has the same location as the client
* - All other replicas
*
*
* CLOSEST_REPLICA = 2;
*/
public static final int CLOSEST_REPLICA_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ReplicaSelection valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ReplicaSelection forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_REPLICA_SELECTION;
case 1: return LEADER_ONLY;
case 2: return CLOSEST_REPLICA;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ReplicaSelection> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ReplicaSelection findValueByNumber(int number) {
return ReplicaSelection.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(6);
}
private static final ReplicaSelection[] VALUES = values();
public static ReplicaSelection valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ReplicaSelection(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.ReplicaSelection)
}
/**
*
* The type of a given table. This is useful in determining whether a
* table/tablet stores user-specified data, as opposed to being a Kudu-internal
* system table.
*
*
* Protobuf enum {@code kudu.TableTypePB}
*/
public enum TableTypePB
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The table stores user data.
*
*
* DEFAULT_TABLE = 0;
*/
DEFAULT_TABLE(0),
/**
*
* The table stores transaction status management metadata.
*
*
* TXN_STATUS_TABLE = 1;
*/
TXN_STATUS_TABLE(1),
;
/**
*
* The table stores user data.
*
*
* DEFAULT_TABLE = 0;
*/
public static final int DEFAULT_TABLE_VALUE = 0;
/**
*
* The table stores transaction status management metadata.
*
*
* TXN_STATUS_TABLE = 1;
*/
public static final int TXN_STATUS_TABLE_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TableTypePB valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TableTypePB forNumber(int value) {
switch (value) {
case 0: return DEFAULT_TABLE;
case 1: return TXN_STATUS_TABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TableTypePB> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TableTypePB findValueByNumber(int number) {
return TableTypePB.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.getDescriptor().getEnumTypes().get(7);
}
private static final TableTypePB[] VALUES = values();
public static TableTypePB valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private TableTypePB(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:kudu.TableTypePB)
}
public interface ColumnTypeAttributesPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnTypeAttributesPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return Whether the precision field is set.
*/
boolean hasPrecision();
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return The precision.
*/
int getPrecision();
/**
* optional int32 scale = 2;
* @return Whether the scale field is set.
*/
boolean hasScale();
/**
* optional int32 scale = 2;
* @return The scale.
*/
int getScale();
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return Whether the length field is set.
*/
boolean hasLength();
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return The length.
*/
int getLength();
/**
* optional int32 specialColCode = 4;
* @return Whether the specialColCode field is set.
*/
boolean hasSpecialColCode();
/**
* optional int32 specialColCode = 4;
* @return The specialColCode.
*/
int getSpecialColCode();
}
/**
*
* Holds detailed attributes for the column. Only certain fields will be set,
* depending on the type of the column.
*
*
* Protobuf type {@code kudu.ColumnTypeAttributesPB}
*/
public static final class ColumnTypeAttributesPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnTypeAttributesPB)
ColumnTypeAttributesPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnTypeAttributesPB.newBuilder() to construct.
private ColumnTypeAttributesPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnTypeAttributesPB() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnTypeAttributesPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnTypeAttributesPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
precision_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
scale_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
length_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
specialColCode_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnTypeAttributesPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnTypeAttributesPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.class, org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder.class);
}
private int bitField0_;
public static final int PRECISION_FIELD_NUMBER = 1;
private int precision_;
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return Whether the precision field is set.
*/
@java.lang.Override
public boolean hasPrecision() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return The precision.
*/
@java.lang.Override
public int getPrecision() {
return precision_;
}
public static final int SCALE_FIELD_NUMBER = 2;
private int scale_;
/**
* optional int32 scale = 2;
* @return Whether the scale field is set.
*/
@java.lang.Override
public boolean hasScale() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 scale = 2;
* @return The scale.
*/
@java.lang.Override
public int getScale() {
return scale_;
}
public static final int LENGTH_FIELD_NUMBER = 3;
private int length_;
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return Whether the length field is set.
*/
@java.lang.Override
public boolean hasLength() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
public static final int SPECIALCOLCODE_FIELD_NUMBER = 4;
private int specialColCode_;
/**
* optional int32 specialColCode = 4;
* @return Whether the specialColCode field is set.
*/
@java.lang.Override
public boolean hasSpecialColCode() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 specialColCode = 4;
* @return The specialColCode.
*/
@java.lang.Override
public int getSpecialColCode() {
return specialColCode_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, precision_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, scale_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(3, length_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, specialColCode_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, precision_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, scale_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, length_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, specialColCode_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnTypeAttributesPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB other = (org.apache.kudu.KuduCommon.ColumnTypeAttributesPB) obj;
if (hasPrecision() != other.hasPrecision()) return false;
if (hasPrecision()) {
if (getPrecision()
!= other.getPrecision()) return false;
}
if (hasScale() != other.hasScale()) return false;
if (hasScale()) {
if (getScale()
!= other.getScale()) return false;
}
if (hasLength() != other.hasLength()) return false;
if (hasLength()) {
if (getLength()
!= other.getLength()) return false;
}
if (hasSpecialColCode() != other.hasSpecialColCode()) return false;
if (hasSpecialColCode()) {
if (getSpecialColCode()
!= other.getSpecialColCode()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPrecision()) {
hash = (37 * hash) + PRECISION_FIELD_NUMBER;
hash = (53 * hash) + getPrecision();
}
if (hasScale()) {
hash = (37 * hash) + SCALE_FIELD_NUMBER;
hash = (53 * hash) + getScale();
}
if (hasLength()) {
hash = (37 * hash) + LENGTH_FIELD_NUMBER;
hash = (53 * hash) + getLength();
}
if (hasSpecialColCode()) {
hash = (37 * hash) + SPECIALCOLCODE_FIELD_NUMBER;
hash = (53 * hash) + getSpecialColCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnTypeAttributesPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Holds detailed attributes for the column. Only certain fields will be set,
* depending on the type of the column.
*
*
* Protobuf type {@code kudu.ColumnTypeAttributesPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnTypeAttributesPB)
org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnTypeAttributesPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnTypeAttributesPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.class, org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
precision_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
scale_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
length_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
specialColCode_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnTypeAttributesPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB build() {
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB buildPartial() {
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB result = new org.apache.kudu.KuduCommon.ColumnTypeAttributesPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.precision_ = precision_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.scale_ = scale_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.length_ = length_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.specialColCode_ = specialColCode_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnTypeAttributesPB) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnTypeAttributesPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnTypeAttributesPB other) {
if (other == org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance()) return this;
if (other.hasPrecision()) {
setPrecision(other.getPrecision());
}
if (other.hasScale()) {
setScale(other.getScale());
}
if (other.hasLength()) {
setLength(other.getLength());
}
if (other.hasSpecialColCode()) {
setSpecialColCode(other.getSpecialColCode());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnTypeAttributesPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int precision_ ;
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return Whether the precision field is set.
*/
@java.lang.Override
public boolean hasPrecision() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return The precision.
*/
@java.lang.Override
public int getPrecision() {
return precision_;
}
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @param value The precision to set.
* @return This builder for chaining.
*/
public Builder setPrecision(int value) {
bitField0_ |= 0x00000001;
precision_ = value;
onChanged();
return this;
}
/**
*
* For decimal columns
*
*
* optional int32 precision = 1;
* @return This builder for chaining.
*/
public Builder clearPrecision() {
bitField0_ = (bitField0_ & ~0x00000001);
precision_ = 0;
onChanged();
return this;
}
private int scale_ ;
/**
* optional int32 scale = 2;
* @return Whether the scale field is set.
*/
@java.lang.Override
public boolean hasScale() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int32 scale = 2;
* @return The scale.
*/
@java.lang.Override
public int getScale() {
return scale_;
}
/**
* optional int32 scale = 2;
* @param value The scale to set.
* @return This builder for chaining.
*/
public Builder setScale(int value) {
bitField0_ |= 0x00000002;
scale_ = value;
onChanged();
return this;
}
/**
* optional int32 scale = 2;
* @return This builder for chaining.
*/
public Builder clearScale() {
bitField0_ = (bitField0_ & ~0x00000002);
scale_ = 0;
onChanged();
return this;
}
private int length_ ;
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return Whether the length field is set.
*/
@java.lang.Override
public boolean hasLength() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return The length.
*/
@java.lang.Override
public int getLength() {
return length_;
}
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @param value The length to set.
* @return This builder for chaining.
*/
public Builder setLength(int value) {
bitField0_ |= 0x00000004;
length_ = value;
onChanged();
return this;
}
/**
*
* For varchar columns
*
*
* optional int32 length = 3;
* @return This builder for chaining.
*/
public Builder clearLength() {
bitField0_ = (bitField0_ & ~0x00000004);
length_ = 0;
onChanged();
return this;
}
private int specialColCode_ ;
/**
* optional int32 specialColCode = 4;
* @return Whether the specialColCode field is set.
*/
@java.lang.Override
public boolean hasSpecialColCode() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional int32 specialColCode = 4;
* @return The specialColCode.
*/
@java.lang.Override
public int getSpecialColCode() {
return specialColCode_;
}
/**
* optional int32 specialColCode = 4;
* @param value The specialColCode to set.
* @return This builder for chaining.
*/
public Builder setSpecialColCode(int value) {
bitField0_ |= 0x00000008;
specialColCode_ = value;
onChanged();
return this;
}
/**
* optional int32 specialColCode = 4;
* @return This builder for chaining.
*/
public Builder clearSpecialColCode() {
bitField0_ = (bitField0_ & ~0x00000008);
specialColCode_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnTypeAttributesPB)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnTypeAttributesPB)
private static final org.apache.kudu.KuduCommon.ColumnTypeAttributesPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnTypeAttributesPB();
}
public static org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnTypeAttributesPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnTypeAttributesPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ColumnSchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnSchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* optional uint32 id = 1;
* @return The id.
*/
int getId();
/**
* required string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* required string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* required string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* required .kudu.DataType type = 3;
* @return Whether the type field is set.
*/
boolean hasType();
/**
* required .kudu.DataType type = 3;
* @return The type.
*/
org.apache.kudu.KuduCommon.DataType getType();
/**
* optional bool is_key = 4 [default = false];
* @return Whether the isKey field is set.
*/
boolean hasIsKey();
/**
* optional bool is_key = 4 [default = false];
* @return The isKey.
*/
boolean getIsKey();
/**
* optional bool is_nullable = 5 [default = false];
* @return Whether the isNullable field is set.
*/
boolean hasIsNullable();
/**
* optional bool is_nullable = 5 [default = false];
* @return The isNullable.
*/
boolean getIsNullable();
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return Whether the readDefaultValue field is set.
*/
boolean hasReadDefaultValue();
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return The readDefaultValue.
*/
com.google.protobuf.ByteString getReadDefaultValue();
/**
* optional bytes write_default_value = 7;
* @return Whether the writeDefaultValue field is set.
*/
boolean hasWriteDefaultValue();
/**
* optional bytes write_default_value = 7;
* @return The writeDefaultValue.
*/
com.google.protobuf.ByteString getWriteDefaultValue();
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return Whether the encoding field is set.
*/
boolean hasEncoding();
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return The encoding.
*/
org.apache.kudu.KuduCommon.EncodingType getEncoding();
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return Whether the compression field is set.
*/
boolean hasCompression();
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return The compression.
*/
org.apache.kudu.Compression.CompressionType getCompression();
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return Whether the cfileBlockSize field is set.
*/
boolean hasCfileBlockSize();
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return The cfileBlockSize.
*/
int getCfileBlockSize();
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return Whether the typeAttributes field is set.
*/
boolean hasTypeAttributes();
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return The typeAttributes.
*/
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getTypeAttributes();
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder getTypeAttributesOrBuilder();
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return Whether the comment field is set.
*/
boolean hasComment();
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The comment.
*/
java.lang.String getComment();
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The bytes for comment.
*/
com.google.protobuf.ByteString
getCommentBytes();
}
/**
*
* TODO: Differentiate between the schema attributes
* that are only relevant to the server (e.g.,
* encoding and compression) and those that also
* matter to the client.
*
*
* Protobuf type {@code kudu.ColumnSchemaPB}
*/
public static final class ColumnSchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnSchemaPB)
ColumnSchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnSchemaPB.newBuilder() to construct.
private ColumnSchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnSchemaPB() {
name_ = "";
type_ = 999;
readDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
writeDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
encoding_ = 0;
compression_ = 0;
comment_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnSchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnSchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readUInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
name_ = bs;
break;
}
case 24: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.DataType value = org.apache.kudu.KuduCommon.DataType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(3, rawValue);
} else {
bitField0_ |= 0x00000004;
type_ = rawValue;
}
break;
}
case 32: {
bitField0_ |= 0x00000008;
isKey_ = input.readBool();
break;
}
case 40: {
bitField0_ |= 0x00000010;
isNullable_ = input.readBool();
break;
}
case 50: {
bitField0_ |= 0x00000020;
readDefaultValue_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
writeDefaultValue_ = input.readBytes();
break;
}
case 64: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType value = org.apache.kudu.KuduCommon.EncodingType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(8, rawValue);
} else {
bitField0_ |= 0x00000080;
encoding_ = rawValue;
}
break;
}
case 72: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType value = org.apache.kudu.Compression.CompressionType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(9, rawValue);
} else {
bitField0_ |= 0x00000100;
compression_ = rawValue;
}
break;
}
case 80: {
bitField0_ |= 0x00000200;
cfileBlockSize_ = input.readInt32();
break;
}
case 90: {
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) != 0)) {
subBuilder = typeAttributes_.toBuilder();
}
typeAttributes_ = input.readMessage(org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(typeAttributes_);
typeAttributes_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
comment_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnSchemaPB.class, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private int id_;
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
public static final int NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object name_;
/**
* required string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3;
private int type_;
/**
* required .kudu.DataType type = 3;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* required .kudu.DataType type = 3;
* @return The type.
*/
@java.lang.Override public org.apache.kudu.KuduCommon.DataType getType() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.DataType result = org.apache.kudu.KuduCommon.DataType.valueOf(type_);
return result == null ? org.apache.kudu.KuduCommon.DataType.UNKNOWN_DATA : result;
}
public static final int IS_KEY_FIELD_NUMBER = 4;
private boolean isKey_;
/**
* optional bool is_key = 4 [default = false];
* @return Whether the isKey field is set.
*/
@java.lang.Override
public boolean hasIsKey() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool is_key = 4 [default = false];
* @return The isKey.
*/
@java.lang.Override
public boolean getIsKey() {
return isKey_;
}
public static final int IS_NULLABLE_FIELD_NUMBER = 5;
private boolean isNullable_;
/**
* optional bool is_nullable = 5 [default = false];
* @return Whether the isNullable field is set.
*/
@java.lang.Override
public boolean hasIsNullable() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_nullable = 5 [default = false];
* @return The isNullable.
*/
@java.lang.Override
public boolean getIsNullable() {
return isNullable_;
}
public static final int READ_DEFAULT_VALUE_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString readDefaultValue_;
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return Whether the readDefaultValue field is set.
*/
@java.lang.Override
public boolean hasReadDefaultValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return The readDefaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReadDefaultValue() {
return readDefaultValue_;
}
public static final int WRITE_DEFAULT_VALUE_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString writeDefaultValue_;
/**
* optional bytes write_default_value = 7;
* @return Whether the writeDefaultValue field is set.
*/
@java.lang.Override
public boolean hasWriteDefaultValue() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional bytes write_default_value = 7;
* @return The writeDefaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getWriteDefaultValue() {
return writeDefaultValue_;
}
public static final int ENCODING_FIELD_NUMBER = 8;
private int encoding_;
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return Whether the encoding field is set.
*/
@java.lang.Override public boolean hasEncoding() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return The encoding.
*/
@java.lang.Override public org.apache.kudu.KuduCommon.EncodingType getEncoding() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType result = org.apache.kudu.KuduCommon.EncodingType.valueOf(encoding_);
return result == null ? org.apache.kudu.KuduCommon.EncodingType.AUTO_ENCODING : result;
}
public static final int COMPRESSION_FIELD_NUMBER = 9;
private int compression_;
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return Whether the compression field is set.
*/
@java.lang.Override public boolean hasCompression() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return The compression.
*/
@java.lang.Override public org.apache.kudu.Compression.CompressionType getCompression() {
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType result = org.apache.kudu.Compression.CompressionType.valueOf(compression_);
return result == null ? org.apache.kudu.Compression.CompressionType.DEFAULT_COMPRESSION : result;
}
public static final int CFILE_BLOCK_SIZE_FIELD_NUMBER = 10;
private int cfileBlockSize_;
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return Whether the cfileBlockSize field is set.
*/
@java.lang.Override
public boolean hasCfileBlockSize() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return The cfileBlockSize.
*/
@java.lang.Override
public int getCfileBlockSize() {
return cfileBlockSize_;
}
public static final int TYPE_ATTRIBUTES_FIELD_NUMBER = 11;
private org.apache.kudu.KuduCommon.ColumnTypeAttributesPB typeAttributes_;
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return Whether the typeAttributes field is set.
*/
@java.lang.Override
public boolean hasTypeAttributes() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return The typeAttributes.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getTypeAttributes() {
return typeAttributes_ == null ? org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance() : typeAttributes_;
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder getTypeAttributesOrBuilder() {
return typeAttributes_ == null ? org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance() : typeAttributes_;
}
public static final int COMMENT_FIELD_NUMBER = 12;
private volatile java.lang.Object comment_;
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return Whether the comment field is set.
*/
@java.lang.Override
public boolean hasComment() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The comment.
*/
@java.lang.Override
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
comment_ = s;
}
return s;
}
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The bytes for comment.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt32(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(3, type_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(4, isKey_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(5, isNullable_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBytes(6, readDefaultValue_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBytes(7, writeDefaultValue_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeEnum(8, encoding_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeEnum(9, compression_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeInt32(10, cfileBlockSize_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(11, getTypeAttributes());
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, comment_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, name_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, type_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, isKey_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isNullable_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, readDefaultValue_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, writeDefaultValue_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, encoding_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, compression_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, cfileBlockSize_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getTypeAttributes());
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, comment_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnSchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnSchemaPB other = (org.apache.kudu.KuduCommon.ColumnSchemaPB) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId()
!= other.getId()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasIsKey() != other.hasIsKey()) return false;
if (hasIsKey()) {
if (getIsKey()
!= other.getIsKey()) return false;
}
if (hasIsNullable() != other.hasIsNullable()) return false;
if (hasIsNullable()) {
if (getIsNullable()
!= other.getIsNullable()) return false;
}
if (hasReadDefaultValue() != other.hasReadDefaultValue()) return false;
if (hasReadDefaultValue()) {
if (!getReadDefaultValue()
.equals(other.getReadDefaultValue())) return false;
}
if (hasWriteDefaultValue() != other.hasWriteDefaultValue()) return false;
if (hasWriteDefaultValue()) {
if (!getWriteDefaultValue()
.equals(other.getWriteDefaultValue())) return false;
}
if (hasEncoding() != other.hasEncoding()) return false;
if (hasEncoding()) {
if (encoding_ != other.encoding_) return false;
}
if (hasCompression() != other.hasCompression()) return false;
if (hasCompression()) {
if (compression_ != other.compression_) return false;
}
if (hasCfileBlockSize() != other.hasCfileBlockSize()) return false;
if (hasCfileBlockSize()) {
if (getCfileBlockSize()
!= other.getCfileBlockSize()) return false;
}
if (hasTypeAttributes() != other.hasTypeAttributes()) return false;
if (hasTypeAttributes()) {
if (!getTypeAttributes()
.equals(other.getTypeAttributes())) return false;
}
if (hasComment() != other.hasComment()) return false;
if (hasComment()) {
if (!getComment()
.equals(other.getComment())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasIsKey()) {
hash = (37 * hash) + IS_KEY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsKey());
}
if (hasIsNullable()) {
hash = (37 * hash) + IS_NULLABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsNullable());
}
if (hasReadDefaultValue()) {
hash = (37 * hash) + READ_DEFAULT_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getReadDefaultValue().hashCode();
}
if (hasWriteDefaultValue()) {
hash = (37 * hash) + WRITE_DEFAULT_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getWriteDefaultValue().hashCode();
}
if (hasEncoding()) {
hash = (37 * hash) + ENCODING_FIELD_NUMBER;
hash = (53 * hash) + encoding_;
}
if (hasCompression()) {
hash = (37 * hash) + COMPRESSION_FIELD_NUMBER;
hash = (53 * hash) + compression_;
}
if (hasCfileBlockSize()) {
hash = (37 * hash) + CFILE_BLOCK_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getCfileBlockSize();
}
if (hasTypeAttributes()) {
hash = (37 * hash) + TYPE_ATTRIBUTES_FIELD_NUMBER;
hash = (53 * hash) + getTypeAttributes().hashCode();
}
if (hasComment()) {
hash = (37 * hash) + COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getComment().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnSchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* TODO: Differentiate between the schema attributes
* that are only relevant to the server (e.g.,
* encoding and compression) and those that also
* matter to the client.
*
*
* Protobuf type {@code kudu.ColumnSchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnSchemaPB)
org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnSchemaPB.class, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnSchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTypeAttributesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
type_ = 999;
bitField0_ = (bitField0_ & ~0x00000004);
isKey_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
isNullable_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
readDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
writeDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
encoding_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
compression_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
cfileBlockSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
if (typeAttributesBuilder_ == null) {
typeAttributes_ = null;
} else {
typeAttributesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
comment_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnSchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPB build() {
org.apache.kudu.KuduCommon.ColumnSchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPB buildPartial() {
org.apache.kudu.KuduCommon.ColumnSchemaPB result = new org.apache.kudu.KuduCommon.ColumnSchemaPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.isKey_ = isKey_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.isNullable_ = isNullable_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.readDefaultValue_ = readDefaultValue_;
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000040;
}
result.writeDefaultValue_ = writeDefaultValue_;
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.encoding_ = encoding_;
if (((from_bitField0_ & 0x00000100) != 0)) {
to_bitField0_ |= 0x00000100;
}
result.compression_ = compression_;
if (((from_bitField0_ & 0x00000200) != 0)) {
result.cfileBlockSize_ = cfileBlockSize_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
if (typeAttributesBuilder_ == null) {
result.typeAttributes_ = typeAttributes_;
} else {
result.typeAttributes_ = typeAttributesBuilder_.build();
}
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
to_bitField0_ |= 0x00000800;
}
result.comment_ = comment_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnSchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnSchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnSchemaPB other) {
if (other == org.apache.kudu.KuduCommon.ColumnSchemaPB.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasName()) {
bitField0_ |= 0x00000002;
name_ = other.name_;
onChanged();
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasIsKey()) {
setIsKey(other.getIsKey());
}
if (other.hasIsNullable()) {
setIsNullable(other.getIsNullable());
}
if (other.hasReadDefaultValue()) {
setReadDefaultValue(other.getReadDefaultValue());
}
if (other.hasWriteDefaultValue()) {
setWriteDefaultValue(other.getWriteDefaultValue());
}
if (other.hasEncoding()) {
setEncoding(other.getEncoding());
}
if (other.hasCompression()) {
setCompression(other.getCompression());
}
if (other.hasCfileBlockSize()) {
setCfileBlockSize(other.getCfileBlockSize());
}
if (other.hasTypeAttributes()) {
mergeTypeAttributes(other.getTypeAttributes());
}
if (other.hasComment()) {
bitField0_ |= 0x00000800;
comment_ = other.comment_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasType()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnSchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnSchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int id_ ;
/**
* optional uint32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional uint32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
return id_;
}
/**
* optional uint32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional uint32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* required string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
/**
* required string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* required string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
name_ = value;
onChanged();
return this;
}
private int type_ = 999;
/**
* required .kudu.DataType type = 3;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* required .kudu.DataType type = 3;
* @return The type.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.DataType getType() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.DataType result = org.apache.kudu.KuduCommon.DataType.valueOf(type_);
return result == null ? org.apache.kudu.KuduCommon.DataType.UNKNOWN_DATA : result;
}
/**
* required .kudu.DataType type = 3;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.apache.kudu.KuduCommon.DataType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* required .kudu.DataType type = 3;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = 999;
onChanged();
return this;
}
private boolean isKey_ ;
/**
* optional bool is_key = 4 [default = false];
* @return Whether the isKey field is set.
*/
@java.lang.Override
public boolean hasIsKey() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool is_key = 4 [default = false];
* @return The isKey.
*/
@java.lang.Override
public boolean getIsKey() {
return isKey_;
}
/**
* optional bool is_key = 4 [default = false];
* @param value The isKey to set.
* @return This builder for chaining.
*/
public Builder setIsKey(boolean value) {
bitField0_ |= 0x00000008;
isKey_ = value;
onChanged();
return this;
}
/**
* optional bool is_key = 4 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsKey() {
bitField0_ = (bitField0_ & ~0x00000008);
isKey_ = false;
onChanged();
return this;
}
private boolean isNullable_ ;
/**
* optional bool is_nullable = 5 [default = false];
* @return Whether the isNullable field is set.
*/
@java.lang.Override
public boolean hasIsNullable() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_nullable = 5 [default = false];
* @return The isNullable.
*/
@java.lang.Override
public boolean getIsNullable() {
return isNullable_;
}
/**
* optional bool is_nullable = 5 [default = false];
* @param value The isNullable to set.
* @return This builder for chaining.
*/
public Builder setIsNullable(boolean value) {
bitField0_ |= 0x00000010;
isNullable_ = value;
onChanged();
return this;
}
/**
* optional bool is_nullable = 5 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsNullable() {
bitField0_ = (bitField0_ & ~0x00000010);
isNullable_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString readDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return Whether the readDefaultValue field is set.
*/
@java.lang.Override
public boolean hasReadDefaultValue() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return The readDefaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReadDefaultValue() {
return readDefaultValue_;
}
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @param value The readDefaultValue to set.
* @return This builder for chaining.
*/
public Builder setReadDefaultValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
readDefaultValue_ = value;
onChanged();
return this;
}
/**
*
* Default values.
* NOTE: as far as clients are concerned, there is only one
* "default value" of a column. The read/write defaults are used
* internally and should not be exposed by any public client APIs.
* When passing schemas to the master for create/alter table,
* specify the default in 'read_default_value'.
* Contrary to this, when the client opens a table, it will receive
* both the read and write defaults, but the *write* default is
* what should be exposed as the "current" default.
*
*
* optional bytes read_default_value = 6;
* @return This builder for chaining.
*/
public Builder clearReadDefaultValue() {
bitField0_ = (bitField0_ & ~0x00000020);
readDefaultValue_ = getDefaultInstance().getReadDefaultValue();
onChanged();
return this;
}
private com.google.protobuf.ByteString writeDefaultValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes write_default_value = 7;
* @return Whether the writeDefaultValue field is set.
*/
@java.lang.Override
public boolean hasWriteDefaultValue() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional bytes write_default_value = 7;
* @return The writeDefaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getWriteDefaultValue() {
return writeDefaultValue_;
}
/**
* optional bytes write_default_value = 7;
* @param value The writeDefaultValue to set.
* @return This builder for chaining.
*/
public Builder setWriteDefaultValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
writeDefaultValue_ = value;
onChanged();
return this;
}
/**
* optional bytes write_default_value = 7;
* @return This builder for chaining.
*/
public Builder clearWriteDefaultValue() {
bitField0_ = (bitField0_ & ~0x00000040);
writeDefaultValue_ = getDefaultInstance().getWriteDefaultValue();
onChanged();
return this;
}
private int encoding_ = 0;
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return Whether the encoding field is set.
*/
@java.lang.Override public boolean hasEncoding() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return The encoding.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.EncodingType getEncoding() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType result = org.apache.kudu.KuduCommon.EncodingType.valueOf(encoding_);
return result == null ? org.apache.kudu.KuduCommon.EncodingType.AUTO_ENCODING : result;
}
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @param value The encoding to set.
* @return This builder for chaining.
*/
public Builder setEncoding(org.apache.kudu.KuduCommon.EncodingType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
encoding_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The following attributes refer to the on-disk storage of the column.
* They won't always be set, depending on context.
*
*
* optional .kudu.EncodingType encoding = 8 [default = AUTO_ENCODING];
* @return This builder for chaining.
*/
public Builder clearEncoding() {
bitField0_ = (bitField0_ & ~0x00000080);
encoding_ = 0;
onChanged();
return this;
}
private int compression_ = 0;
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return Whether the compression field is set.
*/
@java.lang.Override public boolean hasCompression() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return The compression.
*/
@java.lang.Override
public org.apache.kudu.Compression.CompressionType getCompression() {
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType result = org.apache.kudu.Compression.CompressionType.valueOf(compression_);
return result == null ? org.apache.kudu.Compression.CompressionType.DEFAULT_COMPRESSION : result;
}
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @param value The compression to set.
* @return This builder for chaining.
*/
public Builder setCompression(org.apache.kudu.Compression.CompressionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
compression_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .kudu.CompressionType compression = 9 [default = DEFAULT_COMPRESSION];
* @return This builder for chaining.
*/
public Builder clearCompression() {
bitField0_ = (bitField0_ & ~0x00000100);
compression_ = 0;
onChanged();
return this;
}
private int cfileBlockSize_ ;
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return Whether the cfileBlockSize field is set.
*/
@java.lang.Override
public boolean hasCfileBlockSize() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return The cfileBlockSize.
*/
@java.lang.Override
public int getCfileBlockSize() {
return cfileBlockSize_;
}
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @param value The cfileBlockSize to set.
* @return This builder for chaining.
*/
public Builder setCfileBlockSize(int value) {
bitField0_ |= 0x00000200;
cfileBlockSize_ = value;
onChanged();
return this;
}
/**
* optional int32 cfile_block_size = 10 [default = 0];
* @return This builder for chaining.
*/
public Builder clearCfileBlockSize() {
bitField0_ = (bitField0_ & ~0x00000200);
cfileBlockSize_ = 0;
onChanged();
return this;
}
private org.apache.kudu.KuduCommon.ColumnTypeAttributesPB typeAttributes_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB, org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder, org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder> typeAttributesBuilder_;
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return Whether the typeAttributes field is set.
*/
public boolean hasTypeAttributes() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
* @return The typeAttributes.
*/
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB getTypeAttributes() {
if (typeAttributesBuilder_ == null) {
return typeAttributes_ == null ? org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance() : typeAttributes_;
} else {
return typeAttributesBuilder_.getMessage();
}
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public Builder setTypeAttributes(org.apache.kudu.KuduCommon.ColumnTypeAttributesPB value) {
if (typeAttributesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
typeAttributes_ = value;
onChanged();
} else {
typeAttributesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public Builder setTypeAttributes(
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder builderForValue) {
if (typeAttributesBuilder_ == null) {
typeAttributes_ = builderForValue.build();
onChanged();
} else {
typeAttributesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public Builder mergeTypeAttributes(org.apache.kudu.KuduCommon.ColumnTypeAttributesPB value) {
if (typeAttributesBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0) &&
typeAttributes_ != null &&
typeAttributes_ != org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance()) {
typeAttributes_ =
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.newBuilder(typeAttributes_).mergeFrom(value).buildPartial();
} else {
typeAttributes_ = value;
}
onChanged();
} else {
typeAttributesBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public Builder clearTypeAttributes() {
if (typeAttributesBuilder_ == null) {
typeAttributes_ = null;
onChanged();
} else {
typeAttributesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder getTypeAttributesBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getTypeAttributesFieldBuilder().getBuilder();
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
public org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder getTypeAttributesOrBuilder() {
if (typeAttributesBuilder_ != null) {
return typeAttributesBuilder_.getMessageOrBuilder();
} else {
return typeAttributes_ == null ?
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.getDefaultInstance() : typeAttributes_;
}
}
/**
* optional .kudu.ColumnTypeAttributesPB type_attributes = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB, org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder, org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder>
getTypeAttributesFieldBuilder() {
if (typeAttributesBuilder_ == null) {
typeAttributesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnTypeAttributesPB, org.apache.kudu.KuduCommon.ColumnTypeAttributesPB.Builder, org.apache.kudu.KuduCommon.ColumnTypeAttributesPBOrBuilder>(
getTypeAttributes(),
getParentForChildren(),
isClean());
typeAttributes_ = null;
}
return typeAttributesBuilder_;
}
private java.lang.Object comment_ = "";
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return Whether the comment field is set.
*/
public boolean hasComment() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The comment.
*/
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
comment_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return The bytes for comment.
*/
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @param value The comment to set.
* @return This builder for chaining.
*/
public Builder setComment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
comment_ = value;
onChanged();
return this;
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @return This builder for chaining.
*/
public Builder clearComment() {
bitField0_ = (bitField0_ & ~0x00000800);
comment_ = getDefaultInstance().getComment();
onChanged();
return this;
}
/**
*
* The comment for the column.
*
*
* optional string comment = 12;
* @param value The bytes for comment to set.
* @return This builder for chaining.
*/
public Builder setCommentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
comment_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnSchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnSchemaPB)
private static final org.apache.kudu.KuduCommon.ColumnSchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnSchemaPB();
}
public static org.apache.kudu.KuduCommon.ColumnSchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnSchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnSchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ColumnSchemaDeltaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnSchemaDeltaPB)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* optional string new_name = 2;
* @return Whether the newName field is set.
*/
boolean hasNewName();
/**
* optional string new_name = 2;
* @return The newName.
*/
java.lang.String getNewName();
/**
* optional string new_name = 2;
* @return The bytes for newName.
*/
com.google.protobuf.ByteString
getNewNameBytes();
/**
* optional bytes default_value = 4;
* @return Whether the defaultValue field is set.
*/
boolean hasDefaultValue();
/**
* optional bytes default_value = 4;
* @return The defaultValue.
*/
com.google.protobuf.ByteString getDefaultValue();
/**
* optional bool remove_default = 5;
* @return Whether the removeDefault field is set.
*/
boolean hasRemoveDefault();
/**
* optional bool remove_default = 5;
* @return The removeDefault.
*/
boolean getRemoveDefault();
/**
* optional .kudu.EncodingType encoding = 6;
* @return Whether the encoding field is set.
*/
boolean hasEncoding();
/**
* optional .kudu.EncodingType encoding = 6;
* @return The encoding.
*/
org.apache.kudu.KuduCommon.EncodingType getEncoding();
/**
* optional .kudu.CompressionType compression = 7;
* @return Whether the compression field is set.
*/
boolean hasCompression();
/**
* optional .kudu.CompressionType compression = 7;
* @return The compression.
*/
org.apache.kudu.Compression.CompressionType getCompression();
/**
* optional int32 block_size = 8;
* @return Whether the blockSize field is set.
*/
boolean hasBlockSize();
/**
* optional int32 block_size = 8;
* @return The blockSize.
*/
int getBlockSize();
/**
* optional string new_comment = 9;
* @return Whether the newComment field is set.
*/
boolean hasNewComment();
/**
* optional string new_comment = 9;
* @return The newComment.
*/
java.lang.String getNewComment();
/**
* optional string new_comment = 9;
* @return The bytes for newComment.
*/
com.google.protobuf.ByteString
getNewCommentBytes();
}
/**
* Protobuf type {@code kudu.ColumnSchemaDeltaPB}
*/
public static final class ColumnSchemaDeltaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnSchemaDeltaPB)
ColumnSchemaDeltaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnSchemaDeltaPB.newBuilder() to construct.
private ColumnSchemaDeltaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnSchemaDeltaPB() {
name_ = "";
newName_ = "";
defaultValue_ = com.google.protobuf.ByteString.EMPTY;
encoding_ = 999;
compression_ = 999;
newComment_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnSchemaDeltaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnSchemaDeltaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
name_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
newName_ = bs;
break;
}
case 34: {
bitField0_ |= 0x00000004;
defaultValue_ = input.readBytes();
break;
}
case 40: {
bitField0_ |= 0x00000008;
removeDefault_ = input.readBool();
break;
}
case 48: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType value = org.apache.kudu.KuduCommon.EncodingType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(6, rawValue);
} else {
bitField0_ |= 0x00000010;
encoding_ = rawValue;
}
break;
}
case 56: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType value = org.apache.kudu.Compression.CompressionType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(7, rawValue);
} else {
bitField0_ |= 0x00000020;
compression_ = rawValue;
}
break;
}
case 64: {
bitField0_ |= 0x00000040;
blockSize_ = input.readInt32();
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
newComment_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaDeltaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaDeltaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.class, org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NEW_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object newName_;
/**
* optional string new_name = 2;
* @return Whether the newName field is set.
*/
@java.lang.Override
public boolean hasNewName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string new_name = 2;
* @return The newName.
*/
@java.lang.Override
public java.lang.String getNewName() {
java.lang.Object ref = newName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
newName_ = s;
}
return s;
}
}
/**
* optional string new_name = 2;
* @return The bytes for newName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNewNameBytes() {
java.lang.Object ref = newName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEFAULT_VALUE_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString defaultValue_;
/**
* optional bytes default_value = 4;
* @return Whether the defaultValue field is set.
*/
@java.lang.Override
public boolean hasDefaultValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes default_value = 4;
* @return The defaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDefaultValue() {
return defaultValue_;
}
public static final int REMOVE_DEFAULT_FIELD_NUMBER = 5;
private boolean removeDefault_;
/**
* optional bool remove_default = 5;
* @return Whether the removeDefault field is set.
*/
@java.lang.Override
public boolean hasRemoveDefault() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool remove_default = 5;
* @return The removeDefault.
*/
@java.lang.Override
public boolean getRemoveDefault() {
return removeDefault_;
}
public static final int ENCODING_FIELD_NUMBER = 6;
private int encoding_;
/**
* optional .kudu.EncodingType encoding = 6;
* @return Whether the encoding field is set.
*/
@java.lang.Override public boolean hasEncoding() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .kudu.EncodingType encoding = 6;
* @return The encoding.
*/
@java.lang.Override public org.apache.kudu.KuduCommon.EncodingType getEncoding() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType result = org.apache.kudu.KuduCommon.EncodingType.valueOf(encoding_);
return result == null ? org.apache.kudu.KuduCommon.EncodingType.UNKNOWN_ENCODING : result;
}
public static final int COMPRESSION_FIELD_NUMBER = 7;
private int compression_;
/**
* optional .kudu.CompressionType compression = 7;
* @return Whether the compression field is set.
*/
@java.lang.Override public boolean hasCompression() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .kudu.CompressionType compression = 7;
* @return The compression.
*/
@java.lang.Override public org.apache.kudu.Compression.CompressionType getCompression() {
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType result = org.apache.kudu.Compression.CompressionType.valueOf(compression_);
return result == null ? org.apache.kudu.Compression.CompressionType.UNKNOWN_COMPRESSION : result;
}
public static final int BLOCK_SIZE_FIELD_NUMBER = 8;
private int blockSize_;
/**
* optional int32 block_size = 8;
* @return Whether the blockSize field is set.
*/
@java.lang.Override
public boolean hasBlockSize() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional int32 block_size = 8;
* @return The blockSize.
*/
@java.lang.Override
public int getBlockSize() {
return blockSize_;
}
public static final int NEW_COMMENT_FIELD_NUMBER = 9;
private volatile java.lang.Object newComment_;
/**
* optional string new_comment = 9;
* @return Whether the newComment field is set.
*/
@java.lang.Override
public boolean hasNewComment() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional string new_comment = 9;
* @return The newComment.
*/
@java.lang.Override
public java.lang.String getNewComment() {
java.lang.Object ref = newComment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
newComment_ = s;
}
return s;
}
}
/**
* optional string new_comment = 9;
* @return The bytes for newComment.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNewCommentBytes() {
java.lang.Object ref = newComment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newComment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, newName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(4, defaultValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(5, removeDefault_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(6, encoding_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeEnum(7, compression_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(8, blockSize_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, newComment_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, newName_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, defaultValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, removeDefault_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, encoding_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, compression_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, blockSize_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, newComment_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB other = (org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasNewName() != other.hasNewName()) return false;
if (hasNewName()) {
if (!getNewName()
.equals(other.getNewName())) return false;
}
if (hasDefaultValue() != other.hasDefaultValue()) return false;
if (hasDefaultValue()) {
if (!getDefaultValue()
.equals(other.getDefaultValue())) return false;
}
if (hasRemoveDefault() != other.hasRemoveDefault()) return false;
if (hasRemoveDefault()) {
if (getRemoveDefault()
!= other.getRemoveDefault()) return false;
}
if (hasEncoding() != other.hasEncoding()) return false;
if (hasEncoding()) {
if (encoding_ != other.encoding_) return false;
}
if (hasCompression() != other.hasCompression()) return false;
if (hasCompression()) {
if (compression_ != other.compression_) return false;
}
if (hasBlockSize() != other.hasBlockSize()) return false;
if (hasBlockSize()) {
if (getBlockSize()
!= other.getBlockSize()) return false;
}
if (hasNewComment() != other.hasNewComment()) return false;
if (hasNewComment()) {
if (!getNewComment()
.equals(other.getNewComment())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasNewName()) {
hash = (37 * hash) + NEW_NAME_FIELD_NUMBER;
hash = (53 * hash) + getNewName().hashCode();
}
if (hasDefaultValue()) {
hash = (37 * hash) + DEFAULT_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getDefaultValue().hashCode();
}
if (hasRemoveDefault()) {
hash = (37 * hash) + REMOVE_DEFAULT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRemoveDefault());
}
if (hasEncoding()) {
hash = (37 * hash) + ENCODING_FIELD_NUMBER;
hash = (53 * hash) + encoding_;
}
if (hasCompression()) {
hash = (37 * hash) + COMPRESSION_FIELD_NUMBER;
hash = (53 * hash) + compression_;
}
if (hasBlockSize()) {
hash = (37 * hash) + BLOCK_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getBlockSize();
}
if (hasNewComment()) {
hash = (37 * hash) + NEW_COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getNewComment().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnSchemaDeltaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnSchemaDeltaPB)
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaDeltaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaDeltaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.class, org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
newName_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
defaultValue_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
removeDefault_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
encoding_ = 999;
bitField0_ = (bitField0_ & ~0x00000010);
compression_ = 999;
bitField0_ = (bitField0_ & ~0x00000020);
blockSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000040);
newComment_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnSchemaDeltaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB build() {
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB buildPartial() {
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB result = new org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.newName_ = newName_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.defaultValue_ = defaultValue_;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.removeDefault_ = removeDefault_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.encoding_ = encoding_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.compression_ = compression_;
if (((from_bitField0_ & 0x00000040) != 0)) {
result.blockSize_ = blockSize_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
to_bitField0_ |= 0x00000080;
}
result.newComment_ = newComment_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB other) {
if (other == org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasNewName()) {
bitField0_ |= 0x00000002;
newName_ = other.newName_;
onChanged();
}
if (other.hasDefaultValue()) {
setDefaultValue(other.getDefaultValue());
}
if (other.hasRemoveDefault()) {
setRemoveDefault(other.getRemoveDefault());
}
if (other.hasEncoding()) {
setEncoding(other.getEncoding());
}
if (other.hasCompression()) {
setCompression(other.getCompression());
}
if (other.hasBlockSize()) {
setBlockSize(other.getBlockSize());
}
if (other.hasNewComment()) {
bitField0_ |= 0x00000080;
newComment_ = other.newComment_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
private java.lang.Object newName_ = "";
/**
* optional string new_name = 2;
* @return Whether the newName field is set.
*/
public boolean hasNewName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string new_name = 2;
* @return The newName.
*/
public java.lang.String getNewName() {
java.lang.Object ref = newName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
newName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string new_name = 2;
* @return The bytes for newName.
*/
public com.google.protobuf.ByteString
getNewNameBytes() {
java.lang.Object ref = newName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string new_name = 2;
* @param value The newName to set.
* @return This builder for chaining.
*/
public Builder setNewName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
newName_ = value;
onChanged();
return this;
}
/**
* optional string new_name = 2;
* @return This builder for chaining.
*/
public Builder clearNewName() {
bitField0_ = (bitField0_ & ~0x00000002);
newName_ = getDefaultInstance().getNewName();
onChanged();
return this;
}
/**
* optional string new_name = 2;
* @param value The bytes for newName to set.
* @return This builder for chaining.
*/
public Builder setNewNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
newName_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString defaultValue_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes default_value = 4;
* @return Whether the defaultValue field is set.
*/
@java.lang.Override
public boolean hasDefaultValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes default_value = 4;
* @return The defaultValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDefaultValue() {
return defaultValue_;
}
/**
* optional bytes default_value = 4;
* @param value The defaultValue to set.
* @return This builder for chaining.
*/
public Builder setDefaultValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
defaultValue_ = value;
onChanged();
return this;
}
/**
* optional bytes default_value = 4;
* @return This builder for chaining.
*/
public Builder clearDefaultValue() {
bitField0_ = (bitField0_ & ~0x00000004);
defaultValue_ = getDefaultInstance().getDefaultValue();
onChanged();
return this;
}
private boolean removeDefault_ ;
/**
* optional bool remove_default = 5;
* @return Whether the removeDefault field is set.
*/
@java.lang.Override
public boolean hasRemoveDefault() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional bool remove_default = 5;
* @return The removeDefault.
*/
@java.lang.Override
public boolean getRemoveDefault() {
return removeDefault_;
}
/**
* optional bool remove_default = 5;
* @param value The removeDefault to set.
* @return This builder for chaining.
*/
public Builder setRemoveDefault(boolean value) {
bitField0_ |= 0x00000008;
removeDefault_ = value;
onChanged();
return this;
}
/**
* optional bool remove_default = 5;
* @return This builder for chaining.
*/
public Builder clearRemoveDefault() {
bitField0_ = (bitField0_ & ~0x00000008);
removeDefault_ = false;
onChanged();
return this;
}
private int encoding_ = 999;
/**
* optional .kudu.EncodingType encoding = 6;
* @return Whether the encoding field is set.
*/
@java.lang.Override public boolean hasEncoding() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .kudu.EncodingType encoding = 6;
* @return The encoding.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.EncodingType getEncoding() {
@SuppressWarnings("deprecation")
org.apache.kudu.KuduCommon.EncodingType result = org.apache.kudu.KuduCommon.EncodingType.valueOf(encoding_);
return result == null ? org.apache.kudu.KuduCommon.EncodingType.UNKNOWN_ENCODING : result;
}
/**
* optional .kudu.EncodingType encoding = 6;
* @param value The encoding to set.
* @return This builder for chaining.
*/
public Builder setEncoding(org.apache.kudu.KuduCommon.EncodingType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
encoding_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .kudu.EncodingType encoding = 6;
* @return This builder for chaining.
*/
public Builder clearEncoding() {
bitField0_ = (bitField0_ & ~0x00000010);
encoding_ = 999;
onChanged();
return this;
}
private int compression_ = 999;
/**
* optional .kudu.CompressionType compression = 7;
* @return Whether the compression field is set.
*/
@java.lang.Override public boolean hasCompression() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .kudu.CompressionType compression = 7;
* @return The compression.
*/
@java.lang.Override
public org.apache.kudu.Compression.CompressionType getCompression() {
@SuppressWarnings("deprecation")
org.apache.kudu.Compression.CompressionType result = org.apache.kudu.Compression.CompressionType.valueOf(compression_);
return result == null ? org.apache.kudu.Compression.CompressionType.UNKNOWN_COMPRESSION : result;
}
/**
* optional .kudu.CompressionType compression = 7;
* @param value The compression to set.
* @return This builder for chaining.
*/
public Builder setCompression(org.apache.kudu.Compression.CompressionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
compression_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .kudu.CompressionType compression = 7;
* @return This builder for chaining.
*/
public Builder clearCompression() {
bitField0_ = (bitField0_ & ~0x00000020);
compression_ = 999;
onChanged();
return this;
}
private int blockSize_ ;
/**
* optional int32 block_size = 8;
* @return Whether the blockSize field is set.
*/
@java.lang.Override
public boolean hasBlockSize() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional int32 block_size = 8;
* @return The blockSize.
*/
@java.lang.Override
public int getBlockSize() {
return blockSize_;
}
/**
* optional int32 block_size = 8;
* @param value The blockSize to set.
* @return This builder for chaining.
*/
public Builder setBlockSize(int value) {
bitField0_ |= 0x00000040;
blockSize_ = value;
onChanged();
return this;
}
/**
* optional int32 block_size = 8;
* @return This builder for chaining.
*/
public Builder clearBlockSize() {
bitField0_ = (bitField0_ & ~0x00000040);
blockSize_ = 0;
onChanged();
return this;
}
private java.lang.Object newComment_ = "";
/**
* optional string new_comment = 9;
* @return Whether the newComment field is set.
*/
public boolean hasNewComment() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional string new_comment = 9;
* @return The newComment.
*/
public java.lang.String getNewComment() {
java.lang.Object ref = newComment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
newComment_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string new_comment = 9;
* @return The bytes for newComment.
*/
public com.google.protobuf.ByteString
getNewCommentBytes() {
java.lang.Object ref = newComment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
newComment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string new_comment = 9;
* @param value The newComment to set.
* @return This builder for chaining.
*/
public Builder setNewComment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
newComment_ = value;
onChanged();
return this;
}
/**
* optional string new_comment = 9;
* @return This builder for chaining.
*/
public Builder clearNewComment() {
bitField0_ = (bitField0_ & ~0x00000080);
newComment_ = getDefaultInstance().getNewComment();
onChanged();
return this;
}
/**
* optional string new_comment = 9;
* @param value The bytes for newComment to set.
* @return This builder for chaining.
*/
public Builder setNewCommentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
newComment_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnSchemaDeltaPB)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnSchemaDeltaPB)
private static final org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB();
}
public static org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnSchemaDeltaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnSchemaDeltaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaDeltaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.SchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
java.util.List
getColumnsList();
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
org.apache.kudu.KuduCommon.ColumnSchemaPB getColumns(int index);
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
int getColumnsCount();
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
java.util.List extends org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder>
getColumnsOrBuilderList();
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder getColumnsOrBuilder(
int index);
}
/**
* Protobuf type {@code kudu.SchemaPB}
*/
public static final class SchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.SchemaPB)
SchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use SchemaPB.newBuilder() to construct.
private SchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SchemaPB() {
columns_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(
input.readMessage(org.apache.kudu.KuduCommon.ColumnSchemaPB.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_SchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_SchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.SchemaPB.class, org.apache.kudu.KuduCommon.SchemaPB.Builder.class);
}
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
@java.lang.Override
public java.util.List getColumnsList() {
return columns_;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
@java.lang.Override
public int getColumnsCount() {
return columns_.size();
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPB getColumns(int index) {
return columns_.get(index);
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.SchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.SchemaPB other = (org.apache.kudu.KuduCommon.SchemaPB) obj;
if (!getColumnsList()
.equals(other.getColumnsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.SchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.SchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.SchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.SchemaPB)
org.apache.kudu.KuduCommon.SchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_SchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_SchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.SchemaPB.class, org.apache.kudu.KuduCommon.SchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.SchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_SchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB build() {
org.apache.kudu.KuduCommon.SchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB buildPartial() {
org.apache.kudu.KuduCommon.SchemaPB result = new org.apache.kudu.KuduCommon.SchemaPB(this);
int from_bitField0_ = bitField0_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.SchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.SchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.SchemaPB other) {
if (other == org.apache.kudu.KuduCommon.SchemaPB.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getColumnsCount(); i++) {
if (!getColumns(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.SchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.SchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnSchemaPB, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder, org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder> columnsBuilder_;
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public org.apache.kudu.KuduCommon.ColumnSchemaPB getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.ColumnSchemaPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder addColumns(org.apache.kudu.KuduCommon.ColumnSchemaPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.ColumnSchemaPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder addColumns(
org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.apache.kudu.KuduCommon.ColumnSchemaPB> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public java.util.List extends org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.ColumnSchemaPB.getDefaultInstance());
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.ColumnSchemaPB.getDefaultInstance());
}
/**
* repeated .kudu.ColumnSchemaPB columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnSchemaPB, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder, org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnSchemaPB, org.apache.kudu.KuduCommon.ColumnSchemaPB.Builder, org.apache.kudu.KuduCommon.ColumnSchemaPBOrBuilder>(
columns_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.SchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.SchemaPB)
private static final org.apache.kudu.KuduCommon.SchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.SchemaPB();
}
public static org.apache.kudu.KuduCommon.SchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.SchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HostPortPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.HostPortPB)
com.google.protobuf.MessageOrBuilder {
/**
* required string host = 1;
* @return Whether the host field is set.
*/
boolean hasHost();
/**
* required string host = 1;
* @return The host.
*/
java.lang.String getHost();
/**
* required string host = 1;
* @return The bytes for host.
*/
com.google.protobuf.ByteString
getHostBytes();
/**
* required uint32 port = 2;
* @return Whether the port field is set.
*/
boolean hasPort();
/**
* required uint32 port = 2;
* @return The port.
*/
int getPort();
}
/**
* Protobuf type {@code kudu.HostPortPB}
*/
public static final class HostPortPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.HostPortPB)
HostPortPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use HostPortPB.newBuilder() to construct.
private HostPortPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HostPortPB() {
host_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HostPortPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HostPortPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
host_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
port_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_HostPortPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_HostPortPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.HostPortPB.class, org.apache.kudu.KuduCommon.HostPortPB.Builder.class);
}
private int bitField0_;
public static final int HOST_FIELD_NUMBER = 1;
private volatile java.lang.Object host_;
/**
* required string host = 1;
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string host = 1;
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
}
}
/**
* required string host = 1;
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* required uint32 port = 2;
* @return Whether the port field is set.
*/
@java.lang.Override
public boolean hasPort() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required uint32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasHost()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt32(2, port_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, port_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.HostPortPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.HostPortPB other = (org.apache.kudu.KuduCommon.HostPortPB) obj;
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost()
.equals(other.getHost())) return false;
}
if (hasPort() != other.hasPort()) return false;
if (hasPort()) {
if (getPort()
!= other.getPort()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.HostPortPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.HostPortPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.HostPortPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.HostPortPB)
org.apache.kudu.KuduCommon.HostPortPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_HostPortPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_HostPortPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.HostPortPB.class, org.apache.kudu.KuduCommon.HostPortPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.HostPortPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
host_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_HostPortPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.HostPortPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.HostPortPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.HostPortPB build() {
org.apache.kudu.KuduCommon.HostPortPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.HostPortPB buildPartial() {
org.apache.kudu.KuduCommon.HostPortPB result = new org.apache.kudu.KuduCommon.HostPortPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.host_ = host_;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.port_ = port_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.HostPortPB) {
return mergeFrom((org.apache.kudu.KuduCommon.HostPortPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.HostPortPB other) {
if (other == org.apache.kudu.KuduCommon.HostPortPB.getDefaultInstance()) return this;
if (other.hasHost()) {
bitField0_ |= 0x00000001;
host_ = other.host_;
onChanged();
}
if (other.hasPort()) {
setPort(other.getPort());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasHost()) {
return false;
}
if (!hasPort()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.HostPortPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.HostPortPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object host_ = "";
/**
* required string host = 1;
* @return Whether the host field is set.
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string host = 1;
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string host = 1;
* @return The bytes for host.
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string host = 1;
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
host_ = value;
onChanged();
return this;
}
/**
* required string host = 1;
* @return This builder for chaining.
*/
public Builder clearHost() {
bitField0_ = (bitField0_ & ~0x00000001);
host_ = getDefaultInstance().getHost();
onChanged();
return this;
}
/**
* required string host = 1;
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
host_ = value;
onChanged();
return this;
}
private int port_ ;
/**
* required uint32 port = 2;
* @return Whether the port field is set.
*/
@java.lang.Override
public boolean hasPort() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required uint32 port = 2;
* @return The port.
*/
@java.lang.Override
public int getPort() {
return port_;
}
/**
* required uint32 port = 2;
* @param value The port to set.
* @return This builder for chaining.
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
* required uint32 port = 2;
* @return This builder for chaining.
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.HostPortPB)
}
// @@protoc_insertion_point(class_scope:kudu.HostPortPB)
private static final org.apache.kudu.KuduCommon.HostPortPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.HostPortPB();
}
public static org.apache.kudu.KuduCommon.HostPortPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HostPortPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HostPortPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.HostPortPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PartitionSchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionSchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
java.util.List
getHashSchemaList();
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index);
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
int getHashSchemaCount();
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList();
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index);
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return Whether the rangeSchema field is set.
*/
boolean hasRangeSchema();
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return The rangeSchema.
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getRangeSchema();
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder getRangeSchemaOrBuilder();
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
java.util.List
getCustomHashSchemaRangesList();
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getCustomHashSchemaRanges(int index);
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
int getCustomHashSchemaRangesCount();
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder>
getCustomHashSchemaRangesOrBuilderList();
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder getCustomHashSchemaRangesOrBuilder(
int index);
}
/**
*
* The serialized format of a Kudu table partition schema.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB}
*/
public static final class PartitionSchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionSchemaPB)
PartitionSchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use PartitionSchemaPB.newBuilder() to construct.
private PartitionSchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PartitionSchemaPB() {
hashSchema_ = java.util.Collections.emptyList();
customHashSchemaRanges_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PartitionSchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PartitionSchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
hashSchema_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
hashSchema_.add(
input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.PARSER, extensionRegistry));
break;
}
case 18: {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = rangeSchema_.toBuilder();
}
rangeSchema_ = input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rangeSchema_);
rangeSchema_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
customHashSchemaRanges_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
customHashSchemaRanges_.add(
input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
hashSchema_ = java.util.Collections.unmodifiableList(hashSchema_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
customHashSchemaRanges_ = java.util.Collections.unmodifiableList(customHashSchemaRanges_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.Builder.class);
}
public interface ColumnIdentifierPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionSchemaPB.ColumnIdentifierPB)
com.google.protobuf.MessageOrBuilder {
/**
* int32 id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
* int32 id = 1;
* @return The id.
*/
int getId();
/**
* string name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* string name = 2;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 2;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.IdentifierCase getIdentifierCase();
}
/**
*
* A column identifier for partition schemas. In general, the name will be
* used when a client creates the table since column IDs are assigned by the
* master. All other uses of partition schemas will use the numeric column ID.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB.ColumnIdentifierPB}
*/
public static final class ColumnIdentifierPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionSchemaPB.ColumnIdentifierPB)
ColumnIdentifierPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnIdentifierPB.newBuilder() to construct.
private ColumnIdentifierPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnIdentifierPB() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnIdentifierPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnIdentifierPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
identifier_ = input.readInt32();
identifierCase_ = 1;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
identifierCase_ = 2;
identifier_ = bs;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder.class);
}
private int bitField0_;
private int identifierCase_ = 0;
private java.lang.Object identifier_;
public enum IdentifierCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
ID(1),
NAME(2),
IDENTIFIER_NOT_SET(0);
private final int value;
private IdentifierCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IdentifierCase valueOf(int value) {
return forNumber(value);
}
public static IdentifierCase forNumber(int value) {
switch (value) {
case 1: return ID;
case 2: return NAME;
case 0: return IDENTIFIER_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public IdentifierCase
getIdentifierCase() {
return IdentifierCase.forNumber(
identifierCase_);
}
public static final int ID_FIELD_NUMBER = 1;
/**
* int32 id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return identifierCase_ == 1;
}
/**
* int32 id = 1;
* @return The id.
*/
@java.lang.Override
public int getId() {
if (identifierCase_ == 1) {
return (java.lang.Integer) identifier_;
}
return 0;
}
public static final int NAME_FIELD_NUMBER = 2;
/**
* string name = 2;
* @return Whether the name field is set.
*/
public boolean hasName() {
return identifierCase_ == 2;
}
/**
* string name = 2;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = "";
if (identifierCase_ == 2) {
ref = identifier_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8() && (identifierCase_ == 2)) {
identifier_ = s;
}
return s;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = "";
if (identifierCase_ == 2) {
ref = identifier_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (identifierCase_ == 2) {
identifier_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (identifierCase_ == 1) {
output.writeInt32(
1, (int)((java.lang.Integer) identifier_));
}
if (identifierCase_ == 2) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, identifier_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (identifierCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(
1, (int)((java.lang.Integer) identifier_));
}
if (identifierCase_ == 2) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, identifier_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB other = (org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB) obj;
if (!getIdentifierCase().equals(other.getIdentifierCase())) return false;
switch (identifierCase_) {
case 1:
if (getId()
!= other.getId()) return false;
break;
case 2:
if (!getName()
.equals(other.getName())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (identifierCase_) {
case 1:
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
break;
case 2:
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A column identifier for partition schemas. In general, the name will be
* used when a client creates the table since column IDs are assigned by the
* master. All other uses of partition schemas will use the numeric column ID.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB.ColumnIdentifierPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionSchemaPB.ColumnIdentifierPB)
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
identifierCase_ = 0;
identifier_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB build() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB result = new org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (identifierCase_ == 1) {
result.identifier_ = identifier_;
}
if (identifierCase_ == 2) {
result.identifier_ = identifier_;
}
result.bitField0_ = to_bitField0_;
result.identifierCase_ = identifierCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance()) return this;
switch (other.getIdentifierCase()) {
case ID: {
setId(other.getId());
break;
}
case NAME: {
identifierCase_ = 2;
identifier_ = other.identifier_;
onChanged();
break;
}
case IDENTIFIER_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int identifierCase_ = 0;
private java.lang.Object identifier_;
public IdentifierCase
getIdentifierCase() {
return IdentifierCase.forNumber(
identifierCase_);
}
public Builder clearIdentifier() {
identifierCase_ = 0;
identifier_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
* int32 id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return identifierCase_ == 1;
}
/**
* int32 id = 1;
* @return The id.
*/
public int getId() {
if (identifierCase_ == 1) {
return (java.lang.Integer) identifier_;
}
return 0;
}
/**
* int32 id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(int value) {
identifierCase_ = 1;
identifier_ = value;
onChanged();
return this;
}
/**
* int32 id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
if (identifierCase_ == 1) {
identifierCase_ = 0;
identifier_ = null;
onChanged();
}
return this;
}
/**
* string name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return identifierCase_ == 2;
}
/**
* string name = 2;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = "";
if (identifierCase_ == 2) {
ref = identifier_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (identifierCase_ == 2) {
if (bs.isValidUtf8()) {
identifier_ = s;
}
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 2;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = "";
if (identifierCase_ == 2) {
ref = identifier_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (identifierCase_ == 2) {
identifier_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
identifierCase_ = 2;
identifier_ = value;
onChanged();
return this;
}
/**
* string name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
if (identifierCase_ == 2) {
identifierCase_ = 0;
identifier_ = null;
onChanged();
}
return this;
}
/**
* string name = 2;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
identifierCase_ = 2;
identifier_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionSchemaPB.ColumnIdentifierPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionSchemaPB.ColumnIdentifierPB)
private static final org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB();
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnIdentifierPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnIdentifierPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RangeSchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionSchemaPB.RangeSchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
java.util.List
getColumnsList();
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index);
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
int getColumnsCount();
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList();
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index);
}
/**
* Protobuf type {@code kudu.PartitionSchemaPB.RangeSchemaPB}
*/
public static final class RangeSchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionSchemaPB.RangeSchemaPB)
RangeSchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use RangeSchemaPB.newBuilder() to construct.
private RangeSchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RangeSchemaPB() {
columns_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RangeSchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RangeSchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(
input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder.class);
}
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public java.util.List getColumnsList() {
return columns_;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public int getColumnsCount() {
return columns_.size();
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index) {
return columns_.get(index);
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB other = (org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB) obj;
if (!getColumnsList()
.equals(other.getColumnsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.PartitionSchemaPB.RangeSchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionSchemaPB.RangeSchemaPB)
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB build() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB result = new org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB(this);
int from_bitField0_ = bitField0_;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder> columnsBuilder_;
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance());
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance());
}
/**
*
* Column identifiers of columns included in the range. All columns must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>(
columns_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionSchemaPB.RangeSchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionSchemaPB.RangeSchemaPB)
private static final org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB();
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RangeSchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RangeSchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HashBucketSchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionSchemaPB.HashBucketSchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
java.util.List
getColumnsList();
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index);
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
int getColumnsCount();
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList();
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index);
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return Whether the numBuckets field is set.
*/
boolean hasNumBuckets();
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return The numBuckets.
*/
int getNumBuckets();
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return Whether the seed field is set.
*/
boolean hasSeed();
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return The seed.
*/
int getSeed();
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return Whether the hashAlgorithm field is set.
*/
boolean hasHashAlgorithm();
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return The hashAlgorithm.
*/
org.apache.kudu.Hash.HashAlgorithm getHashAlgorithm();
}
/**
* Protobuf type {@code kudu.PartitionSchemaPB.HashBucketSchemaPB}
*/
public static final class HashBucketSchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionSchemaPB.HashBucketSchemaPB)
HashBucketSchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use HashBucketSchemaPB.newBuilder() to construct.
private HashBucketSchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HashBucketSchemaPB() {
columns_ = java.util.Collections.emptyList();
hashAlgorithm_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HashBucketSchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private HashBucketSchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
columns_.add(
input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
numBuckets_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000002;
seed_ = input.readUInt32();
break;
}
case 32: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.apache.kudu.Hash.HashAlgorithm value = org.apache.kudu.Hash.HashAlgorithm.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000004;
hashAlgorithm_ = rawValue;
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder.class);
}
private int bitField0_;
public static final int COLUMNS_FIELD_NUMBER = 1;
private java.util.List columns_;
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public java.util.List getColumnsList() {
return columns_;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList() {
return columns_;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public int getColumnsCount() {
return columns_.size();
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index) {
return columns_.get(index);
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index) {
return columns_.get(index);
}
public static final int NUM_BUCKETS_FIELD_NUMBER = 2;
private int numBuckets_;
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return Whether the numBuckets field is set.
*/
@java.lang.Override
public boolean hasNumBuckets() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return The numBuckets.
*/
@java.lang.Override
public int getNumBuckets() {
return numBuckets_;
}
public static final int SEED_FIELD_NUMBER = 3;
private int seed_;
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return Whether the seed field is set.
*/
@java.lang.Override
public boolean hasSeed() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return The seed.
*/
@java.lang.Override
public int getSeed() {
return seed_;
}
public static final int HASH_ALGORITHM_FIELD_NUMBER = 4;
private int hashAlgorithm_;
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return Whether the hashAlgorithm field is set.
*/
@java.lang.Override public boolean hasHashAlgorithm() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return The hashAlgorithm.
*/
@java.lang.Override public org.apache.kudu.Hash.HashAlgorithm getHashAlgorithm() {
@SuppressWarnings("deprecation")
org.apache.kudu.Hash.HashAlgorithm result = org.apache.kudu.Hash.HashAlgorithm.valueOf(hashAlgorithm_);
return result == null ? org.apache.kudu.Hash.HashAlgorithm.UNKNOWN_HASH : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasNumBuckets()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < columns_.size(); i++) {
output.writeMessage(1, columns_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(2, numBuckets_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt32(3, seed_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(4, hashAlgorithm_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < columns_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, columns_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, numBuckets_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, seed_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, hashAlgorithm_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB other = (org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB) obj;
if (!getColumnsList()
.equals(other.getColumnsList())) return false;
if (hasNumBuckets() != other.hasNumBuckets()) return false;
if (hasNumBuckets()) {
if (getNumBuckets()
!= other.getNumBuckets()) return false;
}
if (hasSeed() != other.hasSeed()) return false;
if (hasSeed()) {
if (getSeed()
!= other.getSeed()) return false;
}
if (hasHashAlgorithm() != other.hasHashAlgorithm()) return false;
if (hasHashAlgorithm()) {
if (hashAlgorithm_ != other.hashAlgorithm_) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getColumnsCount() > 0) {
hash = (37 * hash) + COLUMNS_FIELD_NUMBER;
hash = (53 * hash) + getColumnsList().hashCode();
}
if (hasNumBuckets()) {
hash = (37 * hash) + NUM_BUCKETS_FIELD_NUMBER;
hash = (53 * hash) + getNumBuckets();
}
if (hasSeed()) {
hash = (37 * hash) + SEED_FIELD_NUMBER;
hash = (53 * hash) + getSeed();
}
if (hasHashAlgorithm()) {
hash = (37 * hash) + HASH_ALGORITHM_FIELD_NUMBER;
hash = (53 * hash) + hashAlgorithm_;
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.PartitionSchemaPB.HashBucketSchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionSchemaPB.HashBucketSchemaPB)
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getColumnsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
columnsBuilder_.clear();
}
numBuckets_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
seed_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
hashAlgorithm_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB build() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB result = new org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (columnsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
columns_ = java.util.Collections.unmodifiableList(columns_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.columns_ = columns_;
} else {
result.columns_ = columnsBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.numBuckets_ = numBuckets_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.seed_ = seed_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000004;
}
result.hashAlgorithm_ = hashAlgorithm_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance()) return this;
if (columnsBuilder_ == null) {
if (!other.columns_.isEmpty()) {
if (columns_.isEmpty()) {
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureColumnsIsMutable();
columns_.addAll(other.columns_);
}
onChanged();
}
} else {
if (!other.columns_.isEmpty()) {
if (columnsBuilder_.isEmpty()) {
columnsBuilder_.dispose();
columnsBuilder_ = null;
columns_ = other.columns_;
bitField0_ = (bitField0_ & ~0x00000001);
columnsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getColumnsFieldBuilder() : null;
} else {
columnsBuilder_.addAllMessages(other.columns_);
}
}
}
if (other.hasNumBuckets()) {
setNumBuckets(other.getNumBuckets());
}
if (other.hasSeed()) {
setSeed(other.getSeed());
}
if (other.hasHashAlgorithm()) {
setHashAlgorithm(other.getHashAlgorithm());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasNumBuckets()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List columns_ =
java.util.Collections.emptyList();
private void ensureColumnsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
columns_ = new java.util.ArrayList(columns_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder> columnsBuilder_;
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List getColumnsList() {
if (columnsBuilder_ == null) {
return java.util.Collections.unmodifiableList(columns_);
} else {
return columnsBuilder_.getMessageList();
}
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public int getColumnsCount() {
if (columnsBuilder_ == null) {
return columns_.size();
} else {
return columnsBuilder_.getCount();
}
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB getColumns(int index) {
if (columnsBuilder_ == null) {
return columns_.get(index);
} else {
return columnsBuilder_.getMessage(index);
}
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.set(index, value);
onChanged();
} else {
columnsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder setColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.set(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(value);
onChanged();
} else {
columnsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB value) {
if (columnsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureColumnsIsMutable();
columns_.add(index, value);
onChanged();
} else {
columnsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addColumns(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder builderForValue) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.add(index, builderForValue.build());
onChanged();
} else {
columnsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder addAllColumns(
java.lang.Iterable extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB> values) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, columns_);
onChanged();
} else {
columnsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder clearColumns() {
if (columnsBuilder_ == null) {
columns_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
columnsBuilder_.clear();
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public Builder removeColumns(int index) {
if (columnsBuilder_ == null) {
ensureColumnsIsMutable();
columns_.remove(index);
onChanged();
} else {
columnsBuilder_.remove(index);
}
return this;
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder getColumnsBuilder(
int index) {
return getColumnsFieldBuilder().getBuilder(index);
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder getColumnsOrBuilder(
int index) {
if (columnsBuilder_ == null) {
return columns_.get(index); } else {
return columnsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsOrBuilderList() {
if (columnsBuilder_ != null) {
return columnsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(columns_);
}
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder addColumnsBuilder() {
return getColumnsFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance());
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder addColumnsBuilder(
int index) {
return getColumnsFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.getDefaultInstance());
}
/**
*
* Column identifiers of columns included in the hash. Every column must be
* a component of the primary key.
*
*
* repeated .kudu.PartitionSchemaPB.ColumnIdentifierPB columns = 1;
*/
public java.util.List
getColumnsBuilderList() {
return getColumnsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>
getColumnsFieldBuilder() {
if (columnsBuilder_ == null) {
columnsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.ColumnIdentifierPBOrBuilder>(
columns_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
columns_ = null;
}
return columnsBuilder_;
}
private int numBuckets_ ;
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return Whether the numBuckets field is set.
*/
@java.lang.Override
public boolean hasNumBuckets() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return The numBuckets.
*/
@java.lang.Override
public int getNumBuckets() {
return numBuckets_;
}
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @param value The numBuckets to set.
* @return This builder for chaining.
*/
public Builder setNumBuckets(int value) {
bitField0_ |= 0x00000002;
numBuckets_ = value;
onChanged();
return this;
}
/**
*
* Number of buckets into which columns will be hashed. Must be at least 2.
*
*
* required int32 num_buckets = 2;
* @return This builder for chaining.
*/
public Builder clearNumBuckets() {
bitField0_ = (bitField0_ & ~0x00000002);
numBuckets_ = 0;
onChanged();
return this;
}
private int seed_ ;
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return Whether the seed field is set.
*/
@java.lang.Override
public boolean hasSeed() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return The seed.
*/
@java.lang.Override
public int getSeed() {
return seed_;
}
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @param value The seed to set.
* @return This builder for chaining.
*/
public Builder setSeed(int value) {
bitField0_ |= 0x00000004;
seed_ = value;
onChanged();
return this;
}
/**
*
* Seed value for hash calculation. Administrators may set a seed value
* on a per-table basis in order to randomize the mapping of rows to
* buckets. Setting a seed provides some amount of protection against denial
* of service attacks when the hash bucket columns contain user provided
* input.
*
*
* optional uint32 seed = 3;
* @return This builder for chaining.
*/
public Builder clearSeed() {
bitField0_ = (bitField0_ & ~0x00000004);
seed_ = 0;
onChanged();
return this;
}
private int hashAlgorithm_ = 0;
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return Whether the hashAlgorithm field is set.
*/
@java.lang.Override public boolean hasHashAlgorithm() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return The hashAlgorithm.
*/
@java.lang.Override
public org.apache.kudu.Hash.HashAlgorithm getHashAlgorithm() {
@SuppressWarnings("deprecation")
org.apache.kudu.Hash.HashAlgorithm result = org.apache.kudu.Hash.HashAlgorithm.valueOf(hashAlgorithm_);
return result == null ? org.apache.kudu.Hash.HashAlgorithm.UNKNOWN_HASH : result;
}
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @param value The hashAlgorithm to set.
* @return This builder for chaining.
*/
public Builder setHashAlgorithm(org.apache.kudu.Hash.HashAlgorithm value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
hashAlgorithm_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The hash algorithm to use for calculating the hash bucket.
* NOTE: this is not used yet -- don't expect setting it to have any effect
*
*
* optional .kudu.HashAlgorithm hash_algorithm = 4;
* @return This builder for chaining.
*/
public Builder clearHashAlgorithm() {
bitField0_ = (bitField0_ & ~0x00000008);
hashAlgorithm_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionSchemaPB.HashBucketSchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionSchemaPB.HashBucketSchemaPB)
private static final org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB();
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HashBucketSchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HashBucketSchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RangeWithHashSchemaPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionSchemaPB.RangeWithHashSchemaPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return Whether the rangeBounds field is set.
*/
boolean hasRangeBounds();
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return The rangeBounds.
*/
org.apache.kudu.RowOperations.RowOperationsPB getRangeBounds();
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRangeBoundsOrBuilder();
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
java.util.List
getHashSchemaList();
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index);
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
int getHashSchemaCount();
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList();
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index);
}
/**
*
* This data structure represents a range partition with a custom hash schema.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB.RangeWithHashSchemaPB}
*/
public static final class RangeWithHashSchemaPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionSchemaPB.RangeWithHashSchemaPB)
RangeWithHashSchemaPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use RangeWithHashSchemaPB.newBuilder() to construct.
private RangeWithHashSchemaPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RangeWithHashSchemaPB() {
hashSchema_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RangeWithHashSchemaPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RangeWithHashSchemaPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.apache.kudu.RowOperations.RowOperationsPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = rangeBounds_.toBuilder();
}
rangeBounds_ = input.readMessage(org.apache.kudu.RowOperations.RowOperationsPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rangeBounds_);
rangeBounds_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
hashSchema_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
hashSchema_.add(
input.readMessage(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.PARSER, extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
hashSchema_ = java.util.Collections.unmodifiableList(hashSchema_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder.class);
}
private int bitField0_;
public static final int RANGE_BOUNDS_FIELD_NUMBER = 1;
private org.apache.kudu.RowOperations.RowOperationsPB rangeBounds_;
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return Whether the rangeBounds field is set.
*/
@java.lang.Override
public boolean hasRangeBounds() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return The rangeBounds.
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPB getRangeBounds() {
return rangeBounds_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rangeBounds_;
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
@java.lang.Override
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRangeBoundsOrBuilder() {
return rangeBounds_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rangeBounds_;
}
public static final int HASH_SCHEMA_FIELD_NUMBER = 2;
private java.util.List hashSchema_;
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
@java.lang.Override
public java.util.List getHashSchemaList() {
return hashSchema_;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList() {
return hashSchema_;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
@java.lang.Override
public int getHashSchemaCount() {
return hashSchema_.size();
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index) {
return hashSchema_.get(index);
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index) {
return hashSchema_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getHashSchemaCount(); i++) {
if (!getHashSchema(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getRangeBounds());
}
for (int i = 0; i < hashSchema_.size(); i++) {
output.writeMessage(2, hashSchema_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRangeBounds());
}
for (int i = 0; i < hashSchema_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, hashSchema_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB other = (org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB) obj;
if (hasRangeBounds() != other.hasRangeBounds()) return false;
if (hasRangeBounds()) {
if (!getRangeBounds()
.equals(other.getRangeBounds())) return false;
}
if (!getHashSchemaList()
.equals(other.getHashSchemaList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRangeBounds()) {
hash = (37 * hash) + RANGE_BOUNDS_FIELD_NUMBER;
hash = (53 * hash) + getRangeBounds().hashCode();
}
if (getHashSchemaCount() > 0) {
hash = (37 * hash) + HASH_SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getHashSchemaList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This data structure represents a range partition with a custom hash schema.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB.RangeWithHashSchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionSchemaPB.RangeWithHashSchemaPB)
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRangeBoundsFieldBuilder();
getHashSchemaFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (rangeBoundsBuilder_ == null) {
rangeBounds_ = null;
} else {
rangeBoundsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (hashSchemaBuilder_ == null) {
hashSchema_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
hashSchemaBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB build() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB result = new org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (rangeBoundsBuilder_ == null) {
result.rangeBounds_ = rangeBounds_;
} else {
result.rangeBounds_ = rangeBoundsBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (hashSchemaBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
hashSchema_ = java.util.Collections.unmodifiableList(hashSchema_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.hashSchema_ = hashSchema_;
} else {
result.hashSchema_ = hashSchemaBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.getDefaultInstance()) return this;
if (other.hasRangeBounds()) {
mergeRangeBounds(other.getRangeBounds());
}
if (hashSchemaBuilder_ == null) {
if (!other.hashSchema_.isEmpty()) {
if (hashSchema_.isEmpty()) {
hashSchema_ = other.hashSchema_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureHashSchemaIsMutable();
hashSchema_.addAll(other.hashSchema_);
}
onChanged();
}
} else {
if (!other.hashSchema_.isEmpty()) {
if (hashSchemaBuilder_.isEmpty()) {
hashSchemaBuilder_.dispose();
hashSchemaBuilder_ = null;
hashSchema_ = other.hashSchema_;
bitField0_ = (bitField0_ & ~0x00000002);
hashSchemaBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHashSchemaFieldBuilder() : null;
} else {
hashSchemaBuilder_.addAllMessages(other.hashSchema_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getHashSchemaCount(); i++) {
if (!getHashSchema(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.apache.kudu.RowOperations.RowOperationsPB rangeBounds_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder> rangeBoundsBuilder_;
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return Whether the rangeBounds field is set.
*/
public boolean hasRangeBounds() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
* @return The rangeBounds.
*/
public org.apache.kudu.RowOperations.RowOperationsPB getRangeBounds() {
if (rangeBoundsBuilder_ == null) {
return rangeBounds_ == null ? org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rangeBounds_;
} else {
return rangeBoundsBuilder_.getMessage();
}
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public Builder setRangeBounds(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (rangeBoundsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rangeBounds_ = value;
onChanged();
} else {
rangeBoundsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public Builder setRangeBounds(
org.apache.kudu.RowOperations.RowOperationsPB.Builder builderForValue) {
if (rangeBoundsBuilder_ == null) {
rangeBounds_ = builderForValue.build();
onChanged();
} else {
rangeBoundsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public Builder mergeRangeBounds(org.apache.kudu.RowOperations.RowOperationsPB value) {
if (rangeBoundsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
rangeBounds_ != null &&
rangeBounds_ != org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance()) {
rangeBounds_ =
org.apache.kudu.RowOperations.RowOperationsPB.newBuilder(rangeBounds_).mergeFrom(value).buildPartial();
} else {
rangeBounds_ = value;
}
onChanged();
} else {
rangeBoundsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public Builder clearRangeBounds() {
if (rangeBoundsBuilder_ == null) {
rangeBounds_ = null;
onChanged();
} else {
rangeBoundsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public org.apache.kudu.RowOperations.RowOperationsPB.Builder getRangeBoundsBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRangeBoundsFieldBuilder().getBuilder();
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
public org.apache.kudu.RowOperations.RowOperationsPBOrBuilder getRangeBoundsOrBuilder() {
if (rangeBoundsBuilder_ != null) {
return rangeBoundsBuilder_.getMessageOrBuilder();
} else {
return rangeBounds_ == null ?
org.apache.kudu.RowOperations.RowOperationsPB.getDefaultInstance() : rangeBounds_;
}
}
/**
*
* Row operations containing the lower and upper range bound for the range.
*
*
* optional .kudu.RowOperationsPB range_bounds = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>
getRangeBoundsFieldBuilder() {
if (rangeBoundsBuilder_ == null) {
rangeBoundsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.RowOperations.RowOperationsPB, org.apache.kudu.RowOperations.RowOperationsPB.Builder, org.apache.kudu.RowOperations.RowOperationsPBOrBuilder>(
getRangeBounds(),
getParentForChildren(),
isClean());
rangeBounds_ = null;
}
return rangeBoundsBuilder_;
}
private java.util.List hashSchema_ =
java.util.Collections.emptyList();
private void ensureHashSchemaIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
hashSchema_ = new java.util.ArrayList(hashSchema_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder> hashSchemaBuilder_;
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public java.util.List getHashSchemaList() {
if (hashSchemaBuilder_ == null) {
return java.util.Collections.unmodifiableList(hashSchema_);
} else {
return hashSchemaBuilder_.getMessageList();
}
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public int getHashSchemaCount() {
if (hashSchemaBuilder_ == null) {
return hashSchema_.size();
} else {
return hashSchemaBuilder_.getCount();
}
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index) {
if (hashSchemaBuilder_ == null) {
return hashSchema_.get(index);
} else {
return hashSchemaBuilder_.getMessage(index);
}
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder setHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.set(index, value);
onChanged();
} else {
hashSchemaBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder setHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.set(index, builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder addHashSchema(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.add(value);
onChanged();
} else {
hashSchemaBuilder_.addMessage(value);
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder addHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.add(index, value);
onChanged();
} else {
hashSchemaBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder addHashSchema(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.add(builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder addHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.add(index, builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder addAllHashSchema(
java.lang.Iterable extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB> values) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hashSchema_);
onChanged();
} else {
hashSchemaBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder clearHashSchema() {
if (hashSchemaBuilder_ == null) {
hashSchema_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
hashSchemaBuilder_.clear();
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public Builder removeHashSchema(int index) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.remove(index);
onChanged();
} else {
hashSchemaBuilder_.remove(index);
}
return this;
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder getHashSchemaBuilder(
int index) {
return getHashSchemaFieldBuilder().getBuilder(index);
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index) {
if (hashSchemaBuilder_ == null) {
return hashSchema_.get(index); } else {
return hashSchemaBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList() {
if (hashSchemaBuilder_ != null) {
return hashSchemaBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(hashSchema_);
}
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder addHashSchemaBuilder() {
return getHashSchemaFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance());
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder addHashSchemaBuilder(
int index) {
return getHashSchemaFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance());
}
/**
*
* Hash schema for the range.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 2;
*/
public java.util.List
getHashSchemaBuilderList() {
return getHashSchemaFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaFieldBuilder() {
if (hashSchemaBuilder_ == null) {
hashSchemaBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>(
hashSchema_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
hashSchema_ = null;
}
return hashSchemaBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionSchemaPB.RangeWithHashSchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionSchemaPB.RangeWithHashSchemaPB)
private static final org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB();
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RangeWithHashSchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RangeWithHashSchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int HASH_SCHEMA_FIELD_NUMBER = 1;
private java.util.List hashSchema_;
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
@java.lang.Override
public java.util.List getHashSchemaList() {
return hashSchema_;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList() {
return hashSchema_;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
@java.lang.Override
public int getHashSchemaCount() {
return hashSchema_.size();
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index) {
return hashSchema_.get(index);
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index) {
return hashSchema_.get(index);
}
public static final int RANGE_SCHEMA_FIELD_NUMBER = 2;
private org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB rangeSchema_;
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return Whether the rangeSchema field is set.
*/
@java.lang.Override
public boolean hasRangeSchema() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return The rangeSchema.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getRangeSchema() {
return rangeSchema_ == null ? org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance() : rangeSchema_;
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder getRangeSchemaOrBuilder() {
return rangeSchema_ == null ? org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance() : rangeSchema_;
}
public static final int CUSTOM_HASH_SCHEMA_RANGES_FIELD_NUMBER = 5;
private java.util.List customHashSchemaRanges_;
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
@java.lang.Override
public java.util.List getCustomHashSchemaRangesList() {
return customHashSchemaRanges_;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder>
getCustomHashSchemaRangesOrBuilderList() {
return customHashSchemaRanges_;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
@java.lang.Override
public int getCustomHashSchemaRangesCount() {
return customHashSchemaRanges_.size();
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getCustomHashSchemaRanges(int index) {
return customHashSchemaRanges_.get(index);
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder getCustomHashSchemaRangesOrBuilder(
int index) {
return customHashSchemaRanges_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getHashSchemaCount(); i++) {
if (!getHashSchema(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getCustomHashSchemaRangesCount(); i++) {
if (!getCustomHashSchemaRanges(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < hashSchema_.size(); i++) {
output.writeMessage(1, hashSchema_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getRangeSchema());
}
for (int i = 0; i < customHashSchemaRanges_.size(); i++) {
output.writeMessage(5, customHashSchemaRanges_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < hashSchema_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, hashSchema_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getRangeSchema());
}
for (int i = 0; i < customHashSchemaRanges_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, customHashSchemaRanges_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionSchemaPB other = (org.apache.kudu.KuduCommon.PartitionSchemaPB) obj;
if (!getHashSchemaList()
.equals(other.getHashSchemaList())) return false;
if (hasRangeSchema() != other.hasRangeSchema()) return false;
if (hasRangeSchema()) {
if (!getRangeSchema()
.equals(other.getRangeSchema())) return false;
}
if (!getCustomHashSchemaRangesList()
.equals(other.getCustomHashSchemaRangesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getHashSchemaCount() > 0) {
hash = (37 * hash) + HASH_SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getHashSchemaList().hashCode();
}
if (hasRangeSchema()) {
hash = (37 * hash) + RANGE_SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getRangeSchema().hashCode();
}
if (getCustomHashSchemaRangesCount() > 0) {
hash = (37 * hash) + CUSTOM_HASH_SCHEMA_RANGES_FIELD_NUMBER;
hash = (53 * hash) + getCustomHashSchemaRangesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionSchemaPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The serialized format of a Kudu table partition schema.
*
*
* Protobuf type {@code kudu.PartitionSchemaPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionSchemaPB)
org.apache.kudu.KuduCommon.PartitionSchemaPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionSchemaPB.class, org.apache.kudu.KuduCommon.PartitionSchemaPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionSchemaPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHashSchemaFieldBuilder();
getRangeSchemaFieldBuilder();
getCustomHashSchemaRangesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (hashSchemaBuilder_ == null) {
hashSchema_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
hashSchemaBuilder_.clear();
}
if (rangeSchemaBuilder_ == null) {
rangeSchema_ = null;
} else {
rangeSchemaBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (customHashSchemaRangesBuilder_ == null) {
customHashSchemaRanges_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
customHashSchemaRangesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionSchemaPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionSchemaPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB build() {
org.apache.kudu.KuduCommon.PartitionSchemaPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionSchemaPB result = new org.apache.kudu.KuduCommon.PartitionSchemaPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (hashSchemaBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
hashSchema_ = java.util.Collections.unmodifiableList(hashSchema_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.hashSchema_ = hashSchema_;
} else {
result.hashSchema_ = hashSchemaBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (rangeSchemaBuilder_ == null) {
result.rangeSchema_ = rangeSchema_;
} else {
result.rangeSchema_ = rangeSchemaBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (customHashSchemaRangesBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
customHashSchemaRanges_ = java.util.Collections.unmodifiableList(customHashSchemaRanges_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.customHashSchemaRanges_ = customHashSchemaRanges_;
} else {
result.customHashSchemaRanges_ = customHashSchemaRangesBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionSchemaPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionSchemaPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionSchemaPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionSchemaPB.getDefaultInstance()) return this;
if (hashSchemaBuilder_ == null) {
if (!other.hashSchema_.isEmpty()) {
if (hashSchema_.isEmpty()) {
hashSchema_ = other.hashSchema_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureHashSchemaIsMutable();
hashSchema_.addAll(other.hashSchema_);
}
onChanged();
}
} else {
if (!other.hashSchema_.isEmpty()) {
if (hashSchemaBuilder_.isEmpty()) {
hashSchemaBuilder_.dispose();
hashSchemaBuilder_ = null;
hashSchema_ = other.hashSchema_;
bitField0_ = (bitField0_ & ~0x00000001);
hashSchemaBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHashSchemaFieldBuilder() : null;
} else {
hashSchemaBuilder_.addAllMessages(other.hashSchema_);
}
}
}
if (other.hasRangeSchema()) {
mergeRangeSchema(other.getRangeSchema());
}
if (customHashSchemaRangesBuilder_ == null) {
if (!other.customHashSchemaRanges_.isEmpty()) {
if (customHashSchemaRanges_.isEmpty()) {
customHashSchemaRanges_ = other.customHashSchemaRanges_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.addAll(other.customHashSchemaRanges_);
}
onChanged();
}
} else {
if (!other.customHashSchemaRanges_.isEmpty()) {
if (customHashSchemaRangesBuilder_.isEmpty()) {
customHashSchemaRangesBuilder_.dispose();
customHashSchemaRangesBuilder_ = null;
customHashSchemaRanges_ = other.customHashSchemaRanges_;
bitField0_ = (bitField0_ & ~0x00000004);
customHashSchemaRangesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getCustomHashSchemaRangesFieldBuilder() : null;
} else {
customHashSchemaRangesBuilder_.addAllMessages(other.customHashSchemaRanges_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getHashSchemaCount(); i++) {
if (!getHashSchema(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getCustomHashSchemaRangesCount(); i++) {
if (!getCustomHashSchemaRanges(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionSchemaPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionSchemaPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List hashSchema_ =
java.util.Collections.emptyList();
private void ensureHashSchemaIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
hashSchema_ = new java.util.ArrayList(hashSchema_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder> hashSchemaBuilder_;
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public java.util.List getHashSchemaList() {
if (hashSchemaBuilder_ == null) {
return java.util.Collections.unmodifiableList(hashSchema_);
} else {
return hashSchemaBuilder_.getMessageList();
}
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public int getHashSchemaCount() {
if (hashSchemaBuilder_ == null) {
return hashSchema_.size();
} else {
return hashSchemaBuilder_.getCount();
}
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB getHashSchema(int index) {
if (hashSchemaBuilder_ == null) {
return hashSchema_.get(index);
} else {
return hashSchemaBuilder_.getMessage(index);
}
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder setHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.set(index, value);
onChanged();
} else {
hashSchemaBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder setHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.set(index, builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder addHashSchema(org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.add(value);
onChanged();
} else {
hashSchemaBuilder_.addMessage(value);
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder addHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB value) {
if (hashSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHashSchemaIsMutable();
hashSchema_.add(index, value);
onChanged();
} else {
hashSchemaBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder addHashSchema(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.add(builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder addHashSchema(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder builderForValue) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.add(index, builderForValue.build());
onChanged();
} else {
hashSchemaBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder addAllHashSchema(
java.lang.Iterable extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB> values) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hashSchema_);
onChanged();
} else {
hashSchemaBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder clearHashSchema() {
if (hashSchemaBuilder_ == null) {
hashSchema_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
hashSchemaBuilder_.clear();
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public Builder removeHashSchema(int index) {
if (hashSchemaBuilder_ == null) {
ensureHashSchemaIsMutable();
hashSchema_.remove(index);
onChanged();
} else {
hashSchemaBuilder_.remove(index);
}
return this;
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder getHashSchemaBuilder(
int index) {
return getHashSchemaFieldBuilder().getBuilder(index);
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder getHashSchemaOrBuilder(
int index) {
if (hashSchemaBuilder_ == null) {
return hashSchema_.get(index); } else {
return hashSchemaBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaOrBuilderList() {
if (hashSchemaBuilder_ != null) {
return hashSchemaBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(hashSchema_);
}
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder addHashSchemaBuilder() {
return getHashSchemaFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance());
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder addHashSchemaBuilder(
int index) {
return getHashSchemaFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.getDefaultInstance());
}
/**
*
* Table-wide hash schema. Hash schema for a particular range may be
* overriden by corresponding element in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.HashBucketSchemaPB hash_schema = 1;
*/
public java.util.List
getHashSchemaBuilderList() {
return getHashSchemaFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>
getHashSchemaFieldBuilder() {
if (hashSchemaBuilder_ == null) {
hashSchemaBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.HashBucketSchemaPBOrBuilder>(
hashSchema_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
hashSchema_ = null;
}
return hashSchemaBuilder_;
}
private org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB rangeSchema_;
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder> rangeSchemaBuilder_;
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return Whether the rangeSchema field is set.
*/
public boolean hasRangeSchema() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
* @return The rangeSchema.
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB getRangeSchema() {
if (rangeSchemaBuilder_ == null) {
return rangeSchema_ == null ? org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance() : rangeSchema_;
} else {
return rangeSchemaBuilder_.getMessage();
}
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public Builder setRangeSchema(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB value) {
if (rangeSchemaBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rangeSchema_ = value;
onChanged();
} else {
rangeSchemaBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public Builder setRangeSchema(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder builderForValue) {
if (rangeSchemaBuilder_ == null) {
rangeSchema_ = builderForValue.build();
onChanged();
} else {
rangeSchemaBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public Builder mergeRangeSchema(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB value) {
if (rangeSchemaBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
rangeSchema_ != null &&
rangeSchema_ != org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance()) {
rangeSchema_ =
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.newBuilder(rangeSchema_).mergeFrom(value).buildPartial();
} else {
rangeSchema_ = value;
}
onChanged();
} else {
rangeSchemaBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public Builder clearRangeSchema() {
if (rangeSchemaBuilder_ == null) {
rangeSchema_ = null;
onChanged();
} else {
rangeSchemaBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder getRangeSchemaBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getRangeSchemaFieldBuilder().getBuilder();
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder getRangeSchemaOrBuilder() {
if (rangeSchemaBuilder_ != null) {
return rangeSchemaBuilder_.getMessageOrBuilder();
} else {
return rangeSchema_ == null ?
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.getDefaultInstance() : rangeSchema_;
}
}
/**
*
* Range schema to partition the key space into ranges.
*
*
* optional .kudu.PartitionSchemaPB.RangeSchemaPB range_schema = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder>
getRangeSchemaFieldBuilder() {
if (rangeSchemaBuilder_ == null) {
rangeSchemaBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeSchemaPBOrBuilder>(
getRangeSchema(),
getParentForChildren(),
isClean());
rangeSchema_ = null;
}
return rangeSchemaBuilder_;
}
private java.util.List customHashSchemaRanges_ =
java.util.Collections.emptyList();
private void ensureCustomHashSchemaRangesIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
customHashSchemaRanges_ = new java.util.ArrayList(customHashSchemaRanges_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder> customHashSchemaRangesBuilder_;
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public java.util.List getCustomHashSchemaRangesList() {
if (customHashSchemaRangesBuilder_ == null) {
return java.util.Collections.unmodifiableList(customHashSchemaRanges_);
} else {
return customHashSchemaRangesBuilder_.getMessageList();
}
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public int getCustomHashSchemaRangesCount() {
if (customHashSchemaRangesBuilder_ == null) {
return customHashSchemaRanges_.size();
} else {
return customHashSchemaRangesBuilder_.getCount();
}
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB getCustomHashSchemaRanges(int index) {
if (customHashSchemaRangesBuilder_ == null) {
return customHashSchemaRanges_.get(index);
} else {
return customHashSchemaRangesBuilder_.getMessage(index);
}
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder setCustomHashSchemaRanges(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB value) {
if (customHashSchemaRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.set(index, value);
onChanged();
} else {
customHashSchemaRangesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder setCustomHashSchemaRanges(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder builderForValue) {
if (customHashSchemaRangesBuilder_ == null) {
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.set(index, builderForValue.build());
onChanged();
} else {
customHashSchemaRangesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder addCustomHashSchemaRanges(org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB value) {
if (customHashSchemaRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.add(value);
onChanged();
} else {
customHashSchemaRangesBuilder_.addMessage(value);
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder addCustomHashSchemaRanges(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB value) {
if (customHashSchemaRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.add(index, value);
onChanged();
} else {
customHashSchemaRangesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder addCustomHashSchemaRanges(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder builderForValue) {
if (customHashSchemaRangesBuilder_ == null) {
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.add(builderForValue.build());
onChanged();
} else {
customHashSchemaRangesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder addCustomHashSchemaRanges(
int index, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder builderForValue) {
if (customHashSchemaRangesBuilder_ == null) {
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.add(index, builderForValue.build());
onChanged();
} else {
customHashSchemaRangesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder addAllCustomHashSchemaRanges(
java.lang.Iterable extends org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB> values) {
if (customHashSchemaRangesBuilder_ == null) {
ensureCustomHashSchemaRangesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, customHashSchemaRanges_);
onChanged();
} else {
customHashSchemaRangesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder clearCustomHashSchemaRanges() {
if (customHashSchemaRangesBuilder_ == null) {
customHashSchemaRanges_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
customHashSchemaRangesBuilder_.clear();
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public Builder removeCustomHashSchemaRanges(int index) {
if (customHashSchemaRangesBuilder_ == null) {
ensureCustomHashSchemaRangesIsMutable();
customHashSchemaRanges_.remove(index);
onChanged();
} else {
customHashSchemaRangesBuilder_.remove(index);
}
return this;
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder getCustomHashSchemaRangesBuilder(
int index) {
return getCustomHashSchemaRangesFieldBuilder().getBuilder(index);
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder getCustomHashSchemaRangesOrBuilder(
int index) {
if (customHashSchemaRangesBuilder_ == null) {
return customHashSchemaRanges_.get(index); } else {
return customHashSchemaRangesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public java.util.List extends org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder>
getCustomHashSchemaRangesOrBuilderList() {
if (customHashSchemaRangesBuilder_ != null) {
return customHashSchemaRangesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(customHashSchemaRanges_);
}
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder addCustomHashSchemaRangesBuilder() {
return getCustomHashSchemaRangesFieldBuilder().addBuilder(
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.getDefaultInstance());
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder addCustomHashSchemaRangesBuilder(
int index) {
return getCustomHashSchemaRangesFieldBuilder().addBuilder(
index, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.getDefaultInstance());
}
/**
*
* If the 'custom_hash_schema_ranges' field is empty, the table-wide hash
* schema specified by the 'hash_schema' field is used for all the ranges
* of the table. Otherwise, particular ranges have their hash schema
* as specified by corresponding elements in 'custom_hash_schema_ranges'.
*
*
* repeated .kudu.PartitionSchemaPB.RangeWithHashSchemaPB custom_hash_schema_ranges = 5;
*/
public java.util.List
getCustomHashSchemaRangesBuilderList() {
return getCustomHashSchemaRangesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder>
getCustomHashSchemaRangesFieldBuilder() {
if (customHashSchemaRangesBuilder_ == null) {
customHashSchemaRangesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPB.Builder, org.apache.kudu.KuduCommon.PartitionSchemaPB.RangeWithHashSchemaPBOrBuilder>(
customHashSchemaRanges_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
customHashSchemaRanges_ = null;
}
return customHashSchemaRangesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionSchemaPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionSchemaPB)
private static final org.apache.kudu.KuduCommon.PartitionSchemaPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionSchemaPB();
}
public static org.apache.kudu.KuduCommon.PartitionSchemaPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PartitionSchemaPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PartitionSchemaPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionSchemaPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PartitionPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.PartitionPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return A list containing the hashBuckets.
*/
java.util.List getHashBucketsList();
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return The count of hashBuckets.
*/
int getHashBucketsCount();
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param index The index of the element to return.
* @return The hashBuckets at the given index.
*/
int getHashBuckets(int index);
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return Whether the partitionKeyStart field is set.
*/
boolean hasPartitionKeyStart();
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return The partitionKeyStart.
*/
com.google.protobuf.ByteString getPartitionKeyStart();
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return Whether the partitionKeyEnd field is set.
*/
boolean hasPartitionKeyEnd();
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return The partitionKeyEnd.
*/
com.google.protobuf.ByteString getPartitionKeyEnd();
}
/**
*
* The serialized format of a Kudu table partition.
*
*
* Protobuf type {@code kudu.PartitionPB}
*/
public static final class PartitionPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.PartitionPB)
PartitionPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use PartitionPB.newBuilder() to construct.
private PartitionPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PartitionPB() {
hashBuckets_ = emptyIntList();
partitionKeyStart_ = com.google.protobuf.ByteString.EMPTY;
partitionKeyEnd_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PartitionPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PartitionPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
hashBuckets_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
hashBuckets_.addInt(input.readInt32());
break;
}
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
hashBuckets_ = newIntList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
hashBuckets_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
case 18: {
bitField0_ |= 0x00000001;
partitionKeyStart_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000002;
partitionKeyEnd_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
hashBuckets_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionPB.class, org.apache.kudu.KuduCommon.PartitionPB.Builder.class);
}
private int bitField0_;
public static final int HASH_BUCKETS_FIELD_NUMBER = 1;
private com.google.protobuf.Internal.IntList hashBuckets_;
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return A list containing the hashBuckets.
*/
@java.lang.Override
public java.util.List
getHashBucketsList() {
return hashBuckets_;
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return The count of hashBuckets.
*/
public int getHashBucketsCount() {
return hashBuckets_.size();
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param index The index of the element to return.
* @return The hashBuckets at the given index.
*/
public int getHashBuckets(int index) {
return hashBuckets_.getInt(index);
}
private int hashBucketsMemoizedSerializedSize = -1;
public static final int PARTITION_KEY_START_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString partitionKeyStart_;
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return Whether the partitionKeyStart field is set.
*/
@java.lang.Override
public boolean hasPartitionKeyStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return The partitionKeyStart.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPartitionKeyStart() {
return partitionKeyStart_;
}
public static final int PARTITION_KEY_END_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString partitionKeyEnd_;
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return Whether the partitionKeyEnd field is set.
*/
@java.lang.Override
public boolean hasPartitionKeyEnd() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return The partitionKeyEnd.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPartitionKeyEnd() {
return partitionKeyEnd_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getHashBucketsList().size() > 0) {
output.writeUInt32NoTag(10);
output.writeUInt32NoTag(hashBucketsMemoizedSerializedSize);
}
for (int i = 0; i < hashBuckets_.size(); i++) {
output.writeInt32NoTag(hashBuckets_.getInt(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(2, partitionKeyStart_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(3, partitionKeyEnd_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < hashBuckets_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(hashBuckets_.getInt(i));
}
size += dataSize;
if (!getHashBucketsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
hashBucketsMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, partitionKeyStart_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, partitionKeyEnd_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.PartitionPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.PartitionPB other = (org.apache.kudu.KuduCommon.PartitionPB) obj;
if (!getHashBucketsList()
.equals(other.getHashBucketsList())) return false;
if (hasPartitionKeyStart() != other.hasPartitionKeyStart()) return false;
if (hasPartitionKeyStart()) {
if (!getPartitionKeyStart()
.equals(other.getPartitionKeyStart())) return false;
}
if (hasPartitionKeyEnd() != other.hasPartitionKeyEnd()) return false;
if (hasPartitionKeyEnd()) {
if (!getPartitionKeyEnd()
.equals(other.getPartitionKeyEnd())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getHashBucketsCount() > 0) {
hash = (37 * hash) + HASH_BUCKETS_FIELD_NUMBER;
hash = (53 * hash) + getHashBucketsList().hashCode();
}
if (hasPartitionKeyStart()) {
hash = (37 * hash) + PARTITION_KEY_START_FIELD_NUMBER;
hash = (53 * hash) + getPartitionKeyStart().hashCode();
}
if (hasPartitionKeyEnd()) {
hash = (37 * hash) + PARTITION_KEY_END_FIELD_NUMBER;
hash = (53 * hash) + getPartitionKeyEnd().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.PartitionPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.PartitionPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The serialized format of a Kudu table partition.
*
*
* Protobuf type {@code kudu.PartitionPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.PartitionPB)
org.apache.kudu.KuduCommon.PartitionPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.PartitionPB.class, org.apache.kudu.KuduCommon.PartitionPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.PartitionPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
hashBuckets_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
partitionKeyStart_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
partitionKeyEnd_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_PartitionPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.PartitionPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionPB build() {
org.apache.kudu.KuduCommon.PartitionPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionPB buildPartial() {
org.apache.kudu.KuduCommon.PartitionPB result = new org.apache.kudu.KuduCommon.PartitionPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) != 0)) {
hashBuckets_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.hashBuckets_ = hashBuckets_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.partitionKeyStart_ = partitionKeyStart_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.partitionKeyEnd_ = partitionKeyEnd_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.PartitionPB) {
return mergeFrom((org.apache.kudu.KuduCommon.PartitionPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.PartitionPB other) {
if (other == org.apache.kudu.KuduCommon.PartitionPB.getDefaultInstance()) return this;
if (!other.hashBuckets_.isEmpty()) {
if (hashBuckets_.isEmpty()) {
hashBuckets_ = other.hashBuckets_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureHashBucketsIsMutable();
hashBuckets_.addAll(other.hashBuckets_);
}
onChanged();
}
if (other.hasPartitionKeyStart()) {
setPartitionKeyStart(other.getPartitionKeyStart());
}
if (other.hasPartitionKeyEnd()) {
setPartitionKeyEnd(other.getPartitionKeyEnd());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.PartitionPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.PartitionPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList hashBuckets_ = emptyIntList();
private void ensureHashBucketsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
hashBuckets_ = mutableCopy(hashBuckets_);
bitField0_ |= 0x00000001;
}
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return A list containing the hashBuckets.
*/
public java.util.List
getHashBucketsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(hashBuckets_) : hashBuckets_;
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return The count of hashBuckets.
*/
public int getHashBucketsCount() {
return hashBuckets_.size();
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param index The index of the element to return.
* @return The hashBuckets at the given index.
*/
public int getHashBuckets(int index) {
return hashBuckets_.getInt(index);
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param index The index to set the value at.
* @param value The hashBuckets to set.
* @return This builder for chaining.
*/
public Builder setHashBuckets(
int index, int value) {
ensureHashBucketsIsMutable();
hashBuckets_.setInt(index, value);
onChanged();
return this;
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param value The hashBuckets to add.
* @return This builder for chaining.
*/
public Builder addHashBuckets(int value) {
ensureHashBucketsIsMutable();
hashBuckets_.addInt(value);
onChanged();
return this;
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @param values The hashBuckets to add.
* @return This builder for chaining.
*/
public Builder addAllHashBuckets(
java.lang.Iterable extends java.lang.Integer> values) {
ensureHashBucketsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hashBuckets_);
onChanged();
return this;
}
/**
*
* The hash buckets of the partition. The number of hash buckets must match
* the number of hash dimensions in the partition's schema.
*
*
* repeated int32 hash_buckets = 1 [packed = true];
* @return This builder for chaining.
*/
public Builder clearHashBuckets() {
hashBuckets_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private com.google.protobuf.ByteString partitionKeyStart_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return Whether the partitionKeyStart field is set.
*/
@java.lang.Override
public boolean hasPartitionKeyStart() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return The partitionKeyStart.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPartitionKeyStart() {
return partitionKeyStart_;
}
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @param value The partitionKeyStart to set.
* @return This builder for chaining.
*/
public Builder setPartitionKeyStart(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
partitionKeyStart_ = value;
onChanged();
return this;
}
/**
*
* The encoded start partition key (inclusive).
*
*
* optional bytes partition_key_start = 2;
* @return This builder for chaining.
*/
public Builder clearPartitionKeyStart() {
bitField0_ = (bitField0_ & ~0x00000002);
partitionKeyStart_ = getDefaultInstance().getPartitionKeyStart();
onChanged();
return this;
}
private com.google.protobuf.ByteString partitionKeyEnd_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return Whether the partitionKeyEnd field is set.
*/
@java.lang.Override
public boolean hasPartitionKeyEnd() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return The partitionKeyEnd.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPartitionKeyEnd() {
return partitionKeyEnd_;
}
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @param value The partitionKeyEnd to set.
* @return This builder for chaining.
*/
public Builder setPartitionKeyEnd(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
partitionKeyEnd_ = value;
onChanged();
return this;
}
/**
*
* The encoded end partition key (exclusive).
*
*
* optional bytes partition_key_end = 3;
* @return This builder for chaining.
*/
public Builder clearPartitionKeyEnd() {
bitField0_ = (bitField0_ & ~0x00000004);
partitionKeyEnd_ = getDefaultInstance().getPartitionKeyEnd();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.PartitionPB)
}
// @@protoc_insertion_point(class_scope:kudu.PartitionPB)
private static final org.apache.kudu.KuduCommon.PartitionPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.PartitionPB();
}
public static org.apache.kudu.KuduCommon.PartitionPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PartitionPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PartitionPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.PartitionPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ColumnPredicatePBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB)
com.google.protobuf.MessageOrBuilder {
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return Whether the column field is set.
*/
boolean hasColumn();
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The column.
*/
java.lang.String getColumn();
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The bytes for column.
*/
com.google.protobuf.ByteString
getColumnBytes();
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return Whether the range field is set.
*/
boolean hasRange();
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return The range.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getRange();
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder getRangeOrBuilder();
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return Whether the equality field is set.
*/
boolean hasEquality();
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return The equality.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getEquality();
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder getEqualityOrBuilder();
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return Whether the isNotNull field is set.
*/
boolean hasIsNotNull();
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return The isNotNull.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getIsNotNull();
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder getIsNotNullOrBuilder();
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return Whether the inList field is set.
*/
boolean hasInList();
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return The inList.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getInList();
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder getInListOrBuilder();
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return Whether the isNull field is set.
*/
boolean hasIsNull();
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return The isNull.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getIsNull();
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder getIsNullOrBuilder();
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return Whether the inBloomFilter field is set.
*/
boolean hasInBloomFilter();
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return The inBloomFilter.
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getInBloomFilter();
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder getInBloomFilterOrBuilder();
public org.apache.kudu.KuduCommon.ColumnPredicatePB.PredicateCase getPredicateCase();
}
/**
*
* A predicate that can be applied on a Kudu column.
*
*
* Protobuf type {@code kudu.ColumnPredicatePB}
*/
public static final class ColumnPredicatePB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB)
ColumnPredicatePBOrBuilder {
private static final long serialVersionUID = 0L;
// Use ColumnPredicatePB.newBuilder() to construct.
private ColumnPredicatePB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ColumnPredicatePB() {
column_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ColumnPredicatePB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ColumnPredicatePB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
column_ = bs;
break;
}
case 18: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder subBuilder = null;
if (predicateCase_ == 2) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 2;
break;
}
case 26: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder subBuilder = null;
if (predicateCase_ == 3) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 3;
break;
}
case 34: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder subBuilder = null;
if (predicateCase_ == 4) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 4;
break;
}
case 42: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder subBuilder = null;
if (predicateCase_ == 5) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 5;
break;
}
case 50: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder subBuilder = null;
if (predicateCase_ == 6) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 6;
break;
}
case 58: {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder subBuilder = null;
if (predicateCase_ == 7) {
subBuilder = ((org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_).toBuilder();
}
predicate_ =
input.readMessage(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_);
predicate_ = subBuilder.buildPartial();
}
predicateCase_ = 7;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Builder.class);
}
public interface RangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.Range)
com.google.protobuf.MessageOrBuilder {
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
boolean hasLower();
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return The lower.
*/
com.google.protobuf.ByteString getLower();
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
boolean hasUpper();
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return The upper.
*/
com.google.protobuf.ByteString getUpper();
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.Range}
*/
public static final class Range extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.Range)
RangeOrBuilder {
private static final long serialVersionUID = 0L;
// Use Range.newBuilder() to construct.
private Range(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Range() {
lower_ = com.google.protobuf.ByteString.EMPTY;
upper_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Range();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Range(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
lower_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
upper_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Range_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Range_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder.class);
}
private int bitField0_;
public static final int LOWER_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString lower_;
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
@java.lang.Override
public boolean hasLower() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return The lower.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLower() {
return lower_;
}
public static final int UPPER_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString upper_;
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
@java.lang.Override
public boolean hasUpper() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return The upper.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUpper() {
return upper_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, lower_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, upper_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, lower_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, upper_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.Range)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) obj;
if (hasLower() != other.hasLower()) return false;
if (hasLower()) {
if (!getLower()
.equals(other.getLower())) return false;
}
if (hasUpper() != other.hasUpper()) return false;
if (hasUpper()) {
if (!getUpper()
.equals(other.getUpper())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLower()) {
hash = (37 * hash) + LOWER_FIELD_NUMBER;
hash = (53 * hash) + getLower().hashCode();
}
if (hasUpper()) {
hash = (37 * hash) + UPPER_FIELD_NUMBER;
hash = (53 * hash) + getUpper().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.Range}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.Range)
org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Range_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Range_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
lower_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
upper_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Range_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.Range(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.lower_ = lower_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.upper_ = upper_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.Range)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance()) return this;
if (other.hasLower()) {
setLower(other.getLower());
}
if (other.hasUpper()) {
setUpper(other.getUpper());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString lower_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
@java.lang.Override
public boolean hasLower() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return The lower.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLower() {
return lower_;
}
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @param value The lower to set.
* @return This builder for chaining.
*/
public Builder setLower(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
lower_ = value;
onChanged();
return this;
}
/**
*
* The inclusive lower bound.
*
*
* optional bytes lower = 1 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearLower() {
bitField0_ = (bitField0_ & ~0x00000001);
lower_ = getDefaultInstance().getLower();
onChanged();
return this;
}
private com.google.protobuf.ByteString upper_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
@java.lang.Override
public boolean hasUpper() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return The upper.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUpper() {
return upper_;
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @param value The upper to set.
* @return This builder for chaining.
*/
public Builder setUpper(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
upper_ = value;
onChanged();
return this;
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 2 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearUpper() {
bitField0_ = (bitField0_ & ~0x00000002);
upper_ = getDefaultInstance().getUpper();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.Range)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.Range)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.Range DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.Range();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Range parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Range(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EqualityOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.Equality)
com.google.protobuf.MessageOrBuilder {
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return Whether the value field is set.
*/
boolean hasValue();
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return The value.
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.Equality}
*/
public static final class Equality extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.Equality)
EqualityOrBuilder {
private static final long serialVersionUID = 0L;
// Use Equality.newBuilder() to construct.
private Equality(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Equality() {
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Equality();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Equality(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
value_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Equality_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Equality_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder.class);
}
private int bitField0_;
public static final int VALUE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString value_;
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) obj;
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.Equality}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.Equality)
org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Equality_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Equality_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
value_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_Equality_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance()) return this;
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
value_ = value;
onChanged();
return this;
}
/**
*
* The inclusive lower bound. See comment in Range for notes on the
* encoding.
*
*
* optional bytes value = 1 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000001);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.Equality)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.Equality)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Equality parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Equality(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InListOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.InList)
com.google.protobuf.MessageOrBuilder {
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return A list containing the values.
*/
java.util.List getValuesList();
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return The count of values.
*/
int getValuesCount();
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param index The index of the element to return.
* @return The values at the given index.
*/
com.google.protobuf.ByteString getValues(int index);
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.InList}
*/
public static final class InList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.InList)
InListOrBuilder {
private static final long serialVersionUID = 0L;
// Use InList.newBuilder() to construct.
private InList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InList() {
values_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InList(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
values_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
values_.add(input.readBytes());
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder.class);
}
public static final int VALUES_FIELD_NUMBER = 1;
private java.util.List values_;
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return A list containing the values.
*/
@java.lang.Override
public java.util.List
getValuesList() {
return values_;
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return The count of values.
*/
public int getValuesCount() {
return values_.size();
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param index The index of the element to return.
* @return The values at the given index.
*/
public com.google.protobuf.ByteString getValues(int index) {
return values_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < values_.size(); i++) {
output.writeBytes(1, values_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < values_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(values_.get(i));
}
size += dataSize;
size += 1 * getValuesList().size();
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.InList)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) obj;
if (!getValuesList()
.equals(other.getValuesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getValuesCount() > 0) {
hash = (37 * hash) + VALUES_FIELD_NUMBER;
hash = (53 * hash) + getValuesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.InList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.InList)
org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InList_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.InList(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) != 0)) {
values_ = java.util.Collections.unmodifiableList(values_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.values_ = values_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.InList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance()) return this;
if (!other.values_.isEmpty()) {
if (values_.isEmpty()) {
values_ = other.values_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureValuesIsMutable();
values_.addAll(other.values_);
}
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List values_ = java.util.Collections.emptyList();
private void ensureValuesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
values_ = new java.util.ArrayList(values_);
bitField0_ |= 0x00000001;
}
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return A list containing the values.
*/
public java.util.List
getValuesList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(values_) : values_;
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return The count of values.
*/
public int getValuesCount() {
return values_.size();
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param index The index of the element to return.
* @return The values at the given index.
*/
public com.google.protobuf.ByteString getValues(int index) {
return values_.get(index);
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param index The index to set the value at.
* @param value The values to set.
* @return This builder for chaining.
*/
public Builder setValues(
int index, com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.set(index, value);
onChanged();
return this;
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param value The values to add.
* @return This builder for chaining.
*/
public Builder addValues(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureValuesIsMutable();
values_.add(value);
onChanged();
return this;
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @param values The values to add.
* @return This builder for chaining.
*/
public Builder addAllValues(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureValuesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, values_);
onChanged();
return this;
}
/**
*
* A list of values for the field. See comment in Range for notes on
* the encoding.
*
*
* repeated bytes values = 1 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearValues() {
values_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.InList)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.InList)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.InList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.InList();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InList(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IsNotNullOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.IsNotNull)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.IsNotNull}
*/
public static final class IsNotNull extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.IsNotNull)
IsNotNullOrBuilder {
private static final long serialVersionUID = 0L;
// Use IsNotNull.newBuilder() to construct.
private IsNotNull(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IsNotNull() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IsNotNull();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IsNotNull(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNotNull_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) obj;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.IsNotNull}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.IsNotNull)
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNotNull_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.IsNotNull)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.IsNotNull)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IsNotNull parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IsNotNull(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IsNullOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.IsNull)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.IsNull}
*/
public static final class IsNull extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.IsNull)
IsNullOrBuilder {
private static final long serialVersionUID = 0L;
// Use IsNull.newBuilder() to construct.
private IsNull(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IsNull() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IsNull();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IsNull(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNull_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNull_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) obj;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.IsNull}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.IsNull)
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNull_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNull_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_IsNull_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance()) return this;
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.IsNull)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.IsNull)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IsNull parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IsNull(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface InBloomFilterOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.ColumnPredicatePB.InBloomFilter)
com.google.protobuf.MessageOrBuilder {
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
java.util.List
getBloomFiltersList();
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB getBloomFilters(int index);
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
int getBloomFiltersCount();
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
java.util.List extends org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder>
getBloomFiltersOrBuilderList();
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder getBloomFiltersOrBuilder(
int index);
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
boolean hasLower();
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return The lower.
*/
com.google.protobuf.ByteString getLower();
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
boolean hasUpper();
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return The upper.
*/
com.google.protobuf.ByteString getUpper();
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.InBloomFilter}
*/
public static final class InBloomFilter extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.ColumnPredicatePB.InBloomFilter)
InBloomFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use InBloomFilter.newBuilder() to construct.
private InBloomFilter(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private InBloomFilter() {
bloomFilters_ = java.util.Collections.emptyList();
lower_ = com.google.protobuf.ByteString.EMPTY;
upper_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new InBloomFilter();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InBloomFilter(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
bloomFilters_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
bloomFilters_.add(
input.readMessage(org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.PARSER, extensionRegistry));
break;
}
case 18: {
bitField0_ |= 0x00000001;
lower_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000002;
upper_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
bloomFilters_ = java.util.Collections.unmodifiableList(bloomFilters_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InBloomFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder.class);
}
private int bitField0_;
public static final int BLOOM_FILTERS_FIELD_NUMBER = 1;
private java.util.List bloomFilters_;
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
@java.lang.Override
public java.util.List getBloomFiltersList() {
return bloomFilters_;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
@java.lang.Override
public java.util.List extends org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder>
getBloomFiltersOrBuilderList() {
return bloomFilters_;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
@java.lang.Override
public int getBloomFiltersCount() {
return bloomFilters_.size();
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
@java.lang.Override
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB getBloomFilters(int index) {
return bloomFilters_.get(index);
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
@java.lang.Override
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder getBloomFiltersOrBuilder(
int index) {
return bloomFilters_.get(index);
}
public static final int LOWER_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString lower_;
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
@java.lang.Override
public boolean hasLower() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return The lower.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLower() {
return lower_;
}
public static final int UPPER_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString upper_;
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
@java.lang.Override
public boolean hasUpper() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return The upper.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUpper() {
return upper_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < bloomFilters_.size(); i++) {
output.writeMessage(1, bloomFilters_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(2, lower_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(3, upper_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < bloomFilters_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, bloomFilters_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, lower_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, upper_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter other = (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) obj;
if (!getBloomFiltersList()
.equals(other.getBloomFiltersList())) return false;
if (hasLower() != other.hasLower()) return false;
if (hasLower()) {
if (!getLower()
.equals(other.getLower())) return false;
}
if (hasUpper() != other.hasUpper()) return false;
if (hasUpper()) {
if (!getUpper()
.equals(other.getUpper())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBloomFiltersCount() > 0) {
hash = (37 * hash) + BLOOM_FILTERS_FIELD_NUMBER;
hash = (53 * hash) + getBloomFiltersList().hashCode();
}
if (hasLower()) {
hash = (37 * hash) + LOWER_FIELD_NUMBER;
hash = (53 * hash) + getLower().hashCode();
}
if (hasUpper()) {
hash = (37 * hash) + UPPER_FIELD_NUMBER;
hash = (53 * hash) + getUpper().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.ColumnPredicatePB.InBloomFilter}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB.InBloomFilter)
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InBloomFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getBloomFiltersFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (bloomFiltersBuilder_ == null) {
bloomFilters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
bloomFiltersBuilder_.clear();
}
lower_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
upper_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter result = new org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (bloomFiltersBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
bloomFilters_ = java.util.Collections.unmodifiableList(bloomFilters_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.bloomFilters_ = bloomFilters_;
} else {
result.bloomFilters_ = bloomFiltersBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.lower_ = lower_;
if (((from_bitField0_ & 0x00000004) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.upper_ = upper_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance()) return this;
if (bloomFiltersBuilder_ == null) {
if (!other.bloomFilters_.isEmpty()) {
if (bloomFilters_.isEmpty()) {
bloomFilters_ = other.bloomFilters_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBloomFiltersIsMutable();
bloomFilters_.addAll(other.bloomFilters_);
}
onChanged();
}
} else {
if (!other.bloomFilters_.isEmpty()) {
if (bloomFiltersBuilder_.isEmpty()) {
bloomFiltersBuilder_.dispose();
bloomFiltersBuilder_ = null;
bloomFilters_ = other.bloomFilters_;
bitField0_ = (bitField0_ & ~0x00000001);
bloomFiltersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getBloomFiltersFieldBuilder() : null;
} else {
bloomFiltersBuilder_.addAllMessages(other.bloomFilters_);
}
}
}
if (other.hasLower()) {
setLower(other.getLower());
}
if (other.hasUpper()) {
setUpper(other.getUpper());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List bloomFilters_ =
java.util.Collections.emptyList();
private void ensureBloomFiltersIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
bloomFilters_ = new java.util.ArrayList(bloomFilters_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder> bloomFiltersBuilder_;
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public java.util.List getBloomFiltersList() {
if (bloomFiltersBuilder_ == null) {
return java.util.Collections.unmodifiableList(bloomFilters_);
} else {
return bloomFiltersBuilder_.getMessageList();
}
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public int getBloomFiltersCount() {
if (bloomFiltersBuilder_ == null) {
return bloomFilters_.size();
} else {
return bloomFiltersBuilder_.getCount();
}
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB getBloomFilters(int index) {
if (bloomFiltersBuilder_ == null) {
return bloomFilters_.get(index);
} else {
return bloomFiltersBuilder_.getMessage(index);
}
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder setBloomFilters(
int index, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB value) {
if (bloomFiltersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBloomFiltersIsMutable();
bloomFilters_.set(index, value);
onChanged();
} else {
bloomFiltersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder setBloomFilters(
int index, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder builderForValue) {
if (bloomFiltersBuilder_ == null) {
ensureBloomFiltersIsMutable();
bloomFilters_.set(index, builderForValue.build());
onChanged();
} else {
bloomFiltersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder addBloomFilters(org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB value) {
if (bloomFiltersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBloomFiltersIsMutable();
bloomFilters_.add(value);
onChanged();
} else {
bloomFiltersBuilder_.addMessage(value);
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder addBloomFilters(
int index, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB value) {
if (bloomFiltersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBloomFiltersIsMutable();
bloomFilters_.add(index, value);
onChanged();
} else {
bloomFiltersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder addBloomFilters(
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder builderForValue) {
if (bloomFiltersBuilder_ == null) {
ensureBloomFiltersIsMutable();
bloomFilters_.add(builderForValue.build());
onChanged();
} else {
bloomFiltersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder addBloomFilters(
int index, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder builderForValue) {
if (bloomFiltersBuilder_ == null) {
ensureBloomFiltersIsMutable();
bloomFilters_.add(index, builderForValue.build());
onChanged();
} else {
bloomFiltersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder addAllBloomFilters(
java.lang.Iterable extends org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB> values) {
if (bloomFiltersBuilder_ == null) {
ensureBloomFiltersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bloomFilters_);
onChanged();
} else {
bloomFiltersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder clearBloomFilters() {
if (bloomFiltersBuilder_ == null) {
bloomFilters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
bloomFiltersBuilder_.clear();
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public Builder removeBloomFilters(int index) {
if (bloomFiltersBuilder_ == null) {
ensureBloomFiltersIsMutable();
bloomFilters_.remove(index);
onChanged();
} else {
bloomFiltersBuilder_.remove(index);
}
return this;
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder getBloomFiltersBuilder(
int index) {
return getBloomFiltersFieldBuilder().getBuilder(index);
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder getBloomFiltersOrBuilder(
int index) {
if (bloomFiltersBuilder_ == null) {
return bloomFilters_.get(index); } else {
return bloomFiltersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public java.util.List extends org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder>
getBloomFiltersOrBuilderList() {
if (bloomFiltersBuilder_ != null) {
return bloomFiltersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(bloomFilters_);
}
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder addBloomFiltersBuilder() {
return getBloomFiltersFieldBuilder().addBuilder(
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.getDefaultInstance());
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder addBloomFiltersBuilder(
int index) {
return getBloomFiltersFieldBuilder().addBuilder(
index, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.getDefaultInstance());
}
/**
*
* A list of bloom filters for the field.
*
*
* repeated .kudu.BlockBloomFilterPB bloom_filters = 1;
*/
public java.util.List
getBloomFiltersBuilderList() {
return getBloomFiltersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder>
getBloomFiltersFieldBuilder() {
if (bloomFiltersBuilder_ == null) {
bloomFiltersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPB.Builder, org.apache.kudu.BlockBloomFilter.BlockBloomFilterPBOrBuilder>(
bloomFilters_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
bloomFilters_ = null;
}
return bloomFiltersBuilder_;
}
private com.google.protobuf.ByteString lower_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return Whether the lower field is set.
*/
@java.lang.Override
public boolean hasLower() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return The lower.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLower() {
return lower_;
}
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @param value The lower to set.
* @return This builder for chaining.
*/
public Builder setLower(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
lower_ = value;
onChanged();
return this;
}
/**
*
* lower and upper are optional for InBloomFilter.
* When using both InBloomFilter and Range predicate for the same column the
* merged result can be InBloomFilter within specified range.
* The inclusive lower bound.
*
*
* optional bytes lower = 2 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearLower() {
bitField0_ = (bitField0_ & ~0x00000002);
lower_ = getDefaultInstance().getLower();
onChanged();
return this;
}
private com.google.protobuf.ByteString upper_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return Whether the upper field is set.
*/
@java.lang.Override
public boolean hasUpper() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return The upper.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUpper() {
return upper_;
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @param value The upper to set.
* @return This builder for chaining.
*/
public Builder setUpper(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
upper_ = value;
onChanged();
return this;
}
/**
*
* The exclusive upper bound.
*
*
* optional bytes upper = 3 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearUpper() {
bitField0_ = (bitField0_ & ~0x00000004);
upper_ = getDefaultInstance().getUpper();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB.InBloomFilter)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB.InBloomFilter)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InBloomFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InBloomFilter(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int predicateCase_ = 0;
private java.lang.Object predicate_;
public enum PredicateCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
RANGE(2),
EQUALITY(3),
IS_NOT_NULL(4),
IN_LIST(5),
IS_NULL(6),
IN_BLOOM_FILTER(7),
PREDICATE_NOT_SET(0);
private final int value;
private PredicateCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PredicateCase valueOf(int value) {
return forNumber(value);
}
public static PredicateCase forNumber(int value) {
switch (value) {
case 2: return RANGE;
case 3: return EQUALITY;
case 4: return IS_NOT_NULL;
case 5: return IN_LIST;
case 6: return IS_NULL;
case 7: return IN_BLOOM_FILTER;
case 0: return PREDICATE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PredicateCase
getPredicateCase() {
return PredicateCase.forNumber(
predicateCase_);
}
public static final int COLUMN_FIELD_NUMBER = 1;
private volatile java.lang.Object column_;
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return Whether the column field is set.
*/
@java.lang.Override
public boolean hasColumn() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The column.
*/
@java.lang.Override
public java.lang.String getColumn() {
java.lang.Object ref = column_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
column_ = s;
}
return s;
}
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The bytes for column.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getColumnBytes() {
java.lang.Object ref = column_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
column_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RANGE_FIELD_NUMBER = 2;
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return Whether the range field is set.
*/
@java.lang.Override
public boolean hasRange() {
return predicateCase_ == 2;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return The range.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getRange() {
if (predicateCase_ == 2) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder getRangeOrBuilder() {
if (predicateCase_ == 2) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
public static final int EQUALITY_FIELD_NUMBER = 3;
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return Whether the equality field is set.
*/
@java.lang.Override
public boolean hasEquality() {
return predicateCase_ == 3;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return The equality.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getEquality() {
if (predicateCase_ == 3) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder getEqualityOrBuilder() {
if (predicateCase_ == 3) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
public static final int IS_NOT_NULL_FIELD_NUMBER = 4;
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return Whether the isNotNull field is set.
*/
@java.lang.Override
public boolean hasIsNotNull() {
return predicateCase_ == 4;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return The isNotNull.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getIsNotNull() {
if (predicateCase_ == 4) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder getIsNotNullOrBuilder() {
if (predicateCase_ == 4) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
public static final int IN_LIST_FIELD_NUMBER = 5;
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return Whether the inList field is set.
*/
@java.lang.Override
public boolean hasInList() {
return predicateCase_ == 5;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return The inList.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getInList() {
if (predicateCase_ == 5) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder getInListOrBuilder() {
if (predicateCase_ == 5) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
public static final int IS_NULL_FIELD_NUMBER = 6;
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return Whether the isNull field is set.
*/
@java.lang.Override
public boolean hasIsNull() {
return predicateCase_ == 6;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return The isNull.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getIsNull() {
if (predicateCase_ == 6) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder getIsNullOrBuilder() {
if (predicateCase_ == 6) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
public static final int IN_BLOOM_FILTER_FIELD_NUMBER = 7;
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return Whether the inBloomFilter field is set.
*/
@java.lang.Override
public boolean hasInBloomFilter() {
return predicateCase_ == 7;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return The inBloomFilter.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getInBloomFilter() {
if (predicateCase_ == 7) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder getInBloomFilterOrBuilder() {
if (predicateCase_ == 7) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, column_);
}
if (predicateCase_ == 2) {
output.writeMessage(2, (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_);
}
if (predicateCase_ == 3) {
output.writeMessage(3, (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_);
}
if (predicateCase_ == 4) {
output.writeMessage(4, (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_);
}
if (predicateCase_ == 5) {
output.writeMessage(5, (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_);
}
if (predicateCase_ == 6) {
output.writeMessage(6, (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_);
}
if (predicateCase_ == 7) {
output.writeMessage(7, (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, column_);
}
if (predicateCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_);
}
if (predicateCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_);
}
if (predicateCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_);
}
if (predicateCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_);
}
if (predicateCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_);
}
if (predicateCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.ColumnPredicatePB other = (org.apache.kudu.KuduCommon.ColumnPredicatePB) obj;
if (hasColumn() != other.hasColumn()) return false;
if (hasColumn()) {
if (!getColumn()
.equals(other.getColumn())) return false;
}
if (!getPredicateCase().equals(other.getPredicateCase())) return false;
switch (predicateCase_) {
case 2:
if (!getRange()
.equals(other.getRange())) return false;
break;
case 3:
if (!getEquality()
.equals(other.getEquality())) return false;
break;
case 4:
if (!getIsNotNull()
.equals(other.getIsNotNull())) return false;
break;
case 5:
if (!getInList()
.equals(other.getInList())) return false;
break;
case 6:
if (!getIsNull()
.equals(other.getIsNull())) return false;
break;
case 7:
if (!getInBloomFilter()
.equals(other.getInBloomFilter())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasColumn()) {
hash = (37 * hash) + COLUMN_FIELD_NUMBER;
hash = (53 * hash) + getColumn().hashCode();
}
switch (predicateCase_) {
case 2:
hash = (37 * hash) + RANGE_FIELD_NUMBER;
hash = (53 * hash) + getRange().hashCode();
break;
case 3:
hash = (37 * hash) + EQUALITY_FIELD_NUMBER;
hash = (53 * hash) + getEquality().hashCode();
break;
case 4:
hash = (37 * hash) + IS_NOT_NULL_FIELD_NUMBER;
hash = (53 * hash) + getIsNotNull().hashCode();
break;
case 5:
hash = (37 * hash) + IN_LIST_FIELD_NUMBER;
hash = (53 * hash) + getInList().hashCode();
break;
case 6:
hash = (37 * hash) + IS_NULL_FIELD_NUMBER;
hash = (53 * hash) + getIsNull().hashCode();
break;
case 7:
hash = (37 * hash) + IN_BLOOM_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getInBloomFilter().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.ColumnPredicatePB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A predicate that can be applied on a Kudu column.
*
*
* Protobuf type {@code kudu.ColumnPredicatePB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.ColumnPredicatePB)
org.apache.kudu.KuduCommon.ColumnPredicatePBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.ColumnPredicatePB.class, org.apache.kudu.KuduCommon.ColumnPredicatePB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.ColumnPredicatePB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
column_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
predicateCase_ = 0;
predicate_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_ColumnPredicatePB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.ColumnPredicatePB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB build() {
org.apache.kudu.KuduCommon.ColumnPredicatePB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB buildPartial() {
org.apache.kudu.KuduCommon.ColumnPredicatePB result = new org.apache.kudu.KuduCommon.ColumnPredicatePB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.column_ = column_;
if (predicateCase_ == 2) {
if (rangeBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = rangeBuilder_.build();
}
}
if (predicateCase_ == 3) {
if (equalityBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = equalityBuilder_.build();
}
}
if (predicateCase_ == 4) {
if (isNotNullBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = isNotNullBuilder_.build();
}
}
if (predicateCase_ == 5) {
if (inListBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = inListBuilder_.build();
}
}
if (predicateCase_ == 6) {
if (isNullBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = isNullBuilder_.build();
}
}
if (predicateCase_ == 7) {
if (inBloomFilterBuilder_ == null) {
result.predicate_ = predicate_;
} else {
result.predicate_ = inBloomFilterBuilder_.build();
}
}
result.bitField0_ = to_bitField0_;
result.predicateCase_ = predicateCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.ColumnPredicatePB) {
return mergeFrom((org.apache.kudu.KuduCommon.ColumnPredicatePB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.ColumnPredicatePB other) {
if (other == org.apache.kudu.KuduCommon.ColumnPredicatePB.getDefaultInstance()) return this;
if (other.hasColumn()) {
bitField0_ |= 0x00000001;
column_ = other.column_;
onChanged();
}
switch (other.getPredicateCase()) {
case RANGE: {
mergeRange(other.getRange());
break;
}
case EQUALITY: {
mergeEquality(other.getEquality());
break;
}
case IS_NOT_NULL: {
mergeIsNotNull(other.getIsNotNull());
break;
}
case IN_LIST: {
mergeInList(other.getInList());
break;
}
case IS_NULL: {
mergeIsNull(other.getIsNull());
break;
}
case IN_BLOOM_FILTER: {
mergeInBloomFilter(other.getInBloomFilter());
break;
}
case PREDICATE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.ColumnPredicatePB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.ColumnPredicatePB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int predicateCase_ = 0;
private java.lang.Object predicate_;
public PredicateCase
getPredicateCase() {
return PredicateCase.forNumber(
predicateCase_);
}
public Builder clearPredicate() {
predicateCase_ = 0;
predicate_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object column_ = "";
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return Whether the column field is set.
*/
public boolean hasColumn() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The column.
*/
public java.lang.String getColumn() {
java.lang.Object ref = column_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
column_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return The bytes for column.
*/
public com.google.protobuf.ByteString
getColumnBytes() {
java.lang.Object ref = column_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
column_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @param value The column to set.
* @return This builder for chaining.
*/
public Builder setColumn(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
column_ = value;
onChanged();
return this;
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @return This builder for chaining.
*/
public Builder clearColumn() {
bitField0_ = (bitField0_ & ~0x00000001);
column_ = getDefaultInstance().getColumn();
onChanged();
return this;
}
/**
*
* The predicate column name.
*
*
* optional string column = 1;
* @param value The bytes for column to set.
* @return This builder for chaining.
*/
public Builder setColumnBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
column_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range, org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder> rangeBuilder_;
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return Whether the range field is set.
*/
@java.lang.Override
public boolean hasRange() {
return predicateCase_ == 2;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
* @return The range.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range getRange() {
if (rangeBuilder_ == null) {
if (predicateCase_ == 2) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
} else {
if (predicateCase_ == 2) {
return rangeBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
public Builder setRange(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range value) {
if (rangeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
rangeBuilder_.setMessage(value);
}
predicateCase_ = 2;
return this;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
public Builder setRange(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder builderForValue) {
if (rangeBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
rangeBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 2;
return this;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
public Builder mergeRange(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range value) {
if (rangeBuilder_ == null) {
if (predicateCase_ == 2 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 2) {
rangeBuilder_.mergeFrom(value);
}
rangeBuilder_.setMessage(value);
}
predicateCase_ = 2;
return this;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
public Builder clearRange() {
if (rangeBuilder_ == null) {
if (predicateCase_ == 2) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 2) {
predicateCase_ = 0;
predicate_ = null;
}
rangeBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder getRangeBuilder() {
return getRangeFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder getRangeOrBuilder() {
if ((predicateCase_ == 2) && (rangeBuilder_ != null)) {
return rangeBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 2) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.Range range = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range, org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder>
getRangeFieldBuilder() {
if (rangeBuilder_ == null) {
if (!(predicateCase_ == 2)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.getDefaultInstance();
}
rangeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Range, org.apache.kudu.KuduCommon.ColumnPredicatePB.Range.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.RangeOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.Range) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 2;
onChanged();;
return rangeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality, org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder> equalityBuilder_;
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return Whether the equality field is set.
*/
@java.lang.Override
public boolean hasEquality() {
return predicateCase_ == 3;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
* @return The equality.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality getEquality() {
if (equalityBuilder_ == null) {
if (predicateCase_ == 3) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
} else {
if (predicateCase_ == 3) {
return equalityBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
public Builder setEquality(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality value) {
if (equalityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
equalityBuilder_.setMessage(value);
}
predicateCase_ = 3;
return this;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
public Builder setEquality(
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder builderForValue) {
if (equalityBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
equalityBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 3;
return this;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
public Builder mergeEquality(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality value) {
if (equalityBuilder_ == null) {
if (predicateCase_ == 3 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 3) {
equalityBuilder_.mergeFrom(value);
}
equalityBuilder_.setMessage(value);
}
predicateCase_ = 3;
return this;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
public Builder clearEquality() {
if (equalityBuilder_ == null) {
if (predicateCase_ == 3) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 3) {
predicateCase_ = 0;
predicate_ = null;
}
equalityBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder getEqualityBuilder() {
return getEqualityFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder getEqualityOrBuilder() {
if ((predicateCase_ == 3) && (equalityBuilder_ != null)) {
return equalityBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 3) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.Equality equality = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality, org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder>
getEqualityFieldBuilder() {
if (equalityBuilder_ == null) {
if (!(predicateCase_ == 3)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.getDefaultInstance();
}
equalityBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality, org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.EqualityOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.Equality) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 3;
onChanged();;
return equalityBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder> isNotNullBuilder_;
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return Whether the isNotNull field is set.
*/
@java.lang.Override
public boolean hasIsNotNull() {
return predicateCase_ == 4;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
* @return The isNotNull.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull getIsNotNull() {
if (isNotNullBuilder_ == null) {
if (predicateCase_ == 4) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
} else {
if (predicateCase_ == 4) {
return isNotNullBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
public Builder setIsNotNull(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull value) {
if (isNotNullBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
isNotNullBuilder_.setMessage(value);
}
predicateCase_ = 4;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
public Builder setIsNotNull(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder builderForValue) {
if (isNotNullBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
isNotNullBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 4;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
public Builder mergeIsNotNull(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull value) {
if (isNotNullBuilder_ == null) {
if (predicateCase_ == 4 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 4) {
isNotNullBuilder_.mergeFrom(value);
}
isNotNullBuilder_.setMessage(value);
}
predicateCase_ = 4;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
public Builder clearIsNotNull() {
if (isNotNullBuilder_ == null) {
if (predicateCase_ == 4) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 4) {
predicateCase_ = 0;
predicate_ = null;
}
isNotNullBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder getIsNotNullBuilder() {
return getIsNotNullFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder getIsNotNullOrBuilder() {
if ((predicateCase_ == 4) && (isNotNullBuilder_ != null)) {
return isNotNullBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 4) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.IsNotNull is_not_null = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder>
getIsNotNullFieldBuilder() {
if (isNotNullBuilder_ == null) {
if (!(predicateCase_ == 4)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.getDefaultInstance();
}
isNotNullBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNullOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNotNull) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 4;
onChanged();;
return isNotNullBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList, org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder> inListBuilder_;
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return Whether the inList field is set.
*/
@java.lang.Override
public boolean hasInList() {
return predicateCase_ == 5;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
* @return The inList.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList getInList() {
if (inListBuilder_ == null) {
if (predicateCase_ == 5) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
} else {
if (predicateCase_ == 5) {
return inListBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
public Builder setInList(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList value) {
if (inListBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
inListBuilder_.setMessage(value);
}
predicateCase_ = 5;
return this;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
public Builder setInList(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder builderForValue) {
if (inListBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
inListBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 5;
return this;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
public Builder mergeInList(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList value) {
if (inListBuilder_ == null) {
if (predicateCase_ == 5 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 5) {
inListBuilder_.mergeFrom(value);
}
inListBuilder_.setMessage(value);
}
predicateCase_ = 5;
return this;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
public Builder clearInList() {
if (inListBuilder_ == null) {
if (predicateCase_ == 5) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 5) {
predicateCase_ = 0;
predicate_ = null;
}
inListBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder getInListBuilder() {
return getInListFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder getInListOrBuilder() {
if ((predicateCase_ == 5) && (inListBuilder_ != null)) {
return inListBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 5) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.InList in_list = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList, org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder>
getInListFieldBuilder() {
if (inListBuilder_ == null) {
if (!(predicateCase_ == 5)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.getDefaultInstance();
}
inListBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InList, org.apache.kudu.KuduCommon.ColumnPredicatePB.InList.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InListOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.InList) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 5;
onChanged();;
return inListBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder> isNullBuilder_;
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return Whether the isNull field is set.
*/
@java.lang.Override
public boolean hasIsNull() {
return predicateCase_ == 6;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
* @return The isNull.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull getIsNull() {
if (isNullBuilder_ == null) {
if (predicateCase_ == 6) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
} else {
if (predicateCase_ == 6) {
return isNullBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
public Builder setIsNull(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull value) {
if (isNullBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
isNullBuilder_.setMessage(value);
}
predicateCase_ = 6;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
public Builder setIsNull(
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder builderForValue) {
if (isNullBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
isNullBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 6;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
public Builder mergeIsNull(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull value) {
if (isNullBuilder_ == null) {
if (predicateCase_ == 6 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 6) {
isNullBuilder_.mergeFrom(value);
}
isNullBuilder_.setMessage(value);
}
predicateCase_ = 6;
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
public Builder clearIsNull() {
if (isNullBuilder_ == null) {
if (predicateCase_ == 6) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 6) {
predicateCase_ = 0;
predicate_ = null;
}
isNullBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder getIsNullBuilder() {
return getIsNullFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder getIsNullOrBuilder() {
if ((predicateCase_ == 6) && (isNullBuilder_ != null)) {
return isNullBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 6) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.IsNull is_null = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder>
getIsNullFieldBuilder() {
if (isNullBuilder_ == null) {
if (!(predicateCase_ == 6)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.getDefaultInstance();
}
isNullBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNullOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.IsNull) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 6;
onChanged();;
return isNullBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder> inBloomFilterBuilder_;
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return Whether the inBloomFilter field is set.
*/
@java.lang.Override
public boolean hasInBloomFilter() {
return predicateCase_ == 7;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
* @return The inBloomFilter.
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter getInBloomFilter() {
if (inBloomFilterBuilder_ == null) {
if (predicateCase_ == 7) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
} else {
if (predicateCase_ == 7) {
return inBloomFilterBuilder_.getMessage();
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
public Builder setInBloomFilter(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter value) {
if (inBloomFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
predicate_ = value;
onChanged();
} else {
inBloomFilterBuilder_.setMessage(value);
}
predicateCase_ = 7;
return this;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
public Builder setInBloomFilter(
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder builderForValue) {
if (inBloomFilterBuilder_ == null) {
predicate_ = builderForValue.build();
onChanged();
} else {
inBloomFilterBuilder_.setMessage(builderForValue.build());
}
predicateCase_ = 7;
return this;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
public Builder mergeInBloomFilter(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter value) {
if (inBloomFilterBuilder_ == null) {
if (predicateCase_ == 7 &&
predicate_ != org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance()) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.newBuilder((org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_)
.mergeFrom(value).buildPartial();
} else {
predicate_ = value;
}
onChanged();
} else {
if (predicateCase_ == 7) {
inBloomFilterBuilder_.mergeFrom(value);
}
inBloomFilterBuilder_.setMessage(value);
}
predicateCase_ = 7;
return this;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
public Builder clearInBloomFilter() {
if (inBloomFilterBuilder_ == null) {
if (predicateCase_ == 7) {
predicateCase_ = 0;
predicate_ = null;
onChanged();
}
} else {
if (predicateCase_ == 7) {
predicateCase_ = 0;
predicate_ = null;
}
inBloomFilterBuilder_.clear();
}
return this;
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder getInBloomFilterBuilder() {
return getInBloomFilterFieldBuilder().getBuilder();
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder getInBloomFilterOrBuilder() {
if ((predicateCase_ == 7) && (inBloomFilterBuilder_ != null)) {
return inBloomFilterBuilder_.getMessageOrBuilder();
} else {
if (predicateCase_ == 7) {
return (org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_;
}
return org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
}
/**
* .kudu.ColumnPredicatePB.InBloomFilter in_bloom_filter = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder>
getInBloomFilterFieldBuilder() {
if (inBloomFilterBuilder_ == null) {
if (!(predicateCase_ == 7)) {
predicate_ = org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.getDefaultInstance();
}
inBloomFilterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter.Builder, org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilterOrBuilder>(
(org.apache.kudu.KuduCommon.ColumnPredicatePB.InBloomFilter) predicate_,
getParentForChildren(),
isClean());
predicate_ = null;
}
predicateCase_ = 7;
onChanged();;
return inBloomFilterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.ColumnPredicatePB)
}
// @@protoc_insertion_point(class_scope:kudu.ColumnPredicatePB)
private static final org.apache.kudu.KuduCommon.ColumnPredicatePB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.ColumnPredicatePB();
}
public static org.apache.kudu.KuduCommon.ColumnPredicatePB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ColumnPredicatePB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ColumnPredicatePB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.ColumnPredicatePB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KeyRangePBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.KeyRangePB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return Whether the startPrimaryKey field is set.
*/
boolean hasStartPrimaryKey();
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return The startPrimaryKey.
*/
com.google.protobuf.ByteString getStartPrimaryKey();
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return Whether the stopPrimaryKey field is set.
*/
boolean hasStopPrimaryKey();
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return The stopPrimaryKey.
*/
com.google.protobuf.ByteString getStopPrimaryKey();
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return Whether the sizeBytesEstimates field is set.
*/
boolean hasSizeBytesEstimates();
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return The sizeBytesEstimates.
*/
long getSizeBytesEstimates();
}
/**
*
* The primary key range of a Kudu tablet.
*
*
* Protobuf type {@code kudu.KeyRangePB}
*/
public static final class KeyRangePB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.KeyRangePB)
KeyRangePBOrBuilder {
private static final long serialVersionUID = 0L;
// Use KeyRangePB.newBuilder() to construct.
private KeyRangePB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private KeyRangePB() {
startPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
stopPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new KeyRangePB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private KeyRangePB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
startPrimaryKey_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
stopPrimaryKey_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
sizeBytesEstimates_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_KeyRangePB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_KeyRangePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.KeyRangePB.class, org.apache.kudu.KuduCommon.KeyRangePB.Builder.class);
}
private int bitField0_;
public static final int START_PRIMARY_KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString startPrimaryKey_;
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return Whether the startPrimaryKey field is set.
*/
@java.lang.Override
public boolean hasStartPrimaryKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return The startPrimaryKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStartPrimaryKey() {
return startPrimaryKey_;
}
public static final int STOP_PRIMARY_KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString stopPrimaryKey_;
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return Whether the stopPrimaryKey field is set.
*/
@java.lang.Override
public boolean hasStopPrimaryKey() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return The stopPrimaryKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStopPrimaryKey() {
return stopPrimaryKey_;
}
public static final int SIZE_BYTES_ESTIMATES_FIELD_NUMBER = 3;
private long sizeBytesEstimates_;
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return Whether the sizeBytesEstimates field is set.
*/
@java.lang.Override
public boolean hasSizeBytesEstimates() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return The sizeBytesEstimates.
*/
@java.lang.Override
public long getSizeBytesEstimates() {
return sizeBytesEstimates_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasSizeBytesEstimates()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, startPrimaryKey_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, stopPrimaryKey_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeUInt64(3, sizeBytesEstimates_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, startPrimaryKey_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, stopPrimaryKey_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, sizeBytesEstimates_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.KeyRangePB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.KeyRangePB other = (org.apache.kudu.KuduCommon.KeyRangePB) obj;
if (hasStartPrimaryKey() != other.hasStartPrimaryKey()) return false;
if (hasStartPrimaryKey()) {
if (!getStartPrimaryKey()
.equals(other.getStartPrimaryKey())) return false;
}
if (hasStopPrimaryKey() != other.hasStopPrimaryKey()) return false;
if (hasStopPrimaryKey()) {
if (!getStopPrimaryKey()
.equals(other.getStopPrimaryKey())) return false;
}
if (hasSizeBytesEstimates() != other.hasSizeBytesEstimates()) return false;
if (hasSizeBytesEstimates()) {
if (getSizeBytesEstimates()
!= other.getSizeBytesEstimates()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStartPrimaryKey()) {
hash = (37 * hash) + START_PRIMARY_KEY_FIELD_NUMBER;
hash = (53 * hash) + getStartPrimaryKey().hashCode();
}
if (hasStopPrimaryKey()) {
hash = (37 * hash) + STOP_PRIMARY_KEY_FIELD_NUMBER;
hash = (53 * hash) + getStopPrimaryKey().hashCode();
}
if (hasSizeBytesEstimates()) {
hash = (37 * hash) + SIZE_BYTES_ESTIMATES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSizeBytesEstimates());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.KeyRangePB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.KeyRangePB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The primary key range of a Kudu tablet.
*
*
* Protobuf type {@code kudu.KeyRangePB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.KeyRangePB)
org.apache.kudu.KuduCommon.KeyRangePBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_KeyRangePB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_KeyRangePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.KeyRangePB.class, org.apache.kudu.KuduCommon.KeyRangePB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.KeyRangePB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
startPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
stopPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
sizeBytesEstimates_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_KeyRangePB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.KeyRangePB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.KeyRangePB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.KeyRangePB build() {
org.apache.kudu.KuduCommon.KeyRangePB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.KeyRangePB buildPartial() {
org.apache.kudu.KuduCommon.KeyRangePB result = new org.apache.kudu.KuduCommon.KeyRangePB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
to_bitField0_ |= 0x00000001;
}
result.startPrimaryKey_ = startPrimaryKey_;
if (((from_bitField0_ & 0x00000002) != 0)) {
to_bitField0_ |= 0x00000002;
}
result.stopPrimaryKey_ = stopPrimaryKey_;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.sizeBytesEstimates_ = sizeBytesEstimates_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.KeyRangePB) {
return mergeFrom((org.apache.kudu.KuduCommon.KeyRangePB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.KeyRangePB other) {
if (other == org.apache.kudu.KuduCommon.KeyRangePB.getDefaultInstance()) return this;
if (other.hasStartPrimaryKey()) {
setStartPrimaryKey(other.getStartPrimaryKey());
}
if (other.hasStopPrimaryKey()) {
setStopPrimaryKey(other.getStopPrimaryKey());
}
if (other.hasSizeBytesEstimates()) {
setSizeBytesEstimates(other.getSizeBytesEstimates());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasSizeBytesEstimates()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.KeyRangePB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.KeyRangePB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString startPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return Whether the startPrimaryKey field is set.
*/
@java.lang.Override
public boolean hasStartPrimaryKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return The startPrimaryKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStartPrimaryKey() {
return startPrimaryKey_;
}
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @param value The startPrimaryKey to set.
* @return This builder for chaining.
*/
public Builder setStartPrimaryKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
startPrimaryKey_ = value;
onChanged();
return this;
}
/**
*
* Encoded primary key to begin scanning at (inclusive).
*
*
* optional bytes start_primary_key = 1 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearStartPrimaryKey() {
bitField0_ = (bitField0_ & ~0x00000001);
startPrimaryKey_ = getDefaultInstance().getStartPrimaryKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString stopPrimaryKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return Whether the stopPrimaryKey field is set.
*/
@java.lang.Override
public boolean hasStopPrimaryKey() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return The stopPrimaryKey.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStopPrimaryKey() {
return stopPrimaryKey_;
}
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @param value The stopPrimaryKey to set.
* @return This builder for chaining.
*/
public Builder setStopPrimaryKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
stopPrimaryKey_ = value;
onChanged();
return this;
}
/**
*
* Encoded primary key to stop scanning at (exclusive).
*
*
* optional bytes stop_primary_key = 2 [(.kudu.REDACT) = true];
* @return This builder for chaining.
*/
public Builder clearStopPrimaryKey() {
bitField0_ = (bitField0_ & ~0x00000002);
stopPrimaryKey_ = getDefaultInstance().getStopPrimaryKey();
onChanged();
return this;
}
private long sizeBytesEstimates_ ;
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return Whether the sizeBytesEstimates field is set.
*/
@java.lang.Override
public boolean hasSizeBytesEstimates() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return The sizeBytesEstimates.
*/
@java.lang.Override
public long getSizeBytesEstimates() {
return sizeBytesEstimates_;
}
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @param value The sizeBytesEstimates to set.
* @return This builder for chaining.
*/
public Builder setSizeBytesEstimates(long value) {
bitField0_ |= 0x00000004;
sizeBytesEstimates_ = value;
onChanged();
return this;
}
/**
*
* Number of bytes in chunk.
*
*
* required uint64 size_bytes_estimates = 3;
* @return This builder for chaining.
*/
public Builder clearSizeBytesEstimates() {
bitField0_ = (bitField0_ & ~0x00000004);
sizeBytesEstimates_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.KeyRangePB)
}
// @@protoc_insertion_point(class_scope:kudu.KeyRangePB)
private static final org.apache.kudu.KuduCommon.KeyRangePB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.KeyRangePB();
}
public static org.apache.kudu.KuduCommon.KeyRangePB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public KeyRangePB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new KeyRangePB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.KeyRangePB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TableExtraConfigPBOrBuilder extends
// @@protoc_insertion_point(interface_extends:kudu.TableExtraConfigPB)
com.google.protobuf.MessageOrBuilder {
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return Whether the historyMaxAgeSec field is set.
*/
boolean hasHistoryMaxAgeSec();
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return The historyMaxAgeSec.
*/
int getHistoryMaxAgeSec();
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return Whether the maintenancePriority field is set.
*/
boolean hasMaintenancePriority();
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return The maintenancePriority.
*/
int getMaintenancePriority();
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return Whether the disableCompaction field is set.
*/
boolean hasDisableCompaction();
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return The disableCompaction.
*/
boolean getDisableCompaction();
}
/**
* Protobuf type {@code kudu.TableExtraConfigPB}
*/
public static final class TableExtraConfigPB extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:kudu.TableExtraConfigPB)
TableExtraConfigPBOrBuilder {
private static final long serialVersionUID = 0L;
// Use TableExtraConfigPB.newBuilder() to construct.
private TableExtraConfigPB(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TableExtraConfigPB() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TableExtraConfigPB();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TableExtraConfigPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
historyMaxAgeSec_ = input.readInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
maintenancePriority_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
disableCompaction_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_TableExtraConfigPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_TableExtraConfigPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.TableExtraConfigPB.class, org.apache.kudu.KuduCommon.TableExtraConfigPB.Builder.class);
}
private int bitField0_;
public static final int HISTORY_MAX_AGE_SEC_FIELD_NUMBER = 1;
private int historyMaxAgeSec_;
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return Whether the historyMaxAgeSec field is set.
*/
@java.lang.Override
public boolean hasHistoryMaxAgeSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return The historyMaxAgeSec.
*/
@java.lang.Override
public int getHistoryMaxAgeSec() {
return historyMaxAgeSec_;
}
public static final int MAINTENANCE_PRIORITY_FIELD_NUMBER = 2;
private int maintenancePriority_;
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return Whether the maintenancePriority field is set.
*/
@java.lang.Override
public boolean hasMaintenancePriority() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return The maintenancePriority.
*/
@java.lang.Override
public int getMaintenancePriority() {
return maintenancePriority_;
}
public static final int DISABLE_COMPACTION_FIELD_NUMBER = 3;
private boolean disableCompaction_;
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return Whether the disableCompaction field is set.
*/
@java.lang.Override
public boolean hasDisableCompaction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return The disableCompaction.
*/
@java.lang.Override
public boolean getDisableCompaction() {
return disableCompaction_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, historyMaxAgeSec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, maintenancePriority_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, disableCompaction_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, historyMaxAgeSec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, maintenancePriority_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, disableCompaction_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.apache.kudu.KuduCommon.TableExtraConfigPB)) {
return super.equals(obj);
}
org.apache.kudu.KuduCommon.TableExtraConfigPB other = (org.apache.kudu.KuduCommon.TableExtraConfigPB) obj;
if (hasHistoryMaxAgeSec() != other.hasHistoryMaxAgeSec()) return false;
if (hasHistoryMaxAgeSec()) {
if (getHistoryMaxAgeSec()
!= other.getHistoryMaxAgeSec()) return false;
}
if (hasMaintenancePriority() != other.hasMaintenancePriority()) return false;
if (hasMaintenancePriority()) {
if (getMaintenancePriority()
!= other.getMaintenancePriority()) return false;
}
if (hasDisableCompaction() != other.hasDisableCompaction()) return false;
if (hasDisableCompaction()) {
if (getDisableCompaction()
!= other.getDisableCompaction()) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHistoryMaxAgeSec()) {
hash = (37 * hash) + HISTORY_MAX_AGE_SEC_FIELD_NUMBER;
hash = (53 * hash) + getHistoryMaxAgeSec();
}
if (hasMaintenancePriority()) {
hash = (37 * hash) + MAINTENANCE_PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getMaintenancePriority();
}
if (hasDisableCompaction()) {
hash = (37 * hash) + DISABLE_COMPACTION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDisableCompaction());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.apache.kudu.KuduCommon.TableExtraConfigPB prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code kudu.TableExtraConfigPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:kudu.TableExtraConfigPB)
org.apache.kudu.KuduCommon.TableExtraConfigPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.apache.kudu.KuduCommon.internal_static_kudu_TableExtraConfigPB_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.apache.kudu.KuduCommon.internal_static_kudu_TableExtraConfigPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.apache.kudu.KuduCommon.TableExtraConfigPB.class, org.apache.kudu.KuduCommon.TableExtraConfigPB.Builder.class);
}
// Construct using org.apache.kudu.KuduCommon.TableExtraConfigPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
historyMaxAgeSec_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
maintenancePriority_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
disableCompaction_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.apache.kudu.KuduCommon.internal_static_kudu_TableExtraConfigPB_descriptor;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.TableExtraConfigPB getDefaultInstanceForType() {
return org.apache.kudu.KuduCommon.TableExtraConfigPB.getDefaultInstance();
}
@java.lang.Override
public org.apache.kudu.KuduCommon.TableExtraConfigPB build() {
org.apache.kudu.KuduCommon.TableExtraConfigPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.TableExtraConfigPB buildPartial() {
org.apache.kudu.KuduCommon.TableExtraConfigPB result = new org.apache.kudu.KuduCommon.TableExtraConfigPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.historyMaxAgeSec_ = historyMaxAgeSec_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.maintenancePriority_ = maintenancePriority_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.disableCompaction_ = disableCompaction_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.apache.kudu.KuduCommon.TableExtraConfigPB) {
return mergeFrom((org.apache.kudu.KuduCommon.TableExtraConfigPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.apache.kudu.KuduCommon.TableExtraConfigPB other) {
if (other == org.apache.kudu.KuduCommon.TableExtraConfigPB.getDefaultInstance()) return this;
if (other.hasHistoryMaxAgeSec()) {
setHistoryMaxAgeSec(other.getHistoryMaxAgeSec());
}
if (other.hasMaintenancePriority()) {
setMaintenancePriority(other.getMaintenancePriority());
}
if (other.hasDisableCompaction()) {
setDisableCompaction(other.getDisableCompaction());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.apache.kudu.KuduCommon.TableExtraConfigPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.apache.kudu.KuduCommon.TableExtraConfigPB) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int historyMaxAgeSec_ ;
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return Whether the historyMaxAgeSec field is set.
*/
@java.lang.Override
public boolean hasHistoryMaxAgeSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return The historyMaxAgeSec.
*/
@java.lang.Override
public int getHistoryMaxAgeSec() {
return historyMaxAgeSec_;
}
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @param value The historyMaxAgeSec to set.
* @return This builder for chaining.
*/
public Builder setHistoryMaxAgeSec(int value) {
bitField0_ |= 0x00000001;
historyMaxAgeSec_ = value;
onChanged();
return this;
}
/**
*
* Number of seconds to retain history for tablets in this table,
* including history required to perform diff scans and incremental
* backups. Reads initiated at a snapshot that is older than this
* age will be rejected. Equivalent to --tablet_history_max_age_sec.
*
*
* optional int32 history_max_age_sec = 1;
* @return This builder for chaining.
*/
public Builder clearHistoryMaxAgeSec() {
bitField0_ = (bitField0_ & ~0x00000001);
historyMaxAgeSec_ = 0;
onChanged();
return this;
}
private int maintenancePriority_ ;
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return Whether the maintenancePriority field is set.
*/
@java.lang.Override
public boolean hasMaintenancePriority() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return The maintenancePriority.
*/
@java.lang.Override
public int getMaintenancePriority() {
return maintenancePriority_;
}
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @param value The maintenancePriority to set.
* @return This builder for chaining.
*/
public Builder setMaintenancePriority(int value) {
bitField0_ |= 0x00000002;
maintenancePriority_ = value;
onChanged();
return this;
}
/**
*
* Priority level of a table for maintenance, it will be clamped into
* range [-FLAGS_max_priority_range, FLAGS_max_priority_range] when
* calculate maintenance priority score.
*
*
* optional int32 maintenance_priority = 2;
* @return This builder for chaining.
*/
public Builder clearMaintenancePriority() {
bitField0_ = (bitField0_ & ~0x00000002);
maintenancePriority_ = 0;
onChanged();
return this;
}
private boolean disableCompaction_ ;
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return Whether the disableCompaction field is set.
*/
@java.lang.Override
public boolean hasDisableCompaction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return The disableCompaction.
*/
@java.lang.Override
public boolean getDisableCompaction() {
return disableCompaction_;
}
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @param value The disableCompaction to set.
* @return This builder for chaining.
*/
public Builder setDisableCompaction(boolean value) {
bitField0_ |= 0x00000004;
disableCompaction_ = value;
onChanged();
return this;
}
/**
*
* If set true, the table's data on disk is not compacted.
*
*
* optional bool disable_compaction = 3;
* @return This builder for chaining.
*/
public Builder clearDisableCompaction() {
bitField0_ = (bitField0_ & ~0x00000004);
disableCompaction_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:kudu.TableExtraConfigPB)
}
// @@protoc_insertion_point(class_scope:kudu.TableExtraConfigPB)
private static final org.apache.kudu.KuduCommon.TableExtraConfigPB DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.apache.kudu.KuduCommon.TableExtraConfigPB();
}
public static org.apache.kudu.KuduCommon.TableExtraConfigPB getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TableExtraConfigPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TableExtraConfigPB(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.apache.kudu.KuduCommon.TableExtraConfigPB getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnTypeAttributesPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnTypeAttributesPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnSchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnSchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnSchemaDeltaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnSchemaDeltaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_SchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_SchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_HostPortPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_HostPortPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionSchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionSchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_PartitionPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_PartitionPB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_Range_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_Range_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_Equality_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_Equality_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_InList_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_InList_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_IsNotNull_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_IsNull_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_IsNull_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_ColumnPredicatePB_InBloomFilter_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_KeyRangePB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_KeyRangePB_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_kudu_TableExtraConfigPB_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_kudu_TableExtraConfigPB_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\021kudu_common.proto\022\004kudu\032\024row_operation" +
"s.proto\032\030block_bloom_filter.proto\032\021compr" +
"ession.proto\032\nhash.proto\032\rpb_util.proto\"" +
"b\n\026ColumnTypeAttributesPB\022\021\n\tprecision\030\001" +
" \001(\005\022\r\n\005scale\030\002 \001(\005\022\016\n\006length\030\003 \001(\005\022\026\n\016s" +
"pecialColCode\030\004 \001(\005\"\217\003\n\016ColumnSchemaPB\022\n" +
"\n\002id\030\001 \001(\r\022\014\n\004name\030\002 \002(\t\022\034\n\004type\030\003 \002(\0162\016" +
".kudu.DataType\022\025\n\006is_key\030\004 \001(\010:\005false\022\032\n" +
"\013is_nullable\030\005 \001(\010:\005false\022\032\n\022read_defaul" +
"t_value\030\006 \001(\014\022\033\n\023write_default_value\030\007 \001" +
"(\014\0223\n\010encoding\030\010 \001(\0162\022.kudu.EncodingType" +
":\rAUTO_ENCODING\022?\n\013compression\030\t \001(\0162\025.k" +
"udu.CompressionType:\023DEFAULT_COMPRESSION" +
"\022\033\n\020cfile_block_size\030\n \001(\005:\0010\0225\n\017type_at" +
"tributes\030\013 \001(\0132\034.kudu.ColumnTypeAttribut" +
"esPB\022\017\n\007comment\030\014 \001(\t\"\337\001\n\023ColumnSchemaDe" +
"ltaPB\022\014\n\004name\030\001 \001(\t\022\020\n\010new_name\030\002 \001(\t\022\025\n" +
"\rdefault_value\030\004 \001(\014\022\026\n\016remove_default\030\005" +
" \001(\010\022$\n\010encoding\030\006 \001(\0162\022.kudu.EncodingTy" +
"pe\022*\n\013compression\030\007 \001(\0162\025.kudu.Compressi" +
"onType\022\022\n\nblock_size\030\010 \001(\005\022\023\n\013new_commen" +
"t\030\t \001(\t\"1\n\010SchemaPB\022%\n\007columns\030\001 \003(\0132\024.k" +
"udu.ColumnSchemaPB\"(\n\nHostPortPB\022\014\n\004host" +
"\030\001 \002(\t\022\014\n\004port\030\002 \002(\r\"\253\005\n\021PartitionSchema" +
"PB\022?\n\013hash_schema\030\001 \003(\0132*.kudu.Partition" +
"SchemaPB.HashBucketSchemaPB\022;\n\014range_sch" +
"ema\030\002 \001(\0132%.kudu.PartitionSchemaPB.Range" +
"SchemaPB\022P\n\031custom_hash_schema_ranges\030\005 " +
"\003(\0132-.kudu.PartitionSchemaPB.RangeWithHa" +
"shSchemaPB\032@\n\022ColumnIdentifierPB\022\014\n\002id\030\001" +
" \001(\005H\000\022\016\n\004name\030\002 \001(\tH\000B\014\n\nidentifier\032L\n\r" +
"RangeSchemaPB\022;\n\007columns\030\001 \003(\0132*.kudu.Pa" +
"rtitionSchemaPB.ColumnIdentifierPB\032\241\001\n\022H" +
"ashBucketSchemaPB\022;\n\007columns\030\001 \003(\0132*.kud" +
"u.PartitionSchemaPB.ColumnIdentifierPB\022\023" +
"\n\013num_buckets\030\002 \002(\005\022\014\n\004seed\030\003 \001(\r\022+\n\016has" +
"h_algorithm\030\004 \001(\0162\023.kudu.HashAlgorithm\032\205" +
"\001\n\025RangeWithHashSchemaPB\022+\n\014range_bounds" +
"\030\001 \001(\0132\025.kudu.RowOperationsPB\022?\n\013hash_sc" +
"hema\030\002 \003(\0132*.kudu.PartitionSchemaPB.Hash" +
"BucketSchemaPBJ\004\010\003\020\004J\004\010\004\020\005\"_\n\013PartitionP" +
"B\022\030\n\014hash_buckets\030\001 \003(\005B\002\020\001\022\033\n\023partition" +
"_key_start\030\002 \001(\014\022\031\n\021partition_key_end\030\003 " +
"\001(\014\"\357\004\n\021ColumnPredicatePB\022\016\n\006column\030\001 \001(" +
"\t\022.\n\005range\030\002 \001(\0132\035.kudu.ColumnPredicateP" +
"B.RangeH\000\0224\n\010equality\030\003 \001(\0132 .kudu.Colum" +
"nPredicatePB.EqualityH\000\0228\n\013is_not_null\030\004" +
" \001(\0132!.kudu.ColumnPredicatePB.IsNotNullH" +
"\000\0221\n\007in_list\030\005 \001(\0132\036.kudu.ColumnPredicat" +
"ePB.InListH\000\0221\n\007is_null\030\006 \001(\0132\036.kudu.Col" +
"umnPredicatePB.IsNullH\000\022@\n\017in_bloom_filt" +
"er\030\007 \001(\0132%.kudu.ColumnPredicatePB.InBloo" +
"mFilterH\000\0321\n\005Range\022\023\n\005lower\030\001 \001(\014B\004\210\265\030\001\022" +
"\023\n\005upper\030\002 \001(\014B\004\210\265\030\001\032\037\n\010Equality\022\023\n\005valu" +
"e\030\001 \001(\014B\004\210\265\030\001\032\036\n\006InList\022\024\n\006values\030\001 \003(\014B" +
"\004\210\265\030\001\032\013\n\tIsNotNull\032\010\n\006IsNull\032j\n\rInBloomF" +
"ilter\022/\n\rbloom_filters\030\001 \003(\0132\030.kudu.Bloc" +
"kBloomFilterPB\022\023\n\005lower\030\002 \001(\014B\004\210\265\030\001\022\023\n\005u" +
"pper\030\003 \001(\014B\004\210\265\030\001B\013\n\tpredicate\"k\n\nKeyRang" +
"ePB\022\037\n\021start_primary_key\030\001 \001(\014B\004\210\265\030\001\022\036\n\020" +
"stop_primary_key\030\002 \001(\014B\004\210\265\030\001\022\034\n\024size_byt" +
"es_estimates\030\003 \002(\004\"k\n\022TableExtraConfigPB" +
"\022\033\n\023history_max_age_sec\030\001 \001(\005\022\034\n\024mainten" +
"ance_priority\030\002 \001(\005\022\032\n\022disable_compactio" +
"n\030\003 \001(\010*\246\002\n\010DataType\022\021\n\014UNKNOWN_DATA\020\347\007\022" +
"\t\n\005UINT8\020\000\022\010\n\004INT8\020\001\022\n\n\006UINT16\020\002\022\t\n\005INT1" +
"6\020\003\022\n\n\006UINT32\020\004\022\t\n\005INT32\020\005\022\n\n\006UINT64\020\006\022\t" +
"\n\005INT64\020\007\022\n\n\006STRING\020\010\022\010\n\004BOOL\020\t\022\t\n\005FLOAT" +
"\020\n\022\n\n\006DOUBLE\020\013\022\n\n\006BINARY\020\014\022\023\n\017UNIXTIME_M" +
"ICROS\020\r\022\n\n\006INT128\020\016\022\r\n\tDECIMAL32\020\017\022\r\n\tDE" +
"CIMAL64\020\020\022\016\n\nDECIMAL128\020\021\022\016\n\nIS_DELETED\020" +
"\022\022\013\n\007VARCHAR\020\023\022\010\n\004DATE\020\024*\240\001\n\014EncodingTyp" +
"e\022\025\n\020UNKNOWN_ENCODING\020\347\007\022\021\n\rAUTO_ENCODIN" +
"G\020\000\022\022\n\016PLAIN_ENCODING\020\001\022\023\n\017PREFIX_ENCODI" +
"NG\020\002\022\020\n\014GROUP_VARINT\020\003\022\007\n\003RLE\020\004\022\021\n\rDICT_" +
"ENCODING\020\005\022\017\n\013BIT_SHUFFLE\020\006*l\n\007HmsMode\022\010" +
"\n\004NONE\020\000\022\032\n\026DISABLE_HIVE_METASTORE\020\003\022\031\n\025" +
"ENABLE_HIVE_METASTORE\020\001\022 \n\034ENABLE_METAST" +
"ORE_INTEGRATION\020\002*h\n\027ExternalConsistency" +
"Mode\022%\n!UNKNOWN_EXTERNAL_CONSISTENCY_MOD" +
"E\020\000\022\025\n\021CLIENT_PROPAGATED\020\001\022\017\n\013COMMIT_WAI" +
"T\020\002*^\n\010ReadMode\022\025\n\021UNKNOWN_READ_MODE\020\000\022\017" +
"\n\013READ_LATEST\020\001\022\024\n\020READ_AT_SNAPSHOT\020\002\022\024\n" +
"\020READ_YOUR_WRITES\020\003*?\n\tOrderMode\022\026\n\022UNKN" +
"OWN_ORDER_MODE\020\000\022\r\n\tUNORDERED\020\001\022\013\n\007ORDER" +
"ED\020\002*W\n\020ReplicaSelection\022\035\n\031UNKNOWN_REPL" +
"ICA_SELECTION\020\000\022\017\n\013LEADER_ONLY\020\001\022\023\n\017CLOS" +
"EST_REPLICA\020\002*6\n\013TableTypePB\022\021\n\rDEFAULT_" +
"TABLE\020\000\022\024\n\020TXN_STATUS_TABLE\020\001B\021\n\017org.apa" +
"che.kudu"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
org.apache.kudu.RowOperations.getDescriptor(),
org.apache.kudu.BlockBloomFilter.getDescriptor(),
org.apache.kudu.Compression.getDescriptor(),
org.apache.kudu.Hash.getDescriptor(),
org.apache.kudu.PbUtil.getDescriptor(),
});
internal_static_kudu_ColumnTypeAttributesPB_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_kudu_ColumnTypeAttributesPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnTypeAttributesPB_descriptor,
new java.lang.String[] { "Precision", "Scale", "Length", "SpecialColCode", });
internal_static_kudu_ColumnSchemaPB_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_kudu_ColumnSchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnSchemaPB_descriptor,
new java.lang.String[] { "Id", "Name", "Type", "IsKey", "IsNullable", "ReadDefaultValue", "WriteDefaultValue", "Encoding", "Compression", "CfileBlockSize", "TypeAttributes", "Comment", });
internal_static_kudu_ColumnSchemaDeltaPB_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_kudu_ColumnSchemaDeltaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnSchemaDeltaPB_descriptor,
new java.lang.String[] { "Name", "NewName", "DefaultValue", "RemoveDefault", "Encoding", "Compression", "BlockSize", "NewComment", });
internal_static_kudu_SchemaPB_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_kudu_SchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_SchemaPB_descriptor,
new java.lang.String[] { "Columns", });
internal_static_kudu_HostPortPB_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_kudu_HostPortPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_HostPortPB_descriptor,
new java.lang.String[] { "Host", "Port", });
internal_static_kudu_PartitionSchemaPB_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_kudu_PartitionSchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionSchemaPB_descriptor,
new java.lang.String[] { "HashSchema", "RangeSchema", "CustomHashSchemaRanges", });
internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor =
internal_static_kudu_PartitionSchemaPB_descriptor.getNestedTypes().get(0);
internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionSchemaPB_ColumnIdentifierPB_descriptor,
new java.lang.String[] { "Id", "Name", "Identifier", });
internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor =
internal_static_kudu_PartitionSchemaPB_descriptor.getNestedTypes().get(1);
internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionSchemaPB_RangeSchemaPB_descriptor,
new java.lang.String[] { "Columns", });
internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor =
internal_static_kudu_PartitionSchemaPB_descriptor.getNestedTypes().get(2);
internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionSchemaPB_HashBucketSchemaPB_descriptor,
new java.lang.String[] { "Columns", "NumBuckets", "Seed", "HashAlgorithm", });
internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor =
internal_static_kudu_PartitionSchemaPB_descriptor.getNestedTypes().get(3);
internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionSchemaPB_RangeWithHashSchemaPB_descriptor,
new java.lang.String[] { "RangeBounds", "HashSchema", });
internal_static_kudu_PartitionPB_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_kudu_PartitionPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_PartitionPB_descriptor,
new java.lang.String[] { "HashBuckets", "PartitionKeyStart", "PartitionKeyEnd", });
internal_static_kudu_ColumnPredicatePB_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_kudu_ColumnPredicatePB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_descriptor,
new java.lang.String[] { "Column", "Range", "Equality", "IsNotNull", "InList", "IsNull", "InBloomFilter", "Predicate", });
internal_static_kudu_ColumnPredicatePB_Range_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(0);
internal_static_kudu_ColumnPredicatePB_Range_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_Range_descriptor,
new java.lang.String[] { "Lower", "Upper", });
internal_static_kudu_ColumnPredicatePB_Equality_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(1);
internal_static_kudu_ColumnPredicatePB_Equality_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_Equality_descriptor,
new java.lang.String[] { "Value", });
internal_static_kudu_ColumnPredicatePB_InList_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(2);
internal_static_kudu_ColumnPredicatePB_InList_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_InList_descriptor,
new java.lang.String[] { "Values", });
internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(3);
internal_static_kudu_ColumnPredicatePB_IsNotNull_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_IsNotNull_descriptor,
new java.lang.String[] { });
internal_static_kudu_ColumnPredicatePB_IsNull_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(4);
internal_static_kudu_ColumnPredicatePB_IsNull_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_IsNull_descriptor,
new java.lang.String[] { });
internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor =
internal_static_kudu_ColumnPredicatePB_descriptor.getNestedTypes().get(5);
internal_static_kudu_ColumnPredicatePB_InBloomFilter_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_ColumnPredicatePB_InBloomFilter_descriptor,
new java.lang.String[] { "BloomFilters", "Lower", "Upper", });
internal_static_kudu_KeyRangePB_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_kudu_KeyRangePB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_KeyRangePB_descriptor,
new java.lang.String[] { "StartPrimaryKey", "StopPrimaryKey", "SizeBytesEstimates", });
internal_static_kudu_TableExtraConfigPB_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_kudu_TableExtraConfigPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_kudu_TableExtraConfigPB_descriptor,
new java.lang.String[] { "HistoryMaxAgeSec", "MaintenancePriority", "DisableCompaction", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(org.apache.kudu.PbUtil.rEDACT);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
org.apache.kudu.RowOperations.getDescriptor();
org.apache.kudu.BlockBloomFilter.getDescriptor();
org.apache.kudu.Compression.getDescriptor();
org.apache.kudu.Hash.getDescriptor();
org.apache.kudu.PbUtil.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy