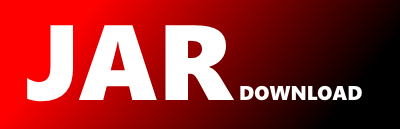
com.cloudconvert.dto.result.FutureAsyncResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudconvert-java Show documentation
Show all versions of cloudconvert-java Show documentation
CloudConvert is an online file converter API - more than 200 different audio, video, document, ebook, archive, image, spreadsheet and presentation formats supported.
package com.cloudconvert.dto.result;
import com.cloudconvert.extractor.ResultExtractor;
import com.fasterxml.jackson.core.type.TypeReference;
import lombok.Builder;
import org.apache.http.HttpResponse;
import org.jetbrains.annotations.Nullable;
import java.io.IOException;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
@Builder
public class FutureAsyncResult extends AsyncResult {
private ResultExtractor resultExtractor;
private Future future;
private TypeReference typeReference;
@Nullable
private Result result;
public Result get() throws InterruptedException, ExecutionException, IOException {
return extractResult(future.get());
}
public Result get(
final long timeout, final TimeUnit timeUnit
) throws InterruptedException, ExecutionException, TimeoutException, IOException {
return extractResult(future.get(timeout, timeUnit));
}
/**
* This method should be synchronized in order to prevent unexpected behavior if multiple threads tries to access an input stream
*/
private synchronized Result extractResult(final HttpResponse httpResponse) throws IOException {
// Cache result, in case user will try to call get() multiple times
if (result == null) {
result = resultExtractor.extract(httpResponse, typeReference);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy