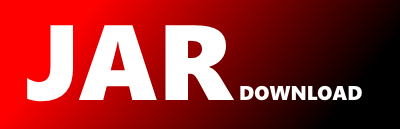
com.cloudhopper.datastore.DataStore Maven / Gradle / Ivy
The newest version!
package com.cloudhopper.datastore;
/*
* #%L
* ch-datastore
* %%
* Copyright (C) 2012 Cloudhopper by Twitter
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.io.File;
/**
* Common interface for a DataStore.
*
* @author joelauer
*/
public interface DataStore {
/**
* Sets the directory the DataStore will use for its files. The files
* actually stored in this directory depends on the underlying DataStore
* implementation.
* @param directory The directory for the DataStore
*/
public void setDirectory(File directory);
/**
* Gets the directory for the DataStore files.
* @return The directory for the DataStore files.
*/
public File getDirectory();
/**
* Sets the name of the DataStore. This name is used within the underlying
* implementation for naming files, databases, etc. For example, a name of
* "queue" may result a file such as "queue.bdb" being created in the
* DataStore directory.
* @param value The name of the DataStore
*/
public void setName(String value);
/**
* Gets the name of the DataStore.
* @return The name of the DataStore.
*/
public String getName();
/**
* Sets if missing directories should be created while opening the DataStore.
* @param value True if any missing directories will be created, or false
* if missing directories will throw a DataStoreFatalException.
*/
public void setCreateMissingDirectories(boolean value);
/**
* Gets if missing directories will be created when opening the DataStore.
* @return True if missing directories will be created, or false if not.
*/
public boolean getCreateMissingDirectories();
/**
* Whether a ShutdownHook will be registered when the DataStore is opened.
* @return True if a ShutdownHook will be registered, otherwise false.
*/
public boolean isShutdownHookEnabled();
/**
* Controls whether a ShutdownHook will be added to properly close
* a DataStore upon JVM shutdown. Offers a simple way to ensure pending
* transactions are committed and the DataStore is properly closed. Setting
* this to false is typically only used during testing to help simulate a
* "crash" of the DataStore.
* @param value If true a ShutdownHook will be added to ensure the DataStore
* is closed during JVM shutdown.
*/
public void setShutdownHookEnabled(boolean value);
/**
* Checks if the DataStore is open.
* @return True if DataStore is open, false if it is closed.
*/
public boolean isOpen();
/**
* Checks if the DataStore is opening. Opening may take a long time if
* the DataStore needs to recover. Therefore, the separate state.
* @return True if DataStore is opening, false if it isn't.
*/
public boolean isOpening();
/**
* Checks if the DataStore is closed (or never opened).
* @return True if DataStore is closed, false if it is open.
*/
public boolean isClosed();
/**
* Checks if the DataStore is closing. Closing may take a long time if
* the DataStore needs to commit a large number of transactions. Therefore, the separate state.
* @return True if DataStore is closing, false if it isn't.
*/
public boolean isClosing();
/**
* Opens the DataStore. Opens or creates the DataStore based on the
* current properties. A DataStore can and should only be opened once.
* An error will be thrown if trying to open twice.
* @throws DataStoreFatalException Thrown if there was an error while opening
* or creating the DateStore.
*/
public void open() throws DataStoreFatalException;
/**
* Closes the DataStore. Executes any cleanup activities to cleanly close
* the DataStore, including committing any transactions that may be open.
* @throws DataStoreFatalException Thrown if there was an error while closing
* the DateStore.
*/
public void close() throws DataStoreFatalException;
/**
* Deletes the DataStore. The DataStore should be closed (or not opened)
* before calling this method. If the DataStore does not exist, this method
* do nothing and return as though it succeeded.
* @throws DataStoreFatalException Thrown if there was an error while deleting
* the DateStore.
*/
public void delete() throws DataStoreFatalException;
/**
* Sets a value in the DataStore referenced by a key. Any previous value
* referenced by this key will be overwritten. The DataStore must be opened
* before calling this method.
* @param key The key of the record
* @param value The value of the record
* @throws DataStoreFatalException Thrown if there was an error while setting
* this record.
*/
public void setRecord(byte[] key, byte[] value) throws DataStoreFatalException;
/**
* Gets a value for a record in the DataStore referenced by a key. This method will
* return a value or throw an exception if the record was not found. The DataStore
* must be opened before calling this method.
* @param key The key of the record
* @return The value of the record
* @throws DataStoreFatalException Thrown if there was an error while getting
* this record.
* @throws RecordNotFoundException Thrown if a record does not exist for
* this key.
*/
public byte[] getRecord(byte[] key) throws DataStoreFatalException, RecordNotFoundException;
/**
* Deletes a record in the DataStore referenced by a key. The DataStore
* must be opened before calling this method.
* @param key The key of the record
* @throws DataStoreFatalException Thrown if there was an error while getting
* this record.
* @throws RecordNotFoundException Thrown if a record does not exist for
* this key.
*/
public void deleteRecord(byte[] key) throws DataStoreFatalException, RecordNotFoundException;
/**
* Checks if this DataStore supports the getAscendingIterator method.
* @return True if this DataStore supports getAscendingIterator or false
* if it does not.
*/
public boolean hasAscendingIteratorSupport();
/**
* Gets an iterator for all records in the data store. The iterator will
* return records in an ascending order by key. Note that not all DataStore
* implementations may support this feature.
* @return A new iterator
* @throws DataStoreFatalException Thrown if there is an error while creating
* the iterator or if the DataStore is not open.
* @see #hasAscendingIteratorSupport()
*/
public DataStoreIterator getAscendingIterator() throws DataStoreFatalException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy