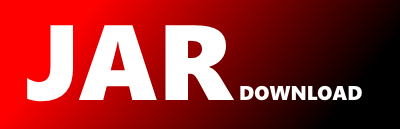
com.cloudhopper.datastore.DataStoreIterator Maven / Gradle / Ivy
The newest version!
package com.cloudhopper.datastore;
/*
* #%L
* ch-datastore
* %%
* Copyright (C) 2012 Cloudhopper by Twitter
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
/**
* Interface for iterating thru every record in a data store. An iterator is
* not thread-safe and external callers of one must take care of synchronization.
* When finished, the iterator must be closed.
*
* @author joelauer
*/
public interface DataStoreIterator {
/**
* A record in a DataStore.
*/
public static class Record {
private byte[] key;
private byte[] value;
public Record(byte[] key, byte[] value) {
this.key = key;
this.value = value;
}
public byte[] getKey() {
return this.key;
}
public byte[] getValue() {
return this.value;
}
}
/**
* Moves the cursor down one record from its current position. A iterator is
* initially positioned before the first record; the first call to the method
* next() makes the first record the current record; the second call makes
* the second record the current record, and so on. Returns false when
* there are no more records.
* @return True if there is another record to process, otherwise false.
* @throws DataStoreFatalException Thrown if there is an error while
* moving the cursor to the next record.
*/
public boolean next() throws DataStoreFatalException;
/**
* Moves the cursor to the given key. Unless this returns false, iteration
* should continue as normal. A call to next() before getRecord() after jump()
* will return the record AFTER the one identified by this key.
* @param key The key to jump to.
* @return true if the key was found.
*/
public boolean jump(byte[] key) throws DataStoreFatalException;
/**
* Gets the current record based on the cursor position.
* @return The current record
* @throws DataStoreFatalException Thrown if there isn't a current record
* such as not calling next() before calling this.
*/
public Record getRecord() throws DataStoreFatalException;
/**
* Closes the iterator and releases any resources.
* @throws DataStoreFatalException Thrown if there is an error while closing
* the iterator.
*/
public void close() throws DataStoreFatalException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy