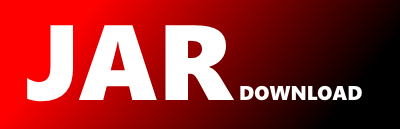
com.cloudhopper.datastore.tokyo.TokyoDataStore Maven / Gradle / Ivy
The newest version!
package com.cloudhopper.datastore.tokyo;
/*
* #%L
* ch-datastore
* %%
* Copyright (C) 2012 Cloudhopper by Twitter
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import com.cloudhopper.commons.util.HexUtil;
import com.cloudhopper.commons.util.StringUtil;
import com.cloudhopper.datastore.*;
import java.io.File;
import java.util.Timer;
import java.util.TimerTask;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import tokyocabinet.BDB;
/**
* Tokyo Cabinet backed implementation of a DataStore.
*
* @author joelauer
*/
public class TokyoDataStore extends BaseDataStore implements AscendingIteratorSupport {
private final static Logger logger = LoggerFactory.getLogger(TokyoDataStore.class);
// path used to generate a full path to the database file
private File path;
// the underlying b-tree database
private BDB bdb;
// for performance, the database is not synchronized on every put() and take()
// if -1, sync() is disabled
// if 0, sync() is called on ever put() and take()
// if > 0, the amount of ms between a sync() call is made, set to 5 seconds by default
private long synchronizeInterval;
private Timer synchronizeTimer;
private TimerTask synchronizeTimerTask;
public TokyoDataStore() {
super("TC-BDB");
// defaults
synchronizeInterval = 5000;
}
/**
* Returns the underlying B-Tree database. If not opened, this will return
* null.
* @return Null if not open, otherwise the B-Tree database.
*/
public BDB getDatabase() {
return bdb;
}
/**
* Gets the interval between sync() calls to underlying Tokyo cabinet db.
* For performance, by default, the database is not synchronized on every
* put() and take(). If -1, sync() is disabled. If 0, sync() is called on
* every put() and take(). Throughput will be dramatically decreased, but
* it'll guarantee the data makes it into the file. If > 0, its the length
* of ms between a sync() call is made, set to 5 seconds by default
* @return The synchronization interval in milliseconds
*/
public long getSynchronizeInterval() {
return synchronizeInterval;
}
/**
*
* @throws QueueStoreException Thrown if the data store is already open
* when this method is called.
*/
public void setSynchronizeInterval(long value) {
this.synchronizeInterval = value;
}
private File getDatabaseFile() {
return new File(getDirectory(), getName() + ".bdb");
}
@Override
public void doDelete() throws DataStoreFatalException {
File databaseFile = getDatabaseFile();
try {
databaseFile.delete();
} catch (Exception e) {
throw new DataStoreFatalException("Unable to delete " + databaseFile.getPath(), e);
}
}
@Override
public void doOpen() throws DataStoreFatalException {
// create a tokyo cabinet backed queue
bdb = new BDB();
// just leave comparison as LEXICAL, don't set it to anything else
/*
// comparator that does a byte at a time, up to the length of the shorter key
bdb.setcomparator(new tokyocabinet.BDBCMP() {
public int compare(byte[] a, byte[] b) {
int itlen = a.length;
if (itlen > b.length) itlen = b.length;
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy