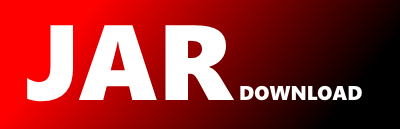
com.cloudimpl.outstack.spring.security.BearerTokenServerAuthenticationEntryPoint Maven / Gradle / Ivy
/*
* Copyright 2021 nuwan.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cloudimpl.outstack.spring.security;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.springframework.core.io.buffer.DataBuffer;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.server.reactive.ServerHttpResponse;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.oauth2.core.OAuth2AuthenticationException;
import org.springframework.security.oauth2.core.OAuth2Error;
import org.springframework.security.oauth2.server.resource.BearerTokenError;
import org.springframework.security.web.server.ServerAuthenticationEntryPoint;
import org.springframework.util.StringUtils;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
/**
*
* @author nuwan
*/
public final class BearerTokenServerAuthenticationEntryPoint implements ServerAuthenticationEntryPoint {
private ObjectMapper mapper = new ObjectMapper(); //TODO remove this
private String realmName;
public void setRealmName(String realmName) {
this.realmName = realmName;
}
@Override
public Mono commence(ServerWebExchange exchange, AuthenticationException authException) {
return Mono.defer(() -> {
try {
HttpStatus status = getStatus(authException);
Map parameters = createParameters(authException);
String wwwAuthenticate = computeWWWAuthenticateHeaderValue(parameters);
Mono buffer = Mono.just(mapper.writeValueAsBytes(new Error(authException.getMessage()))).map(b -> exchange.getResponse().bufferFactory().wrap(b));
exchange.getResponse().getHeaders().add("Content-Type", "application/json");
ServerHttpResponse response = exchange.getResponse();
response.getHeaders().set(HttpHeaders.WWW_AUTHENTICATE, wwwAuthenticate);
response.getHeaders().set("X-Error", authException.getMessage());
response.setStatusCode(status);
// return response.setComplete();
return response.writeWith(buffer);
} catch (JsonProcessingException ex) {
Logger.getLogger(PlatformAuthenticationSuccessHandler.class.getName()).log(Level.SEVERE, null, ex);
return Mono.error(ex);
}
});
}
private Map createParameters(AuthenticationException authException) {
Map parameters = new LinkedHashMap<>();
if (this.realmName != null) {
parameters.put("realm", this.realmName);
}
if (authException instanceof OAuth2AuthenticationException) {
OAuth2Error error = ((OAuth2AuthenticationException) authException).getError();
parameters.put("error", error.getErrorCode());
if (StringUtils.hasText(error.getDescription())) {
parameters.put("error_description", error.getDescription());
}
if (StringUtils.hasText(error.getUri())) {
parameters.put("error_uri", error.getUri());
}
if (error instanceof BearerTokenError) {
BearerTokenError bearerTokenError = (BearerTokenError) error;
if (StringUtils.hasText(bearerTokenError.getScope())) {
parameters.put("scope", bearerTokenError.getScope());
}
}
}
return parameters;
}
private HttpStatus getStatus(AuthenticationException authException) {
if (authException instanceof OAuth2AuthenticationException) {
OAuth2Error error = ((OAuth2AuthenticationException) authException).getError();
if (error instanceof BearerTokenError) {
return ((BearerTokenError) error).getHttpStatus();
}
}
return HttpStatus.UNAUTHORIZED;
}
private static String computeWWWAuthenticateHeaderValue(Map parameters) {
StringBuilder wwwAuthenticate = new StringBuilder();
wwwAuthenticate.append("Bearer");
if (!parameters.isEmpty()) {
wwwAuthenticate.append(" ");
int i = 0;
for (Map.Entry entry : parameters.entrySet()) {
wwwAuthenticate.append(entry.getKey()).append("=\"").append(entry.getValue()).append("\"");
if (i != parameters.size() - 1) {
wwwAuthenticate.append(", ");
}
i++;
}
}
return wwwAuthenticate.toString();
}
public static final class Error
{
private String error;
public Error(String error) {
this.error = error;
}
public String getError() {
return error;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy