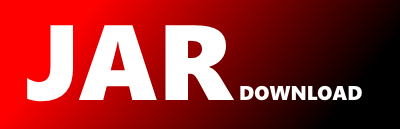
com.cloudinary.transformation.BaseExpression Maven / Gradle / Ivy
package com.cloudinary.transformation;
import com.cloudinary.Transformation;
import com.cloudinary.utils.ObjectUtils;
import com.cloudinary.utils.StringUtils;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* Defines an expression used in transformation parameter values
*
* @param Children must define themselves as T
*/
public abstract class BaseExpression {
public static final Map OPERATORS = ObjectUtils.asMap(
"=", "eq",
"!=", "ne",
"<", "lt",
">", "gt",
"<=", "lte",
">=", "gte",
"&&", "and",
"||", "or",
"*", "mul",
"/", "div",
"+", "add",
"-", "sub",
"^", "pow"
);
public static final Map PREDEFINED_VARS = ObjectUtils.asMap(
"width", "w",
"height", "h",
"initialWidth", "iw",
"initialHeight", "ih",
"aspect_ratio", "ar",
"initial_aspect_ratio", "iar",
"aspectRatio", "ar",
"initialAspectRatio", "iar",
"page_count", "pc",
"pageCount", "pc",
"face_count", "fc",
"faceCount", "fc",
"current_page", "cp",
"currentPage", "cp",
"tags", "tags",
"pageX", "px",
"pageY", "py",
"duration","du",
"initial_duration","idu",
"initialDuration","idu"
);
private static final Pattern PATTERN = getPattern();
private static final Pattern USER_VARIABLE_PATTERN = Pattern.compile("\\$_*[^_]+");
protected List expressions = null;
protected Transformation parent = null;
protected BaseExpression() {
expressions = new ArrayList();
}
/**
* Normalize an expression string, replace "nice names" with their coded values and spaces with "_".
*
* @param expression an expression
* @return a parsed expression
*/
public static String normalize(Object expression) {
if (expression == null) {
return null;
}
// If it's a number it's not an expression
if (expression instanceof Number){
return String.valueOf(expression);
}
String conditionStr = StringUtils.mergeToSingleUnderscore(String.valueOf(expression));
Matcher m = USER_VARIABLE_PATTERN.matcher(conditionStr);
StringBuilder builder = new StringBuilder();
int lastMatchEnd = 0;
while (m.find()) {
String beforeMatch = conditionStr.substring(lastMatchEnd, m.start());
builder.append(normalizeBuiltins(beforeMatch));
builder.append(m.group());
lastMatchEnd = m.end();
}
builder.append(normalizeBuiltins(conditionStr.substring(lastMatchEnd)));
return builder.toString();
}
private static String normalizeBuiltins(String input) {
String replacement;
Matcher matcher = PATTERN.matcher(input);
StringBuffer result = new StringBuffer(input.length());
while (matcher.find()) {
if (OPERATORS.containsKey(matcher.group())) {
replacement = (String) OPERATORS.get(matcher.group());
} else if (PREDEFINED_VARS.containsKey(matcher.group())) {
replacement = (String) PREDEFINED_VARS.get(matcher.group());
} else {
replacement = matcher.group();
}
matcher.appendReplacement(result, replacement);
}
matcher.appendTail(result);
return result.toString();
}
/**
* @return a regex pattern for operators and predefined vars as /((operators)(?=[ _])|variables)/
*/
private static Pattern getPattern() {
String pattern;
final ArrayList operators = new ArrayList(OPERATORS.keySet());
Collections.sort(operators, Collections.reverseOrder());
StringBuilder sb = new StringBuilder("((");
for (String op : operators) {
sb.append(Pattern.quote(op)).append("|");
}
sb.deleteCharAt(sb.length() - 1);
sb.append(")(?=[ _])|(?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy