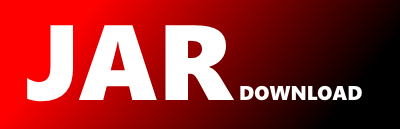
com.cloudinary.Api Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudinary-dummy-core Show documentation
Show all versions of cloudinary-dummy-core Show documentation
Cloudinary is a cloud service that offers a solution to a web application's entire image management pipeline. Upload images to the cloud. Automatically perform smart image resizing, cropping and conversion without installing any complex software. Integrate Facebook or Twitter profile image extraction in a snap, in any dimension and style to match your websiteâs graphics requirements. Images are seamlessly delivered through a fast CDN, and much much more. This Java library allows to easily integrate with Cloudinary in Java applications.
package com.cloudinary;
import java.util.*;
import com.cloudinary.api.ApiResponse;
import com.cloudinary.api.AuthorizationRequired;
import com.cloudinary.api.exceptions.*;
import com.cloudinary.metadata.MetadataField;
import com.cloudinary.metadata.MetadataDataSource;
import com.cloudinary.strategies.AbstractApiStrategy;
import com.cloudinary.utils.ObjectUtils;
import com.cloudinary.utils.StringUtils;
import org.cloudinary.json.JSONArray;
@SuppressWarnings({"rawtypes", "unchecked"})
public class Api {
public AbstractApiStrategy getStrategy() {
return strategy;
}
public enum HttpMethod {GET, POST, PUT, DELETE;}
public final static Map> CLOUDINARY_API_ERROR_CLASSES = new HashMap>();
static {
CLOUDINARY_API_ERROR_CLASSES.put(400, BadRequest.class);
CLOUDINARY_API_ERROR_CLASSES.put(401, AuthorizationRequired.class);
CLOUDINARY_API_ERROR_CLASSES.put(403, NotAllowed.class);
CLOUDINARY_API_ERROR_CLASSES.put(404, NotFound.class);
CLOUDINARY_API_ERROR_CLASSES.put(409, AlreadyExists.class);
CLOUDINARY_API_ERROR_CLASSES.put(420, RateLimited.class);
CLOUDINARY_API_ERROR_CLASSES.put(500, GeneralError.class);
}
public final Cloudinary cloudinary;
private AbstractApiStrategy strategy;
protected ApiResponse callApi(HttpMethod method, Iterable uri, Map params, Map options) throws Exception {
return this.strategy.callApi(method, uri, params, options);
}
public Api(Cloudinary cloudinary, AbstractApiStrategy strategy) {
this.cloudinary = cloudinary;
this.strategy = strategy;
this.strategy.init(this);
}
public ApiResponse ping(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("ping"), ObjectUtils.emptyMap(), options);
}
public ApiResponse usage(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
final List uri = new ArrayList();
uri.add("usage");
Object date = options.get("date");
if (date != null) {
if (date instanceof Date) {
date = ObjectUtils.toUsageApiDateFormat((Date) date);
}
uri.add(date.toString());
}
return callApi(HttpMethod.GET, uri, ObjectUtils.emptyMap(), options);
}
public ApiResponse resourceTypes(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("resources"), ObjectUtils.emptyMap(), options);
}
public ApiResponse resources(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"));
List uri = new ArrayList();
uri.add("resources");
uri.add(resourceType);
if (type != null)
uri.add(type);
ApiResponse response = callApi(HttpMethod.GET, uri, ObjectUtils.only(options, "next_cursor", "direction", "max_results", "prefix", "tags", "context", "moderations", "start_at", "metadata"), options);
return response;
}
public ApiResponse resourcesByTag(String tag, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", resourceType, "tags", tag), ObjectUtils.only(options, "next_cursor", "direction", "max_results", "tags", "context", "moderations", "metadata"), options);
return response;
}
public ApiResponse resourcesByContext(String key, Map options) throws Exception {
return resourcesByContext(key, null, options);
}
public ApiResponse resourcesByContext(String key, String value, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
Map params = ObjectUtils.only(options, "next_cursor", "direction", "max_results", "tags", "context", "moderations", "metadata");
params.put("key", key);
if (StringUtils.isNotBlank(value)) {
params.put("value", value);
}
return callApi(HttpMethod.GET, Arrays.asList("resources", resourceType, "context"), params, options);
}
public ApiResponse resourceByAssetID(String assetId, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map params = ObjectUtils.only(options, "tags", "context", "moderations");
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", assetId), params, options);
return response;
}
public ApiResponse resourcesByAssetIDs(Iterable assetIds, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map params = ObjectUtils.only(options, "public_ids", "tags", "context", "moderations");
params.put("asset_ids", assetIds);
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", "by_asset_ids"), params, options);
return response;
}
public ApiResponse resourcesByIds(Iterable publicIds, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = ObjectUtils.only(options, "tags", "context", "moderations");
params.put("public_ids", publicIds);
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", resourceType, type), params, options);
return response;
}
public ApiResponse resourcesByModeration(String kind, String status, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", resourceType, "moderations", kind, status), ObjectUtils.only(options, "next_cursor", "direction", "max_results", "tags", "context", "moderations", "metadata"), options);
return response;
}
public ApiResponse resource(String public_id, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
ApiResponse response = callApi(HttpMethod.GET, Arrays.asList("resources", resourceType, type, public_id),
ObjectUtils.only(options, "exif", "colors", "faces", "coordinates",
"image_metadata", "pages", "phash", "max_results", "quality_analysis", "cinemagraph_analysis",
"accessibility_analysis", "versions"), options);
return response;
}
public ApiResponse update(String public_id, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = new HashMap();
Util.processWriteParameters(options, params);
params.put("moderation_status", options.get("moderation_status"));
params.put("notification_url", options.get("notification_url"));
ApiResponse response = callApi(HttpMethod.POST, Arrays.asList("resources", resourceType, type, public_id),
params, options);
return response;
}
public ApiResponse deleteResources(Iterable publicIds, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = ObjectUtils.only(options, "keep_original", "invalidate", "next_cursor", "transformations");
params.put("public_ids", publicIds);
return callApi(HttpMethod.DELETE, Arrays.asList("resources", resourceType, type), params, options);
}
public ApiResponse deleteDerivedByTransformation(Iterable publicIds, List transformations, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = ObjectUtils.only(options, "invalidate", "next_cursor");
params.put("keep_original", true);
params.put("public_ids", publicIds);
params.put("transformations", Util.buildEager(transformations));
return callApi(HttpMethod.DELETE, Arrays.asList("resources", resourceType, type), params, options);
}
public ApiResponse deleteResourcesByPrefix(String prefix, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = ObjectUtils.only(options, "keep_original", "invalidate", "next_cursor");
params.put("prefix", prefix);
return callApi(HttpMethod.DELETE, Arrays.asList("resources", resourceType, type), params, options);
}
public ApiResponse deleteResourcesByTag(String tag, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
return callApi(HttpMethod.DELETE, Arrays.asList("resources", resourceType, "tags", tag), ObjectUtils.only(options, "keep_original", "invalidate", "next_cursor"), options);
}
public ApiResponse deleteAllResources(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map filtered = ObjectUtils.only(options, "keep_original", "invalidate", "next_cursor");
filtered.put("all", true);
return callApi(HttpMethod.DELETE, Arrays.asList("resources", resourceType, type), filtered, options);
}
public ApiResponse deleteDerivedResources(Iterable derivedResourceIds, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.DELETE, Arrays.asList("derived_resources"), ObjectUtils.asMap("derived_resource_ids", derivedResourceIds), options);
}
public ApiResponse tags(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
return callApi(HttpMethod.GET, Arrays.asList("tags", resourceType), ObjectUtils.only(options, "next_cursor", "max_results", "prefix"), options);
}
public ApiResponse transformations(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("transformations"), ObjectUtils.only(options, "next_cursor", "max_results", "named"), options);
}
public ApiResponse transformation(String transformation, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map map = ObjectUtils.only(options, "next_cursor", "max_results");
map.put("transformation", transformation);
return callApi(HttpMethod.GET, Arrays.asList("transformations"), map, options);
}
public ApiResponse deleteTransformation(String transformation, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map updates = ObjectUtils.asMap("transformation", transformation);
return callApi(HttpMethod.DELETE, Arrays.asList("transformations"), updates, options);
}
// updates - currently only supported update are:
// "allowed_for_strict": boolean flag
// "unsafe_update": transformation string
public ApiResponse updateTransformation(String transformation, Map updates, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
updates.put("transformation", transformation);
return callApi(HttpMethod.PUT, Arrays.asList("transformations"), updates, options);
}
public ApiResponse createTransformation(String name, String definition, Map options) throws Exception {
return callApi(HttpMethod.POST,
Arrays.asList("transformations"),
ObjectUtils.asMap("transformation", definition, "name", name), options);
}
public ApiResponse uploadPresets(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("upload_presets"), ObjectUtils.only(options, "next_cursor", "max_results"), options);
}
public ApiResponse uploadPreset(String name, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("upload_presets", name), ObjectUtils.only(options, "max_results"), options);
}
public ApiResponse deleteUploadPreset(String name, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
return callApi(HttpMethod.DELETE, Arrays.asList("upload_presets", name), ObjectUtils.emptyMap(), options);
}
public ApiResponse updateUploadPreset(String name, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map params = Util.buildUploadParams(options);
Util.clearEmpty(params);
params.putAll(ObjectUtils.only(options, "unsigned", "disallow_public_id", "live"));
return callApi(HttpMethod.PUT, Arrays.asList("upload_presets", name), params, options);
}
public ApiResponse createUploadPreset(Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
Map params = Util.buildUploadParams(options);
Util.clearEmpty(params);
params.putAll(ObjectUtils.only(options, "name", "unsigned", "disallow_public_id", "live"));
return callApi(HttpMethod.POST, Arrays.asList("upload_presets"), params, options);
}
public ApiResponse rootFolders(Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("folders"),
extractParams(options, Arrays.asList("max_results", "next_cursor")),
options);
}
public ApiResponse subFolders(String ofFolderPath, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("folders", ofFolderPath),
extractParams(options, Arrays.asList("max_results", "next_cursor")),
options);
}
//Creates an empty folder
public ApiResponse createFolder(String folderName, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.POST, Arrays.asList("folders", folderName), ObjectUtils.emptyMap(), options);
}
public ApiResponse restore(Iterable publicIds, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
String type = ObjectUtils.asString(options.get("type"), "upload");
Map params = new HashMap();
params.put("public_ids", publicIds);
params.put("versions", options.get("versions"));
ApiResponse response = callApi(HttpMethod.POST, Arrays.asList("resources", resourceType, type, "restore"), params, options);
return response;
}
public ApiResponse uploadMappings(Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("upload_mappings"),
ObjectUtils.only(options, "next_cursor", "max_results"), options);
}
public ApiResponse uploadMapping(String name, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.GET, Arrays.asList("upload_mappings"), ObjectUtils.asMap("folder", name), options);
}
public ApiResponse deleteUploadMapping(String name, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
return callApi(HttpMethod.DELETE, Arrays.asList("upload_mappings"), ObjectUtils.asMap("folder", name), options);
}
public ApiResponse updateUploadMapping(String name, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
Map params = new HashMap();
params.put("folder", name);
params.putAll(ObjectUtils.only(options, "template"));
return callApi(HttpMethod.PUT, Arrays.asList("upload_mappings"), params, options);
}
public ApiResponse createUploadMapping(String name, Map options) throws Exception {
if (options == null)
options = ObjectUtils.emptyMap();
Map params = new HashMap();
params.put("folder", name);
params.putAll(ObjectUtils.only(options, "template"));
return callApi(HttpMethod.POST, Arrays.asList("upload_mappings"), params, options);
}
public ApiResponse publishByPrefix(String prefix, Map options) throws Exception {
return publishResource("prefix", prefix, options);
}
public ApiResponse publishByTag(String tag, Map options) throws Exception {
return publishResource("tag", tag, options);
}
public ApiResponse publishByIds(Iterable publicIds, Map options) throws Exception {
return publishResource("public_ids", publicIds, options);
}
private ApiResponse publishResource(String byKey, Object value, Map options) throws Exception {
if (options == null) options = ObjectUtils.emptyMap();
String resourceType = ObjectUtils.asString(options.get("resource_type"), "image");
List uri = new ArrayList();
uri.add("resources");
uri.add(resourceType);
uri.add("publish_resources");
Map params = new HashMap();
params.put(byKey, value);
params.putAll(ObjectUtils.only(options, "invalidate", "overwrite", "type"));
return callApi(HttpMethod.POST, uri, params, options);
}
/**
* Create a new streaming profile
*
* @param name the of the profile
* @param displayName the display name of the profile
* @param representations a collection of Maps with a transformation key
* @param options additional options
* @return the new streaming profile
* @throws Exception an exception
*/
public ApiResponse createStreamingProfile(String name, String displayName, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy