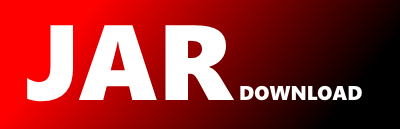
com.clusterra.iam.rest.avatar.AvatarController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-iam-rest Show documentation
Show all versions of clusterra-iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.avatar;
import com.clusterra.iam.avatar.application.AvatarData;
import com.clusterra.iam.avatar.application.AvatarImageConverter;
import com.clusterra.iam.avatar.application.AvatarImageResizeException;
import com.clusterra.iam.avatar.application.AvatarNotFoundException;
import com.clusterra.iam.avatar.application.AvatarService;
import com.clusterra.iam.session.application.SessionLobStorage;
import com.clusterra.rest.util.ResponseMessage;
import org.apache.commons.lang3.Validate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
/**
* Created with IntelliJ IDEA.
*
* @author Denis Kuchugurov
* Date: 23.12.13
*/
@Controller
@RequestMapping(value = "/iam/avatars")
public class AvatarController {
@Autowired
private AvatarService avatarService;
@Autowired
private SessionLobStorage sessionLobStorage;
@RequestMapping(value = "/{id}/image24", method = RequestMethod.GET, produces = "image/png")
public ResponseEntity image24(@PathVariable String id) throws AvatarNotFoundException {
AvatarData avatar = avatarService.find(id);
byte[] result = avatar.getImage24();
HttpHeaders headers = new HttpHeaders();
headers.setContentLength(result.length);
setCacheHeaders(headers);
return new ResponseEntity<>(result, headers, HttpStatus.OK);
}
private static void setCacheHeaders(HttpHeaders headers) {
Integer seconds = 60 * 60 * 24 * 500;
headers.setCacheControl("max-age=" + seconds);
headers.setExpires(System.currentTimeMillis() + seconds * 1000L);
}
@RequestMapping(value = "/{id}/image48", method = RequestMethod.GET, produces = "image/png")
public ResponseEntity image48(@PathVariable String id) throws AvatarNotFoundException {
AvatarData avatar = avatarService.find(id);
byte[] result = avatar.getImage48();
HttpHeaders headers = new HttpHeaders();
headers.setContentLength(result.length);
setCacheHeaders(headers);
return new ResponseEntity<>(result, headers, HttpStatus.OK);
}
@RequestMapping(value = "/{id}/image128", method = RequestMethod.GET, produces = "image/png")
public ResponseEntity image128(@PathVariable String id) throws AvatarNotFoundException {
AvatarData avatar = avatarService.find(id);
byte[] result = avatar.getImage128();
HttpHeaders headers = new HttpHeaders();
headers.setContentLength(result.length);
setCacheHeaders(headers);
return new ResponseEntity<>(result, headers, HttpStatus.OK);
}
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public ResponseEntity upload(@RequestParam(required = false) String token,
@RequestParam(required = false) MultipartFile file) throws IOException, AvatarImageResizeException, InvalidImageFileException {
Validate.notNull(token, "token is null");
AvatarValidator.validate(file);
ByteArrayResource resource = new ByteArrayResource(file.getBytes());
byte[] image = AvatarImageConverter.getAvatarData(resource).getImage128();
sessionLobStorage.store(token, AvatarSessionKey.UPLOAD_AVATAR_SESSION_KEY, image);
return new ResponseEntity<>(ResponseMessage.message("uploaded " + file.getOriginalFilename()), HttpStatus.OK);
}
@RequestMapping(value = "/uploaded", method = RequestMethod.GET, produces = "image/png")
public ResponseEntity uploaded(@RequestParam(required = false) String token) {
Validate.notNull(token, "token is null");
byte[] result = (byte[]) sessionLobStorage.retrieve(token, AvatarSessionKey.UPLOAD_AVATAR_SESSION_KEY);
HttpHeaders headers = new HttpHeaders();
headers.setContentLength(result.length);
return new ResponseEntity<>(result, headers, HttpStatus.OK);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy