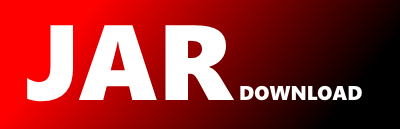
com.clusterra.iam.rest.session.SessionController Maven / Gradle / Ivy
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.session;
import com.clusterra.iam.core.application.user.UserDisabledException;
import com.clusterra.iam.core.domain.model.tenant.Tenant;
import com.clusterra.iam.session.tracker.AuthenticationService;
import com.clusterra.iam.core.application.tenant.TenantDisabledException;
import com.clusterra.iam.core.application.tenant.TenantId;
import com.clusterra.iam.core.application.tenant.TenantNotFoundException;
import com.clusterra.iam.core.application.tenant.TenantQueryService;
import com.clusterra.iam.core.application.tracker.IdentityTrackerLifeCycle;
import com.clusterra.iam.core.application.user.UserId;
import com.clusterra.iam.session.application.SessionRegistry;
import com.clusterra.iam.session.application.SessionToken;
import com.clusterra.iam.session.tracker.InvalidCredentialsException;
import com.clusterra.rest.util.ResponseMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
/**
* @author Alexander Fyodorov
* @author Denis Kuchugurov
*/
@RestController
@RequestMapping(value = "/iam/sessions", produces = {MediaType.APPLICATION_JSON_VALUE})
public class SessionController {
@Autowired
private AuthenticationService authenticationService;
@Autowired
private SessionRegistry sessionRegistry;
@Autowired
private SessionTokenResolver sessionTokenResolver;
@Autowired
private IdentityTrackerLifeCycle identityTrackerLifeCycle;
@Autowired
private TenantQueryService tenantQueryService;
@RequestMapping(value = "", method = RequestMethod.POST)
public ResponseEntity login(HttpServletRequest request, @RequestBody Credentials credentials) throws InvalidCredentialsException, TenantNotFoundException, TenantDisabledException, UserDisabledException {
UserId userId = authenticationService.authenticate(credentials.login, credentials.password);
SessionToken sessionToken = sessionRegistry.registerSession(userId.getId(), request.getRemoteAddr());
Tenant tenant = tenantQueryService.findByUser(userId);
identityTrackerLifeCycle.startTracking(userId, new TenantId(tenant.getId()));
return new ResponseEntity<>(sessionToken, HttpStatus.CREATED);
}
@RequestMapping(value = "", method = RequestMethod.DELETE)
public ResponseEntity logout(HttpServletRequest request) {
sessionRegistry.invalidateSession(sessionTokenResolver.resolve(request));
identityTrackerLifeCycle.stopTracking();
return new ResponseEntity<>(ResponseMessage.message("logout"), HttpStatus.NO_CONTENT);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy