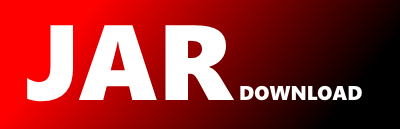
com.clusterra.iam.rest.tenant.TenantController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-iam-rest Show documentation
Show all versions of clusterra-iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.tenant;
import com.clusterra.iam.core.application.tenant.InvalidTenantNameException;
import com.clusterra.iam.core.application.tenant.TenantId;
import com.clusterra.iam.core.application.user.EmailAlreadyExistsException;
import com.clusterra.iam.core.application.user.InvalidEmailException;
import com.clusterra.iam.core.domain.model.tenant.Tenant;
import com.clusterra.iam.rest.tenant.pod.ActivationPod;
import com.clusterra.iam.rest.tenant.pod.SignUpPod;
import com.clusterra.iam.rest.tenant.pod.UpdatePod;
import com.clusterra.iam.rest.tenant.resource.TenantResource;
import com.clusterra.iam.rest.tenant.resource.TenantResourceAssembler;
import com.clusterra.rest.util.ResponseMessage;
import com.clusterra.iam.core.application.tenant.InvalidTenantActivationTokenException;
import com.clusterra.iam.core.application.tenant.TenantAlreadyExistsException;
import com.clusterra.iam.core.application.tenant.TenantCommandService;
import com.clusterra.iam.core.application.tenant.TenantNotFoundException;
import com.clusterra.iam.core.application.tenant.TenantQueryService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.web.PageableDefault;
import org.springframework.data.web.PagedResourcesAssembler;
import org.springframework.hateoas.PagedResources;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindException;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
/**
* @author Denis Kuchugurov
*/
@RequestMapping(value = "/iam/tenants", produces = {MediaType.APPLICATION_JSON_VALUE})
@RestController
public class TenantController {
@Autowired
private TenantCommandService tenantCommandService;
@Autowired
private TenantQueryService tenantQueryService;
@Autowired
private TenantResourceAssembler tenantResourceAssembler;
@RequestMapping(value = "/sign-up", method = RequestMethod.POST)
public ResponseEntity signUp(@Valid @RequestBody SignUpPod pod,
BindingResult bindingResult) throws EmailAlreadyExistsException, TenantAlreadyExistsException, InvalidEmailException, BindException, InvalidTenantNameException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
tenantCommandService.signUp(pod.getName(), pod.getEmail());
return new ResponseEntity<>(ResponseMessage.message("tenant signed-up, please expect email with registration instructions on " + pod.getEmail()), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/check-activation-token", method = RequestMethod.GET)
public ResponseEntity checkActivationTokenValid(
@RequestParam(required = false) String activationToken) throws InvalidTenantActivationTokenException {
tenantQueryService.checkActivationToken(activationToken);
return new ResponseEntity<>(ResponseMessage.message("tenant activation token is valid"), HttpStatus.OK);
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public ResponseEntity get(@PathVariable String id) throws TenantNotFoundException {
Tenant tenant = tenantQueryService.find(new TenantId(id));
return new ResponseEntity<>(tenantResourceAssembler.toResource(tenant), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/search", method = RequestMethod.GET)
public ResponseEntity> search(@PageableDefault Pageable pageable,
@RequestParam(required = false) String searchBy,
PagedResourcesAssembler assembler) {
Page page = tenantQueryService.findAll(pageable, searchBy);
return new ResponseEntity<>(assembler.toResource(page, tenantResourceAssembler), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/activate", method = RequestMethod.PUT)
public ResponseEntity activate(@Valid @RequestBody ActivationPod pod,
BindingResult bindingResult) throws InvalidTenantActivationTokenException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
String activationToken = pod.getActivationToken();
Tenant tenant = tenantCommandService.activate(activationToken, pod.getLogin(), pod.getPassword(), pod.getFirstName(), pod.getLastName());
return new ResponseEntity<>(tenantResourceAssembler.toResource(tenant), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/{id}/update", method = RequestMethod.PUT)
public ResponseEntity update(@PathVariable String id, @Valid @RequestBody UpdatePod pod, BindingResult bindingResult) throws TenantAlreadyExistsException, TenantNotFoundException, BindException, InvalidTenantNameException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
Tenant tenant = tenantCommandService.update(new TenantId(id), pod.getName(), pod.getDescription(), pod.getWebSite(), pod.getLanguage(), pod.getLocation());
return new ResponseEntity<>(tenantResourceAssembler.toResource(tenant), HttpStatus.ACCEPTED);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy