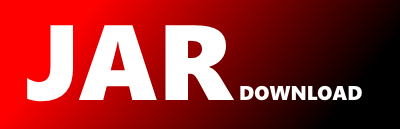
com.clusterra.iam.rest.user.resource.UserResourceAssembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-iam-rest Show documentation
Show all versions of clusterra-iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.user.resource;
import com.clusterra.iam.avatar.application.AvatarImageResizeException;
import com.clusterra.iam.core.application.user.LoginAlreadyExistsException;
import com.clusterra.iam.avatar.application.AvatarNotFoundException;
import com.clusterra.iam.core.application.membership.AuthorizedMembershipService;
import com.clusterra.iam.core.application.role.RoleDescriptor;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.iam.core.application.user.InvalidUserActivationTokenException;
import com.clusterra.iam.core.application.user.UserId;
import com.clusterra.iam.core.application.user.UserNotFoundException;
import com.clusterra.iam.core.domain.model.user.User;
import com.clusterra.iam.rest.avatar.AvatarController;
import com.clusterra.iam.rest.avatar.InvalidImageFileException;
import com.clusterra.iam.rest.user.UserAvatarController;
import com.clusterra.iam.rest.user.UserController;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.hateoas.mvc.ResourceAssemblerSupport;
import org.springframework.stereotype.Component;
import org.springframework.validation.BindException;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPut;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created by dkuchugurov on 02.05.2014.
*/
@Component
public class UserResourceAssembler extends ResourceAssemblerSupport {
@Autowired
private AuthorizedMembershipService service;
public UserResourceAssembler() {
super(UserController.class, UserResource.class);
}
@Override
protected UserResource instantiateResource(User entity) {
List roles = service.findRolesByUser(new UserId(entity.getId()));
return new UserResource(
entity.getId(),
entity.getPerson().getFirstName(),
entity.getPerson().getLastName(),
entity.getPerson().getDisplayName(),
entity.getLogin(),
entity.getPerson().getContactInformation().getEmail(),
entity.getPerson().getAvatarId(),
entity.isEnabled(), toNames(roles));
}
private static List toNames(List roles) {
List result = new ArrayList<>(roles.size());
for (RoleDescriptor role : roles) {
result.add(role.getRoleName());
}
return result;
}
@Override
public UserResource toResource(User user) {
try {
UserResource resource = createResourceWithId(user.getId(), user);
if (user.hasActivationToken()) {
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).activate(null, null)).withRel("user.activate")));
}
if (user.isEnabled()) {
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).disable(user.getId())).withRel("user.disable")));
}
if (!user.isEnabled()) {
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).enable(user.getId())).withRel("user.enable")));
}
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).changePassword(null, null)).withRel("user.password.change")));
if (user.hasActivationToken()) {
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).cancelInvitation(user.getId())).withRel("user.invitation.cancel")));
}
resource.add(linkWithMethodPut(linkTo(methodOn(UserController.class).changeName(user.getId(), null, null)).withRel("user.name.change")));
resource.add(linkWithMethodPut(linkTo(methodOn(UserAvatarController.class).change(user.getId(), null)).withRel("user.avatar.change")));
resource.add(linkWithMethodPut(linkTo(methodOn(AvatarController.class).upload(null, null)).withRel("iam.avatars.upload")));
resource.add(linkTo(methodOn(AvatarController.class).image24(user.getPerson().getAvatarId())).withRel("user.avatar.image24"));
resource.add(linkTo(methodOn(AvatarController.class).image48(user.getPerson().getAvatarId())).withRel("user.avatar.image48"));
resource.add(linkTo(methodOn(AvatarController.class).image128(user.getPerson().getAvatarId())).withRel("user.avatar.image128"));
return resource;
} catch (BindException | IOException | InvalidImageFileException | AvatarNotFoundException | NotAuthenticatedException | AvatarImageResizeException | UserNotFoundException | InvalidUserActivationTokenException | LoginAlreadyExistsException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy