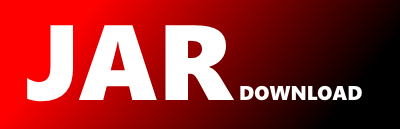
com.clusterra.iam.rest.IamDiscoverController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-iam-rest Show documentation
Show all versions of clusterra-iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest;
import com.clusterra.iam.rest.session.SessionController;
import com.clusterra.rest.util.RestMethods;
import com.clusterra.iam.rest.avatar.AvatarController;
import com.clusterra.iam.rest.tenant.TenantController;
import com.clusterra.iam.rest.user.UserController;
import org.springframework.hateoas.Link;
import org.springframework.hateoas.Links;
import org.springframework.hateoas.ResourceSupport;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodDelete;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodGet;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPost;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPut;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created with IntelliJ IDEA.
*
* @author Denis Kuchugurov
* Date: 04.12.13
*/
@RestController
@RequestMapping(value = "/iam", produces = {MediaType.APPLICATION_JSON_VALUE})
public class IamDiscoverController {
@RequestMapping(value = "", method = RequestMethod.GET)
public ResourceSupport root() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodGet(linkTo(TenantController.class).withRel("iam.tenants")));
links.add(linkWithMethodGet(linkTo(UserController.class).withRel("iam.users")));
links.add(linkWithMethodGet(linkTo(AvatarController.class).withRel("iam.avatars")));
links.add(linkWithMethodGet(linkTo(SessionController.class).withRel("iam.sessions")));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/tenants", method = RequestMethod.GET)
public ResourceSupport tenants() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(methodOn(TenantController.class).signUp(null, null)).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodDelete(linkTo(TenantController.class).withRel(RestMethods.DELETE.getName())));
links.add(linkTo(methodOn(TenantController.class).search(null, null, null)).withRel("iam.tenants.search"));
links.add(linkTo(methodOn(TenantController.class).search(null, "", null)).withRel("iam.tenants.search.by"));
links.add(linkWithMethodPut(linkTo(methodOn(TenantController.class).activate(null, null)).withRel("iam.tenants.activate")));
links.add(linkTo(methodOn(TenantController.class).checkActivationTokenValid(null)).withRel("iam.tenants.is-activation-token-valid"));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/users", method = RequestMethod.GET)
public ResourceSupport users() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(methodOn(UserController.class).invite(null)).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodDelete(linkTo(UserController.class).withRel(RestMethods.DELETE.getName())));
links.add(linkTo(methodOn(UserController.class).profile()).withRel("iam.users.profile"));
links.add(linkTo(methodOn(UserController.class).checkActivationTokenValid(null)).withRel("iam.users.is-activation-token-valid"));
links.add(linkTo(methodOn(UserController.class).checkPasswordTokenValid(null)).withRel("iam.users.is-password-token-valid"));
links.add(linkWithMethodPut(linkTo(methodOn(UserController.class).forgotPassword(null, null)).withRel("iam.users.password.forgot")));
links.add(linkWithMethodPut(linkTo(methodOn(UserController.class).resetPassword(null, null)).withRel("iam.users.password.reset")));
links.add(linkTo(methodOn(UserController.class).search(null, null, null, null)).withRel("iam.users.search"));
links.add(linkTo(methodOn(UserController.class).search(null, null, "", null)).withRel("iam.users.search.by"));
links.add(linkWithMethodPut(linkTo(methodOn(UserController.class).activate(null, null)).withRel("iam.users.activate")));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/avatars", method = RequestMethod.GET)
public ResourceSupport avatars() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(AvatarController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodDelete(linkTo(AvatarController.class).withRel(RestMethods.DELETE.getName())));
links.add(linkTo(methodOn(AvatarController.class).upload(null, null)).withRel("iam.avatars.upload"));
links.add(linkTo(methodOn(AvatarController.class).uploaded(null)).withRel("iam.avatars.uploaded"));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/sessions", method = RequestMethod.GET)
public ResourceSupport sessions() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(methodOn(SessionController.class).login(null, null)).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodDelete(linkTo(methodOn(SessionController.class).logout(null)).withRel(RestMethods.DELETE.getName())));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy