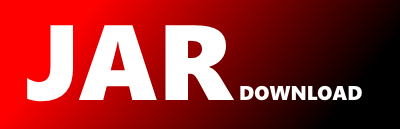
com.clusterra.iam.rest.user.UserController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-iam-rest Show documentation
Show all versions of clusterra-iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.user;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.iam.core.application.user.EmailAlreadyExistsException;
import com.clusterra.iam.core.application.user.InvalidEmailException;
import com.clusterra.iam.core.application.user.InvalidUserActivationTokenException;
import com.clusterra.iam.core.application.user.LoginAlreadyExistsException;
import com.clusterra.iam.core.application.user.UserDisabledException;
import com.clusterra.iam.core.application.user.UserId;
import com.clusterra.iam.core.domain.model.user.User;
import com.clusterra.iam.rest.user.pod.LoginPod;
import com.clusterra.iam.rest.user.pod.PasswordResetPod;
import com.clusterra.iam.rest.user.pod.UpdateNamePod;
import com.clusterra.iam.rest.user.pod.UserActivationPod;
import com.clusterra.iam.rest.user.pod.UserInvitationPod;
import com.clusterra.iam.rest.user.pod.UserStatusEnum;
import com.clusterra.iam.rest.user.resource.UserResource;
import com.clusterra.iam.rest.user.resource.UserResourceAssembler;
import com.clusterra.rest.util.ResponseMessage;
import org.apache.commons.lang3.Validate;
import com.clusterra.iam.core.application.tenant.TenantNotFoundException;
import com.clusterra.iam.core.application.tracker.IdentityTracker;
import com.clusterra.iam.core.application.user.InvalidPasswordTokenException;
import com.clusterra.iam.core.application.user.UserCommandService;
import com.clusterra.iam.core.application.user.UserNotFoundException;
import com.clusterra.iam.core.application.user.UserQueryService;
import com.clusterra.iam.rest.user.pod.PasswordChangePod;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Sort;
import org.springframework.data.web.PageableDefault;
import org.springframework.data.web.PagedResourcesAssembler;
import org.springframework.hateoas.PagedResources;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindException;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
import java.util.ArrayList;
import java.util.List;
/**
* @author Denis Kuchugurov
*/
@RestController
@RequestMapping(value = "/iam/users", produces = {MediaType.APPLICATION_JSON_VALUE})
public class UserController {
@Autowired
private IdentityTracker identityTracker;
@Autowired
private UserQueryService userQueryService;
@Autowired
private UserCommandService userCommandService;
@Autowired
private UserResourceAssembler userResourceAssembler;
@RequestMapping(value = "/invite", method = RequestMethod.POST)
public ResponseEntity invite(@RequestBody UserInvitationPod pod) throws InvalidEmailException, EmailAlreadyExistsException, TenantNotFoundException, NotAuthenticatedException {
Validate.notNull(pod, "pod is null");
Validate.notEmpty(pod.getEmail(), "email is empty");
userCommandService.invite(identityTracker.currentTenant(), pod.getEmail());
return new ResponseEntity<>(ResponseMessage.message("user invited, please expect email with registration instructions on " + pod.getEmail()), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/profile", method = RequestMethod.GET)
public ResponseEntity profile() throws UserNotFoundException, NotAuthenticatedException {
try {
UserId userId = identityTracker.currentUser();
User user = userQueryService.findUser(userId);
UserResource resource = userResourceAssembler.toResource(user);
return new ResponseEntity<>(resource, HttpStatus.OK);
} catch (NotAuthenticatedException e) {
return new ResponseEntity<>(HttpStatus.UNAUTHORIZED);
}
}
@RequestMapping(value = "/activate", method = RequestMethod.PUT)
public ResponseEntity activate(@Valid @RequestBody UserActivationPod pod,
BindingResult bindingResult) throws InvalidUserActivationTokenException, LoginAlreadyExistsException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
String login = pod.getLogin();
String password = pod.getPassword();
String firstName = pod.getFirstName();
String lastName = pod.getLastName();
String activationToken = pod.getActivationToken();
User user = userCommandService.activate(activationToken, login, password, firstName, lastName);
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.OK);
}
@RequestMapping(value = "/password/reset", method = RequestMethod.PUT)
public ResponseEntity resetPassword(@Valid @RequestBody PasswordResetPod pod,
BindingResult bindingResult) throws InvalidPasswordTokenException, UserDisabledException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
String passwordToken = pod.getPasswordToken();
String password = pod.getPassword();
User user = userCommandService.resetPassword(passwordToken, password);
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.OK);
}
@RequestMapping(value = "/password/change", method = RequestMethod.PUT)
public ResponseEntity changePassword(@Valid @RequestBody PasswordChangePod pod,
BindingResult bindingResult) throws NotAuthenticatedException, UserNotFoundException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
User user = userCommandService.changePassword(identityTracker.currentUser(), pod.getPassword());
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.OK);
}
@RequestMapping(value = "/forgot-password", method = RequestMethod.PUT)
public ResponseEntity forgotPassword(@Valid @RequestBody LoginPod pod,
BindingResult bindingResult) throws UserDisabledException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
userCommandService.forgotPassword(pod.getLogin());
return new ResponseEntity<>(ResponseMessage.message("password reset requested, please expect instructions on user account's email"), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/{id}/change/name", method = RequestMethod.PUT)
public ResponseEntity changeName(@PathVariable String id, @Valid @RequestBody UpdateNamePod pod,
BindingResult bindingResult) throws UserNotFoundException, NotAuthenticatedException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
User user = userCommandService.updateName(identityTracker.currentUser(), pod.getFirstName(), pod.getLastName());
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.OK);
}
@RequestMapping(value = "/check-activation-token", method = RequestMethod.GET)
public ResponseEntity checkActivationTokenValid(
@RequestParam(required = false) String activationToken) throws InvalidUserActivationTokenException {
userQueryService.checkActivationToken(activationToken);
return new ResponseEntity<>(ResponseMessage.message("user activation token is valid"), HttpStatus.OK);
}
@RequestMapping(value = "/check-password-token", method = RequestMethod.GET)
public ResponseEntity checkPasswordTokenValid(
@RequestParam(required = false) String passwordToken) throws InvalidPasswordTokenException {
User user = userQueryService.findByPasswordToken(passwordToken);
return new ResponseEntity<>(new LoginPod(user.getLogin()), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/disable", method = RequestMethod.PUT)
public ResponseEntity disable(@PathVariable String id) throws UserNotFoundException {
User user = userCommandService.disable(new UserId(id));
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/{id}/enable", method = RequestMethod.PUT)
public ResponseEntity enable(@PathVariable String id) throws UserNotFoundException {
User user = userCommandService.enable(new UserId(id));
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/{id}/cancel-invitation", method = RequestMethod.PUT)
public ResponseEntity cancelInvitation(@PathVariable String id) throws UserNotFoundException {
User user = userCommandService.cancelInvitation(new UserId(id));
return new ResponseEntity<>(userResourceAssembler.toResource(user), HttpStatus.ACCEPTED);
}
@RequestMapping(value = "/search", method = RequestMethod.GET)
public ResponseEntity> search(@PageableDefault Pageable pageable,
@RequestParam(required = false) UserStatusEnum status,
@RequestParam(required = false) String searchBy,
PagedResourcesAssembler assembler) throws NotAuthenticatedException {
pageable = fixSorting(pageable);
Page page;
if (UserStatusEnum.ACTIVATED.equals(status)) {
page = userQueryService.findActivated(identityTracker.currentTenant(), pageable, searchBy);
} else if (UserStatusEnum.INVITED.equals(status)) {
page = userQueryService.findInvited(identityTracker.currentTenant(), pageable, searchBy);
} else {
page = userQueryService.findAll(identityTracker.currentTenant(), pageable, searchBy);
}
PagedResources pagedResources = assembler.toResource(page, userResourceAssembler);
return new ResponseEntity<>(pagedResources, HttpStatus.OK);
}
private static Pageable fixSorting(Pageable pageable) {
if (pageable.getSort() == null) {
return pageable;
}
List orders = new ArrayList<>(1);
for (Sort.Order order : pageable.getSort()) {
switch (order.getProperty()) {
case "firstName":
orders.add(new Sort.Order(order.getDirection(), "person.firstName"));
break;
case "lastName":
orders.add(new Sort.Order(order.getDirection(), "person.lastName"));
break;
case "email":
orders.add(new Sort.Order(order.getDirection(), "person.contactInformation.email"));
break;
case "enabled":
orders.add(new Sort.Order(order.getDirection(), "status"));
break;
default:
orders.add(order);
}
}
return new PageRequest(pageable.getPageNumber(), pageable.getPageSize(), new Sort(orders));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy