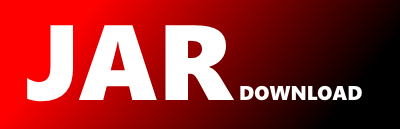
com.clusterra.pmbok.document.domain.model.term.TermAssociation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-document Show documentation
Show all versions of clusterra-pmbok-document Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.document.domain.model.term;
import org.apache.commons.lang3.Validate;
import com.clusterra.iam.core.application.tenant.TenantId;
import com.clusterra.pmbok.document.domain.model.document.Document;
import org.hibernate.annotations.GenericGenerator;
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import javax.persistence.UniqueConstraint;
import java.util.Date;
/**
* Created by Denis Kuchugurov on 28.05.2014.
*/
@Entity
@Table(name = "pmb_doc_term_association", uniqueConstraints = @UniqueConstraint(columnNames = {"termId", "document_id"}))
public class TermAssociation {
@Id
@GeneratedValue(generator = "base64")
@GenericGenerator(name = "base64", strategy = "com.clusterra.hibernate.base64.Base64IdGenerator")
private String id;
@Basic
@Column(nullable = false)
private String tenantId;
@Basic
@CreatedDate
private Date createdDate;
@Basic
@CreatedBy
private String createdByUserId;
@Basic
@Column(nullable = false)
private String termId;
@ManyToOne
private Document document;
TermAssociation() {
}
public TermAssociation(TenantId tenantId, String termId, Document document) {
Validate.notNull(tenantId);
Validate.notNull(termId);
Validate.notNull(document);
this.tenantId = tenantId.getId();
this.termId = termId;
this.document = document;
}
public String getId() {
return id;
}
public Date getCreatedDate() {
return createdDate;
}
public String getCreatedByUserId() {
return createdByUserId;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TermAssociation that = (TermAssociation) o;
if (id != null ? !id.equals(that.id) : that.id != null) return false;
return true;
}
@Override
public int hashCode() {
return id != null ? id.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy