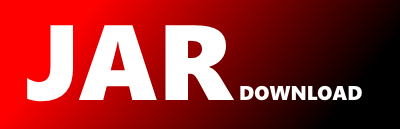
com.clusterra.pmbok.document.domain.service.template.TemplateDomainServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-document Show documentation
Show all versions of clusterra-pmbok-document Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.document.domain.service.template;
import com.clusterra.iam.core.application.tenant.TenantId;
import com.clusterra.pmbok.document.domain.model.document.Version;
import com.clusterra.pmbok.document.domain.model.template.SectionTemplateAlreadyExistsException;
import com.clusterra.pmbok.document.domain.model.template.SectionTemplateNotFoundException;
import com.clusterra.pmbok.document.domain.model.template.Template;
import com.clusterra.pmbok.document.domain.model.template.TemplateId;
import com.clusterra.pmbok.document.domain.model.template.event.TemplateCreatedEvent;
import com.clusterra.pmbok.document.domain.model.template.event.TemplateDeletedEvent;
import com.clusterra.pmbok.document.domain.model.template.event.TemplateDeletingEvent;
import com.clusterra.pmbok.document.domain.model.template.repo.SectionTemplateRepository;
import com.clusterra.pmbok.document.domain.model.template.repo.TemplateRepository;
import com.clusterra.pmbok.document.domain.model.template.repo.TemplateSearchBySpecification;
import com.clusterra.pmbok.document.domain.model.template.repo.TemplateTenantSpecification;
import com.clusterra.pmbok.document.domain.model.template.section.SectionTemplate;
import com.clusterra.pmbok.document.domain.model.template.section.SectionTemplateId;
import com.clusterra.pmbok.document.domain.model.template.section.SectionType;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.Validate;
import com.clusterra.pmbok.document.domain.model.template.event.TemplateUpdatedEvent;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.data.jpa.domain.Specifications;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Propagation;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
import java.util.Set;
/**
* @author Denis Kuchugurov.
* 11.07.2014.
*/
@Service
@Transactional(propagation = Propagation.MANDATORY)
public class TemplateDomainServiceImpl implements TemplateDomainService {
@Autowired
private TemplateRepository templateRepository;
@Autowired
private SectionTemplateRepository sectionTemplateRepository;
@Autowired
private ApplicationEventPublisher publisher;
public Template createTemplate(TenantId tenantId, Integer majorVersion, Integer minorVersion, String name) throws TemplateAlreadyExistsException {
Validate.notNull(tenantId);
Validate.notNull(majorVersion);
Validate.notNull(minorVersion);
Validate.notNull(name);
Validate.inclusiveBetween(Integer.valueOf(0), Integer.valueOf(100), majorVersion);
Validate.inclusiveBetween(Integer.valueOf(0), Integer.valueOf(100), minorVersion);
Version version = new Version(majorVersion, minorVersion);
Template existing = templateRepository.findByTenantIdByName(tenantId.getId(), name);
if (existing != null) {
throw new TemplateAlreadyExistsException(tenantId, name);
}
Template template = templateRepository.save(new Template(tenantId, version, name));
publisher.publishEvent(new TemplateCreatedEvent(this, template.getTemplateId()));
return template;
}
public Template updateTemplateName(TemplateId templateId, String name) throws TemplateNotFoundException {
Validate.notNull(name);
Validate.notNull(templateId);
Template template = findDocumentTemplate(templateId);
template.setName(name);
templateRepository.save(template);
return template;
}
public void deleteTemplate(TemplateId templateId) throws TemplateNotFoundException {
Validate.notNull(templateId);
Template template = findDocumentTemplate(templateId);
publisher.publishEvent(new TemplateDeletingEvent(this, template.getTemplateId()));
template.removeAllSections(sectionTemplateRepository);
templateRepository.delete(template);
publisher.publishEvent(new TemplateDeletedEvent(this, template.getTemplateId()));
}
public Template findBy(TemplateId templateId) throws TemplateNotFoundException {
return findDocumentTemplate(templateId);
}
public Template findByName(TenantId tenantId, String name) {
Validate.notNull(tenantId);
return templateRepository.findByTenantIdByName(tenantId.getId(), name);
}
public Page findBy(TenantId tenantId, Pageable pageable, String searchBy) {
Validate.notNull(tenantId);
Specification tenantSpec = new TemplateTenantSpecification(tenantId.getId());
Specifications specifications = Specifications.where(tenantSpec);
if (!StringUtils.isEmpty(searchBy)) {
specifications = specifications.and(new TemplateSearchBySpecification(searchBy));
}
return templateRepository.findAll(specifications, pageable);
}
public List findAllTemplates(TenantId tenantId) {
Validate.notNull(tenantId);
return templateRepository.findByTenantId(tenantId.getId());
}
public void markReady(TemplateId templateId) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
template.markReady();
}
public Set findSectionTypesUsedBy(TemplateId templateId) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
return sectionTemplateRepository.findSectionTypesUsedBy(template);
}
private Template findDocumentTemplate(TemplateId templateId) throws TemplateNotFoundException {
Validate.notNull(templateId);
Template template = templateRepository.findOne(templateId.getId());
if (template == null) {
throw new TemplateNotFoundException(templateId);
}
return template;
}
public SectionTemplate addTextSection(TemplateId templateId, String name) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
SectionTemplate textSection = template.addTextSection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return textSection;
}
public SectionTemplate addTitleSection(TemplateId templateId, String name) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
SectionTemplate textSection = template.addTitleSection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return textSection;
}
public SectionTemplate addTocSection(TemplateId templateId, String name) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
SectionTemplate textSection = template.addTocSection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return textSection;
}
public SectionTemplate findSectionTemplateBy(SectionTemplateId sectionTemplateId) throws SectionTemplateNotFoundException {
SectionTemplate sectionTemplate = sectionTemplateRepository.findOne(sectionTemplateId.getId());
if (sectionTemplate == null) {
throw new SectionTemplateNotFoundException(sectionTemplateId);
}
return sectionTemplate;
}
public SectionTemplate addHistorySection(TemplateId templateId, String name) throws TemplateNotFoundException, SectionTemplateAlreadyExistsException {
Template template = findDocumentTemplate(templateId);
SectionTemplate historySection = template.addHistorySection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return historySection;
}
public SectionTemplate addTermSection(TemplateId templateId, String name) throws TemplateNotFoundException, SectionTemplateAlreadyExistsException {
Template template = findDocumentTemplate(templateId);
SectionTemplate termSection = template.addTermSection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return termSection;
}
public SectionTemplate addReferenceSection(TemplateId templateId, String name) throws TemplateNotFoundException, SectionTemplateAlreadyExistsException {
Template template = findDocumentTemplate(templateId);
SectionTemplate referenceSection = template.addReferenceSection(name, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return referenceSection;
}
public void removeSection(TemplateId templateId, SectionTemplateId sectionTemplateId) throws TemplateNotFoundException, SectionTemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
template.removeSection(sectionTemplateId, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
}
public List findSectionTemplates(TemplateId templateId) throws TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
return sectionTemplateRepository.findBy(template);
}
public SectionTemplate updateSectionOrder(TemplateId templateId, SectionTemplateId sectionTemplateId, Integer orderIndex) throws SectionTemplateNotFoundException, TemplateNotFoundException {
Template template = findDocumentTemplate(templateId);
SectionTemplate setOrder = template.setOrder(sectionTemplateId, orderIndex, sectionTemplateRepository);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return setOrder;
}
public SectionTemplate updateSectionName(TemplateId templateId, SectionTemplateId sectionTemplateId, String name) throws TemplateNotFoundException, SectionTemplateNotFoundException {
SectionTemplate sectionTemplate = sectionTemplateRepository.findOne(sectionTemplateId.getId());
if (sectionTemplate == null) {
throw new SectionTemplateNotFoundException(sectionTemplateId);
}
sectionTemplate.setName(name);
publisher.publishEvent(new TemplateUpdatedEvent(this, templateId));
return sectionTemplate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy