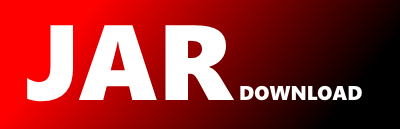
com.clusterra.pmbok.document.domain.model.document.Document Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-document Show documentation
Show all versions of clusterra-pmbok-document Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.document.domain.model.document;
import com.clusterra.iam.core.application.tenant.TenantId;
import com.clusterra.iam.core.application.user.UserId;
import com.clusterra.pmbok.document.domain.model.template.Template;
import com.clusterra.pmbok.project.domain.model.ProjectId;
import com.clusterra.pmbok.project.domain.model.ProjectVersionId;
import org.apache.commons.lang3.Validate;
import org.hibernate.annotations.GenericGenerator;
import org.springframework.data.annotation.CreatedBy;
import org.springframework.data.annotation.CreatedDate;
import org.springframework.data.annotation.LastModifiedBy;
import org.springframework.data.annotation.LastModifiedDate;
import org.springframework.data.jpa.domain.support.AuditingEntityListener;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.EntityListeners;
import javax.persistence.EnumType;
import javax.persistence.Enumerated;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import javax.persistence.ManyToOne;
import javax.persistence.Table;
import java.util.Date;
/**
* Created by dkuchugurov on 26.03.2014.
*/
@Entity
@EntityListeners({AuditingEntityListener.class})
@Table(name = "pmb_doc")
@Inheritance(strategy = InheritanceType.JOINED)
public class Document {
@Id
@GeneratedValue(generator = "base64")
@GenericGenerator(name = "base64", strategy = "com.clusterra.hibernate.base64.Base64IdGenerator")
private String id;
@Basic
@Enumerated(EnumType.STRING)
@Column(nullable = false)
private Status status;
@Basic
@Column(nullable = false)
private String tenantId;
@Basic
@CreatedDate
private Date createdDate;
@Basic
@CreatedBy
private String createdByUserId;
@Basic
@LastModifiedDate
private Date modifiedDate;
@Basic
@LastModifiedBy
private String modifiedByUserId;
@ManyToOne
private Template template;
@Basic
@Column(nullable = false)
private String projectVersionId;
@Basic
@Column(nullable = false)
private String projectId;
@Basic
private int revision;
@Basic
private int touchCounter;
Document() {
}
public Document(Template template, TenantId tenantId, ProjectId projectId, ProjectVersionId projectVersionId) {
Validate.notNull(tenantId, "tenantId is null");
Validate.notNull(projectVersionId, "projectVersionId is null");
Validate.notNull(template, "template is null");
Validate.notNull(projectId, "projectId is null");
this.tenantId = tenantId.getId();
this.projectVersionId = projectVersionId.getId();
this.projectId = projectId.getId();
this.template = template;
this.status = Status.NEW;
this.touchCounter = 0;
}
public void touch() {
touchCounter++;
}
public DocumentId getDocumentId() {
return new DocumentId(id);
}
public ProjectId getProjectId() {
return new ProjectId(projectId);
}
public void publish() {
this.status = Status.PUBLISHED;
incrementVersion();
}
public void approve() {
this.status = Status.APPROVED;
}
public void edit() {
this.status = Status.EDITING;
}
public TenantId getTenantId() {
return new TenantId(tenantId);
}
public Date getCreatedDate() {
return createdDate;
}
public UserId getCreatedByUserId() {
return new UserId(createdByUserId);
}
public Date getModifiedDate() {
return modifiedDate;
}
public UserId getModifiedByUserId() {
return new UserId(modifiedByUserId);
}
public ProjectVersionId getProjectVersionId() {
return new ProjectVersionId(projectVersionId);
}
public Template getTemplate() {
return template;
}
private void incrementVersion() {
revision++;
}
public Integer getRevision() {
return revision;
}
public Status getStatus() {
return status;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Document document = (Document) o;
if (id != null ? !id.equals(document.id) : document.id != null) return false;
return true;
}
@Override
public int hashCode() {
return id != null ? id.hashCode() : 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy