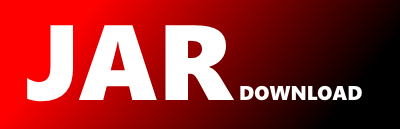
com.clusterra.pmbok.rest.PmbokDiscoverController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-rest-api Show documentation
Show all versions of clusterra-pmbok-rest-api Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.rest;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.pmbok.document.domain.service.template.TemplateNotFoundException;
import com.clusterra.pmbok.rest.document.DocumentController;
import com.clusterra.pmbok.rest.project.ProjectController;
import com.clusterra.pmbok.rest.reference.ReferenceController;
import com.clusterra.pmbok.rest.reference.ReferenceUploadController;
import com.clusterra.pmbok.rest.template.TemplateController;
import com.clusterra.pmbok.rest.term.TermController;
import com.clusterra.pmbok.rest.term.TermUploadController;
import com.clusterra.rest.util.RestMethods;
import org.springframework.hateoas.Link;
import org.springframework.hateoas.Links;
import org.springframework.hateoas.ResourceSupport;
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.List;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodGet;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPost;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created with IntelliJ IDEA.
*
* @author Denis Kuchugurov
* Date: 04.12.13
*/
@RestController
@RequestMapping(value = "/pmbok", produces = {MediaType.APPLICATION_JSON_VALUE})
public class PmbokDiscoverController {
@RequestMapping(value = "", method = RequestMethod.GET)
public ResourceSupport root() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodGet(linkTo(ProjectController.class).withRel(RelConstants.PROJECTS)));
links.add(linkWithMethodGet(linkTo(DocumentController.class).withRel(RelConstants.DOCUMENTS)));
links.add(linkWithMethodGet(linkTo(ReferenceController.class).withRel(RelConstants.REFERENCES)));
links.add(linkWithMethodGet(linkTo(TermController.class).withRel(RelConstants.TERMS)));
links.add(linkWithMethodGet(linkTo(TemplateController.class).withRel(RelConstants.TEMPLATES)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/projects", method = RequestMethod.GET)
public ResourceSupport projects() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(ProjectController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodGet(linkTo(methodOn(ProjectController.class).get("ID")).withRel(RelConstants.PROJECTS_BY_ID)));
links.add(linkWithMethodGet(linkTo(methodOn(ProjectController.class).searchProjects(null, null, null)).withRel(RelConstants.PROJECTS_SEARCH)));
links.add(linkWithMethodGet(linkTo(methodOn(ProjectController.class).searchProjects(null, "", null)).withRel(RelConstants.PROJECTS_SEARCH_BY)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/documents", method = RequestMethod.GET)
public ResourceSupport documents() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(DocumentController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodGet(linkTo(methodOn(DocumentController.class).get("ID")).withRel(RelConstants.DOCUMENTS_BY_ID)));
links.add(linkWithMethodGet(linkTo(methodOn(DocumentController.class).search(null, null, null, null, null)).withRel(RelConstants.DOCUMENTS_SEARCH)));
links.add(linkWithMethodGet(linkTo(methodOn(DocumentController.class).search(null, null, null, "", null)).withRel(RelConstants.DOCUMENTS_SEARCH_BY)));
links.add(linkWithMethodGet(linkTo(methodOn(DocumentController.class).search(null, null, "", null, null)).withRel(RelConstants.DOCUMENTS_SEARCH_BY_PROJECT_VERSION)));
links.add(linkWithMethodGet(linkTo(methodOn(DocumentController.class).search(null, "", null, null, null)).withRel(RelConstants.DOCUMENTS_SEARCH_BY_PROJECT)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/references", method = RequestMethod.GET)
public ResourceSupport references() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(ReferenceController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodGet(linkTo(methodOn(ReferenceController.class).search(null, null, null, null)).withRel(RelConstants.REFERENCES_SEARCH)));
links.add(linkWithMethodGet(linkTo(methodOn(ReferenceController.class).search(null, "", null, null)).withRel(RelConstants.REFERENCES_SEARCH_BY)));
links.add(linkWithMethodGet(linkTo(methodOn(ReferenceController.class).search(null, null, "", null)).withRel(RelConstants.REFERENCES_SEARCH_BY_SECTION)));
links.add(linkWithMethodPost(linkTo(methodOn(ReferenceUploadController.class).upload(null, null)).withRel(RelConstants.REFERENCES_UPLOAD)));
links.add(linkWithMethodPost(linkTo(methodOn(ReferenceUploadController.class).load(null)).withRel(RelConstants.REFERENCES_LOAD)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/terms", method = RequestMethod.GET)
public ResourceSupport terms() throws Exception {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(TermController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodGet(linkTo(methodOn(TermController.class).search(null, null, null, null)).withRel(RelConstants.TERMS_SEARCH)));
links.add(linkWithMethodGet(linkTo(methodOn(TermController.class).search(null, "", null, null)).withRel(RelConstants.TERMS_SEARCH_BY)));
links.add(linkWithMethodGet(linkTo(methodOn(TermController.class).search(null, null, "", null)).withRel(RelConstants.TERMS_SEARCH_BY_SECTION)));
links.add(linkWithMethodPost(linkTo(methodOn(TermUploadController.class).upload(null, null)).withRel(RelConstants.TERMS_UPLOAD)));
links.add(linkWithMethodPost(linkTo(methodOn(TermUploadController.class).load(null)).withRel(RelConstants.TERMS_LOAD)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
@RequestMapping(value = "/templates", method = RequestMethod.GET)
public ResourceSupport templates() throws NotAuthenticatedException, TemplateNotFoundException {
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(TemplateController.class).withRel(RestMethods.CREATE.getName())));
links.add(linkWithMethodGet(linkTo(methodOn(TemplateController.class).get("ID")).withRel(RelConstants.TEMPLATES_BY_ID)));
links.add(linkWithMethodGet(linkTo(methodOn(TemplateController.class).search(null, null, null)).withRel(RelConstants.TEMPLATES_SEARCH)));
links.add(linkWithMethodGet(linkTo(methodOn(TemplateController.class).search(null, "", null)).withRel(RelConstants.TEMPLATES_SEARCH_BY)));
ResourceSupport result = new ResourceSupport();
result.add(new Links(links));
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy