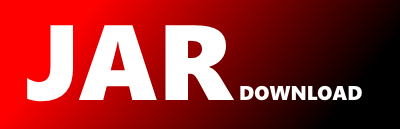
com.clusterra.pmbok.rest.project.ProjectController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-rest-api Show documentation
Show all versions of clusterra-pmbok-rest-api Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright 2012 - 2013 www.equmo.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.rest.project;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.pmbok.document.application.document.DocumentQueryService;
import com.clusterra.pmbok.document.application.template.TemplateService;
import com.clusterra.pmbok.document.domain.model.template.Template;
import com.clusterra.pmbok.project.application.ProjectCommandService;
import com.clusterra.pmbok.project.application.ProjectQueryService;
import com.clusterra.pmbok.project.domain.model.Project;
import com.clusterra.pmbok.project.domain.model.ProjectId;
import com.clusterra.pmbok.project.domain.model.ProjectVersion;
import com.clusterra.pmbok.project.domain.model.ProjectVersionId;
import com.clusterra.pmbok.project.domain.model.stakeholder.Stakeholder;
import com.clusterra.pmbok.project.domain.model.stakeholder.StakeholderClassification;
import com.clusterra.pmbok.project.domain.model.stakeholder.StakeholderType;
import com.clusterra.pmbok.project.domain.service.IncorrectProjectVersionException;
import com.clusterra.pmbok.project.domain.service.ProjectNotFoundException;
import com.clusterra.pmbok.project.domain.service.ProjectVersionNotFoundException;
import com.clusterra.pmbok.rest.project.pod.ProjectPod;
import com.clusterra.pmbok.rest.project.pod.ProjectVersionPod;
import com.clusterra.pmbok.rest.project.pod.StakeholderPod;
import com.clusterra.pmbok.rest.project.resource.DocumentTypeResource;
import com.clusterra.pmbok.rest.project.resource.DocumentTypeResourceAssembler;
import com.clusterra.pmbok.rest.project.resource.ProjectResource;
import com.clusterra.pmbok.rest.project.resource.ProjectResourceAssembler;
import com.clusterra.pmbok.rest.project.resource.ProjectVersionResource;
import com.clusterra.pmbok.rest.project.resource.ProjectVersionResourceAssembler;
import com.clusterra.rest.util.ResponseMessage;
import com.clusterra.rest.util.RestMethods;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.web.PageableDefault;
import org.springframework.data.web.PagedResourcesAssembler;
import org.springframework.hateoas.ExposesResourceFor;
import org.springframework.hateoas.Link;
import org.springframework.hateoas.PagedResources;
import org.springframework.hateoas.Resources;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindException;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPost;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created with IntelliJ IDEA.
*
* @author Denis Kuchugurov
* Date: 04.12.13
*/
@RestController
@ExposesResourceFor(Project.class)
@RequestMapping(value = "pmbok/projects", produces = {MediaType.APPLICATION_JSON_VALUE})
public class ProjectController {
@Autowired
private ProjectCommandService projectCommandService;
@Autowired
private ProjectQueryService projectQueryService;
@Autowired
private DocumentQueryService documentQueryService;
@Autowired
private TemplateService templateService;
@Autowired
private ProjectResourceAssembler projectResourceAssembler;
@Autowired
private ProjectVersionResourceAssembler projectVersionResourceAssembler;
@Autowired
private DocumentTypeResourceAssembler documentTypeResourceAssembler;
@RequestMapping(value = "", method = RequestMethod.POST)
public ResponseEntity createProject(@Valid @RequestBody ProjectPod projectPod, BindingResult bindingResult) throws BindException, NotAuthenticatedException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
Project project = projectCommandService.createProject(projectPod.getName());
return new ResponseEntity<>(projectResourceAssembler.toResource(project), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public ResponseEntity get(@PathVariable String id) throws ProjectNotFoundException {
Project project = projectQueryService.findBy(new ProjectId(id));
return new ResponseEntity<>(projectResourceAssembler.toResource(project), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/stakeholders", method = RequestMethod.POST)
public ResponseEntity addStakeholder(@PathVariable String id, @RequestBody StakeholderPod pod, BindingResult bindingResult) throws ProjectNotFoundException, BindException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
Project project = projectQueryService.findBy(new ProjectId(id));
project = projectCommandService.addStakeholder(new ProjectId(id), new Stakeholder(project.getTenantId(), pod.getName(), StakeholderType.INTERNAL, pod.getRole(), StakeholderClassification.NEUTRAL));
return new ResponseEntity<>(projectResourceAssembler.toResource(project), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/stakeholders/{stakeholderId}", method = RequestMethod.DELETE)
public ResponseEntity removeStakeholder(@PathVariable String id, @PathVariable String stakeholderId) throws ProjectNotFoundException, BindException {
Project project = projectQueryService.findBy(new ProjectId(id));
Stakeholder toRemove = null;
for (Stakeholder stakeholder : project.getStakeholders()) {
if (stakeholder.getId().equals(stakeholderId)) {
toRemove = stakeholder;
break;
}
}
if (toRemove != null) {
project = projectCommandService.removeStakeholder(new ProjectId(id), toRemove);
}
return new ResponseEntity<>(projectResourceAssembler.toResource(project), HttpStatus.OK);
}
@RequestMapping(value = "/{id}", method = RequestMethod.DELETE)
public ResponseEntity delete(@PathVariable String id) throws ProjectNotFoundException {
projectCommandService.deleteProject(new ProjectId(id));
return new ResponseEntity<>(ResponseMessage.message("deleted"), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/search", method = RequestMethod.GET)
public ResponseEntity> searchProjects(
@PageableDefault Pageable pageable,
@RequestParam(required = false) String searchBy,
PagedResourcesAssembler assembler) throws NotAuthenticatedException {
Page pageResult = projectQueryService.findBy(pageable, searchBy);
PagedResources resources = assembler.toResource(pageResult, projectResourceAssembler);
return new ResponseEntity<>(resources, HttpStatus.OK);
}
@RequestMapping(value = "/{id}/versions", method = RequestMethod.POST)
public ResponseEntity createVersion(@PathVariable String id,
@Valid @RequestBody ProjectVersionPod projectVersionPod,
BindingResult bindingResult) throws ProjectNotFoundException, BindException, IncorrectProjectVersionException, NotAuthenticatedException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
ProjectVersion projectVersion = projectCommandService.createVersion(new ProjectId(id), projectVersionPod.getLabel(), projectVersionPod.getValue(), projectVersionPod.getIsMain());
return new ResponseEntity<>(projectVersionResourceAssembler.toResource(projectVersion), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/versions", method = RequestMethod.GET)
public ResponseEntity> getVersions(@PathVariable String id) throws ProjectNotFoundException {
try {
List projectVersions = projectQueryService.findAllVersionsBy(new ProjectId(id));
List links = new ArrayList<>();
links.add(linkWithMethodPost(linkTo(methodOn(ProjectController.class).createVersion(id, null, null)).withRel(RestMethods.CREATE.getName())));
return new ResponseEntity<>(new Resources<>(projectVersionResourceAssembler.toResources(projectVersions), links), HttpStatus.OK);
} catch (BindException | IncorrectProjectVersionException | NotAuthenticatedException e) {
throw new RuntimeException(e);
}
}
@RequestMapping(value = "/versions/{versionId}", method = RequestMethod.GET)
public ResponseEntity getVersion(@PathVariable String versionId) throws ProjectVersionNotFoundException {
ProjectVersion projectVersion = projectQueryService.findVersionBy(new ProjectVersionId(versionId));
return new ResponseEntity<>(projectVersionResourceAssembler.toResource(projectVersion), HttpStatus.OK);
}
@RequestMapping(value = "/versions/{versionId}", method = RequestMethod.DELETE)
public ResponseEntity deleteVersion(@PathVariable String versionId) throws ProjectVersionNotFoundException {
projectCommandService.deleteVersion(new ProjectVersionId(versionId));
return new ResponseEntity<>(ResponseMessage.message("deleted"), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/versions/{versionId}/available-document-types", method = RequestMethod.GET)
public ResponseEntity> docTypes(@PathVariable String versionId) throws ProjectVersionNotFoundException, NotAuthenticatedException {
List result = templateService.findAllTemplates();
Set existing = documentQueryService.findUsedTemplates(new ProjectVersionId(versionId));
result.removeAll(existing);
return new ResponseEntity<>(documentTypeResourceAssembler.toResources(result), HttpStatus.OK);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy