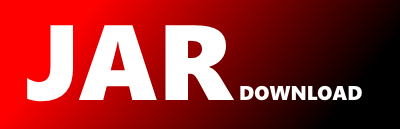
com.clusterra.pmbok.rest.project.resource.ProjectResourceAssembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-rest-api Show documentation
Show all versions of clusterra-pmbok-rest-api Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.rest.project.resource;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.iam.core.application.user.UserNotFoundException;
import com.clusterra.iam.core.application.user.UserQueryService;
import com.clusterra.pmbok.project.domain.service.ProjectNotFoundException;
import com.clusterra.pmbok.project.domain.service.ProjectVersionNotFoundException;
import com.clusterra.pmbok.rest.RelConstants;
import com.clusterra.pmbok.rest.document.DocumentController;
import com.clusterra.pmbok.rest.project.ProjectController;
import com.clusterra.pmbok.project.domain.model.Project;
import com.clusterra.rest.util.RestMethods;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.hateoas.mvc.ControllerLinkBuilder;
import org.springframework.hateoas.mvc.ResourceAssemblerSupport;
import org.springframework.stereotype.Component;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodDelete;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodGet;
import static com.clusterra.rest.util.LinkWithMethodBuilder.linkWithMethodPost;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created by dkuchugurov on 02.05.2014.
*/
@Component
public class ProjectResourceAssembler extends ResourceAssemblerSupport {
@Autowired
private UserQueryService userQueryService;
public ProjectResourceAssembler() {
super(ProjectController.class, ProjectResource.class);
}
@Override
protected ProjectResource instantiateResource(Project entity) {
try {
String createdBy = userQueryService.findUser(entity.getCreatedByUserId()).getPerson().getDisplayName();
String modifiedBy = userQueryService.findUser(entity.getModifiedByUserId()).getPerson().getDisplayName();
return new ProjectResource(
entity.getProjectId().getId(),
entity.getName(),
createdBy,
entity.getCreatedDate(),
modifiedBy,
entity.getModifiedDate()
);
} catch (UserNotFoundException e) {
throw new RuntimeException(e);
}
}
@Override
public ProjectResource toResource(Project project) {
try {
ProjectResource resource = createResourceWithId(project.getProjectId().getId(), project);
resource.add(linkWithMethodDelete(linkTo(methodOn(ProjectController.class).delete(project.getProjectId().getId())).withRel(RestMethods.DELETE.getName())));
resource.add(linkWithMethodGet(ControllerLinkBuilder.linkTo(methodOn(DocumentController.class).search(null, project.getProjectId().getId(), null, null, null)).withRel(RelConstants.PROJECT_SEARCH_DOCUMENTS)));
resource.add(linkWithMethodGet(linkTo(methodOn(ProjectController.class).getVersions(project.getProjectId().getId())).withRel(RelConstants.PROJECT_VERSIONS)));
resource.add(linkWithMethodPost(linkTo(methodOn(ProjectController.class).getVersions(project.getProjectId().getId())).withRel(RelConstants.PROJECT_VERSION_CREATE)));
return resource;
} catch (NotAuthenticatedException | ProjectNotFoundException | ProjectVersionNotFoundException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy