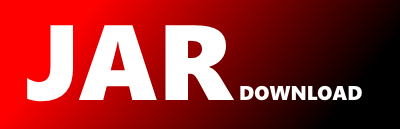
com.clusterra.pmbok.rest.template.TemplateController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-rest-api Show documentation
Show all versions of clusterra-pmbok-rest-api Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright (c) 2014.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.rest.template;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.pmbok.document.domain.model.template.SectionTemplateAlreadyExistsException;
import com.clusterra.pmbok.document.domain.model.template.TemplateId;
import com.clusterra.pmbok.document.domain.model.template.section.SectionTemplate;
import com.clusterra.pmbok.document.domain.model.template.section.SectionTemplateId;
import com.clusterra.pmbok.document.domain.model.template.section.SectionType;
import com.clusterra.pmbok.document.domain.service.template.TemplateAlreadyExistsException;
import com.clusterra.pmbok.document.application.template.TemplateService;
import com.clusterra.pmbok.document.domain.model.template.SectionTemplateNotFoundException;
import com.clusterra.pmbok.document.domain.model.template.Template;
import com.clusterra.pmbok.document.domain.service.template.TemplateNotFoundException;
import com.clusterra.pmbok.rest.template.resource.SectionTemplateResource;
import com.clusterra.pmbok.rest.template.resource.SectionTemplateResourceAssembler;
import com.clusterra.pmbok.rest.template.resource.SectionTypeResource;
import com.clusterra.pmbok.rest.template.resource.SectionTypeResourceAssembler;
import com.clusterra.pmbok.rest.template.resource.TemplateResource;
import com.clusterra.pmbok.rest.template.resource.TemplateResourceAssembler;
import com.clusterra.rest.util.ResponseMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.MessageSource;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.web.PageableDefault;
import org.springframework.data.web.PagedResourcesAssembler;
import org.springframework.hateoas.PagedResources;
import org.springframework.hateoas.Resources;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.BindException;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.validation.Valid;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Locale;
import java.util.Set;
/**
* @author Denis Kuchugurov.
* 14.07.2014.
*/
@RestController
@RequestMapping(value = "pmbok/templates", produces = {MediaType.APPLICATION_JSON_VALUE})
public class TemplateController {
@Autowired
private TemplateService templateService;
@Autowired
private TemplateResourceAssembler templateResourceAssembler;
@Autowired
private SectionTemplateResourceAssembler sectionTemplateResourceAssembler;
@Autowired
private SectionTypeResourceAssembler sectionTypeResourceAssembler;
@Autowired
private MessageSource messageSource;
@RequestMapping(value = "", method = RequestMethod.POST)
public ResponseEntity create(@Valid @RequestBody TemplatePod pod, BindingResult bindingResult) throws BindException, NotAuthenticatedException, TemplateAlreadyExistsException {
if (bindingResult.hasErrors()) {
throw new BindException(bindingResult);
}
Template template = templateService.createTemplate(pod.getMajorVersion(), pod.getMinorVersion(), pod.getName());
return new ResponseEntity<>(templateResourceAssembler.toResource(template), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}", method = RequestMethod.PUT)
public ResponseEntity updateTemplateName(@PathVariable String id, @Valid @RequestBody NamePod pod) throws TemplateNotFoundException {
Template template = templateService.updateTemplateName(new TemplateId(id), pod.getName());
return new ResponseEntity<>(templateResourceAssembler.toResource(template), HttpStatus.OK);
}
@RequestMapping(value = "/{id}", method = RequestMethod.DELETE)
public ResponseEntity delete(@PathVariable String id) throws TemplateNotFoundException {
templateService.deleteTemplate(new TemplateId(id));
return new ResponseEntity<>(ResponseMessage.message("template deleted"), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public ResponseEntity get(@PathVariable String id) throws TemplateNotFoundException {
Template template = templateService.findBy(new TemplateId(id));
return new ResponseEntity<>(templateResourceAssembler.toResource(template), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/sections/add-history", method = RequestMethod.POST)
public ResponseEntity addHistorySection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.history.title", null, "title_", locale);
SectionTemplate section = templateService.addHistorySection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/add-reference", method = RequestMethod.POST)
public ResponseEntity addReferenceSection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.reference.title", null, "title_", locale);
SectionTemplate section = templateService.addReferenceSection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/add-term", method = RequestMethod.POST)
public ResponseEntity addTermSection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.term.title", null, "title_", locale);
SectionTemplate section = templateService.addTermSection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/add-text", method = RequestMethod.POST)
public ResponseEntity addTextSection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.plaint-text.title", null, "title_", locale);
SectionTemplate section = templateService.addTextSection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/add-contents", method = RequestMethod.POST)
public ResponseEntity addContentsSection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.contents.title", null, "title_", locale);
SectionTemplate section = templateService.addTocSection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/add-title", method = RequestMethod.POST)
public ResponseEntity addTitleSection(@PathVariable String id, Locale locale) throws SectionTemplateAlreadyExistsException, TemplateNotFoundException {
String name = messageSource.getMessage("document.section.title.title", null, "title_", locale);
SectionTemplate section = templateService.addTitleSection(new TemplateId(id), name);
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(section), HttpStatus.CREATED);
}
@RequestMapping(value = "/{id}/sections/{sectionTemplateId}", method = RequestMethod.DELETE)
public ResponseEntity removeSection(@PathVariable String id, @PathVariable String sectionTemplateId) throws TemplateNotFoundException, SectionTemplateNotFoundException {
templateService.removeSection(new TemplateId(id), new SectionTemplateId(sectionTemplateId));
return new ResponseEntity<>(ResponseMessage.message("section removed"), HttpStatus.NO_CONTENT);
}
@RequestMapping(value = "/{id}/sections/{sectionTemplateId}", method = RequestMethod.GET)
public ResponseEntity getSection(@PathVariable String id, @PathVariable String sectionTemplateId) throws TemplateNotFoundException, SectionTemplateNotFoundException {
SectionTemplate template = templateService.findSectionTemplateBy(new SectionTemplateId(sectionTemplateId));
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(template), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/sections", method = RequestMethod.GET)
public ResponseEntity> getSections(@PathVariable String id) throws TemplateNotFoundException {
List sectionTemplates = templateService.findSectionTemplates(new TemplateId(id));
List resources = sectionTemplateResourceAssembler.toResources(sectionTemplates);
return new ResponseEntity<>(resources, HttpStatus.OK);
}
@RequestMapping(value = "/{id}/sections/{sectionTemplateId}/update-order", method = RequestMethod.PUT)
public ResponseEntity updateSectionOrder(@PathVariable String id, @PathVariable String sectionTemplateId, @Valid @RequestBody SectionOrderPod pod) throws TemplateNotFoundException, SectionTemplateNotFoundException {
SectionTemplate sectionTemplate = templateService.updateSectionOrder(new TemplateId(id), new SectionTemplateId(sectionTemplateId), pod.getOrderIndex());
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(sectionTemplate), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/sections/{sectionTemplateId}/update-name", method = RequestMethod.PUT)
public ResponseEntity updateSectionName(@PathVariable String id, @PathVariable String sectionTemplateId, @Valid @RequestBody NamePod pod) throws TemplateNotFoundException, SectionTemplateNotFoundException {
SectionTemplate sectionTemplate = templateService.updateSectionName(new TemplateId(id), new SectionTemplateId(sectionTemplateId), pod.getName());
return new ResponseEntity<>(sectionTemplateResourceAssembler.toResource(sectionTemplate), HttpStatus.OK);
}
@RequestMapping(value = "/search", method = RequestMethod.GET)
public ResponseEntity> search(@PageableDefault Pageable pageable,
@RequestParam(required = false) String searchBy,
PagedResourcesAssembler assembler) throws NotAuthenticatedException {
Page page = templateService.findBy(pageable, searchBy);
return new ResponseEntity<>(assembler.toResource(page, templateResourceAssembler), HttpStatus.OK);
}
@RequestMapping(value = "/{id}/available-section-types")
public ResponseEntity> getAvailableSectionTypes(@PathVariable String id) throws TemplateNotFoundException {
List all = new ArrayList<>(Arrays.asList(SectionType.values()));
Set used = templateService.findSectionTypesUsedBy(new TemplateId(id));
used.remove(SectionType.SECTION_TEXT);
all.removeAll(used);
return new ResponseEntity<>(new Resources<>(sectionTypeResourceAssembler.toResources(all)), HttpStatus.OK);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy