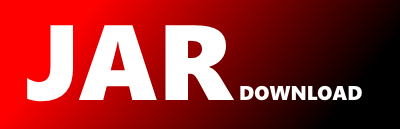
com.clusterra.pmbok.rest.term.TermUploadController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of clusterra-pmbok-rest-api Show documentation
Show all versions of clusterra-pmbok-rest-api Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
/*
* Copyright 2014 www.equmo.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.pmbok.rest.term;
import com.clusterra.pmbok.rest.uploadcsv.CsvFileValidator;
import com.clusterra.pmbok.rest.uploadcsv.CsvSessionKey;
import com.clusterra.pmbok.rest.uploadcsv.InvalidCsvFileException;
import com.clusterra.pmbok.term.application.csv.TermCsvLoader;
import org.apache.commons.lang3.Validate;
import com.clusterra.iam.core.application.tenant.TenantNotFoundException;
import com.clusterra.iam.core.application.tracker.IdentityTracker;
import com.clusterra.iam.core.application.tracker.NotAuthenticatedException;
import com.clusterra.iam.core.protect.Protected;
import com.clusterra.iam.session.application.SessionLobStorage;
import com.clusterra.pmbok.term.application.csv.InvalidCsvTermContentException;
import com.clusterra.pmbok.term.application.csv.TermCsvLoaderException;
import com.clusterra.rest.util.ResponseMessage;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.support.DefaultMessageSourceResolvable;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
/**
* Created with IntelliJ IDEA.
*
* @author Denis Kuchugurov
* Date: 05.02.14
*/
@RestController
@RequestMapping(value = "pmbok/terms")
public class TermUploadController {
@Autowired
private IdentityTracker identityTracker;
@Autowired
private SessionLobStorage sessionLobStorage;
@Autowired
private TermCsvLoader termCsvLoader;
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public ResponseEntity upload(@RequestParam(required = false) String token,
@RequestParam(required = false) MultipartFile file) throws IOException, InvalidCsvFileException, InvalidCsvTermContentException {
Validate.notEmpty(token);
Validate.notNull(file, "file is null, make sure Content-Disposition:name='file'");
CsvFileValidator.validate(file);
byte[] bytes = file.getBytes();
termCsvLoader.validateContent(bytes);
sessionLobStorage.store(token, CsvSessionKey.UPLOAD_CSV_SESSION_KEY, bytes);
return new ResponseEntity<>(file.getOriginalFilename(), HttpStatus.OK);
}
@RequestMapping(value = "/load", method = RequestMethod.POST)
@Protected(actions = "See Personal Details")
public ResponseEntity load(@RequestParam(required = false) String token) throws TermCsvLoaderException, NotAuthenticatedException, TenantNotFoundException {
byte[] result = (byte[]) sessionLobStorage.retrieve(token, CsvSessionKey.UPLOAD_CSV_SESSION_KEY);
try {
termCsvLoader.loadFromCsv(identityTracker.currentTenant(), result);
} finally {
sessionLobStorage.remove(token, CsvSessionKey.UPLOAD_CSV_SESSION_KEY);
}
return new ResponseEntity<>(ResponseMessage.message("loaded"), HttpStatus.OK);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy