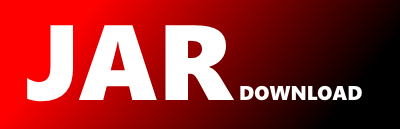
com.clusterra.iam.rest.tenant.resource.TenantResourceAssembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iam-rest Show documentation
Show all versions of iam-rest Show documentation
A application used as an example on how to set up pushing its components to the Central Repository.
The newest version!
/*
* Copyright (c) 2015 the original author or authors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.clusterra.iam.rest.tenant.resource;
import com.clusterra.iam.avatar.application.AvatarImageResizeException;
import com.clusterra.iam.core.domain.model.tenant.Tenant;
import com.clusterra.iam.avatar.application.AvatarNotFoundException;
import com.clusterra.iam.core.application.tenant.InvalidTenantNameException;
import com.clusterra.iam.core.application.tenant.TenantAlreadyExistsException;
import com.clusterra.iam.core.application.tenant.TenantNotFoundException;
import com.clusterra.iam.rest.avatar.AvatarController;
import com.clusterra.iam.rest.avatar.InvalidImageFileException;
import com.clusterra.iam.rest.tenant.TenantAvatarController;
import com.clusterra.iam.rest.tenant.TenantController;
import org.springframework.hateoas.mvc.ResourceAssemblerSupport;
import org.springframework.stereotype.Component;
import org.springframework.validation.BindException;
import java.io.IOException;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.linkTo;
import static org.springframework.hateoas.mvc.ControllerLinkBuilder.methodOn;
/**
* Created by dkuchugurov on 02.05.2014.
*/
@Component
public class TenantResourceAssembler extends ResourceAssemblerSupport {
public TenantResourceAssembler() {
super(TenantController.class, TenantResource.class);
}
@Override
protected TenantResource instantiateResource(Tenant entity) {
return new TenantResource(
entity.getId(),
entity.getName(),
entity.getDescription(),
entity.getWebSite(),
entity.getLocation(),
entity.getLanguage(),
entity.getCreatedDate(),
entity.getLastModifiedDate(),
entity.getStatus().getName(),
entity.getAvatarId());
}
@Override
public TenantResource toResource(Tenant tenant) {
try {
TenantResource resource = createResourceWithId(tenant.getId(), tenant);
resource.add(linkTo(methodOn(TenantController.class).update(tenant.getId(), null, null)).withRel("tenant.update"));
resource.add(linkTo(methodOn(TenantAvatarController.class).change(tenant.getId(), null)).withRel("tenant.avatar.change"));
resource.add(linkTo(methodOn(AvatarController.class).upload(null, null)).withRel("iam.avatars.upload"));
resource.add(linkTo(methodOn(AvatarController.class).image24(tenant.getAvatarId())).withRel("tenant.avatar.image24"));
resource.add(linkTo(methodOn(AvatarController.class).image48(tenant.getAvatarId())).withRel("tenant.avatar.image48"));
resource.add(linkTo(methodOn(AvatarController.class).image128(tenant.getAvatarId())).withRel("tenant.avatar.image128"));
return resource;
} catch (IOException | InvalidImageFileException | AvatarImageResizeException | AvatarNotFoundException | BindException | TenantNotFoundException | TenantAlreadyExistsException | InvalidTenantNameException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy