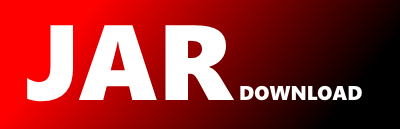
com.cmonbaby.http.core.HttpHelper Maven / Gradle / Ivy
Show all versions of http_lower Show documentation
package com.cmonbaby.http.core;
import android.text.TextUtils;
import android.util.Log;
import com.cmonbaby.http.dialog.HttpLoadable;
import com.cmonbaby.http.schedulers.AndroidSchedulers;
import rx.Observable;
import rx.Observer;
import rx.Subscription;
import rx.functions.Action0;
import rx.functions.Action1;
import rx.schedulers.Schedulers;
/**
* Author: Simon
*
QO: 8950764
*
Email: [email protected]
*
WebSize: https://www.cmonbaby.com
*
Version: 1.0.0
*
Date: 2020/12/28
*
Description: 普通请求
*/
public class HttpHelper {
private final Observable observable; // 从retrofit返回的Observable。被观察者
private final Action0 before; // http请求开始的时候一些界面上的处理
private final Action1 filter; // http请求完成时过滤,比如isSuccess = false,调用onError()
private final HttpLoadable loadable; // 对于activity和fragment loading的处理
private final String dialogTitle; // loading标题
private String dialogContent; // loading内容摘要
private final HttpCallback callback; // Http请求回调
private HttpHelper(Builder builder) {
this.observable = builder.observable;
this.before = builder.before;
this.filter = builder.filter;
this.loadable = builder.loadable;
this.dialogTitle = builder.dialogTitle;
this.dialogContent = builder.dialogContent;
this.callback = builder.callback;
}
private Subscription startWork() {
if (observable == null) {
Log.e("初始化observable错误", "observable == null");
return null;
}
if (callback == null) {
Log.e("初始化callback错误", "请实现:callback(new HttpCallback())方法");
return null;
}
if (TextUtils.isEmpty(dialogContent)) dialogContent = "加载中,请稍候……";
Observer httpCallback = new Observer() {
@Override
public void onCompleted() {
if (loadable != null && !loadable.isKeepShowing()) loadable.dismissHttpDialog();
}
@Override
public void onError(Throwable e) {
if (loadable != null) loadable.dismissHttpDialog();
callback.onError(e);
}
@Override
public void onNext(T t) {
callback.onSuccessful(t);
}
};
return observable.subscribeOn(Schedulers.io()) // 是http请求的操作是在io线程中调用,而不是UI线程中。
.observeOn(AndroidSchedulers.mainThread()) // 确保subscriber的回调,即onNext、onError和onCompleted是在Ui线程中回调
.doOnSubscribe(() -> {
if (loadable != null) loadable.showDialog(); // 如果不为空,就进行loading的show操作
if (loadable != null) loadable.setHttpDialogTitle(dialogTitle);
if (loadable != null) loadable.setHttpDialogContent(dialogContent);
if (before != null) before.call(); // 进行额外的界面处理,在http请求开始的时候
}) // http请求开始时,一些初始化的操作。比如loading界面的显示
.subscribeOn(AndroidSchedulers.mainThread()) // 这里切换到UI线程,确保doOnSubscribe在UI线程中进行,以便subscribe回调是在UI线程中进行。
.doOnNext(t -> { // 处理
if (filter != null) filter.call(t); // 进行额外的界面处理,在http请求开始的时候
})
.subscribe(httpCallback);
}
public static final class Builder {
private final Observable observable; // 从retrofit返回的Observable。被观察者
private Action0 before; // http请求开始的时候一些界面上的处理
private Action1 filter; // http请求完成时过滤,比如isSuccess = false,调用onError()
private HttpLoadable loadable; // 对于activity和fragment loading的处理
private String dialogTitle; // loading标题
private String dialogContent; // loading内容摘要
private HttpCallback callback; // Http请求回调
private Builder(Observable observable) {
this.observable = observable;
}
public static Builder builder(Observable observable) {
return new Builder<>(observable);
}
public Builder before(Action0 before) {
this.before = before;
return this;
}
public Builder filter(Action1 filter) {
this.filter = filter;
return this;
}
public Builder loadable(HttpLoadable loadable) {
this.loadable = loadable;
return this;
}
public Builder dialogTitle(String dialogTitle) {
this.dialogTitle = dialogTitle;
return this;
}
public Builder dialogContent(String dialogContent) {
this.dialogContent = dialogContent;
return this;
}
public Builder callback(HttpCallback callback) {
this.callback = callback;
return this;
}
private HttpHelper request() {
return new HttpHelper<>(this);
}
public Subscription toSubscribe() {
HttpHelper httpHelper = request();
return httpHelper.startWork();
}
}
}