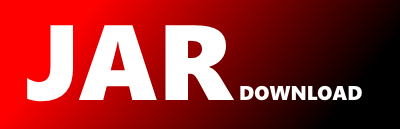
com.cmonbaby.http.exception.ExceptionHandle Maven / Gradle / Ivy
Show all versions of http_lower Show documentation
package com.cmonbaby.http.exception;
import android.net.ParseException;
import com.alibaba.fastjson.JSONException;
import org.apache.http.conn.ConnectTimeoutException;
import java.net.ConnectException;
import java.net.UnknownHostException;
/**
* Author: Simon
*
QO: 8950764
*
Email: [email protected]
*
WebSize: https://www.cmonbaby.com
*
Version: 1.0.0
*
Date: 2020/12/28
*
Description: 异常捕获处理
*/
public class ExceptionHandle {
private static final int UNAUTHORIZED = 401;
private static final int FORBIDDEN = 403;
private static final int NOT_FOUND = 404;
private static final int REQUEST_TIMEOUT = 408;
private static final int INTERNAL_SERVER_ERROR = 500;
private static final int BAD_GATEWAY = 502;
private static final int SERVICE_UNAVAILABLE = 503;
private static final int GATEWAY_TIMEOUT = 504;
public static ResponeThrowable handleException(Throwable e) {
ResponeThrowable ex;
if (e instanceof HttpException) {
HttpException httpException = (HttpException) e;
ex = new ResponeThrowable(e, ERROR.HTTP_ERROR);
switch (httpException.code()) {
case UNAUTHORIZED:
case FORBIDDEN:
case NOT_FOUND:
case REQUEST_TIMEOUT:
case GATEWAY_TIMEOUT:
case INTERNAL_SERVER_ERROR:
case BAD_GATEWAY:
case SERVICE_UNAVAILABLE:
default:
ex.message = "网络错误";
break;
}
return ex;
} else if (e instanceof ServerException) {
ServerException resultException = (ServerException) e;
ex = new ResponeThrowable(resultException, resultException.code);
ex.message = resultException.message + e.getMessage();
return ex;
} else if (e instanceof JSONException
|| e instanceof ParseException) {
ex = new ResponeThrowable(e, ERROR.PARSE_ERROR);
ex.message = "解析错误 >>> " + e.getMessage();
return ex;
} else if (e instanceof ConnectException) {
ex = new ResponeThrowable(e, ERROR.NETWORD_ERROR);
ex.message = "连接失败 >>> " + e.getMessage();
return ex;
} else if (e instanceof UnknownHostException) {
ex = new ResponeThrowable(e, ERROR.NETWORD_ERROR);
ex.message = "网络错误 >>> " + e.getMessage();
return ex;
} else if (e instanceof javax.net.ssl.SSLHandshakeException) {
ex = new ResponeThrowable(e, ERROR.SSL_ERROR);
ex.message = "证书验证失败 >>> " + e.getMessage();
return ex;
} else if (e instanceof ConnectTimeoutException){ // HttpClient已取消
ex = new ResponeThrowable(e, ERROR.TIMEOUT_ERROR);
ex.message = "请求超时 >>> " + e.getMessage();
return ex;
} else if (e instanceof java.net.SocketTimeoutException) {
ex = new ResponeThrowable(e, ERROR.TIMEOUT_ERROR);
ex.message = "服务器响应超时 >>> " + e.getMessage();
return ex;
} else if (e instanceof NullPointerException) {
ex = new ResponeThrowable(e, ERROR.UNKNOWN);
ex.message = "NullPointerException >>> " + e.getMessage();
return ex;
} else {
ex = new ResponeThrowable(e, ERROR.UNKNOWN);
ex.message = "未知错误 >>> " + e.getMessage();
return ex;
}
}
/**
* 约定异常
*/
public static class ERROR {
/**
* 未知错误
*/
public static final int UNKNOWN = 1000;
/**
* 解析错误
*/
static final int PARSE_ERROR = 1001;
/**
* 网络错误
*/
static final int NETWORD_ERROR = 1002;
/**
* 协议出错
*/
static final int HTTP_ERROR = 1003;
/**
* 证书出错
*/
static final int SSL_ERROR = 1005;
/**
* 连接超时
*/
static final int TIMEOUT_ERROR = 1006;
}
public static class ResponeThrowable extends Exception {
public int code;
public String message;
public ResponeThrowable(Throwable throwable, int code) {
super(throwable);
this.code = code;
}
}
private static class ServerException extends RuntimeException {
public int code;
String message;
}
}