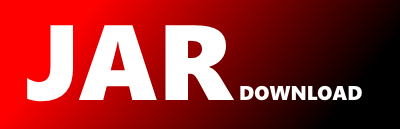
com.cmonbaby.http.rxjava.BodyOnSubscribe Maven / Gradle / Ivy
Show all versions of http_lower Show documentation
package com.cmonbaby.http.rxjava;
import retrofit2.HttpException;
import retrofit2.Response;
import rx.Observable.OnSubscribe;
import rx.Subscriber;
import rx.exceptions.Exceptions;
import rx.exceptions.OnCompletedFailedException;
import rx.exceptions.OnErrorFailedException;
import rx.exceptions.OnErrorNotImplementedException;
/**
* Author: Simon
*
QO: 8950764
*
Email: [email protected]
*
WebSize: https://www.cmonbaby.com
*
Version: 1.0.0
*
Date: 2020/12/28
*
Description:
*/
final class BodyOnSubscribe implements OnSubscribe {
private final OnSubscribe> upstream;
BodyOnSubscribe(OnSubscribe> upstream) {
this.upstream = upstream;
}
@Override
public void call(Subscriber super T> subscriber) {
upstream.call(new BodySubscriber<>(subscriber));
}
private static class BodySubscriber extends Subscriber> {
private final Subscriber super R> subscriber;
/**
* Indicates whether a terminal event has been sent to {@link #subscriber}.
*/
private boolean subscriberTerminated;
BodySubscriber(Subscriber super R> subscriber) {
super(subscriber);
this.subscriber = subscriber;
}
@Override
public void onNext(Response response) {
if (response.isSuccessful()) {
subscriber.onNext(response.body());
} else {
subscriberTerminated = true;
Throwable t = new HttpException(response);
try {
subscriber.onError(t);
} catch (OnCompletedFailedException
| OnErrorFailedException
| OnErrorNotImplementedException e) {
e.printStackTrace();
} catch (Throwable inner) {
Exceptions.throwIfFatal(inner);
inner.printStackTrace();
}
}
}
@Override
public void onError(Throwable throwable) {
if (!subscriberTerminated) {
subscriber.onError(throwable);
} else {
// This should never happen! onNext handles and forwards errors automatically.
throw new AssertionError("This should never happen! Report as a Retrofit bug with the full stacktrace.");
}
}
@Override
public void onCompleted() {
if (!subscriberTerminated) {
subscriber.onCompleted();
}
}
}
}