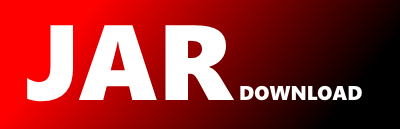
codacy.test.docker.impl.PrivilegedSpotifyDockerCommandExecutor.scala Maven / Gradle / Ivy
package codacy.test.docker.impl
import java.util
import java.util.Collections
import com.spotify.docker.client.DockerClient
import com.spotify.docker.client.messages.{ContainerConfig, HostConfig, PortBinding}
import com.whisk.docker._
import com.whisk.docker.impl.spotify.SpotifyDockerCommandExecutor
import scala.jdk.CollectionConverters._
import scala.concurrent.{ExecutionContext, Future}
/**
* This re-implements method [[SpotifyDockerCommandExecutor.createContainer]] in order to run containers in privileged mode
* The method is an exact copy of the original with the exception of adding `.privileged(true)` to the `hostConfig`
*/
class PrivilegedSpotifyDockerCommandExecutor(host: String, client: DockerClient)
extends SpotifyDockerCommandExecutor(host, client) {
override def createContainer(spec: DockerContainer)(implicit ec: ExecutionContext): Future[String] = {
val portBindings: Map[String, util.List[PortBinding]] = spec.bindPorts.map {
case (guestPort, DockerPortMapping(Some(hostPort), address)) =>
guestPort.toString -> Collections.singletonList(PortBinding.of(address, hostPort))
case (guestPort, DockerPortMapping(None, address)) =>
guestPort.toString -> Collections.singletonList(PortBinding.randomPort(address))
}
val binds: Seq[String] = spec.volumeMappings.map { volumeMapping =>
val rw = if (volumeMapping.rw) ":rw" else ""
volumeMapping.host + ":" + volumeMapping.container + rw
}
val hostConfig = {
val hostConfigBase =
HostConfig.builder().portBindings(portBindings.asJava).binds(binds.asJava)
val links = spec.links.map {
case ContainerLink(container, alias) => s"${container.name.get}:$alias"
}
val hostConfigBuilder =
if (links.isEmpty) hostConfigBase else hostConfigBase.links(links.asJava)
hostConfigBuilder
.withOption(spec.networkMode) {
case (config, networkMode) => config.networkMode(networkMode)
}
.withOption(spec.hostConfig.flatMap(_.tmpfs)) {
case (config, value) => config.tmpfs(value.asJava)
}
.withOption(spec.hostConfig.flatMap(_.memory)) {
case (config, memory) => config.memory(memory)
}
.withOption(spec.hostConfig.flatMap(_.memoryReservation)) {
case (config, reservation) => config.memoryReservation(reservation)
}
.privileged(true) // The line that was added
.build()
}
val containerConfig = ContainerConfig
.builder()
.image(spec.image)
.hostConfig(hostConfig)
.exposedPorts(spec.bindPorts.map(_._1.toString).toSeq: _*)
.tty(spec.tty)
.attachStdin(spec.stdinOpen)
.env(spec.env: _*)
.withOption(spec.user) { case (config, user) => config.user(user) }
.withOption(spec.hostname) { case (config, hostname) => config.hostname(hostname) }
.withOption(spec.command) { case (config, command) => config.cmd(command: _*) }
.withOption(spec.entrypoint) {
case (config, entrypoint) => config.entrypoint(entrypoint: _*)
}
.build()
val creation = Future(
spec.name.fold(client.createContainer(containerConfig))(client.createContainer(containerConfig, _))
)
creation.map(_.id)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy