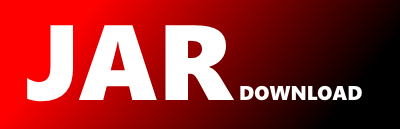
com.codacy.scoobydoo.web.WebElementWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scooby-doo Show documentation
Show all versions of scooby-doo Show documentation
A comics character for me
The newest version!
package com.codacy.scoobydoo.web;
import com.codacy.scoobydoo.Configuration;
import com.codacy.scoobydoo.LoggingHelper;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
public class WebElementWrapper {
private final By locator;
private final WebElement element;
private final List elements;
private final ScreenShotHelper screenShotHelper;
private final WebDriver driver;
private final LoggingHelper loggingHelper = new LoggingHelper();
public WebElementWrapper(WebDriver driver, Configuration configuration, By locator) {
this.locator = locator;
this.driver = driver;
this.element = driver.findElement(locator);
this.elements = driver.findElements(locator);
this.screenShotHelper = new ScreenShotHelper((TakesScreenshot) driver, configuration,loggingHelper);
turnOnHighlight();
}
public WebElement getElement() {
return element;
}
public List getElements() {
return elements;
}
public void click() {
try {
screenShotHelper.takesScreenshotOnAction("Clicking on element " + locator.toString());
element.click();
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to click with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public boolean isDisplayed() {
try {
screenShotHelper.takesScreenshotOnAction("Displaying element " + locator.toString());
return element.isDisplayed();
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to display with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public void sendKeys(CharSequence... key) {
try {
screenShotHelper.takesScreenshotOnAction("Send Keys to element " + locator.toString());
element.sendKeys(key);
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to sendKeys to element with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public void clear() {
try {
screenShotHelper.takesScreenshotOnAction("Clear element " + locator.toString());
element.clear();
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to clear element with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public String getText() {
try {
screenShotHelper.takesScreenshotOnAction("Get getText from element " + locator.toString());
return element.getText();
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to getText to element with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public String getAttribute(String attribute) {
try {
screenShotHelper.takesScreenshotOnAction("Get attribute " + attribute + " from element " + locator.toString());
return element.getAttribute(attribute);
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to get attribute " + attribute + " from element with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
public void clearAndSendKeys(CharSequence... key) {
clear();
sendKeys(key);
}
private void turnOnHighlight() {
scriptExecutor(driver, element, "arguments[0].style.border='3px solid red'");
}
private void turnOffHighlight() {
try {
scriptExecutor(driver, element, "arguments[0].style.border='none'");
} catch (Exception e) {
loggingHelper.info("Between Pages!");
}
}
private void scriptExecutor(WebDriver driver, WebElement element, String script) {
((JavascriptExecutor) driver).executeScript(script, element);
}
public void jsClick() {
try {
screenShotHelper.takesScreenshotOnAction("Clicking on element with javascript executor" + locator.toString());
scriptExecutor(driver, element, "arguments[0].click()");
} catch (Exception e) {
screenShotHelper.takesScreenshotOnError("Failed to click with javascript executor with the following exception:", e);
throw e;
} finally {
turnOffHighlight();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy