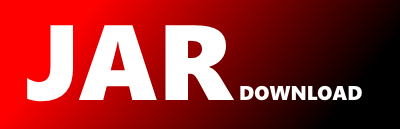
com.codahale.metrics.health.HealthCheckRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metrics-healthchecks Show documentation
Show all versions of metrics-healthchecks Show documentation
An addition to Metrics which provides the ability to run application-specific health checks,
allowing you to check your application's heath in production.
The newest version!
package com.codahale.metrics.health;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.*;
import java.util.concurrent.*;
import static com.codahale.metrics.health.HealthCheck.Result;
/**
* A registry for health checks.
*/
public class HealthCheckRegistry {
private static final Logger LOGGER = LoggerFactory.getLogger(HealthCheckRegistry.class);
private final ConcurrentMap healthChecks;
/**
* Creates a new {@link HealthCheckRegistry}.
*/
public HealthCheckRegistry() {
this.healthChecks = new ConcurrentHashMap();
}
/**
* Registers an application {@link HealthCheck}.
*
* @param name the name of the health check
* @param healthCheck the {@link HealthCheck} instance
*/
public void register(String name, HealthCheck healthCheck) {
healthChecks.putIfAbsent(name, healthCheck);
}
/**
* Unregisters the application {@link HealthCheck} with the given name.
*
* @param name the name of the {@link HealthCheck} instance
*/
public void unregister(String name) {
healthChecks.remove(name);
}
/**
* Returns a set of the names of all registered health checks.
*
* @return the names of all registered health checks
*/
public SortedSet getNames() {
return Collections.unmodifiableSortedSet(new TreeSet(healthChecks.keySet()));
}
/**
* Runs the health check with the given name.
*
* @param name the health check's name
* @return the result of the health check
* @throws NoSuchElementException if there is no health check with the given name
*/
public HealthCheck.Result runHealthCheck(String name) throws NoSuchElementException {
final HealthCheck healthCheck = healthChecks.get(name);
if (healthCheck == null) {
throw new NoSuchElementException("No health check named " + name + " exists");
}
return healthCheck.execute();
}
/**
* Runs the registered health checks and returns a map of the results.
*
* @return a map of the health check results
*/
public SortedMap runHealthChecks() {
final SortedMap results = new TreeMap();
for (Map.Entry entry : healthChecks.entrySet()) {
final Result result = entry.getValue().execute();
results.put(entry.getKey(), result);
}
return Collections.unmodifiableSortedMap(results);
}
/**
* Runs the registered health checks in parallel and returns a map of the results.
*
* @return a map of the health check results
*/
public SortedMap runHealthChecks(ExecutorService executor) {
final Map> futures = new HashMap>();
for (final Map.Entry entry : healthChecks.entrySet()) {
futures.put(entry.getKey(), executor.submit(new Callable() {
@Override
public Result call() throws Exception {
return entry.getValue().execute();
}
}));
}
final SortedMap results = new TreeMap();
for (Map.Entry> entry : futures.entrySet()) {
try {
results.put(entry.getKey(), entry.getValue().get());
} catch (Exception e) {
LOGGER.warn("Error executing health check {}", entry.getKey(), e);
}
}
return Collections.unmodifiableSortedMap(results);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy