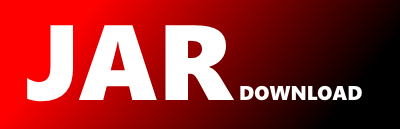
com.codbex.kronos.parser.hdbcalculationview.ndb.bimodeldatafoundation.LogicalJoin Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2022 codbex or an codbex affiliate company and contributors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v2.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v20.html
*
* SPDX-FileCopyrightText: 2022 codbex or an codbex affiliate company and contributors
* SPDX-License-Identifier: EPL-2.0
*/
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.11.26 at 10:54:28 AM EET
//
package com.codbex.kronos.parser.hdbcalculationview.ndb.bimodeldatafoundation;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.codbex.kronos.parser.hdbcalculationview.ndb.basemodelbase.FeaturedHierarchyReference;
import com.codbex.kronos.parser.hdbcalculationview.ndb.basemodelbase.TemporalJoinProperties;
/**
* Models the join betweeb two logical objects - e.g. the measure group of a cube and a shared dimension
*
*
* Java class for LogicalJoin complex type.
*
* The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="LogicalJoin">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="attributes" type="{http://www.sap.com/ndb/BiModelDataFoundation.ecore}AttributeRefs"/>
* <element name="associatedAttributeNames" type="{http://www.sap.com/ndb/BiModelDataFoundation.ecore}AttributeNames"/>
* <element name="properties" type="{http://www.sap.com/ndb/BiModelDataFoundation.ecore}JoinProperties"/>
* <element name="temporalJoinProperties" type="{http://www.sap.com/ndb/BaseModelBase.ecore}TemporalJoinProperties" minOccurs="0"/>
* <element name="associatedAttributeFeatures" type="{http://www.sap.com/ndb/BiModelDataFoundation.ecore}FeaturedAttributeReferences" minOccurs="0"/>
* <element name="associatedHierarchyFeature" type="{http://www.sap.com/ndb/BaseModelBase.ecore}FeaturedHierarchyReference" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="associatedObjectUri" use="required" type="{http://www.sap.com/ndb/RepositoryModelResource.ecore}RepositoryUri" />
* <attribute name="languageAttributeName" type="{http://www.sap.com/ndb/RepositoryModelResource.ecore}AlphanumericName" />
* <attribute name="useDimensionViewHierarchies" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "LogicalJoin", propOrder = {
"attributes",
"associatedAttributeNames",
"properties",
"temporalJoinProperties",
"associatedAttributeFeatures",
"associatedHierarchyFeature"
})
public class LogicalJoin {
/** The attributes. */
@XmlElement(required = true)
protected AttributeRefs attributes;
/** The associated attribute names. */
@XmlElement(required = true)
protected AttributeNames associatedAttributeNames;
/** The properties. */
@XmlElement(required = true)
protected JoinProperties properties;
/** The temporal join properties. */
protected TemporalJoinProperties temporalJoinProperties;
/** The associated attribute features. */
protected FeaturedAttributeReferences associatedAttributeFeatures;
/** The associated hierarchy feature. */
protected List associatedHierarchyFeature;
/** The associated object uri. */
@XmlAttribute(name = "associatedObjectUri", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String associatedObjectUri;
/** The language attribute name. */
@XmlAttribute(name = "languageAttributeName")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String languageAttributeName;
/** The use dimension view hierarchies. */
@XmlAttribute(name = "useDimensionViewHierarchies")
protected Boolean useDimensionViewHierarchies;
/**
* Gets the value of the attributes property.
*
* @return possible object is
* {@link AttributeRefs }
*/
public AttributeRefs getAttributes() {
return attributes;
}
/**
* Sets the value of the attributes property.
*
* @param value allowed object is
* {@link AttributeRefs }
*/
public void setAttributes(AttributeRefs value) {
this.attributes = value;
}
/**
* Gets the value of the associatedAttributeNames property.
*
* @return possible object is
* {@link AttributeNames }
*/
public AttributeNames getAssociatedAttributeNames() {
return associatedAttributeNames;
}
/**
* Sets the value of the associatedAttributeNames property.
*
* @param value allowed object is
* {@link AttributeNames }
*/
public void setAssociatedAttributeNames(AttributeNames value) {
this.associatedAttributeNames = value;
}
/**
* Gets the value of the properties property.
*
* @return possible object is
* {@link JoinProperties }
*/
public JoinProperties getProperties() {
return properties;
}
/**
* Sets the value of the properties property.
*
* @param value allowed object is
* {@link JoinProperties }
*/
public void setProperties(JoinProperties value) {
this.properties = value;
}
/**
* Gets the value of the temporalJoinProperties property.
*
* @return possible object is
* {@link TemporalJoinProperties }
*/
public TemporalJoinProperties getTemporalJoinProperties() {
return temporalJoinProperties;
}
/**
* Sets the value of the temporalJoinProperties property.
*
* @param value allowed object is
* {@link TemporalJoinProperties }
*/
public void setTemporalJoinProperties(TemporalJoinProperties value) {
this.temporalJoinProperties = value;
}
/**
* Gets the value of the associatedAttributeFeatures property.
*
* @return possible object is
* {@link FeaturedAttributeReferences }
*/
public FeaturedAttributeReferences getAssociatedAttributeFeatures() {
return associatedAttributeFeatures;
}
/**
* Sets the value of the associatedAttributeFeatures property.
*
* @param value allowed object is
* {@link FeaturedAttributeReferences }
*/
public void setAssociatedAttributeFeatures(FeaturedAttributeReferences value) {
this.associatedAttributeFeatures = value;
}
/**
* Gets the value of the associatedHierarchyFeature property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the associatedHierarchyFeature property.
*
*
* For example, to add a new item, do as follows:
*
* getAssociatedHierarchyFeature().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FeaturedHierarchyReference }
*
* @return the associated hierarchy feature
*/
public List getAssociatedHierarchyFeature() {
if (associatedHierarchyFeature == null) {
associatedHierarchyFeature = new ArrayList();
}
return this.associatedHierarchyFeature;
}
/**
* Gets the value of the associatedObjectUri property.
*
* @return possible object is
* {@link String }
*/
public String getAssociatedObjectUri() {
return associatedObjectUri;
}
/**
* Sets the value of the associatedObjectUri property.
*
* @param value allowed object is
* {@link String }
*/
public void setAssociatedObjectUri(String value) {
this.associatedObjectUri = value;
}
/**
* Gets the value of the languageAttributeName property.
*
* @return possible object is
* {@link String }
*/
public String getLanguageAttributeName() {
return languageAttributeName;
}
/**
* Sets the value of the languageAttributeName property.
*
* @param value allowed object is
* {@link String }
*/
public void setLanguageAttributeName(String value) {
this.languageAttributeName = value;
}
/**
* Gets the value of the useDimensionViewHierarchies property.
*
* @return possible object is
* {@link Boolean }
*/
public Boolean isUseDimensionViewHierarchies() {
return useDimensionViewHierarchies;
}
/**
* Sets the value of the useDimensionViewHierarchies property.
*
* @param value allowed object is
* {@link Boolean }
*/
public void setUseDimensionViewHierarchies(Boolean value) {
this.useDimensionViewHierarchies = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy