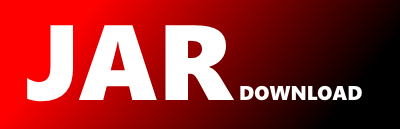
com.codbex.kronos.parser.hdbcalculationview.ndb.bimodelvariable.DerivationRule Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2022 codbex or an codbex affiliate company and contributors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v2.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v20.html
*
* SPDX-FileCopyrightText: 2022 codbex or an codbex affiliate company and contributors
* SPDX-License-Identifier: EPL-2.0
*/
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.11.26 at 10:54:28 AM EET
//
package com.codbex.kronos.parser.hdbcalculationview.ndb.bimodelvariable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import com.codbex.kronos.parser.hdbcalculationview.ndb.bimodeldatafoundation.ColumnFilter;
/**
* Defines how the derived parameter is automatically filled at runtime.
* -Parameter might be filled by reading from a table column. Then the resourceUri must point to a table and resultElementName must be filled.
* Optionally columnFilter can be provided.
* -Parameter might be filled by a procedure or scalar function which has exactly one scalar output parameter.
* Then resourceUri must point to the procedure/function and (optionally) variableMapping can be filled
*
*
* Java class for DerivationRule complex type.
*
* The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="DerivationRule">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="resourceUri" type="{http://www.sap.com/ndb/RepositoryModelResource.ecore}RepositoryUri"/>
* <element name="procedureName" type="{http://www.sap.com/ndb/RepositoryModelResource.ecore}FQName"/>
* <element name="scalarFunctionName" type="{http://www.sap.com/ndb/RepositoryModelResource.ecore}FQName"/>
* </choice>
* <choice>
* <sequence>
* <element name="resultElementName" type="{http://www.sap.com/ndb/BaseModelBase.ecore}DbName" minOccurs="0"/>
* <element name="columnFilter" type="{http://www.sap.com/ndb/BiModelDataFoundation.ecore}ColumnFilter" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <element name="variableMapping" type="{http://www.sap.com/ndb/BiModelVariable.ecore}AbstractVariableMapping" maxOccurs="unbounded" minOccurs="0"/>
* </choice>
* </sequence>
* <attribute name="inputEnabled" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DerivationRule", propOrder = {
"resourceUri",
"procedureName",
"scalarFunctionName",
"resultElementName",
"columnFilter",
"variableMapping"
})
public class DerivationRule {
/** The resource uri. */
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String resourceUri;
/** The procedure name. */
protected String procedureName;
/** The scalar function name. */
protected String scalarFunctionName;
/** The result element name. */
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String resultElementName;
/** The column filter. */
protected List columnFilter;
/** The variable mapping. */
protected List variableMapping;
/** The input enabled. */
@XmlAttribute(name = "inputEnabled")
protected Boolean inputEnabled;
/**
* Gets the value of the resourceUri property.
*
* @return possible object is
* {@link String }
*/
public String getResourceUri() {
return resourceUri;
}
/**
* Sets the value of the resourceUri property.
*
* @param value allowed object is
* {@link String }
*/
public void setResourceUri(String value) {
this.resourceUri = value;
}
/**
* Gets the value of the procedureName property.
*
* @return possible object is
* {@link String }
*/
public String getProcedureName() {
return procedureName;
}
/**
* Sets the value of the procedureName property.
*
* @param value allowed object is
* {@link String }
*/
public void setProcedureName(String value) {
this.procedureName = value;
}
/**
* Gets the value of the scalarFunctionName property.
*
* @return possible object is
* {@link String }
*/
public String getScalarFunctionName() {
return scalarFunctionName;
}
/**
* Sets the value of the scalarFunctionName property.
*
* @param value allowed object is
* {@link String }
*/
public void setScalarFunctionName(String value) {
this.scalarFunctionName = value;
}
/**
* Gets the value of the resultElementName property.
*
* @return possible object is
* {@link String }
*/
public String getResultElementName() {
return resultElementName;
}
/**
* Sets the value of the resultElementName property.
*
* @param value allowed object is
* {@link String }
*/
public void setResultElementName(String value) {
this.resultElementName = value;
}
/**
* Gets the value of the columnFilter property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the columnFilter property.
*
*
* For example, to add a new item, do as follows:
*
* getColumnFilter().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ColumnFilter }
*
* @return the column filter
*/
public List getColumnFilter() {
if (columnFilter == null) {
columnFilter = new ArrayList();
}
return this.columnFilter;
}
/**
* Gets the value of the variableMapping property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the variableMapping property.
*
*
* For example, to add a new item, do as follows:
*
* getVariableMapping().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link AbstractVariableMapping }
*
* @return the variable mapping
*/
public List getVariableMapping() {
if (variableMapping == null) {
variableMapping = new ArrayList();
}
return this.variableMapping;
}
/**
* Gets the value of the inputEnabled property.
*
* @return possible object is
* {@link Boolean }
*/
public Boolean isInputEnabled() {
return inputEnabled;
}
/**
* Sets the value of the inputEnabled property.
*
* @param value allowed object is
* {@link Boolean }
*/
public void setInputEnabled(Boolean value) {
this.inputEnabled = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy