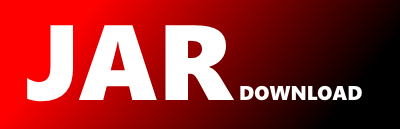
com.codbex.kronos.parser.hdbcalculationview.ndb.datamodelhierarchy.HierarchyDescendantsParameterization Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2022 codbex or an codbex affiliate company and contributors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v2.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v20.html
*
* SPDX-FileCopyrightText: 2022 codbex or an codbex affiliate company and contributors
* SPDX-License-Identifier: EPL-2.0
*/
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.11.26 at 10:54:28 AM EET
//
package com.codbex.kronos.parser.hdbcalculationview.ndb.datamodelhierarchy;
import java.math.BigInteger;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Returns all descendants of a set of start nodes in a hierarchy.
* Docs: https://help.sap.com/viewer/4fe29514fd584807ac9f2a04f6754767/2.0.02/en-US/589208b0582245a782a1f3667a5b80c1.html
*
*
* Java class for HierarchyDescendantsParameterization complex type.
*
* The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="HierarchyDescendantsParameterization">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="start" type="{http://www.sap.com/ndb/DataModelHierarchy.ecore}Start" minOccurs="0"/>
* <element name="startWhere" type="{http://www.sap.com/ndb/DataModelType.ecore}ExpressionString" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="distance" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="distanceParameter" type="{http://www.sap.com/ndb/BaseModelBase.ecore}DbName" minOccurs="0"/>
* </choice>
* <choice minOccurs="0">
* <element name="distanceFrom" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="distanceFromParameter" type="{http://www.sap.com/ndb/BaseModelBase.ecore}DbName" minOccurs="0"/>
* <element name="distanceTo" type="{http://www.w3.org/2001/XMLSchema}integer" minOccurs="0"/>
* <element name="distanceToParameter" type="{http://www.sap.com/ndb/BaseModelBase.ecore}DbName" minOccurs="0"/>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "HierarchyDescendantsParameterization", propOrder = {
"start",
"startWhere",
"distance",
"distanceParameter",
"distanceFrom",
"distanceFromParameter",
"distanceTo",
"distanceToParameter"
})
@XmlSeeAlso({
HierarchyAncestorsParameterization.class
})
public class HierarchyDescendantsParameterization {
/** The start. */
protected Start start;
/** The start where. */
protected String startWhere;
/** The distance. */
protected BigInteger distance;
/** The distance parameter. */
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String distanceParameter;
/** The distance from. */
protected BigInteger distanceFrom;
/** The distance from parameter. */
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String distanceFromParameter;
/** The distance to. */
protected BigInteger distanceTo;
/** The distance to parameter. */
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "token")
protected String distanceToParameter;
/**
* Gets the value of the start property.
*
* @return possible object is
* {@link Start }
*/
public Start getStart() {
return start;
}
/**
* Sets the value of the start property.
*
* @param value allowed object is
* {@link Start }
*/
public void setStart(Start value) {
this.start = value;
}
/**
* Gets the value of the startWhere property.
*
* @return possible object is
* {@link String }
*/
public String getStartWhere() {
return startWhere;
}
/**
* Sets the value of the startWhere property.
*
* @param value allowed object is
* {@link String }
*/
public void setStartWhere(String value) {
this.startWhere = value;
}
/**
* Gets the value of the distance property.
*
* @return possible object is
* {@link BigInteger }
*/
public BigInteger getDistance() {
return distance;
}
/**
* Sets the value of the distance property.
*
* @param value allowed object is
* {@link BigInteger }
*/
public void setDistance(BigInteger value) {
this.distance = value;
}
/**
* Gets the value of the distanceParameter property.
*
* @return possible object is
* {@link String }
*/
public String getDistanceParameter() {
return distanceParameter;
}
/**
* Sets the value of the distanceParameter property.
*
* @param value allowed object is
* {@link String }
*/
public void setDistanceParameter(String value) {
this.distanceParameter = value;
}
/**
* Gets the value of the distanceFrom property.
*
* @return possible object is
* {@link BigInteger }
*/
public BigInteger getDistanceFrom() {
return distanceFrom;
}
/**
* Sets the value of the distanceFrom property.
*
* @param value allowed object is
* {@link BigInteger }
*/
public void setDistanceFrom(BigInteger value) {
this.distanceFrom = value;
}
/**
* Gets the value of the distanceFromParameter property.
*
* @return possible object is
* {@link String }
*/
public String getDistanceFromParameter() {
return distanceFromParameter;
}
/**
* Sets the value of the distanceFromParameter property.
*
* @param value allowed object is
* {@link String }
*/
public void setDistanceFromParameter(String value) {
this.distanceFromParameter = value;
}
/**
* Gets the value of the distanceTo property.
*
* @return possible object is
* {@link BigInteger }
*/
public BigInteger getDistanceTo() {
return distanceTo;
}
/**
* Sets the value of the distanceTo property.
*
* @param value allowed object is
* {@link BigInteger }
*/
public void setDistanceTo(BigInteger value) {
this.distanceTo = value;
}
/**
* Gets the value of the distanceToParameter property.
*
* @return possible object is
* {@link String }
*/
public String getDistanceToParameter() {
return distanceToParameter;
}
/**
* Sets the value of the distanceToParameter property.
*
* @param value allowed object is
* {@link String }
*/
public void setDistanceToParameter(String value) {
this.distanceToParameter = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy