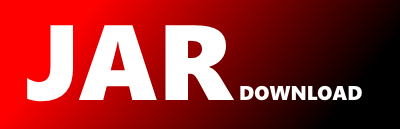
com.codeborne.selenide.WebDriverConditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenide-core Show documentation
Show all versions of selenide-core Show documentation
Selenide = concise API for Selenium WebDriver
package com.codeborne.selenide;
import com.codeborne.selenide.conditions.webdriver.CurrentFrameUrl;
import com.codeborne.selenide.conditions.webdriver.CurrentFrameUrlContaining;
import com.codeborne.selenide.conditions.webdriver.CurrentFrameUrlStartingWith;
import com.codeborne.selenide.conditions.webdriver.NumberOfWindows;
import com.codeborne.selenide.conditions.webdriver.Title;
import com.codeborne.selenide.conditions.webdriver.Url;
import com.codeborne.selenide.conditions.webdriver.UrlContaining;
import com.codeborne.selenide.conditions.webdriver.UrlStartingWith;
import org.openqa.selenium.WebDriver;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nonnull;
/**
* @since 5.23.0
*/
public class WebDriverConditions {
@CheckReturnValue
@Nonnull
public static ObjectCondition url(String expectedUrl) {
return new Url(expectedUrl);
}
@CheckReturnValue
@Nonnull
public static ObjectCondition urlStartingWith(String expectedUrl) {
return new UrlStartingWith(expectedUrl);
}
@CheckReturnValue
@Nonnull
public static ObjectCondition urlContaining(String expectedUrl) {
return new UrlContaining(expectedUrl);
}
@CheckReturnValue
@Nonnull
public static ObjectCondition currentFrameUrl(String expectedUrl) {
return new CurrentFrameUrl(expectedUrl);
}
@CheckReturnValue
@Nonnull
public static ObjectCondition currentFrameUrlStartingWith(String expectedUrl) {
return new CurrentFrameUrlStartingWith(expectedUrl);
}
@CheckReturnValue
@Nonnull
public static ObjectCondition currentFrameUrlContaining(String expectedUrl) {
return new CurrentFrameUrlContaining(expectedUrl);
}
/**
* Check that the number of windows/tabs in the browser is as expected.
* Example:
* {@code webdriver().shouldHave(numberOfWindows(2)) }
*/
@CheckReturnValue
@Nonnull
public static ObjectCondition numberOfWindows(int numberOfWindows) {
return new NumberOfWindows(numberOfWindows);
}
/**
* @since 5.25.0
*/
@CheckReturnValue
@Nonnull
public static ObjectCondition title(String expectedTitle) {
return new Title(expectedTitle);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy