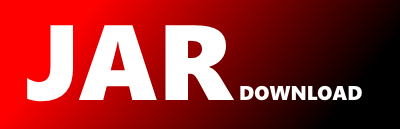
com.codeborne.selenide.WebElementsCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenide-core Show documentation
Show all versions of selenide-core Show documentation
Selenide = concise API for Selenium WebDriver
The newest version!
package com.codeborne.selenide;
import com.codeborne.selenide.ex.UIAssertionError;
import com.codeborne.selenide.impl.CollectionSource;
import org.jspecify.annotations.Nullable;
import org.openqa.selenium.WebElement;
import java.util.List;
import static com.codeborne.selenide.CheckResult.Verdict.ACCEPT;
import static java.lang.System.lineSeparator;
public abstract class WebElementsCondition {
@Nullable
protected String explanation;
public CheckResult check(Driver driver, List elements) {
throw new UnsupportedOperationException("Implement one of 'check' methods in your condition");
}
/**
* The most powerful way to implement condition.
* Can check the collection using JavaScript or any other effective means.
* Also, can return "actual values" in the returned {@link CheckResult} object.
*/
public CheckResult check(CollectionSource collection) {
List elements = collection.getElements();
return check(collection.driver(), elements);
}
/**
* Override this method if you want to customize error class or description
*/
public void fail(CollectionSource collection, CheckResult lastCheckResult, @Nullable Exception cause, long timeoutMs) {
throw new UIAssertionError(
errorMessage() +
lineSeparator() + "Actual: " + lastCheckResult.getActualValue() +
lineSeparator() + "Expected: " + expectedValue() +
(explanation == null ? "" : lineSeparator() + "Because: " + explanation) +
lineSeparator() + "Collection: " + collection.description(),
toString(), lastCheckResult.getActualValue()
);
}
public String errorMessage() {
return "Collection check failed";
}
public String expectedValue() {
return toString();
}
@Override
public abstract String toString();
/**
* Use for explaining the reason of condition:
* WHY you think this collection should match that condition?
*/
public WebElementsCondition because(String explanation) {
this.explanation = explanation;
return this;
}
public boolean missingElementsSatisfyCondition() {
return false;
}
/**
* Using "or" checks in tests is probably a flag of bad test design.
* Consider splitting this "or" check into two different methods or tests.
* @see NOT RECOMMENDED
*/
public WebElementsCondition or(WebElementsCondition alternative) {
return new WebElementsCondition() {
@Override
public CheckResult check(CollectionSource collection) {
CheckResult r1 = WebElementsCondition.this.check(collection);
return r1.verdict() == ACCEPT ? r1 : alternative.check(collection);
}
@Override
public String toString() {
return "%s OR %s".formatted(WebElementsCondition.this, alternative);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy