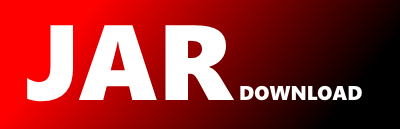
com.codename1.designer.DataEditor Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2008, 2010, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores
* CA 94065 USA or visit www.oracle.com if you need additional information or
* have any questions.
*/
package com.codename1.designer;
import com.codename1.ui.resource.util.BlockingAction;
import com.codename1.designer.ResourceEditorView;
import com.codename1.ui.util.EditableResources;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import javax.swing.JOptionPane;
/**
* The data entry in the resource editor, allows placing an arbitrary file
* in the resource file
*
* @author Shai Almog
*/
public class DataEditor extends BaseForm {
private EditableResources resources;
private String name;
private long fileSize = -1;
private SelectDataFileAction selectFile = new SelectDataFileAction();
/** Creates new form DataEditor */
public DataEditor(EditableResources resources, String name) {
this.resources = resources;
this.name = name;
initComponents();
fileSize = resources.getDataSize(name);
sizeLabel.setText("File Size : " + Long.toString(fileSize) + " Bytes");
sizeLabel.setVisible(fileSize >= 0);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
sizeLabel = new javax.swing.JLabel();
setName("Form"); // NOI18N
sizeLabel.setForeground(new java.awt.Color(255, 0, 0));
sizeLabel.setText("0 Bytes");
sizeLabel.setFocusable(false);
sizeLabel.setName("sizeLabel"); // NOI18N
org.jdesktop.layout.GroupLayout layout = new org.jdesktop.layout.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(layout.createSequentialGroup()
.addContainerGap()
.add(sizeLabel, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, 380, Short.MAX_VALUE)
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(layout.createSequentialGroup()
.add(55, 55, 55)
.add(sizeLabel)
.addContainerGap(242, Short.MAX_VALUE))
);
}// //GEN-END:initComponents
public void selectDataFile(ResourceEditorView view) {
selectFile.view = view;
selectFile.actionPerformed(null);
}
class SelectDataFileAction extends BlockingAction {
private File[] selection;
private ResourceEditorView view;
public SelectDataFileAction() {
putValue(NAME, "Select File");
}
public void start() {
File[] files = ResourceEditorView.showOpenFileChooser(true, "All Files", "");
if(files != null && files.length > 0) {
selection = files;
}
}
@Override
public void exectute() {
if(selection != null) {
for(File currentFile : selection) {
try {
DataInputStream d = new DataInputStream(new FileInputStream(currentFile));
byte[] buffer = new byte[(int)currentFile.length()];
fileSize = buffer.length;
d.readFully(buffer);
resources.setData(currentFile.getName(), buffer);
d.close();
if(view != null) {
view.setSelectedResource(currentFile.getName());
}
} catch(IOException ioErr) {
fileSize = -1;
ioErr.printStackTrace();
JOptionPane.showMessageDialog(DataEditor.this, "There was an error reading the file:\n" + ioErr, "IO Error", JOptionPane.ERROR_MESSAGE);
}
sizeLabel.setText("File Size : " + Long.toString(fileSize) + " Bytes");
sizeLabel.setVisible(fileSize >= 0);
}
} else {
fileSize = -1;
}
}
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel sizeLabel;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy