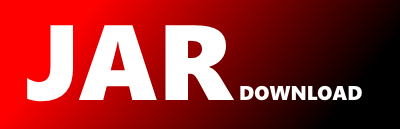
com.codename1.designer.ImageBorderWizardTabbedPane Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2008, 2010, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores
* CA 94065 USA or visit www.oracle.com if you need additional information or
* have any questions.
*/
package com.codename1.designer;
import com.codename1.ui.util.EditableResources;
import java.io.IOException;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
/**
* Part of the image border wizard in the theme
*
* @author Shai Almog
*/
public class ImageBorderWizardTabbedPane extends javax.swing.JPanel {
private ImageBorderCuttingWizard cutting;
private ImageBorderWizard design;
private ImageBorderAppliesToWizard appliesTo;
private boolean generated;
/** Creates new form ImageBorderWizardTabbedPane */
public ImageBorderWizardTabbedPane(EditableResources res, String theme) {
initComponents();
try {
help.setPage(getClass().getResource("/help/9patchHelp.html"));
} catch (IOException ex) {
ex.printStackTrace();
}
design = new ImageBorderWizard();
appliesTo = new ImageBorderAppliesToWizard(res, theme);
cutting = new ImageBorderCuttingWizard(res, theme, design, appliesTo);
jPanel1.add(java.awt.BorderLayout.CENTER, design);
jPanel2.add(java.awt.BorderLayout.CENTER, cutting);
jPanel3.add(java.awt.BorderLayout.CENTER, appliesTo);
}
public void addToAppliesToList(String uiid) {
appliesTo.addToList(uiid);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
ok = new javax.swing.JButton();
close = new javax.swing.JButton();
jTabbedPane1 = new javax.swing.JTabbedPane();
jPanel1 = new javax.swing.JPanel();
jPanel2 = new javax.swing.JPanel();
jPanel3 = new javax.swing.JPanel();
jScrollPane1 = new javax.swing.JScrollPane();
help = new javax.swing.JTextPane();
apply = new javax.swing.JButton();
FormListener formListener = new FormListener();
ok.setText("OK");
ok.setName("ok"); // NOI18N
ok.addActionListener(formListener);
close.setText("Cancel");
close.setName("close"); // NOI18N
close.addActionListener(formListener);
jTabbedPane1.setName("jTabbedPane1"); // NOI18N
jPanel1.setName("jPanel1"); // NOI18N
jPanel1.setLayout(new java.awt.BorderLayout());
jTabbedPane1.addTab("Create Image", jPanel1);
jPanel2.setName("jPanel2"); // NOI18N
jPanel2.setLayout(new java.awt.BorderLayout());
jTabbedPane1.addTab("Cut Image", jPanel2);
jPanel3.setName("jPanel3"); // NOI18N
jPanel3.setLayout(new java.awt.BorderLayout());
jTabbedPane1.addTab("Apply To", jPanel3);
jScrollPane1.setName("jScrollPane1"); // NOI18N
help.setContentType("text/html");
help.setEditable(false);
help.setName("help"); // NOI18N
jScrollPane1.setViewportView(help);
jTabbedPane1.addTab("Help", jScrollPane1);
apply.setText("Apply");
apply.setName("apply"); // NOI18N
apply.addActionListener(formListener);
org.jdesktop.layout.GroupLayout layout = new org.jdesktop.layout.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(layout.createSequentialGroup()
.addContainerGap()
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(jTabbedPane1, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, 380, Short.MAX_VALUE)
.add(org.jdesktop.layout.GroupLayout.TRAILING, layout.createSequentialGroup()
.add(close)
.addPreferredGap(org.jdesktop.layout.LayoutStyle.UNRELATED)
.add(apply)
.addPreferredGap(org.jdesktop.layout.LayoutStyle.UNRELATED)
.add(ok)))
.addContainerGap())
);
layout.linkSize(new java.awt.Component[] {close, ok}, org.jdesktop.layout.GroupLayout.HORIZONTAL);
layout.setVerticalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(org.jdesktop.layout.GroupLayout.TRAILING, layout.createSequentialGroup()
.addContainerGap()
.add(jTabbedPane1, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, 249, Short.MAX_VALUE)
.addPreferredGap(org.jdesktop.layout.LayoutStyle.RELATED)
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.BASELINE)
.add(ok)
.add(apply)
.add(close))
.addContainerGap())
);
}
// Code for dispatching events from components to event handlers.
private class FormListener implements java.awt.event.ActionListener {
FormListener() {}
public void actionPerformed(java.awt.event.ActionEvent evt) {
if (evt.getSource() == ok) {
ImageBorderWizardTabbedPane.this.okActionPerformed(evt);
}
else if (evt.getSource() == close) {
ImageBorderWizardTabbedPane.this.closeActionPerformed(evt);
}
else if (evt.getSource() == apply) {
ImageBorderWizardTabbedPane.this.applyActionPerformed(evt);
}
}
}// //GEN-END:initComponents
private void okActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_okActionPerformed
generated = true;
cutting.generate();
SwingUtilities.windowForComponent(this).dispose();
}//GEN-LAST:event_okActionPerformed
private void closeActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_closeActionPerformed
/*if(!generated) {
if(JOptionPane.showConfirmDialog(this, "Are you sure you want to close the window without generating?", "Are You Sure?", JOptionPane.YES_NO_OPTION) !=
JOptionPane.YES_OPTION) {
return;
}
}*/
SwingUtilities.windowForComponent(this).dispose();
}//GEN-LAST:event_closeActionPerformed
private void applyActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_applyActionPerformed
generated = true;
cutting.generate();
}//GEN-LAST:event_applyActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton apply;
private javax.swing.JButton close;
private javax.swing.JTextPane help;
private javax.swing.JPanel jPanel1;
private javax.swing.JPanel jPanel2;
private javax.swing.JPanel jPanel3;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JTabbedPane jTabbedPane1;
private javax.swing.JButton ok;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy