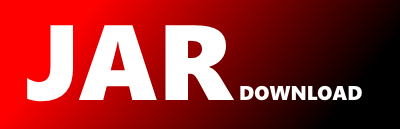
com.codename1.designer.InputModeKeyEditor Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2008, 2010, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores
* CA 94065 USA or visit www.oracle.com if you need additional information or
* have any questions.
*/
package com.codename1.designer;
import javax.swing.SpinnerNumberModel;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
/**
* Allows editing input modes for the text field
*
* @author Shai Almog
*/
public class InputModeKeyEditor extends javax.swing.JPanel {
private boolean lock;
/** Creates new form InputModeKeyEditor */
public InputModeKeyEditor(int key, String value) {
initComponents();
keycode.setModel(new SpinnerNumberModel(0, Integer.MIN_VALUE, Integer.MAX_VALUE, 1));
toggle.setText(value);
keycode.setValue(key);
character.setText("" + ((char)key));
character.getDocument().addDocumentListener(new DocumentListener() {
public void insertUpdate(DocumentEvent e) {
changedUpdate(e);
}
public void removeUpdate(DocumentEvent e) {
changedUpdate(e);
}
public void changedUpdate(DocumentEvent e) {
if(!lock) {
lock = true;
String c = character.getText();
if(c.length() > 0) {
keycode.setValue(new Integer(c.charAt(0) & 0xffffff));
}
lock = false;
}
}
});
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
keycode = new javax.swing.JSpinner();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
toggle = new javax.swing.JTextField();
jScrollPane1 = new javax.swing.JScrollPane();
help = new javax.swing.JTextPane();
character = new javax.swing.JFormattedTextField();
FormListener formListener = new FormListener();
jLabel1.setText("Keycode");
jLabel1.setName("jLabel1"); // NOI18N
keycode.setName("keycode"); // NOI18N
keycode.addChangeListener(formListener);
jLabel2.setText("Character");
jLabel2.setName("jLabel2"); // NOI18N
jLabel3.setText("Toggle Between");
jLabel3.setName("jLabel3"); // NOI18N
toggle.setColumns(10);
toggle.setName("toggle"); // NOI18N
jScrollPane1.setName("jScrollPane1"); // NOI18N
help.setContentType("text/html");
help.setEditable(false);
help.setText("\r\n \r\n\r\n \r\n \r\n \r\n \rKey input mode toggles the string characters given when the keycode/character is pressed.\nE.g. When the character '2' is pressed on a typical feature phone abc2 characters will replace\none another with repeater presses\n
\r\n \r\n\r\n"); // NOI18N
help.setName("help"); // NOI18N
jScrollPane1.setViewportView(help);
try {
character.setFormatterFactory(new javax.swing.text.DefaultFormatterFactory(new javax.swing.text.MaskFormatter("*")));
} catch (java.text.ParseException ex) {
ex.printStackTrace();
}
character.setName("character"); // NOI18N
character.addActionListener(formListener);
org.jdesktop.layout.GroupLayout layout = new org.jdesktop.layout.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(layout.createSequentialGroup()
.addContainerGap()
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(jLabel1)
.add(jLabel2)
.add(jLabel3))
.addPreferredGap(org.jdesktop.layout.LayoutStyle.RELATED)
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(toggle, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE)
.add(keycode, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE)
.add(character, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(org.jdesktop.layout.LayoutStyle.RELATED)
.add(jScrollPane1, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, 217, Short.MAX_VALUE))
);
layout.linkSize(new java.awt.Component[] {character, keycode, toggle}, org.jdesktop.layout.GroupLayout.HORIZONTAL);
layout.setVerticalGroup(
layout.createParallelGroup(org.jdesktop.layout.GroupLayout.LEADING)
.add(layout.createSequentialGroup()
.addContainerGap()
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.BASELINE)
.add(jLabel1)
.add(keycode, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(org.jdesktop.layout.LayoutStyle.RELATED)
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.BASELINE)
.add(jLabel2)
.add(character, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(org.jdesktop.layout.LayoutStyle.RELATED)
.add(layout.createParallelGroup(org.jdesktop.layout.GroupLayout.BASELINE)
.add(jLabel3)
.add(toggle, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, org.jdesktop.layout.GroupLayout.PREFERRED_SIZE)))
.add(jScrollPane1, org.jdesktop.layout.GroupLayout.DEFAULT_SIZE, 156, Short.MAX_VALUE)
);
}
// Code for dispatching events from components to event handlers.
private class FormListener implements java.awt.event.ActionListener, javax.swing.event.ChangeListener {
FormListener() {}
public void actionPerformed(java.awt.event.ActionEvent evt) {
if (evt.getSource() == character) {
InputModeKeyEditor.this.characterActionPerformed(evt);
}
}
public void stateChanged(javax.swing.event.ChangeEvent evt) {
if (evt.getSource() == keycode) {
InputModeKeyEditor.this.keycodeStateChanged(evt);
}
}
}// //GEN-END:initComponents
public int getKeycode() {
return ((Number)keycode.getValue()).intValue();
}
public String getToggle() {
return toggle.getText();
}
private void keycodeStateChanged(javax.swing.event.ChangeEvent evt) {//GEN-FIRST:event_keycodeStateChanged
if(!lock) {
lock = true;
char c = (char)((Number)keycode.getValue()).intValue();
character.setText("" + c);
lock = false;
}
}//GEN-LAST:event_keycodeStateChanged
private void characterActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_characterActionPerformed
if(!lock) {
lock = true;
String c = character.getText();
if(c.length() > 0) {
keycode.setValue(new Integer(c.charAt(0) & 0xffffff));
}
lock = false;
}
}//GEN-LAST:event_characterActionPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JFormattedTextField character;
private javax.swing.JTextPane help;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JSpinner keycode;
private javax.swing.JTextField toggle;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy