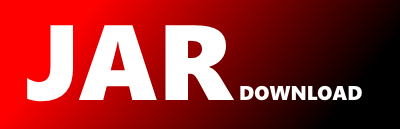
com.codepine.api.testrail.model.Plan Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 27 13:39:31 PST 2016
/*
* The MIT License (MIT)
*
* Copyright (c) 2015 Kunal Shah
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.codepine.api.testrail.model;
import com.codepine.api.testrail.TestRail;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonView;
import java.util.Date;
import java.util.List;
/**
* TestRail plan.
*/
public class Plan {
private int id;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.Update.class})
private String name;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.Update.class})
private String description;
private String url;
private int projectId;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.Update.class})
private Integer milestoneId;
private Integer assignedtoId;
private Date createdOn;
private int createdBy;
@JsonProperty
private boolean isCompleted;
private Date completedOn;
private int passedCount;
private int blockedCount;
private int untestedCount;
private int retestCount;
private int failedCount;
private int customStatus1Count;
private int customStatus2Count;
private int customStatus3Count;
private int customStatus4Count;
private int customStatus5Count;
private int customStatus6Count;
private int customStatus7Count;
@JsonView({TestRail.Plans.Add.class})
private List entries;
public static class Entry {
private String id;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class, TestRail.Plans.UpdateEntry.class})
private String name;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class})
private Integer suiteId;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class, TestRail.Plans.UpdateEntry.class})
private Integer assignedtoId;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class, TestRail.Plans.UpdateEntry.class})
private Boolean includeAll;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class, TestRail.Plans.UpdateEntry.class})
private List caseIds;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class})
private List configIds;
@JsonView({TestRail.Plans.Add.class, TestRail.Plans.AddEntry.class})
private List runs;
public static class Run extends com.codepine.api.testrail.model.Run {
private String entryId;
private int entryIndex;
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Run() {
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getEntryId() {
return this.entryId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getEntryIndex() {
return this.entryIndex;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Run setEntryId(final String entryId) {
this.entryId = entryId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Run setEntryIndex(final int entryIndex) {
this.entryIndex = entryIndex;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Plan.Entry.Run)) return false;
final Run other = (Run)o;
if (!other.canEqual((Object)this)) return false;
if (!super.equals(o)) return false;
final Object this$entryId = this.getEntryId();
final Object other$entryId = other.getEntryId();
if (this$entryId == null ? other$entryId != null : !this$entryId.equals(other$entryId)) return false;
if (this.getEntryIndex() != other.getEntryIndex()) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Plan.Entry.Run;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final Object $entryId = this.getEntryId();
result = result * PRIME + ($entryId == null ? 0 : $entryId.hashCode());
result = result * PRIME + this.getEntryIndex();
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Plan.Entry.Run(super=" + super.toString() + ", entryId=" + this.getEntryId() + ", entryIndex=" + this.getEntryIndex() + ")";
}
}
public Boolean isIncludeAll() {
return getIncludeAll();
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry() {
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getId() {
return this.id;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getName() {
return this.name;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getSuiteId() {
return this.suiteId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getAssignedtoId() {
return this.assignedtoId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getCaseIds() {
return this.caseIds;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getConfigIds() {
return this.configIds;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getRuns() {
return this.runs;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setId(final String id) {
this.id = id;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setName(final String name) {
this.name = name;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setSuiteId(final Integer suiteId) {
this.suiteId = suiteId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setAssignedtoId(final Integer assignedtoId) {
this.assignedtoId = assignedtoId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setIncludeAll(final Boolean includeAll) {
this.includeAll = includeAll;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setCaseIds(final List caseIds) {
this.caseIds = caseIds;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setConfigIds(final List configIds) {
this.configIds = configIds;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Entry setRuns(final List runs) {
this.runs = runs;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Plan.Entry)) return false;
final Entry other = (Entry)o;
if (!other.canEqual((Object)this)) return false;
final Object this$id = this.getId();
final Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final Object this$name = this.getName();
final Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final Object this$suiteId = this.getSuiteId();
final Object other$suiteId = other.getSuiteId();
if (this$suiteId == null ? other$suiteId != null : !this$suiteId.equals(other$suiteId)) return false;
final Object this$assignedtoId = this.getAssignedtoId();
final Object other$assignedtoId = other.getAssignedtoId();
if (this$assignedtoId == null ? other$assignedtoId != null : !this$assignedtoId.equals(other$assignedtoId)) return false;
final Object this$includeAll = this.getIncludeAll();
final Object other$includeAll = other.getIncludeAll();
if (this$includeAll == null ? other$includeAll != null : !this$includeAll.equals(other$includeAll)) return false;
final Object this$caseIds = this.getCaseIds();
final Object other$caseIds = other.getCaseIds();
if (this$caseIds == null ? other$caseIds != null : !this$caseIds.equals(other$caseIds)) return false;
final Object this$configIds = this.getConfigIds();
final Object other$configIds = other.getConfigIds();
if (this$configIds == null ? other$configIds != null : !this$configIds.equals(other$configIds)) return false;
final Object this$runs = this.getRuns();
final Object other$runs = other.getRuns();
if (this$runs == null ? other$runs != null : !this$runs.equals(other$runs)) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Plan.Entry;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $id = this.getId();
result = result * PRIME + ($id == null ? 0 : $id.hashCode());
final Object $name = this.getName();
result = result * PRIME + ($name == null ? 0 : $name.hashCode());
final Object $suiteId = this.getSuiteId();
result = result * PRIME + ($suiteId == null ? 0 : $suiteId.hashCode());
final Object $assignedtoId = this.getAssignedtoId();
result = result * PRIME + ($assignedtoId == null ? 0 : $assignedtoId.hashCode());
final Object $includeAll = this.getIncludeAll();
result = result * PRIME + ($includeAll == null ? 0 : $includeAll.hashCode());
final Object $caseIds = this.getCaseIds();
result = result * PRIME + ($caseIds == null ? 0 : $caseIds.hashCode());
final Object $configIds = this.getConfigIds();
result = result * PRIME + ($configIds == null ? 0 : $configIds.hashCode());
final Object $runs = this.getRuns();
result = result * PRIME + ($runs == null ? 0 : $runs.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Plan.Entry(id=" + this.getId() + ", name=" + this.getName() + ", suiteId=" + this.getSuiteId() + ", assignedtoId=" + this.getAssignedtoId() + ", includeAll=" + this.getIncludeAll() + ", caseIds=" + this.getCaseIds() + ", configIds=" + this.getConfigIds() + ", runs=" + this.getRuns() + ")";
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
private Boolean getIncludeAll() {
return this.includeAll;
}
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan() {
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getId() {
return this.id;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getName() {
return this.name;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getDescription() {
return this.description;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getUrl() {
return this.url;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getProjectId() {
return this.projectId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getMilestoneId() {
return this.milestoneId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getAssignedtoId() {
return this.assignedtoId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Date getCreatedOn() {
return this.createdOn;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCreatedBy() {
return this.createdBy;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Date getCompletedOn() {
return this.completedOn;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getPassedCount() {
return this.passedCount;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getBlockedCount() {
return this.blockedCount;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getUntestedCount() {
return this.untestedCount;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getRetestCount() {
return this.retestCount;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getFailedCount() {
return this.failedCount;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus1Count() {
return this.customStatus1Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus2Count() {
return this.customStatus2Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus3Count() {
return this.customStatus3Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus4Count() {
return this.customStatus4Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus5Count() {
return this.customStatus5Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus6Count() {
return this.customStatus6Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCustomStatus7Count() {
return this.customStatus7Count;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List getEntries() {
return this.entries;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setId(final int id) {
this.id = id;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setName(final String name) {
this.name = name;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setDescription(final String description) {
this.description = description;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setUrl(final String url) {
this.url = url;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setProjectId(final int projectId) {
this.projectId = projectId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setMilestoneId(final Integer milestoneId) {
this.milestoneId = milestoneId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setAssignedtoId(final Integer assignedtoId) {
this.assignedtoId = assignedtoId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCreatedOn(final Date createdOn) {
this.createdOn = createdOn;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCreatedBy(final int createdBy) {
this.createdBy = createdBy;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCompleted(final boolean isCompleted) {
this.isCompleted = isCompleted;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCompletedOn(final Date completedOn) {
this.completedOn = completedOn;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setPassedCount(final int passedCount) {
this.passedCount = passedCount;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setBlockedCount(final int blockedCount) {
this.blockedCount = blockedCount;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setUntestedCount(final int untestedCount) {
this.untestedCount = untestedCount;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setRetestCount(final int retestCount) {
this.retestCount = retestCount;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setFailedCount(final int failedCount) {
this.failedCount = failedCount;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus1Count(final int customStatus1Count) {
this.customStatus1Count = customStatus1Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus2Count(final int customStatus2Count) {
this.customStatus2Count = customStatus2Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus3Count(final int customStatus3Count) {
this.customStatus3Count = customStatus3Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus4Count(final int customStatus4Count) {
this.customStatus4Count = customStatus4Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus5Count(final int customStatus5Count) {
this.customStatus5Count = customStatus5Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus6Count(final int customStatus6Count) {
this.customStatus6Count = customStatus6Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setCustomStatus7Count(final int customStatus7Count) {
this.customStatus7Count = customStatus7Count;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Plan setEntries(final List entries) {
this.entries = entries;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Plan)) return false;
final Plan other = (Plan)o;
if (!other.canEqual((Object)this)) return false;
if (this.getId() != other.getId()) return false;
final Object this$name = this.getName();
final Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final Object this$description = this.getDescription();
final Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final Object this$url = this.getUrl();
final Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
if (this.getProjectId() != other.getProjectId()) return false;
final Object this$milestoneId = this.getMilestoneId();
final Object other$milestoneId = other.getMilestoneId();
if (this$milestoneId == null ? other$milestoneId != null : !this$milestoneId.equals(other$milestoneId)) return false;
final Object this$assignedtoId = this.getAssignedtoId();
final Object other$assignedtoId = other.getAssignedtoId();
if (this$assignedtoId == null ? other$assignedtoId != null : !this$assignedtoId.equals(other$assignedtoId)) return false;
final Object this$createdOn = this.getCreatedOn();
final Object other$createdOn = other.getCreatedOn();
if (this$createdOn == null ? other$createdOn != null : !this$createdOn.equals(other$createdOn)) return false;
if (this.getCreatedBy() != other.getCreatedBy()) return false;
if (this.isCompleted() != other.isCompleted()) return false;
final Object this$completedOn = this.getCompletedOn();
final Object other$completedOn = other.getCompletedOn();
if (this$completedOn == null ? other$completedOn != null : !this$completedOn.equals(other$completedOn)) return false;
if (this.getPassedCount() != other.getPassedCount()) return false;
if (this.getBlockedCount() != other.getBlockedCount()) return false;
if (this.getUntestedCount() != other.getUntestedCount()) return false;
if (this.getRetestCount() != other.getRetestCount()) return false;
if (this.getFailedCount() != other.getFailedCount()) return false;
if (this.getCustomStatus1Count() != other.getCustomStatus1Count()) return false;
if (this.getCustomStatus2Count() != other.getCustomStatus2Count()) return false;
if (this.getCustomStatus3Count() != other.getCustomStatus3Count()) return false;
if (this.getCustomStatus4Count() != other.getCustomStatus4Count()) return false;
if (this.getCustomStatus5Count() != other.getCustomStatus5Count()) return false;
if (this.getCustomStatus6Count() != other.getCustomStatus6Count()) return false;
if (this.getCustomStatus7Count() != other.getCustomStatus7Count()) return false;
final Object this$entries = this.getEntries();
final Object other$entries = other.getEntries();
if (this$entries == null ? other$entries != null : !this$entries.equals(other$entries)) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Plan;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getId();
final Object $name = this.getName();
result = result * PRIME + ($name == null ? 0 : $name.hashCode());
final Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 0 : $description.hashCode());
final Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 0 : $url.hashCode());
result = result * PRIME + this.getProjectId();
final Object $milestoneId = this.getMilestoneId();
result = result * PRIME + ($milestoneId == null ? 0 : $milestoneId.hashCode());
final Object $assignedtoId = this.getAssignedtoId();
result = result * PRIME + ($assignedtoId == null ? 0 : $assignedtoId.hashCode());
final Object $createdOn = this.getCreatedOn();
result = result * PRIME + ($createdOn == null ? 0 : $createdOn.hashCode());
result = result * PRIME + this.getCreatedBy();
result = result * PRIME + (this.isCompleted() ? 79 : 97);
final Object $completedOn = this.getCompletedOn();
result = result * PRIME + ($completedOn == null ? 0 : $completedOn.hashCode());
result = result * PRIME + this.getPassedCount();
result = result * PRIME + this.getBlockedCount();
result = result * PRIME + this.getUntestedCount();
result = result * PRIME + this.getRetestCount();
result = result * PRIME + this.getFailedCount();
result = result * PRIME + this.getCustomStatus1Count();
result = result * PRIME + this.getCustomStatus2Count();
result = result * PRIME + this.getCustomStatus3Count();
result = result * PRIME + this.getCustomStatus4Count();
result = result * PRIME + this.getCustomStatus5Count();
result = result * PRIME + this.getCustomStatus6Count();
result = result * PRIME + this.getCustomStatus7Count();
final Object $entries = this.getEntries();
result = result * PRIME + ($entries == null ? 0 : $entries.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Plan(id=" + this.getId() + ", name=" + this.getName() + ", description=" + this.getDescription() + ", url=" + this.getUrl() + ", projectId=" + this.getProjectId() + ", milestoneId=" + this.getMilestoneId() + ", assignedtoId=" + this.getAssignedtoId() + ", createdOn=" + this.getCreatedOn() + ", createdBy=" + this.getCreatedBy() + ", isCompleted=" + this.isCompleted() + ", completedOn=" + this.getCompletedOn() + ", passedCount=" + this.getPassedCount() + ", blockedCount=" + this.getBlockedCount() + ", untestedCount=" + this.getUntestedCount() + ", retestCount=" + this.getRetestCount() + ", failedCount=" + this.getFailedCount() + ", customStatus1Count=" + this.getCustomStatus1Count() + ", customStatus2Count=" + this.getCustomStatus2Count() + ", customStatus3Count=" + this.getCustomStatus3Count() + ", customStatus4Count=" + this.getCustomStatus4Count() + ", customStatus5Count=" + this.getCustomStatus5Count() + ", customStatus6Count=" + this.getCustomStatus6Count() + ", customStatus7Count=" + this.getCustomStatus7Count() + ", entries=" + this.getEntries() + ")";
}
@JsonIgnore
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean isCompleted() {
return this.isCompleted;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy