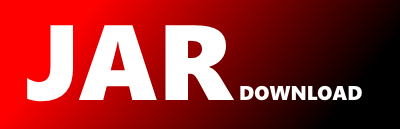
com.codepine.api.testrail.model.Project Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 27 13:39:31 PST 2016
/*
* The MIT License (MIT)
*
* Copyright (c) 2015 Kunal Shah
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
*
*/
package com.codepine.api.testrail.model;
import com.codepine.api.testrail.TestRail;
import com.fasterxml.jackson.annotation.JsonView;
import java.util.Date;
/**
* TestRail project.
*/
public class Project {
private int id;
@JsonView({TestRail.Projects.Add.class, TestRail.Projects.Update.class})
private String name;
@JsonView({TestRail.Projects.Add.class, TestRail.Projects.Update.class})
private String announcement;
@JsonView({TestRail.Projects.Add.class, TestRail.Projects.Update.class})
private Boolean showAnnouncement;
@JsonView(TestRail.Projects.Update.class)
private Boolean isCompleted;
private Date completedOn;
private String url;
@JsonView({TestRail.Projects.Add.class, TestRail.Projects.Update.class})
private Integer suiteMode;
public Boolean isCompleted() {
return getIsCompleted();
}
public Project setCompleted(boolean isCompleted) {
return setIsCompleted(isCompleted);
}
public Boolean isShowAnnouncement() {
return getShowAnnouncement();
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project() {
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getId() {
return this.id;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getName() {
return this.name;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getAnnouncement() {
return this.announcement;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Date getCompletedOn() {
return this.completedOn;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getUrl() {
return this.url;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getSuiteMode() {
return this.suiteMode;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setId(final int id) {
this.id = id;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setName(final String name) {
this.name = name;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setAnnouncement(final String announcement) {
this.announcement = announcement;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setShowAnnouncement(final Boolean showAnnouncement) {
this.showAnnouncement = showAnnouncement;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setCompletedOn(final Date completedOn) {
this.completedOn = completedOn;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setUrl(final String url) {
this.url = url;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Project setSuiteMode(final Integer suiteMode) {
this.suiteMode = suiteMode;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Project)) return false;
final Project other = (Project)o;
if (!other.canEqual((Object)this)) return false;
if (this.getId() != other.getId()) return false;
final Object this$name = this.getName();
final Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final Object this$announcement = this.getAnnouncement();
final Object other$announcement = other.getAnnouncement();
if (this$announcement == null ? other$announcement != null : !this$announcement.equals(other$announcement)) return false;
final Object this$showAnnouncement = this.getShowAnnouncement();
final Object other$showAnnouncement = other.getShowAnnouncement();
if (this$showAnnouncement == null ? other$showAnnouncement != null : !this$showAnnouncement.equals(other$showAnnouncement)) return false;
final Object this$isCompleted = this.getIsCompleted();
final Object other$isCompleted = other.getIsCompleted();
if (this$isCompleted == null ? other$isCompleted != null : !this$isCompleted.equals(other$isCompleted)) return false;
final Object this$completedOn = this.getCompletedOn();
final Object other$completedOn = other.getCompletedOn();
if (this$completedOn == null ? other$completedOn != null : !this$completedOn.equals(other$completedOn)) return false;
final Object this$url = this.getUrl();
final Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final Object this$suiteMode = this.getSuiteMode();
final Object other$suiteMode = other.getSuiteMode();
if (this$suiteMode == null ? other$suiteMode != null : !this$suiteMode.equals(other$suiteMode)) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Project;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getId();
final Object $name = this.getName();
result = result * PRIME + ($name == null ? 0 : $name.hashCode());
final Object $announcement = this.getAnnouncement();
result = result * PRIME + ($announcement == null ? 0 : $announcement.hashCode());
final Object $showAnnouncement = this.getShowAnnouncement();
result = result * PRIME + ($showAnnouncement == null ? 0 : $showAnnouncement.hashCode());
final Object $isCompleted = this.getIsCompleted();
result = result * PRIME + ($isCompleted == null ? 0 : $isCompleted.hashCode());
final Object $completedOn = this.getCompletedOn();
result = result * PRIME + ($completedOn == null ? 0 : $completedOn.hashCode());
final Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 0 : $url.hashCode());
final Object $suiteMode = this.getSuiteMode();
result = result * PRIME + ($suiteMode == null ? 0 : $suiteMode.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Project(id=" + this.getId() + ", name=" + this.getName() + ", announcement=" + this.getAnnouncement() + ", showAnnouncement=" + this.getShowAnnouncement() + ", isCompleted=" + this.getIsCompleted() + ", completedOn=" + this.getCompletedOn() + ", url=" + this.getUrl() + ", suiteMode=" + this.getSuiteMode() + ")";
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
private Boolean getShowAnnouncement() {
return this.showAnnouncement;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
private Boolean getIsCompleted() {
return this.isCompleted;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
private Project setIsCompleted(final Boolean isCompleted) {
this.isCompleted = isCompleted;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy