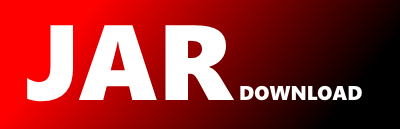
com.codepine.api.testrail.model.Result Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 27 13:39:31 PST 2016
/*
* The MIT License (MIT)
*
* Copyright (c) 2015 Kunal Shah
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.codepine.api.testrail.model;
import com.codepine.api.testrail.TestRail;
import com.codepine.api.testrail.internal.CsvToListDeserializer;
import com.codepine.api.testrail.internal.ListToCsvSerializer;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonView;
import com.fasterxml.jackson.core.JsonGenerationException;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.databind.SerializerProvider;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.databind.ser.std.StdKeySerializer;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import lombok.Getter;
import lombok.NonNull;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import static com.codepine.api.testrail.model.Field.Type;
/**
* TestRail result.
*/
public class Result {
private static final String CUSTOM_FIELD_KEY_PREFIX = "custom_";
private int id;
private int testId;
@JsonView({TestRail.Results.AddListForCases.class})
private Integer caseId;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private Integer statusId;
private Date createdOn;
private int createdBy;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private Integer assignedtoId;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private String comment;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private String version;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private String elapsed;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
@JsonSerialize(using = ListToCsvSerializer.class)
@JsonDeserialize(using = CsvToListDeserializer.class)
private java.util.List defects;
@JsonView({TestRail.Results.Add.class, TestRail.Results.AddForCase.class, TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
@JsonIgnore
private Map customFields;
/**
* Add a defect.
*
* @param defect defect to be added
* @return this instance for chaining
*/
public Result addDefect(@NonNull String defect) {
if (defect == null) {
throw new NullPointerException("defect");
}
Preconditions.checkArgument(!defect.isEmpty(), "defect cannot be empty");
java.util.List defects = getDefects();
if (defects == null) {
defects = new ArrayList<>();
}
defects.add(defect);
setDefects(defects);
return this;
}
@JsonAnyGetter
@JsonSerialize(keyUsing = CustomFieldSerializer.class)
public Map getCustomFields() {
return Objects.firstNonNull(customFields, Collections.emptyMap());
}
/**
* Add a custom field.
*
* @param key the name of the custom field with or without "custom_" prefix
* @param value the value of the custom field
* @return result instance for chaining
*/
public Result addCustomField(String key, Object value) {
if (customFields == null) {
customFields = new HashMap<>();
}
customFields.put(key.replaceFirst(CUSTOM_FIELD_KEY_PREFIX, ""), value);
return this;
}
/**
* Support for forward compatibility and extracting custom fields.
*
* @param key the name of the unknown field
* @param value the value of the unkown field
*/
@JsonAnySetter
private void addUnknownField(String key, Object value) {
if (key.startsWith(CUSTOM_FIELD_KEY_PREFIX)) {
addCustomField(key, value);
}
}
/**
* Get custom field.
* Use Java Type Inference, to get the value with correct type. Refer to {@link Type} for a map of TestRail field types to Java types.
*
* @param key the system name of custom field
* @param the type of returned value
* @return the value of the custom field
*/
public T getCustomField(String key) {
return (T)getCustomFields().get(key);
}
/**
* Serializer for custom fields.
*/
private static class CustomFieldSerializer extends StdKeySerializer {
@Override
public void serialize(Object o, JsonGenerator jsonGenerator, SerializerProvider serializerProvider) throws IOException, JsonGenerationException {
super.serialize(CUSTOM_FIELD_KEY_PREFIX + o, jsonGenerator, serializerProvider);
}
}
/**
* Wrapper for list of {@code Result}s for internal use.
*/
public static class List {
@JsonView({TestRail.Results.AddList.class, TestRail.Results.AddListForCases.class})
private java.util.List results;
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.util.List getResults() {
return this.results;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List setResults(final java.util.List results) {
this.results = results;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Result.List)) return false;
final List other = (List)o;
if (!other.canEqual((Object)this)) return false;
final Object this$results = this.getResults();
final Object other$results = other.getResults();
if (this$results == null ? other$results != null : !this$results.equals(other$results)) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Result.List;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final Object $results = this.getResults();
result = result * PRIME + ($results == null ? 0 : $results.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Result.List(results=" + this.getResults() + ")";
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List() {
}
@java.beans.ConstructorProperties({"results"})
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public List(final java.util.List results) {
this.results = results;
}
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result() {
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getId() {
return this.id;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getTestId() {
return this.testId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getCaseId() {
return this.caseId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getStatusId() {
return this.statusId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Date getCreatedOn() {
return this.createdOn;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int getCreatedBy() {
return this.createdBy;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Integer getAssignedtoId() {
return this.assignedtoId;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getComment() {
return this.comment;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getVersion() {
return this.version;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String getElapsed() {
return this.elapsed;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public java.util.List getDefects() {
return this.defects;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setId(final int id) {
this.id = id;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setTestId(final int testId) {
this.testId = testId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setCaseId(final Integer caseId) {
this.caseId = caseId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setStatusId(final Integer statusId) {
this.statusId = statusId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setCreatedOn(final Date createdOn) {
this.createdOn = createdOn;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setCreatedBy(final int createdBy) {
this.createdBy = createdBy;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setAssignedtoId(final Integer assignedtoId) {
this.assignedtoId = assignedtoId;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setComment(final String comment) {
this.comment = comment;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setVersion(final String version) {
this.version = version;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setElapsed(final String elapsed) {
this.elapsed = elapsed;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setDefects(final java.util.List defects) {
this.defects = defects;
return this;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public Result setCustomFields(final Map customFields) {
this.customFields = customFields;
return this;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public boolean equals(final Object o) {
if (o == this) return true;
if (!(o instanceof Result)) return false;
final Result other = (Result)o;
if (!other.canEqual((Object)this)) return false;
if (this.getId() != other.getId()) return false;
if (this.getTestId() != other.getTestId()) return false;
final Object this$caseId = this.getCaseId();
final Object other$caseId = other.getCaseId();
if (this$caseId == null ? other$caseId != null : !this$caseId.equals(other$caseId)) return false;
final Object this$statusId = this.getStatusId();
final Object other$statusId = other.getStatusId();
if (this$statusId == null ? other$statusId != null : !this$statusId.equals(other$statusId)) return false;
final Object this$createdOn = this.getCreatedOn();
final Object other$createdOn = other.getCreatedOn();
if (this$createdOn == null ? other$createdOn != null : !this$createdOn.equals(other$createdOn)) return false;
if (this.getCreatedBy() != other.getCreatedBy()) return false;
final Object this$assignedtoId = this.getAssignedtoId();
final Object other$assignedtoId = other.getAssignedtoId();
if (this$assignedtoId == null ? other$assignedtoId != null : !this$assignedtoId.equals(other$assignedtoId)) return false;
final Object this$comment = this.getComment();
final Object other$comment = other.getComment();
if (this$comment == null ? other$comment != null : !this$comment.equals(other$comment)) return false;
final Object this$version = this.getVersion();
final Object other$version = other.getVersion();
if (this$version == null ? other$version != null : !this$version.equals(other$version)) return false;
final Object this$elapsed = this.getElapsed();
final Object other$elapsed = other.getElapsed();
if (this$elapsed == null ? other$elapsed != null : !this$elapsed.equals(other$elapsed)) return false;
final Object this$defects = this.getDefects();
final Object other$defects = other.getDefects();
if (this$defects == null ? other$defects != null : !this$defects.equals(other$defects)) return false;
final Object this$customFields = this.getCustomFields();
final Object other$customFields = other.getCustomFields();
if (this$customFields == null ? other$customFields != null : !this$customFields.equals(other$customFields)) return false;
return true;
}
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
protected boolean canEqual(final Object other) {
return other instanceof Result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + this.getId();
result = result * PRIME + this.getTestId();
final Object $caseId = this.getCaseId();
result = result * PRIME + ($caseId == null ? 0 : $caseId.hashCode());
final Object $statusId = this.getStatusId();
result = result * PRIME + ($statusId == null ? 0 : $statusId.hashCode());
final Object $createdOn = this.getCreatedOn();
result = result * PRIME + ($createdOn == null ? 0 : $createdOn.hashCode());
result = result * PRIME + this.getCreatedBy();
final Object $assignedtoId = this.getAssignedtoId();
result = result * PRIME + ($assignedtoId == null ? 0 : $assignedtoId.hashCode());
final Object $comment = this.getComment();
result = result * PRIME + ($comment == null ? 0 : $comment.hashCode());
final Object $version = this.getVersion();
result = result * PRIME + ($version == null ? 0 : $version.hashCode());
final Object $elapsed = this.getElapsed();
result = result * PRIME + ($elapsed == null ? 0 : $elapsed.hashCode());
final Object $defects = this.getDefects();
result = result * PRIME + ($defects == null ? 0 : $defects.hashCode());
final Object $customFields = this.getCustomFields();
result = result * PRIME + ($customFields == null ? 0 : $customFields.hashCode());
return result;
}
@Override
@SuppressWarnings("all")
@javax.annotation.Generated("lombok")
public String toString() {
return "Result(id=" + this.getId() + ", testId=" + this.getTestId() + ", statusId=" + this.getStatusId() + ", createdOn=" + this.getCreatedOn() + ", createdBy=" + this.getCreatedBy() + ", assignedtoId=" + this.getAssignedtoId() + ", comment=" + this.getComment() + ", version=" + this.getVersion() + ", elapsed=" + this.getElapsed() + ", defects=" + this.getDefects() + ", customFields=" + this.getCustomFields() + ")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy