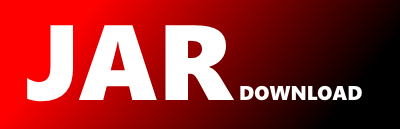
com.windowsazure.samples.PropertyCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa4azure Show documentation
Show all versions of jpa4azure Show documentation
jpa4azure, implements a subset of the JPA specification using Azure Storage for pesisting beans. see http://jpa4azure.codeplex.com for more information.
The newest version!
package com.windowsazure.samples;
import java.util.Date;
import java.util.Iterator;
import java.util.Vector;
import com.windowsazure.samples.Property;
public final class PropertyCollection implements Iterable> {
public PropertyCollection() {
properties = new Vector>();
}
public PropertyCollection(String partitionKey, String rowKey) {
this(partitionKey, rowKey, new Date());
}
public PropertyCollection(String partitionKey, String rowKey, Date timestamp) {
properties = new Vector>();
setPartitionKey(partitionKey);
setRowKey(rowKey);
setTimestamp(timestamp);
}
public void add(Property> newProperty) {
Property> property = getProperty(newProperty.getName());
if (property != null)
properties.remove(property);
properties.add(newProperty);
}
public int getCount() {
return properties.size();
}
public String getPartitionKey() {
return this.getProperty(Property.PARTITION_KEY).getValue();
}
public Property> getProperty(int index) {
return properties.get(index);
}
@SuppressWarnings("unchecked")
public Property getProperty(String name) {
for (Property> property : properties) {
if (property.getName().equals(name)) {
return (Property) property;
}
}
return null;
}
public String getRowKey() {
return this.getProperty(Property.ROW_KEY).getValue();
}
public Date getTimestamp() {
return this.getProperty(Property.TIMESTAMP).getValue();
}
@Override
public Iterator> iterator() {
return properties.iterator();
}
public void setPartitionKey(String partitionKey) {
add(Property.newProperty(Property.PARTITION_KEY, partitionKey));
}
public void setRowKey(String rowKey) {
add(Property.newProperty(Property.ROW_KEY, rowKey));
}
public void setTimestamp(Date date) {
add(Property.newProperty(Property.TIMESTAMP, date));
}
private Vector> properties;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy