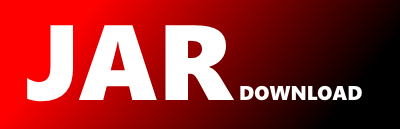
com.codepoetics.fluvius.flows.TargetCapture Maven / Gradle / Ivy
package com.codepoetics.fluvius.flows;
import com.codepoetics.fluvius.api.scratchpad.Key;
/**
* The stage in the fluent API where we have captured the output Key to which a Flow will write its result.
*
* @param
© 2015 - 2024 Weber Informatics LLC | Privacy Policy